Huffman compression in C++ is an efficient algorithm for lossless data compression that uses variable-length codes based on the frequencies of characters to minimize the overall data size.
Here’s a simple code snippet demonstrating a basic implementation of Huffman coding in C++:
#include <iostream>
#include <queue>
#include <unordered_map>
#include <string>
using namespace std;
// Structure to represent a node in the Huffman Tree
struct Node {
char data;
int frequency;
Node *left, *right;
Node(char data, int frequency) : data(data), frequency(frequency), left(nullptr), right(nullptr) {}
};
// Custom comparator for priority queue
struct compare {
bool operator()(Node* left, Node* right) {
return left->frequency > right->frequency;
}
};
// Function to generate Huffman Codes
void generateCodes(Node* root, string str, unordered_map<char, string>& huffmanCodes) {
if (!root) return;
if (root->left == nullptr && root->right == nullptr) {
huffmanCodes[root->data] = str;
}
generateCodes(root->left, str + "0", huffmanCodes);
generateCodes(root->right, str + "1", huffmanCodes);
}
// Function to build the Huffman Tree
Node* buildHuffmanTree(unordered_map<char, int> freq) {
priority_queue<Node*, vector<Node*>, compare> minHeap;
for (auto pair : freq) {
minHeap.push(new Node(pair.first, pair.second));
}
while (minHeap.size() > 1) {
Node* left = minHeap.top(); minHeap.pop();
Node* right = minHeap.top(); minHeap.pop();
Node* newNode = new Node('$', left->frequency + right->frequency);
newNode->left = left;
newNode->right = right;
minHeap.push(newNode);
}
return minHeap.top();
}
int main() {
unordered_map<char, int> freq = {{'a', 5}, {'b', 2}, {'c', 1}, {'d', 1}};
Node* root = buildHuffmanTree(freq);
unordered_map<char, string> huffmanCodes;
generateCodes(root, "", huffmanCodes);
for (auto pair : huffmanCodes) {
cout << pair.first << ": " << pair.second << endl;
}
return 0;
}
What is Huffman Compression?
Huffman Compression is a widely-used algorithm for lossless data compression. Developed by David A. Huffman in 1952, it is an efficient method that assigns variable-length codes to input characters, making frequently occurring characters occupy less space than those that are infrequent.
The advantage of Huffman Compression lies in its ability to reduce the overall size of data, which is particularly crucial in contexts where storage space or bandwidth is a concern. The algorithm effectively reduces file sizes without sacrificing the original content, making it an essential component in compressing data that needs to be retrieved in its original form.
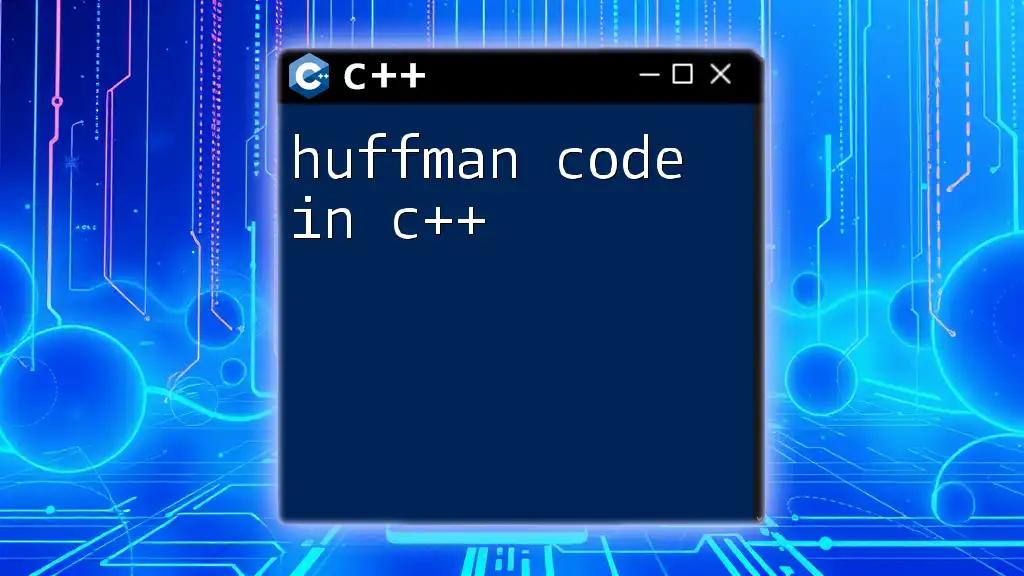
Applications of Huffman Compression
Huffman Compression finds application in various domains, including:
- File Compression: Used in formats like JPEG and PNG for reducing image sizes.
- Data Transmission: Essential in reducing the amount of data sent over networks, improving speed.
- Text Encoding: Commonly applied in file formats such as ZIP and GZIP to compress textual data.
- Programming Libraries: Integration into languages and APIs that require efficient data encoding and decoding.
Understanding how Huffman Compression works can empower developers to optimize their applications for better performance and reduced costs.
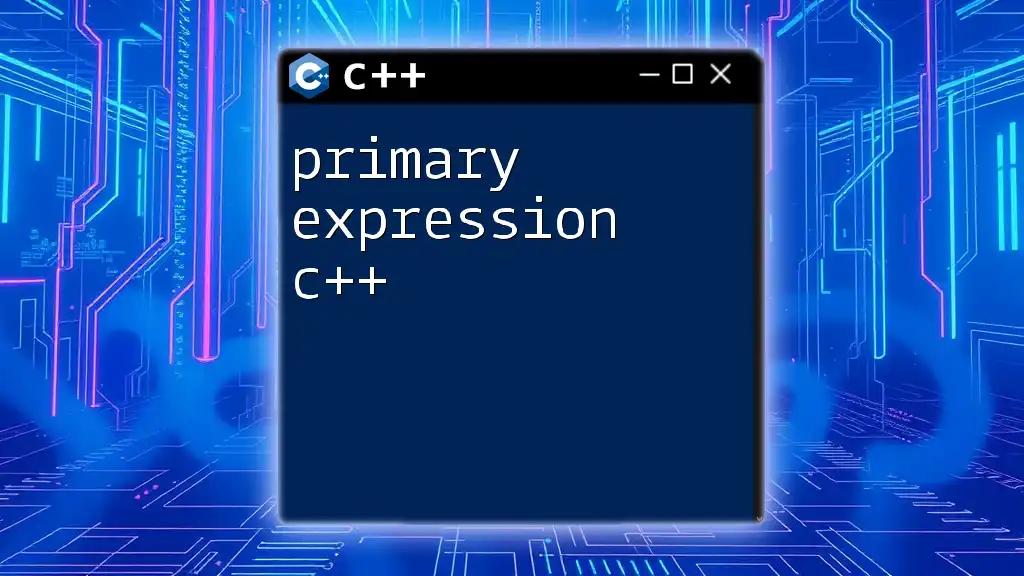
Understanding the Basics of C++
Introduction to C++
C++ is a powerful programming language that combines the functional features of C with object-oriented programming capabilities. Its versatility and performance make it an ideal choice for implementing algorithms like Huffman Compression. C++ utilizes rich data structures, efficient memory management, and object-oriented principles that facilitate complex algorithm design.
Key Concepts in C++
Data Structures
C++ provides foundational data structures crucial for implementing Huffman Compression:
-
Trees: In Huffman Compression, a binary tree is utilized to represent characters and their corresponding codes. Each leaf node of the tree represents a unique character, while internal nodes represent combined frequencies.
-
Priority Queues: A priority queue is used to manage the nodes during the tree construction based on character frequency. This ensures that the least frequent characters are positioned higher in the tree, facilitating efficient coding.
File Handling
File handling is essential in this algorithm since Huffman Compression often takes input from files and outputs the compressed data back to files.
- Reading Files: C++ provides functionalities for reading text or binary files using streams.
- Writing Files: Similarly, writing to files allows us to store the compressed output successfully.

The Huffman Compression Algorithm
Steps to Implement Huffman Compression
Step 1: Build the Frequency Table
A frequency table is constructed to determine how often each character appears in the input data. This serves as the cornerstone of the Huffman algorithm and guides the next steps in the process.
#include <iostream>
#include <unordered_map>
#include <string>
void buildFrequencyTable(const std::string& input, std::unordered_map<char, int>& freqTable) {
for (char ch : input) {
freqTable[ch]++;
}
}
Step 2: Build the Huffman Tree
The Huffman Tree is constructed using the frequency table. A min-heap (or priority queue) is employed to efficiently combine nodes and form the tree based on character frequencies.
#include <queue>
struct Node {
char ch;
int freq;
Node* left;
Node* right;
// Constructor
Node(char character, int frequency) : ch(character), freq(frequency), left(nullptr), right(nullptr) {}
};
struct Compare {
bool operator()(Node* l, Node* r) {
return l->freq > r->freq; // Min-heap
}
};
Node* buildHuffmanTree(const std::unordered_map<char, int>& freqTable) {
std::priority_queue<Node*, std::vector<Node*>, Compare> minHeap;
// Creating nodes from frequency table
for (const auto& pair : freqTable) {
minHeap.push(new Node(pair.first, pair.second));
}
// Building the tree
while (minHeap.size() > 1) {
Node* left = minHeap.top();
minHeap.pop();
Node* right = minHeap.top();
minHeap.pop();
Node* combined = new Node('\0', left->freq + right->freq);
combined->left = left;
combined->right = right;
minHeap.push(combined);
}
return minHeap.top(); // Root of the Huffman Tree
}
Step 3: Generate Huffman Codes
After constructing the tree, the next step is to generate the Huffman codes for each character based on their position in the tree. This is achieved through a recursive traversal of the tree.
void generateCodes(Node* root, const std::string& str, std::unordered_map<char, std::string>& huffmanCodes) {
if (!root) return;
// Leaf node
if (!root->left && !root->right) {
huffmanCodes[root->ch] = str;
}
generateCodes(root->left, str + "0", huffmanCodes);
generateCodes(root->right, str + "1", huffmanCodes);
}
Step 4: Encode the Input Text
With the Huffman codes generated, you can now encode the original text. This process involves mapping each character in the text to its respective Huffman code.
std::string encode(const std::string& input, const std::unordered_map<char, std::string>& huffmanCodes) {
std::string encodedString;
for (char ch : input) {
encodedString += huffmanCodes.at(ch);
}
return encodedString;
}
Step 5: Decode the Encoded String
Decoding the encoded string using the Huffman Tree entails traversing the tree based on the bits in the encoded string. This ensures that you accurately retrieve the original characters.
std::string decode(Node* root, const std::string& encodedStr) {
std::string decodedString;
Node* current = root;
for (char bit : encodedStr) {
if (bit == '0') {
current = current->left;
} else {
current = current->right;
}
// If we reached a leaf node
if (!current->left && !current->right) {
decodedString += current->ch;
current = root; // Reset to root
}
}
return decodedString;
}
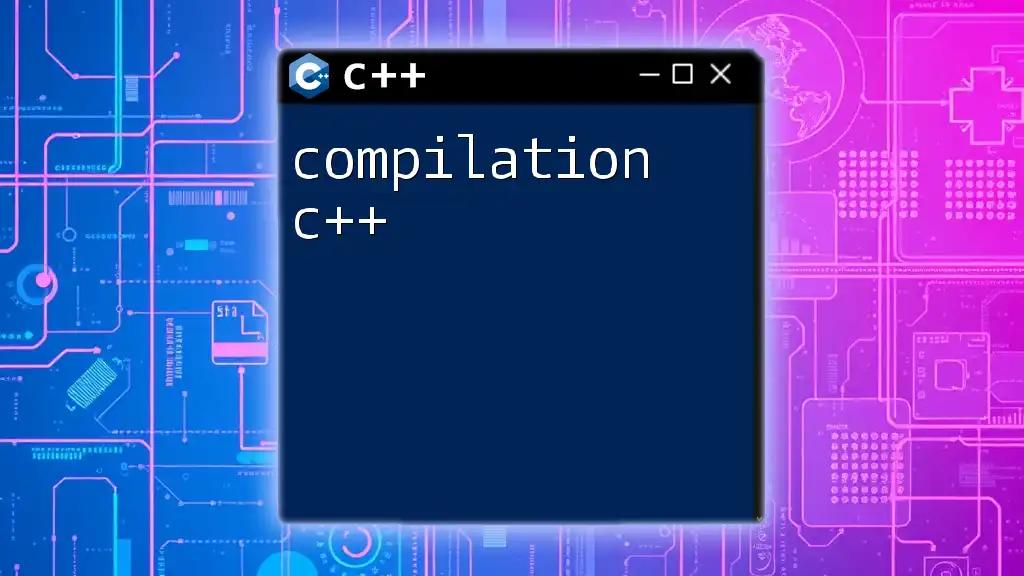
Example Implementation
Full C++ Code Example
Integrating all pieces together, the following code snippet provides a complete example of implementing Huffman Compression in C++.
#include <iostream>
#include <unordered_map>
#include <queue>
#include <string>
// Node structure for the Huffman Tree
struct Node {
char ch;
int freq;
Node* left;
Node* right;
// Constructor
Node(char character, int frequency) : ch(character), freq(frequency), left(nullptr), right(nullptr) {}
};
// Comparator for the priority queue
struct Compare {
bool operator()(Node* l, Node* r) {
return l->freq > r->freq; // Min-heap
}
};
// Build frequency table
void buildFrequencyTable(const std::string& input, std::unordered_map<char, int>& freqTable) {
for (char ch : input) {
freqTable[ch]++;
}
}
// Build Huffman tree
Node* buildHuffmanTree(const std::unordered_map<char, int>& freqTable) {
std::priority_queue<Node*, std::vector<Node*>, Compare> minHeap;
for (const auto& pair : freqTable) {
minHeap.push(new Node(pair.first, pair.second));
}
while (minHeap.size() > 1) {
Node* left = minHeap.top();
minHeap.pop();
Node* right = minHeap.top();
minHeap.pop();
Node* combined = new Node('\0', left->freq + right->freq);
combined->left = left;
combined->right = right;
minHeap.push(combined);
}
return minHeap.top();
}
// Generate Huffman codes
void generateCodes(Node* root, const std::string& str, std::unordered_map<char, std::string>& huffmanCodes) {
if (!root) return;
if (!root->left && !root->right) {
huffmanCodes[root->ch] = str;
}
generateCodes(root->left, str + "0", huffmanCodes);
generateCodes(root->right, str + "1", huffmanCodes);
}
// Encode the input text
std::string encode(const std::string& input, const std::unordered_map<char, std::string>& huffmanCodes) {
std::string encodedString;
for (char ch : input) {
encodedString += huffmanCodes.at(ch);
}
return encodedString;
}
// Decode the encoded string
std::string decode(Node* root, const std::string& encodedStr) {
std::string decodedString;
Node* current = root;
for (char bit : encodedStr) {
if (bit == '0') {
current = current->left;
} else {
current = current->right;
}
if (!current->left && !current->right) {
decodedString += current->ch;
current = root;
}
}
return decodedString;
}
int main() {
std::string input = "huffman compression in c++ is very efficient";
// Build frequency table
std::unordered_map<char, int> freqTable;
buildFrequencyTable(input, freqTable);
// Build Huffman Tree
Node* root = buildHuffmanTree(freqTable);
// Generate Huffman Codes
std::unordered_map<char, std::string> huffmanCodes;
generateCodes(root, "", huffmanCodes);
// Encode the input text
std::string encodedStr = encode(input, huffmanCodes);
std::cout << "Encoded String: " << encodedStr << std::endl;
// Decode the encoded string
std::string decodedStr = decode(root, encodedStr);
std::cout << "Decoded String: " << decodedStr << std::endl;
return 0;
}
Steps to Compile and Run
- Save the above code into a file, e.g., `huffman.cpp`.
- Open a terminal and navigate to the directory where the file is saved.
- Compile the code using the command:
g++ -o huffman huffman.cpp
- Run the executable with:
./huffman

Conclusion
In summary, Huffman Compression in C++ grants developers a powerful tool for optimizing data storage and transmission. This algorithm effectively reduces file sizes without compromising the data, making it critical for various applications, from web development to data science.
To further enhance your understanding, consider exploring additional resources such as books and online courses on data structures and algorithms. Practicing with different datasets can also dramatically improve your proficiency in C++ and efficiency in implementing algorithms.
References
Additional academic papers and resources will provide a deeper insight into Huffman Compression and related algorithms. Exploring these materials will help you understand the breadth of capabilities that data compression offers.