A boolean expression in C++ is an expression that evaluates to either `true` or `false`, often used in control flow statements like `if` and loops.
bool isAdult(int age) {
return age >= 18; // Evaluates to true if age is 18 or older
}
What Are Boolean Expressions?
Boolean expressions are fundamental to programming and represent values that can be either true or false. In C++, boolean expressions form the backbone of decision-making processes, allowing programmers to implement logic in their code. They can simplify complex problems by providing a clear mechanism to evaluate conditions.
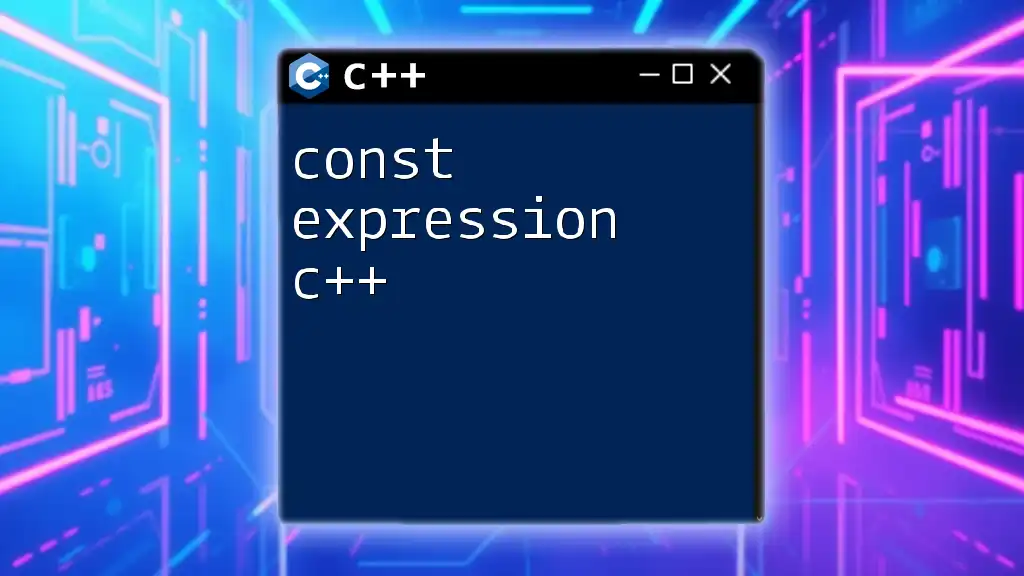
Why Use Boolean Expressions in C++?
Utilizing boolean expressions in C++ enables logical decision-making within your code. By integrating these expressions, you can control the flow of program execution. For instance, when working with conditional statements, boolean expressions determine whether specific blocks of code should run based on given conditions.
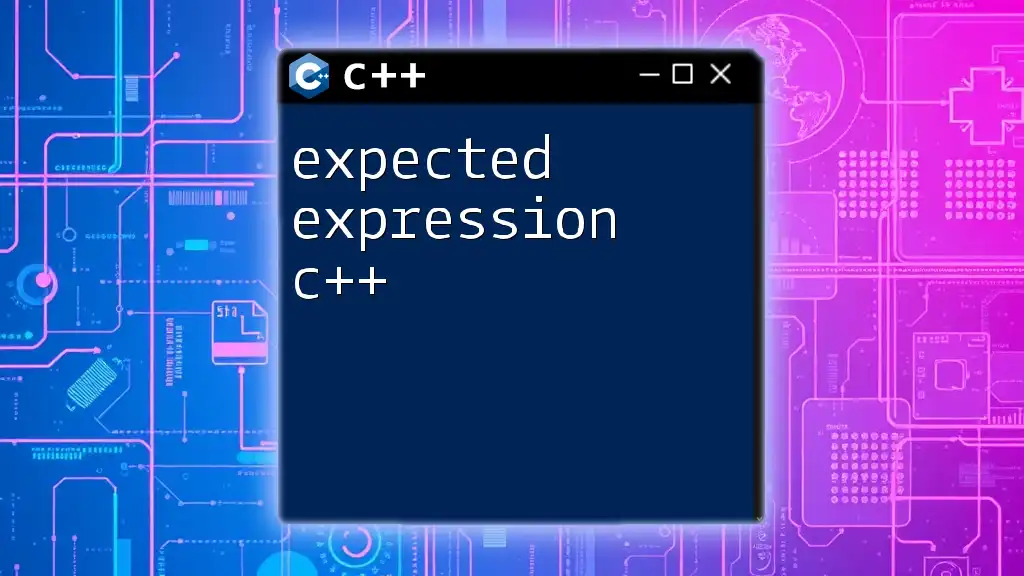
The Boolean Data Type in C++
The boolean data type in C++, defined by the `bool` keyword, consists of only two possible values: true and false. Understanding this datatype is pivotal for effective programming, as it directly relates to how conditions are evaluated.
How to Declare Boolean Variables
Declaring a boolean variable is straightforward. Here’s how to do it:
bool isRaining = false;
bool hasLicense = true;
Initializing boolean variables upon declaration is generally considered best practice to prevent unexpected behavior during program execution.
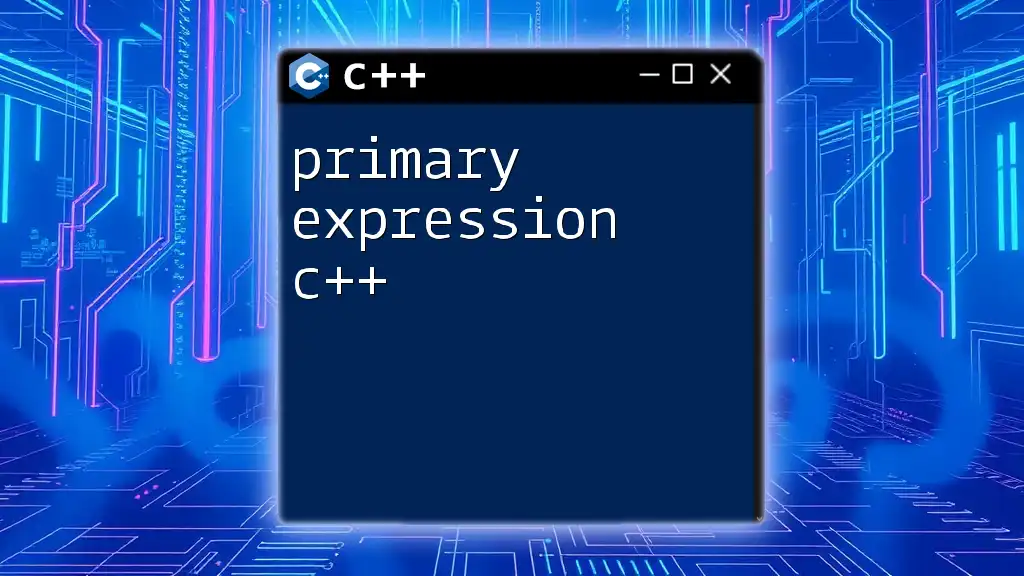
Basic Operators for Boolean Expressions in C++
C++ includes several logical operators essential for constructing boolean expressions. Understanding these operators is crucial for effective programming.
Logical Operators
The three primary logical operators in C++ are AND (&&), OR (||), and NOT (!).
-
AND Operator (`&&`): This operator returns true only if both operands are true.
bool a = true; bool b = false; bool result = a && b; // result will be false
-
OR Operator (`||`): The OR operator returns true if at least one of the operands is true.
bool a = true; bool b = false; bool result = a || b; // result will be true
-
NOT Operator (`!`): This operator reverses the truth value of its operand. It yields true for false operands and false for true ones.
bool a = true; bool result = !a; // result will be false
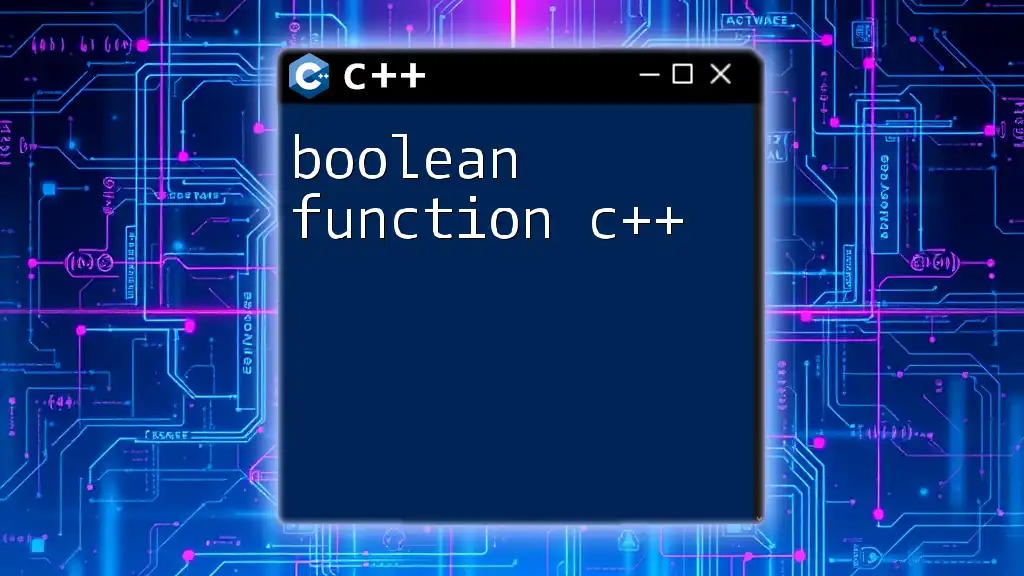
Complex Boolean Expressions in C++
Combining boolean expressions allows you to make sophisticated decisions within your code. You can use multiple logical operators in tandem to create complex structures.
Example of a Complex Boolean Expression
Consider the following example where we evaluate multiple conditions:
bool a = true;
bool b = false;
bool c = true;
bool result = (a && b) || (b && c); // result will be false
Short-Circuit Evaluation
Short-circuit evaluation refers to the process where C++ stops evaluating an expression as soon as the result is determined. For instance, in an AND expression (a && b), if `a` is false, `b` will not be evaluated since the overall expression can never result in true. This enhances performance and prevents unnecessary computations, especially in complex expressions.
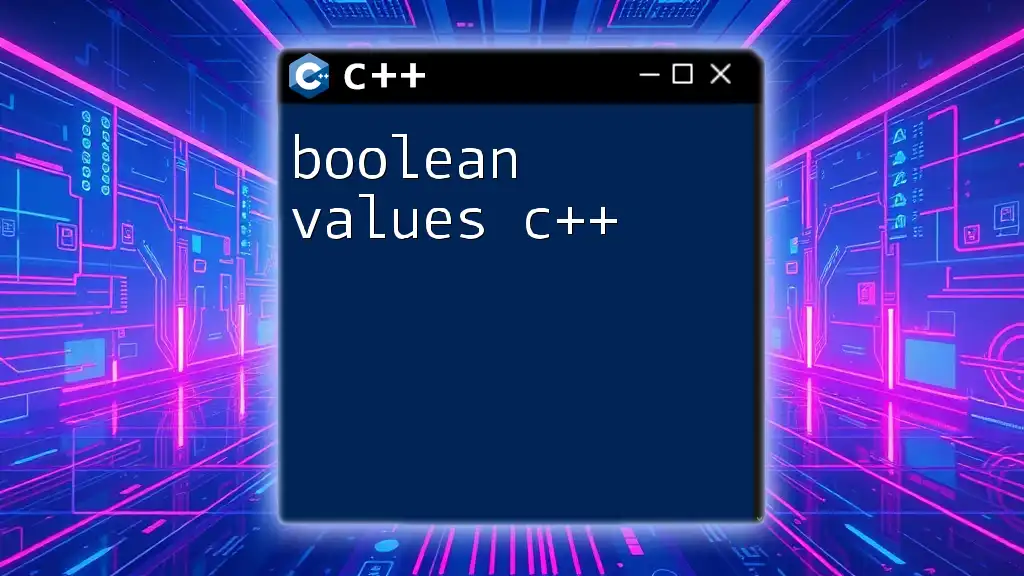
Using Boolean Expressions in Control Flow
Boolean expressions play a pivotal role in control flow statements such as `if`, `else if`, and `else`. These statements utilize boolean conditions to dictate which block of code executes.
Example of Control Flow Using Boolean Expressions
Here’s a simple example that demonstrates how boolean expressions can direct program flow:
int age = 20;
if (age >= 18) {
cout << "Adult";
} else {
cout << "Minor";
}
In this example, the program checks if `age` is greater than or equal to 18. If true, it prints "Adult"; otherwise, it prints "Minor".
Switch Statement and Boolean Expressions
While `switch` statements primarily support integer values, boolean expressions can influence the flow of a program indirectly. They often accompany `if` statements that lead into case statements based on initial conditions, driving program behavior.
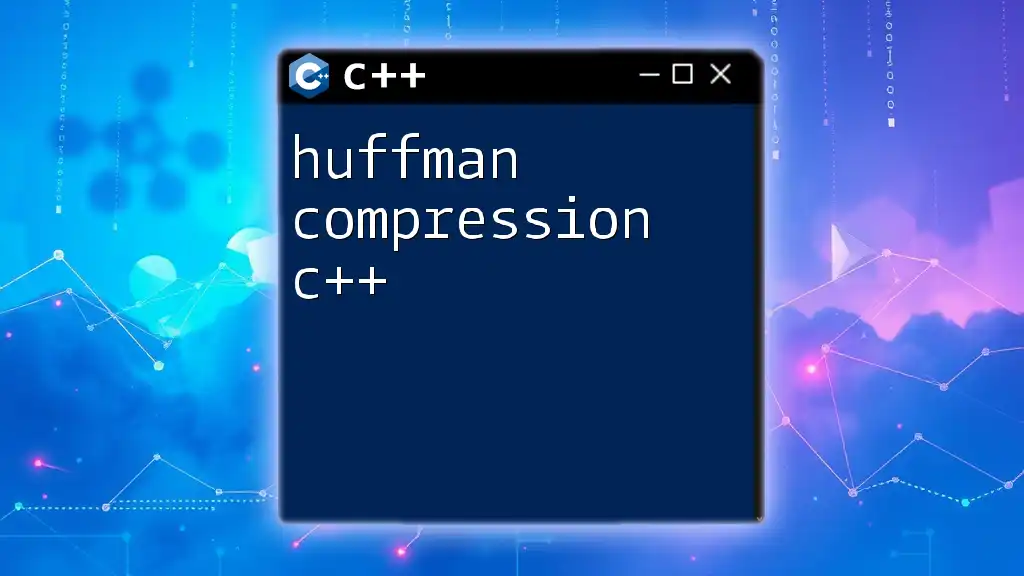
Best Practices for Using Boolean Expressions
Adhering to best practices when employing boolean expressions can significantly improve code clarity and maintainability.
Clarity and Readability
Always aim for clarity. A well-structured boolean expression should convey its intention clearly. Avoid using double negatives, which can confuse readers and maintainers of your code. For example, instead of writing:
if (!notAllowed) {
// logic
}
Use a straightforward approach:
if (allowed) {
// logic
}
Consistency in Naming Conventions
Establish a consistent naming convention for your boolean variables. Names should clearly express what the condition represents. For example, use `isUserLoggedIn` instead of `x`, making your code self-documenting.
Logical Grouping
When dealing with complex boolean expressions, grouping conditions logically enhances understanding. Using parentheses helps delineate sections of the expression, leading to clearer, more structured code.
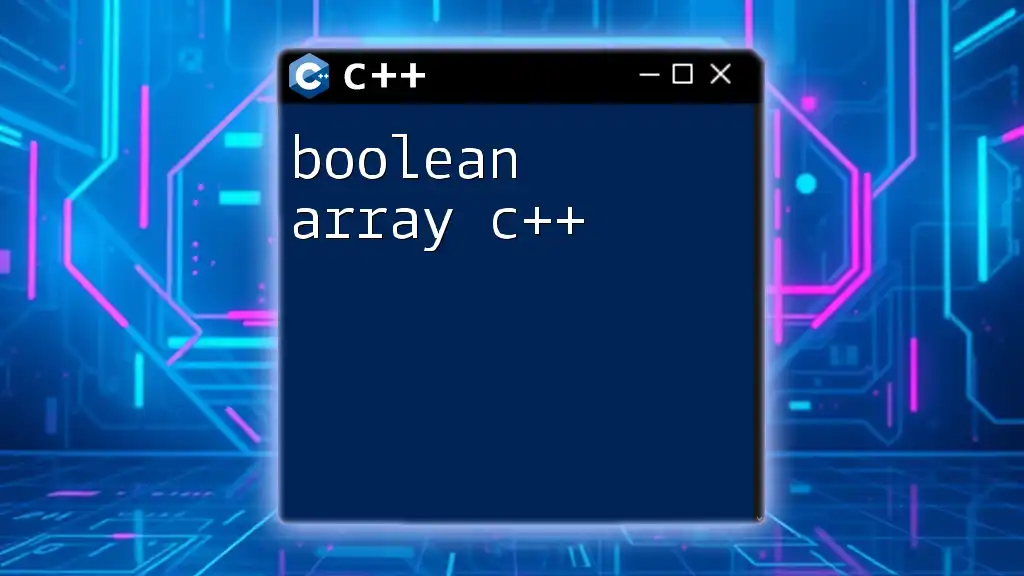
Common Pitfalls and Errors
Be aware of common mistakes when working with boolean expressions to avoid unexpected results:
- Confusion with AND/OR Conditions: It’s easy to misinterpret how AND and OR operators behave. Always check your logic carefully, as mixing them up can lead to incorrect conclusions.
Debugging Boolean Expressions
Debugging boolean expressions may sometimes pose a challenge. Using print statements to display the values of variables and expressions can help clarify how your program is evaluating conditions. Tools such as debuggers and logging can also assist in tracking down issues related to boolean logic.
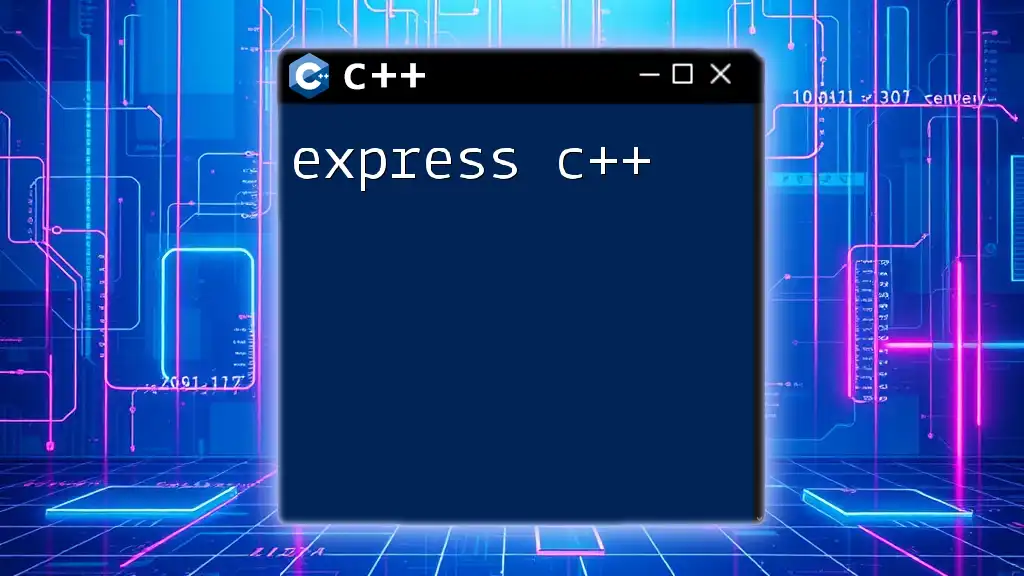
Recap of Key Takeaways
In summary, boolean expressions are a powerful feature of C++ that allows for logical decision-making. Understanding the boolean data type, operators, and application in control flow is fundamental for any C++ programmer. By incorporating best practices and being mindful of common pitfalls, you can write more effective, clear, and maintainable code.
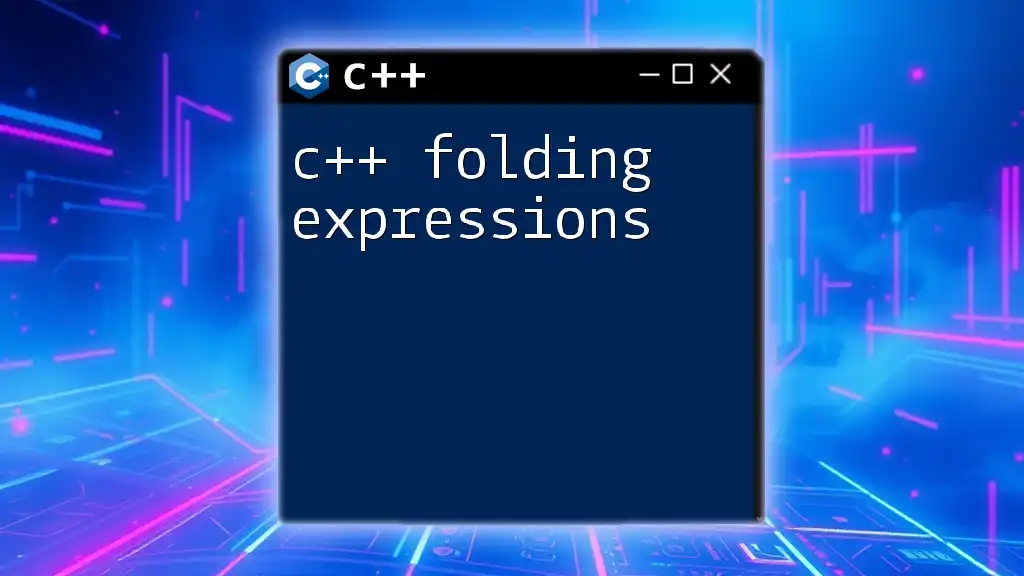
Encouragement to Practice
As with any skill, practice is key. Experiment with boolean expressions in your C++ code to see how they can streamline decision-making and improve your overall programming efficiency. Dive into projects that challenge your understanding, and watch your proficiency grow!
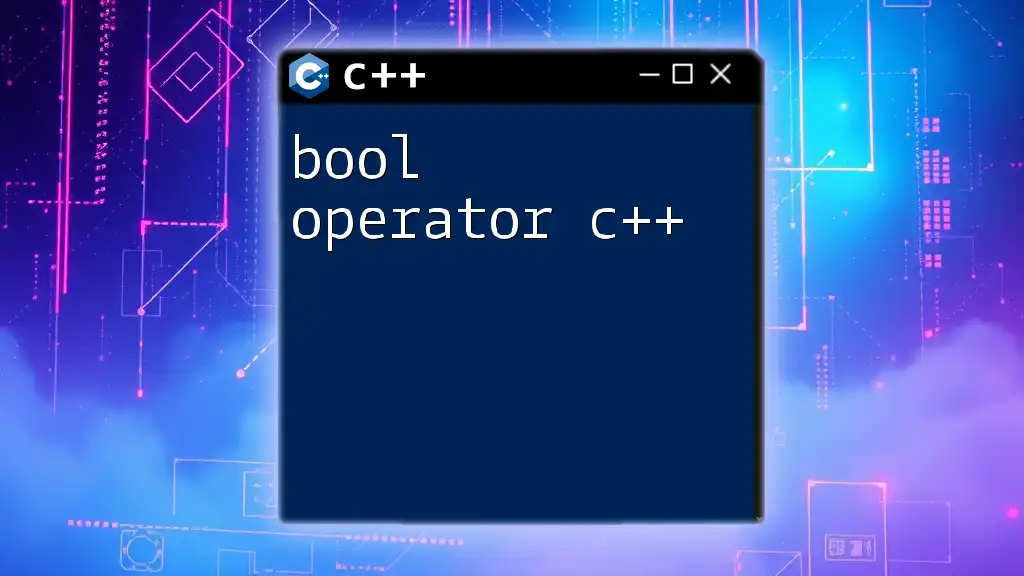
Further Reading and Resources
To continue your journey into boolean expressions in C++, consider exploring books and online courses dedicated to C++. Additionally, the official C++ documentation is a valuable resource for deepening your understanding of boolean expressions and related concepts. Happy coding!