A primary expression in C++ is a fundamental building block of the language, representing the simplest form of expressions such as literals, variables, or function calls that can be evaluated to produce a value.
Here’s a code snippet demonstrating various primary expressions:
int a = 5; // Literal
float b = 7.5; // Float literal
char c = 'x'; // Character literal
bool d = true; // Boolean literal
int sum = a + 10; // Variable expression using a primary expression
Understanding Expressions in C++
In C++, expressions are combinations of variables, literals, operators, and function calls that are evaluated to produce a value. Expressions are vital in programming, as they form the building blocks of code functionality. Understanding the foundational elements of expressions, specifically primary expressions, is essential for mastering C++ programming.
Components of Expressions
Expressions can be simple or complex, and they usually consist of the following components:
- Variables: Identifiers that hold data values, allowing for dynamic programming.
- Operators: Symbols that specify the operation to perform (for example, arithmetic operations like `+` and `-`).
- Literals: Fixed values that do not change during execution—such as numbers and characters.
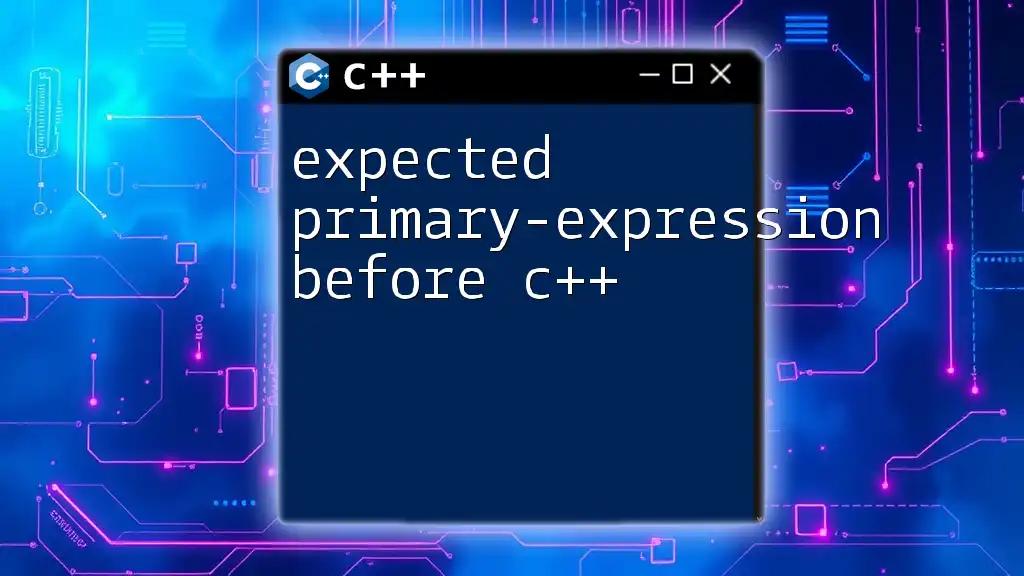
What are Primary Expressions?
Primary expressions form the simplest units of expressions in C++. They can stand alone and are essential for building more complex expressions. Understanding primary expressions is key to understanding how the C++ compiler interprets and executes code.
Types of Primary Expressions
Primary expressions include various types:
-
Literals: These are constant values coded directly into the source. C++ supports several types of literals:
- Integer literals (e.g., `42`)
- Floating-point literals (e.g., `3.14`)
- Character literals (e.g., `'A'`)
- String literals (e.g., `"Hello, World!"`)
Example:
int num = 10; // Integer literal double pi = 3.14; // Floating-point literal char letter = 'A'; // Character literal
-
Identifiers: The names we assign to variables, functions, and other elements in C++. Identifiers must follow specific naming conventions and can consist of letters, digits, and underscores, but cannot start with a digit.
Example:
int score = 100; // 'score' is an identifier
-
Punctuators and Parentheses: In C++, punctuators such as parentheses `()`, braces `{}`, and brackets `[]` can also serve as primary expressions. They help define the structure of more complex expressions.
Example:
int result = (2 + 3) * 5; // Parentheses in use
-
Function Calls: Function calls are primary expressions that perform an action and return a value. They can be particularly powerful when combined with other expressions.
Example:
int square(int x) { return x * x; } int val = square(5); // Function call as primary expression
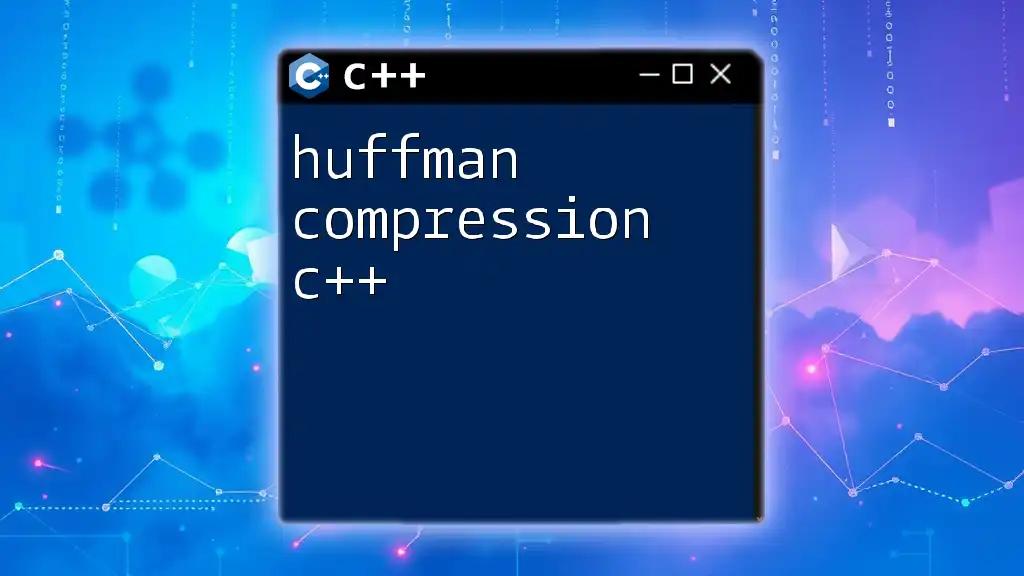
Evaluating Primary Expressions
Understanding how C++ evaluates primary expressions is crucial for effective programming. When the compiler encounters a primary expression, it evaluates it to determine its value. This evaluation occurs at runtime, which means the variables must have valid values at that point for the expression to produce the correct result.
Order of Evaluation
C++ generally follows an order of operations similar to that of math, which affects how expressions get evaluated. For primary expressions, it's essential to understand that they are evaluated first before being combined with other expressions.
Practical Examples
Utilizing primary expressions effectively can enhance clarity and performance in code. Here are some practical examples:
Example Code Snippet:
int a = 5;
int b = 10;
int sum = a + b; // 'a' and 'b' as primary expressions
Here, `a` and `b` are primary expressions that participate in calculating `sum`.
Primary Expressions in Context
Primary expressions serve as the basis for more complex expressions. They can be nested and combined with operators, other primary expressions, and function calls to create advanced logic in your code.
Example:
int x = 10, y = 20;
int total = (x * 2) + (y / 2); // Primary expressions within a larger expression
In this example, the primary expressions `x` and `y` work within the arithmetic operations to calculate `total`.
Nested Primary Expressions
Primary expressions can also be nested within one another. This is common in more complex expressions where clarity and proper evaluation order are essential.
Example:
int result = (x + (y * 2)); // Nested primary expressions
In this scenario, `y * 2` is evaluated first, which is then added to `x`.
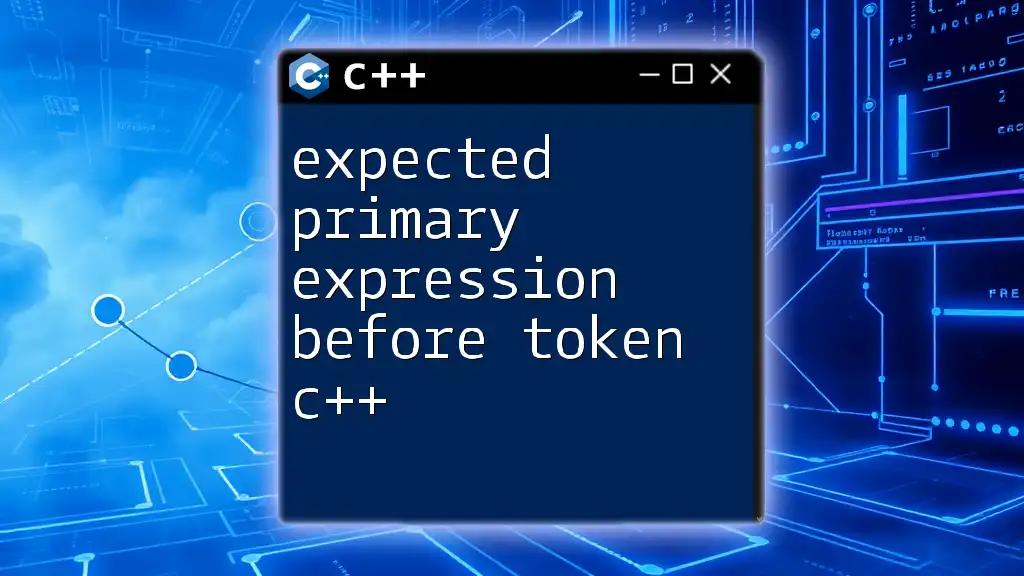
Common Errors and Best Practices
While working with primary expressions, new programmers often encounter common pitfalls.
Common Mistakes with Primary Expressions
-
Missing Semicolons: Forgetting to end statements with semicolons is a common error that can lead to compilation errors.
Example of an error:
// Incorrect: Missing semicolon int value = 10
-
Variable Initialization Errors: Trying to use uninitialized variables. Always ensure that variables have been assigned a value before usage.
Best Practices
To write effective primary expressions, keep the following tips in mind:
- Clarity over Complexity: Write simple, clear expressions. Avoid overly convoluted logic that can confuse future readers (or yourself later on).
- Proper Naming Conventions: Use meaningful identifiers that convey the purpose of the variable, improving code readability and maintainability.
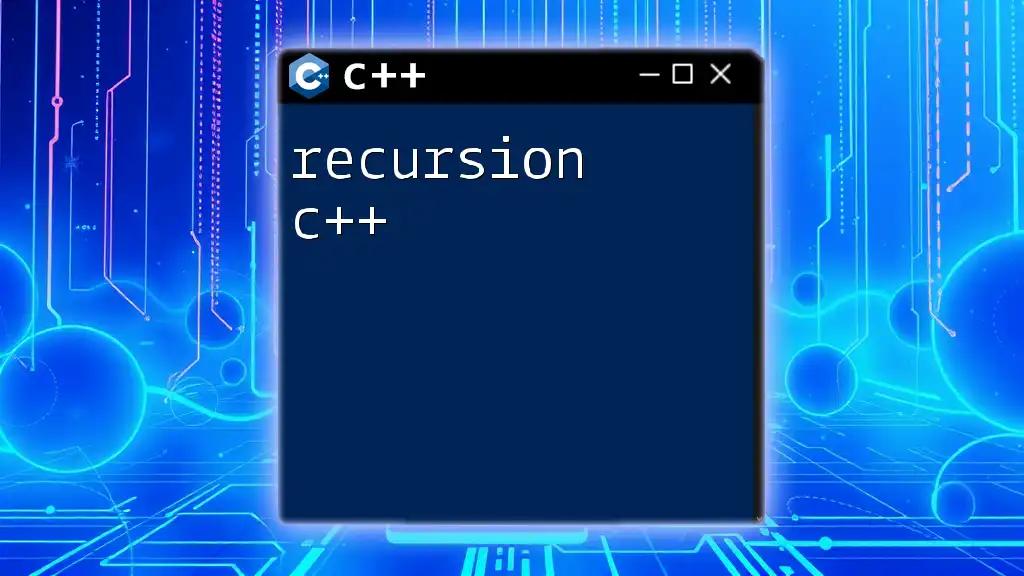
Conclusion
To master C++, an understanding of primary expressions is integral. These elements serve as the foundation for building complex expressions and executing various programming tasks. By grasping the intricacies of literals, identifiers, function calls, and the evaluation process, you will enhance your ability to write efficient, readable code.
Experiment with primary expressions in your projects and continue to explore the vast capabilities of C++. Each line of code you write brings you closer to proficiency in C++ programming.
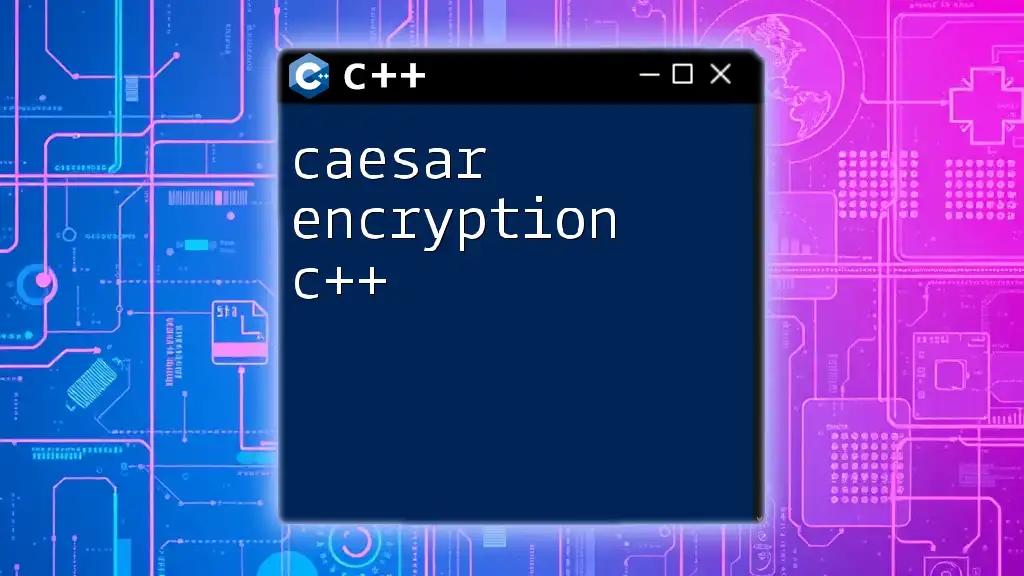
Additional Resources
As you enhance your knowledge, consider exploring recommended reading material, including books, online tutorials, and C++ documentation. These resources can provide deeper insights into the world of complex expressions, data structures, and more in C++.

Call to Action
Now that you have a comprehensive understanding of primary expressions in C++, it’s time to practice! Try experimenting with different expressions and observe how they behave within your code. Utilize online code sandboxes to refine your skills further!