Recursion in C++ is a programming technique where a function calls itself to solve smaller instances of the same problem, often used for tasks like calculating factorials or traversing data structures.
Here's a simple example of a recursive function to calculate the factorial of a number:
#include <iostream>
using namespace std;
int factorial(int n) {
if (n <= 1) return 1; // base case
return n * factorial(n - 1); // recursive case
}
int main() {
int number = 5;
cout << "Factorial of " << number << " is " << factorial(number) << endl;
return 0;
}
Understanding Recursion in C++
What is Recursion?
Recursion is a programming technique where a function calls itself in order to solve a problem. This method enables the development of elegant solutions for complex problems by breaking them down into smaller, more manageable subproblems. In C++, recursion plays a significant role in diverse algorithmic designs such as sorting, searching, and traversing data structures.
One of the major benefits of recursion is its ability to simplify the code. It often results in less code compared to iterative solutions. However, understanding recursion also comes with a downside: it can lead to performance issues and is sometimes harder to debug.
Key Concepts of Recursion
To effectively utilize recursion in C++, it is crucial to grasp some fundamental concepts:
-
Base Case: This is the condition under which the function stops calling itself. It prevents infinite recursion and is essential for the function to complete.
-
Recursive Case: This is where the function continues to call itself while making progress towards the base case. It is crucial to ensure that each recursive call brings the function closer to its base case.
-
Stack Overflow Consideration: Every recursive call consumes stack space. If too many calls are made before reaching the base case, the program may run out of stack space, leading to a stack overflow error.
-
Call Stack: Understanding how the call stack operates is vital. When a function calls itself, a new frame is pushed onto the call stack, and once a return statement is executed, that frame is popped off the stack.

C++ Recursion Function
Structure of a C++ Recursive Function
In C++, a recursive function generally has a straightforward structure. It consists of both a base case and a recursive case. As an example, let's implement a simple recursive function to calculate the factorial of a number:
#include <iostream>
using namespace std;
int factorial(int n) {
// Base case
if (n <= 1) return 1;
// Recursive case
return n * factorial(n - 1);
}
int main() {
int num = 5;
cout << "Factorial of " << num << " is " << factorial(num) << endl;
return 0;
}
In this example, `factorial` is the recursive function. The base case occurs when `n` is less than or equal to 1, returning 1. The recursive case multiplies `n` by the result of calling `factorial` with `n - 1`.
How C++ Handles Recursive Functions
C++ handles each recursive call by allocating memory on the call stack. For each function invocation, a new stack frame is created. This frame contains local variables and the state of the call. When the function completes, this frame is removed from the stack, allowing the previous state to resume. An understanding of memory usage in C++ recursion is paramount, especially when handling large input values.
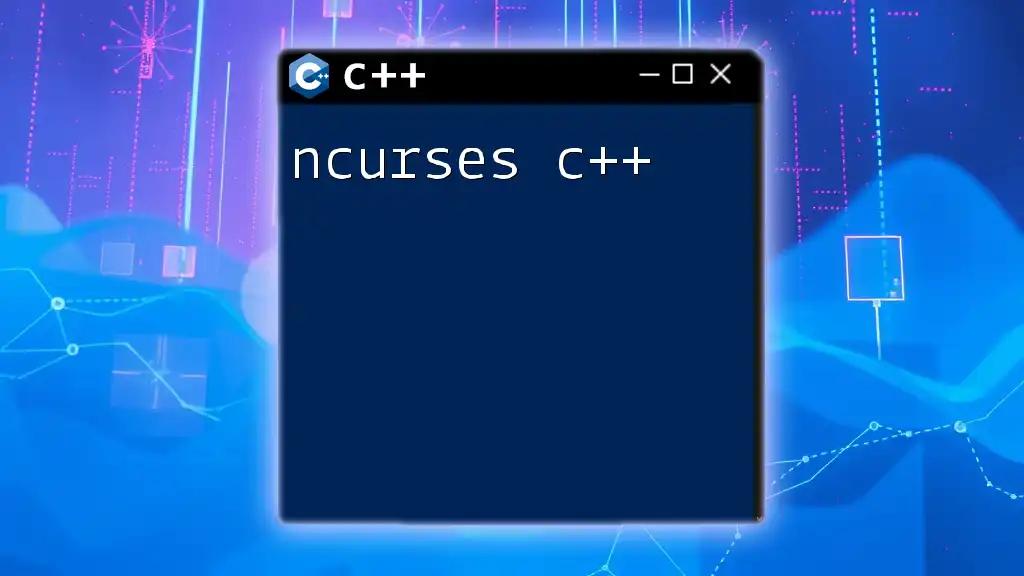
Implementing Recursive Methods in C++
Common Examples of Recursive Methods
Calculating Fibonacci Numbers
The Fibonacci sequence is another classic example of recursion. Each number in the series is the sum of the two preceding ones, starting from 0 and 1. Here's how to implement it in C++:
int fibonacci(int n) {
// Base case
if (n <= 1) return n;
// Recursive case
return fibonacci(n - 1) + fibonacci(n - 2);
}
In this case, the base case returns `n` for values `0` and `1`. For other values, the function recursively calls itself to sum the two previous Fibonacci numbers.
The Tower of Hanoi
The Tower of Hanoi is a puzzle that consists of three rods and a number of disks of different sizes. The objective is to move the entire stack to another rod, following specific rules. Here’s a C++ implementation of the solution:
void towerOfHanoi(int n, char fromRod, char toRod, char auxRod) {
// Base case
if (n == 1) {
cout << "Move disk 1 from rod " << fromRod << " to rod " << toRod << endl;
return;
}
// Recursive case
towerOfHanoi(n - 1, fromRod, auxRod, toRod);
cout << "Move disk " << n << " from rod " << fromRod << " to rod " << toRod << endl;
towerOfHanoi(n - 1, auxRod, toRod, fromRod);
}
In this function, the base case occurs when there is only one disk to move. The recursive case handles the transfer of `n - 1` disks to the auxiliary rod, moves the largest disk directly to the destination rod, and then recursively moves the disks from the auxiliary rod to the destination.
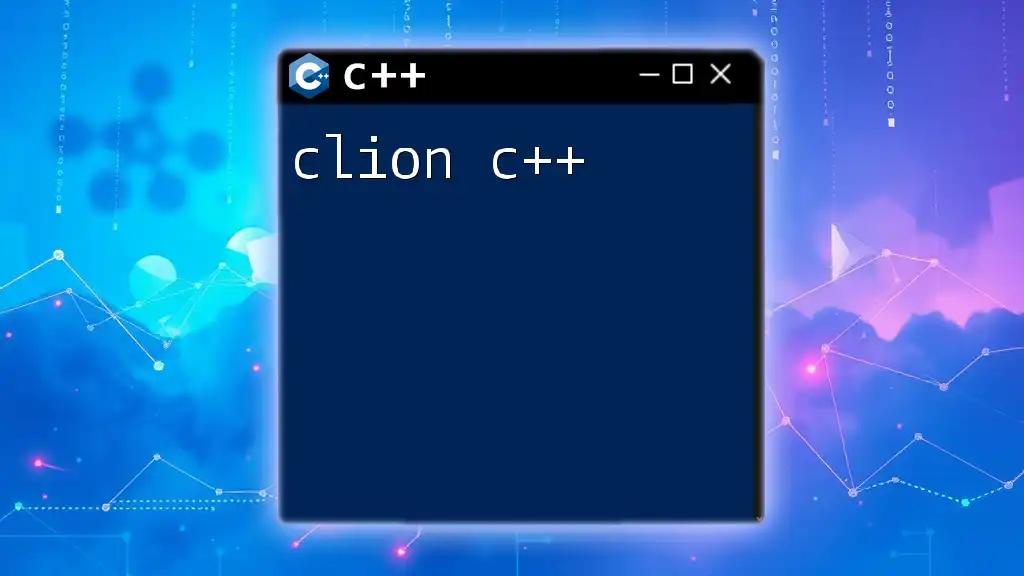
C++ Recursion in Detail
Advantages of Using Recursion
One of the prominent benefits of using recursion is elegance. Recursive solutions often require fewer lines of code, making them easier to read and maintain. Complex problems can be solved using a straightforward recursive method instead of convoluted looping constructs.
Disadvantages of Recursion
However, recursion comes with its challenges. The major downsides include:
-
Performance Issues: Recursive functions tend to have an overhead due to multiple function calls, consuming more CPU time compared to their iterative counterparts.
-
Risk of Stack Overflow: If the depth of recursion is too great (i.e., too many recursive calls before reaching the base case), the program risks crashing due to stack overflow.
-
Tail Recursion Optimization: While some languages optimize tail-recursive functions to avoid additional stack memory consumption, C++ does not guarantee such optimizations. Careful design is required to manage resource usage.
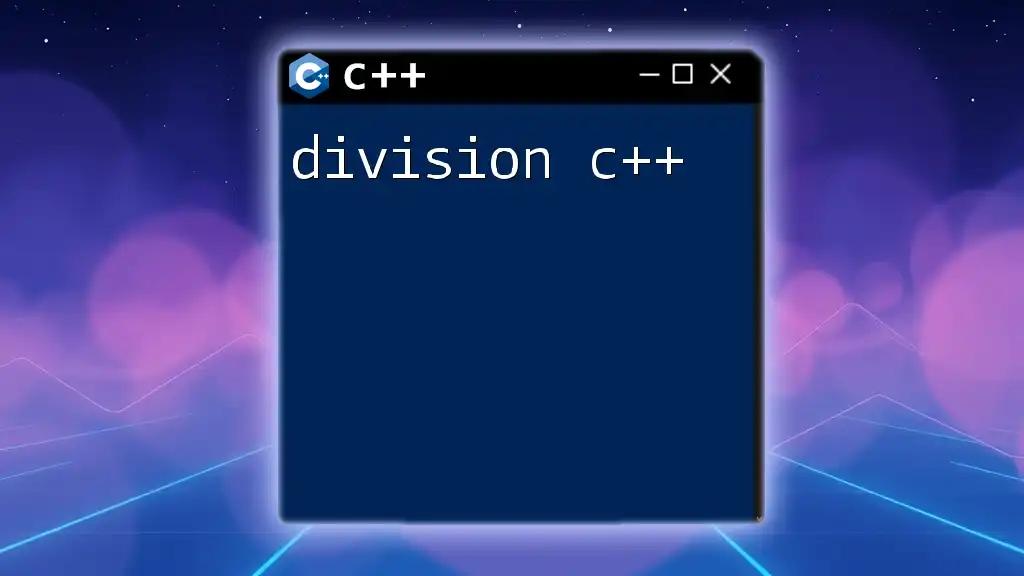
Best Practices for Writing Recursive Functions in C++
Tips for Effective Recursive Function Design
To write effective recursive functions, consider the following best practices:
-
Always Define a Clear Base Case: Ensure that your function has well-defined stopping criteria.
-
Ensure Progress Towards the Base Case: Each recursive call should advance towards the base condition to avoid infinite loops.
-
Avoid Unnecessary Calculations: Use techniques such as memoization to store results of expensive function calls to improve performance.
Debugging Recursive Functions
Debugging recursion can be challenging. Here are some techniques to aid in the process:
-
Trace with Print Statements: Use print statements generously to follow the flow of recursive calls, observing the values of variables at different stages.
-
Limit Input Size: When testing, start with small input sizes to verify correctness before scaling up.
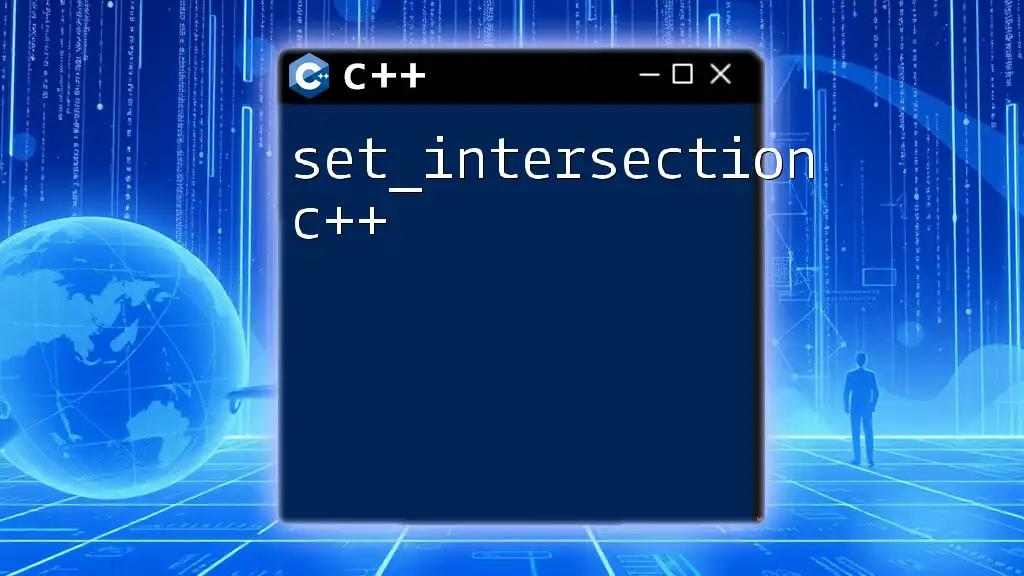
Conclusion
Recursion in C++ presents a powerful tool for solving complex problems with elegant and readable code. Understanding its concept, advantages, and challenges is essential for any programmer.
To master recursion, practice implementing various problems and explore innovative solutions using this technique. Leveraging the array of resources available, including online platforms and coding challenges, can aid in deepening your understanding and proficiency in recursion.