Recursion in C++ is a programming technique where a function calls itself directly or indirectly to solve a problem, often simplifying complex tasks like calculating factorials or traversing data structures.
Here’s a simple example of a recursive function to calculate the factorial of a number:
#include <iostream>
int factorial(int n) {
return (n == 0) ? 1 : n * factorial(n - 1);
}
int main() {
int number = 5;
std::cout << "Factorial of " << number << " is " << factorial(number) << std::endl;
return 0;
}
What is Recursion?
Recursion is a programming technique where a function calls itself to solve smaller instances of a problem. It’s often used to break down complex problems into simpler, more manageable sub-problems. This method can lead to more elegant and concise code, especially for tasks that can be defined in terms of smaller instances of the same task.
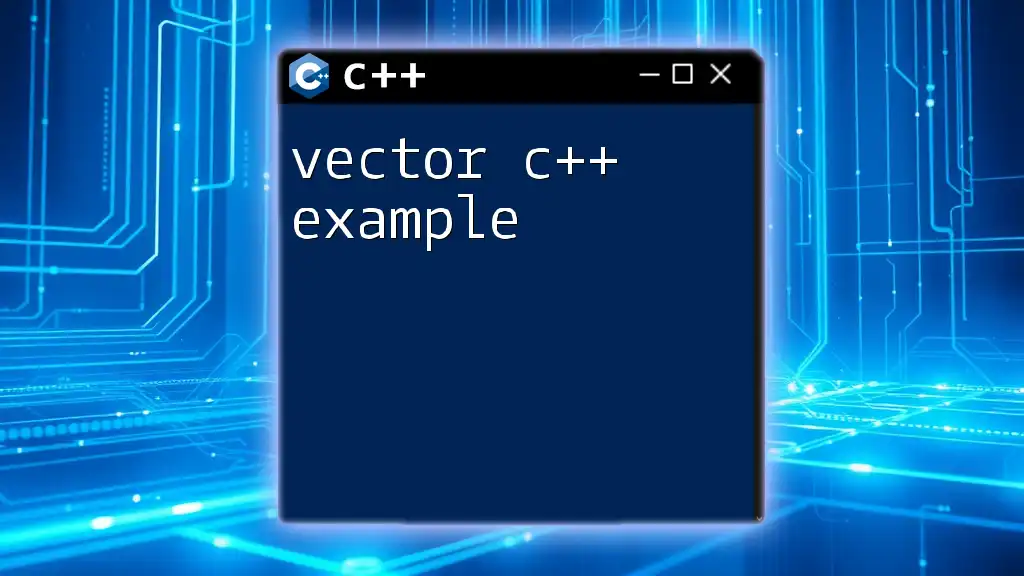
How Recursion Works
A recursive function typically consists of two main components:
- Base Case: This is a condition under which the recursive function will stop calling itself. It is crucial to prevent infinite recursion, which can lead to stack overflow errors.
- Recursive Case: This is where the function calls itself with modified arguments, gradually moving towards the base case.
Understanding how recursion utilizes stack memory is essential. Each function call is pushed onto the call stack. When the base case is reached, the function begins to return values, which pop off the stack in reverse order.
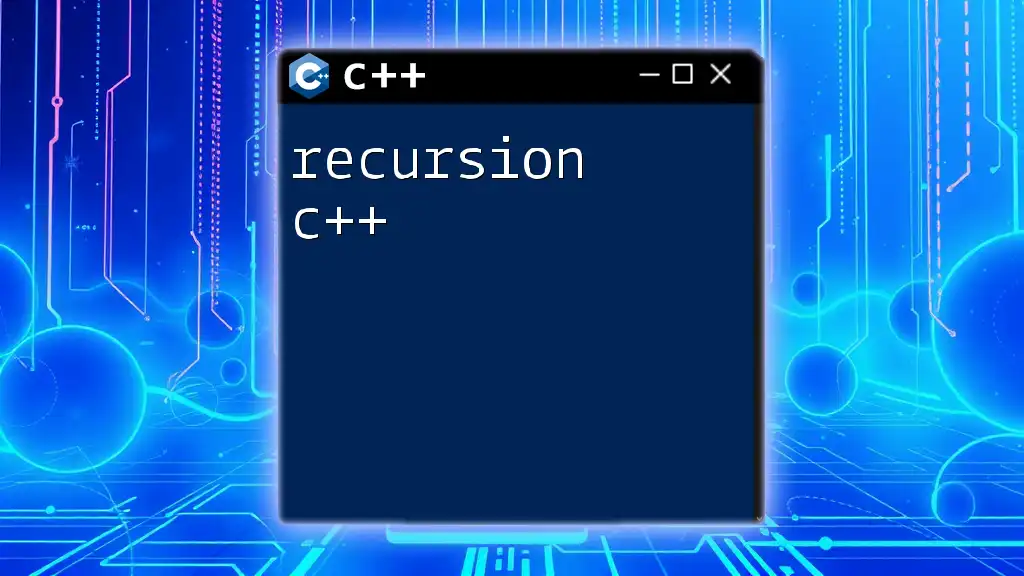
Understanding C++ Recursion Functions
Defining a C++ Recursion Function
In C++, a recursion function is defined just like any other function, with the key difference being that it calls itself. The typical syntax includes the return type, function name, parameters, and the body, which contains conditions for both the base and recursive cases.
Common Characteristics of Recursion
Recursion can often lead to simpler and more readable code, particularly when problems display a clear pattern. However, it also has its downsides, such as memory usage and the potential for reduced performance.
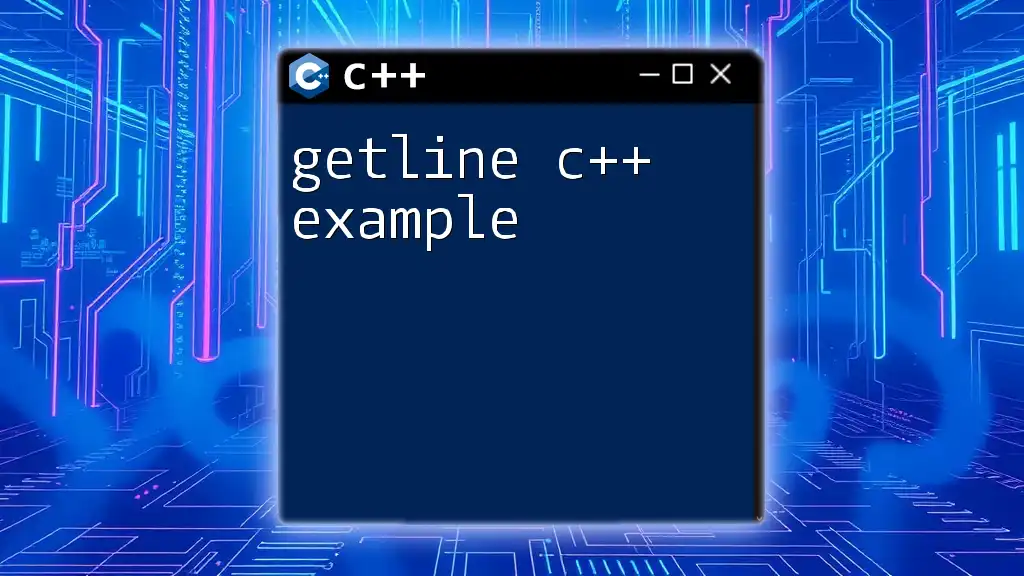
Practical Examples of Recursion in C++
Factorial of a Number
A classic example of recursion is calculating the factorial of a number. Factorial is defined as the product of all positive integers up to a given number `n`.
int factorial(int n) {
if (n <= 1) return 1; // Base case
return n * factorial(n - 1); // Recursive case
}
In the above example, when `factorial(5)` is called, the function evaluates `5 * factorial(4)`, and this pattern continues until it reaches the base case. Thus, the calculation proceeds as follows:
`5 * 4 * 3 * 2 * 1 = 120`.
Fibonacci Sequence
Another well-known example is generating Fibonacci numbers, where each number is the sum of the two preceding ones.
int fibonacci(int n) {
if (n <= 1) return n; // Base case
return fibonacci(n - 1) + fibonacci(n - 2); // Recursive case
}
Calling `fibonacci(5)` leads to a series of recursive calls:
`fibonacci(5) = fibonacci(4) + fibonacci(3)`, and so on. This continues until it hits the base case of `n = 0` or `n = 1`, yielding the result: `0, 1, 1, 2, 3, 5`.
Finding the Greatest Common Divisor (GCD)
The GCD of two integers is the largest number that divides both without leaving a remainder. The Euclidean algorithm is a classic recursion approach for finding the GCD.
int gcd(int a, int b) {
if (b == 0) return a; // Base case
return gcd(b, a % b); // Recursive case
}
By calling `gcd(48, 18)`, the function follows the path:
`gcd(48, 18) → gcd(18, 12) → gcd(12, 6) → gcd(6, 0)`, eventually returning `6`.
Reverse a String Using Recursion
Reversing a string is another interesting problem that can be solved using recursion.
std::string reverseString(const std::string& str) {
if (str.empty()) return str; // Base case
return str.back() + reverseString(str.substr(0, str.size() - 1)); // Recursive case
}
When `reverseString("hello")` is called, the function will work its way back through the string, ultimately reconstructing it into `"olleh"`.
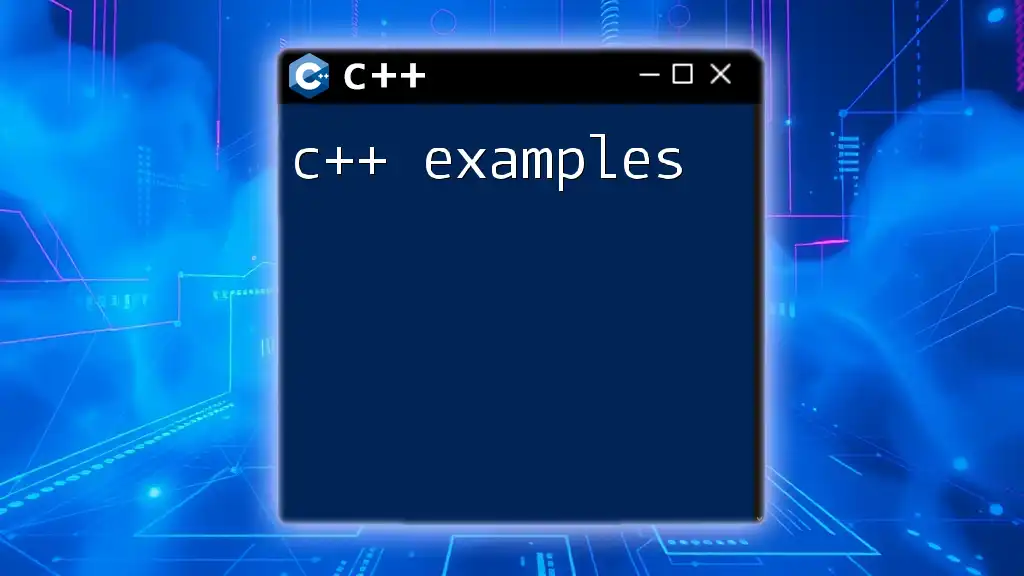
Advantages of Using Recursion
Simplicity and Clarity
One of the main advantages of recursion is simplicity. Many problems, especially those related to data structures like trees and graphs, are often easier to express with recursion due to their inherently recursive nature.
Divide-and-Conquer Approach
Recursion often embraces the divide-and-conquer strategy, splitting a problem into sub-problems, solving them independently, and then combining results. This method is seen in algorithms such as quicksort and mergesort.
Memory and Stack Depth Considerations
While recursion provides elegant solutions, it has memory usage implications. Each function call consumes stack space, which may lead to stack overflow if the recursion depth is too great, particularly for deeply recursive functions.
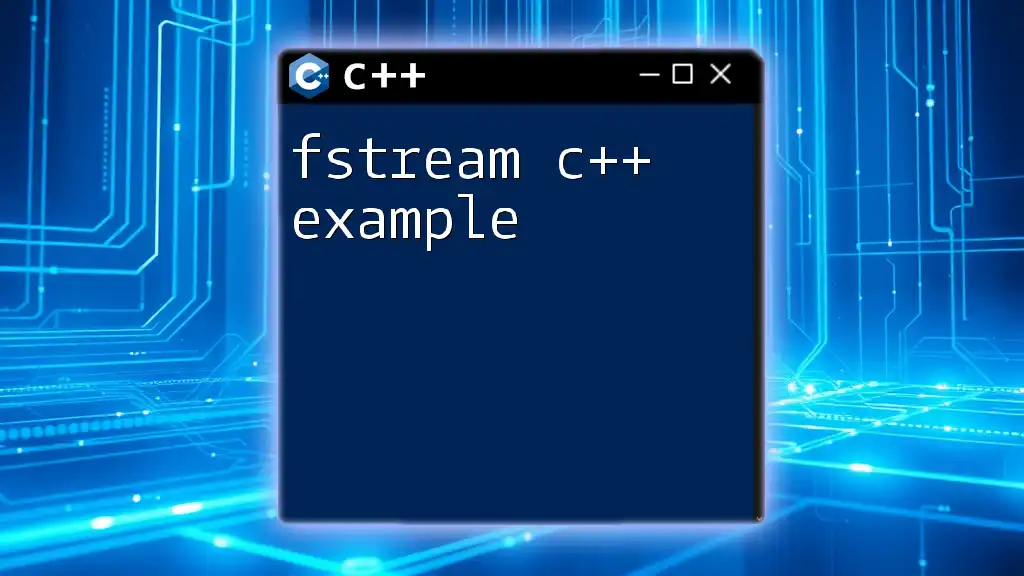
Common Pitfalls & How to Avoid Them
Excessive Recursion Depth
Stack overflow is a major risk associated with recursion. Always ensure that your base cases are correct and reachable. It's vital to analyze the efficiency of your recursive solutions.
Performance Concerns
Although recursion can simplify coding, it is not always the most efficient approach. Utilize memoization or dynamic programming to optimize recursive algorithms, especially in cases like calculating Fibonacci numbers, where overlapping subproblems can lead to excessive redundant calculations.
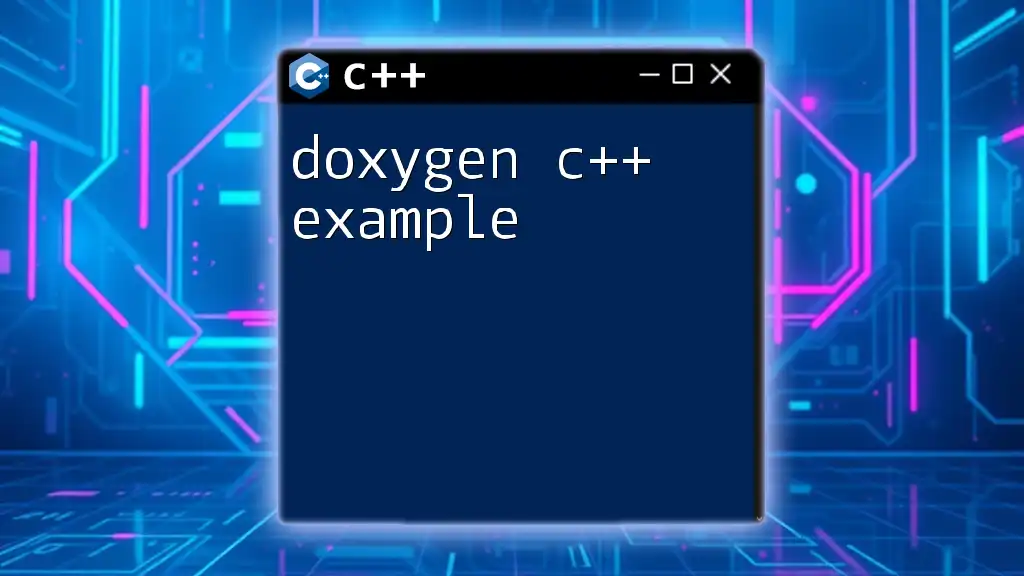
Conclusion
Recursion is a powerful concept in C++. The examples provided showcase how recursion can solve various problems elegantly, although it is essential to be aware of potential pitfalls such as stack overflow and performance issues. Engaging with these recursion C++ examples can deepen your understanding and help you develop efficient algorithms.
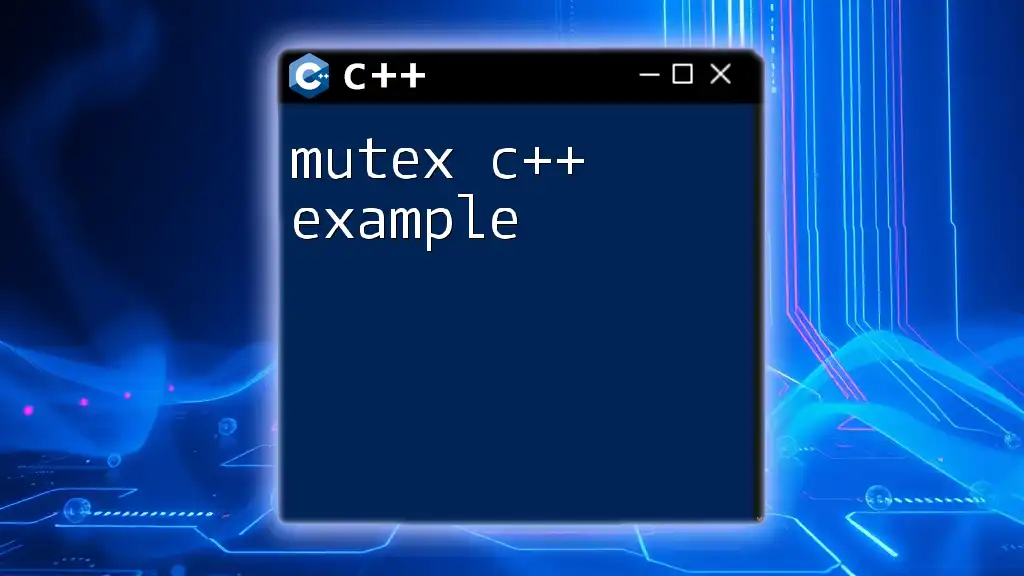
Further Reading Resources
For readers keen on expanding their knowledge, consider exploring online coding platforms or C++ documentation. Practical exercises will significantly enhance your grasp of recursion and its applications in C++. Happy coding!
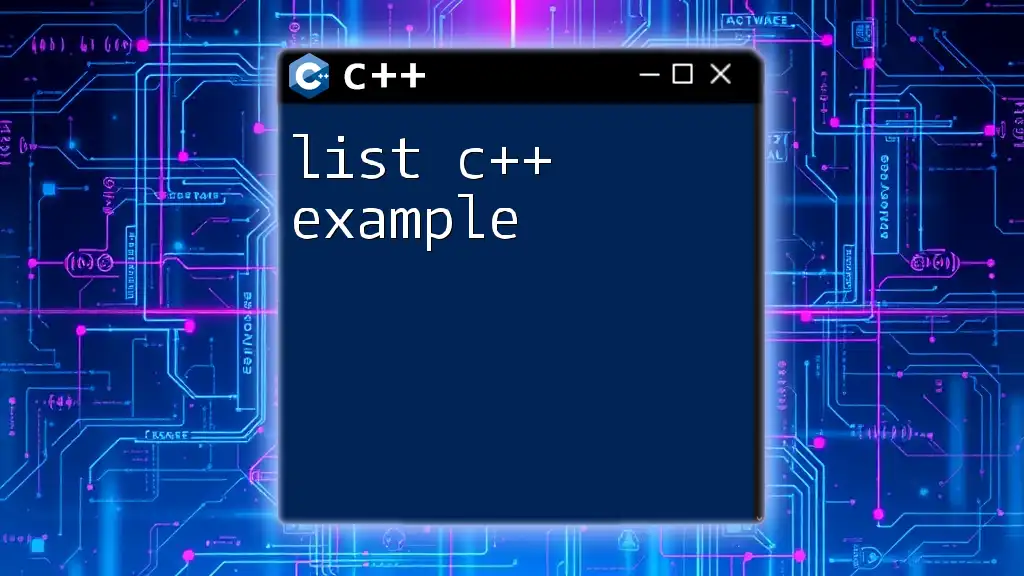
FAQs
What is the difference between recursion and iteration?
Recursion calls a function within itself, while iteration uses loops. Recursion can be more intuitive for problems defined recursively, but iteration can be more memory efficient.
How can I optimize my recursive functions?
You can use techniques such as memoization, where you cache results of expensive function calls and return the cached result when the same inputs occur again.
Are there scenarios where recursion is not appropriate?
Yes, recursion may not be suitable for all problems, particularly those that require a large number of recursive calls or involve deep recursion due to limitations in stack size. In these cases, iterative solutions may be more efficient.