The `getline` function in C++ is used to read a line of text from an input stream, and it can be utilized as shown in the following example:
#include <iostream>
#include <string>
int main() {
std::string line;
std::cout << "Enter a line of text: ";
getline(std::cin, line);
std::cout << "You entered: " << line << std::endl;
return 0;
}
What is getline in C++?
The `getline` function in C++ is a powerful tool for reading string input from the user. Unlike traditional input techniques that can break up user input at whitespace, `getline` reads an entire line of text, making it perfect for capturing input that may contain spaces or special characters. This becomes especially relevant in situations where the user's input is not limited to single words, such as names or addresses.
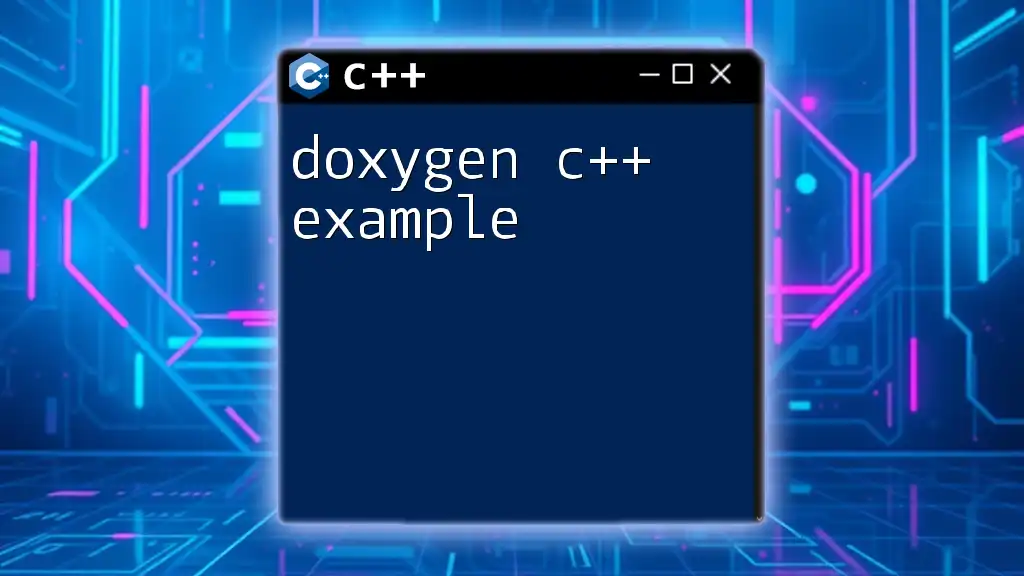
Overview of the Use Cases for getline
When to Use getline Instead of Standard Input Methods
- Capturing Complete Lines: When you need to capture an entire line of input, including spaces (e.g., a full address).
- Reading User Responses: For quiz or survey applications where participants may provide detailed responses.
- Parsing Formatted Data: When working with configuration files or CSV data where fields are separated by line breaks rather than whitespace.
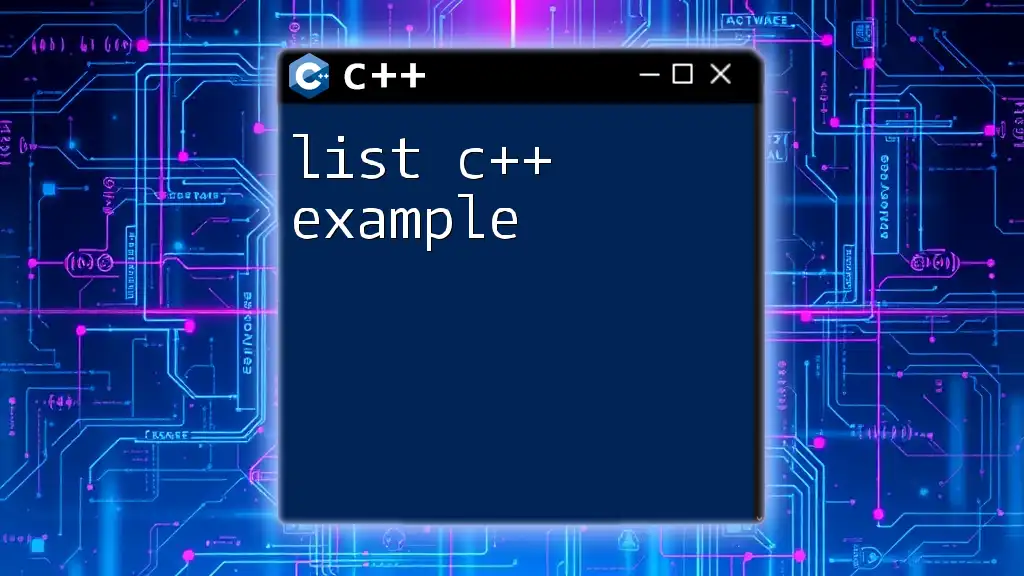
Understanding the Syntax of getline
Basic Syntax of getline
The general structure for `getline` is as follows:
std::getline(std::istream& is, std::string& str);
Parameters Explained
- istream& is: This parameter specifies the input stream from which data is read. It could be standard input (e.g., `std::cin`) or a file input stream.
- string& str: This is where the content of the line is stored. After the function executes, `str` will contain the entire line that was read, allowing you to manipulate or use it as needed.
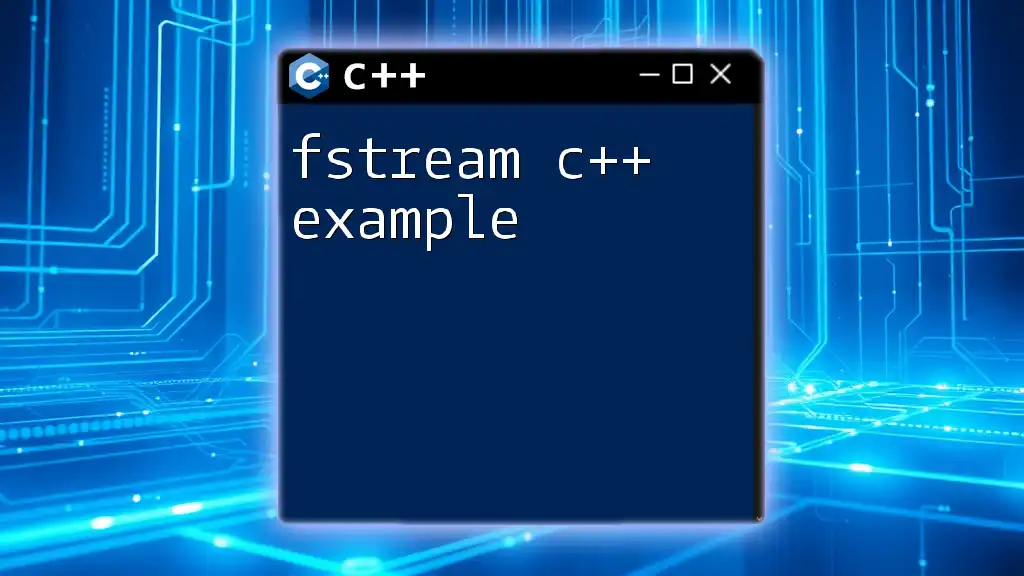
How to Use getline in C++
Basic Example of Using getline
Here’s a simple example demonstrating how to use `getline`:
#include <iostream>
#include <string>
int main() {
std::string input;
std::cout << "Enter a sentence: ";
std::getline(std::cin, input);
std::cout << "You entered: " << input << std::endl;
return 0;
}
This code snippet prompts the user to enter a sentence and reads that entire line, including any spaces.
Handling Whitespace and Line Breaks
One of the key features of `getline` is its ability to handle whitespace. Unlike `std::cin`, which stops reading input at the first whitespace, `getline` allows spaces to be included in the input.
std::string fullLine;
std::cout << "Enter a full line with spaces: ";
std::getline(std::cin, fullLine);
If the user inputs, "Hello, world!", the entire phrase is captured in `fullLine`.
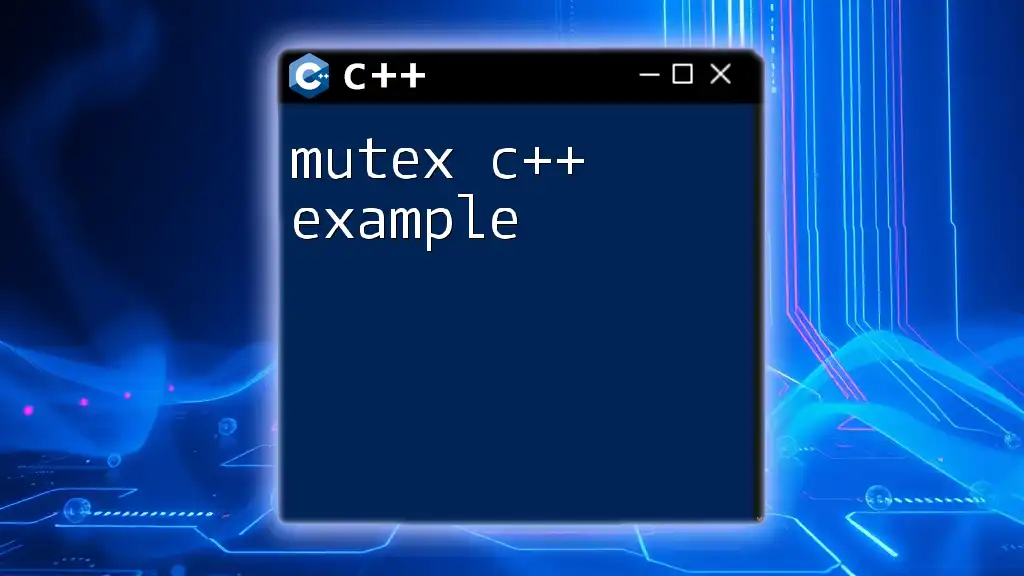
Advanced Usage of getline
Using getline with Different Data Types
Often, you might want to convert the string input into different data types, such as integers or floats. After taking input with `getline`, you can perform conversion using functions such as `std::stoi` for integers or `std::stof` for floats.
std::string input;
std::cout << "Enter a number: ";
std::getline(std::cin, input);
int number = std::stoi(input); // Convert string to int
std::cout << "You entered the number: " << number << std::endl;
Incorporating Delimiters in getline
`getline` can also accept a third parameter, which allows you to specify a delimiter other than the default newline character. This is helpful in parsing structured inputs.
std::string field;
std::cout << "Enter comma-separated values: ";
std::getline(std::cin, field, ','); // Reads until ','
std::cout << "First value: " << field << std::endl;
In this example, only the text before the first comma will be read into `field`.
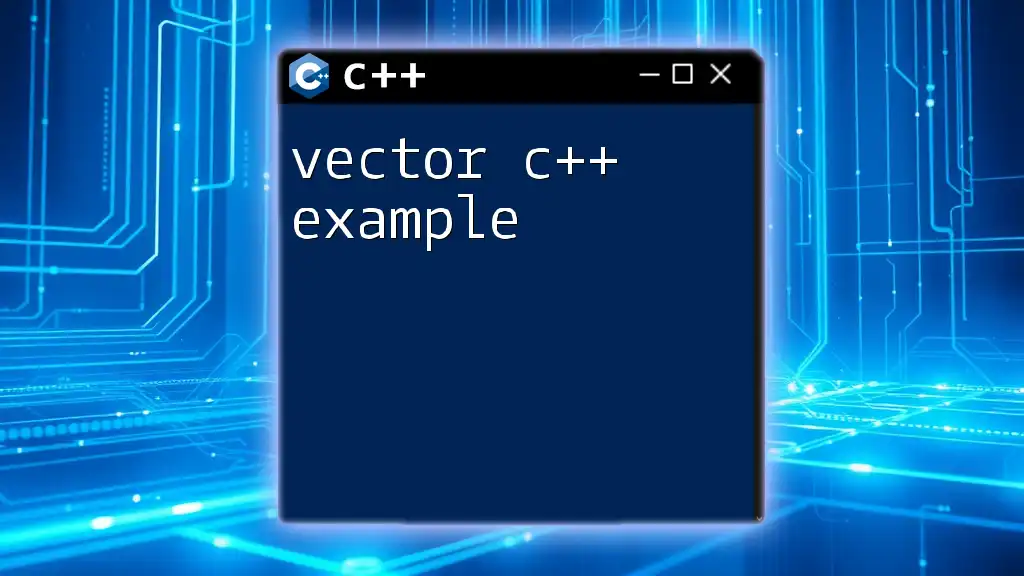
Error Handling with getline
Common Pitfalls with getline
Even though `getline` is user-friendly, it can have its own pitfalls. If the user inputs a non-string value or if the stream is in a fail state (e.g., there's an attempt to read from a closed file), it can lead to unexpected behavior.
Ensuring Safe Input
To ensure input validity, you can implement checks. For instance, you can check if `getline` succeeds in reading and if the input is not empty.
std::string input;
std::cout << "Enter something: ";
if (std::getline(std::cin, input) && !input.empty()) {
std::cout << "You entered: " << input << std::endl;
} else {
std::cout << "No input received!" << std::endl;
}
In this snippet, if the input is empty or reading fails, an appropriate message is displayed.
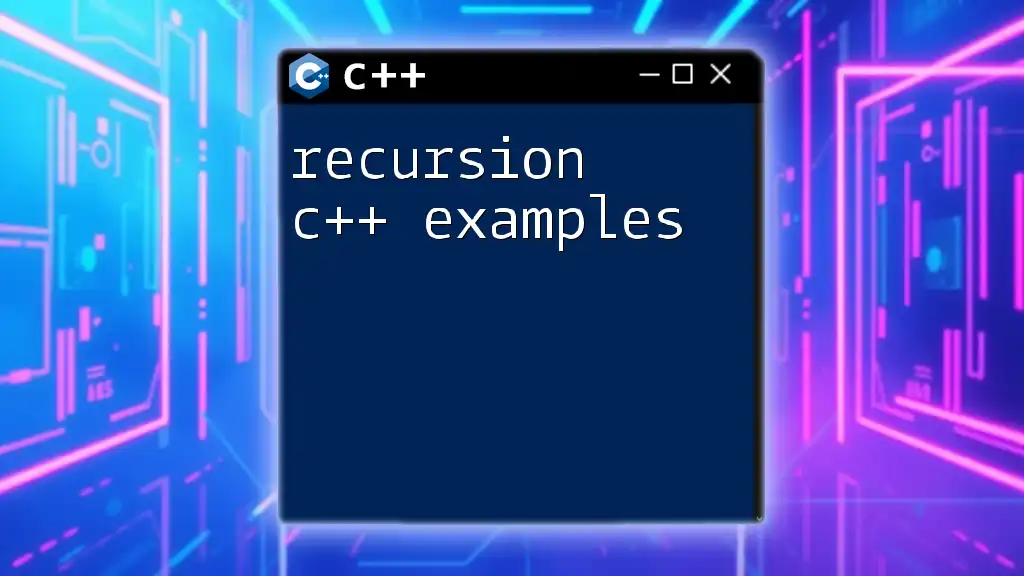
Practical Applications of getline
Real-World Scenarios Where getline is Useful
`getline` is often used in applications requiring multiple lines of user input, such as chat applications, form entries, and data collection tools.
Code Example: Reading Multiple Lines of Input
You can use a loop to read multiple lines from the user until a certain condition is met. Below is an example of reading multiple lines of text:
std::string line;
std::cout << "Enter text (type 'exit' to finish): " << std::endl;
while (std::getline(std::cin, line) && line != "exit") {
std::cout << "You entered: " << line << std::endl;
}
This code allows users to enter text continuously until typing 'exit' stops the input process.
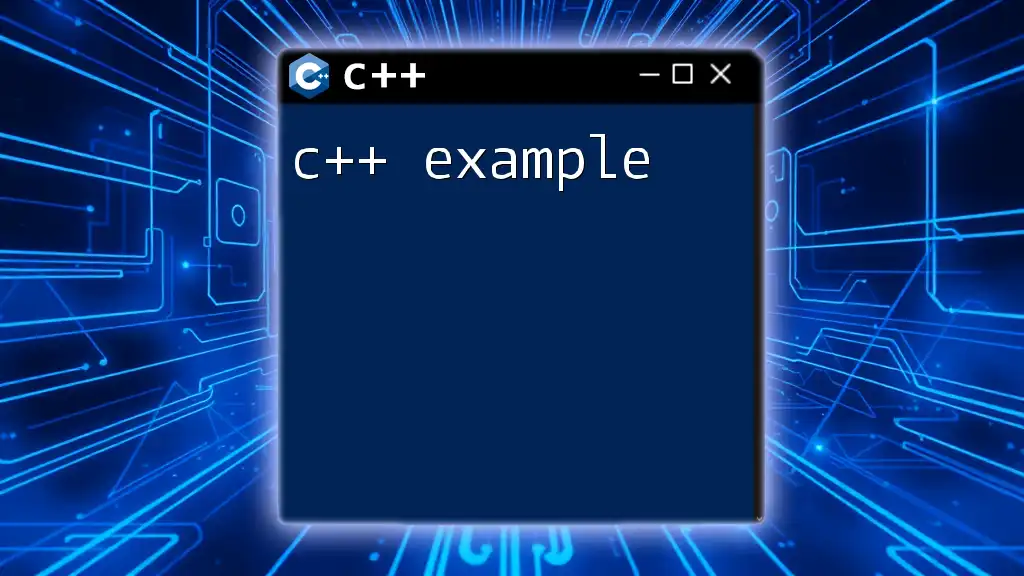
Performance Considerations
Impact of getline on Performance
While `getline` is a robust solution for user input, be mindful of its performance, especially when reading large volumes of data. It allocates memory dynamically for strings, so repeated calls could lead to inefficiencies in memory management.
Comparison with Other Input Techniques
Using `getline` provides a clear advantage over the standard extraction operator (`>>`) when you need to read entire lines of input, including whitespace. Although `cin >>` can be more performant for single word inputs, it falls short for full line captures.
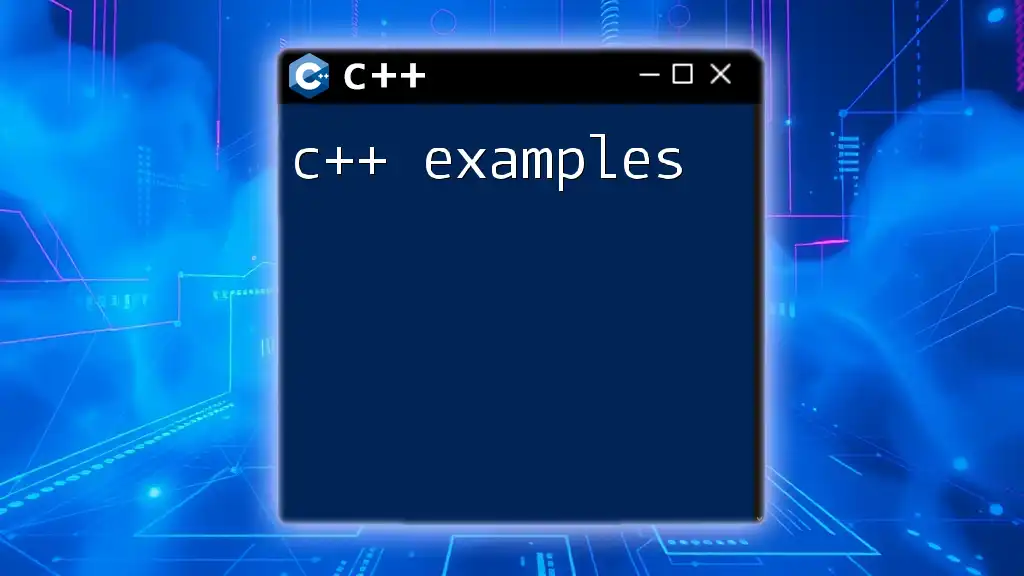
Conclusion
In summary, `getline` is a versatile function for reading user input in C++. Its ability to capture an entire line, including spaces, makes it particularly useful in a variety of scenarios—from user interactivity to data parsing. By practicing different usage patterns with `getline`, you'll enhance your input handling capabilities in C++ programs.
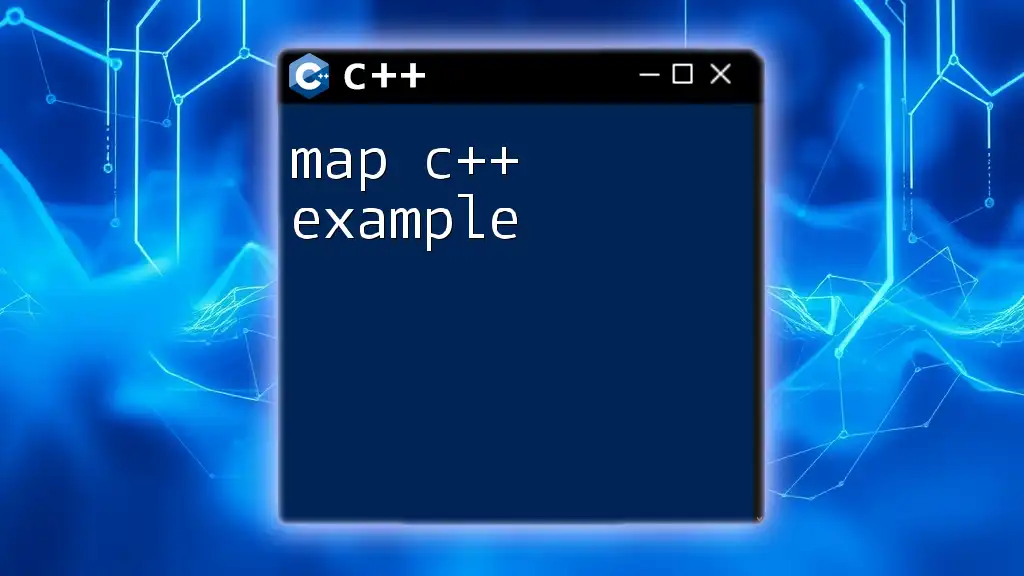
Call to Action
Engage with our community! Share your own experiences and examples of using `getline` in C++. Also, don't miss out on our upcoming workshops and tutorials focused on modern C++ commands and techniques designed to make your programming journey more efficient and enjoyable.