Beginning C++ game programming involves learning the fundamentals of C++ to create engaging games, utilizing basic concepts such as variables, control flow, and functions.
Here’s a simple example that demonstrates how to create a basic game loop in C++:
#include <iostream>
int main() {
bool isPlaying = true;
while (isPlaying) {
std::cout << "Playing the game! (Type '0' to quit)\n";
int input;
std::cin >> input;
if (input == 0) {
isPlaying = false;
}
}
std::cout << "Game over!\n";
return 0;
}
Understanding Game Programming
What is Game Programming?
Game programming encompasses the processes and techniques involved in creating video games, including writing the source code, developing algorithms, and implementing game mechanics. It serves as a critical facet of game development, translating concepts and designs into an interactive experience for players. Whether for casual games or complex AAA titles, the developer's choice of programming language significantly influences the outcome.
The Role of C++ in Game Development
Why Choose C++? C++ is a powerhouse in the gaming industry, renowned for its performance and efficiency. Unlike higher-level languages, C++ provides greater control over system resources—crucial for real-time games where speed is paramount. It is the primary language for many industry-standard game engines, like Unreal Engine, which thrives on C++ for its core functionality. Furthermore, familiarity with C++ affords developers access to a plethora of libraries and frameworks specifically designed for game programming, making it an indispensable tool in a developer's arsenal.
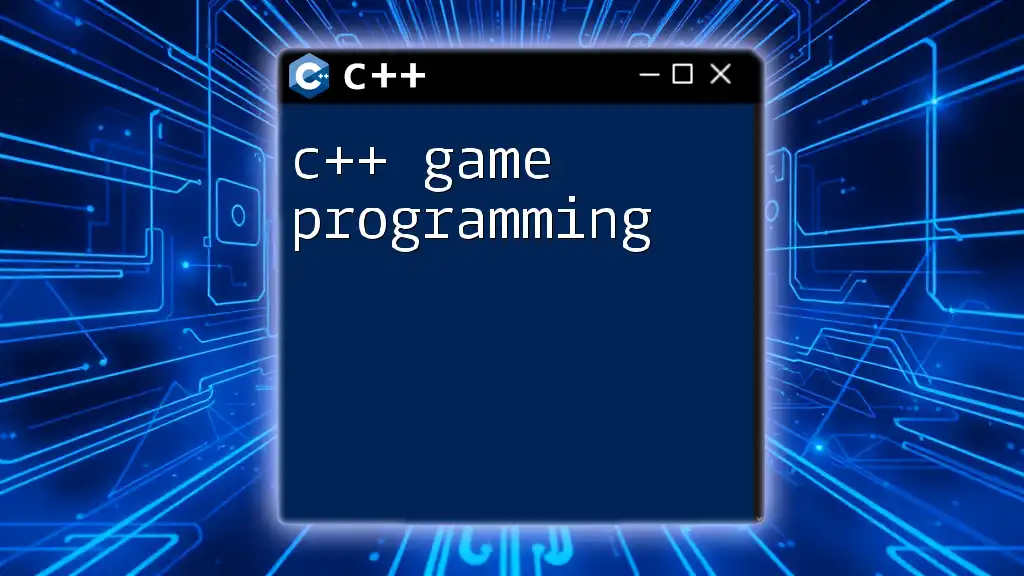
Getting Started with C++
Setting Up Your Development Environment
Choosing a Text Editor or IDE
Selecting a robust Integrated Development Environment (IDE) or text editor is the first step toward effective coding in C++. Options include:
- Visual Studio: A powerful IDE with extensive features, perfect for C++ game development.
- Code::Blocks: An open-source option that is lightweight and user-friendly.
- CLion: A versatile cross-platform IDE that includes various C++ tools.
Make sure to download and install the IDE according to the provided installation guide. Proper setup makes coding smoother and more efficient.
Installing C++ Compiler
To execute C++ code, developers must install a compiler. Two popular options are:
- GCC (GNU Compiler Collection): A versatile compiler widely used on various platforms.
- MSVC (Microsoft Visual C++): Integrated into Visual Studio, offering robust debugging and profiling tools.
Follow the official documentation to ensure proper installation.
Writing Your First C++ Program
Basic Structure of a C++ Program
Every C++ program has a structure that includes headers, a main function, and other components necessary for operation. Here’s a simple example:
#include <iostream>
int main() {
std::cout << "Hello, C++ Game Programming!" << std::endl;
return 0;
}
Explanation:
- `#include <iostream>`: This includes the standard input-output stream, essential for handling inputs and outputs.
- `int main()`: Defines the main function—this is the entry point of the program.
- `std::cout`: Utilizes the output stream to display text on the console.
This foundational structure is critical for any further C++ development.
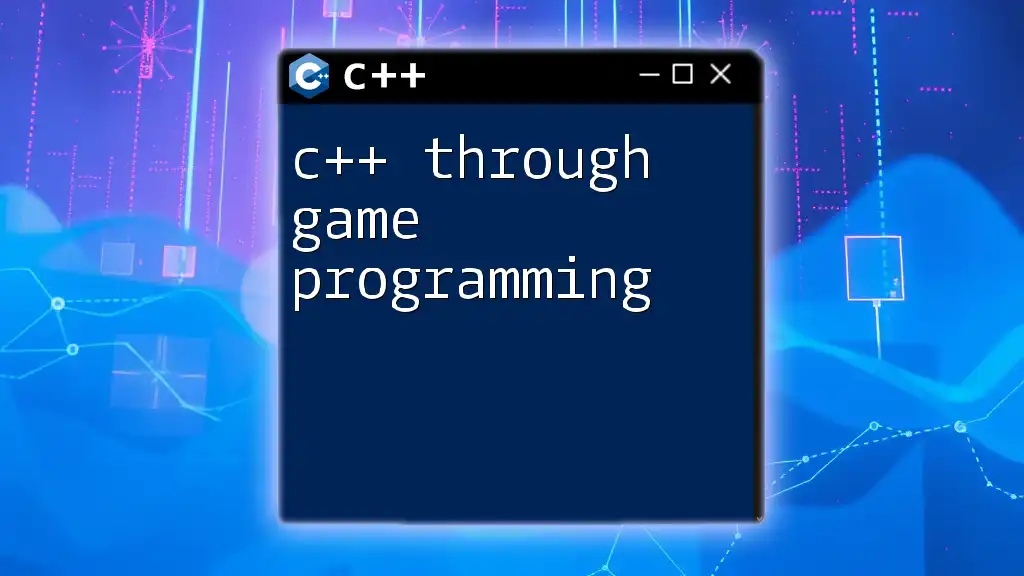
Understanding Basic C++ Concepts
C++ Syntax and Structure
Variables and Data Types
C++ supports multiple data types including `int`, `float`, `double`, `char`, and `bool`. Understanding these types enables developers to store and manipulate data effectively.
Examples:
int playerScore = 0; // Integer for score
float playerHealth = 100.0f; // Float for health
char playerSymbol = 'A'; // Character for representation
Local vs. Global Variables:
- Local variables are declared within functions and cannot be accessed outside.
- Global variables can be accessed from any function, but excessive use can lead to conflicts and make debugging challenging.
Control Structures
If Statements and Loops
Control structures in C++ enable conditional execution and iteration.
Example of If-Else Statement:
if (playerScore > 100) {
std::cout << "You are a high scorer!" << std::endl;
} else {
std::cout << "Keep trying!" << std::endl;
}
Loops enable repetition, crucial for tasks such as continuously checking player input. Here’s a simple loop example:
for (int i = 0; i < 5; i++) {
std::cout << "This is loop iteration " << i << std::endl;
}
Functions and Parameters
Functions encapsulate code for reusability. They can accept parameters and return values, streamlining programs. Here’s a basic example of a function:
int add(int a, int b) {
return a + b;
}
// Function call
int sum = add(5, 3);
Understanding functions empowers developers to organize code better and avoid repetition, which is crucial when building large game systems.
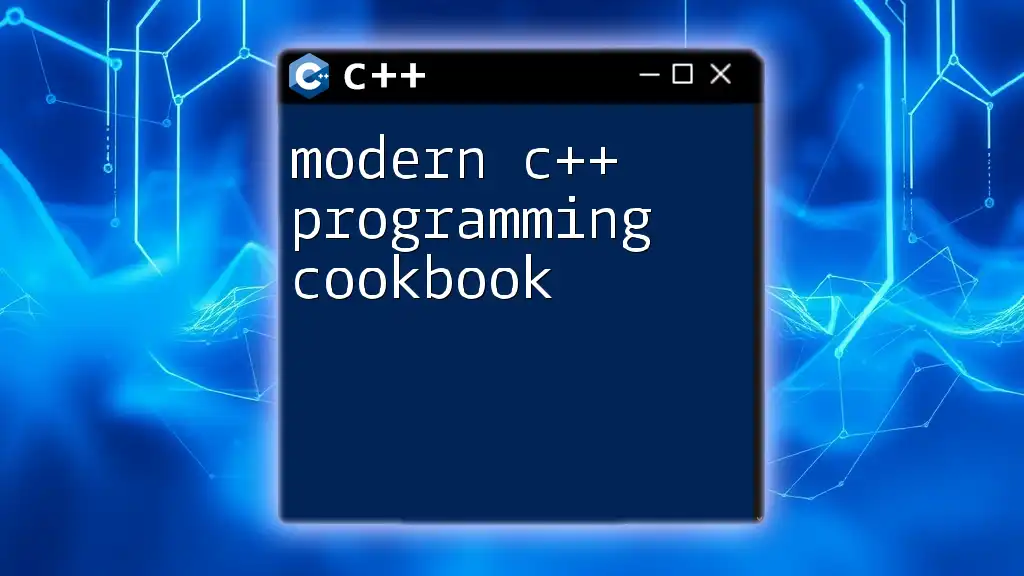
Basic Game Programming Concepts
Game Loop Structure
The game loop is the heart of any game, constantly running to update the game status, render graphics, and manage player inputs. It usually consists of initialization, the main loop, and shutdown procedures. Here’s a basic outline:
while (gameIsRunning) {
processInput();
updateGame(); // Update game state
renderGraphics(); // Render to the screen
}
Each iteration captures player actions, updates the game state, and redraws the graphics, ensuring dynamic engagement.
Handling User Input
In game programming, capturing user input is essential. C++ can utilize libraries like SDL or SFML for advanced input handling. Here's a simple approach using pseudo-code:
if (keyPressed("UP")) {
// Move player up
} else if (keyPressed("DOWN")) {
// Move player down
}
This example exhibits how to respond to keyboard inputs, driving character movement based on player choices.
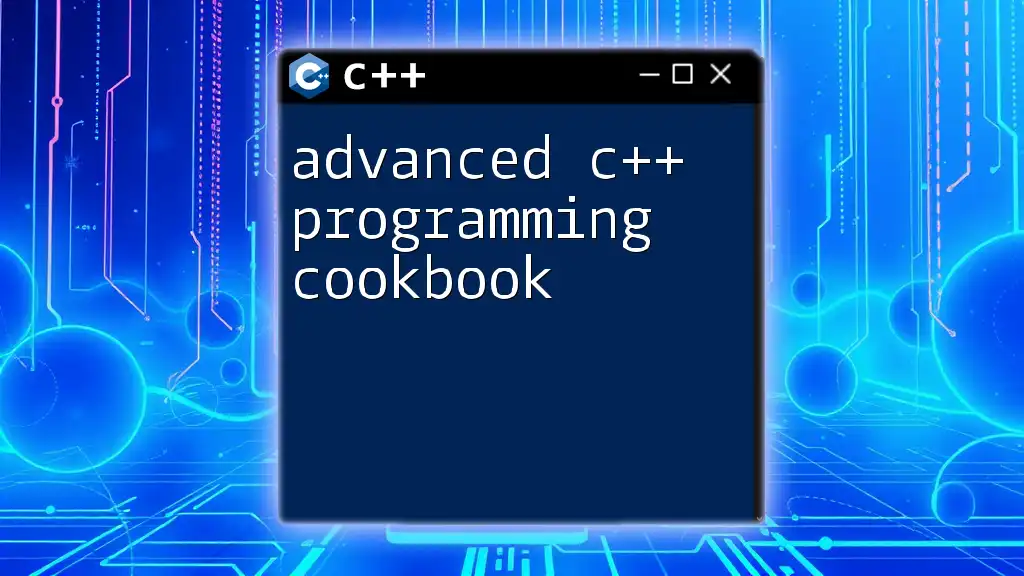
Building a Simple Game
Developing a Text-Based Game
Concept of a Text Adventure
Creating a text-based game is an enriching introduction to programming logic and control flow. Players interact through text, making choices that lead to different outcomes.
Example Game: Guess the Number
Game Design Overview
In this game, players must guess a randomly generated number within a specific range. Incorporating loops and conditionals allows for continuous guessing until the correct number is found.
Code Example
Here’s the complete C++ code for a simple "Guess the Number" game:
#include <iostream>
#include <cstdlib>
#include <ctime>
int main() {
std::srand(static_cast<unsigned int>(std::time(nullptr)));
int numberToGuess = std::rand() % 100 + 1;
int playerGuess;
do {
std::cout << "Enter your guess (1-100): ";
std::cin >> playerGuess;
if (playerGuess > numberToGuess) {
std::cout << "Too high! Try again.\n";
} else if (playerGuess < numberToGuess) {
std::cout << "Too low! Try again.\n";
}
} while (playerGuess != numberToGuess);
std::cout << "Congratulations! You guessed the number.\n";
return 0;
}
Explanation:
- The game initializes by generating a random number between 1 and 100.
- Using a do-while loop, players input guesses until they guess correctly, with feedback after each attempt to help guide their next guess.
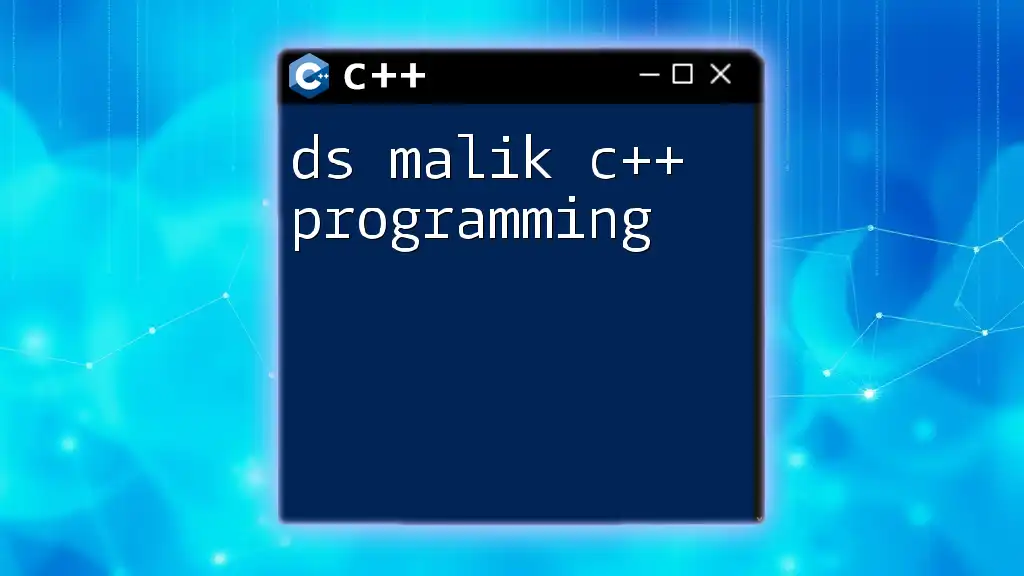
Moving Forward
Introduction to Game Engines
What is a Game Engine? A game engine is software designed to aid in the development of video games, offering tools for graphics rendering, physics simulation, sound integration, and more, which significantly streamlines the development process compared to coding everything from scratch. Engines like Unity and Unreal Engine extend their flexibility and powerful capabilities to developers.
Learning Resources
To further hone your skills in C++ and game programming, explore the following resources:
- Books: Look into titles like "Programming Principles and Practice Using C++" by Bjarne Stroustrup.
- Online Courses: Platforms like Udemy and Coursera offer comprehensive C++ courses tailored for beginners.
- Tutorials: Participate in game-specific tutorials on YouTube or dedicated programming websites.
Getting Involved in the Community
Joining programming communities can be immensely beneficial. Engage with fellow learners on platforms like Stack Overflow, Reddit, and GitHub. Sharing your journey and asking questions invites collaboration and accelerated learning.
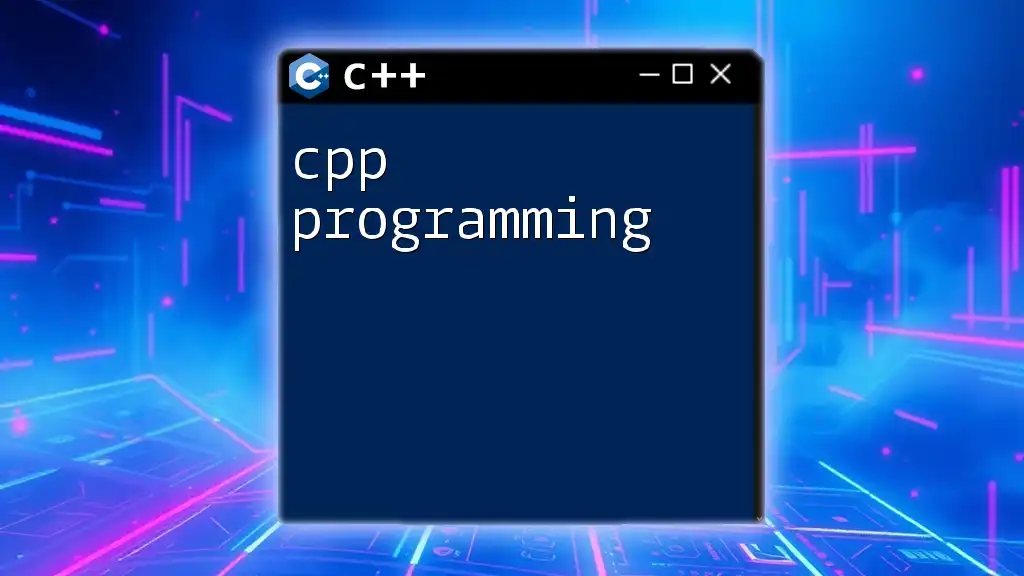
Conclusion
In summary, this guide has illuminated the fundamentals of beginning C++ game programming. From setting up the environment to understanding core concepts and creating a simple game, you now possess the foundational tools to embark on your game development journey.
Encouragement for Continuous Learning: Keep experimenting, exploring new libraries, and building projects. Each step deepens your understanding of both C++ and the complexities of game design.
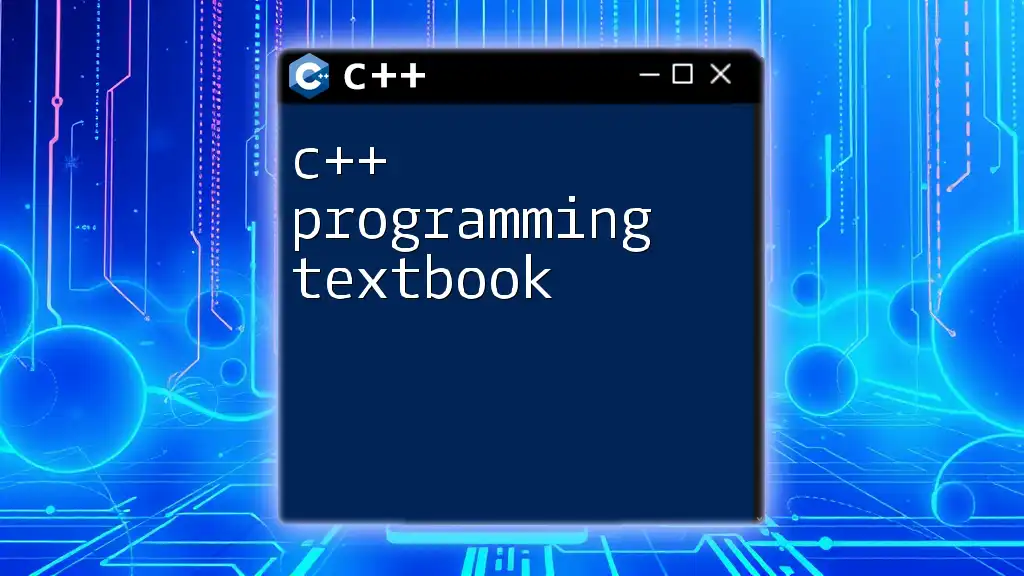
Call to Action
Now that you have a solid grounding in beginning C++ game programming, it’s time to try your ideas. Build your games, iterate on your designs, and most importantly, have fun while learning!