A C++ programming textbook is a comprehensive guide that provides learners with essential concepts, syntax, and practical examples to master the C++ programming language efficiently.
Here’s a simple code snippet demonstrating a basic C++ program that prints "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is a C++ Textbook?
A C++ programming textbook is a structured educational resource designed to guide individuals in learning the C++ programming language. These textbooks are crucial for providing a comprehensive understanding of C++, covering both foundational and advanced topics. They often include definitions, explanations, and examples that facilitate a systematic learning approach, making them an essential tool for aspiring programmers.
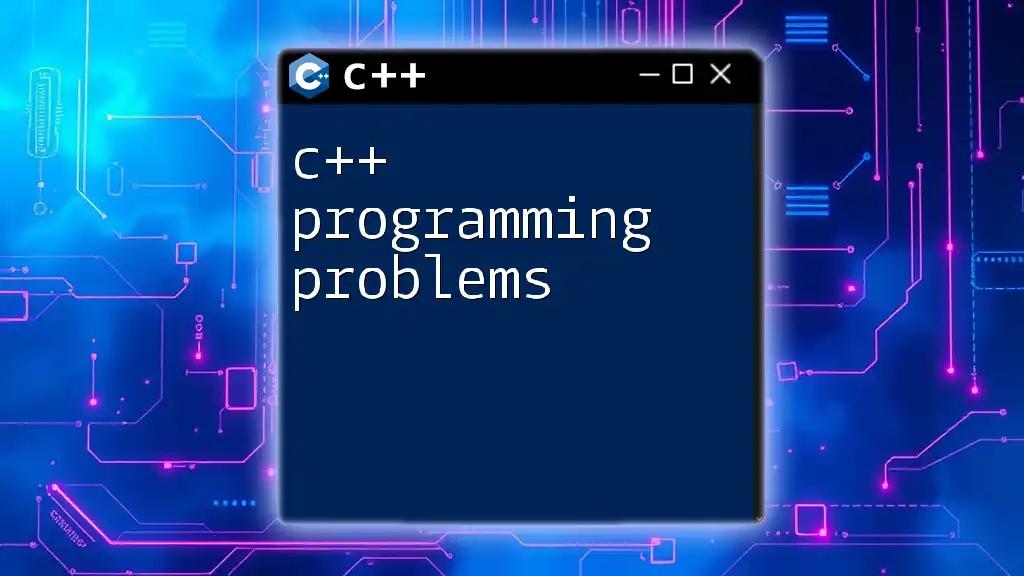
Why Choose a C++ Coding Book?
While there are myriad resources available online, choosing a C++ coding book offers unique advantages. Textbooks are curated by expert authors, ensuring that the material is not only informative but also pedagogically sound. Unlike fragmented online articles or videos, a textbook provides a cohesive narrative that builds upon previous concepts, allowing learners to progressively deepen their understanding.
The Role of Printed vs. Digital Textbooks
Both printed and digital formats have their benefits. Printed books provide an engaging tactile experience, allowing you to annotate and flip through pages without distractions. In contrast, digital textbooks are often more portable and searchable, providing instant access to glossaries and references. Ultimately, the choice between them depends on personal learning preferences and accessibility.
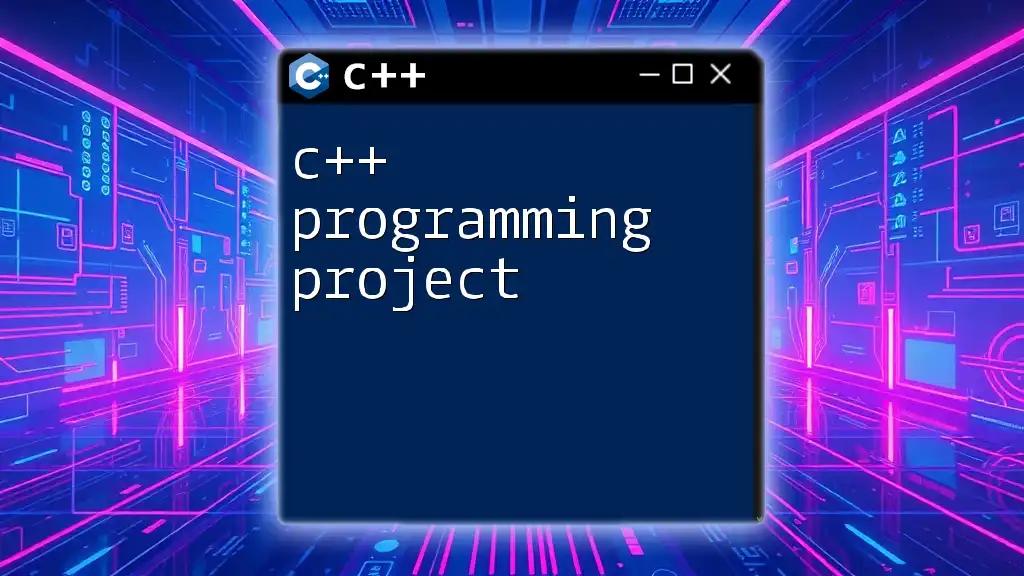
Key Features to Look for in a C++ Textbook
When selecting a C++ programming textbook, consider the following key features:
Beginner-Friendly Language
A good textbook will use clear and approachable language. It should break down complex concepts into understandable segments, making it easier for beginners to grasp the fundamentals of C++. For example, instead of diving straight into intricate syntax, it may first explain basic programming concepts.
Practical Exercises and Hands-On Projects
Practical exercises are a cornerstone of effective learning. A quality textbook will include hands-on projects and exercises that reinforce the concepts covered in the chapters. This practical application is vital for cementing your understanding.
Here's a simple C++ program that calculates the sum of two numbers:
#include <iostream>
using namespace std;
int main() {
int a, b, sum;
cout << "Enter two numbers: ";
cin >> a >> b;
sum = a + b;
cout << "Sum: " << sum << endl;
return 0;
}
In this example, the program introduces basic I/O operations and arithmetic, allowing learners to engage with C++ directly.
Comprehensive Coverage of C++ Concepts
A well-structured C++ programming textbook should cover a wide array of topics, such as:
- Variables and Data Types
- Control Structures and Loops
- Functions and Recursion
- Object-Oriented Programming (OOP) principles
These core subjects form the backbone of C++ programming and should be adequately addressed.
Illustrations and Visual Aids
Visual aids such as diagrams and flowcharts can greatly enhance understanding. They offer a different angle of approach, helping to visualize how concepts fit together. Information presented in a visually appealing manner tends to be more memorable.
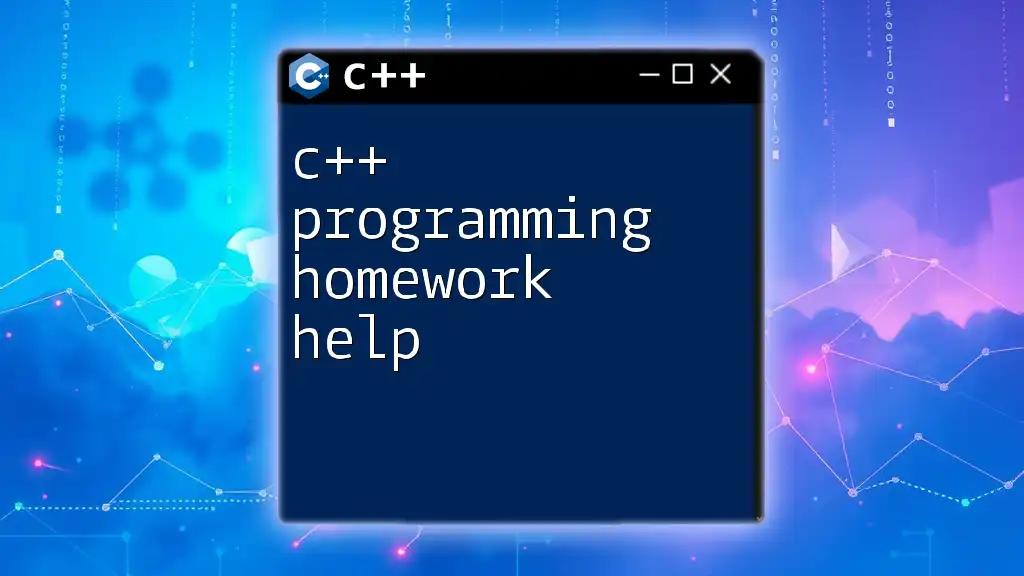
Recommended C++ Textbooks
When it comes to learning C++, a selection of textbooks stands out for both beginners and advanced learners.
Top C++ Coding Books for Beginners
One highly regarded book is "C++ Primer" by Stanley B. Lippman. This textbook is celebrated for its thorough explanations and practical examples, making it suitable for newcomers. Another seminal work, "Accelerated C++" by Andrew Koenig and Barbara E. Moo, focuses on teaching C++ features through practical applications, ensuring that learners are equipped with real-world programming skills right from the start.
Intermediate and Advanced C++ Textbooks
For those looking to deepen their expertise, consider "Effective C++" by Scott Meyers. This book contains guidelines and best practices for writing efficient and robust C++ code, making it an invaluable resource for intermediate programmers. Additionally, "The C++ Programming Language" by Bjarne Stroustrup, the creator of C++, serves as an essential reference guide. It comprehensively covers the language and its standard library, offering insights that only the language designer could provide.
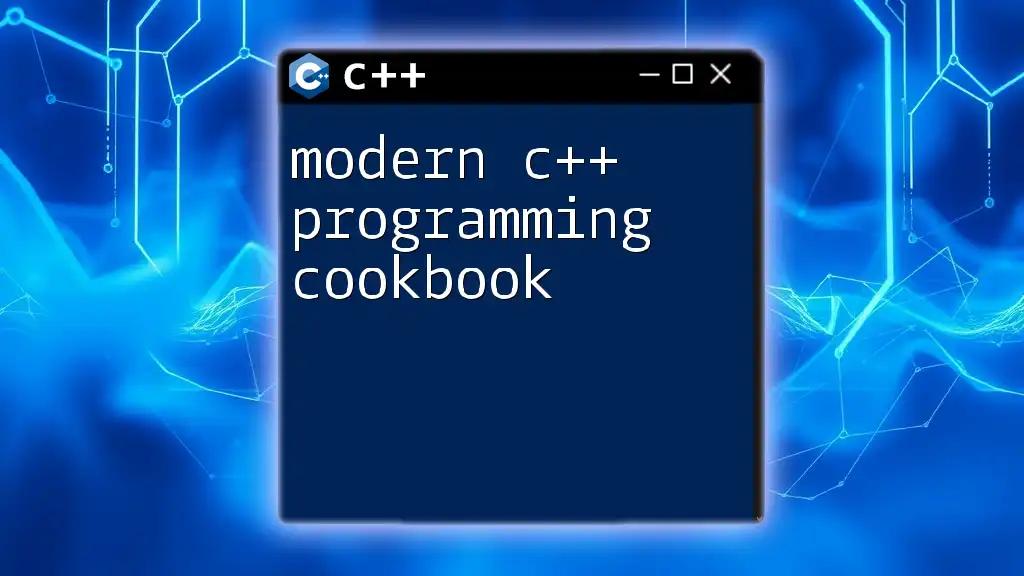
How to Effectively Use a C++ Textbook
To maximize your learning from a C++ programming textbook, it’s essential to adopt effective study strategies.
Creating a Study Schedule
Establish a study schedule that balances reading theoretical concepts and completing practical exercises. This balanced approach ensures that you don't just understand the theory but can also apply it effectively.
Note-Taking Strategies for Programming Books
Effective note-taking can drastically improve retention. Consider summarizing each chapter's key points and jotting down any code snippets that you find particularly insightful. This will create a personalized reference guide for future use.
Engaging with Exercises and Challenges
Engagement with practical challenges is fundamental to learning. Try to solve textbook problems and implement additional projects that interest you. For instance, you can design a simple class in C++ for educational purposes:
class Student {
public:
string name;
int age;
void displayInfo() {
cout << "Name: " << name << ", Age: " << age << endl;
}
};
Implementing such classes will help solidify your understanding of object-oriented programming principles in C++.
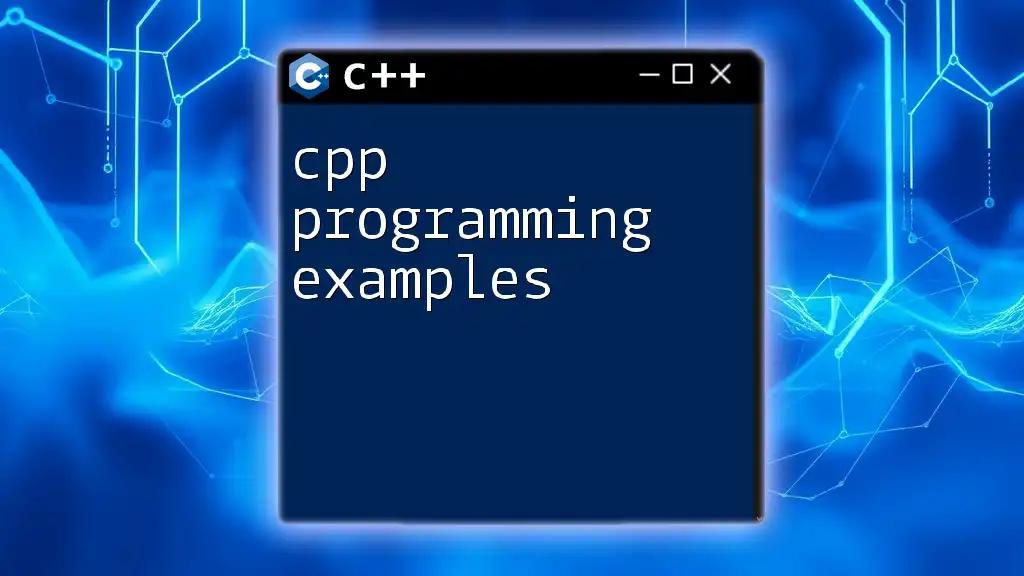
The Importance of a Good C++ Textbook in Your Learning Journey
A well-chosen C++ programming textbook serves as a foundation in your programming education. It offers structured learning and access to a wealth of knowledge that can help you navigate both the basics and complexities of C++.
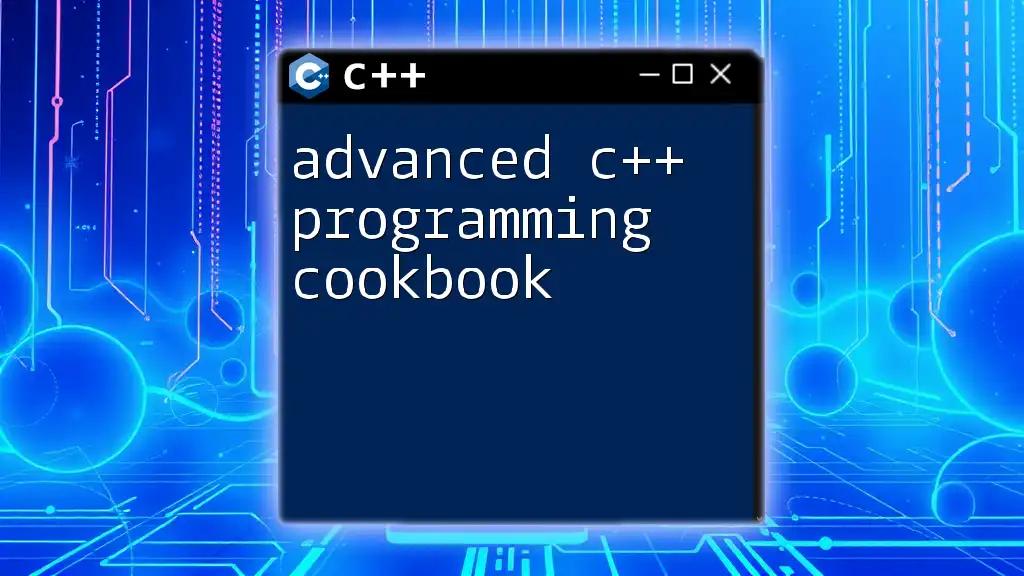
Where to Purchase C++ Textbooks
Purchasing a C++ coding book is relatively straightforward. You can explore popular online retailers such as Amazon and Barnes & Noble, which offer a wide selection of programming textbooks. Additionally, many local bookstores may carry the essential texts. Libraries and educational institutions can also provide access to a plethora of titles without requiring purchase, making learning more accessible.
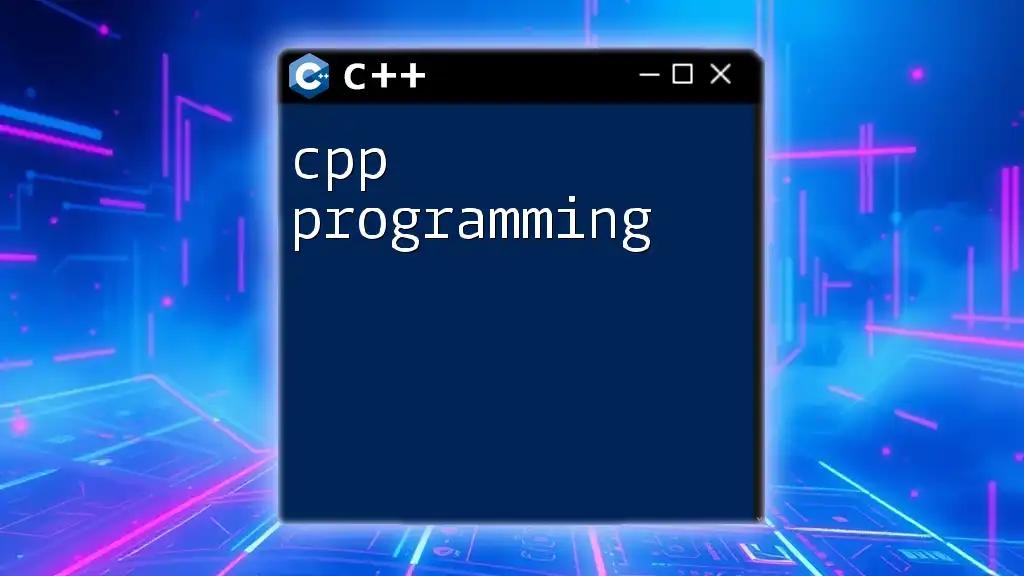
Additional Resources
It's beneficial to explore supplementary online platforms that reinforce your C++ learning. Websites such as Codecademy and LeetCode provide interactive exercises that can complement your textbook studies. Engaging with communities on platforms like Reddit or Stack Overflow allows for sharing knowledge and solving problems collectively.
Cheat sheets and reference guides are also invaluable for quick access to C++ syntax and commands, acting as a shortcut to refresh your memory on specific topics.
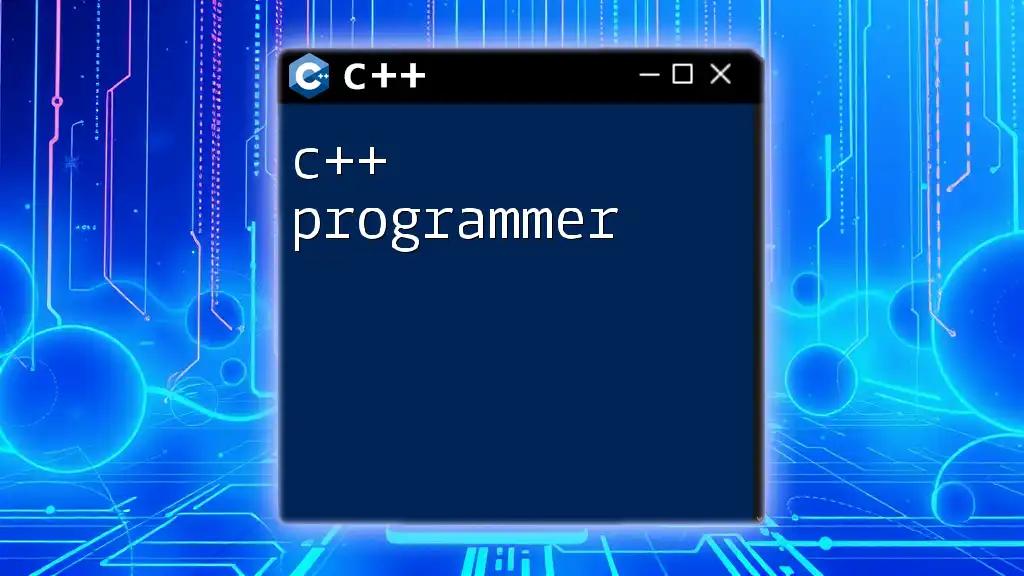
Get Started on Learning C++ Today!
Now that you have an overview of what a C++ programming textbook entails and key considerations to keep in mind, it's time to embark on your C++ learning journey. Choose a textbook that resonates with your learning style and immerse yourself in the fascinating world of programming. The skills you acquire now can open up countless opportunities in the tech industry!