C++ programming language examples showcase fundamental concepts through concise code snippets, helping learners quickly grasp key syntax and commands. Here’s an example of a simple "Hello, World!" program in C++:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding C++ Programming Language
Overview of C++
C++ is a powerful, high-performance programming language that extends the C programming language, primarily known for its object-oriented features. Developed by Bjarne Stroustrup in the early 1980s, C++ allows programmers to achieve greater flexibility and efficiency via abstraction, encapsulation, inheritance, and polymorphism.
Key features of C++ include:
- Object-Oriented Programming (OOP): C++ supports OOP principles, making it easier to manage complex programs by organizing code into reusable objects.
- Flexibility: C++ is versatile, used in various domains from game development to systems programming.
- Performance: The language provides low-level memory manipulation capabilities, resulting in high execution speeds.
When to Use C++
C++ is particularly suitable for:
- Game Development: Its performance advantages make it a natural choice for building real-time graphics and gaming engines.
- System Software: Operating systems, device drivers, and embedded systems often require C++ for efficiency.
- High-Performance Applications: Applications such as financial modeling and simulations benefit from the control C++ allows over system resources.
When comparing it to languages like Python or Java, C++ stands out in scenarios where performance and resource management are critical.
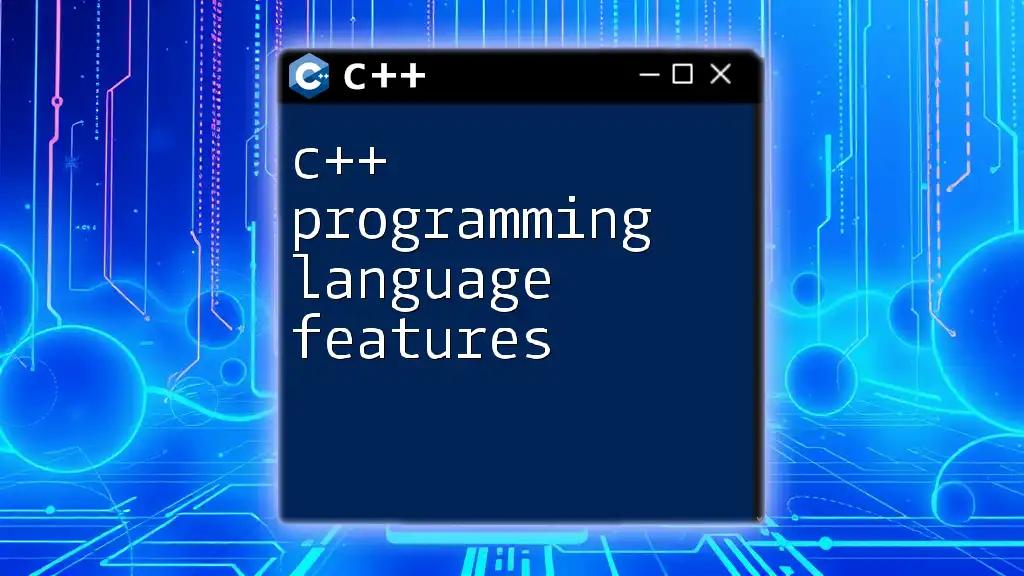
C++ Basics
Setting Up Your C++ Environment
Getting started with C++ involves setting up an integrated development environment (IDE). Recommended options include:
- Visual Studio: A robust IDE with excellent debugging tools.
- Code::Blocks: Lightweight and easy to configure.
- CLion: A powerful IDE with smart features for C++ development.
Install any of the above IDEs and configure them according to your operating system guidelines.
Writing Your First C++ Program
Every programmer begins with the classic "Hello, World!" program, showcasing the basic structure of a C++ application:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In this snippet:
- `#include <iostream>` includes the standard input/output stream library.
- `int main()` defines the main function from which execution begins.
- `std::cout` is used for output, and `std::endl` signifies the end of a line.
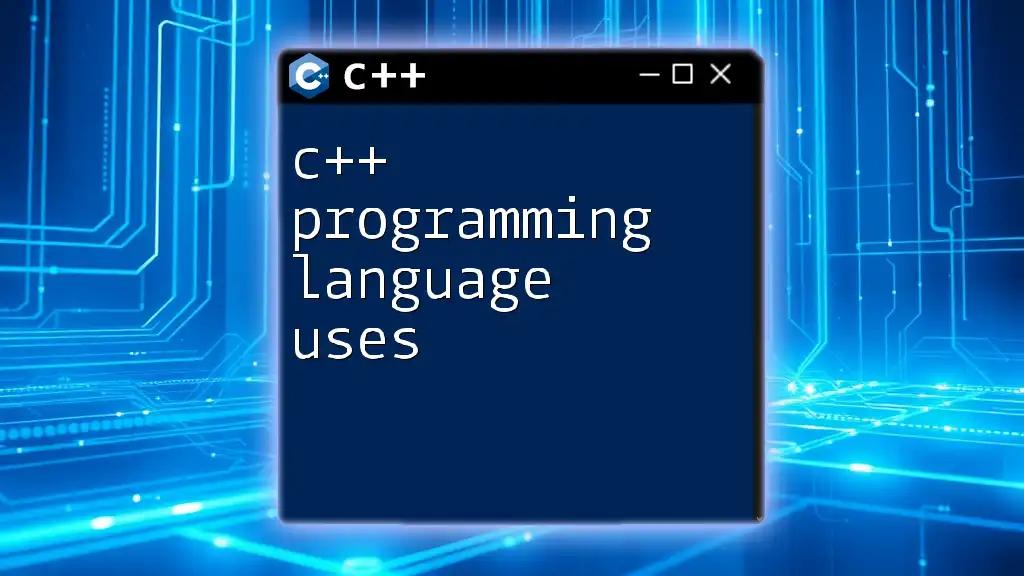
Core Concepts in C++
Variables and Data Types
Understanding Variables
A variable in C++ is a storage location identified by a variable name that holds data. They allow us to create flexible and dynamic applications.
Data Types in C++
C++ supports various data types, essential for defining the nature of data. Here are some of the most common:
- int: For integer values.
- float: For floating-point numbers.
- double: For double-precision floating-point numbers.
- char: For character data.
- string: For sequences of characters.
Here's how you can declare different types of variables:
int age = 30;
float salary = 45000.50;
char grade = 'A';
std::string name = "John Doe";
In this code, each variable is declared with its data type, demonstrating how easy it is to represent various kinds of data in C++.
Control Structures
Conditional Statements
Conditional statements control the execution flow based on specific conditions. The if-else statement is a basic example:
if (age >= 18) {
std::cout << "You are an adult." << std::endl;
} else {
std::cout << "You are a minor." << std::endl;
}
This structure evaluates whether the `age` variable qualifies for adulthood, outputting the appropriate message.
Loops
Loops allow for repetitive execution of code. Two commonly used types are for loops and while loops.
For Loops
for (int i = 0; i < 5; i++) {
std::cout << "Iteration: " << i << std::endl;
}
In this snippet, the loop prints the iteration count from 0 to 4.
While Loops
int countdown = 5;
while (countdown > 0) {
std::cout << countdown << std::endl;
countdown--;
}
This example demonstrates how the while loop continues executing until the countdown variable reaches 0.
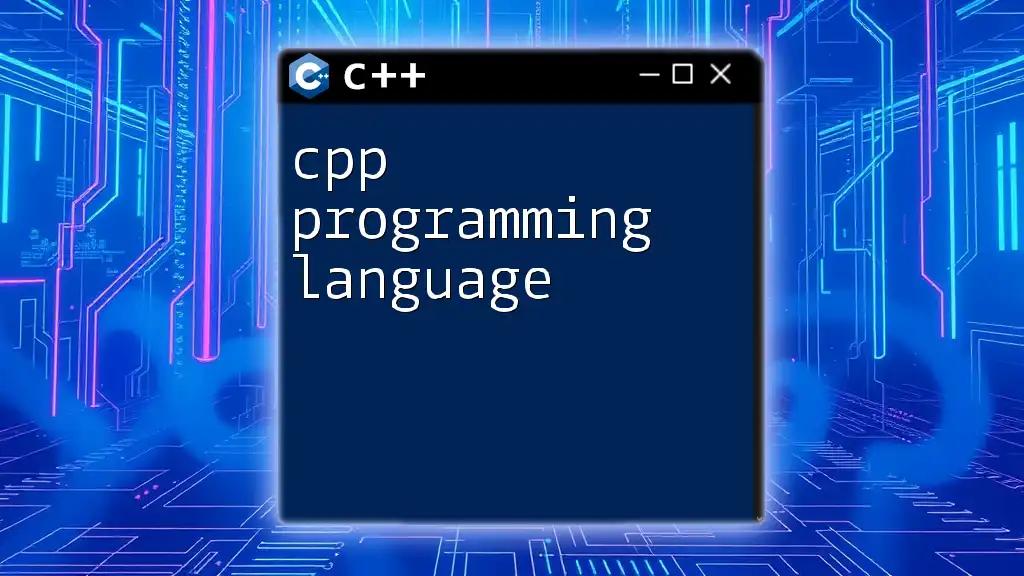
Functions in C++
Creating and Using Functions
Functions are essential for modular programming, allowing code reuse and organization. Here’s how to create a simple function:
int add(int a, int b) {
return a + b;
}
This function receives two integers, adds them, and returns the result. Functions help enhance code readability and maintainability.
Function Overloading
C++ supports function overloading, allowing multiple functions with the same name but different parameters. Here’s an illustration:
int multiply(int a, int b) {
return a * b;
}
double multiply(double a, double b) {
return a * b;
}
In this example, we define two `multiply` functions, one for integers and another for double values, showcasing the ability to handle different data types seamlessly.
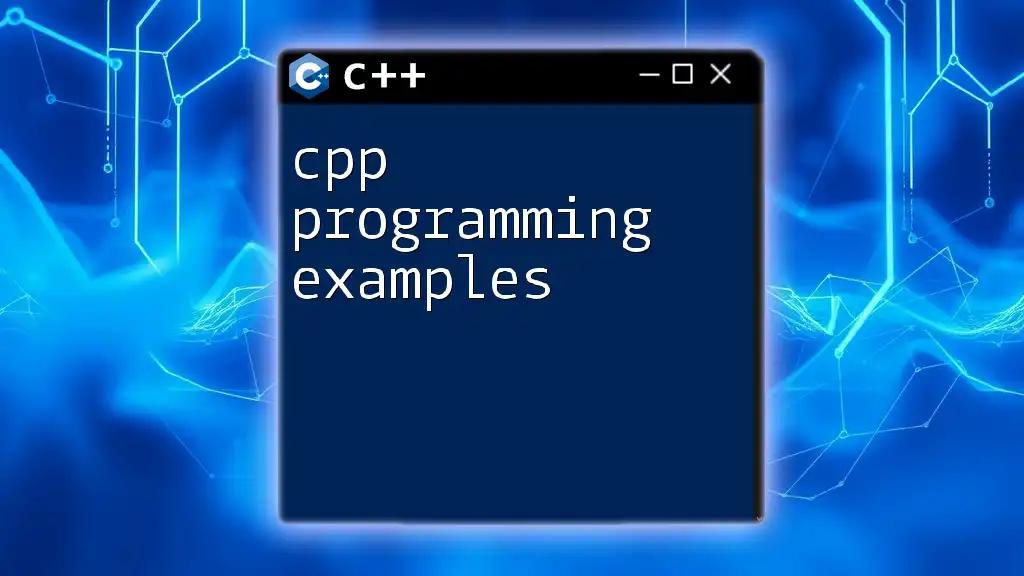
Object-Oriented Programming in C++
Classes and Objects
C++ supports classes, which are blueprints for creating objects. Here's a sample class definition:
class Car {
public:
std::string model;
int year;
void display() {
std::cout << "Model: " << model << ", Year: " << year << std::endl;
}
};
In this snippet:
- `Car` is a class with attributes and methods.
- The `display` function outputs the car’s details when called.
Inheritance
Inheritance helps in reusing code by creating a new class based on an existing class. Here’s an example:
class Vehicle {
public:
void start() {
std::cout << "Vehicle started." << std::endl;
}
};
class Bike : public Vehicle {
public:
void ringBell() {
std::cout << "Bike bell rings!" << std::endl;
}
};
Here, `Bike` inherits from `Vehicle`, allowing it to use the `start` method while also introducing its functionality.
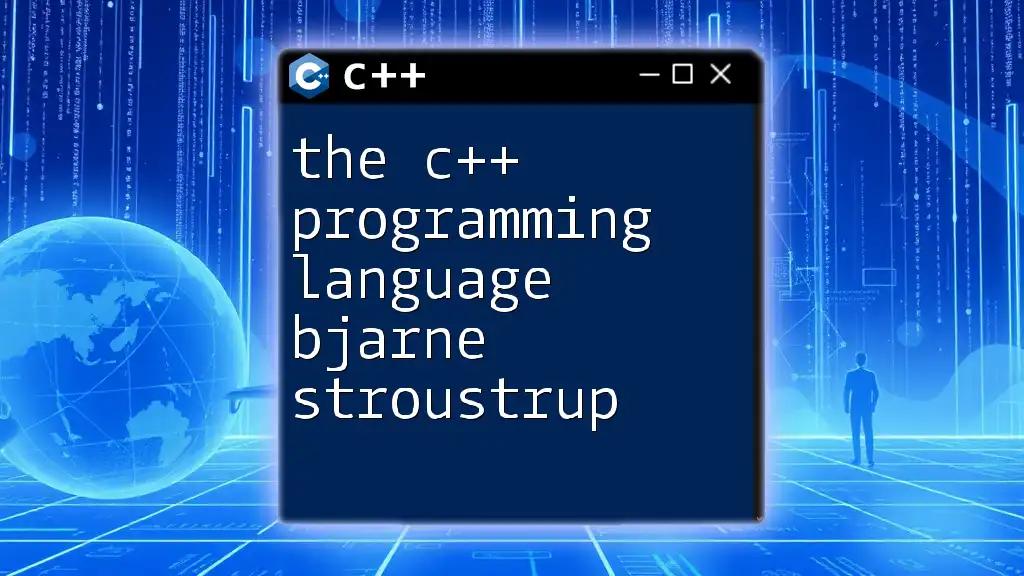
Advanced C++ Concepts
Templates
Templates allow functions and classes to operate with generic types, facilitating code reuse. Here’s a template function example:
template <typename T>
T getMax(T a, T b) {
return (a > b) ? a : b;
}
This template can be used to find the maximum of various data types, promoting flexibility in coding.
Exception Handling
C++ provides a robust mechanism for exception handling, enabling the graceful handling of errors. Here’s a basic example:
try {
throw std::runtime_error("An error occurred");
} catch (const std::exception& e) {
std::cout << "Caught an exception: " << e.what() << std::endl;
}
In this code, any runtime errors can be caught and processed without crashing the program, enhancing reliability.
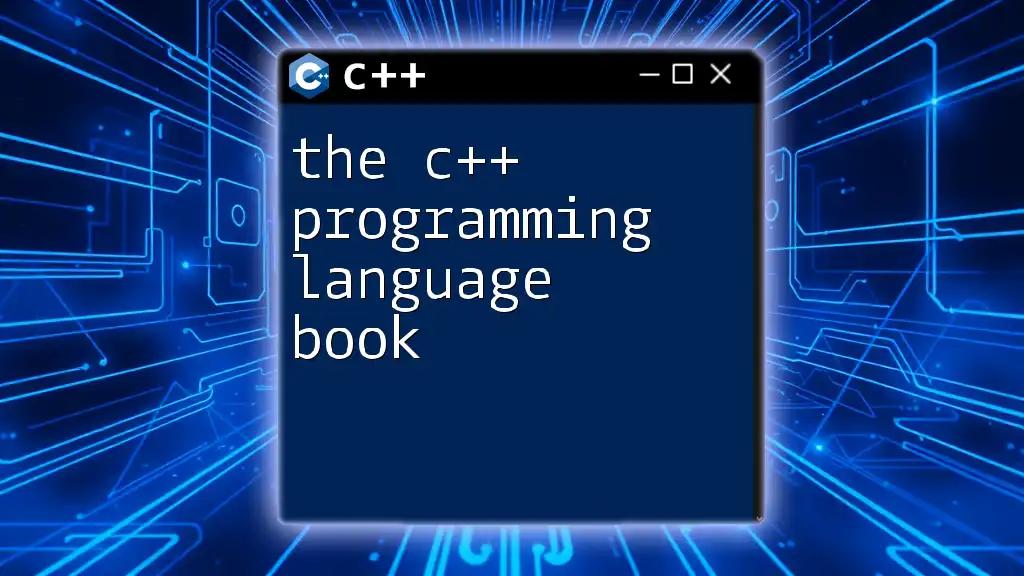
C++ Standard Library
Introduction to STL
The Standard Template Library (STL) is a powerful library in C++ that provides a rich set of functions and data structures. It simplifies complex programming tasks and enhances code efficiency.
Common Data Structures in STL
Vectors
Vectors are dynamic arrays that can resize themselves. Here's an example:
#include <vector>
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (int num : numbers) {
std::cout << num << std::endl;
}
This snippet illustrates how to create a vector and iterate through its elements. Vectors are preferable for scenarios where the size of the collection may change during runtime.
Maps
Maps provide an associative array data structure, where each key is associated with a value:
#include <map>
std::map<std::string, int> ageMap;
ageMap["Alice"] = 30;
ageMap["Bob"] = 25;
In this example, a map is utilized to store names with their corresponding ages, showcasing how to access data efficiently.
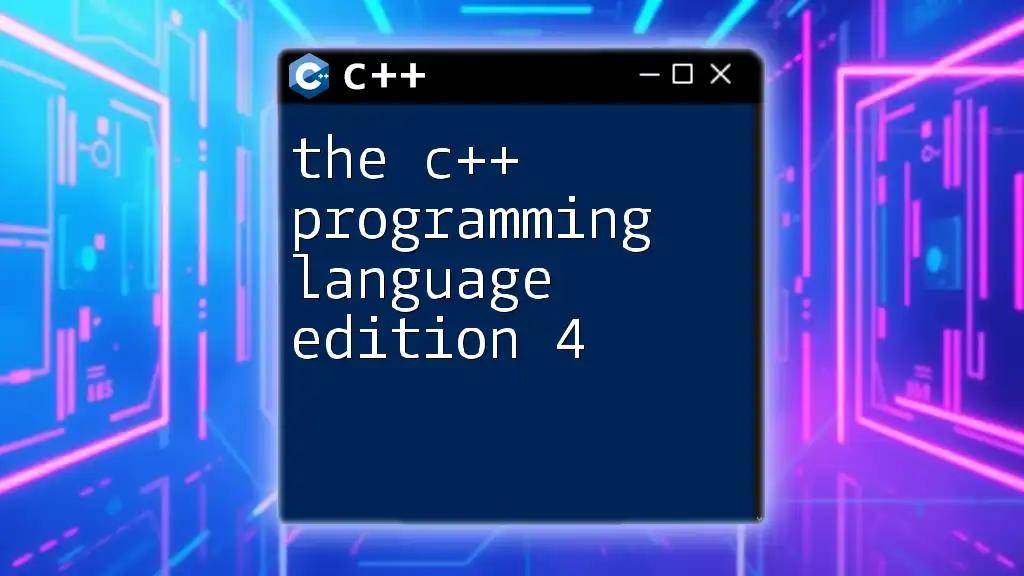
Conclusion
This guide highlights a range of C++ programming language examples that illustrate foundational and advanced concepts. From basic syntax to complex OOP principles, mastering these concepts empowers you to write efficient C++ code tailored to your programming needs.
As you continue your learning journey, remember that practice is crucial. Engage with real projects and coding tasks, and don't hesitate to explore deeper into the vast C++ ecosystem. Happy coding!
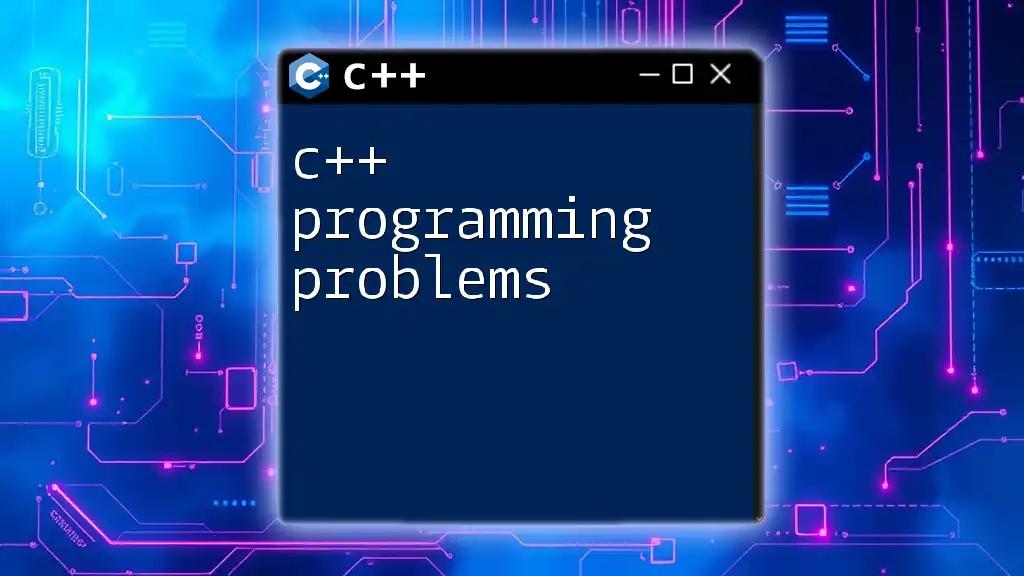
Resources for Further Learning
To further enhance your understanding of C++, consider seeking out a variety of resources, including:
- Books: "The C++ Programming Language" by Bjarne Stroustrup is a classic.
- Online Courses: Platforms like Coursera and Udacity offer comprehensive C++ courses.
- Communities and Forums: Engage with C++ communities on Stack Overflow, GitHub, and Reddit to exchange ideas and solutions.
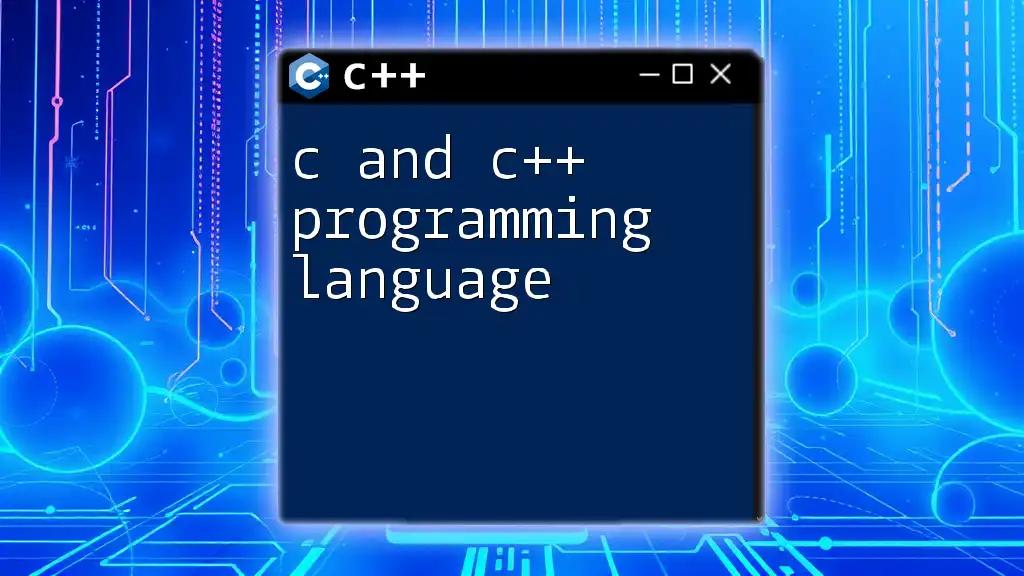
Call to Action
If you’re keen on mastering C++, consider enrolling in our expertly guided courses. Join our community and start your journey today!