A subroutine in C++ is a reusable block of code that performs a specific task, allowing for better organization and efficiency in programming. Here's an example of a simple subroutine that calculates the square of a number:
#include <iostream>
using namespace std;
int square(int x) {
return x * x;
}
int main() {
int num = 5;
cout << "The square of " << num << " is " << square(num) << endl;
return 0;
}
Understanding C++ Subroutines
What is a Subroutine?
A subroutine is a set of instructions designed to perform a frequently used operation. In programming, it allows you to break down complex processes into smaller, manageable pieces of code. This enhances the readability and maintainability of your code. In C++, subroutines are typically implemented as functions.
Types of Subroutines in C++
Subroutines can generally be categorized into:
- Functions: Standalone blocks of code that may accept inputs (parameters) and return a value.
- Methods: Functions that are associated with objects in object-oriented programming.
- Inline Functions: This type of function suggests to the compiler to expand the function inline at the call site rather than calling it normally, which can reduce function-call overhead.
- Recursive Functions: Functions that call themselves in order to solve a problem.
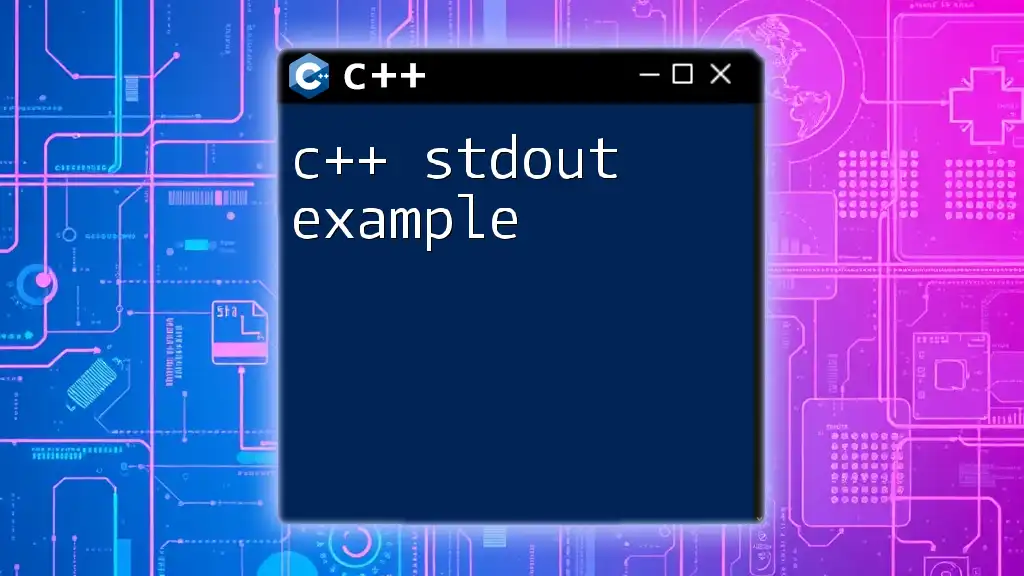
How to Create a Function in C++
Basic Syntax of a C++ Function
Creating a function in C++ involves understanding its basic syntax. Each function typically consists of four important components:
- Return Type: The type of value that the function returns.
- Function Name: Identifier for the function.
- Parameter List: Value(s) passed into the function, defined within parentheses.
- Function Body: The set of instructions the function executes, enclosed in braces.
The general structure can be represented as follows:
return_type function_name(parameter_list) {
// function body
}
Example of a Simple Function
Let’s look at a basic example of a function that adds two integers:
int add(int a, int b) {
return a + b;
}
In this example, `add` is the function name, `int` is the return type, and it accepts two integer parameters.
Calling a Function
Once you have defined a function, you can invoke it anywhere in your code. For example, to call the `add` function, you would do the following:
int result = add(5, 10);
Here, `result` will store the sum of 5 and 10 calculated by the `add` function.
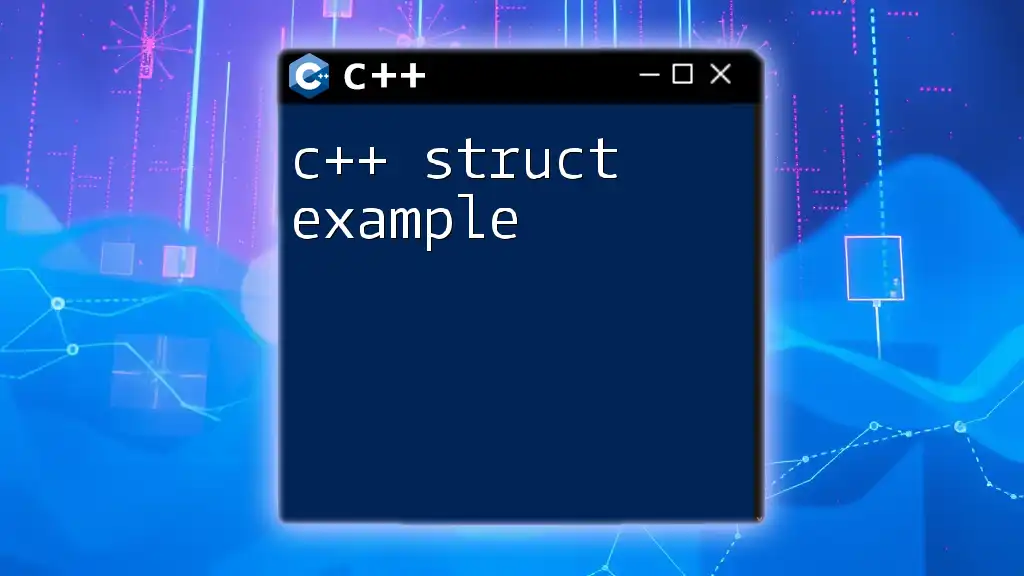
Function Parameters and Return Types
Understanding Function Parameters
Parameters play a critical role in functions as they allow you to pass values into them. There are two main types of parameters:
- Value-based parameters: These are passed by value, meaning the function receives a copy of the actual values.
- Reference-based parameters: Also known as pass-by-reference, the function receives a reference to the actual values, allowing it to modify them.
Here’s an illustrative example of a function that prints the sum of two integers:
void printSum(int a, int b) {
std::cout << "Sum: " << (a + b) << std::endl;
}
Return Types Explained
Every function must specify a return type. This defines what type of data the function will return. For example, a function returning a double can be created as follows:
double computeArea(double radius) {
return 3.14 * radius * radius;
}
When this function is called with a radius, it calculates and returns the area of a circle.
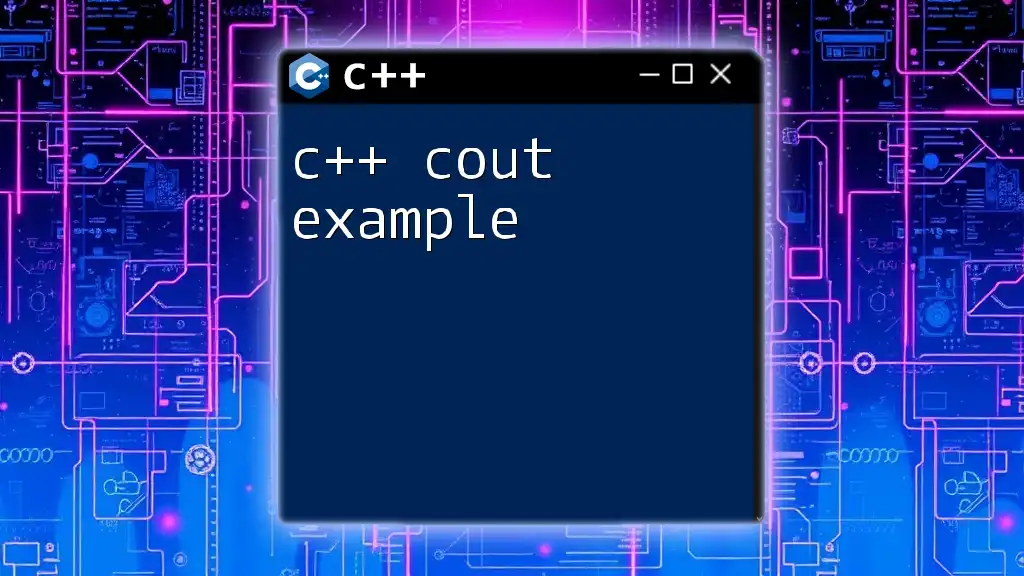
Creating Functions with Multiple Parameters
Example of Function with Multiple Parameters
A function can accept multiple parameters, making it versatile. Here’s an example of a function that displays the information of a person:
void displayInfo(std::string name, int age, double height) {
std::cout << "Name: " << name << ", Age: " << age << ", Height: " << height << " meters" << std::endl;
}
In this case, `displayInfo` takes three parameters and prints a formatted string with the provided details.
How to Call Functions with Multiple Parameters
When invoking this function, you need to pass all required arguments:
displayInfo("Alice", 30, 1.65);
This will output: `Name: Alice, Age: 30, Height: 1.65 meters`.
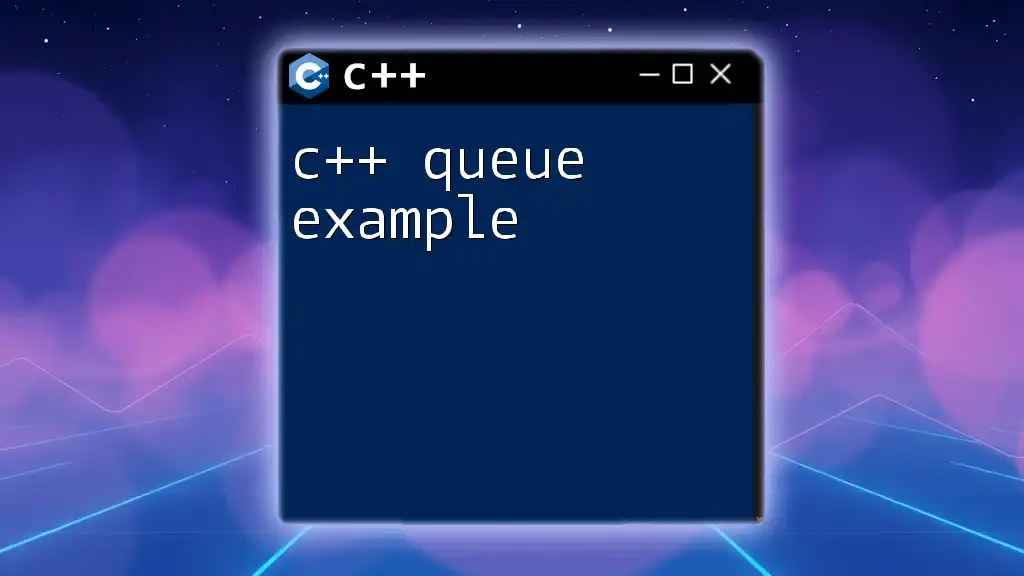
Function Overloading in C++
What is Function Overloading?
Function overloading allows you to define multiple functions with the same name but different parameter types or counts. This is beneficial as it improves code readability and organization by allowing similar functions to be grouped together.
Example of Function Overloading
Consider the following example that demonstrates function overloading for a multiplication operation:
int multiply(int a, int b) {
return a * b;
}
double multiply(double a, double b) {
return a * b;
}
Both `multiply` functions perform multiplication, but one handles integers, while the other handles doubles.
When to Use Function Overloading
Function overloading is particularly useful when you have multiple ways to perform a similar operation, thus avoiding the need to come up with entirely different names for each variation.
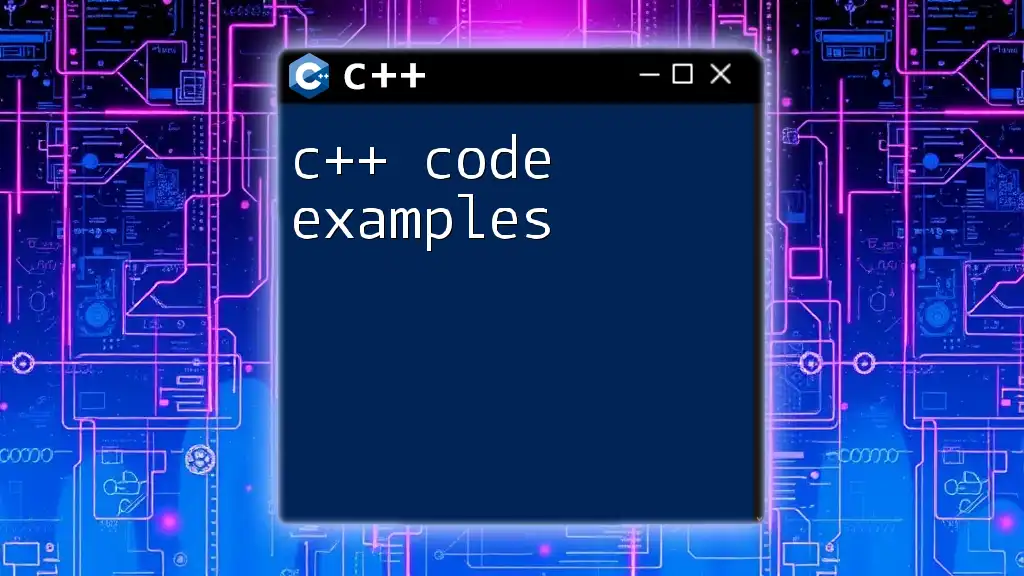
Recursive Functions
What is Recursion?
Recursion refers to the technique where a function calls itself in order to solve a problem. This approach is often used to solve problems that can be broken down into smaller, similar problems.
Creating a Recursive Function
A classic example of recursion is calculating the factorial of a number:
int factorial(int n) {
return (n == 1) ? 1 : n * factorial(n - 1);
}
This function checks if `n` is 1 (the base case), and if not, it calls itself with `n - 1`.
Advantages and Disadvantages of Recursion
While recursion provides elegant solutions for complex problems, it comes with its own drawbacks, such as increased memory usage due to the call stack and potential for stack overflow in deep recursions.
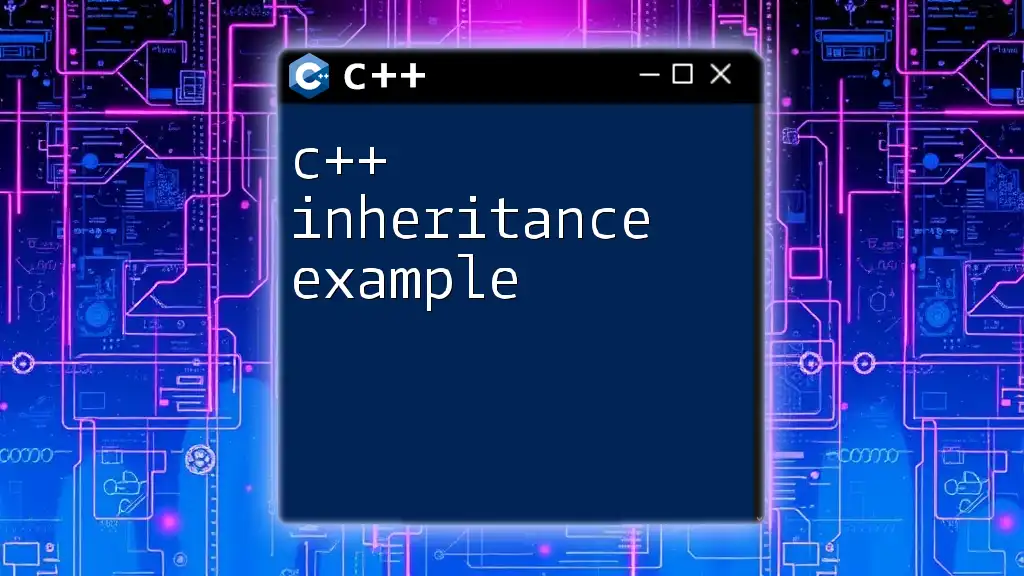
Best Practices for Writing Functions in C++
Keeping Functions Concise and Focused
To enhance your code's maintainability, aim to keep functions concise and focused. Each function should ideally perform one specific task. This not only improves code readability but also simplifies debugging and testing.
Utilizing Comments Effectively
Comments in functions add clarity and context. Use them to explain the purpose of complex code segments or to outline the function’s behavior.
Testing and Debugging Functions
Testing is crucial for affirming that your functions behave as expected. Implement unit tests to automate testing each function, ensuring that any changes to the codebase do not introduce new bugs.
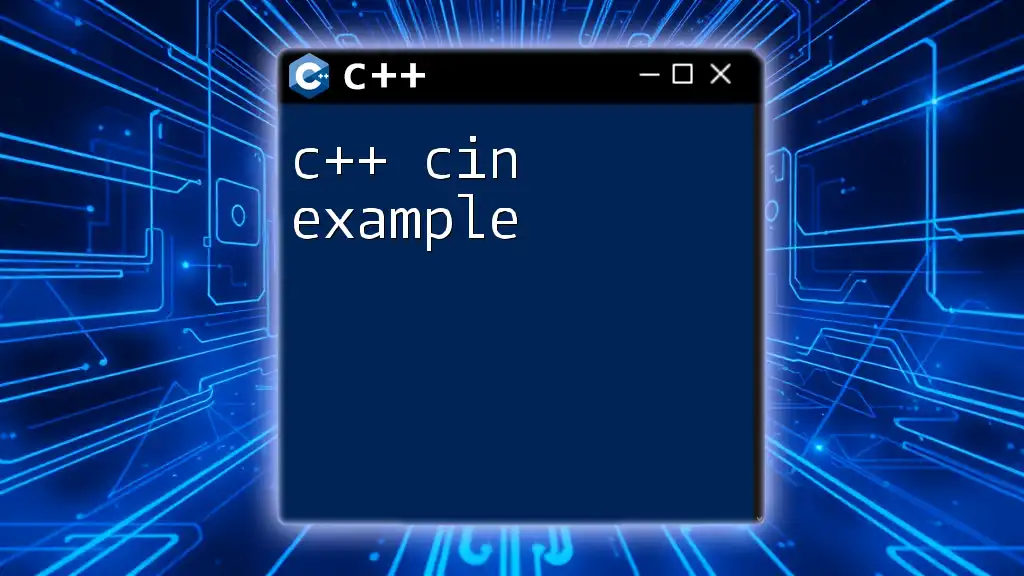
Conclusion
In summary, C++ subroutines, represented as functions, are powerful tools in programming that help reduce complexity, enhance readability, and facilitate code reusability. By mastering the art of creating and using functions, you'll significantly elevate your coding skills. Practicing by implementing your own functions will provide further insights and strengthen your programming foundation.