In C++, the `cout` command is used to output data to the standard console, and here's a simple example demonstrating its usage:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is C++?
C++ is a powerful programming language that builds on the foundation of C, introducing object-oriented features. Developed by Bjarne Stroustrup in the late 1970s, it has evolved significantly over the years, making it one of the most widely used languages for application and software development today.
Some key features of C++ include:
- Object-Oriented Programming (OOP): Supports encapsulation, inheritance, and polymorphism.
- Performance: Provides low-level memory manipulation features, making it suitable for performance-critical applications.
- Standard Template Library (STL): Offers a collection of pre-built templates for common data structures and algorithms.
C++ plays a crucial role in various domains, including game development, system programming, and high-performance applications.
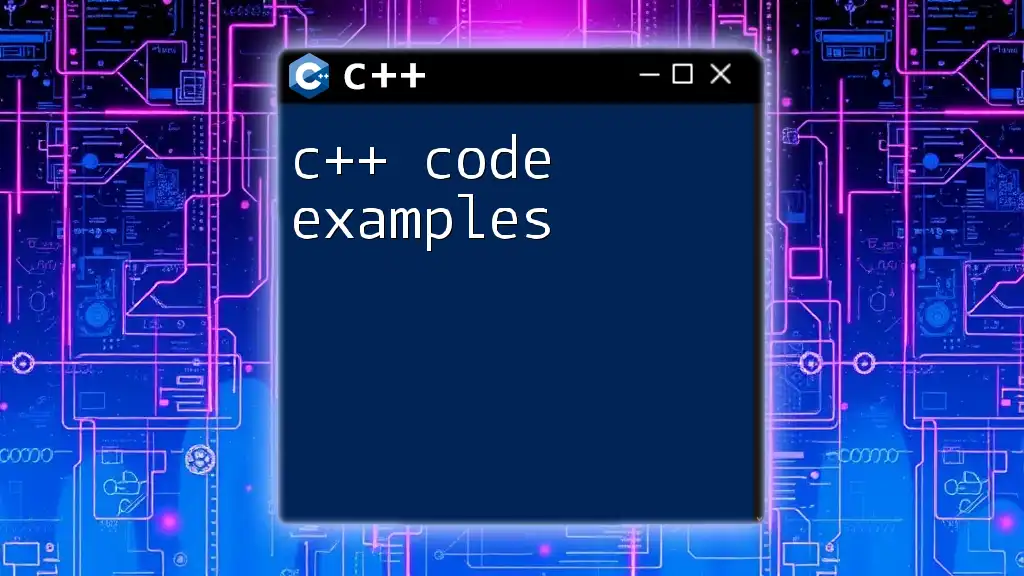
Understanding Output in C++
Output mechanisms are essential in programming, primarily for displaying information and debugging purposes. Console output in C++ allows developers to view the results of their code execution, making `cout` a vital element for any C++ programmer.
What is std::cout?
The `std::cout` object is part of the C++ standard library and is used to perform output operations. It is defined in the `<iostream>` header file and belongs to the `std` namespace, which means that every time you use `cout`, you typically prefix it with `std::`.
Namespace std
Namespaces are a way to group identifiers and avoid naming conflicts. The `std` namespace encompasses all components of the C++ Standard Library, allowing you to use its features without worrying about clashes with custom-defined functions or variables.
Overview of std::cout
`std::cout` stands for "standard character output." It utilizes the `<<` operator, known as the stream insertion operator, to send data to the console. This operator allows you to chain multiple outputs together in a single statement.
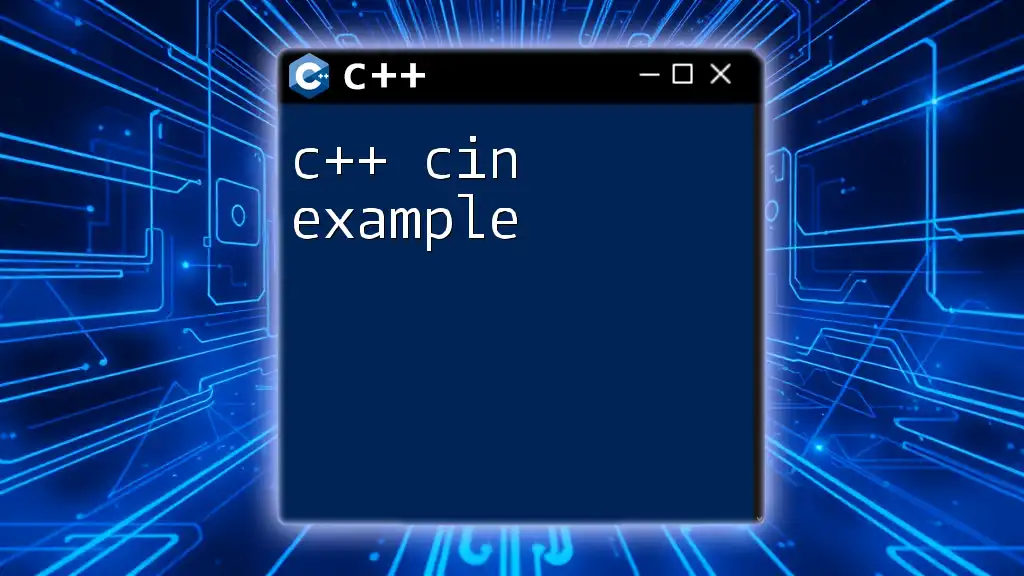
Basic Usage of std::cout
Using `std::cout` is straightforward, but understanding its syntax is essential for effective outputting of messages and data types.
Syntax of std::cout
The basic syntax for using `cout` is as follows:
std::cout << [data to output] << std::endl;
Where `[data to output]` can be various data types, including integers, floating-point numbers, characters, strings, and so on.
Example: Simple Output
Here’s a simple example that demonstrates how to use `std::cout` to display a message.
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In this code, `std::cout` sends the string "Hello, World!" to the console, followed by `std::endl`, which flushes the output buffer and moves the cursor to the next line. The output will be:
Hello, World!
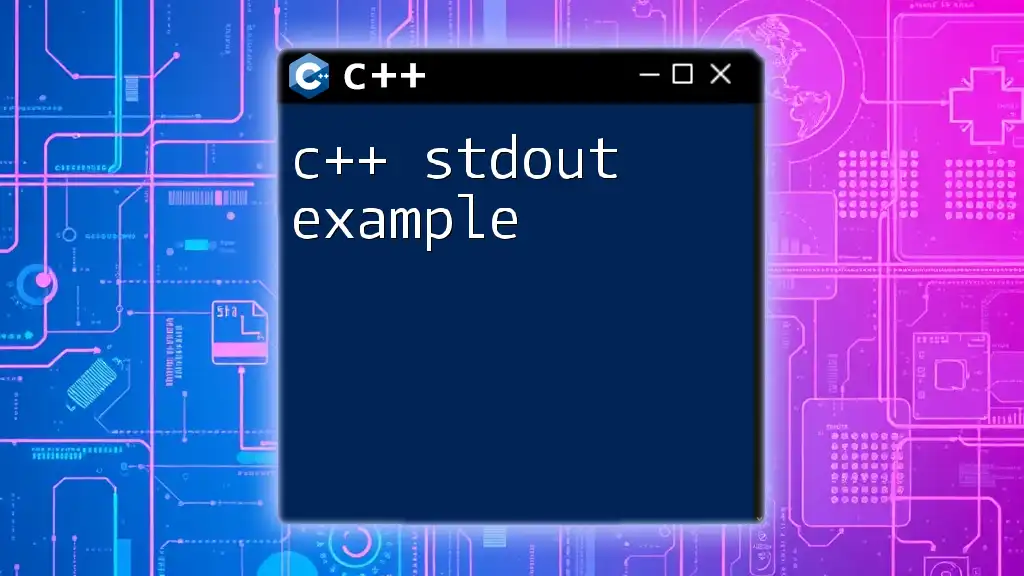
Working with Different Data Types
`std::cout` can handle a variety of data types, making it versatile. Let's explore how to use `cout` for different data types in C++.
Outputting Integers
You can seamlessly output integers using `cout`. For instance:
int age = 30;
std::cout << "I am " << age << " years old." << std::endl;
In this example, the variable `age` is inserted into the output stream, resulting in:
I am 30 years old.
Outputting Floating-point Numbers
When dealing with decimals, `std::cout` processes floating-point numbers with equal ease:
double pi = 3.14;
std::cout << "Value of Pi: " << pi << std::endl;
This outputs:
Value of Pi: 3.14
Outputting Characters and Strings
You can also output single characters and strings using `std::cout`:
char initial = 'J';
std::cout << "My initial is: " << initial << std::endl;
std::string name = "John";
std::cout << "My name is: " << name << std::endl;
The output will be:
My initial is: J
My name is: John
In this case, notice that we included the `<string>` header to use the `string` data type.
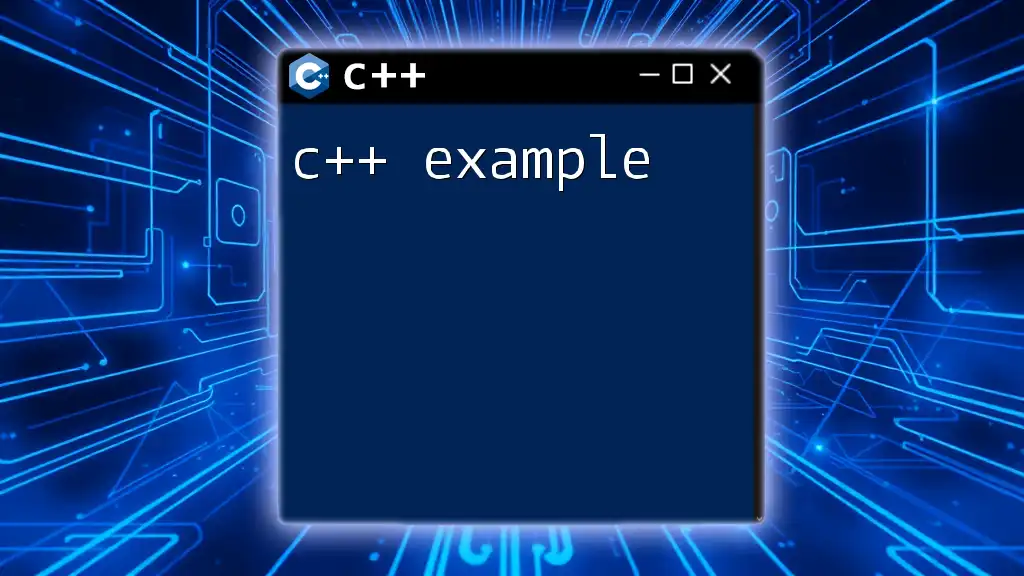
Chaining and Formatting Output
One of the strengths of `cout` is its ability to chain multiple outputs together and format them according to your needs.
Chaining cout Statements
Chaining allows you to concatenate multiple outputs in a single line:
std::cout << "The number is " << age << " and Pi is approximately " << pi << "." << std::endl;
This results in:
The number is 30 and Pi is approximately 3.14.
Formatting with std::endl vs. "\n"
While both `std::endl` and `"\n"` can create new lines, they function differently. Using `std::endl` not only adds a new line but also flushes the output buffer, which can be crucial in scenarios where immediate output is required. On the other hand, using `"\n"` simply adds a new line without flushing.
Manipulating Output Formats
For more tailored output, especially with floating-point numbers, you can use formatting manipulators like `std::fixed` and `std::setprecision` from the `<iomanip>` header:
#include <iostream>
#include <iomanip>
double price = 9.99;
std::cout << std::fixed << std::setprecision(2) << "Price: $" << price << std::endl;
This code ensures that the output is displayed with two decimal places:
Price: $9.99
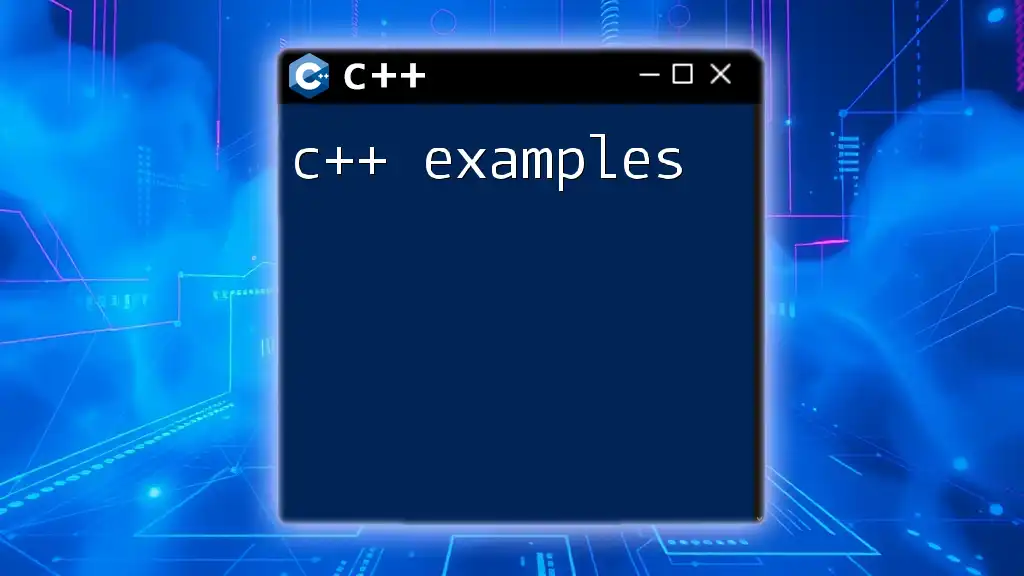
Common Errors with std::cout
While using `std::cout` is relatively straightforward, beginners often encounter some common pitfalls. Understanding these can save you time and frustration.
Missing Headers
One of the most frequent errors is forgetting to include the `<iostream>` header file. Without this, the compiler won't recognize `std::cout`, leading to compilation errors.
Wrong Operator Usage
Another common mistake is misusing the `<<` operator. It is vital to ensure that you are appending and not trying to overwrite `cout` with an assignment operator (`=`).
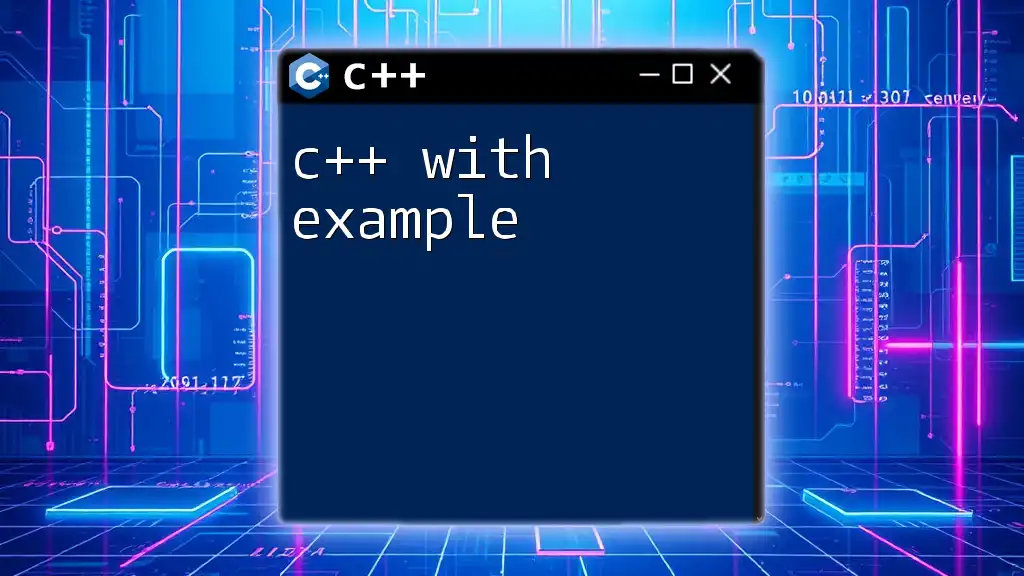
Conclusion
In summary, `std::cout` is a fundamental part of C++ programming that enables you to display outputs efficiently. By mastering its use, you can significantly enhance your debugging and development capabilities. Practice creating your own c++ cout examples to fully grasp the potential of this powerful output stream.
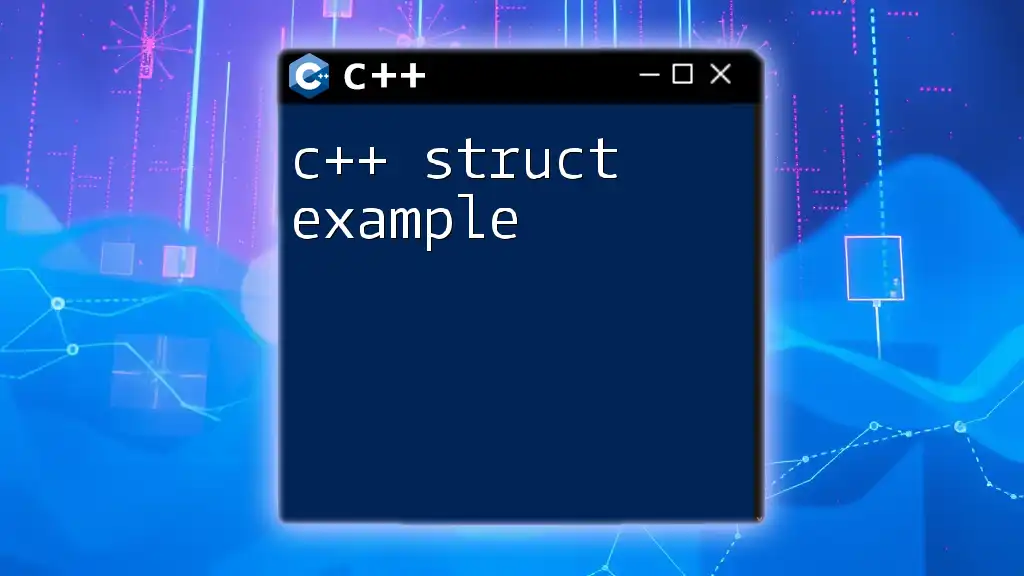
FAQs about C++ cout
What is the difference between std::cout and printf?
- std::cout is type-safe and integrates smoothly with C++ objects, especially user-defined types. printf, on the other hand, is a C-style function that requires format specifiers.
- You can leverage C++ objects and manipulators with std::cout, making it more flexible for various data types.
Can I use printf in C++?
Yes, you can use `printf` in C++, but keep in mind that it is a part of the C standard library. Use it for C-style strings and legacy code, but prefer `std::cout` for modern C++ development.
How do I handle special characters in output?
Special characters can be incorporated into your output using escape sequences. For example, to include a tab or a new line, you can use:
std::cout << "Hello,\nWorld!" << std::endl; // Outputs 'Hello,'
// on one line, 'World!' on the next.
Understanding how to effectively utilize `std::cout` is essential as you progress in your C++ programming journey. So, get familiar with its capabilities and enrich your coding practice!