The `setw` manipulator in C++ is used to set the width of the next output field, ensuring that the output is formatted to a specified width for better readability.
Here's an example code snippet demonstrating its use:
#include <iostream>
#include <iomanip> // Include for setw
int main() {
std::cout << std::setw(10) << "Hello" << std::setw(10) << "World" << std::endl;
return 0;
}
Introduction to C++ setw
What is `setw`?
`setw` is a manipulator in C++ used to set the width of the output field for the next value to be output. This is crucial for organizing console output, especially when dealing with tabular data. Without proper formatting, output can appear disorganized, making it difficult for users to read.
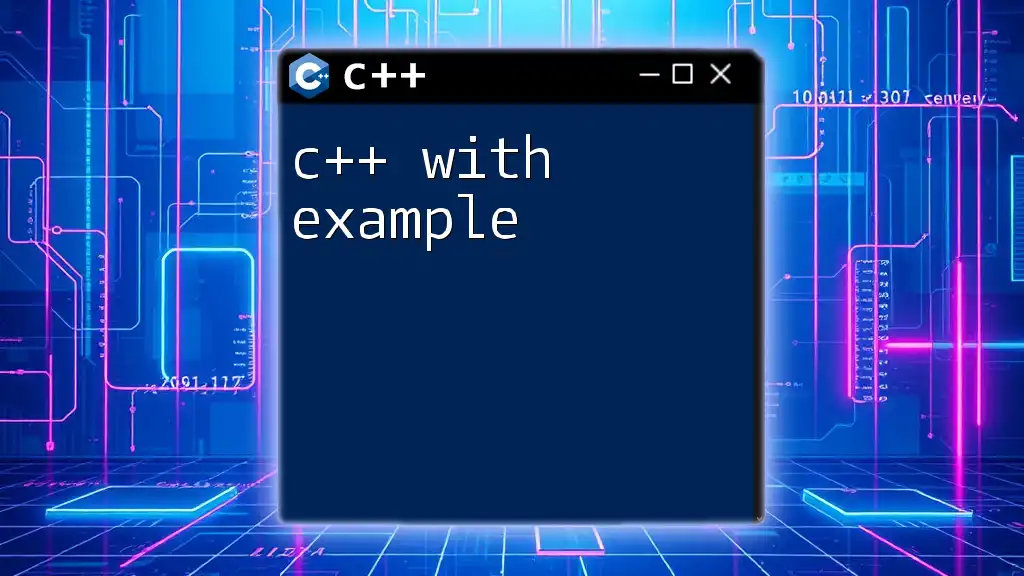
Understanding Input/Output in C++
Basics of Input/Output Streams
In C++, the `iostream` library provides functionalities for input and output operations. The most commonly used objects are `cin` for input and `cout` for output.
Need for Formatting Output
Formatting becomes imperative when displaying data in a user-friendly manner, particularly when values do not align properly. A well-structured output enhances readability, allowing users to consume information efficiently.
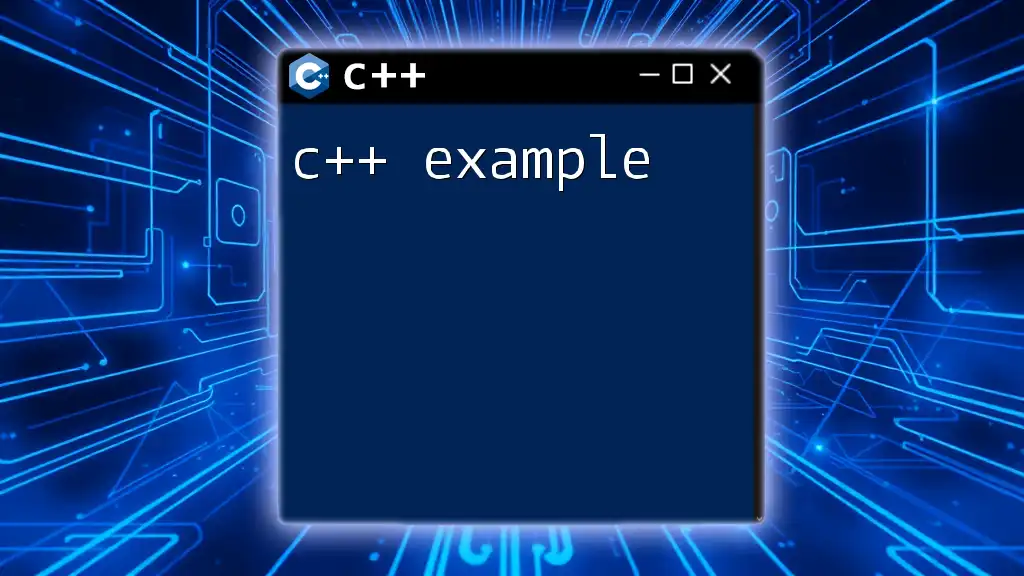
The `setw` Manipulator
Definition and Purpose
The `setw` manipulator is defined in the `iomanip` header file and allows you to specify the width of the output field. It is essential for creating neatly organized outputs, especially when displaying data in columns. By using `setw`, you ensure that all elements align properly, regardless of their data type.
Header Files Required
To use `setw`, include the following header file in your C++ program:
#include <iomanip>
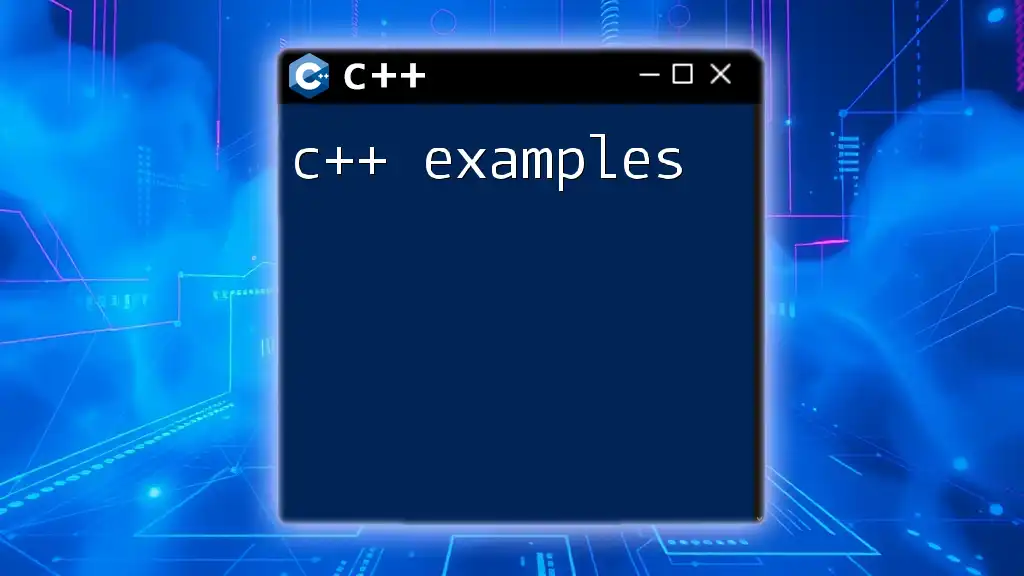
Usage of `setw` in C++
Basic Syntax
To use `setw`, simply call it before the value you wish to output. The syntax looks like this:
std::cout << std::setw(width) << value;
This indicates that the output will occupy a minimum width specified by `width`.
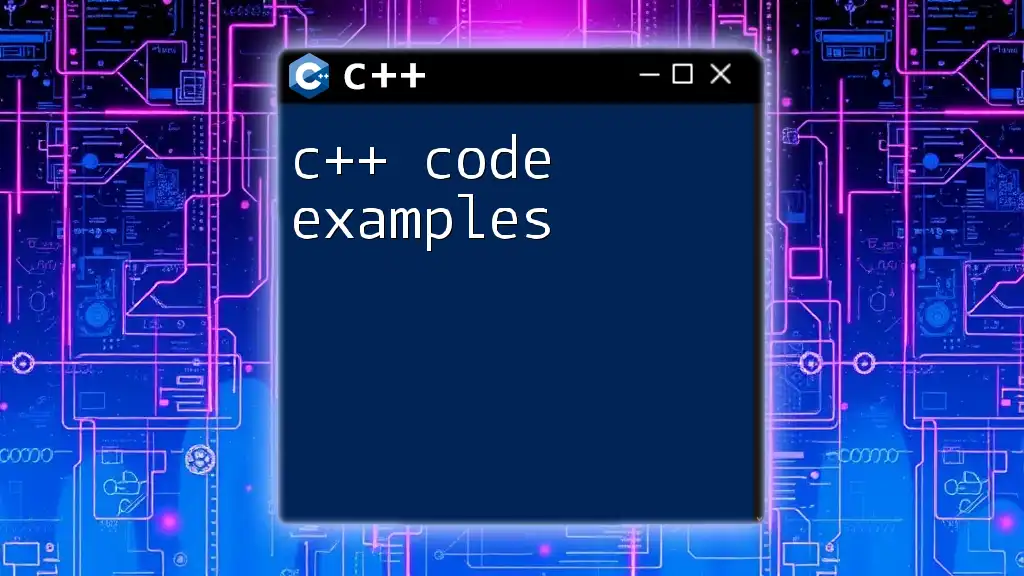
Examples of `setw` in Action
Simple Console Output Example
One of the simplest uses of `setw` can be observed in displaying a table of items and prices:
#include <iostream>
#include <iomanip>
int main() {
std::cout << std::setw(10) << "Item" << std::setw(10) << "Price" << std::endl;
std::cout << std::setw(10) << "Apple" << std::setw(10) << 1.50 << std::endl;
std::cout << std::setw(10) << "Banana" << std::setw(10) << 0.75 << std::endl;
return 0;
}
Explanation of Output:
In the above example, the output will be aligned into two columns— the first for the item names and the second for their prices. The width of 10 characters ensures that both columns are sufficiently spaced, resulting in a clean and organized output.
Practical Data Display Example
For a more complex example, consider formatting names, ages, and salaries:
#include <iostream>
#include <iomanip>
int main() {
std::cout << std::setw(15) << "Name" << std::setw(10) << "Age" << std::setw(15) << "Salary" << std::endl;
std::cout << std::setw(15) << "John Doe" << std::setw(10) << 28 << std::setw(15) << 55000.50 << std::endl;
std::cout << std::setw(15) << "Jane Smith" << std::setw(10) << 32 << std::setw(15) << 65000.00 << std::endl;
return 0;
}
Explanation of Formatted Output:
This snippet creates a neat and organized display of names, ages, and salaries. Each entry is aligned correctly, ensuring readability and professionalism.
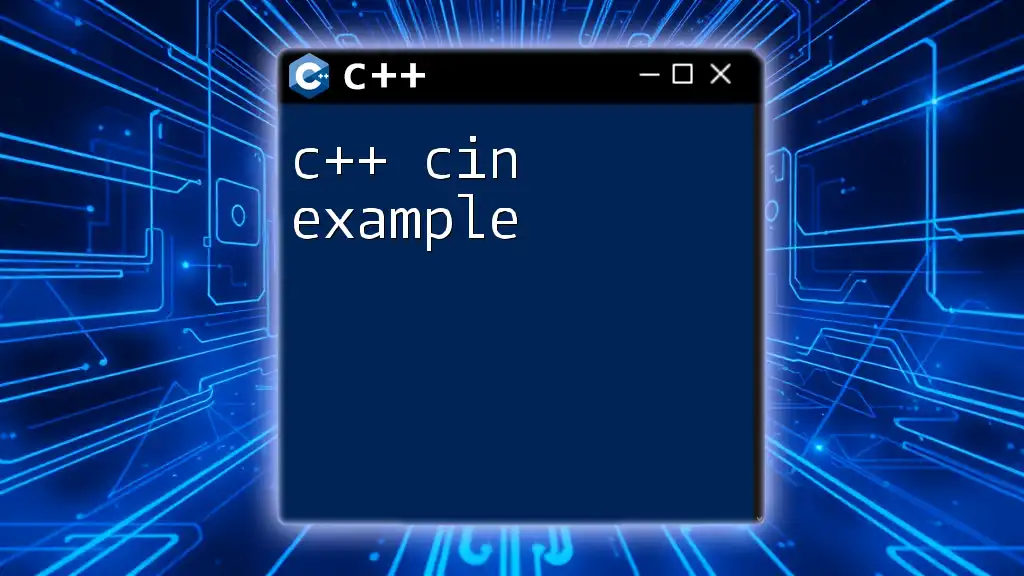
Combining `setw` with Other Manipulators
Using Multiple Manipulators
You can enhance your output further by combining `setw` with other manipulators like `setprecision` and `fixed`. For example:
#include <iostream>
#include <iomanip>
int main() {
double pi = 3.14159;
std::cout << std::setw(10) << std::fixed << std::setprecision(2) << pi << std::endl;
return 0;
}
Discussion on Precision and Alignment:
This code snippet demonstrates how to set the total width of the output and the precision for floating point numbers. The output will be right-aligned within the specified width, maintaining both alignment and the desired number of decimal places.
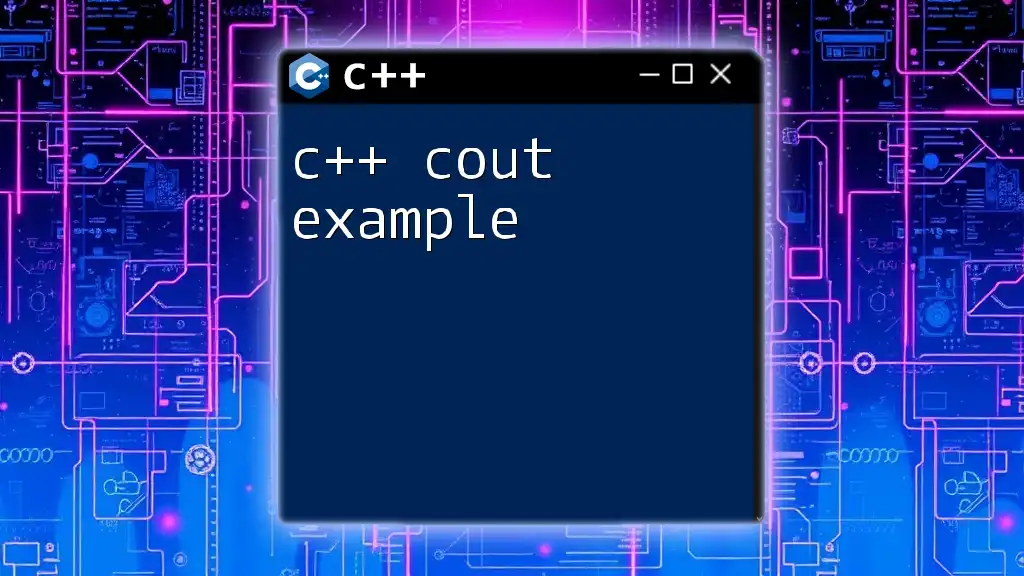
Common Pitfalls with `setw`
Not Resetting Width After Use
One common misunderstanding is that `setw` only applies to the next output. It does not reset automatically for subsequent outputs. If you use `setw` once and make another output call without specifying the width again, that output will not be formatted:
std::cout << std::setw(10) << "Item"; // Sets width
std::cout << "Price"; // Price appears without width adjustment
Impact of Integer vs. String Output
When using different data types, remember that the width set by `setw` can lead to unexpected spacing if they vary. For instance, the display of `int` versus `string` types can lead to misaligned columns if not controlled properly.
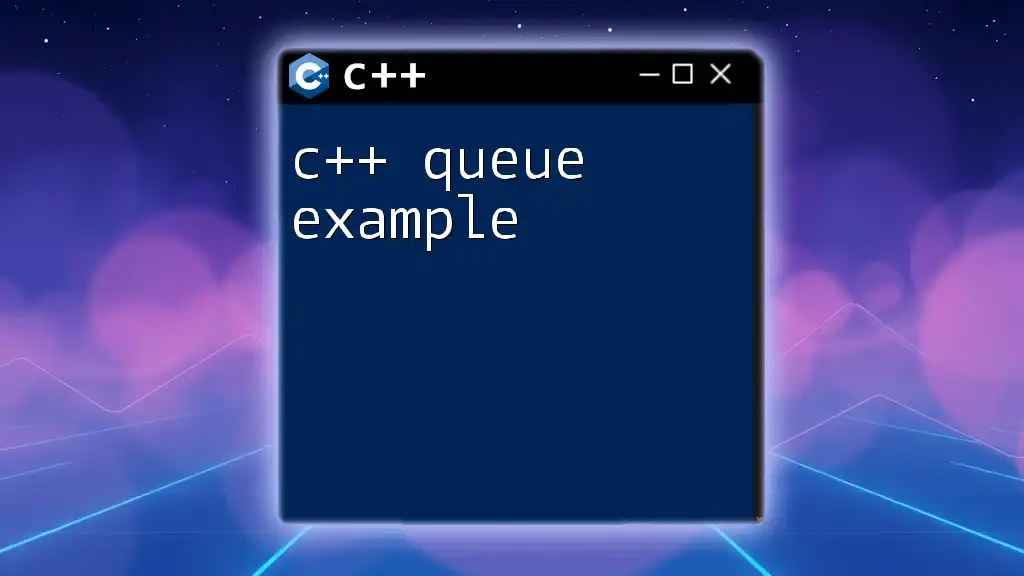
Best Practices When Using `setw`
Choosing Appropriate Width
When using `setw`, consider the longest item you expect in a column to avoid truncation. A good practice is to pad a little extra space, ensuring that dynamically changing data still aligns well.
Consistent Formatting for User Interfaces
Maintaining consistency in your output formatting is key to a good user experience. Use the same width for similar types of outputs throughout your program—this fosters better readability and professionalism.
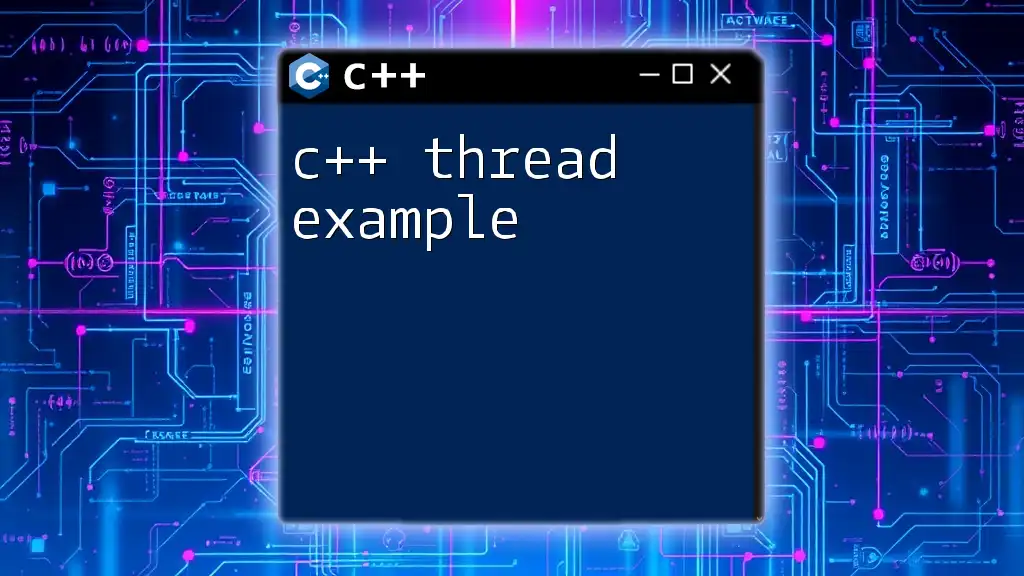
Conclusion
In summary, the `setw` manipulator in C++ is a powerful tool for structuring console output in a readable manner. Through its ability to set output field widths, it allows for neat organization of data, improving user interaction. Practicing its application with various data types and formats will help solidify your understanding and effectiveness in presenting data in your C++ programs.
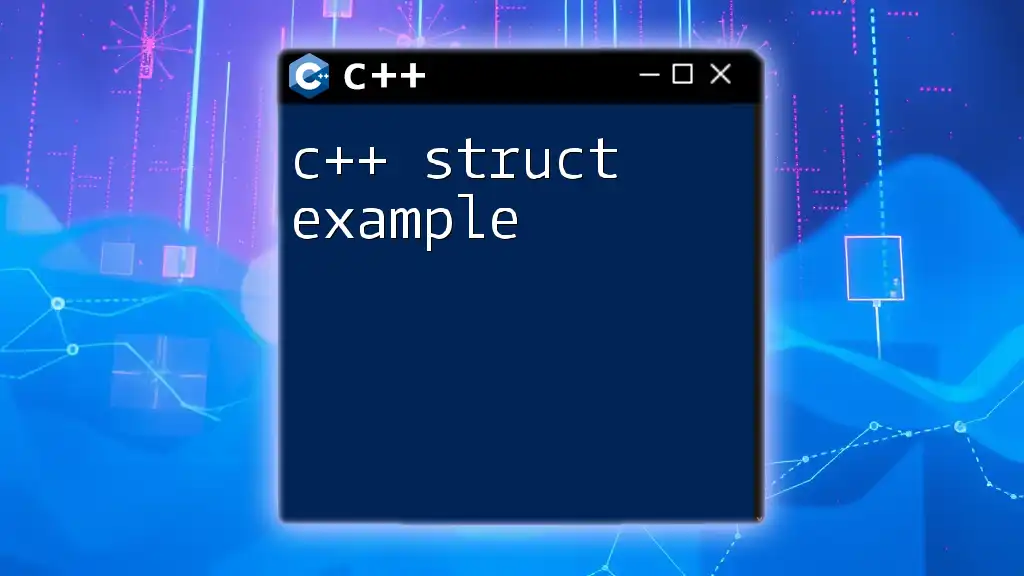
Call to Action
To further enhance your skills, explore additional resources such as online tutorials, coding books, and practice exercises focused on C++ output formatting. Consistent practice will make you proficient with manipulators like `setw` and more.