C++ sample problems provide practical exercises to help learners quickly grasp the syntax and commands of the C++ programming language.
Here’s a simple code snippet that demonstrates how to reverse a string in C++:
#include <iostream>
#include <string>
void reverseString(std::string& str) {
int n = str.length();
for (int i = 0; i < n / 2; i++) {
std::swap(str[i], str[n - i - 1]);
}
}
int main() {
std::string str = "Hello, World!";
reverseString(str);
std::cout << str << std::endl; // Output: !dlroW ,olleH
return 0;
}
Understanding Programming Practice Problems in C++
What Are Programming Practice Problems?
Programming practice problems are challenges designed to enhance your coding abilities by allowing you to apply theoretical knowledge in a practical context. In the realm of C++, these problems facilitate the development of your algorithmic thinking and enhance your understanding of key programming concepts, such as data structures, control flow, and functions. Solving these problems is essential for anyone looking to excel in C++ programming, as they help build confidence and skill set.
Categories of C++ Practice Problems
C++ sample problems can be categorized based on their complexity, ensuring that learners at all levels can find challenges that suit their skills.
Basic Level Problems often focus on fundamental concepts, providing a solid foundation for beginners. These problems assist in understanding the syntax and basic constructs of C++, allowing new programmers to write simple and effective code.
Intermediate Level Problems introduce more complex scenarios that require a combination of knowledge and creativity. These problems may involve logical thinking and a deeper understanding of algorithms and data types.
Advanced Level Problems represent the pinnacle of programming challenge, requiring proficiency in C++ and often demanding the use of advanced algorithms, performance optimization, and smart coding practices.
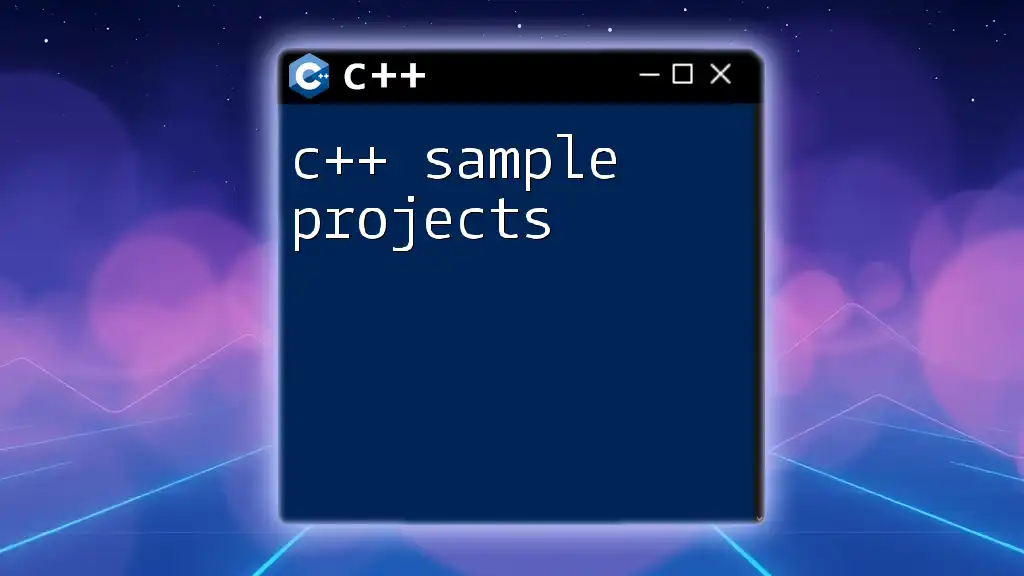
Sample C++ Programming Practice Problems
Basic Level Problems
Problem: Hello World
Description
The quintessential first step into the world of programming is the "Hello, World!" program—the simplest code that outputs a message to the console.
Code Example
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Explanation
In this code, we include the `iostream` header to utilize the standard input-output stream. The function `main()` serves as the entry point of the program, where `std::cout` is used to print a message to the console. The `<<` operator directs the output stream, and `std::endl` flushes the output buffer.
Problem: Simple Calculator
Description
Next, we delve into a simple calculator program, which accepts two numbers and performs basic arithmetic operations.
Code Example
#include <iostream>
int main() {
double num1, num2;
char operation;
std::cout << "Enter first number: ";
std::cin >> num1;
std::cout << "Enter second number: ";
std::cin >> num2;
std::cout << "Enter operator (+, -, *, /): ";
std::cin >> operation;
switch(operation) {
case '+':
std::cout << num1 + num2;
break;
case '-':
std::cout << num1 - num2;
break;
case '*':
std::cout << num1 * num2;
break;
case '/':
if(num2 != 0)
std::cout << num1 / num2;
else
std::cout << "Division by zero!";
break;
default:
std::cout << "Invalid operator!";
}
return 0;
}
Explanation
This program begins by prompting the user for input. We receive two numbers and an operator. The `switch` statement then evaluates the operator to determine which arithmetic operation to perform. The program includes error handling for division by zero, illustrating how C++ can manage exceptions logically.
Intermediate Level Problems
Problem: Check Palindrome
Description
The next challenge is developing a program to verify if a string is a palindrome, a word that reads the same forwards and backward.
Code Example
#include <iostream>
#include <string>
bool isPalindrome(const std::string& str) {
std::string revStr(str.rbegin(), str.rend());
return str == revStr;
}
int main() {
std::string input;
std::cout << "Enter a word: ";
std::cin >> input;
if (isPalindrome(input))
std::cout << "The word is a palindrome.";
else
std::cout << "The word is not a palindrome.";
return 0;
}
Explanation
The function `isPalindrome` takes a string and creates a reversed version using an iterator which helps understand the key concept of string manipulation in C++. The comparison between the original and reversed strings determines if the input is indeed a palindrome.
Problem: FizzBuzz Challenge
Description
The FizzBuzz challenge instructs programmers to loop through numbers 1 to 100 and print "Fizz" for numbers divisible by 3, "Buzz" for multiples of 5, and "FizzBuzz" for multiples of both.
Code Example
#include <iostream>
int main() {
for (int i = 1; i <= 100; ++i) {
if (i % 3 == 0 && i % 5 == 0)
std::cout << "FizzBuzz\n";
else if (i % 3 == 0)
std::cout << "Fizz\n";
else if (i % 5 == 0)
std::cout << "Buzz\n";
else
std::cout << i << "\n";
}
return 0;
}
Explanation
This code demonstrates the use of loops and conditional statements in C++. The nested `if-else` structure evaluates the cube conditions and outputs the corresponding results, showcasing fundamental concepts necessary for C++.
Advanced Level Problems
Problem: Fibonacci Sequence
Description
Developing a program to generate the Fibonacci sequence requires understanding both recursion and iteration.
Code Example
#include <iostream>
void fibonacci(int n) {
int t1 = 0, t2 = 1, nextTerm = 0;
for (int i = 1; i <= n; ++i) {
std::cout << t1 << ", ";
nextTerm = t1 + t2;
t1 = t2;
t2 = nextTerm;
}
}
int main() {
int n;
std::cout << "Enter the number of terms: ";
std::cin >> n;
fibonacci(n);
return 0;
}
Explanation
Here, a simple yet effective iterative method is employed to compute the Fibonacci series. Each term is computed based on the two preceding terms. This program also introduces concepts around functions, parameter passing, and iterative logic.
Problem: Sorting Algorithms
Description
Sorting algorithms are important for organizing data, and implementing bubble sort is a classic exercise in algorithm design.
Code Example
#include <iostream>
void bubbleSort(int arr[], int n) {
for (int i = 0; i < n-1; ++i)
for (int j = 0; j < n-i-1; ++j)
if (arr[j] > arr[j+1])
std::swap(arr[j], arr[j+1]);
}
int main() {
int arr[] = {32, 12, 45, 67, 23};
int n = sizeof(arr)/sizeof(arr[0]);
bubbleSort(arr, n);
std::cout << "Sorted array: ";
for (int i : arr) std::cout << i << " ";
return 0;
}
Explanation
The bubble sort function iteratively compares adjacent elements, swapping them if they are in the wrong order. This example encapsulates the essence of algorithmic efficiency and the importance of understanding sorting techniques.
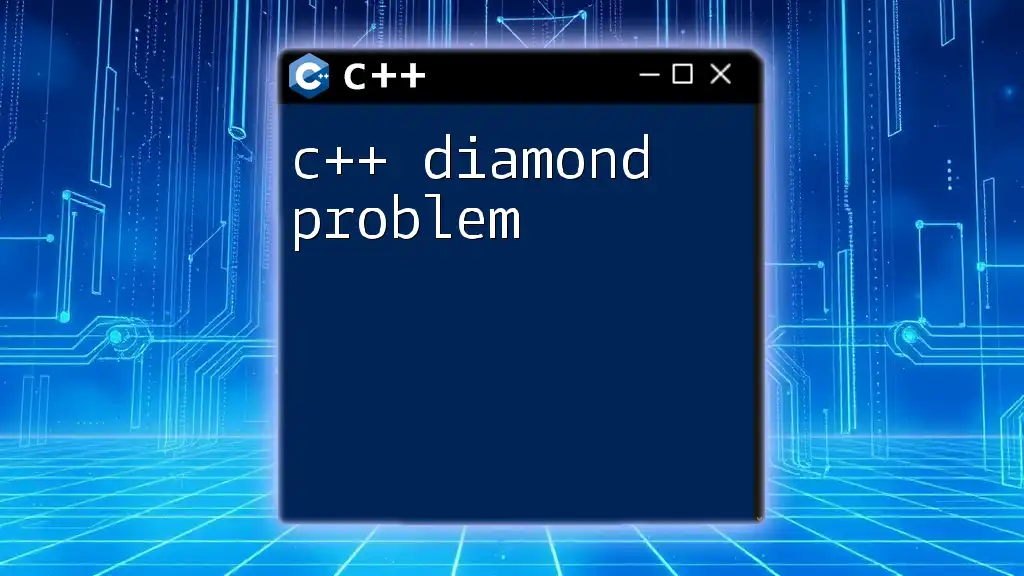
Conclusion
Recap of C++ Practice Problems
By engaging with these C++ sample problems, programmers can progressively enhance their coding skills from basic to advanced levels. Each problem serves as a building block, contributing to a more robust understanding of programming concepts and best practices.
Encouragement for Continued Learning
Remember, the more you practice, the more proficient you will become. Continue to challenge yourself with various problems to solidify your knowledge and expand your skills in C++.
Resources for Additional Practice
For those seeking further practice, numerous resources, including books, websites, and coding platforms, are available that offer extensive collections of C++ programming challenges.
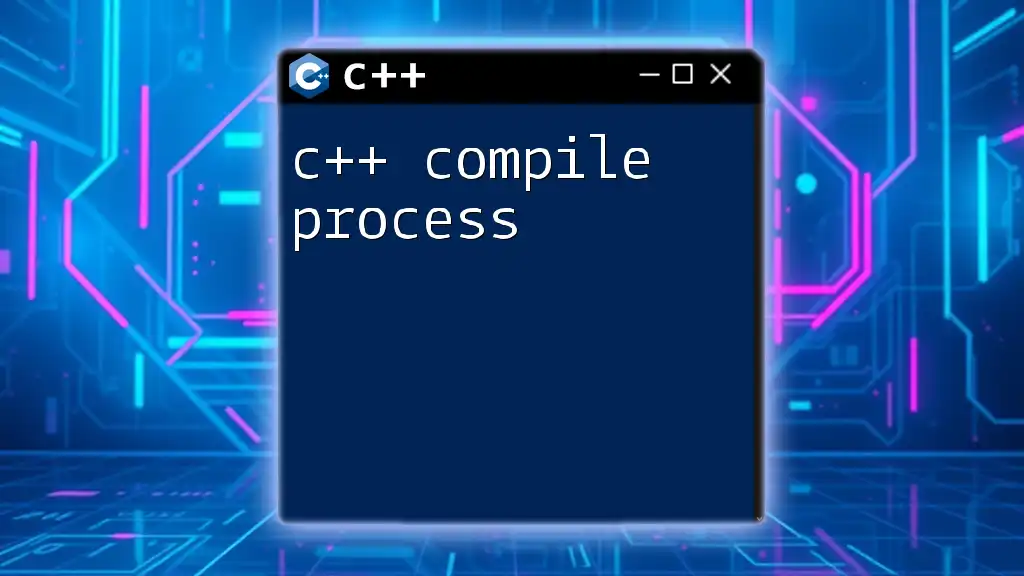
Call to Action
Stay engaged and continue your learning journey! Subscribe to our updates for more valuable tutorials and practice problems in C++, and join our vibrant community of learners striving for success in programming.