The C++ diamond problem occurs in multiple inheritance when a derived class inherits from two classes that both inherit from a common base class, leading to ambiguity about which base class's methods or properties to use.
class Base {
public:
void show() {
std::cout << "Base class show()" << std::endl;
}
};
class DerivedA : public Base {
public:
void show() {
std::cout << "DerivedA class show()" << std::endl;
}
};
class DerivedB : public Base {
public:
void show() {
std::cout << "DerivedB class show()" << std::endl;
}
};
class Diamond : public DerivedA, public DerivedB {
// Diamond will cause ambiguity when calling show() method
};
int main() {
Diamond d;
// d.show(); // This will cause an error due to ambiguity
return 0;
}
Understanding the Diamond Problem in C++
What is the Diamond Problem?
The C++ diamond problem arises in the context of multiple inheritance when a derived class inherits from two base classes that both inherit from a common superclass. This creates a "diamond" shape in the inheritance hierarchy. When a member function from the shared base class is called, it can cause ambiguity since there are two paths to reach that member, leading to confusion in which implementation should be invoked.
Why Does the Diamond Problem Occur?
The diamond problem occurs due to the nature of multiple inheritance. When a class (let's call it `D`) inherits from two classes (`B` and `C`), both of which inherit from a common base class (`A`), the compiler cannot clearly determine which version of the members of `A` should be used by `D`. This ambiguity can lead to unexpected behaviors and compile-time errors, which is why it’s crucial to handle it correctly.
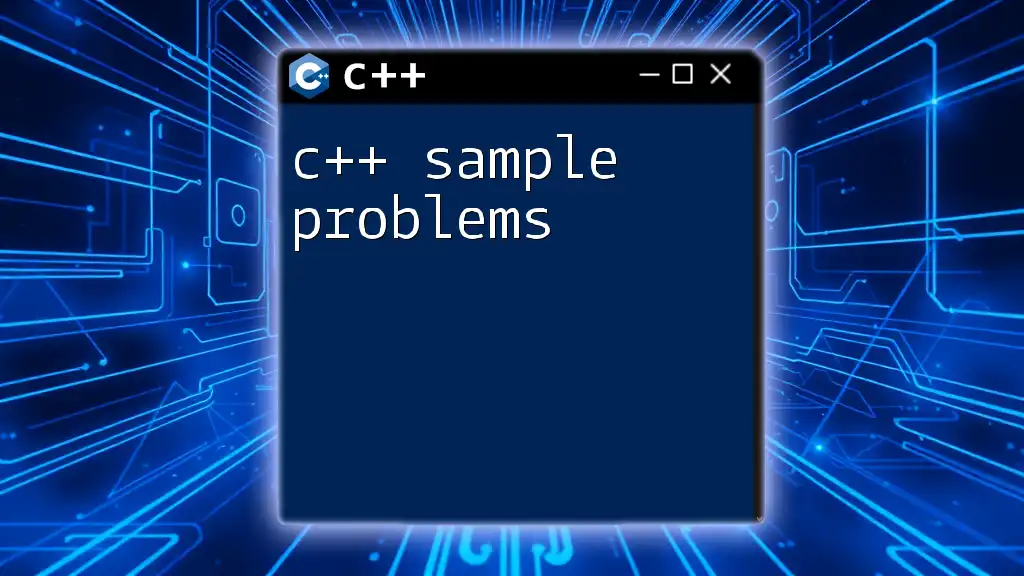
Example of the Diamond Problem
Setting Up the Problem
Consider a simple scenario in C++ setup where the diamond problem is illustrated through the following code:
class A {
public:
void display() { std::cout << "Class A" << std::endl; }
};
class B : public A {
};
class C : public A {
};
class D : public B, public C {
};
In this code snippet:
- Class `A` has a member function `display()`.
- Class `B` and class `C` both inherit from `A`.
- Class `D` inherits from both `B` and `C`.
Complications Arising from the Diamond Problem
When you attempt to call the `display()` function on an object of class `D`, you encounter an ambiguity:
D obj;
obj.display(); // Error: call of a member function 'display' is ambiguous
The compiler throws an error because it cannot determine whether to invoke `B::display()` or `C::display()`. This situation exemplifies the core issue behind the C++ diamond problem: ambiguous member function calls when there are multiple paths to a base class member.
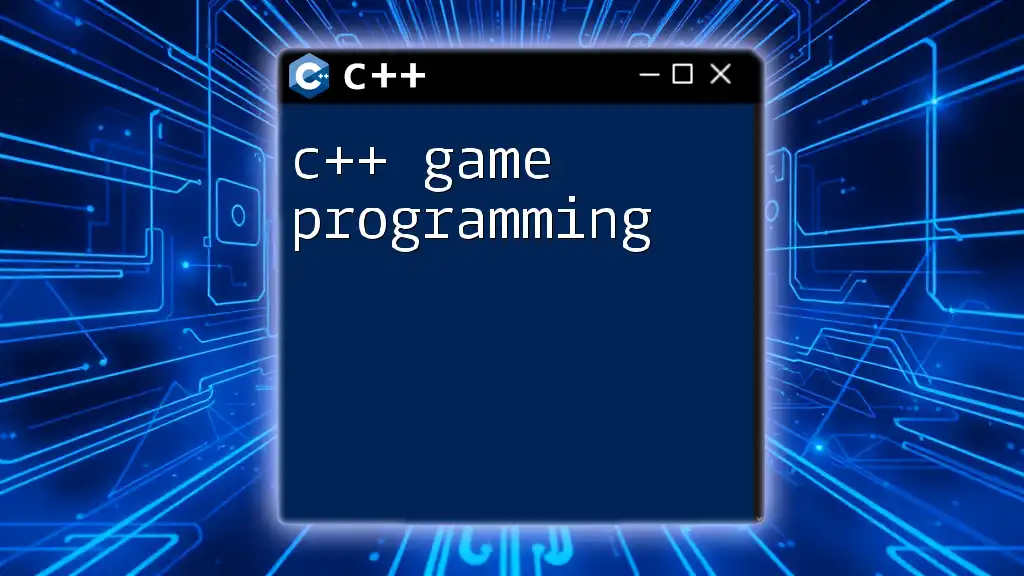
Resolving the Diamond Problem
Introduction to Virtual Inheritance
To resolve the diamond problem effectively, C++ provides the concept of virtual inheritance. This ensures that when a class inherits from a base class virtually, only one instance of the base class is created, regardless of how many times it is inherited down the chain.
Implementing Virtual Inheritance
Let’s see how to implement virtual inheritance to eliminate ambiguity:
class A {
public:
void display() { std::cout << "Class A" << std::endl; }
};
class B : virtual public A {
};
class C : virtual public A {
};
class D : public B, public C {
};
In this modified code:
- The classes `B` and `C` inherit from `A` using the `virtual` keyword.
- This signifies that any class inheriting from `B` or `C` will refer to the same instance of `A`.
Practical Example
With this setup, we can now create an object of class `D` and call the `display()` method without encountering ambiguity:
D obj;
obj.display(); // Works without ambiguity
Here, `obj.display()` will correctly call the `display()` function from `A`, demonstrating how virtual inheritance effectively resolves the diamond problem, allowing a single, unambiguous reference to the base class.
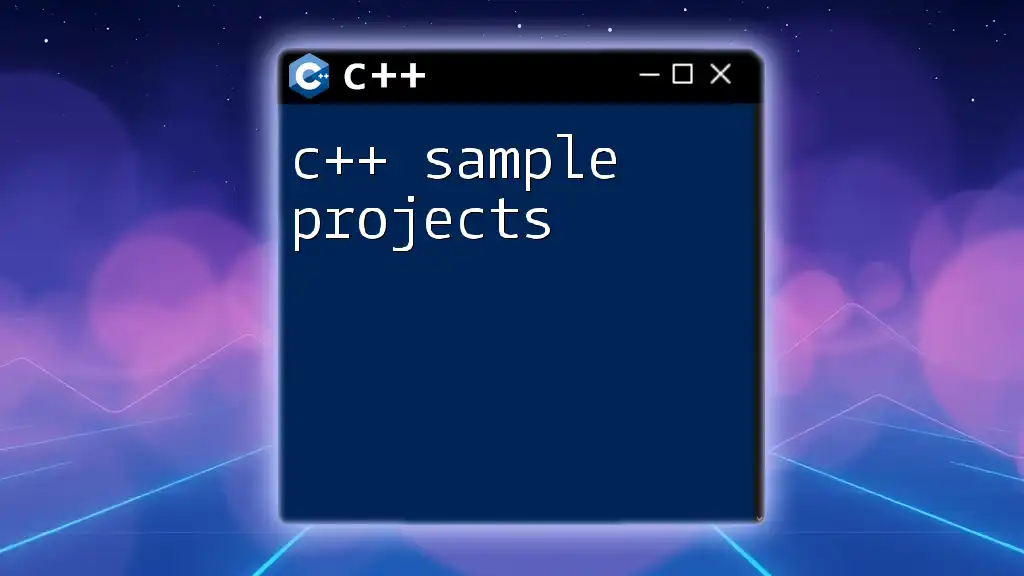
Advantages and Disadvantages of Virtual Inheritance
Advantages
- Reduces redundancy: Prevents multiple instances of a base class in the inheritance hierarchy.
- Ensures a single instance: Guarantees that only one instance of a base class is used, which simplifies memory management and behavior in complex class hierarchies.
Disadvantages
- Increased complexity: The implementation and understanding of virtual inheritance can introduce more complexity in class design. Developers need to be clear on how virtual inheritance affects object construction and initialization.
- Potential performance overhead: There may be slight performance penalties due to the extra pointer indirection that virtual inheritance introduces, particularly in complex inheritance structures.
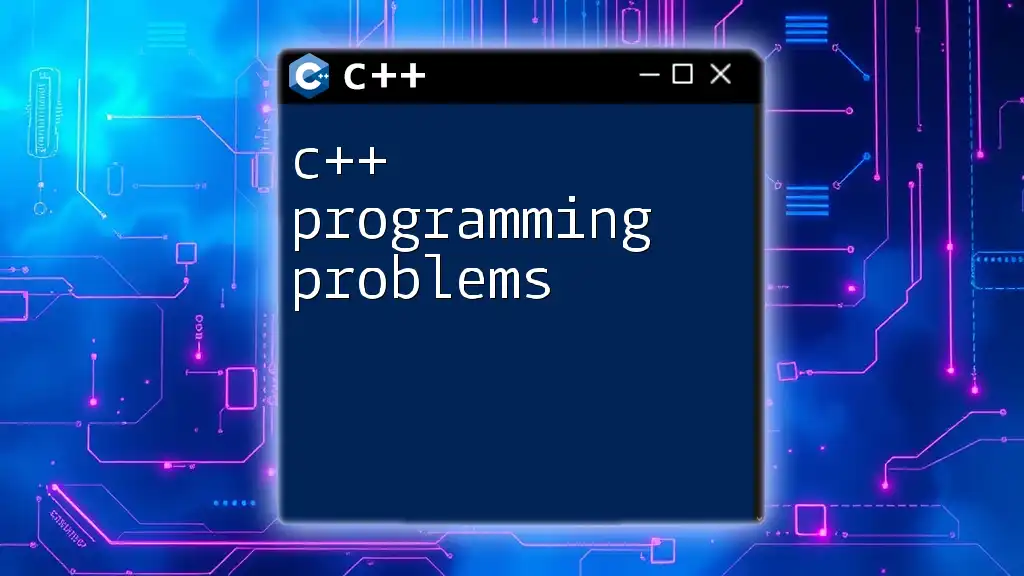
Conclusion
Key Takeaways
The C++ diamond problem poses significant challenges in multiple inheritance scenarios, leading to ambiguity when accessing base class members. However, through the use of virtual inheritance, developers can elegantly resolve these issues, promoting clarity and efficiency in class designs. Understanding the diamond problem and its solutions is essential for effective C++ programming, especially in large-scale applications that utilize complex class hierarchies.
Further Reading
For those interested in deepening their understanding of C++ inheritance, further resources include comprehensive books on C++ design patterns, articles from reputable coding websites, and online courses focused on advanced C++ programming concepts.
FAQs
It's important to address common questions about the diamond problem in C++ to reinforce understanding and clarify any uncertainties that may arise as one explores inheritance and object-oriented programming in the language.