A C++ memory pool is a fixed-size block of memory that is preallocated for dynamic memory allocation, allowing for efficient reuse of memory blocks and reducing fragmentation.
Here’s a simple example of how to implement a memory pool in C++:
#include <iostream>
#include <vector>
class MemoryPool {
public:
MemoryPool(size_t size) {
pool.resize(size);
freeBlocks.reserve(size);
for (size_t i = 0; i < size; ++i) {
freeBlocks.push_back(&pool[i]);
}
}
void* allocate() {
if (freeBlocks.empty()) {
return nullptr; // no memory left
}
void* block = freeBlocks.back();
freeBlocks.pop_back();
return block;
}
void deallocate(void* block) {
freeBlocks.push_back(block);
}
private:
std::vector<char> pool;
std::vector<void*> freeBlocks;
};
int main() {
MemoryPool pool(5);
void* block1 = pool.allocate();
pool.deallocate(block1);
return 0;
}
What is a Memory Pool?
A memory pool is a technique used in programming to manage memory allocation more efficiently, allowing multiple objects of similar sizes to be allocated and deallocated from a pre-allocated block of memory. This method minimizes the overhead associated with frequent allocations and deallocations from the heap, thus enhancing performance and reducing fragmentation.
Memory pools are particularly useful in scenarios where the same size objects are created and destroyed repeatedly, such as in game engines or real-time applications. By utilizing a memory pool, a programmer can avoid the pitfalls of traditional dynamic memory management.
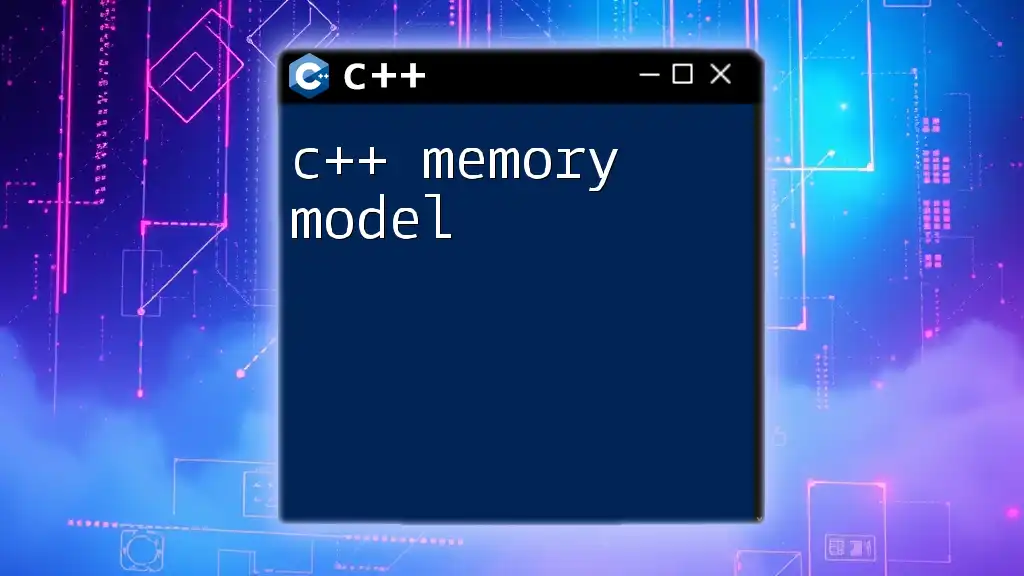
Advantages of Using Memory Pools
The benefits of using a C++ memory pool are substantial.
-
Performance Improvements: With memory pools, the process of allocation is faster since the pool contains pre-allocated memory chunks. Consequently, there is no need to request memory from the OS.
-
Reduced Fragmentation: Using pools helps mitigate memory fragmentation by allocating fixed-size blocks. This consistent allocation strategy helps keep memory usage efficient.
-
Improved Speed: Deallocation is also expedited as the memory is simply returned to the pool without the need for complex operations.
-
Optimized Memory Usage: By pooling objects of similar sizes, you can avoid wasting memory on large blocks that may go underutilized.
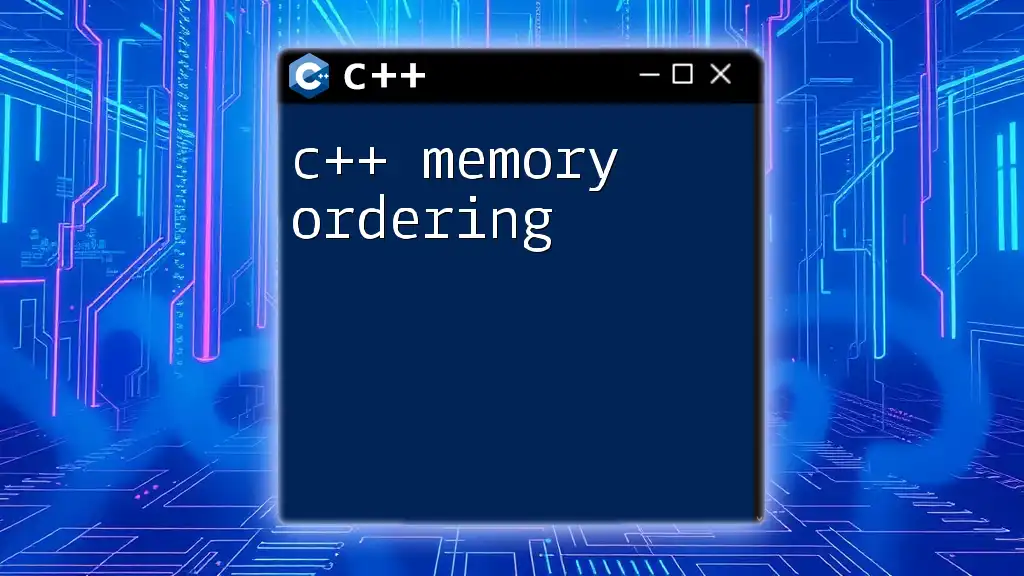
Understanding C++ Memory Management
Basics of C++ Memory Management
Memory management in C++ primarily revolves around two types of storage: stack and heap.
- The stack is where local variables are stored and managed automatically when they go out of scope.
- The heap, on the other hand, is used for dynamic memory allocation, where memory must be explicitly managed by the programmer.
In C++, developers use pointers and references to access and manipulate memory. Understanding the distinctions between manual and automatic memory management is crucial for a programmer, as improper management can lead to various issues.
Common Memory Management Issues
Despite its power, C++ memory management is rife with challenges:
- Memory Leaks: Occur when memory is allocated but never freed, resulting in wasted memory.
- Fragmentation: Happens when memory is allocated and deallocated frequently, leading to small unusable segments of memory.
- Overhead of Frequent Allocations: The performance can degrade if many allocations take place back-to-back, as the OS incurs costs for managing these operations.
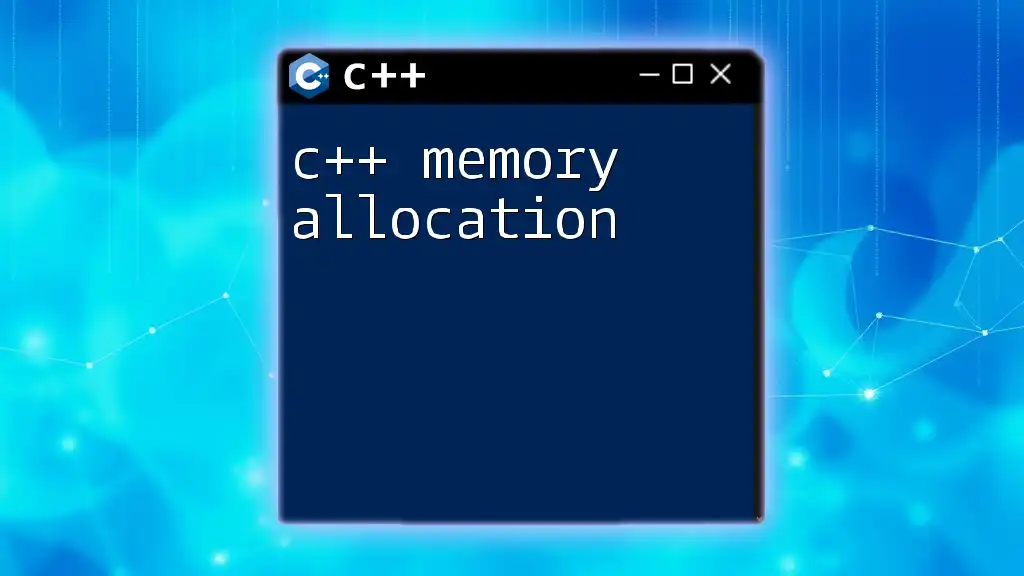
Implementing a Memory Pool in C++
Designing a Simple Memory Pool
Creating a basic memory pool involves designing key components that allow efficient management of memory blocks.
- The block size and alignment are crucial factors. For instance, aligning to the size of the target architecture can prevent performance penalties.
- Proper management of free blocks is necessary for effective memory retrieval.
Code Snippet: Basic Memory Pool Implementation
class MemoryPool {
public:
MemoryPool(size_t size);
~MemoryPool();
void* allocate();
void deallocate(void* ptr);
private:
char* pool; // memory pool
std::vector<void*> freeBlocks; // free block list
};
Allocating Memory from the Pool
Implementing the `allocate` Function
In the allocation function, the objective is to find a free block and return it. If necessary, the function can also split larger blocks into smaller chunks to fulfill the request.
Code Snippet: Allocating Memory
void* MemoryPool::allocate() {
if (freeBlocks.empty()) {
// Pool is exhausted
return nullptr;
}
void* block = freeBlocks.back();
freeBlocks.pop_back();
return block;
}
Deallocating Memory back to the Pool
Implementing the `deallocate` Function
Deallocation is straightforward: simply return the block back to the free block list. However, care must be taken to avoid double freeing, which can lead to undefined behaviors.
Code Snippet: Deallocating Memory
void MemoryPool::deallocate(void* ptr) {
freeBlocks.push_back(ptr);
}
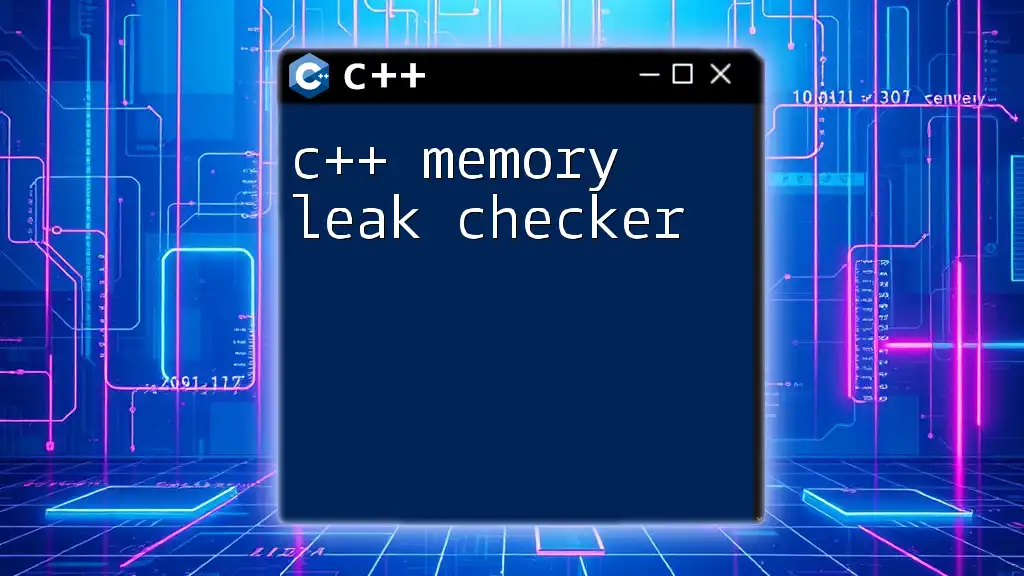
Advanced Memory Pool Concepts
Customizing the Memory Pool
As projects grow in complexity, the need to customize the memory pool becomes apparent.
Combining Multiple Pools
To manage different object sizes efficiently, one might choose to combine multiple pools. This strategy allows for handling diverse allocation requests more effectively.
Pool Growth and Shrinkage
Dynamically adjusting the size of the pool is another advanced concept. This adaptation involves managing memory when pools become empty or nearly empty to optimize resource usage.
Thread-Safety in Memory Pools
In multi-threaded environments, ensuring that allocation and deallocation are thread-safe is paramount. Implementing locks or using atomic operations can safeguard against race conditions, maintaining stability across concurrent accesses.
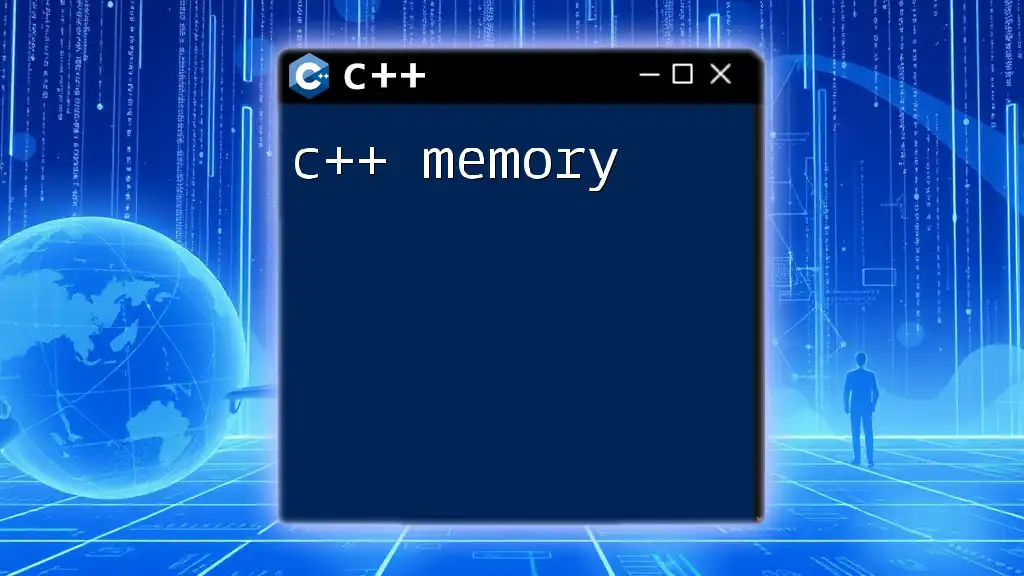
Challenges and Considerations
Potential Pitfalls of Memory Pools
Despite their advantages, memory pools come with challenges. The overhead of maintaining the pool can sometimes outweigh the benefits, particularly for small applications.
Debugging memory-related issues can also become more complex as pools obscure typical patterns in memory management.
Performance Considerations
When considering whether to implement a memory pool, it’s wise to benchmark it against traditional allocation strategies to understand the actual performance gains. Case studies across various applications highlight countless scenarios where memory pools significantly helped improve speed and memory efficiency.
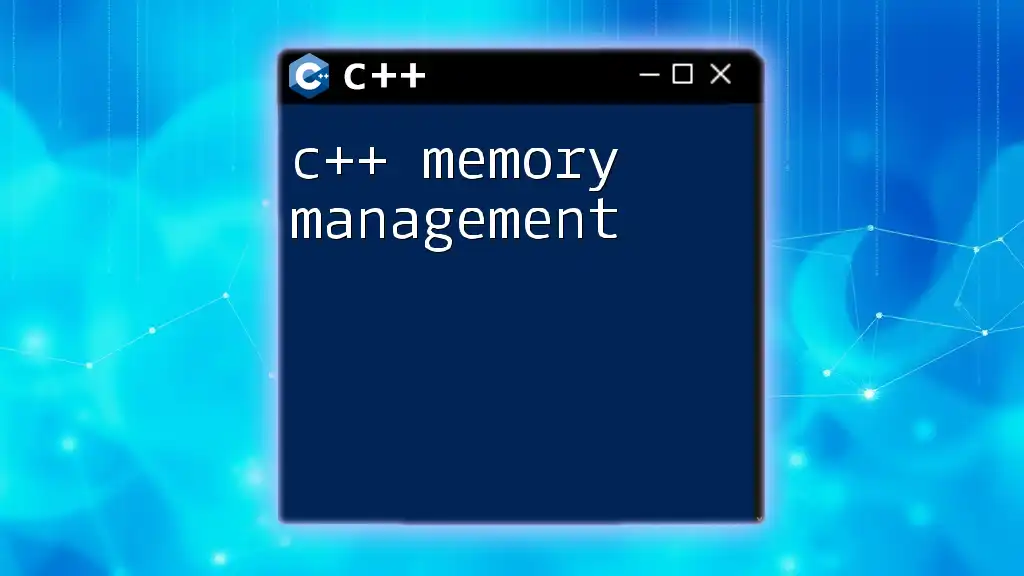
Conclusion
In summary, a C++ memory pool is a powerful tool for optimizing memory management in applications requiring frequent allocations of similar-sized objects. By leveraging the advantages of memory pools, programmers can create faster and more efficient applications that are less prone to fragmentation and memory leaks.
For those keen on advancing their C++ skills, implementing a memory pool can be a substantial step towards mastering memory management, paving the way for higher-performing applications.
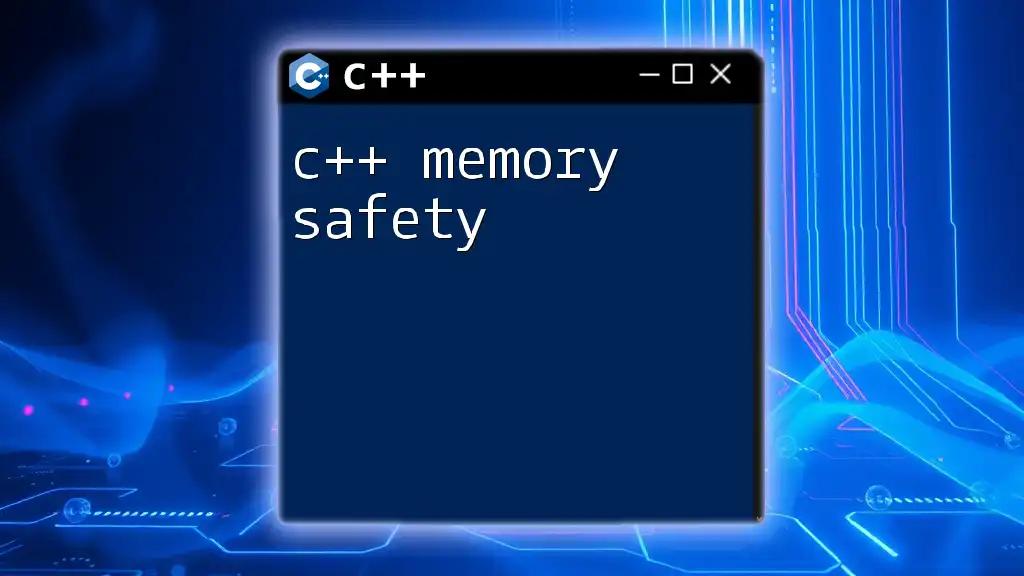
Additional Resources
To further your understanding of memory management in C++, consider exploring books and online resources that offer insight into advanced techniques and practical applications. Engaging with code examples via platforms like GitHub can also reinforce your knowledge and enable real-world application.