The `memcmp` function in C++ compares two blocks of memory and returns an integer indicating their relationship, which can be used to determine if they are equal, or which one is greater.
#include <cstring> // Include for memcmp
int main() {
const char* str1 = "Hello";
const char* str2 = "World";
int result = memcmp(str1, str2, 5); // Compare the first 5 characters
return result; // result < 0 if str1 < str2, 0 if equal, > 0 if str1 > str2
}
Understanding memory comparison
What is memory comparison?
Memory comparison refers to the process of evaluating the contents of one memory location against another. In the context of C++, all data is ultimately represented in memory; thus, understanding how to perform a comparison at the memory level is crucial for systems programming and performance optimization.
Why use memcmp?
The `memcmp` function is essential in situations where you need to quickly and efficiently compare raw memory blocks. This can include comparing strings, arrays, or complex data structures. Unlike traditional looping constructs that inspect one element at a time, `memcmp` is designed to compare multiple bytes simultaneously, making it a preferred choice for performance-critical applications.
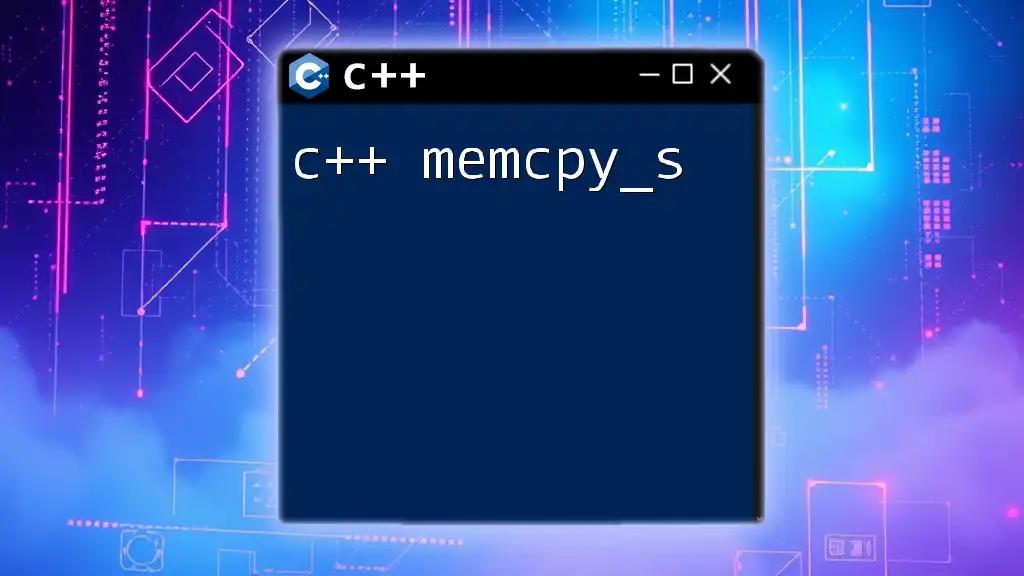
The `memcmp` function
Definition of memcmp
The `memcmp` function is defined in the `<cstring>` library. Its primary purpose is to compare a specified number of bytes from two blocks of memory. The function signature is as follows:
int memcmp(const void *ptr1, const void *ptr2, size_t num);
Here, `ptr1` and `ptr2` are pointers to the memory blocks you wish to compare, and `num` represents the number of bytes to compare.
Parameters explained
-
ptr1 and ptr2
These are pointers pointing to the memory locations you want to compare. Since `memcmp` operates on raw memory, the data types of the pointers can be anything, but generally, they are frequently used with `char` pointers or arrays. -
num
This parameter specifies the total number of bytes to compare. It is crucial to ensure that this value does not exceed the actual size of the memory blocks being compared; otherwise, it can lead to undefined behavior.
Return value
The return value of `memcmp` is an integer that provides insight into the relationship between the two memory blocks:
- A return value of less than zero indicates that the first block (`ptr1`) is less than the second block (`ptr2`).
- A return value of zero signifies that both blocks are identical.
- A return value of greater than zero indicates that the first block is greater than the second block.

Practical examples of memcmp
Basic usage
To demonstrate the use of `memcmp`, let's consider a straightforward case where we compare two character arrays. This example illustrates a simple string comparison.
#include <iostream>
#include <cstring>
int main() {
const char *str1 = "Hello";
const char *str2 = "Hello";
int result = memcmp(str1, str2, strlen(str1));
if (result == 0) {
std::cout << "Strings are equal." << std::endl;
} else {
std::cout << "Strings are not equal." << std::endl;
}
return 0;
}
In this example, since both strings are identical, `memcmp` returns 0, indicating equality.
Comparing numerical arrays
Next, we can apply `memcmp` to compare numerical arrays, which involves inspecting blocks of integers directly.
#include <iostream>
#include <cstring>
int main() {
int arr1[] = {1, 2, 3, 4, 5};
int arr2[] = {1, 2, 3, 4, 6};
int result = memcmp(arr1, arr2, sizeof(arr1));
if (result == 0) {
std::cout << "Arrays are equal." << std::endl;
} else {
std::cout << "Arrays are not equal." << std::endl;
}
return 0;
}
Here, `memcmp` helps efficiently determine that `arr1` and `arr2` are not equal, as the last elements differ.
Using memcmp with structures
When comparing structures, `memcmp` may also prove useful. This is essential for quickly determining if two objects of the same structure are equivalent.
#include <iostream>
#include <cstring>
struct Point {
int x;
int y;
};
int main() {
Point p1 = {1, 2};
Point p2 = {1, 2};
int result = memcmp(&p1, &p2, sizeof(Point));
if (result == 0) {
std::cout << "Structures are equal." << std::endl;
} else {
std::cout << "Structures are not equal." << std::endl;
}
return 0;
}
Since both `Point` structures have the same values, `memcmp` deems them equal.
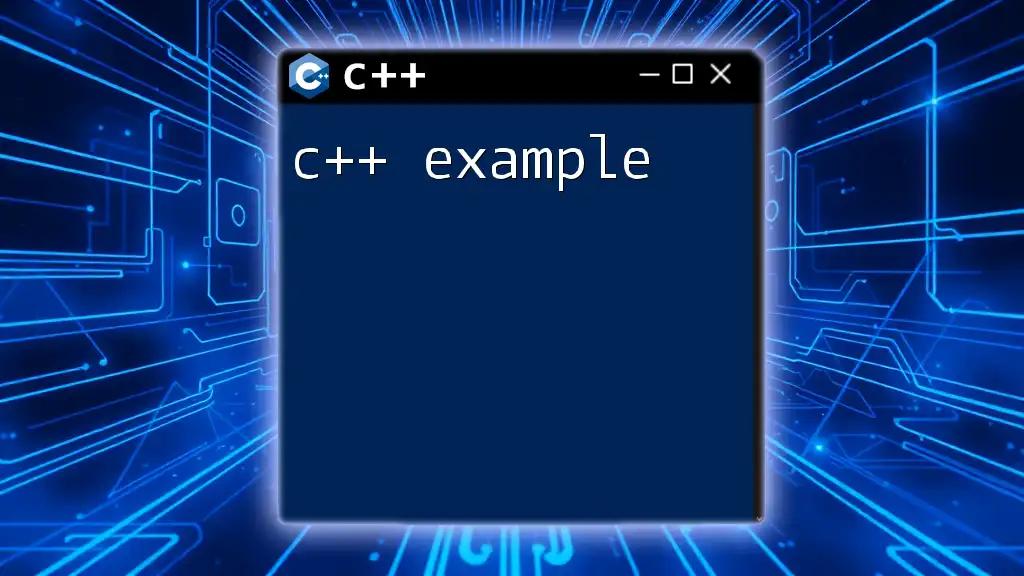
Common pitfalls and best practices
Understanding data alignment and padding
When dealing with structures, it’s crucial to remember that memory alignment and padding can introduce discrepancies. Different compilers may add padding bytes for alignment reasons, potentially leading to inaccurate results when using `memcmp`. Always analyze the structure layout to understand how `memcmp` will behave.
Avoiding type mismatches
Type compatibility is vital when comparing memory. Ensure that the types of data being pointed to match the types being compared. Mismatched types can lead to unpredictable outcomes.
When not to use memcmp
While `memcmp` is powerful, it should not replace string comparison functions for character strings. Using `strcmp` or `strncmp` is more appropriate in cases where you’re working with null-terminated strings to correctly consider string semantics.

Optimizations and performance considerations
Performance comparison with other methods
`memcmp` often outperforms traditional loops through memory, especially for large data structures. Due to its implementation, which may take advantage of processor optimizations, using `memcmp` can lead to significant performance gains when comparing large blocks of memory.
Memory access patterns
Optimize your memory comparisons by ensuring that the memory being accessed is contiguous and aligned. Fragmented memory access can cause cache misses, leading to slower performance. Structuring data with this in mind can enhance the efficacy of `memcmp`.

Conclusion
The `memcmp` function is a powerful tool in the C++ programmer's arsenal, allowing for efficient and straightforward memory comparisons. By understanding its syntax, functionality, and potential pitfalls, you can leverage `memcmp` to enhance the performance of your applications. Always remember to evaluate when it's appropriate to use `memcmp` and consider further learning resources to deepen your grasp of memory management in C++.