C++ memory management involves the allocation and deallocation of memory dynamically during runtime using operators like `new` and `delete`.
Here's a simple code snippet that demonstrates dynamic memory allocation in C++:
#include <iostream>
int main() {
int* ptr = new int; // allocate memory for an integer
*ptr = 42; // assign a value to the allocated memory
std::cout << *ptr << std::endl; // output the value
delete ptr; // free the allocated memory
return 0;
}
Types of Memory in C++
Stack Memory
Stack memory is a region of memory that stores temporary variables created by functions. It follows a Last In, First Out (LIFO) structure, meaning the last variable added to the stack is the first one to be removed.
When a function is called, a new block of stack memory is allocated for its local variables. As soon as the function exits, this memory is reclaimed automatically, making stack memory management fast and efficient. However, stack size is limited, and large allocations can lead to stack overflow.
Example: Local Variables in a Function
void stackExample() {
int a = 10; // 'a' is stored in stack
// 'a' is automatically destroyed when the function exits
}
Heap Memory
In contrast, heap memory is used for dynamic memory allocation, where the size and lifespan of objects are determined at runtime. This flexibility allows developers to allocate memory based on the program’s needs but comes with the responsibility of managing that memory manually.
Heap memory is managed using the operators `new` and `delete`. Memory allocated on the heap must be explicitly deallocated to avoid memory leaks.
Example: Using `new` and `delete`
void heapExample() {
int* ptr = new int; // dynamically allocated memory for an integer
*ptr = 20; // setting value
delete ptr; // freeing the allocated memory
}

Memory Allocation in C++
Static vs. Dynamic Allocation
Memory allocation in C++ can be static or dynamic. Static allocation occurs when the size of a variable is known at compile time. For instance, arrays defined with a fixed size belong to this category. On the other hand, dynamic allocation allows for the creation of data structures that can grow or shrink as needed, often responding to user input or system conditions.
The table below helps identify some key differences:
-
Static Allocation
- Predetermined size at compile-time
- Stack-based memory
- Automatically deallocated when the scope ends
-
Dynamic Allocation
- Size can be determined at runtime
- Heap-based memory
- Must be explicitly deallocated using `delete` to avoid memory leaks
Examples Illustrating Both Methods
// Static Allocation
int staticArray[5]; // memory allocated at compile time
// Dynamic Allocation
int* dynamicArray = new int[5]; // memory allocated at runtime

The C++ Memory Model
Understanding the C++ memory model is crucial for effective memory management. The memory layout in C++ consists of several regions:
- Code Segment: Stores compiled code.
- Data Segment: Contains global and static variables.
- Stack Segment: Handles function calls and local variables.
- Heap Segment: Manages dynamically allocated memory.
This model underpins how you should allocate memory and the potential impact on performance.

Memory Leaks and Their Prevention
Understanding Memory Leaks
A memory leak occurs when a program allocates memory but fails to release it back to the system. Over time, these leaks can accumulate, reducing the available memory and potentially leading to program crashes.
Detecting Memory Leaks
Several tools can help detect memory leaks, including Valgrind and AddressSanitizer. Valgrind works by running a program and reporting memory usage and leaks, providing valuable information for debugging.
Preventing Memory Leaks
Properly managing memory is key to preventing leaks. This includes using `delete` for each `new` and being careful when handling pointer variables. Adopting modern C++ practices, such as using smart pointers, can also mitigate these issues.
Example: Correct Use of `delete`
void exampleLeak() {
int* leakPtr = new int[10];
// Memory leak if delete is not called
delete[] leakPtr; // properly freeing memory prevents leak
}
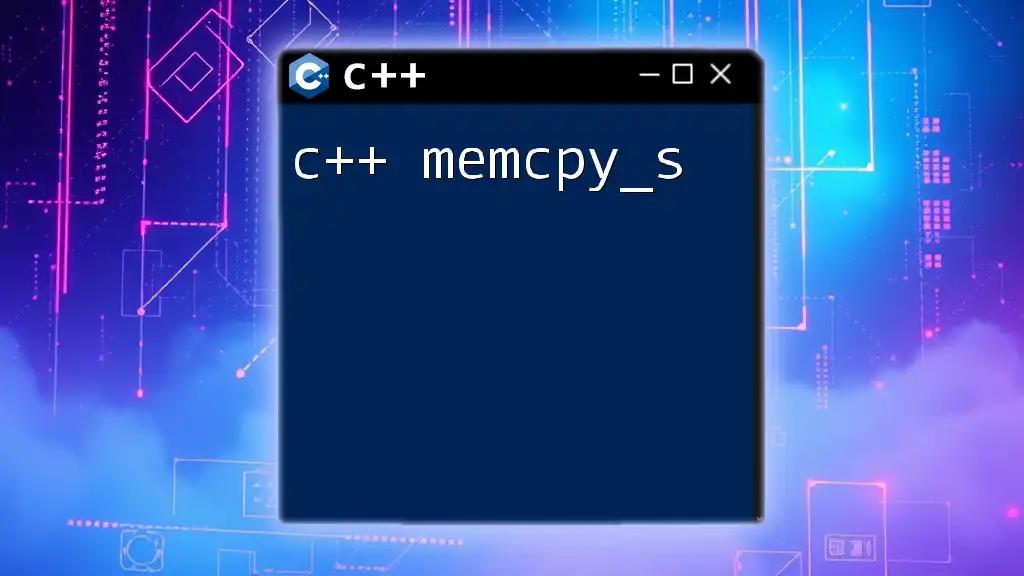
Smart Pointers in C++
What Are Smart Pointers?
Smart pointers are template classes in C++ that manage the lifecycle of dynamic objects, ensuring that memory is automatically released. They prevent common memory management issues, including leaks and dangling pointers.
Types of Smart Pointers
There are several types of smart pointers in C++, including:
- `std::unique_ptr`: Represents exclusive ownership of a resource and automatically deallocates when out of scope.
- `std::shared_ptr`: Allows multiple pointers to own the same resource, managing its lifetime based on the number of owners.
- `std::weak_ptr`: A non-owning reference that observes a shared pointer without affecting its lifetime.
Using Smart Pointers
Using smart pointers can dramatically simplify memory management. Here’s an example utilizing `std::unique_ptr`:
#include <memory>
void smartPointerExample() {
std::unique_ptr<int> uniquePtr = std::make_unique<int>(5);
// uniquePtr automatically frees memory when it goes out of scope
}

The Role of Constructors and Destructors
Understanding Constructors
Constructors are special member functions that execute upon object creation, often initializing memory. Each time an object is instantiated, its constructor runs to set up the initial state, including memory setup.
class MyClass {
public:
MyClass() {
// Constructor initializes memory or other resources
}
};
Understanding Destructors
Destructors perform cleanup operations when an object is destroyed. They are crucial for freeing resources allocated during the object’s lifetime.
class MyClass {
public:
~MyClass() {
// Destructor frees memory or other resources
}
};
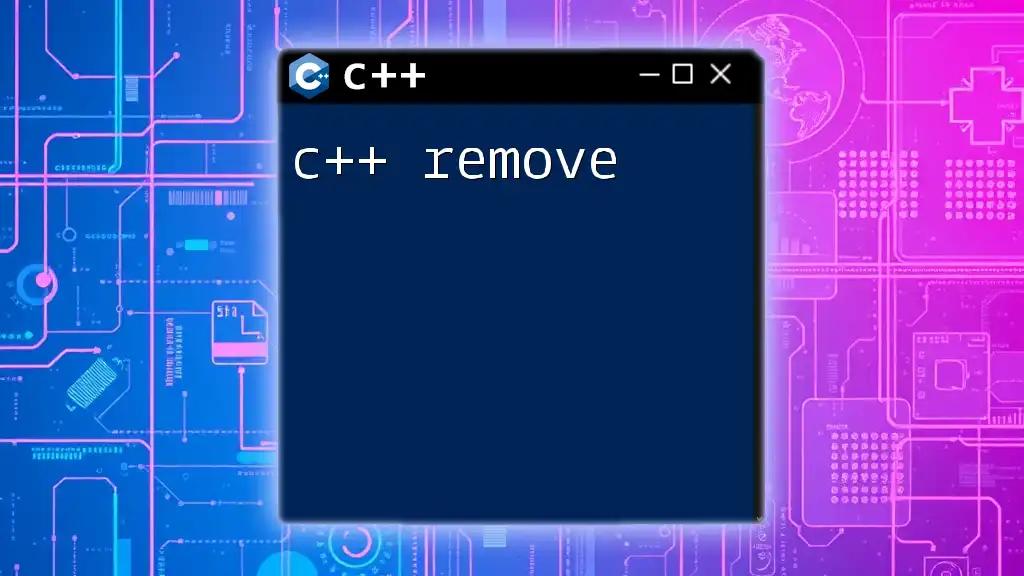
Conclusion
In summary, effective C++ memory management is vital for building robust applications. By understanding the differences between stack and heap memory, mastering allocation methods, and employing smart pointers, developers can significantly reduce memory-related issues. Always remember that good memory management practices lead not only to a more efficient program but also a better user experience overall.

Additional Resources
To deepen your understanding of C++ memory management, consider exploring books on advanced C++ techniques, online tutorials, and engaging with communities that focus on C++ programming. These resources can further enhance your skills in managing memory effectively.