The C++ memory model defines how threads interact through memory, specifying the visibility and ordering of operations to ensure reliable concurrent programming.
#include <iostream>
#include <thread>
#include <atomic>
std::atomic<int> shared_counter(0);
void increment() {
for (int i = 0; i < 1000; ++i) {
shared_counter++;
}
}
int main() {
std::thread t1(increment);
std::thread t2(increment);
t1.join();
t2.join();
std::cout << "Final counter value: " << shared_counter.load() << std::endl;
return 0;
}
Understanding Memory Models in C++
The C++ memory model defines how variables can be read and written in a multi-threaded environment, ensuring consistency and predictability when multiple threads interact. Unlike other programming languages, C++ presents a nuanced view of memory, acknowledging the need for fine-grained control in system-level programming.
Understanding memory models is crucial for developers, as improper handling can lead to subtle bugs and unpredictable behavior. The core aspect of the C++ memory model revolves around atomicity, memory ordering, and visibility across threads.
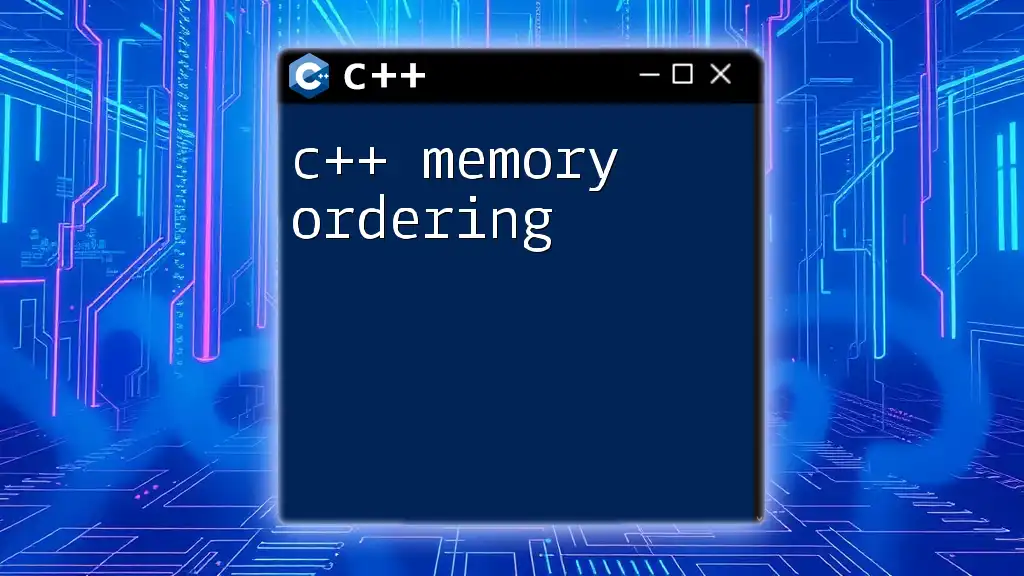
The Role of C++ Memory Model in Multithreading
As software systems become increasingly concurrent, the risk of data races and race conditions grows. C++ provides a memory model designed to address these issues effectively. By defining how memory is accessed and manipulated in the context of multiple threads, the C++ memory model establishes guidelines that help ensure thread safety.
In multi-threading, it’s essential to ensure that when one thread updates a value, other threads see this update correctly. The memory model facilitates this through concepts like memory visibility and ordering, thus allowing developers to write safer and more efficient code.
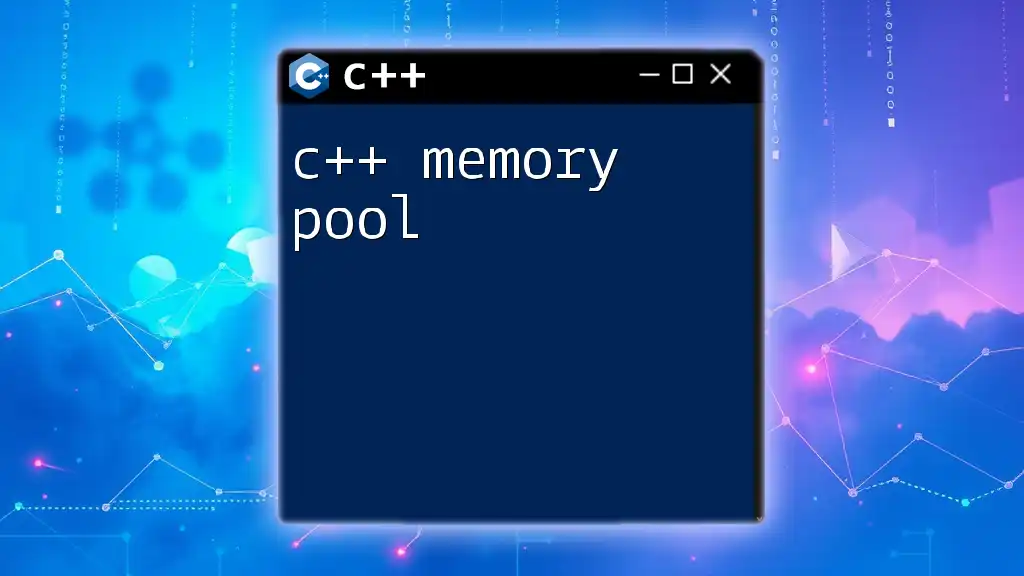
Key Concepts of C++ Memory Model
Atomic Operations
Atomic operations are the foundation of the C++ memory model. They allow variables to be modified by multiple threads without interference. C++11 introduced support for atomic types via the `<atomic>` header, enabling developers to conduct operations that are guaranteed to be performed entirely or not at all.
For example, when using `std::atomic`, the operations performed on atomic types are inherently thread-safe. Here's a simple code snippet illustrating the concept:
#include <atomic>
#include <iostream>
#include <thread>
std::atomic<int> counter{0};
void increment() {
for (int i = 0; i < 1000; ++i) {
counter++;
}
}
int main() {
std::thread t1(increment);
std::thread t2(increment);
t1.join();
t2.join();
std::cout << "Final counter value: " << counter.load() << std::endl;
}
In this example, we use `std::atomic<int>` to ensure that the `counter` variable is incremented safely across two threads. Without atomic types, concurrent updates could corrupt the data.
Memory Order
In the realm of atomic operations, memory order dictates how memory interactions are perceived by different threads. C++ offers several memory orderings that allow developers to fine-tune performance while maintaining correctness:
- `memory_order_relaxed`: Provides no synchronization or ordering guarantees. It is solely about atomicity.
- `memory_order_acquire`: Ensures no reads or writes can be reordered before this operation.
- `memory_order_release`: Guarantees that all writes before this operation are visible to other threads that perform an acquire on the same atomic variable.
- `memory_order_seq_cst`: Establishes a total, global order in which all operations appear to execute.
Here’s a code example demonstrating how different memory ordering affects operations:
#include <atomic>
std::atomic<int> flag{0};
void producer() {
flag.store(1, std::memory_order_release); // Release
}
void consumer() {
while (flag.load(std::memory_order_acquire) == 0) {} // Acquire
}
In this case, the `producer` thread’s writes made before the release will be visible to the `consumer` thread once it performs the acquire operation.
Happens-Before Relationship
The happens-before relationship is a key concept in the C++ memory model, providing a way to determine which operations are guaranteed to be visible to others. If one operation happens before another in the execution order, then the effects of the first operation are visible to the second.
This relationship impacts how we reason about multithreading. Here’s a sample illustrating the happens-before principle:
#include <thread>
#include <iostream>
#include <atomic>
std::atomic<int> x{0}, y{0};
std::atomic<int> z{0};
void write() {
x.store(1, std::memory_order_relaxed);
z.store(1, std::memory_order_relaxed);
}
void read() {
if (z.load(std::memory_order_relaxed) == 1) {
// This read may see the value of x as uninitialized or outdated.
int r1 = y.load(std::memory_order_relaxed);
}
}
Here, the relationship between the operations can lead to unexpected readings of `x` when `z` is read. Understanding such relationships is vital for creating reliable multithreaded applications.
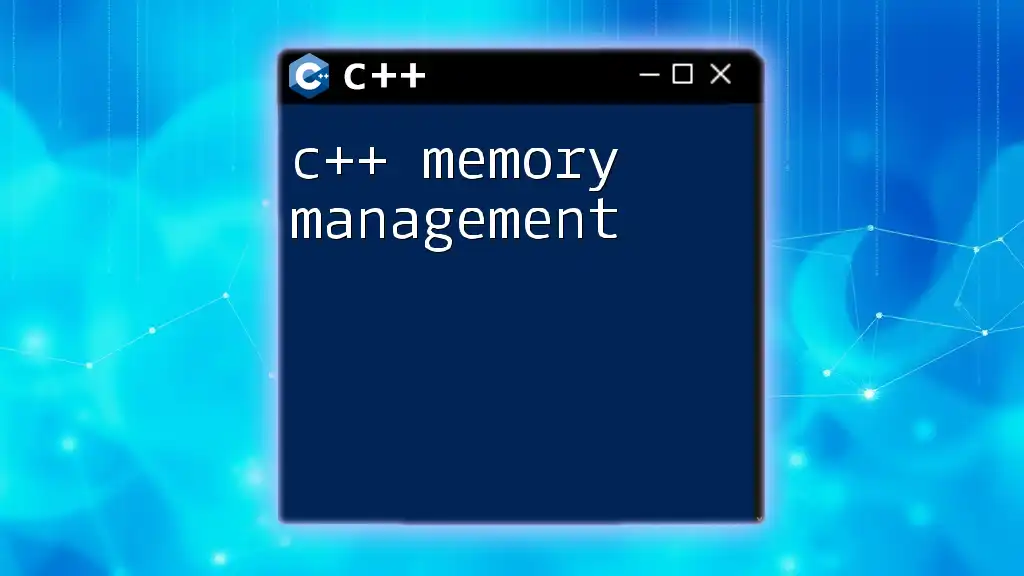
Memory Model Guarantees
Sequential Consistency
Sequential consistency is a strong memory model guarantee that ensures operations appear to execute in a single linear order. This model facilitates reasoning about code behavior, making concurrent programming easier for developers. However, it often comes at the cost of performance due to its stringent requirements.
Relaxed Memory Models
In some scenarios, developers may opt for relaxed memory models, which allow for more extensive optimization at the expense of potential complexity. These models are particularly useful in performance-critical applications but require a deep understanding of the implications. For instance:
#include <thread>
#include <iostream>
#include <atomic>
std::atomic<int> a{0}, b{0};
void thread1() {
a.store(1, std::memory_order_relaxed);
b.store(1, std::memory_order_relaxed);
}
void thread2() {
if (b.load(std::memory_order_relaxed) == 1) {
std::cout << a.load(std::memory_order_relaxed) << std::endl; // May output 0
}
}
In this example, the relaxed model may allow `thread2` to read a value of `0` for `a`, leading to an unexpected behavior due to the lack of a clear happens-before relationship.
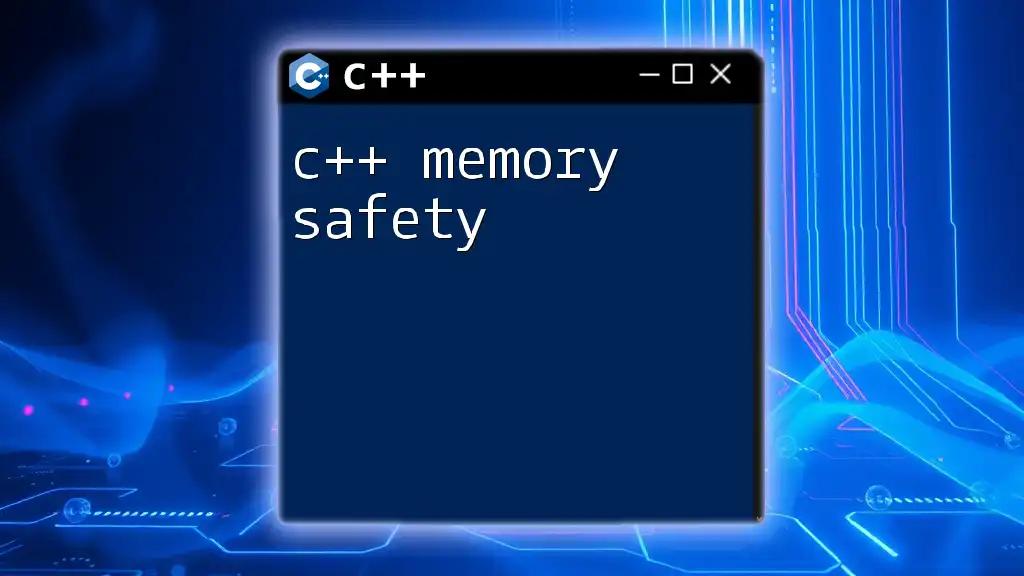
Best Practices for Using C++ Memory Models
To navigate the complexities of the C++ memory model effectively, developers should adhere to a few best practices:
- Always use atomic types when data is shared between threads to prevent race conditions.
- Choose the appropriate memory order based on whether you require strong guarantees or can accept relaxed behavior for performance.
- Be conscious of the happens-before relationships when designing multi-threaded code to avoid unpredictable results.
These practices can significantly enhance both the safety and performance of concurrent applications.
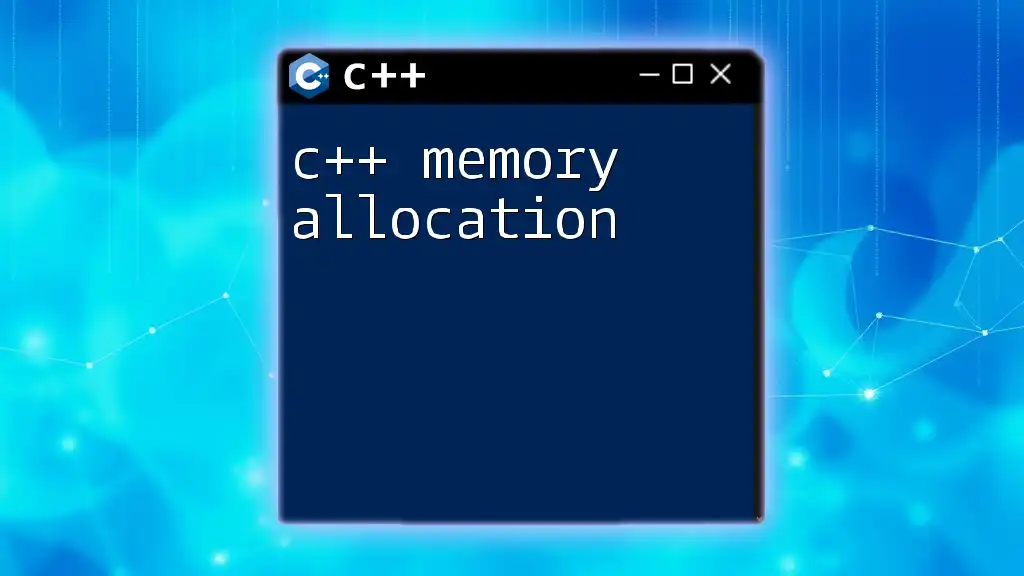
Conclusion
The C++ memory model is a powerful framework that empowers developers to write efficient multi-threaded programs with predictability. By mastering concepts like atomic operations, memory order, and the happens-before relationship, one can create applications that harness the full power of multi-core processors while avoiding common pitfalls associated with concurrent programming. Understanding and applying the principles of the C++ memory model is essential for anyone looking to excel in modern software development.
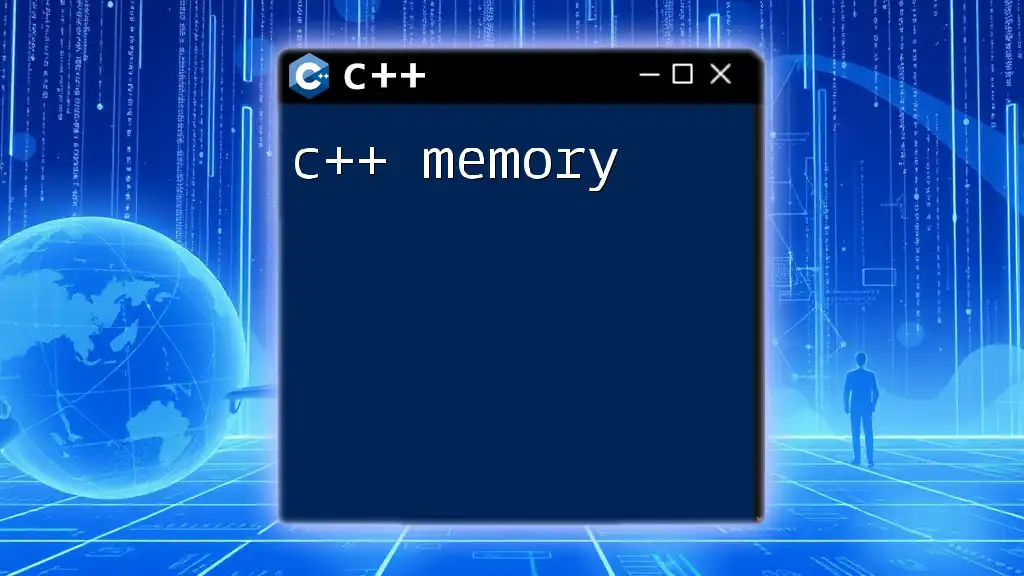
Additional Resources
For those looking to dive deeper into the intricacies of the C++ memory model, consider exploring further readings, community forums, and engaging with C++ enthusiasts to refine your understanding and application of these concepts in your programming endeavors.