A C++ module is a way to group related code and declarations into a single entity that can be imported into other parts of a program, promoting better organization and faster compilation.
Here's a simple example of a C++ module:
// my_module.cppm
export module my_module;
export int add(int a, int b) {
return a + b;
}
To use this module in another file, you would write:
// main.cpp
import my_module;
#include <iostream>
int main() {
std::cout << "Sum: " << add(3, 4) << std::endl;
return 0;
}
Understanding C++ Modules
What are C++ Modules?
C++ modules represent a significant enhancement in the way C++ code is organized, compiled, and utilized. A C++ module is a way to encapsulate functionality, allowing developers to create a clear boundary around code to improve organization and prevent naming collisions. Unlike traditional header files, which can lead to problems like multiple definitions and lengthy compilation times due to dependencies not being properly managed, modules provide a structured and efficient alternative.
Historically, the need for modules arose from the complexities introduced by large codebases, where proper management of dependencies and inclusions became challenging. C++ modules simplify this by defining a clear interface for how code can be used.
Benefits of Using C++ Modules
The shift to using C++ modules brings multiple advantages:
-
Improved Compile Times: Modules reduce the need for repeated parsing of the same code. By precompiling the module interfaces, subsequent compilations of the same modules are noticeably faster.
-
Enhanced Code Organization and Readability: A module encapsulates its functionality and exposes only the necessary parts to the outside world, leading to a more organized codebase. It promotes better coding practices by making interfaces clear.
-
Namespace Management and Encapsulation of Dependencies: Modules help in managing namespaces more effectively. They prevent naming conflicts between different parts of a codebase by containing their definitions within a module's scope.

C++20 Modules Overview
What’s New in C++20?
The C++20 standard introduced numerous features that modernized the language. One of the more revolutionary updates is the introduction of C++ modules. This change aims to address long-standing issues with the traditional include model used in C++. With modular programming, code becomes easier to manage, understand, and scale.
The Role of Modules in C++20
In the context of C++20, modules are designed to replace or enhance the use of the preprocessor directives, primarily for header files. This means that modules not only provide a cleaner approach to including files, but also contribute to better performance and robustness in software development.
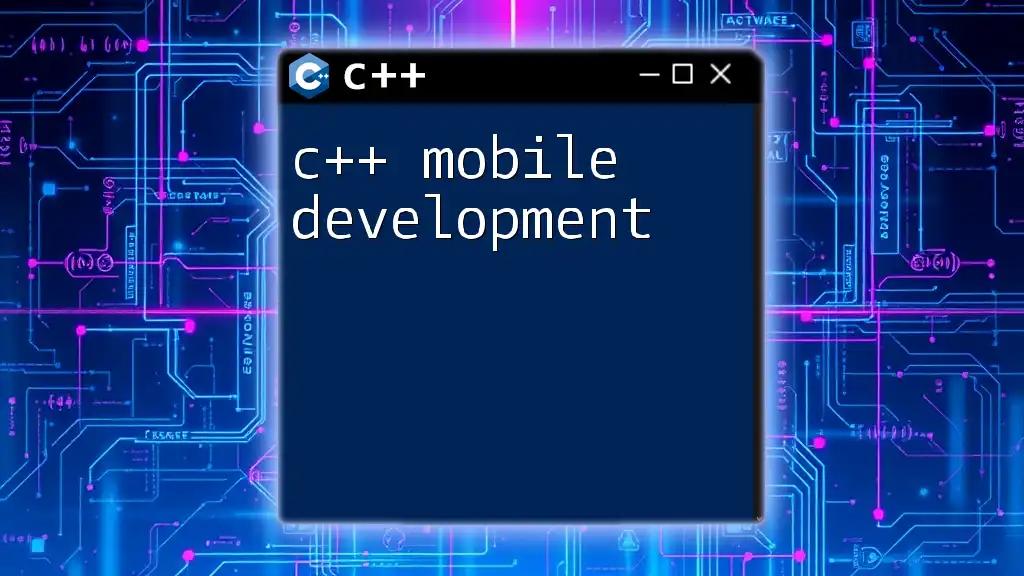
Module Creation and Usage
Basic Structure of a C++ Module
Creating a C++ module follows a simple syntax. A module is declared using the keyword `module`, and its contents are typically separated into interface and implementation sections. Here’s a minimal example of how to define a module:
export module MathOperations; // Declaration
export int add(int a, int b) { return a + b; } // Function definition
In this example, we introduce a module called `MathOperations` that encapsulates an `add` function.
Exporting and Importing Modules
A key feature of modules is the ability to export functions and types so that they can be used in other parts of the program. To use a module, you employ the `import` keyword:
import MathOperations; // Importing the module
int result = add(5, 3); // Using the imported function
This import statement allows us to access the `add` function from the `MathOperations` module directly.
Module Interface vs. Implementation
Normally, a module is divided into an interface and an implementation. The interface contains declarations that are exported, while the implementation contains the actual function definitions. This separation is crucial for managing larger codebases, as it allows for more straightforward modifications and builds.
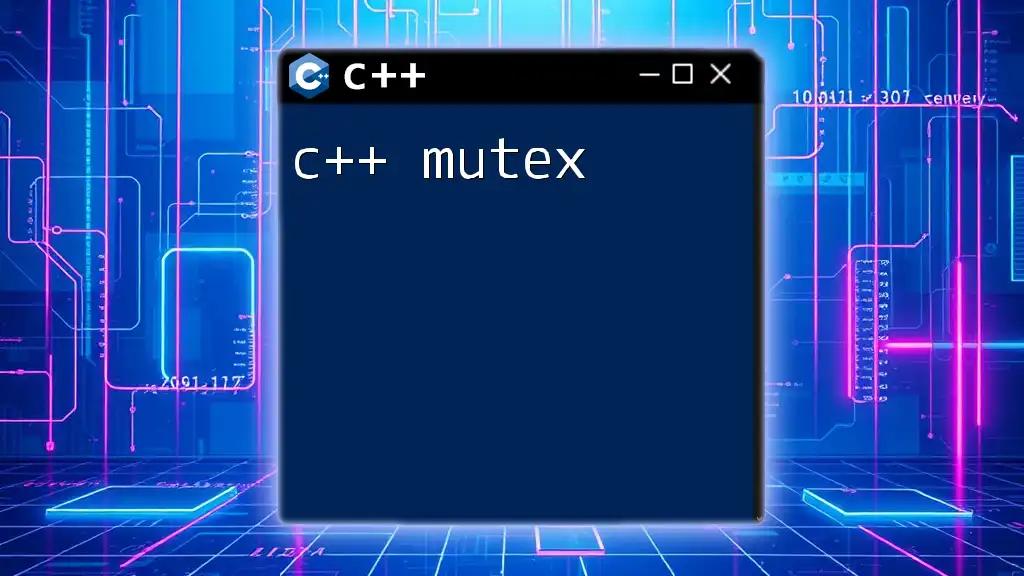
Practical Example of C++ Modules
Building a Simple Project using Modules
Let’s consider a simple project that utilizes C++ modules to manage basic mathematical operations.
To start, here is how you can set up your project directory:
mkdir MathProject
cd MathProject
touch MathOperations.cppm // Creating module interface file
Code Snippet: Setting Up the Project
Now, create the module interface in the `MathOperations.cppm` file:
export module MathOperations; // Declaration
export int add(int a, int b);
export int subtract(int a, int b);
Next, you would implement the module in a separate file, say `MathOperations.ipp`:
module MathOperations; // Implementation file
int add(int a, int b) { return a + b; }
int subtract(int a, int b) { return a - b; }
Example Usage
Now, in your main program file, you would import the module and use the functions defined in your `MathOperations`:
import MathOperations;
int main() {
int sum = add(10, 5);
int difference = subtract(10, 5);
}
This clear separation of declaration and implementation not only streamlines your code, but also solidifies its structure.
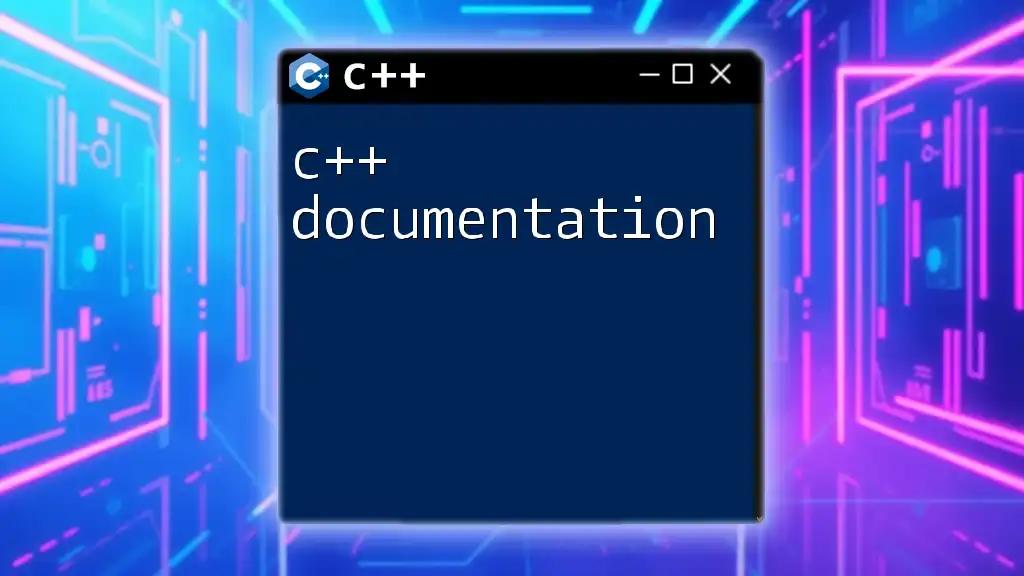
Advanced Module Features
Nested Modules
Nested modules allow for a hierarchy of modules, providing even finer control over the visibility and access of functionality within a more extensive software system. By creating nested modules, you can encapsulate related functionalities together, enhancing modularization.
Module Partitioning
In scenarios where functionality grows, partitioning allows the separation of a module into smaller pieces while remaining parts of a single module. This concept becomes particularly useful for large projects. Using partitions helps manage complexity without losing the benefits of module encapsulation. You can define a partition like this:
module MathOperations:Simple; // Partition declaration

Troubleshooting Common Module Issues
Common Errors and Solutions
Developers new to modules often encounter specific issues such as:
-
Compilation errors related to module imports: Ensure modules are correctly imported and that there are no circular dependencies.
-
Linker errors when using modules: Verify all files are properly linked and that the module definitions have been included during compilation.
Best Practices for Module Usage
To avoid common pitfalls while using C++ modules, adhere to these best practices:
-
Keep the interface and implementation separated to reduce dependencies when changes are made.
-
Maintain clear naming conventions for modules to improve readability and avoid naming conflicts.
-
Document module functionality clearly to aid other developers in understanding the interface without delving deeply into the implementation.

Future of C++ Modules
Trends and Expectations
C++ modules are expected to evolve as the C++ community continues to adopt them. With upcoming features in C++23, we can anticipate further enhancements that will build upon C++20 modules, potentially introducing more refined functionalities.
Conclusion
C++ modules mark a significant evolutionary step for the language, enhancing how developers organize, compile, and manage their code. By adopting modules, programmers can create more maintainable and efficient codebases. Embracing this new feature will undoubtedly foster a smoother development experience and lay the groundwork for the future of programming in C++.

Additional Resources
Recommended Learning Materials
For those looking to deepen their understanding of C++ modules, consider exploring books focused on C++20, enrolling in online courses, and participating in discussions on forums. Engaging with the C++ community can provide valuable insights and resources related to best practices and advanced techniques concerning modules.