C++ mobile development involves using the C++ programming language to create applications for mobile platforms, leveraging libraries such as Qt for cross-platform compatibility.
Here’s a simple code snippet demonstrating how to create a basic mobile application using C++ with the Qt framework:
#include <QApplication>
#include <QPushButton>
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
QPushButton button("Hello, C++ Mobile Development!");
button.resize(300, 100);
button.show();
return app.exec();
}
Why Choose C++ for Mobile Development?
Performance and Efficiency
C++ is revered in the development community for its performance and efficiency. When it comes to mobile applications, where limited resources and battery life are critical factors, C++ shines by producing faster and more optimized code. The language allows developers to control system resources directly, leading to smoother user experiences. For instance, many gaming applications and performance-driven apps utilize C++ due to its ability to handle complex calculations and render graphics efficiently.
Cross-Platform Development
Another significant advantage is the ability to create cross-platform applications. With C++, developers write code once and deploy it to multiple platforms such as iOS and Android. This can dramatically reduce development time and costs when compared to languages that require platform-specific coding, like Java for Android or Swift for iOS.
Access to Native Libraries
C++ offers seamless access to a wide array of native libraries available for different platforms. This allows developers to leverage existing code and functionality, enabling faster development cycles. For example, you might employ native libraries for graphics rendering to enhance your app's performance and visual quality. Here’s a code snippet showing how to link C++ code to native libraries:
extern "C" {
#include "nativeLib.h" // Example header file for a native library
}
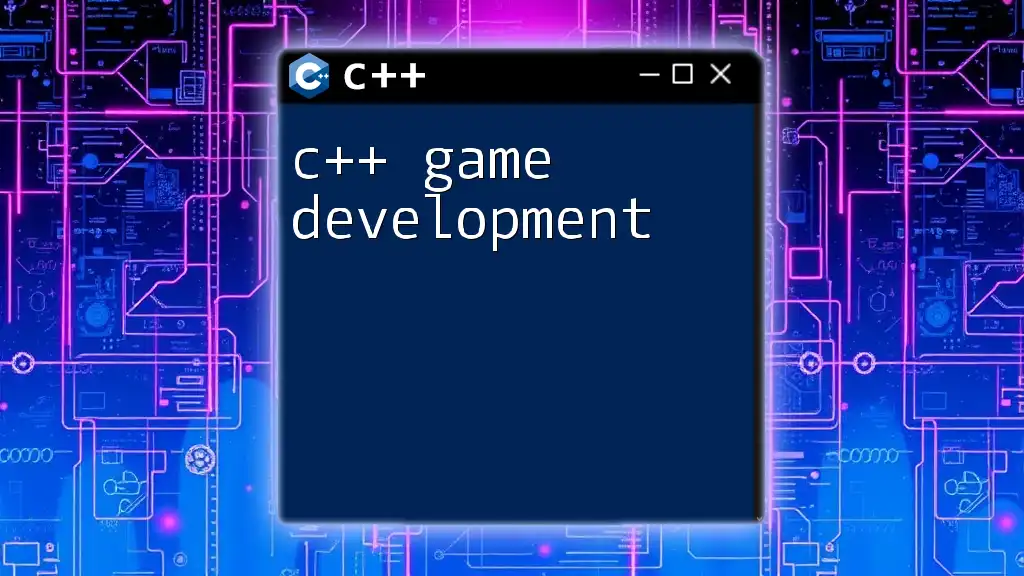
Getting Started with C++ Mobile App Development
Setting Up Your Development Environment
To start developing in C++, you need a robust development environment. Recommended IDEs include Visual Studio, CLion, and Qt Creator. These tools provide integrated debugging, code completion, and project management features that streamline the development process.
You'll also need to install the appropriate C++ compiler (like GCC or Clang) depending on your chosen platform.
Basic C++ Mobile Application Structure
Understanding the basic structure of a C++ mobile application is crucial. Generally, a project includes several key components:
- Source Files: Where your C++ code resides.
- Headers: Declarations of functions and classes.
- Resources: Images, icons, and other assets.
Here’s a simple code snippet illustrating the basic structure of a `main.cpp` file:
#include <iostream>
#include "App.h" // Your application header
int main() {
App myApp; // Create your app instance
myApp.run(); // Start the application
return 0;
}
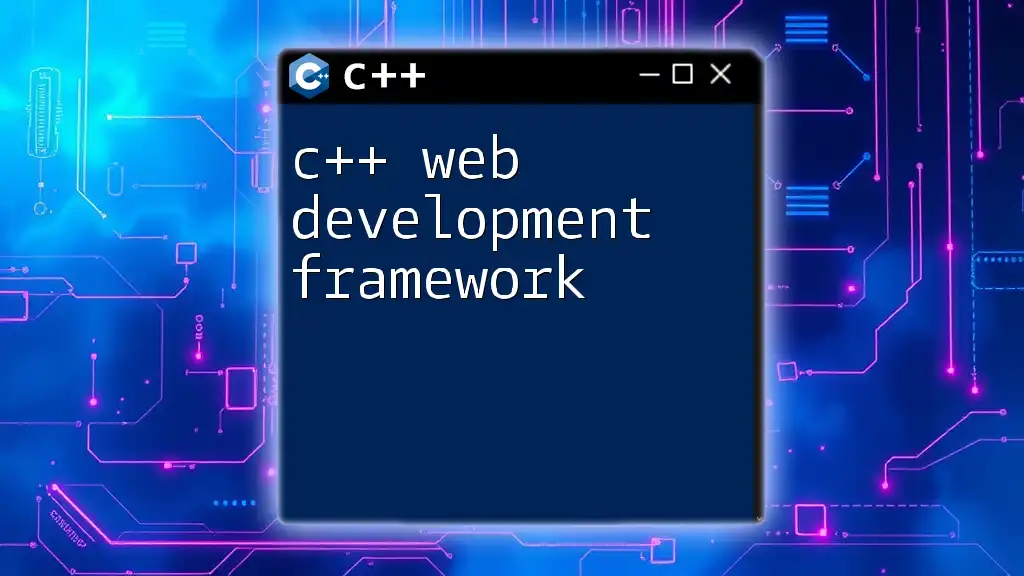
Mobile Development Frameworks that Support C++
Introduction to Mobile Frameworks
Several frameworks bolster C++ mobile development, each offering unique features to enhance building applications. Understanding these frameworks can significantly ease the development process.
C++ Frameworks
Qt for Mobile Development
Qt is one of the most popular frameworks for mobile development with C++. It enables developers to create cross-platform applications with stunning UIs. Qt provides a set of tools and libraries that expedite development while preserving code maintainability.
Here’s a code snippet to create a simple Qt application:
#include <QApplication>
#include <QPushButton>
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
QPushButton button("Hello, World!");
button.resize(200, 100);
button.show();
return app.exec();
}
Cocos2d-x for Game Development
For those focused on game development, Cocos2d-x is an excellent framework. It specializes in building 2D games and ensures high performance on mobile devices. The framework is highly portable and offers a rich set of features, such as animations and physics engines.
Below is a basic setup for a Cocos2d-x game:
#include "cocos2d.h"
USING_NS_CC;
class HelloWorld : public Scene {
public:
virtual bool init() {
auto label = Label::createWithTTF("Hello Cocos2d-x", "fonts/Marker Felt.ttf", 24);
label->setPosition(Vec2(Director::getInstance()->getVisibleSize() / 2));
this->addChild(label);
return true;
}
};
int main(int argc, char** argv) {
// Initialize the application
return Application::getInstance()->run();
}
Unreal Engine
Unreal Engine stands out in providing a robust environment for both C++ and game development. With its powerful graphics capabilities and extensive community support, it's suitable for developing high-end games and graphics-intensive applications.
Key Concepts in C++ Mobile Development
Object-Oriented Programming in C++
C++ is an object-oriented programming language, making it essential to embrace OOP principles when developing. Classes allow you to create objects that embody data and behavior, which can simplify complex applications.
For example, you can define a simple class for a mobile app like:
class Task {
public:
std::string taskName;
bool isCompleted;
Task(std::string name) : taskName(name), isCompleted(false) {}
void complete() {
isCompleted = true;
}
};
Memory Management
In mobile development, managing memory efficiently is crucial. C++ offers fine-grained control over memory allocation and deallocation via pointers and dynamic memory management techniques. This can help your application use memory wisely, ensuring that it runs smoothly. Here’s how to use smart pointers:
#include <memory>
class TaskManager {
public:
void addTask(std::unique_ptr<Task> task) {
// Add task to the task manager
}
};
Concurrency and Multithreading
Mobile applications must often deal with multiple tasks at once. C++ provides threading capabilities to create responsive applications. You can create new threads as follows:
#include <thread>
void backgroundTask() {
// Perform background operation
}
int main() {
std::thread t(backgroundTask);
t.join(); // Wait for the thread to finish
return 0;
}
User Interface Design
Creating an engaging user interface using C++ often involves using frameworks like Qt or Cocos2d-x that handle UI elements. Integrating responsive design principles ensures users have a seamless experience. A simple UI setup within Qt can look like this:
QPushButton *myButton = new QPushButton("Click Me", this);
connect(myButton, &QPushButton::clicked, this, &MyClass::onButtonClick);
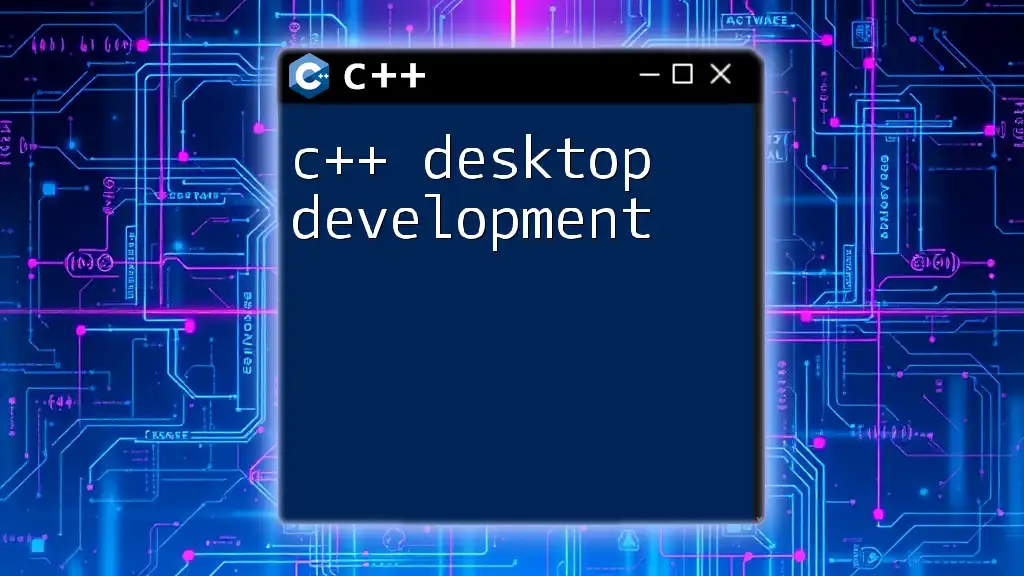
Building a Simple C++ Mobile App
Step-by-Step Walkthrough
To put your knowledge into practice, let's build a simple "To-Do List" application as an example. This project will contain basic functionalities, such as adding and removing tasks.
Setting up the Project
Start by creating a new project in Qt Creator or another preferred IDE designed for mobile development.
Designing the UI
Utilize a design tool to create your user interface. You can define the main window and necessary buttons such as 'Add Task' and 'Remove Task.'
Implementing Core Functionalities
Add functionality to add and remove tasks from your list. For instance, you may define a method to handle adding tasks:
void MyClass::addTask(const std::string &taskName) {
tasks.push_back(Task(taskName)); // Add a new task
}
Testing and Debugging the App
It’s crucial to regularly test your app on different devices to catch and resolve bugs early. Both emulators and real devices offer insight into how your application performs across various platforms.
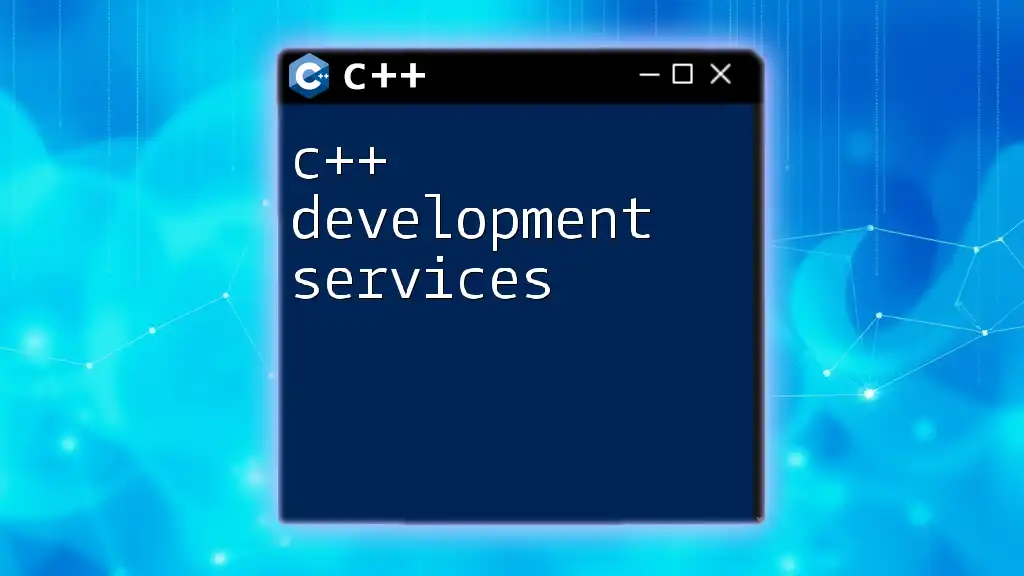
Deploying Your C++ Mobile Application
Platforms for Deployment
When it's time to deploy, you'll typically target platforms such as Android and iOS. Each platform has its requirements, so understanding how to build for these environments is critical. For instance, Android uses the Android NDK, while iOS has Xcode.
App Store Guidelines
Familiarizing yourself with app submission processes is vital. Each app store has guidelines regarding app functionality, security, and performance. Addressing these standards improves the likelihood of successful submissions.
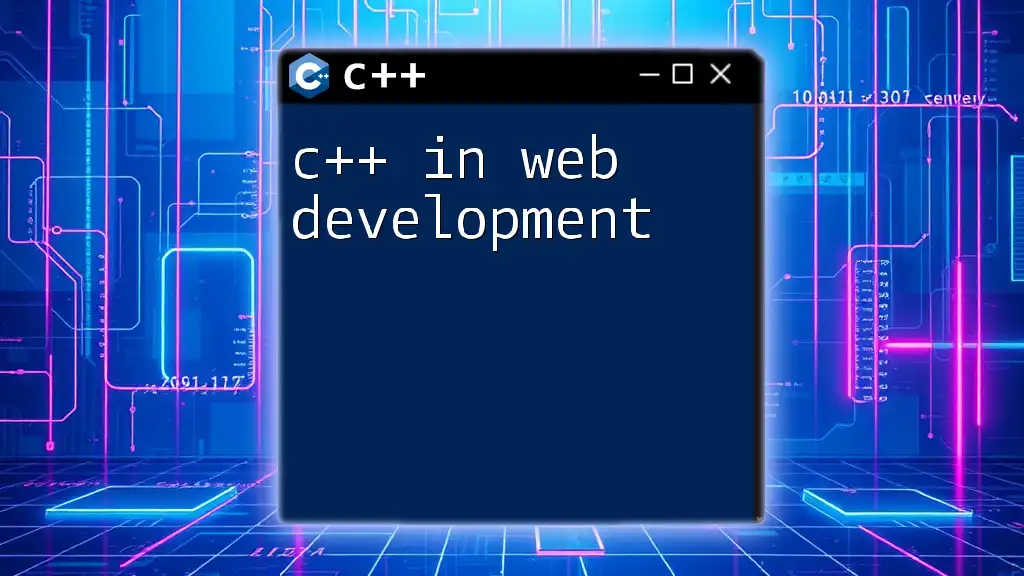
Challenges in C++ Mobile Development
Common Issues and Resolutions
Developers may face several challenges, such as compatibility issues across platforms or dealing with performance bottlenecks. It’s important to test extensively and use profiling tools to identify problem areas in your application.
Maintenance and Updates
Maintaining your application involves regularly updating it for performance improvements and adapting to new OS versions. Establish a feedback loop from users to guide the update process effectively.
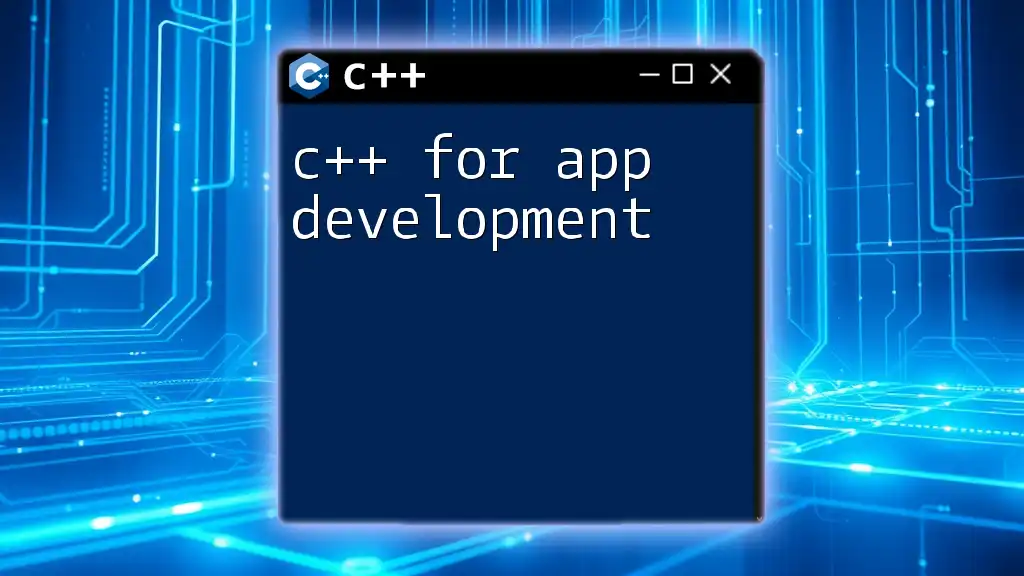
Future Trends in C++ Mobile Development
Emerging Technologies
The future of mobile development is focused on incorporating AI and machine learning into applications. C++ is well-positioned to leverage algorithms and models for performance-intensive tasks.
C++20 and Beyond
The introduction of new features in C++20, such as modules and coroutines, enhances performance and improves code organization. Familiarizing yourself with these features can significantly benefit your mobile applications.
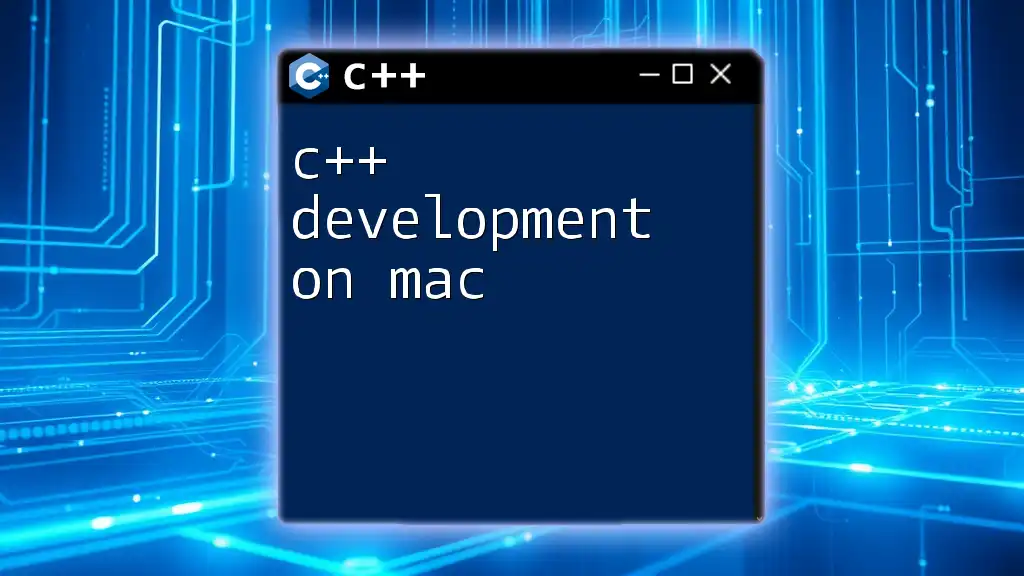
Conclusion
C++ is a powerful tool in mobile development, offering unmatched performance and versatility. By understanding frameworks, embracing object-oriented programming, and mastering best practices, you can create efficient and engaging mobile applications.
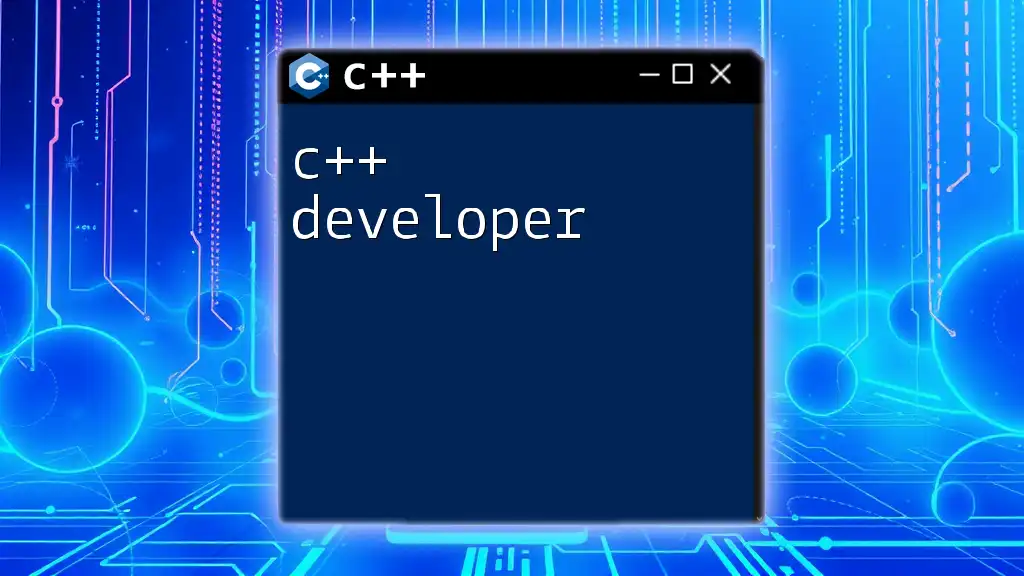
Call to Action
If you're eager to dive deeper into C++ mobile development, consider enrolling in our upcoming courses. Share your experiences with C++ or any projects you've created—community collaboration fosters learning and innovation!