A C++ backend developer specializes in building server-side applications using C++, focusing on efficient data processing and system-level functionality.
Here's a simple example of a C++ command that demonstrates a basic backend operation, such as reading user input and printing a response:
#include <iostream>
int main() {
std::string userInput;
std::cout << "Enter your name: ";
std::getline(std::cin, userInput);
std::cout << "Hello, " << userInput << "!" << std::endl;
return 0;
}
What is Backend Development?
Backend development is the part of web development that deals with server-side operations. It is responsible for managing database interactions, server logic, and application programming interfaces (APIs). Essentially, while frontend developers focus on the user interface and experience, backend developers ensure that the server, application, and database communicate seamlessly.
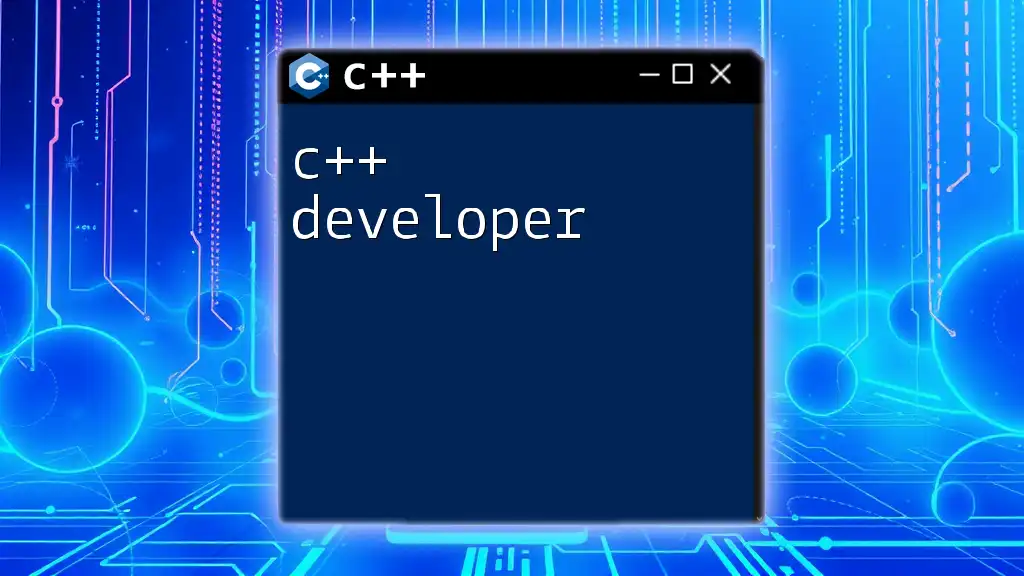
Why Choose C++ for Backend?
Choosing C++ for backend development comes with several significant advantages. Performance is one of the most critical reasons—C++ allows developers to write code that runs extremely quickly, which is crucial for applications that require high efficiency and speed. Additionally, C++ has a wide array of libraries specifically designed for various functionalities, making it a robust choice for building complex applications.
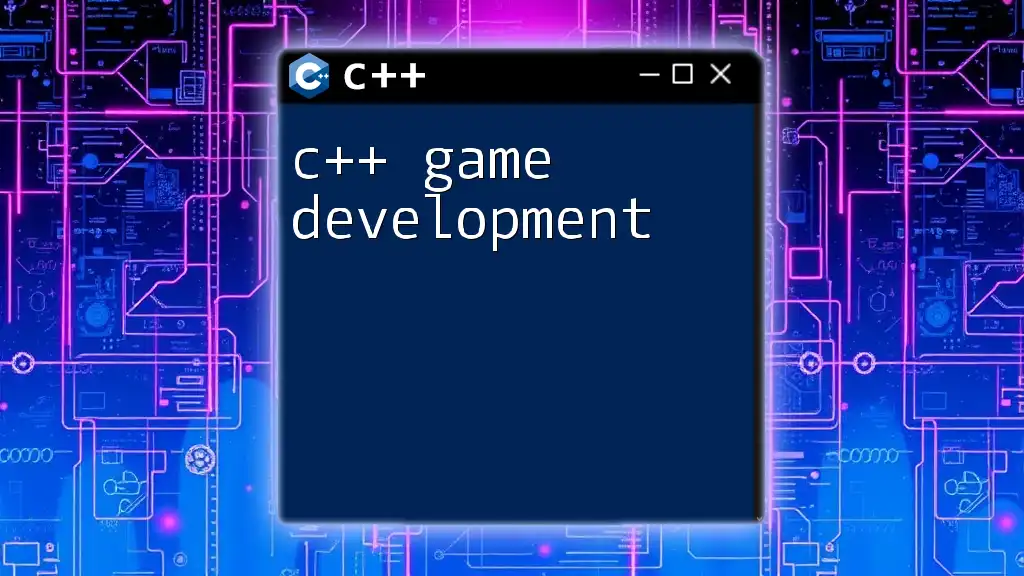
Essential Skills for C++ Backend Developers
Proficiency in C++ Programming
To become a successful C++ backend developer, a strong grasp of C++ syntax and foundational concepts is indispensable. This includes understanding variables, data types, loops, and conditional statements.
Key features of C++ relevant to backend development include:
- Memory Management: Understanding pointers, references, and dynamic memory allocation.
- Standard Template Library (STL): Utilizing data structures like vectors, lists, and maps, which can significantly reduce development time.
Understanding Object-Oriented Programming (OOP)
C++ is an object-oriented programming language. Thus, an understanding of OOP principles is crucial for a backend developer. The four core principles of OOP are:
- Encapsulation: Bundling data with the methods that operate on it, restricting access to some components.
- Inheritance: Allowing a new class to inherit properties and behavior from an existing class.
- Polymorphism: Enabling objects to be treated as instances of their parent class, enhancing flexibility and reusability.
Familiarity with C++ Standard Library
The C++ Standard Library is a powerful feature set that includes data structures, algorithms, and iterators. Familiarity with it can drastically reduce development time.
Example of using STL for data structures:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (int num : numbers) {
std::cout << num << " ";
}
return 0;
}
Knowledge of Databases
A C++ backend developer must understand how to interact with databases, both relational and non-relational. Familiarity with SQL databases such as MySQL or PostgreSQL is vital.
Code Snippet: Connecting to a SQL database using C++:
#include <mysql/mysql.h>
int main() {
MYSQL *conn;
conn = mysql_init(NULL);
if (conn == NULL) {
std::cerr << "mysql_init() failed\n";
return EXIT_FAILURE;
}
// Connect to the database
if (mysql_real_connect(conn, "host", "user", "password", "db_name", 0, NULL, 0) == NULL) {
std::cerr << "mysql_real_connect() failed\n";
mysql_close(conn);
return EXIT_FAILURE;
}
// Perform database operations here...
mysql_close(conn);
return EXIT_SUCCESS;
}
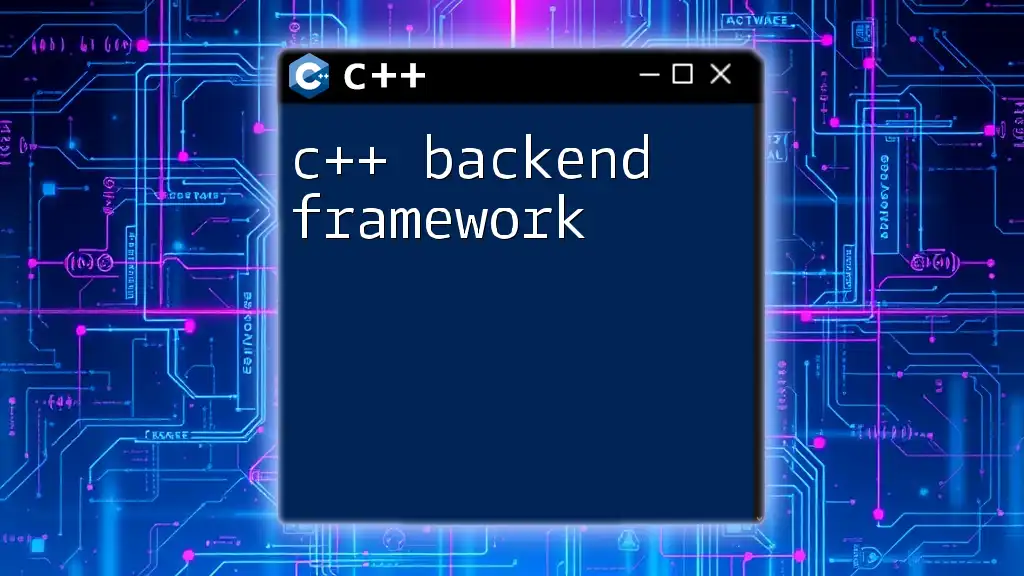
Tools and Technologies for C++ Backend Development
Integrated Development Environments (IDEs)
The choice of IDE can significantly influence productivity. Popular IDEs for C++ development include Visual Studio and CLion. These environments provide features like intelligent code completion, debugging tools, and project management capabilities that enhance development efficiency.
Build Systems and Package Managers
Using build systems and package managers optimizes project management. CMake is widely used in C++ projects for automating the build process. It simplifies the creation of makefiles and project files.
Example of a simple CMake configuration for a C++ project:
cmake_minimum_required(VERSION 3.10)
project(MyProject)
set(CMAKE_CXX_STANDARD 17)
add_executable(MyExecutable main.cpp)
vcpkg is a powerful package manager that simplifies the management of C++ libraries, providing easy access to thousands of libraries.
Version Control Systems
Using a version control system like Git is crucial for every C++ backend developer. It allows collaborative development, tracking changes in code, and managing versions effectively.
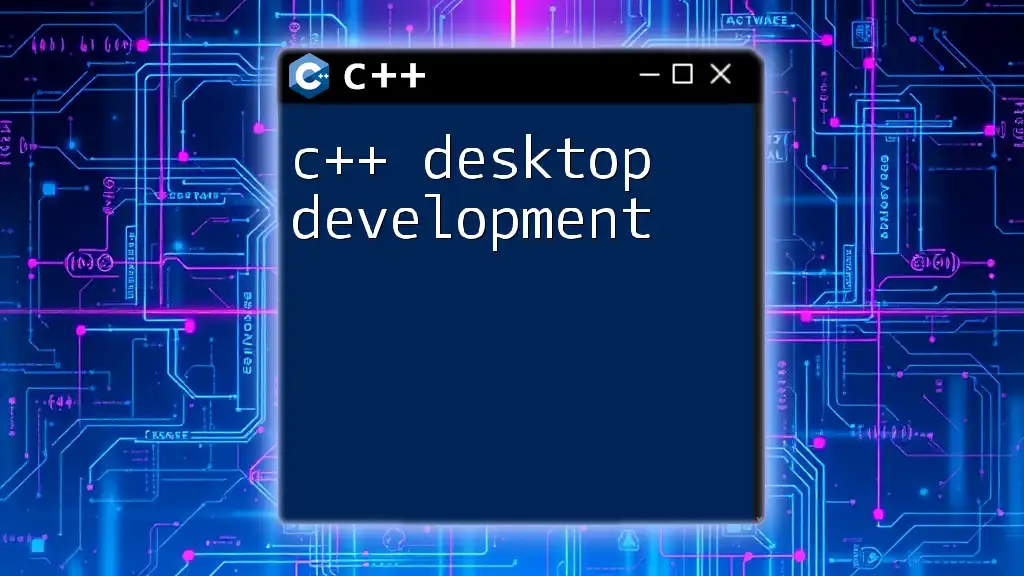
C++ Frameworks for Backend Development
Introduction to C++ Web Frameworks
Frameworks streamline the development of web applications by providing pre-built modules and functionalities. Among the many frameworks available, Pistache, Crow, and Wt stand out for C++ backend development.
Deep Dive into Crow Framework
Crow is an excellent choice for building REST APIs in C++. It is lightweight and easy to use, providing features like routing, templating, and JSON serialization.
Code Snippet: Creating a simple REST API with Crow:
#include "crow_all.h"
int main() {
crow::SimpleApp app;
CROW_ROUTE(app, "/hello/<string>")
([](const std::string &name) {
return "Hello, " + name;
});
app.port(18080).multithreaded().run();
}
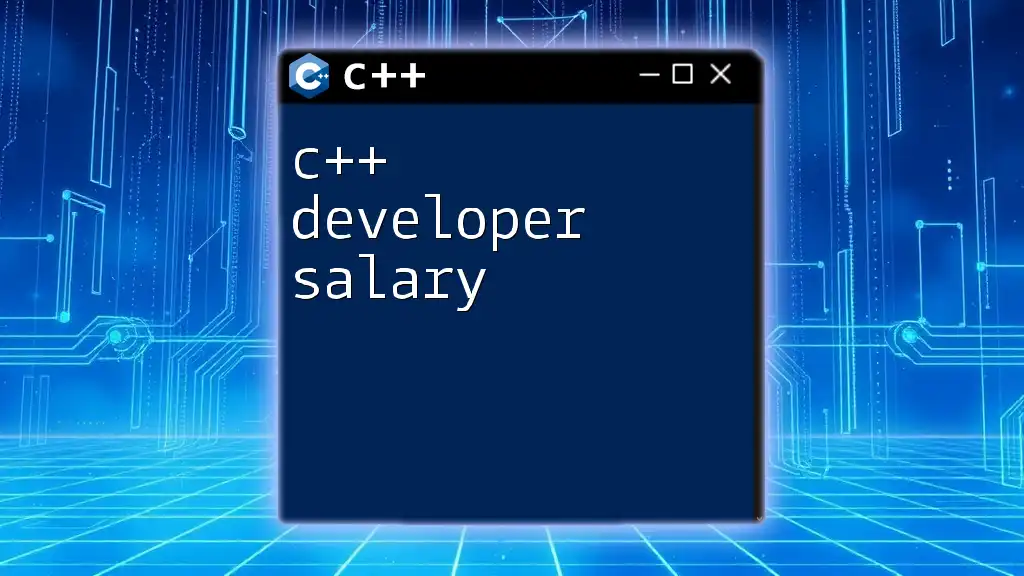
Best Practices for C++ Backend Development
Code Quality and Maintainability
Maintaining high code quality is crucial in backend development. Follow coding conventions and adopt a clean code philosophy, which promotes readability and maintainability. Tools like linters can help in this regard.
Error Handling and Debugging Techniques
Effective error handling is essential. C++ supports exception handling, which should be incorporated into your coding practices. Additionally, mastering debugging tools available in your IDE can streamline fixing issues during development.
Performance Optimization
C++ provides powerful features for optimization. Regular profiling of your code aids in identifying bottlenecks. Techniques like using appropriate data structures, avoiding unnecessary copies, and optimizing loops can boost performance significantly.
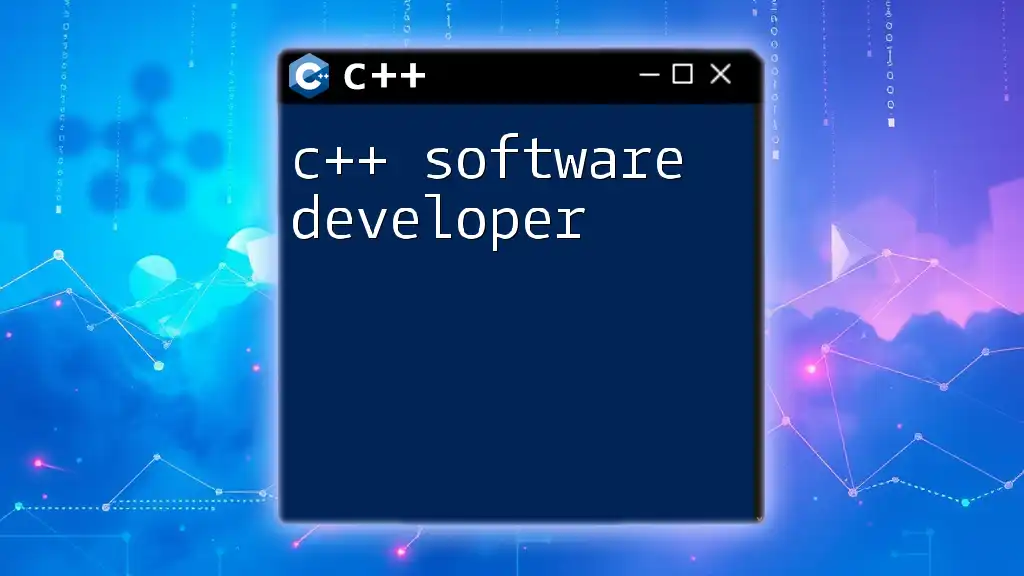
Real-World Applications of C++ Backend Development
Case Studies
Several companies use C++ for backend development due to its speed and efficiency. Industries such as gaming, finance, and telecommunications make extensive use of C++ to handle mission-critical applications.
Emerging Trends and Future
As technology advances, the demand for high-performance applications is growing. With cloud computing and IoT on the rise, C++ backend developers are in a strategic position to utilize their skills in building productive systems and solutions.
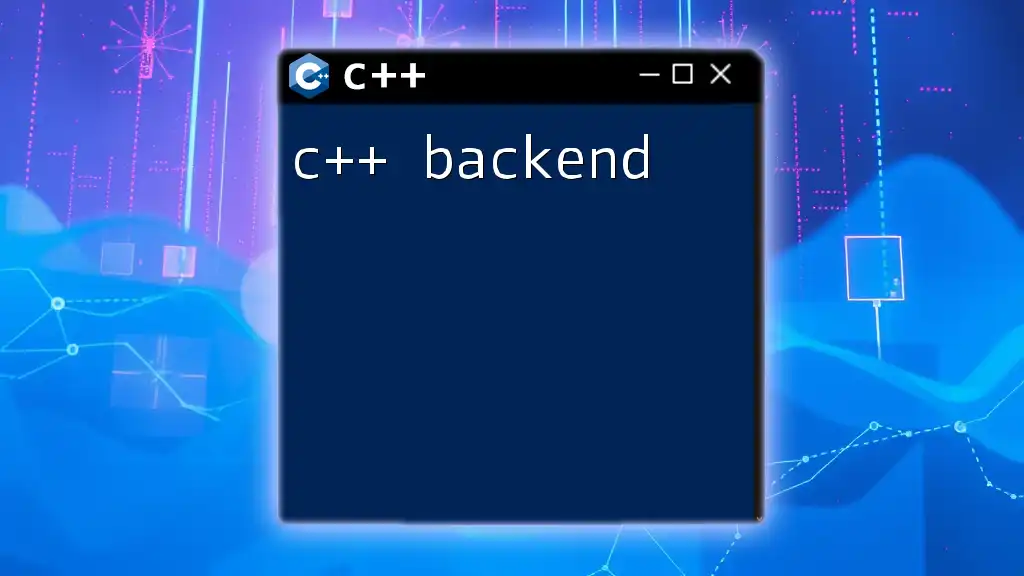
Resources for Continuous Learning
Books and Tutorials
Reading materials like "C++ Primer" and "Effective Modern C++" are excellent resources for deepening your understanding of C++. Online tutorials from platforms like Codecademy help build hands-on experience.
Online Courses and Coding Bootcamps
Platforms like Coursera and edX offer web development bootcamps focusing on C++. These programs often provide project-based learning, which can significantly enhance your understanding and abilities.
Communities and Forums
Engaging with communities such as Stack Overflow, Reddit’s C++ subreddit, and GitHub allows you to connect with fellow developers, share knowledge, and seek help when needed.
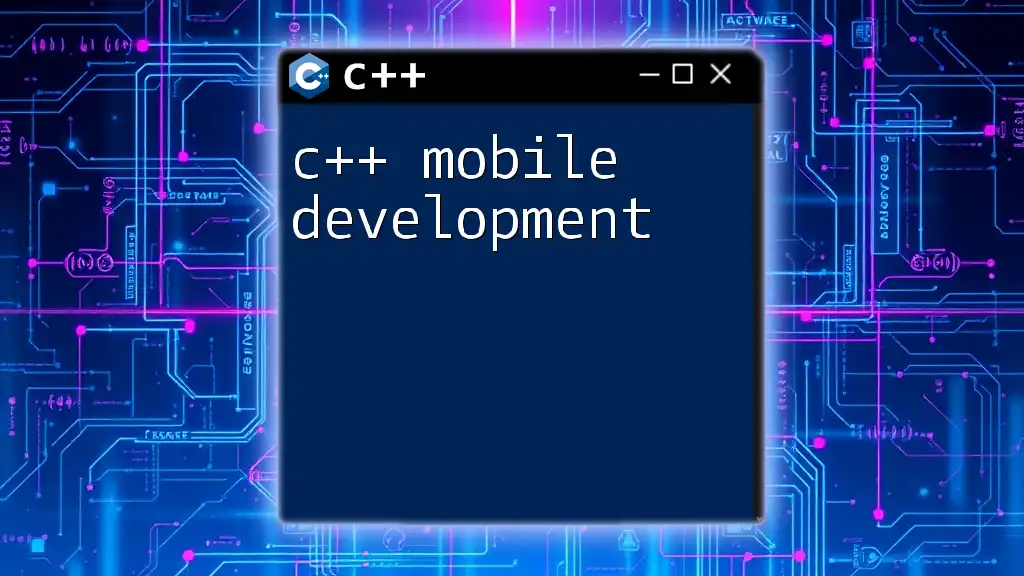
Conclusion
In summary, becoming a C++ backend developer requires a strong foundation in C++ programming, an understanding of OOP, and familiarity with tools and frameworks. Adopting best practices can lead to high-quality, efficient code. With the current demand for skilled C++ developers, there are endless opportunities to explore in various industries. Now is the ideal time to start your journey in C++ backend development, leveraging your skills and knowledge to build impactful applications.