A C++ backend framework provides a structured environment for developing server-side applications by simplifying complex tasks such as handling requests, managing databases, and routing.
Here's a simple example of a C++ web server using the Crow framework:
#include "crow_all.h"
int main()
{
crow::SimpleApp app;
CROW_ROUTE(app, "/")([](){
return "Hello, C++ Backend!";
});
app.port(18080).multithreaded().run();
}
Why Choose C++ for Backend Development?
C++ is renowned for its performance and efficiency, making it an attractive choice for backend development. As a statically typed language, C++ allows developers to catch many errors at compile-time, enhancing the reliability of applications.
Performance and Efficiency
C++ is a compiled language that translates code directly into machine instructions, resulting in extremely fast execution times. This is particularly beneficial for backend systems, which often manage large volumes of data and requests.
System-Level Programming
C++ provides developers with low-level access to system resources and memory management. This capability allows for fine-tuned optimization when working with performance-critical applications.
Strong Typing and Compile-Time Checks
The strong typing of C++ helps prevent many types of bugs. The compiler can catch errors before the code runs, reducing runtime exceptions and contributing to a smoother user experience.
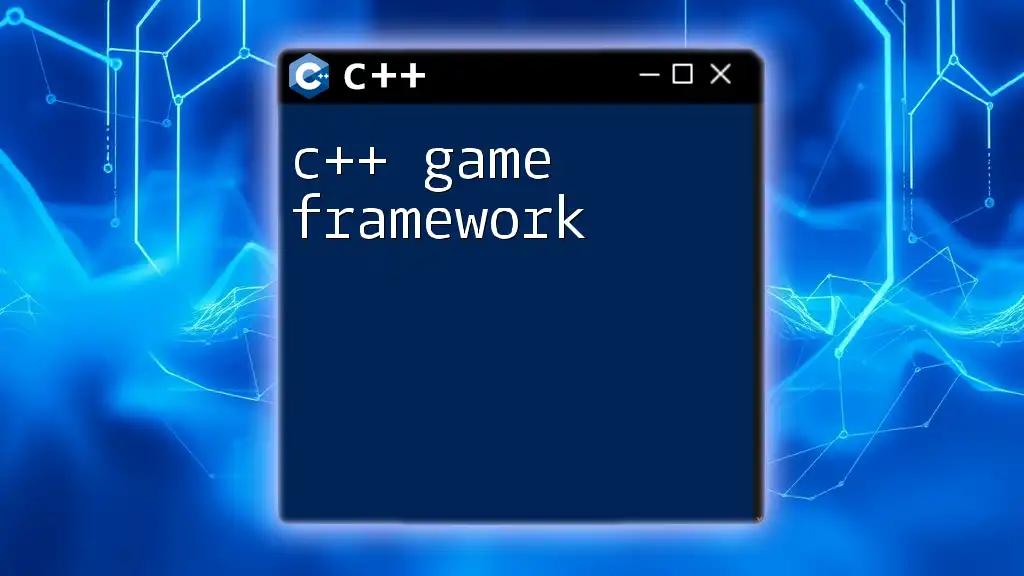
Overview of Popular C++ Backend Frameworks
Understanding the available C++ backend frameworks is crucial for selecting the right one for your project. Below is a brief overview of some popular frameworks.
Boost.Beast
Boost.Beast provides HTTP and WebSocket functionalities built on top of Boost.Asio.
- When to Use: Ideal for projects requiring a robust network protocol implementation.
Example: A simple HTTP server using Boost.Beast might look like:
#include <boost/beast.hpp>
#include <boost/asio.hpp>
using namespace boost::beast;
using namespace boost::asio;
void http_server() {
io_context io;
// Server code goes here
}
cpprestsdk (C++ REST SDK)
The C++ REST SDK, also known as cpprestsdk, is designed for building RESTful applications.
- Features: It supports URI parsing, JSON serialization, and asynchronous operations.
Example: Creating a simple REST endpoint might look like this:
#include <cpprest/http_listener.h>
void handle_get() {
// Handle HTTP GET requests
}
int main() {
web::http::experimental::listener::http_listener listener(U("http://localhost:8080"));
listener.support(web::http::methods::GET, handle_get);
listener
.open()
.then([](){ std::wcout << L"Starting to listen at: http://localhost:8080\n"; })
.wait();
}
Wt (pronounced "witty")
Wt is a web toolkit for creating interactive web applications in C++.
- Unique Features: It provides excellent support for server-side rendering and a rich user interface.
Example: A basic Wt application can be set up like so:
#include <Wt/WApplication.h>
#include <Wt/WText.h>
class MyApp : public Wt::WApplication {
public:
MyApp(const Wt::WEnvironment& env) : Wt::WApplication(env) {
setTitle("Hello Wt");
root()->addWidget(std::make_unique<Wt::WText>("Hello, World!"));
}
};
int main(int argc, char** argv) {
return Wt::WRun(argc, argv, &MyApp);
}
Crow
Crow is a microframework for C++ that is lightweight and easy to use.
- Strengths: It offers high performance and simplicity, making it a good choice for small to medium applications.
Example: Creating a basic web application with Crow looks like this:
#include "crow_all.h"
int main() {
crow::SimpleApp app;
CROW_ROUTE(app, "/")([](){
return "Hello Crow!";
});
app.port(18080).multithreaded().run();
}
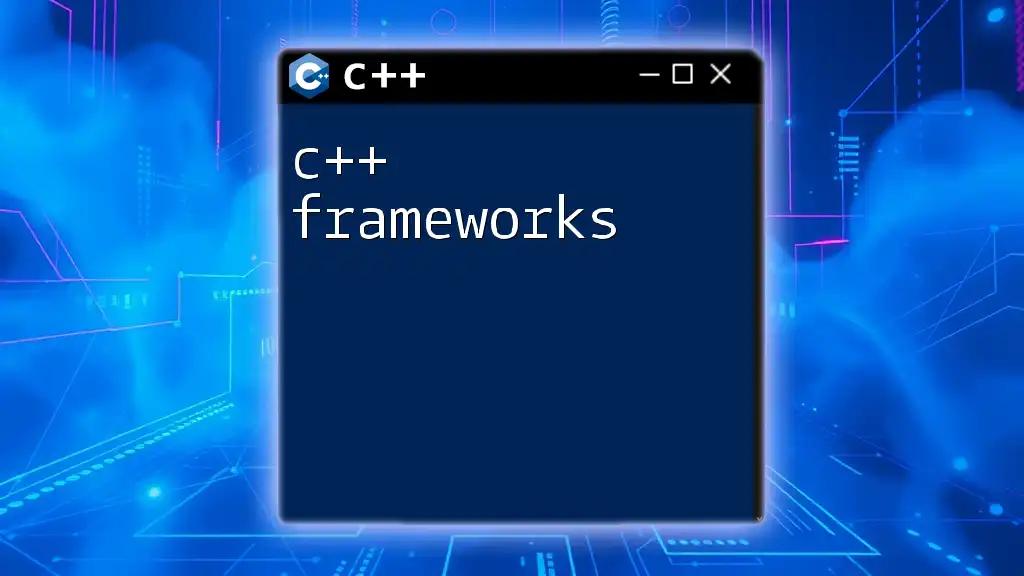
Key Features of C++ Backend Frameworks
Asynchronous Programming
Asynchronous programming is essential in today's web applications to improve performance and resource management.
To handle asynchronous requests, C++ frameworks often use callback mechanisms or futures. Here's an example of a simple async task:
#include <iostream>
#include <future>
void async_task() {
std::cout << "This runs asynchronously!" << std::endl;
}
int main() {
auto future = std::async(std::launch::async, async_task);
future.get(); // Wait for the async_task to complete
}
RESTful API Development
Creating a RESTful API in C++ involves defining routes, controllers, and handling responses.
A RESTful service typically adheres to certain architectural principles, such as stateless communication. Here's how you might set up a basic API endpoint:
CROW_ROUTE(app, "/api/data")([](){
return R"({"key": "value"})"; // JSON response
});
Database Integration
Database integration is fundamental to backend development. Common databases used with C++ include SQLite, MySQL, and PostgreSQL.
Using an Object-Relational Mapping (ORM) library, such as ODB, can simplify database interactions. Here's an example snippet for establishing a database connection:
#include <odb/sqlite/database.hxx>
odb::sqlite::database db("my_database.db", SQLITE_OPEN_READWRITE | SQLITE_OPEN_CREATE);
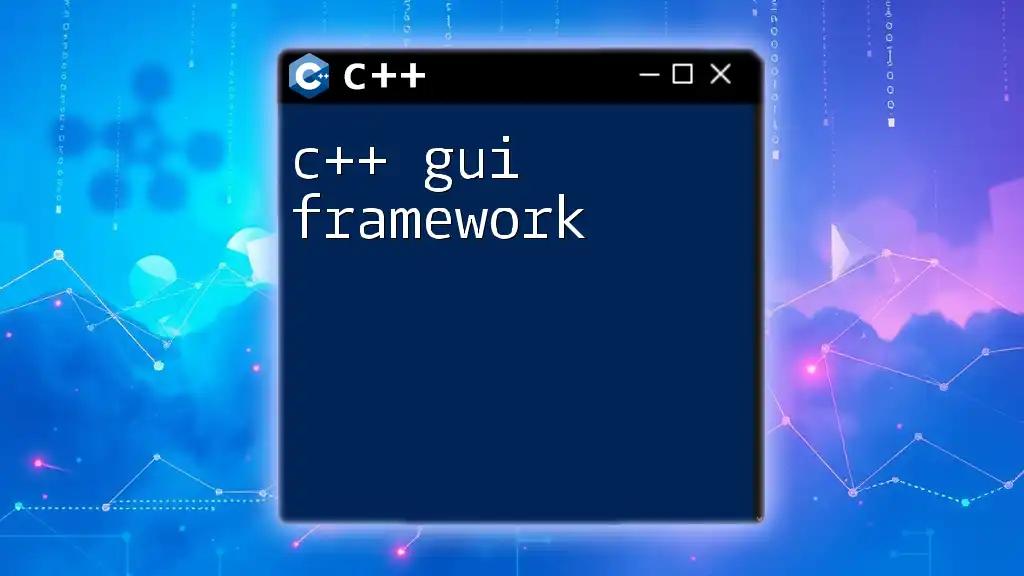
Best Practices for C++ Backend Development
Proper Memory Management
C++ gives developers the power of manual memory management, which can lead to performance gains if done correctly. Using smart pointers like `std::shared_ptr` and `std::unique_ptr` can help manage memory effectively and prevent leaks.
Modular Code Structure
Organizing your code into a modular structure is crucial for maintainability. A sample directory structure for a C++ backend application may look like this:
/myapp
/src
/include
/lib
/tests
Error Handling and Logging
Robust error handling is essential in any backend system. C++ provides mechanisms such as exceptions for capturing errors.
Integrating logging libraries, such as spdlog, can help in tracing activities and errors. Here's a simple error handling example:
try {
// Some code that may throw
} catch (const std::exception &e) {
spdlog::error("Exception: {}", e.what());
}

Performance Optimization Techniques in C++
Profiling and Measuring Performance
Profiling your C++ application is vital for identifying bottlenecks. Tools like Valgrind and gprof can help you gather performance metrics, allowing you to refine your application for better performance.
Multi-threading and Concurrency
C++ supports multi-threading, allowing developers to leverage multi-core processors for concurrent processing. Here's a simple example using `std::thread`:
#include <thread>
#include <iostream>
void thread_function() {
std::cout << "Running in a separate thread." << std::endl;
}
int main() {
std::thread my_thread(thread_function);
my_thread.join(); // Wait for the thread to finish
}

Conclusion
C++ backend frameworks offer numerous advantages, including high performance, efficient resource management, and flexibility. As you explore these frameworks, consider the requirements of your project and choose accordingly. Building efficient, robust applications requires a solid understanding of the language and the frameworks at hand, but the rewards are well worth the effort.

Additional Resources
For further learning, consider diving into recommended C++ backend development books, online courses, and engaging with communities and forums that cater to C++ developers. Your journey in mastering a C++ backend framework is just beginning, and there are plenty of resources to help mitigate the challenges ahead.
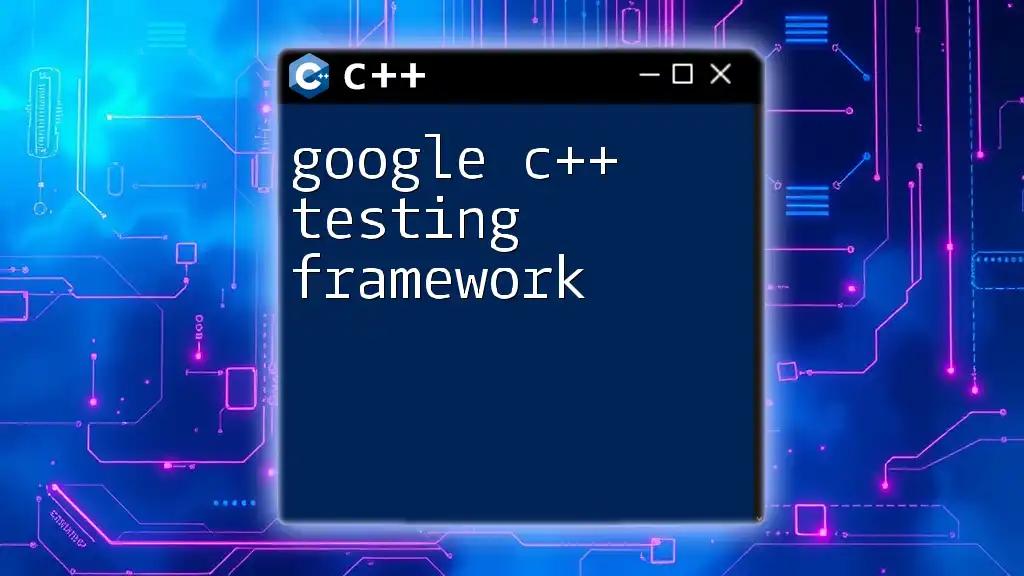
Final Thoughts
The future of C++ in backend development looks promising, with increasing interest in its capabilities to handle high-performance applications. As technologies evolve, so too will the frameworks that support them, making it essential for developers to keep abreast of trends and advancements in the field.