The ACE (Adaptive Communicative Environment) framework in C++ provides a powerful toolkit for building concurrent and networked applications with ease and efficiency.
Here’s a simple example of using ACE to set up a basic TCP server that listens for incoming connections:
#include <ace/ACE.h>
#include <ace/Service_Config.h>
#include <ace/SOCK_Acceptor.h>
#include <ace/SOCK_Stream.h>
#include <iostream>
int main() {
ACE_INET_Addr addr(12345); // Listening on port 12345
ACE_SOCK_Acceptor acceptor(addr);
ACE_SOCK_Stream clientStream;
std::cout << "Server is running and waiting for connections..." << std::endl;
if (acceptor.accept(clientStream) == -1) {
std::cerr << "Accept failed" << std::endl;
return 1;
}
std::cout << "Accepted a connection!" << std::endl;
// Close the streams
clientStream.close();
acceptor.close();
return 0;
}
Understanding the Ace Framework
What is Ace Framework?
The Ace Framework is a powerful and flexible C++ framework designed for networked and real-time applications. It provides robust support for handling asynchronous operations, which allows developers to write high-performance applications without deep knowledge of the underlying asynchronous programming models. Originally developed to support distributed application development, the framework offers both high-level abstractions and low-level interfaces for fine-tuning performance.
Key Features of Ace Framework
-
Asynchronous programming: One of the standout features of the Ace Framework is its support for asynchronous programming. By utilizing event-driven programming models, developers can handle multiple tasks concurrently without blocking main execution threads, leading to more responsive applications.
-
Network communication support: The Ace Framework simplifies the development of networked applications with built-in support for various communication protocols, primarily focusing on TCP and UDP. This flexibility allows developers to create various application types, from simple chat servers to complex data-driven applications.
-
Cross-platform capabilities: Development with the Ace Framework allows for cross-platform compatibility, meaning your applications can run on multiple operating systems such as Windows, Linux, and macOS. This makes it easier to reach a broader audience without extensive platform-specific modifications.
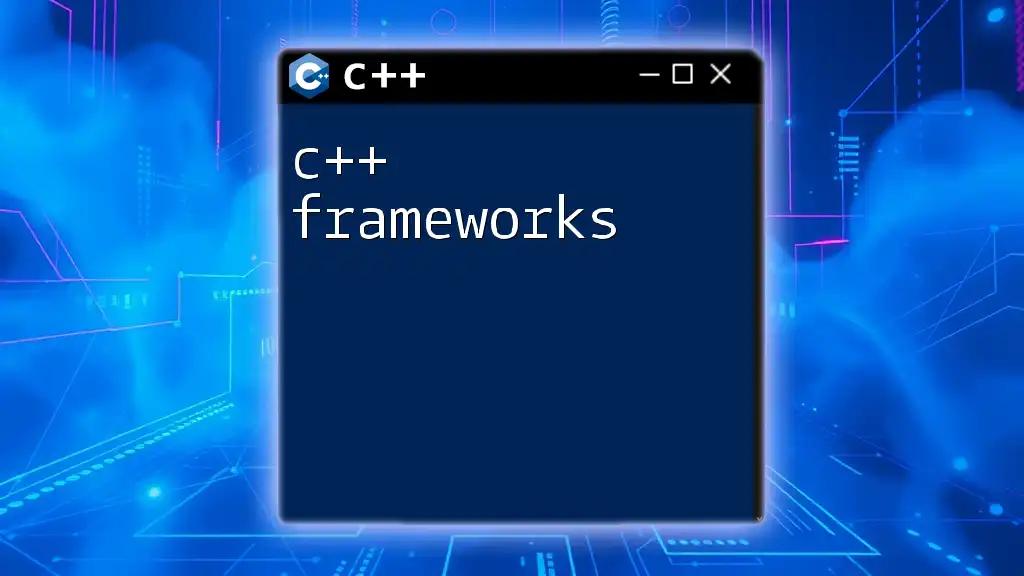
Setting Up the Development Environment
Required Tools
To begin working with the Ace Framework, it is crucial to choose the right set of tools. Several Integrated Development Environments (IDEs) like Visual Studio, Code::Blocks, and Eclipse support C++ and have the necessary features for large-scale development. Additionally, being familiar with various compilers such as GCC and Clang can enhance your ease of development.
Installation Guide
Here is a step-by-step guide to installing the Ace Framework:
-
Download the Ace Framework: You can obtain the latest version of the framework from its official website or repository.
-
Compile the Framework: Use your chosen compiler to build the library. Follow the provided build instructions to ensure it compiles correctly.
-
Linking Ace in Your C++ Project: After successful installation, you must link the framework within your project settings. This usually involves including the path to Ace headers and linking against the Ace libraries in your IDE’s project settings.
-
Common Pitfalls: Some users might encounter issues such as unresolved dependencies or incompatible library versions. Carefully reading through the installation documentation can save you from such headaches.
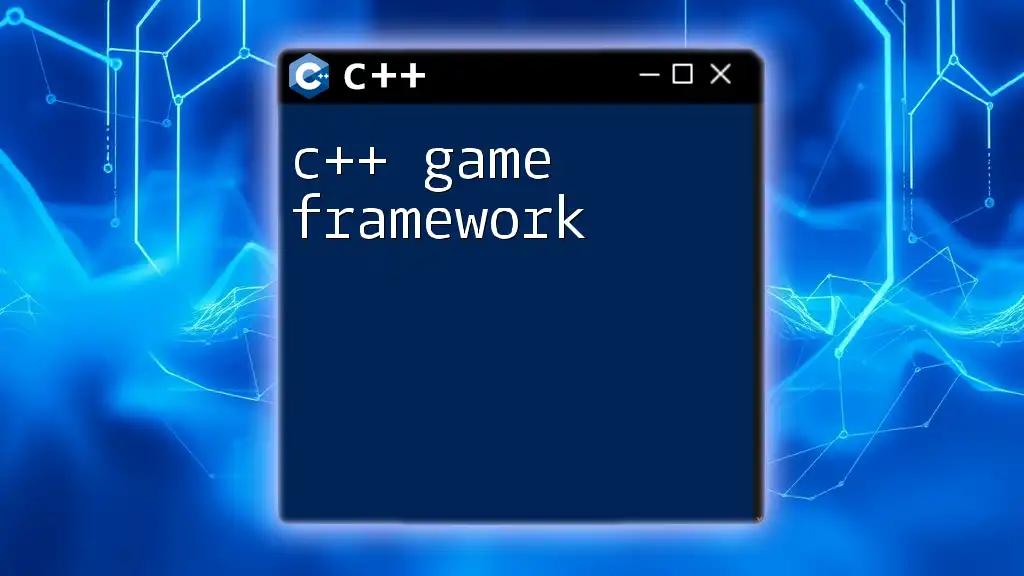
Basic Concepts in Ace Framework
Event Handling
The Ace Framework utilizes an object-oriented approach to manage events through the ACE_Event_Handler class. This class is crucial for implementing event-driven designs where handling input/output operations efficiently is necessary.
For example, here’s how you can define a simple event handler class:
class MyEventHandler : public ACE_Event_Handler {
public:
virtual int handle_input(ACE_HANDLE fd) {
// Handle input events here, e.g., read data
return 0; // Return 0 on success
}
};
Synchronous vs Asynchronous Operations
Understanding the difference between synchronous and asynchronous operations is critical when using the Ace Framework. In a synchronous model, tasks are executed sequentially, which can lead to blocking behavior. Conversely, asynchronous models allow tasks to run concurrently, significantly improving application responsiveness.
When to use each model:
- Use synchronous operations for simplified control flows where tasks are dependent on one another.
- Opt for asynchronous operations in high-load scenarios where throughput and responsiveness are prime concerns.
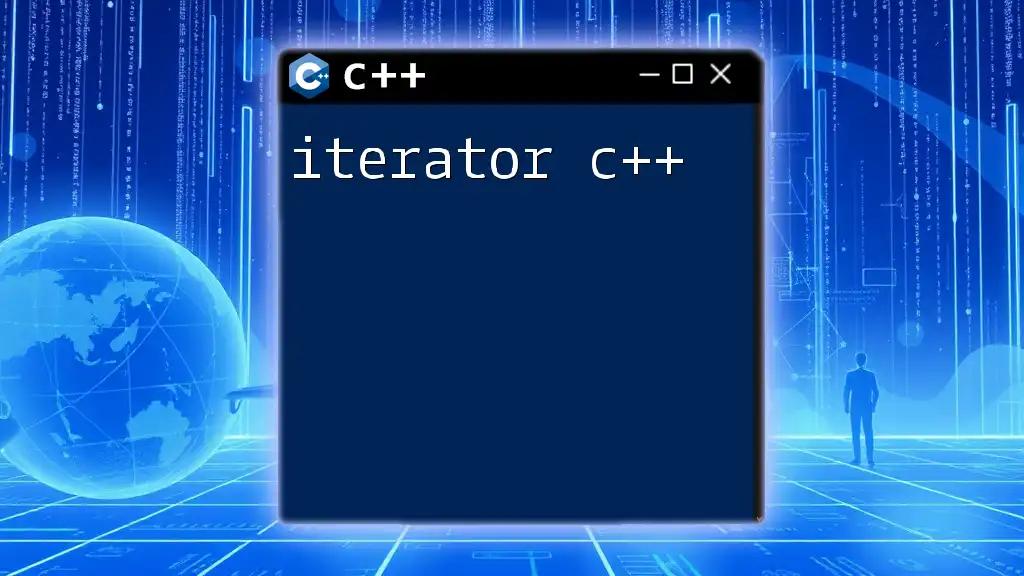
Using the Ace Framework
Creating a Simple Server
Here's a practical exercise in using the Ace Framework to create a basic TCP server. This example outlines how to initialize the ACE library, set up a server socket, and handle incoming connections.
#include <ace/ACE.h>
#include <ace/SOCK_Acceptor.h>
#include <ace/SOCK_Stream.h>
#include <iostream>
int main(int argc, char* argv[]) {
ACE_INET_Addr addr(12345); // Use port 12345
ACE_SOCK_Acceptor acceptor(addr);
std::cout << "Server is running and waiting for connections...\n";
while (true) {
ACE_SOCK_Stream peer_stream;
if (acceptor.accept(peer_stream) == -1) {
std::cerr << "Failed to accept connection." << std::endl;
continue; // Move to the next iteration
}
std::cout << "Connection accepted!" << std::endl;
// Here you would handle the peer connection
peer_stream.close(); // Make sure to close the stream
}
return 0;
}
Building a Client Application
Now, let’s walk through creating a TCP client that connects to the server:
#include <ace/ACE.h>
#include <ace/SOCK_Connector.h>
#include <ace/SOCK_Stream.h>
#include <iostream>
int main(int argc, char* argv[]) {
ACE_INET_Addr server_addr("127.0.0.1:12345"); // Server IP and port
ACE_SOCK_Connector connector;
ACE_SOCK_Stream peer_stream;
if (connector.connect(peer_stream, server_addr) == -1) {
std::cerr << "Connection failed." << std::endl;
return 1;
}
std::cout << "Connected to server!" << std::endl;
// Here, you can implement data sending/receiving logic
peer_stream.close(); // Close the stream after use
return 0;
}

Advanced Features of Ace Framework
Timers in Ace
Timers are essential for handling delays in applications, making it possible to implement timing-based operations seamlessly. The ACE_Timer_Queue allows you to manage timed events effectively. Here's a general use case for timers:
ACE_Timer_Queue timer_queue;
// Scheduling a timer
timer_queue.schedule(timer_handler, "Hello", tick_interval);
Specialized Event Handling
To handle multiple types of events simultaneously, the Ace Framework includes ACE_Reactor, which manages events through a demultiplexing strategy. This ensures you can manage various input sources effectively.
A simple event registration with the reactor would look like this:
ACE_Reactor reactor;
reactor.register_handler(signal_handler, ACE_Event_Handler::READ_MASK);
reactor.run_reactor_event_loop();
This code sets up an event loop that will continuously listen for events to handle.
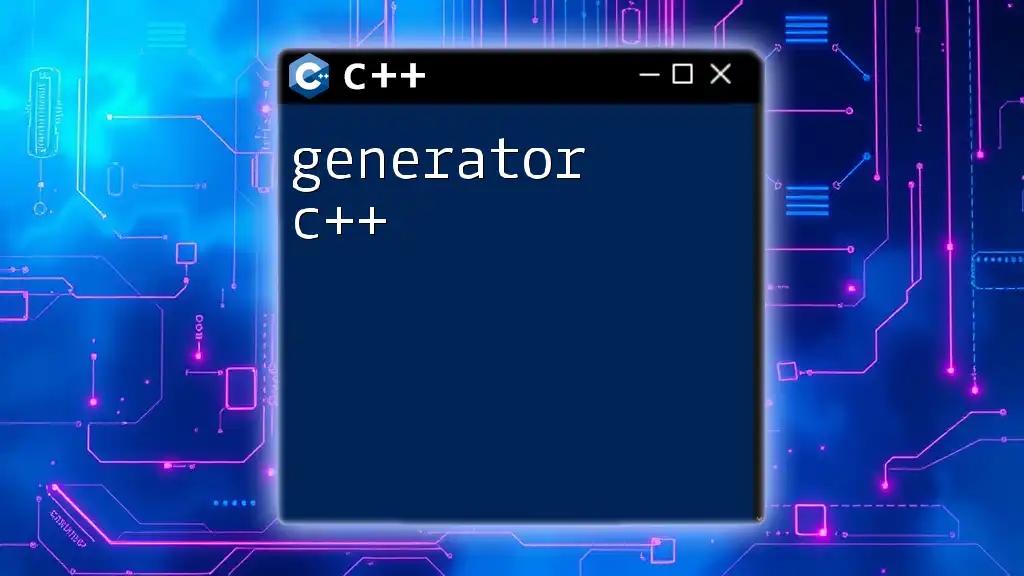
Debugging and Troubleshooting
Common Issues and Solutions
Newer developers often make basic mistakes, such as improper connection handling or failing to clean up resources. Ensuring that you manage connections and free resources like file descriptors or memory is crucial for stable applications.
Example Debugging Session
When debugging a multi-threaded application using Ace, employing tools like GDB can provide insight into concurrent thread operations. Setting breakpoints and stepping through your code can help identify race conditions or deadlock situations common in asynchronous programming.

Best Practices
Code organization is vital for maintaining readability and scalability. Structure your code using classes and functions that encapsulate specific tasks or functionalities. It allows for easier debugging and testing, particularly when working on complex applications.
Resource management cannot be overstated in importance. To prevent memory leaks or exhausting system resources, ensure proper cleanup using destructors or resource management classes from the Ace Framework.
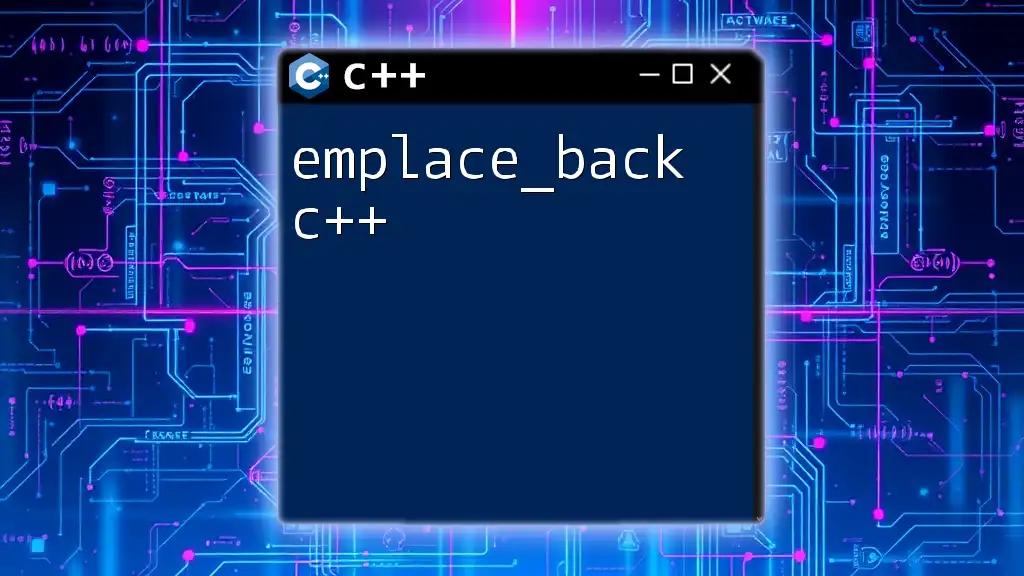
Conclusion
The Ace Framework for C++ provides developers with a powerful set of tools for building networked and real-time applications. By understanding the core concepts and features, you can harness its capabilities to create scalable and efficient software solutions. The journey doesn't end here; consider exploring community forums and discussions for ongoing support and knowledge sharing. Embrace the nuances of the Ace Framework, and leverage its strengths to push the boundaries of your C++ applications.
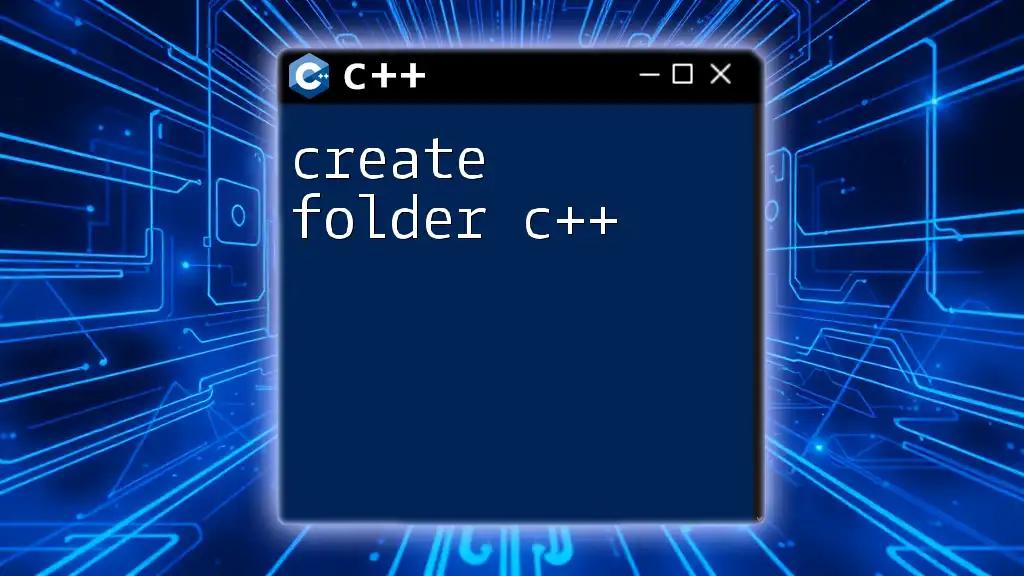
Additional Resources
For further learning, consider checking out the official documentation, relevant books, or online courses dedicated to advanced C++ development and the Ace Framework. Engaging with the community through forums can also enhance your learning experience and provide valuable support as you develop your projects.