An accessor in C++ is a public member function that allows controlled access to private class attributes, thereby promoting encapsulation.
class Example {
private:
int value;
public:
// Accessor
int getValue() const {
return value;
}
};
Understanding Accessor Methods in C++
An accessor method in C++ is a special type of method that allows you to retrieve the values of private member variables while maintaining encapsulation. These methods can significantly improve code readability and maintainability by providing a controlled way to access an object's internal state.
What is an Accessor Method?
Accessor methods are typically named using the get prefix followed by the name of the variable they access. For example, if you have a private variable called `width`, the accessor for it would be `getWidth()`. This clear naming convention enhances the understanding of your code. Here's a simple example of an accessor method:
class Rectangle {
private:
int width;
int height;
public:
// Accessor method
int getWidth() const { return width; }
int getHeight() const { return height; }
};
In this example, `getWidth()` and `getHeight()` return the values of the private members `width` and `height`, respectively. The `const` keyword indicates that these methods do not modify the object’s state.
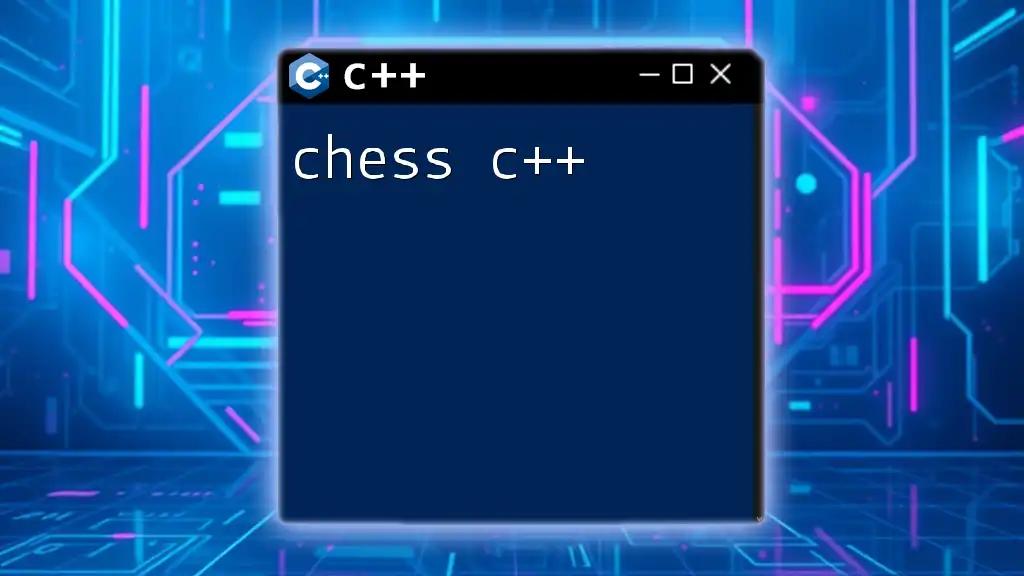
Accessors and Mutators in C++
Definition of Mutator Methods
While accessors are used for retrieving values, mutator methods, often prefixed with set, allow you to modify the values of private member variables. Combining accessors and mutators encapsulates the state of the object, providing a clear interface for interacting with the object's properties.
Differences Between Accessors and Mutators
The main difference lies in their functionality:
- Accessors retrieve data
- Mutators change data
Example: Accessors and Mutators in Action
Here’s an enhanced example demonstrating both accessors and mutators:
class Rectangle {
private:
int width;
int height;
public:
// Accessors
int getWidth() const { return width; }
int getHeight() const { return height; }
// Mutators
void setWidth(int w) { width = w; }
void setHeight(int h) { height = h; }
};
In this class, `setWidth(int w)` and `setHeight(int h)` allow external code to set the values of `width` and `height`, while `getWidth()` and `getHeight()` provide access to these values without exposing the member variables directly.
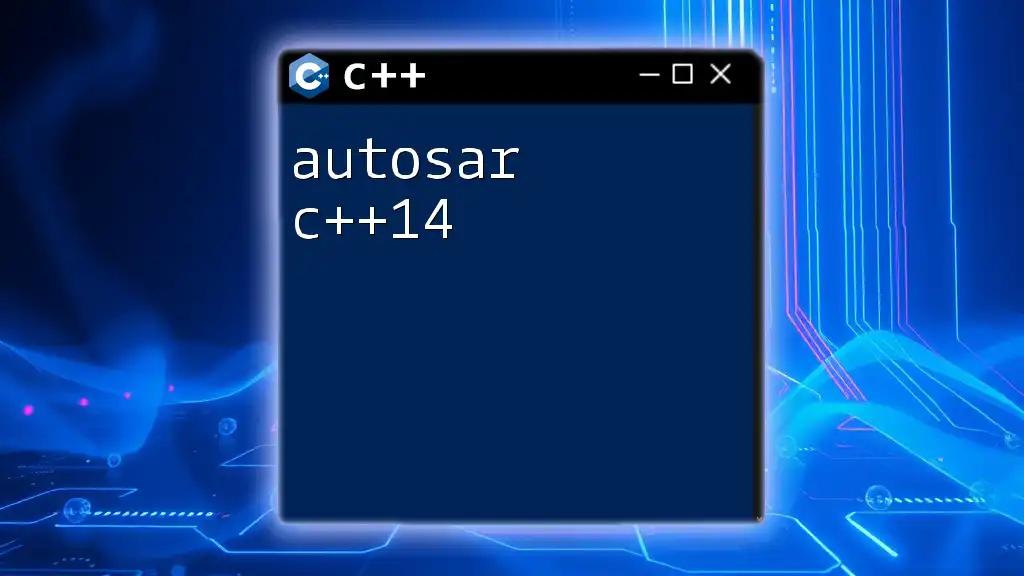
Creating Accessor Methods in C++
How to Define Accessor Methods
To define an accessor method, follow these steps:
- Decide the member variable you want to expose.
- Create a public method with a name prefixed by get.
- Make sure the method returns the appropriate type and is marked as `const` if it does not change the object's state.
Best Practices for Writing Accessors
- Use `const`: Always mark accessor methods as `const` to convey that these methods will not modify the object's data.
- Avoid Unnecessary Computations: Keep accessor methods straightforward; they should merely return the value of the private member variable.
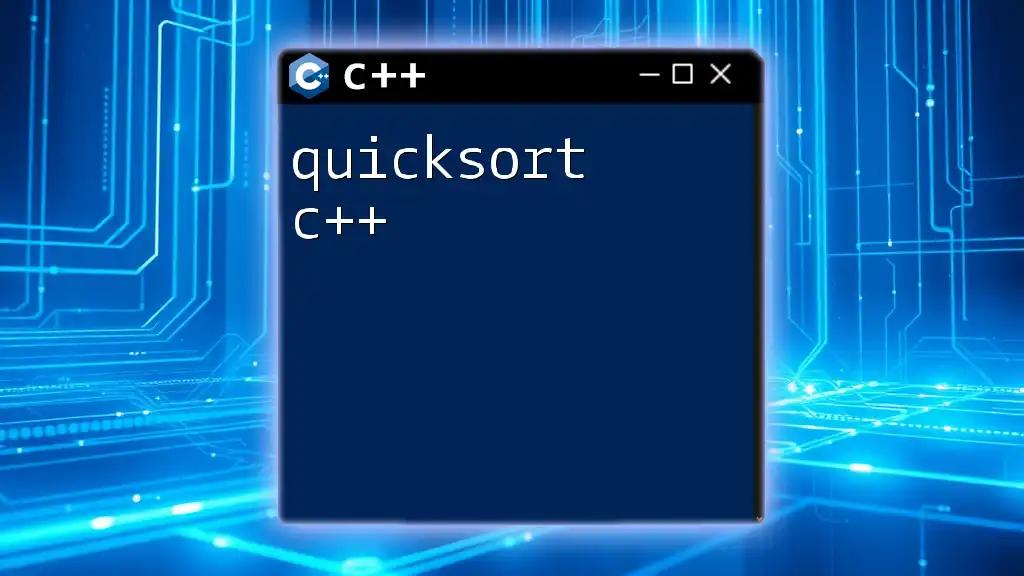
Advantages of Using Accessor Methods
Promoting Data Encapsulation
Accessors promote encapsulation by restricting direct access to class members. This ensures that any data-related logic remains within the class, maintaining its integrity.
Simplifying Code Maintenance
When you need to change how data is stored or validated, you can modify accessors without affecting the code that uses these methods. This aspect significantly enhances the maintainability of your code.
Testing and Debugging
Accessor methods can facilitate unit testing. You can independently verify that objects contain the correct values by calling these methods during tests, ensuring they behave as expected.
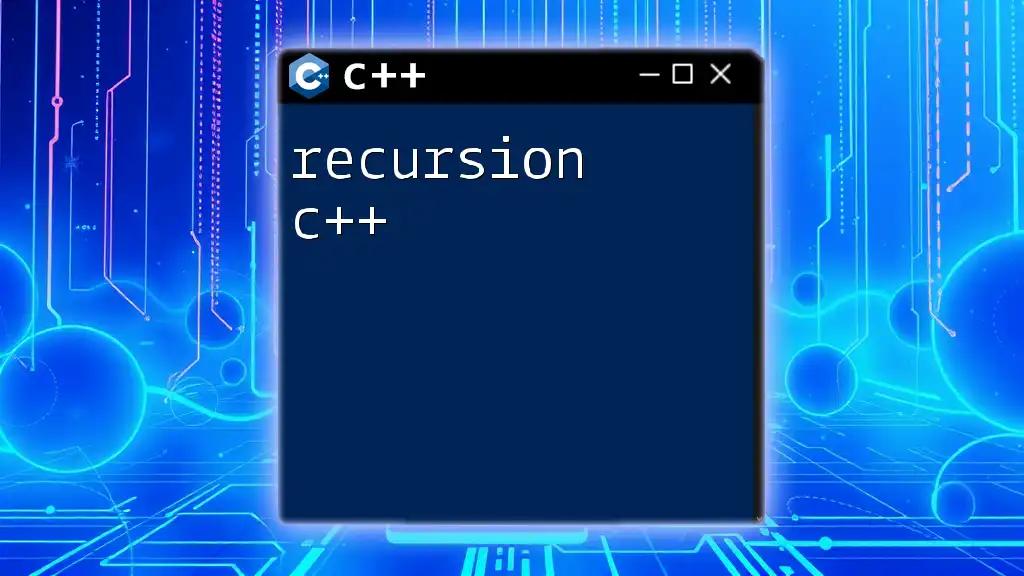
Common Mistakes with Accessor Methods
Forgetting `const` in Accessor Methods
One frequent mistake is omitting the `const` qualifier in accessor methods. This can lead to unintended modifications and break the promise that these methods won't alter the object state, which is a fundamental practice in C++.
Misusing Accessors to Modify State
Implementing logic to modify the state of the object within an accessor is a violation of its purpose. Accessors should strictly return values and should not contain code that changes internal attributes.
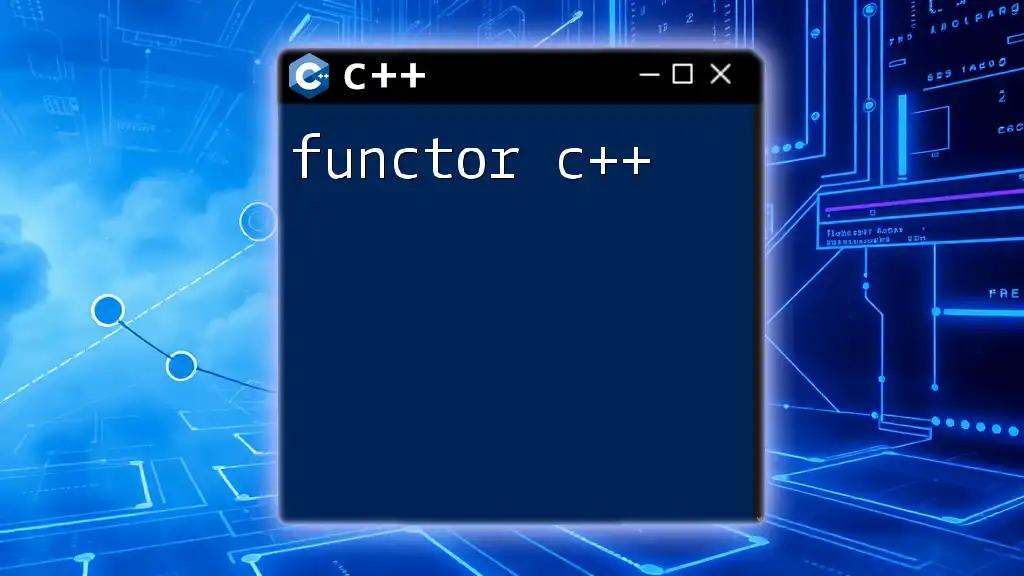
Practical Examples of Accessor Methods in C++
Example 1: Accessing Object Properties
Here’s another organization-focused example that uses accessors for an employee class:
class Person {
private:
std::string name;
int age;
public:
// Accessor methods
std::string getName() const { return name; }
int getAge() const { return age; }
};
In this case, `getName()` and `getAge()` are used to access the values of the private member variables `name` and `age`.
Example 2: Accessors with Collections
Accessors can also be used to handle collections. Consider the following example:
class Employee {
private:
std::vector<std::string> tasks;
public:
// Accessor method for retrieving tasks
const std::vector<std::string>& getTasks() const { return tasks; }
void addTask(const std::string& task) { tasks.push_back(task); }
};
In this case, `getTasks()` returns a reference to the tasks vector, ensuring that the integrity of the employee's tasks is maintained while allowing access to the underlying data.
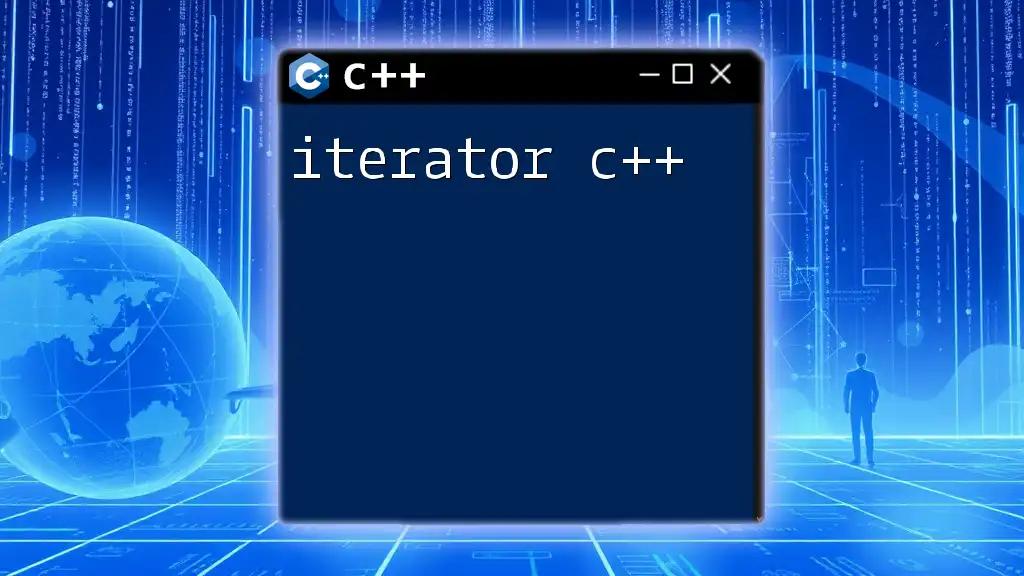
Conclusion
In summary, accessor methods in C++ are an essential part of object-oriented programming, promoting encapsulation and improving code maintainability. By following best practices and understanding the distinctions between accessors and mutators, you can create robust and readable code structures. Implementing accessors will not only streamline your code but also enhance its structure and reliability.