In C++, a `runtime_error` is an exception that indicates an error that occurs while the program is running, typically due to logical issues, invalid operations, or external factors, and can be caught using a try-catch block.
Here's a code snippet demonstrating how to throw and catch a `runtime_error`:
#include <iostream>
#include <stdexcept>
int main() {
try {
throw std::runtime_error("This is a runtime error!");
} catch (const std::runtime_error& e) {
std::cout << "Caught a runtime_error: " << e.what() << std::endl;
}
return 0;
}
Understanding `runtime_error` in C++
What is `runtime_error`?
`runtime_error` is a standard exception class in C++ that is used to signal errors that occur during the execution of a program. This class is a part of the `<stdexcept>` header and serves as a base class for all runtime exceptions. It allows developers to define specific issues that arise at runtime, making it easier to identify problems and debug applications.
Importance of Handling Runtime Errors
Efficient handling of runtime errors is crucial for maintaining a reliable application. When runtime errors occur, they can lead to unexpected application behavior or crashes, negatively affecting the user experience. By using `runtime_error` and managing exceptions properly, developers can improve application stability, provide meaningful feedback to users, and create more robust applications.
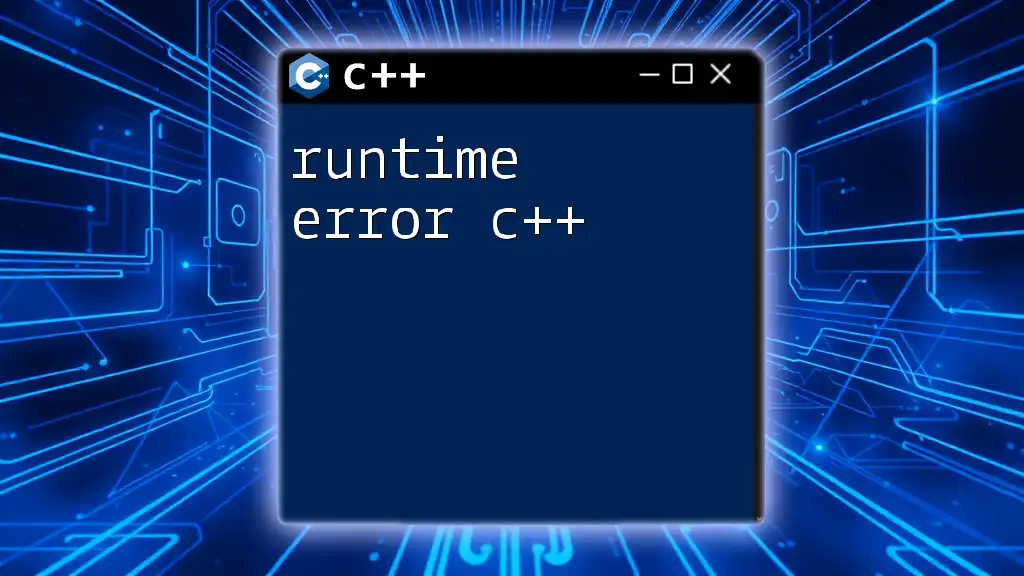
When to Use `runtime_error`
Common Scenarios for Triggering `runtime_error`
`runtime_error` is typically thrown in situations where certain logical conditions become invalid during the execution of the program. Common cases include:
- Invalid operations: Performing operations that do not yield valid results, such as dividing by zero.
- Resource unavailability: Trying to access resources, like files or network connections, that are not available or cannot be opened.
- Logic errors: Issues that are not evident during compilation but surface when particular conditions are met at runtime.
When Not to Use `runtime_error`
Not every error situation should be managed using `runtime_error`. It is important to differentiate between compile-time errors (which the compiler can catch) and runtime errors. In some cases, developer-defined exceptions or other standard exceptions may be more appropriate. For instance, consider using `std::invalid_argument` for function parameters or `std::out_of_range` for accessing out-of-bounds elements in data structures.
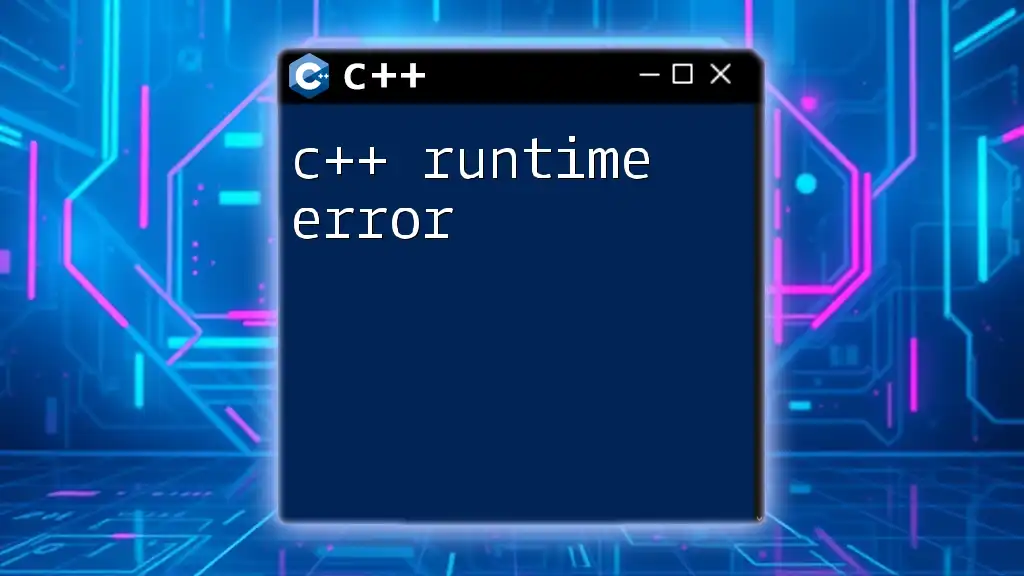
How to Implement `runtime_error` in C++
Including the Required Header
To utilize `runtime_error`, make sure to include the appropriate header in your code:
#include <stdexcept>
This line brings in the necessary components to use exception handling.
Basic Syntax of `runtime_error`
Throwing a `runtime_error` is straightforward. Use the `throw` statement to signal an error condition. The syntax is as follows:
throw std::runtime_error("Error message here");
In this line, the message within the quotation marks should describe the nature of the error, guiding developers or users on what went wrong.
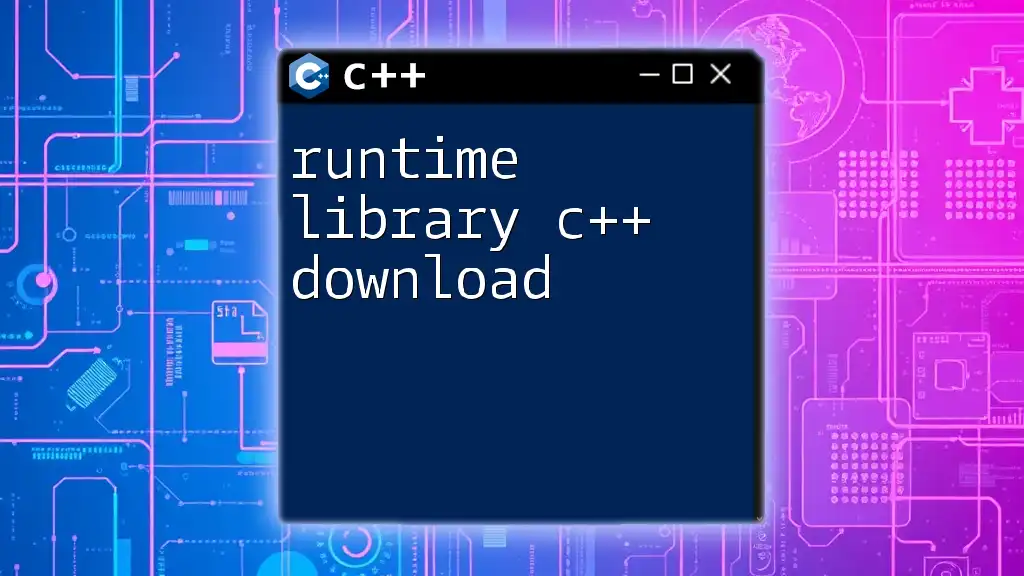
Creating Custom Exceptions with `runtime_error`
Defining a Custom Exception Class
Sometimes, the built-in exceptions do not suffice, and custom exceptions become necessary. Create a custom exception class by extending `runtime_error` as shown below:
class MyCustomError : public std::runtime_error {
public:
MyCustomError(const std::string& msg) : std::runtime_error(msg) {}
};
This allows you to define specific error cases pertinent to your application while leveraging all the benefits of standard runtime error handling.
Throwing a Custom Exception
You can throw a custom exception in the same way you would throw a standard runtime error:
throw MyCustomError("This is a custom runtime error.");
This helps in creating a clearer error handling mechanism and enhances the readability of your codebase.
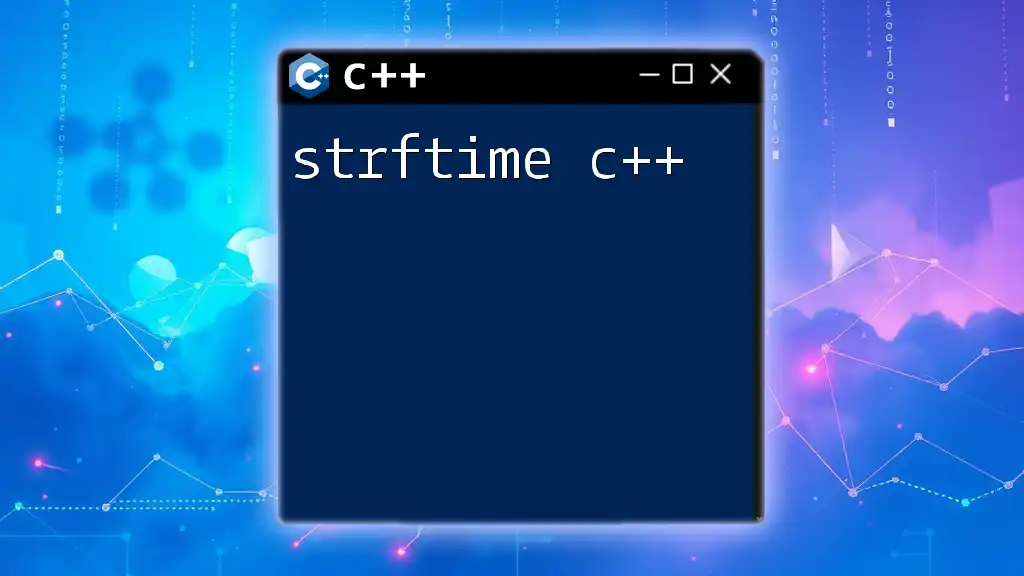
Best Practices for Using `runtime_error`
Clear and Descriptive Error Messages
When throwing exceptions, specificity is key. Clear and descriptive messages help developers identify and troubleshoot issues quickly. For instance, instead of using a vague message like "Error occurred," it's better to specify: "Failed to open file: myfile.txt."
Documenting Your Exceptions
Good documentation practices include outlining which functions may throw exceptions and under what circumstances. This should be consistent throughout your code. By using in-code comments and external documentation, you enhance maintainability and readability.
Consistency in Error Handling
Maintain a consistent approach to exception handling across your application. Use `try-catch` blocks appropriately to catch exceptions and handle them gracefully. Here’s an example:
try {
// Code that might throw
throw std::runtime_error("An error occurred.");
} catch (const std::runtime_error& e) {
std::cerr << "Caught runtime_error: " << e.what() << std::endl;
}
In this example, if a `runtime_error` is thrown, it is caught, and the message is printed to standard error, providing immediate feedback about the error.
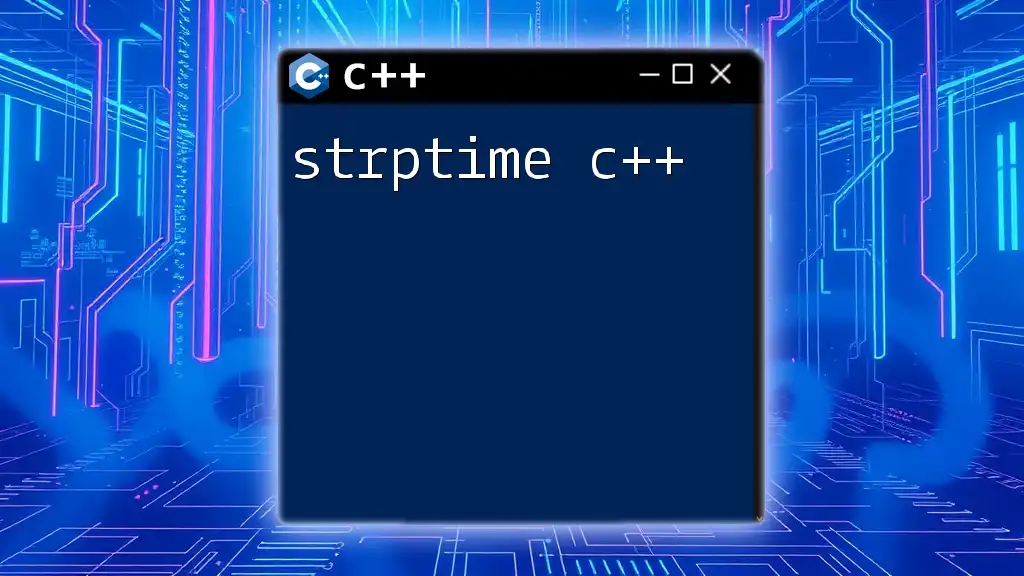
Debugging Runtime Errors
Utilizing Debugging Tools
Leveraging debugging tools can streamline the process of identifying and fixing runtime errors. Most Integrated Development Environments (IDEs) offer debugging features, such as breakpoints, step execution, and variable inspection that can assist in examining the state of the application at the point an error occurs.
Logging Errors
Implementing logging is essential for effective debugging. Logging runtime errors allows developers to track unexpected behavior or failures in production applications. A simple logging implementation could look like this:
std::cerr << "Error: " << e.what() << std::endl;
By capturing and storing error messages, you can analyze them later to gain insights into recurring issues and fix bugs systematically.
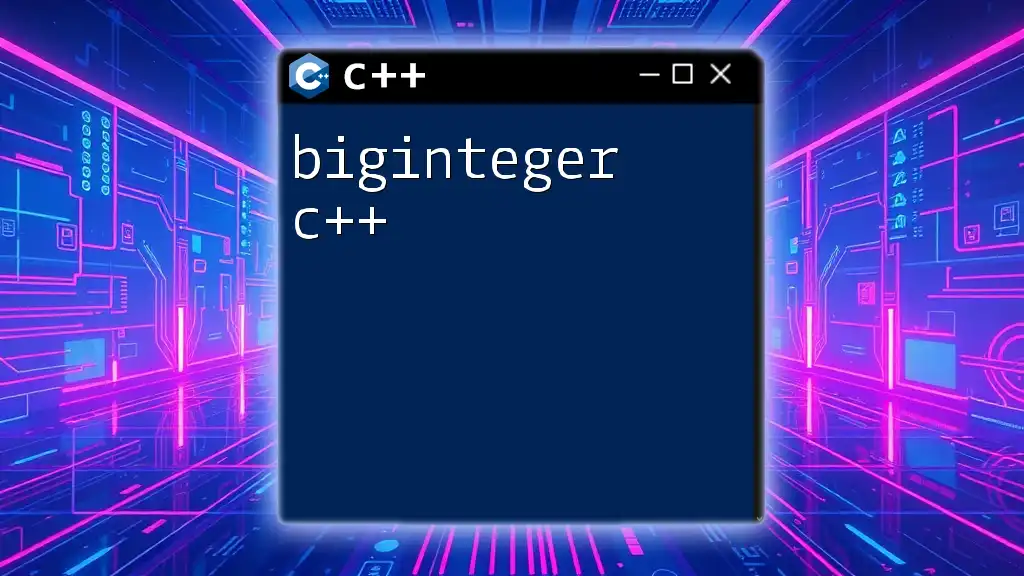
Real-world Examples
Example 1: File Handling
A common scenario where `runtime_error` is useful involves file handling. If a program attempts to open a file that doesn't exist, it can throw a `runtime_error` as follows:
std::ifstream file("non_existent_file.txt");
if (!file) {
throw std::runtime_error("File could not be opened.");
}
This snippet checks if the file was successfully opened and raises an error if it wasn't, thus providing clarity on the issue.
Example 2: Division Operation
Another scenario is performing division operations. Here’s how you can handle a potential division by zero error:
double divide(double numerator, double denominator) {
if (denominator == 0) {
throw std::runtime_error("Division by zero is undefined.");
}
return numerator / denominator;
}
This example checks the denominator before performing the division, ensuring that a runtime error is thrown with a clear message if the denominator is zero.
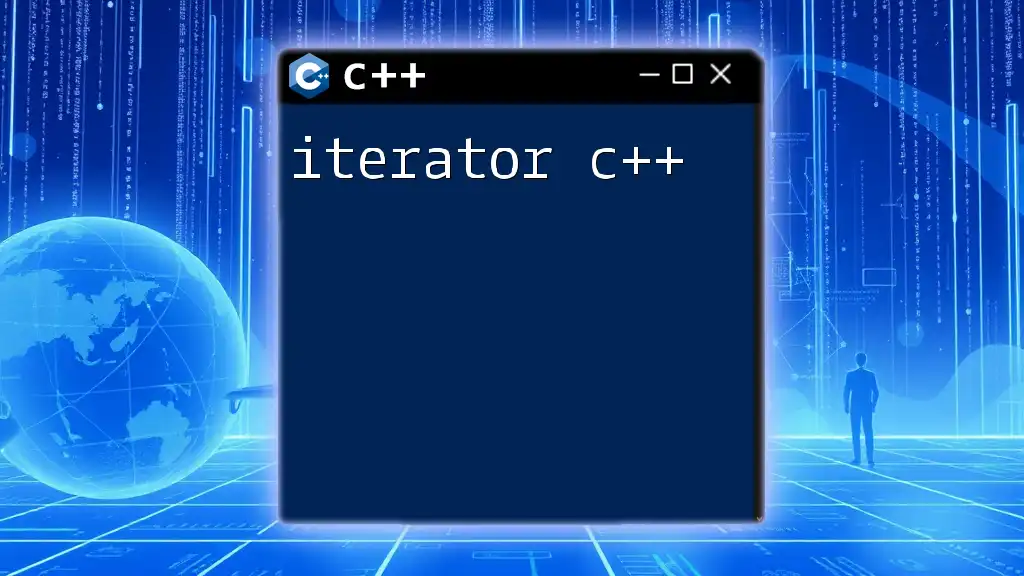
Conclusion
Recap of Key Points
In summary, `runtime_error c++` provides an effective way to handle errors that aren't caught until the program is running. By carefully implementing `runtime_error`, defining custom exceptions, and following best practices, developers can enhance application reliability and user satisfaction.
Encouraging Further Learning
For those interested in mastering exception handling in C++, numerous resources are available, including books and online courses that delve into advanced practices and patterns. Engaging with these materials can significantly boost your programming proficiency.
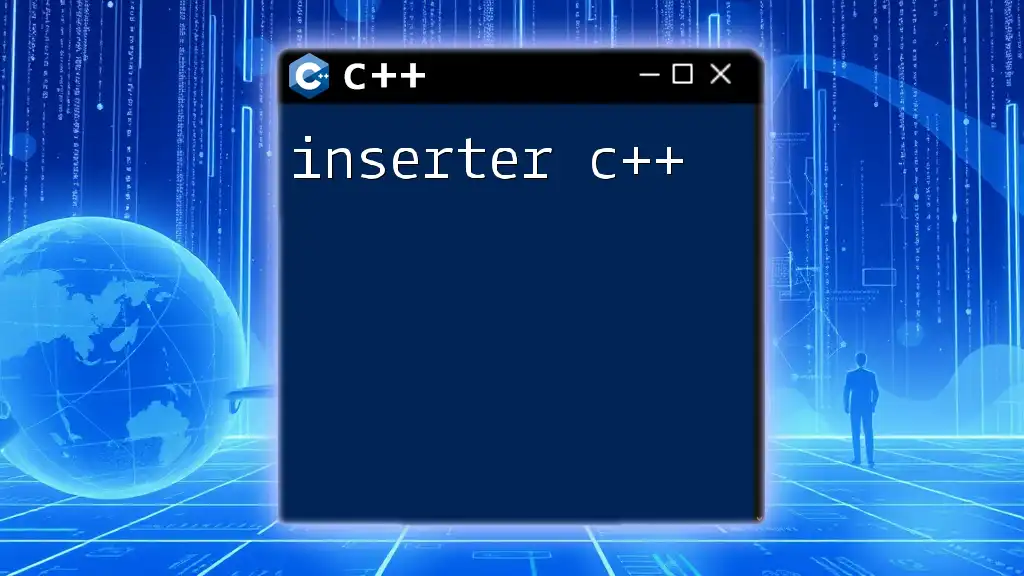
Additional Resources
Recommended Books and Online Courses
Exploring titles that focus on C++ exception handling and best coding practices can deepen your understanding and skills. Look for online platforms that offer specialized courses tailored to exception management in C++.
Community and Support
Participating in C++ forums and communities can be invaluable for developers seeking to learn and share knowledge. Platforms like Stack Overflow and Reddit offer places to ask questions and collaborate with peers, enriching your learning experience.