The `unique_lock` in C++ is a smart pointer that provides exclusive ownership of a mutex, enabling convenient and efficient locking mechanisms, particularly for managing resource access in multithreaded environments.
#include <iostream>
#include <thread>
#include <mutex>
std::mutex mtx;
void print_id(int id) {
std::unique_lock<std::mutex> lock(mtx); // Lock the mutex
std::cout << "Thread " << id << " is running\n";
// lock will be automatically released when going out of scope
}
int main() {
std::thread threads[10];
for (int i = 0; i < 10; ++i) {
threads[i] = std::thread(print_id, i);
}
for (auto& th : threads) {
th.join();
}
return 0;
}
What is `std::unique_lock`?
`std::unique_lock` is a C++ class designed as a flexible and powerful mechanism for managing locks in multithreaded environments. This smart lock offers features that provide better control than its simpler counterpart, `std::lock_guard`.
Why Use `unique_lock`?
The primary advantage of using `unique_lock` lies in its versatility. Unlike `lock_guard`, which is simpler but less flexible, `unique_lock` allows for:
- Deferred locking, where you can create the lock without immediately acquiring it.
- Timed locking, which allows you to try to lock with a timeout.
- Ownership transfer, enabling you to move the lock between threads or scopes safely.
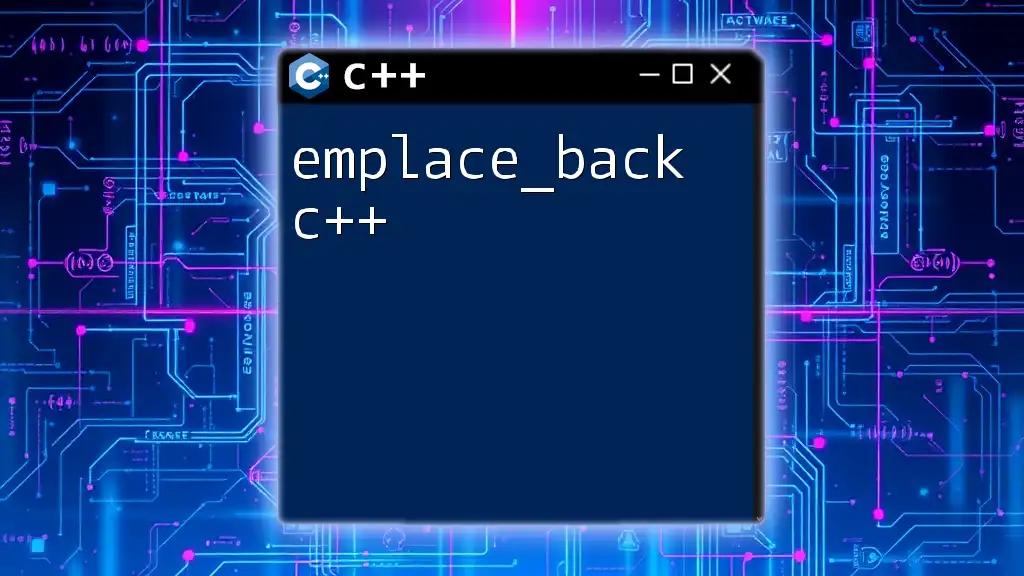
Understanding Mutexes
Understanding Mutexes
A mutex (mutual exclusion) is a synchronization primitive that allows multiple threads to share the same resource (e.g., memory) safely without causing data corruption. By blocking access to a resource, a mutex ensures that only one thread can access the shared resource at any one time.
Types of Mutexes in C++
In C++, several types of mutexes are available:
- `std::mutex`: A basic mutex with no additional features.
- `std::timed_mutex`: Similar to `std::mutex`, but allows for timed locking attempts.
- `std::recursive_mutex`: Allows the same thread to reacquire a lock it already holds.
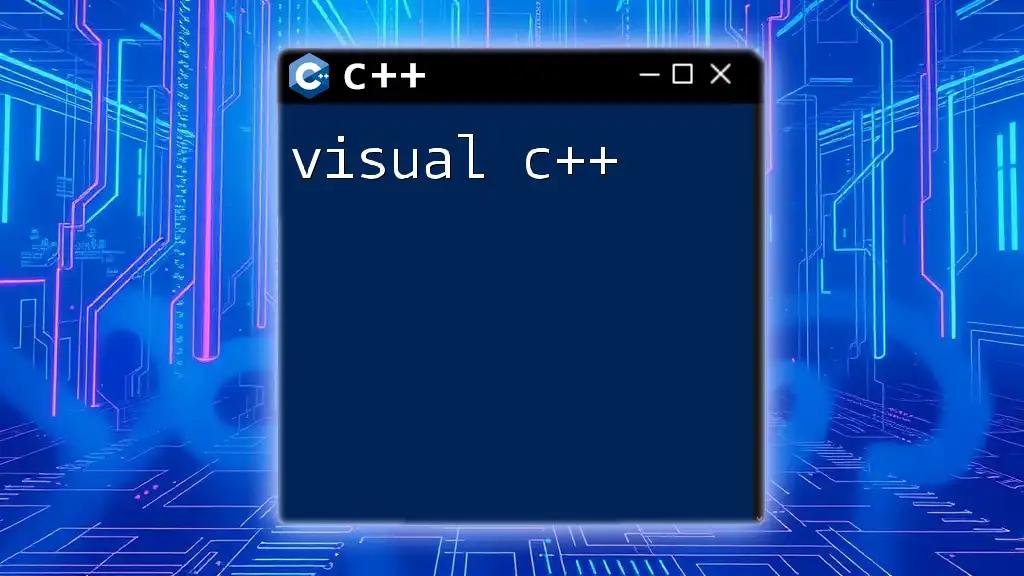
Deep Dive into `std::unique_lock`
Constructing a `unique_lock`
The typical syntax to construct a `unique_lock` is straightforward. The lock is initialized alongside a mutex:
std::mutex mtx;
std::unique_lock<std::mutex> lock(mtx);
In this example, when `lock` is created, it immediately acquires the mutex `mtx`. This is ideal for scoped locking, ensuring that the mutex is released when the `unique_lock` goes out of scope.
Locking and Unlocking
Locking happens automatically upon creation, but it can also be managed explicitly. You can check if a lock is currently held by calling the `.owns_lock()` member function. For instance:
if (lock.owns_lock()) {
// Safe to access shared resources
}
You can manually unlock the mutex by calling `lock.unlock()`, which can be useful when you need to release the lock before the lock object is destroyed.
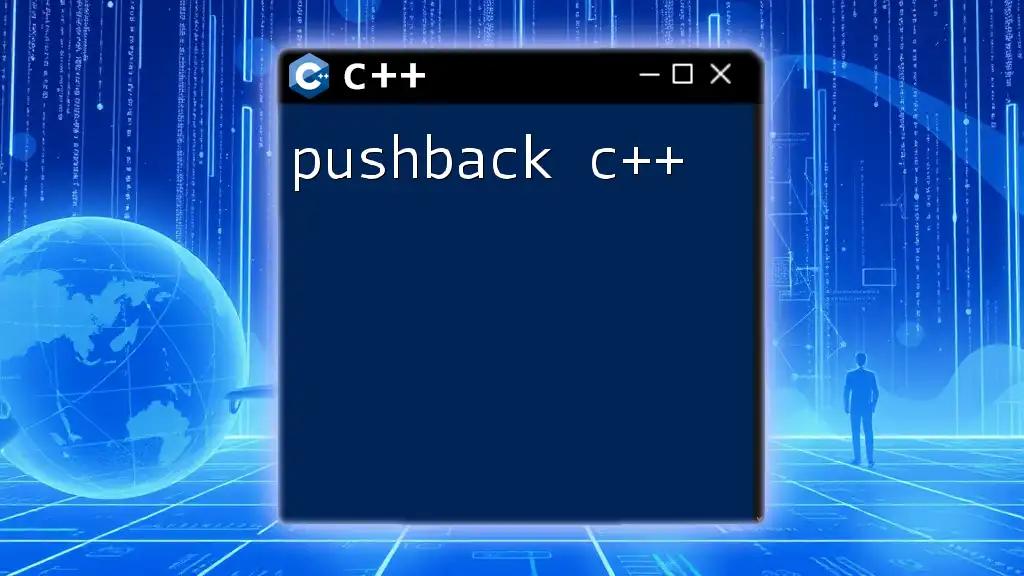
Unique Features of `std::unique_lock`
Deferred Locking
One of the most compelling features of `unique_lock` is the ability to defer locking. This is useful when you want to set up the lock without immediately acquiring it. You can do this by creating a `unique_lock` with the `std::defer_lock` option:
std::unique_lock<std::mutex> lock(mtx, std::defer_lock);
lock.lock(); // Explicitly lock the mutex when required
Timed Locking
`std::unique_lock` also supports timed locking, allowing you to attempt to acquire a lock with a specified timeout duration. This can prevent a thread from waiting indefinitely:
if (lock.try_lock_for(std::chrono::seconds(1))) {
// Do work
} else {
// Handle the timeout scenario
}
Transfer of Ownership
Ownership transfer allows one `unique_lock` to take over the lock from another. This is useful in scenarios where you need to move the lock between different scopes or threads without having to re-lock the mutex:
std::unique_lock<std::mutex> lock1(mtx);
std::unique_lock<std::mutex> lock2(std::move(lock1)); // lock1 is now empty
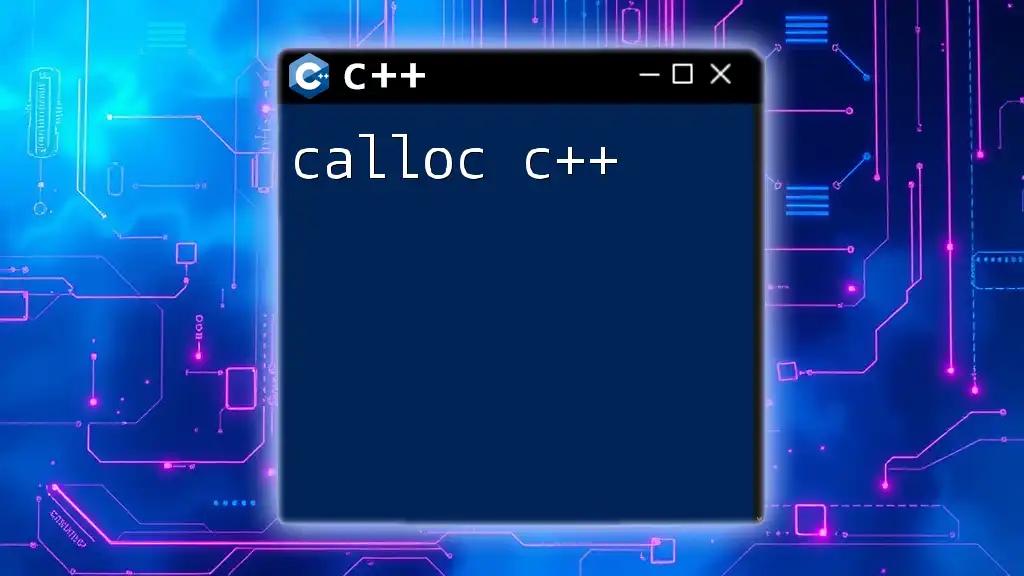
Performance Considerations
When to Prefer `unique_lock` Over `lock_guard`
You should consider using `unique_lock` when you require features like deferred locking, timed locking, or ownership transfer. If your locking needs are straightforward (immediate and scoped), `std::lock_guard` may suffice and usually provides slightly better performance due to its simplicity.
Managing Lock Contention
To avoid deadlocks and improve performance with locks:
- Always try to lock in a consistent order across your codebase.
- Use timeout options where applicable to prevent threads from blocking indefinitely.
- Minimize the duration that locks are held to reduce contention.

Common Pitfalls and Best Practices
Common Mistakes with `std::unique_lock`
Mistakes often happen when:
- A `unique_lock` is destructed without unlocking its associated mutex, leading to potential deadlock.
- The same lock is attempted to be locked multiple times without being unlocked first.
Best Practices
- Scoped Locking: Always encapsulate your `unique_lock` usage in the smallest possible scope to automatically release locks.
- Exception Safety: Make sure your code handles exceptions that could lead to the failure of critical sections where locks are held.

Real-World Examples
Simple Producer-Consumer Pattern Using `unique_lock`
Consider a simple producer-consumer scenario where a shared queue is accessed by multiple threads. The producer adds items to the queue, while the consumer removes them:
std::queue<int> q;
std::mutex mtx;
void producer() {
std::unique_lock<std::mutex> lock(mtx);
q.push(1); // Produce an item
}
In this code sample, by using `std::unique_lock`, we ensure that the access to the queue `q` is synchronized. If multiple producers attempt to add items simultaneously, the mutex protects the shared resource.
Complex Scenarios
More complex scenarios can involve multiple threads interacting with various shared resources. Using `unique_lock` makes it easier to manage multiple mutexes and ensure that only one resource is accessed at a time.
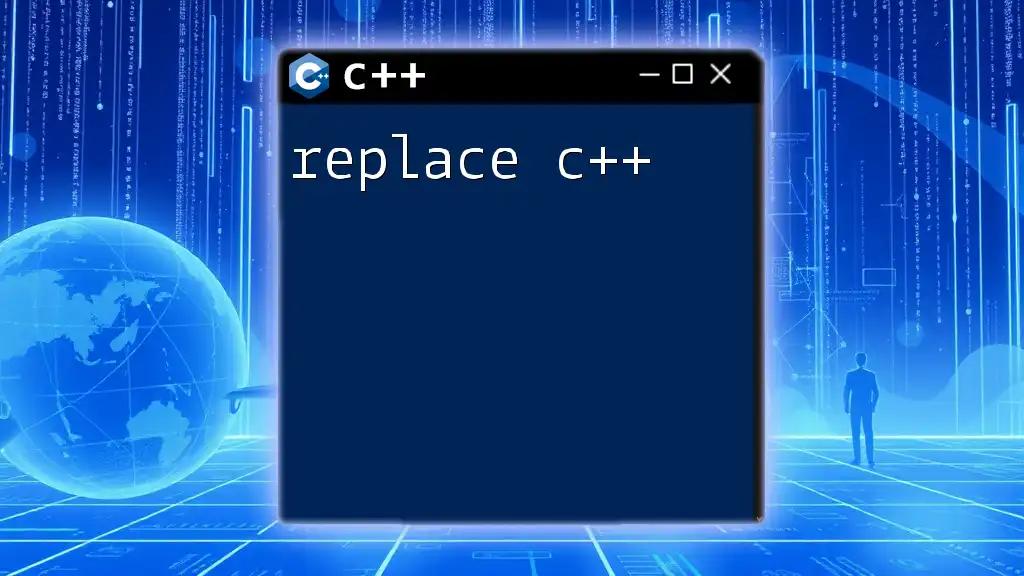
Summary of `std::unique_lock`
In summary, `std::unique_lock` is a powerful tool for managing thread synchronization in C++. It allows for greater flexibility and control compared to other locking mechanisms, making it suitable for a range of applications from simple to complex multithreaded environments.

Further Learning and Resources
For those looking to deepen their understanding of multithreading in C++, consider exploring additional resources such as the official C++ documentation, advanced concurrency tutorials, and practical coding exercises to enhance your skills in using `unique_lock` and other synchronization primitives in C++.
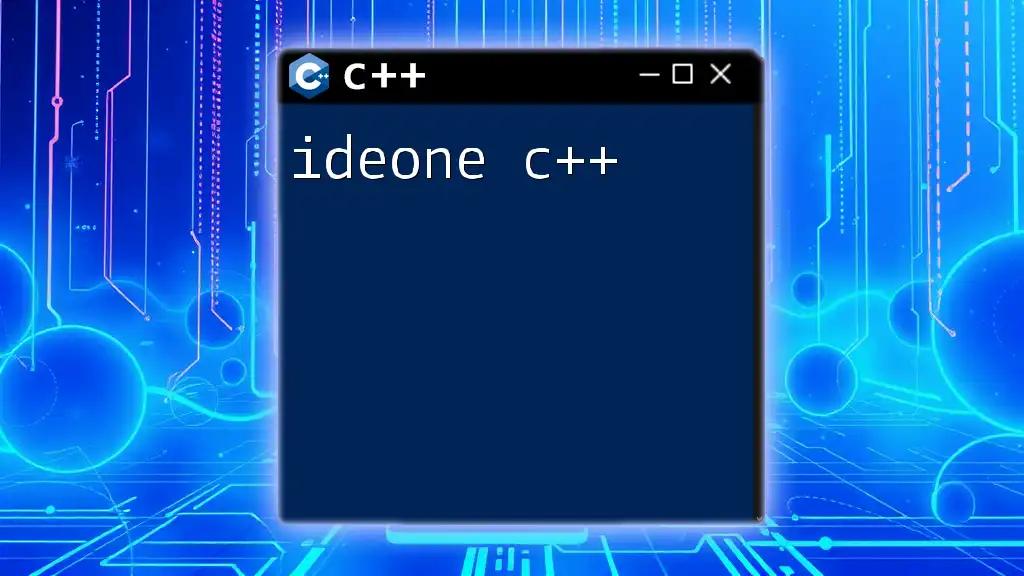
Frequently Asked Questions About `std::unique_lock`
As you explore `std::unique_lock`, you may have common questions such as:
-
What happens if I try to unlock a `unique_lock` that is not locked?
- Calling `unlock()` on an unlocked `unique_lock` can lead to undefined behavior. Ensure the lock is held before unlocking.
-
Can I lock a mutex from multiple threads using `std::unique_lock`?
- No, once locked, a mutex can only be handled by one thread at a time. Attempting to lock it again from another thread will block or throw an error, depending on the lock strategy used.
By understanding these concepts and practices, you can make full use of `unique_lock` in your C++ projects, ensuring safe and efficient multithreaded programming.