FILO (First In, Last Out) is a principle often used in data structures like stacks, where the last element added is the first one to be removed.
Here's a simple example of using a stack in C++ to demonstrate the FILO principle:
#include <iostream>
#include <stack>
int main() {
std::stack<int> myStack;
// Pushing elements onto the stack
myStack.push(1);
myStack.push(2);
myStack.push(3);
// Popping elements from the stack
while (!myStack.empty()) {
std::cout << myStack.top() << std::endl; // Last pushed element is printed first
myStack.pop();
}
return 0;
}
Understanding FILO Concept
FILO stands for First In, Last Out, a foundational principle in data handling that plays a crucial role in various programming scenarios. Unlike FIFO (First In, First Out), where elements are processed in the order they arrive, FILO ensures that the newest element is the first one to be accessed. This concept is fundamental to the stack data structure, making it essential for any C++ programmer to grasp.
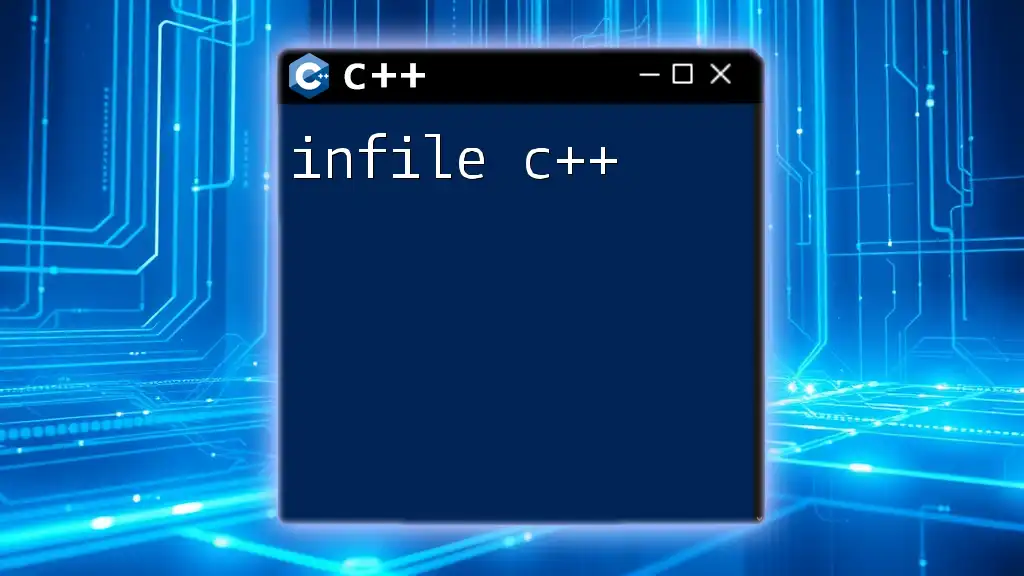
Importance of FILO in Programming
Understanding and utilizing FILO is vital as it provides a way to manage data dynamically and efficiently. In programming, FILO allows for operations like backtracking, undo functionality, and even certain parsing tasks. By mastering this concept, one can elegantly solve complex problems and optimize various algorithms.
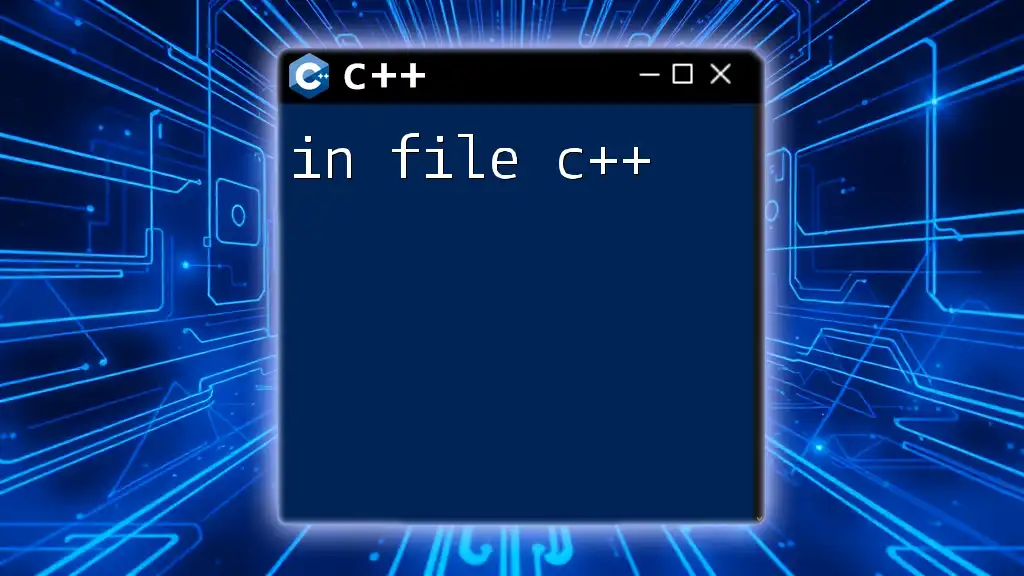
The Stack Data Structure
Overview of Stack
A stack is a linear data structure that follows the FILO principle. It comprises two primary operations: push, to add an element to the top of the stack, and pop, to remove the element from the top.
Key characteristics of a stack include:
- LIFO structure: The last element added is the first one to be removed.
- Dynamic size: A stack can grow and shrink as elements are added or removed.
Real-World Applications of Stacks
Stacks can be found in various real-world applications, including:
- Undo features in software: Actions are stored on a stack, allowing users to revert to the most recent state.
- Expression evaluation: Compilers use stacks to evaluate expressions and manage operation precedence.
- Function calls in programming: The call stack maintains the execution context for function calls and returns.
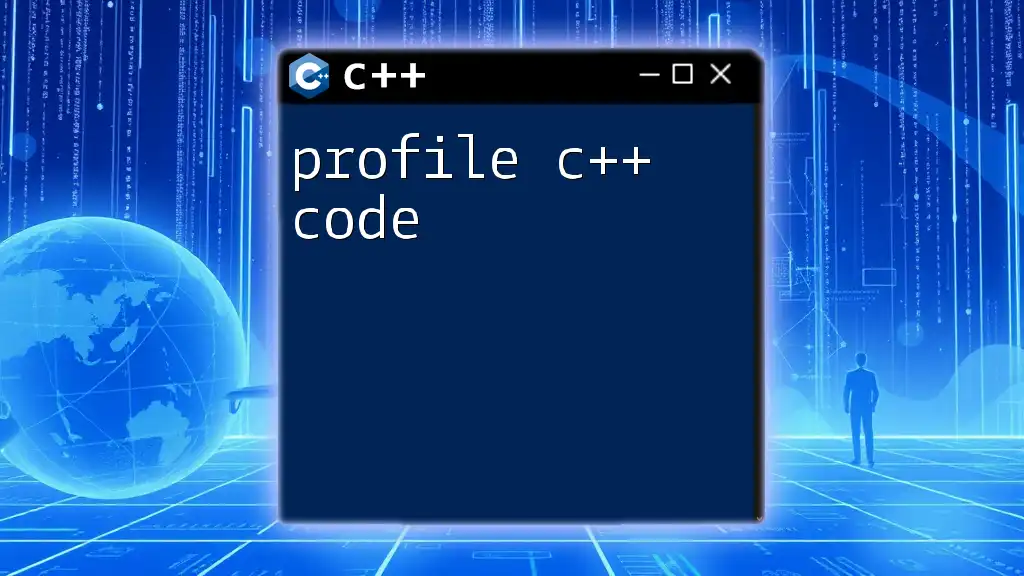
Implementing a Stack in C++
Basic Stack Operations
To fully leverage the filo c++ concept, one must understand the basic stack operations:
- Push: Place an element at the top of the stack.
- Pop: Remove the top element from the stack.
- Peek: Retrieve the top element without removing it.
- isEmpty: Check if the stack is empty.
Code Snippet: Basic Stack Implementation
Here's a simple implementation of a stack using C++'s Standard Template Library (STL):
#include <iostream>
#include <stack>
class Stack {
private:
std::stack<int> data;
public:
void push(int value) {
data.push(value);
}
void pop() {
if (!data.empty()) {
data.pop();
}
}
int top() {
return data.top();
}
bool isEmpty() {
return data.empty();
}
};
In this implementation, we've defined a class `Stack` that leverages the STL stack to manage integer values. The class consists of methods to push, pop, check the top element, and verify if the stack is empty.
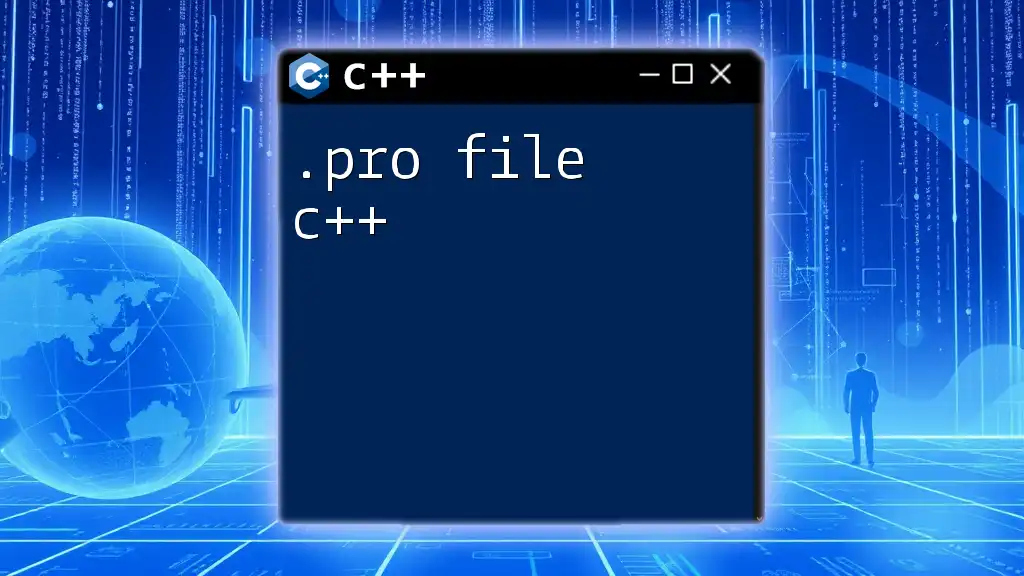
Advanced Stack Functions
Custom Stack Implementation
While STL stacks are convenient, sometimes you might want to build your own stack from scratch for educational purposes or specific use cases. This allows for greater control over the underlying structure and memory management.
Code Snippet: Custom Stack Class
Here’s how to create a custom stack class without using STL:
#include <iostream>
class CustomStack {
int* arr;
int top;
int capacity;
public:
CustomStack(int size) {
arr = new int[size];
capacity = size;
top = -1;
}
void push(int value) {
if (top == capacity - 1) {
std::cout << "Stack Overflow!\n";
return;
}
arr[++top] = value;
}
void pop() {
if (top == -1) {
std::cout << "Stack Underflow!\n";
return;
}
top--;
}
int peek() {
if (top != -1) {
return arr[top];
}
throw std::out_of_range("Stack is empty!");
}
bool isEmpty() {
return top == -1;
}
};
In this code, we define a `CustomStack` class that manages its memory allocation, allowing for basic push, pop, and peek operations while handling potential overflow and underflow scenarios.
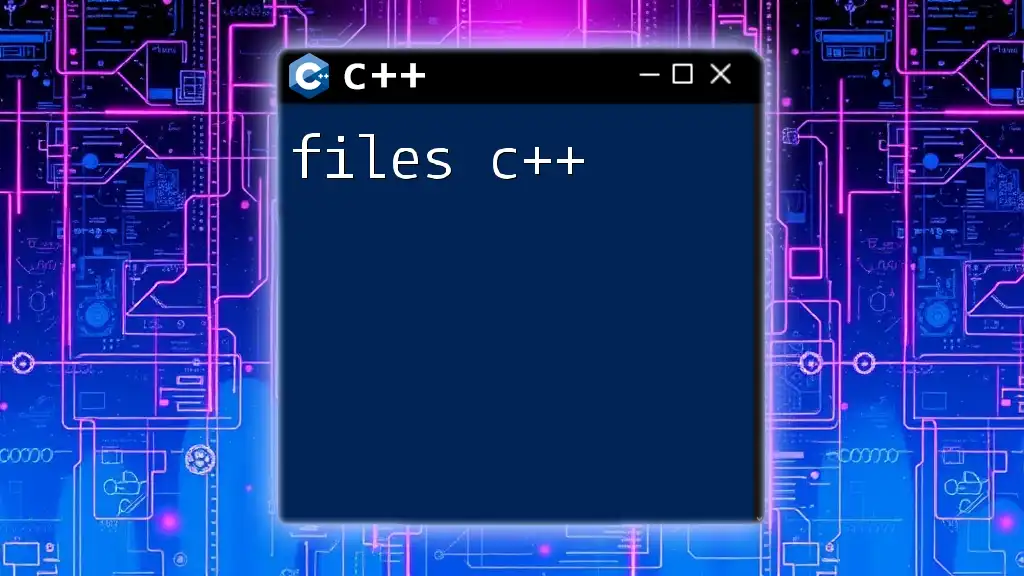
Use Cases of FILO in Algorithms
Depth-First Search (DFS)
The Depth-First Search (DFS) algorithm exemplifies the use of FILO. In DFS, a stack is crucial for keeping track of the nodes visited during traversal. As we encounter a new node, we push it onto the stack. When we need to backtrack, we pop from the stack, adhering to the FILO principle.
Backtracking Algorithms
FILO is also extensively used in backtracking algorithms, where the goal is to explore all potential solutions to a problem. The stack keeps track of choices made, allowing the algorithm to revert to previous states easily. This approach is useful in solving problems like the N-Queens puzzle or Sudoku.
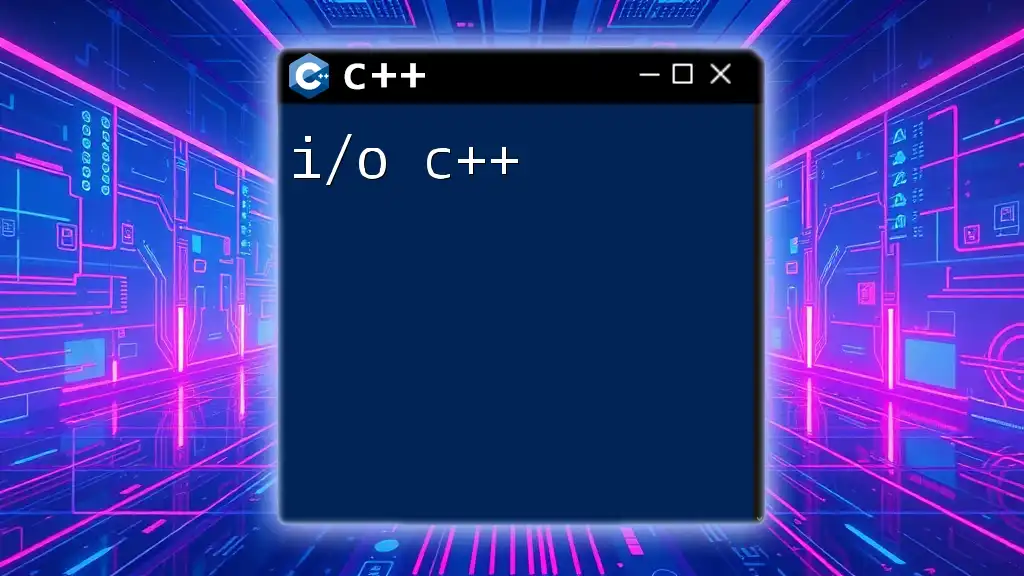
Common Pitfalls and Best Practices
Memory Management with Stacks
When managing memory with stacks, especially in custom implementations, it's imperative to handle memory correctly to avoid leaks. Always ensure that dynamically allocated memory is freed once no longer needed.
Debugging Stack Issues
Common errors in stack management include:
- Stack Overflow: Occurs when attempting to push onto a full stack. Always check size before pushing.
- Stack Underflow: Happens when popping from an empty stack. Implement checks to prevent this.
- Memory Leaks: Use tools like Valgrind to track memory allocation issues.
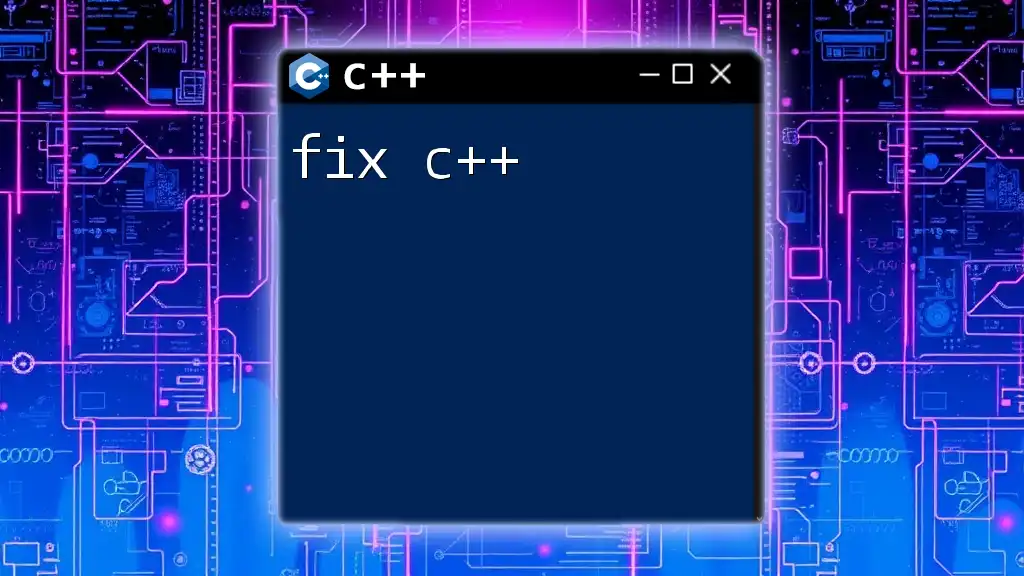
Conclusion
The filo c++ concept is fundamental in understanding how stacks operate and how they can be implemented efficiently in C++. By mastering FILO principles, one can tackle a variety of algorithms and data structure problems effectively.
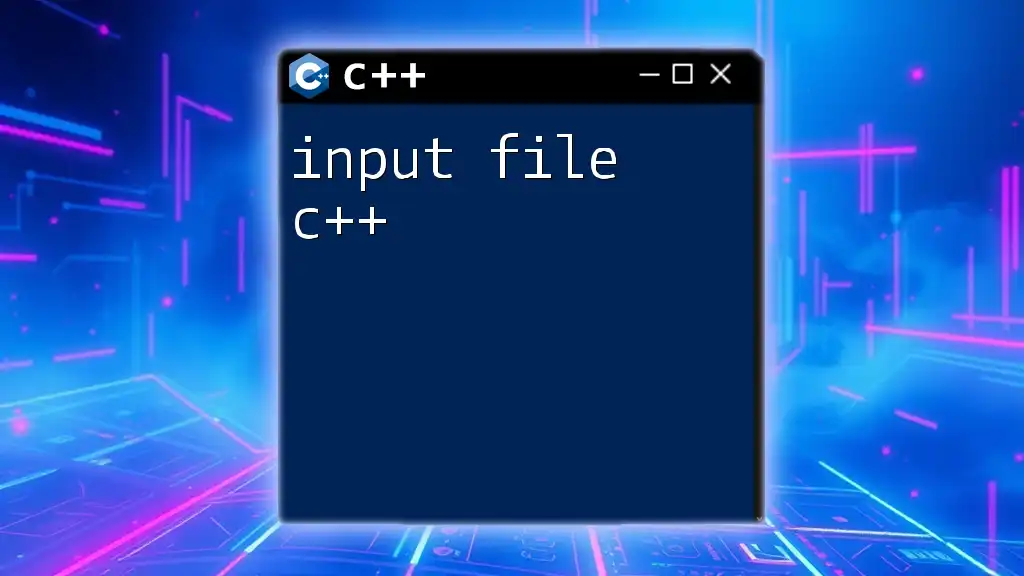
Further Reading and Resources
For those seeking to delve deeper into C++ and FILO concepts, numerous resources are available, ranging from tutorials and documentation to community forums where one can ask questions and share knowledge.
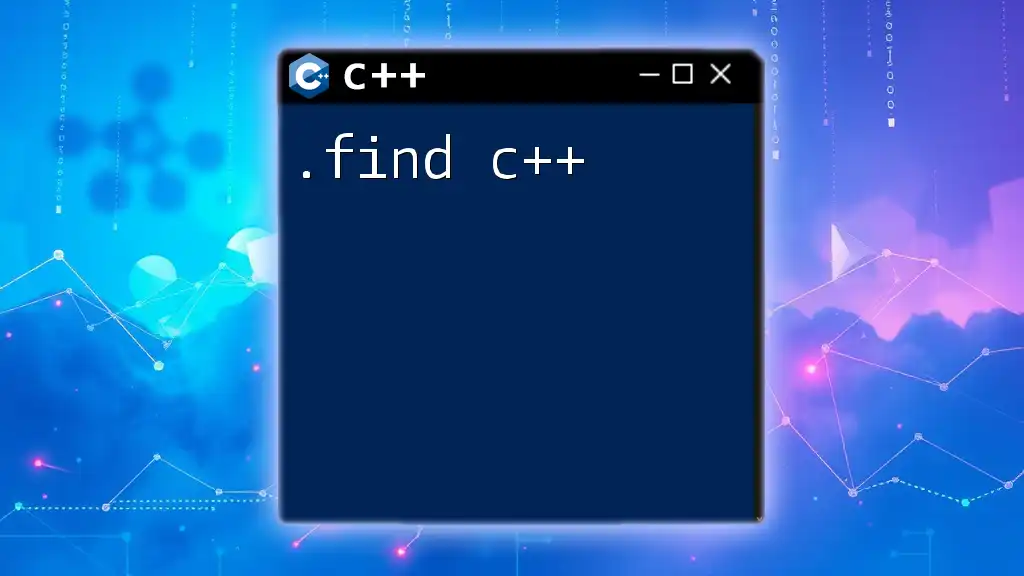
Call to Action
Practice implementing your own stack and experiment with different algorithms relying on FILO principles. Consider joining forums or online classes to further enhance your understanding of C++ and data structures.