In C++, overflow occurs when an operation exceeds the storage capacity of the data type, leading to unexpected results; for example, adding two large integers.
Here's a code snippet that demonstrates integer overflow:
#include <iostream>
#include <limits>
int main() {
int maxInt = std::numeric_limits<int>::max();
int overflowedInt = maxInt + 1; // This will cause overflow
std::cout << "Max Int: " << maxInt << "\n";
std::cout << "Overflowed Int: " << overflowedInt << "\n"; // Output will not be as expected
return 0;
}
Understanding Overflow in C++
What is Overflow?
Overflow occurs when an arithmetic operation produces a value outside the allowed range that can be represented by a data type. In programming, particularly in C++, this often leads to unexpected behavior or bugs. For example, when adding two integers, if the sum exceeds the maximum value that an `int` can hold, the result wraps around to the minimum value, causing a logical error.
Why Does Overflow Matter in C++?
Ignoring overflow can lead to serious implications in software development. Bugs resulting from overflow can corrupt data, create vulnerabilities, or compromise the security of an application. Understanding and handling overflow effectively is crucial for any C++ developer. By doing so, programmers can ensure their applications behave as expected, maintain data integrity, and safeguard against potential attacks that exploit these weaknesses.
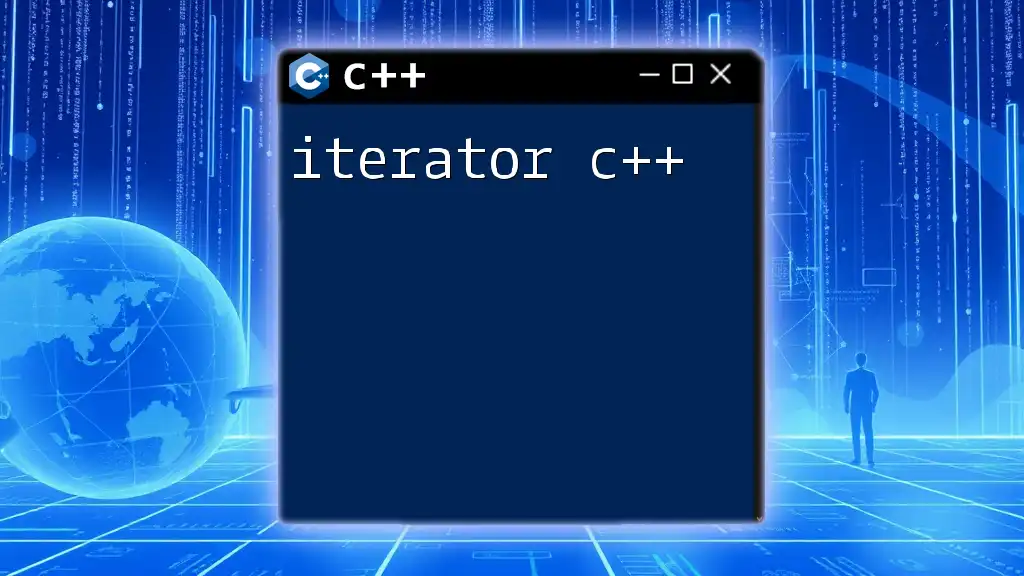
Types of Overflow in C++
Integer Overflow
Integer overflow is a specific instance of overflow that occurs when an operation on integers exceeds the maximum or minimum limit of the integer type. In C++, the behavior resulting from integer overflow is technically undefined, meaning the output can vary from one compilation or execution to another, leading to unpredictable results.
Code Snippet: Simple Integer Overflow Example
#include <iostream>
#include <limits>
int main() {
int x = std::numeric_limits<int>::max(); // Max int value
std::cout << "Max int value: " << x << std::endl;
x = x + 1; // This causes overflow
std::cout << "After overflow: " << x << std::endl; // Undefined behavior
return 0;
}
In this example, when we attempt to add 1 to the maximum integer, the result wraps around—this can lead to catastrophic failures if not handled properly.
Floating-Point Overflow
Floating-point values also have their own limits. An overflow occurs in a floating-point context when a calculation results in a number too large for that format to express. This will often result in a representation of infinity.
Code Snippet: Floating-Point Overflow Example
#include <iostream>
#include <limits>
int main() {
float largeFloat = std::numeric_limits<float>::max();
std::cout << "Max float value: " << largeFloat << std::endl;
largeFloat *= 2; // This can cause overflow
std::cout << "After overflow: " << largeFloat << std::endl; // May output inf
return 0;
}
In this case, multiplying the maximum representable floating-point value by 2 results in the output of `inf` (infinity). Handling this situation is critical, especially in applications requiring numerical accuracy.
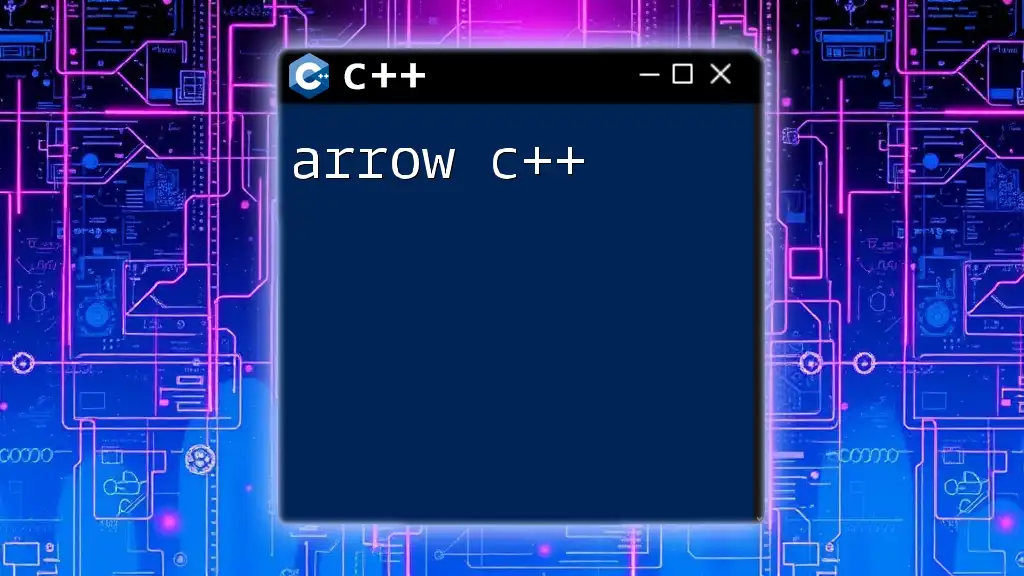
Detecting Overflow in C++
Compiler Warnings and Static Analysis Tools
One way to detect potential overflow is to leverage compiler warnings. Most modern C++ compilers, like GCC or Clang, offer options to enable warnings that can catch issues related to overflow when compiling code.
Static analysis tools such as CPPcheck or Clang Static Analyzer can help identify parts of the code that are prone to overflow bugs even before runtime. Using these tools can significantly reduce the risk of overflow-related issues in your application.
Runtime Overflow Detection
In addition to compile-time checks, implementing runtime overflow detection can create safeguard mechanisms in your code against unexpected behavior. Custom functions can be crafted to verify whether an operation will result in an overflow.
Code Snippet: Overflow Detection Function
#include <iostream>
#include <limits>
#include <stdexcept>
bool willAdditionOverflow(int a, int b) {
if (a > 0 && b > std::numeric_limits<int>::max() - a) return true;
if (a < 0 && b < std::numeric_limits<int>::min() - a) return true;
return false;
}
int main() {
int a = 1000000000;
int b = 2000000000;
if (willAdditionOverflow(a, b)) {
std::cout << "Addition would overflow!" << std::endl;
} else {
std::cout << "Result: " << (a + b) << std::endl;
}
return 0;
}
In this example, the function `willAdditionOverflow` checks whether adding two integers might cause an overflow. In doing so, developers can confidently handle potential errors.
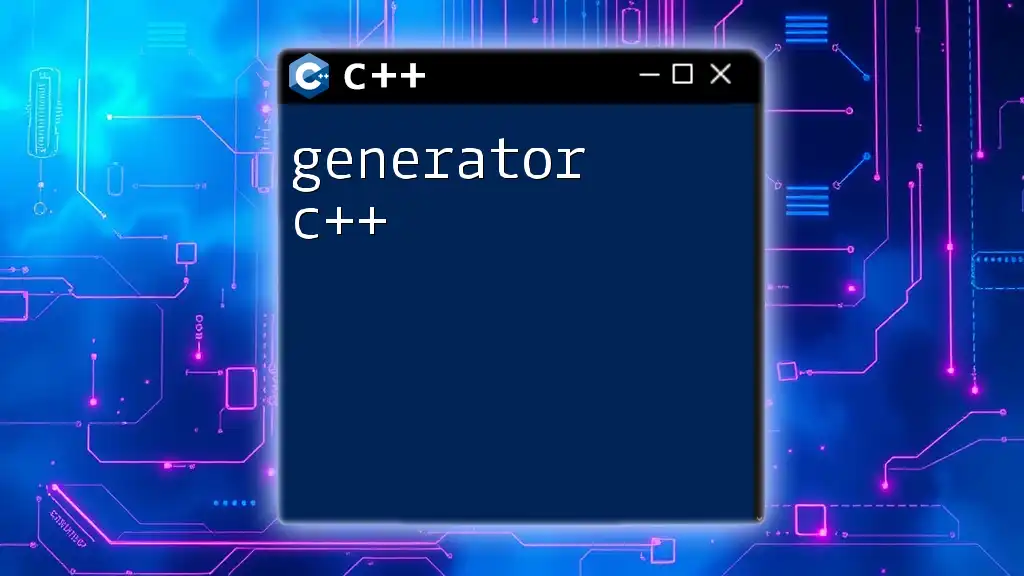
Preventing Overflow in C++
Using Safe Libraries and Alternative Data Types
One effective strategy for preventing overflow is to utilize safe libraries or data types. Libraries like Boost.Multiprecision allow for calculations with arbitrary-precision types, effectively eliminating the risk of overflow by allocating sufficient memory for large numbers.
Moreover, considering larger data types, such as `long long` instead of regular `int`, can provide a larger range, helping to mitigate overflow risks in scenarios where large numbers are involved.
Best Practices for Writing Overflow-Safe Code
To minimize the chances of overflow, the following best practices should be observed during coding:
- Validate inputs: Ensure that the data being processed is within acceptable limits before performing calculations.
- Use assertions: Implement assertions to check for conditions that might lead to overflow before operations are executed.
- Check bounds: Always perform bound checks before performing arithmetic operations that could exceed data type limits.
By adopting these practices, developers can significantly enhance the safety of their code.
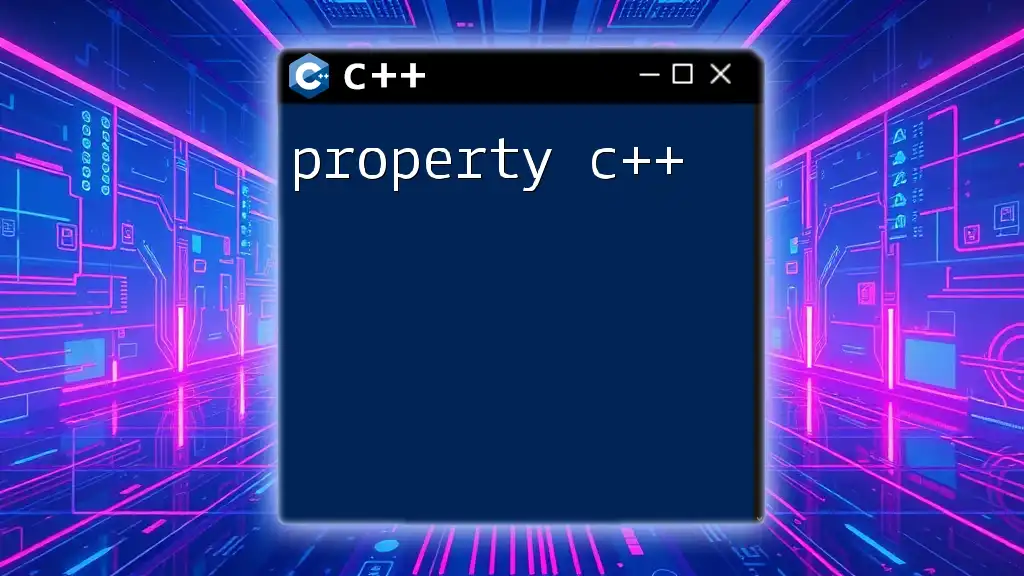
Overflow in Real-World Applications
Security Implications of Overflow
Overflow is not just a technical issue; it also poses serious security implications. Attackers understand that overflow vulnerabilities can lead to exploits—injecting malicious code, manipulating memory, or even causing denial-of-service attacks. Recognizing overflow as a potential attack vector is crucial for robust application security.
Case Study: Overflow Bugs in Software History
Historically, several high-profile security vulnerabilities have stemmed from overflow bugs. For example, the Morris Worm in 1988 exploited a buffer overflow in a widely used program, leading to significant system failures. Understanding such instances reinforces the need for vigilance regarding overflow handling.
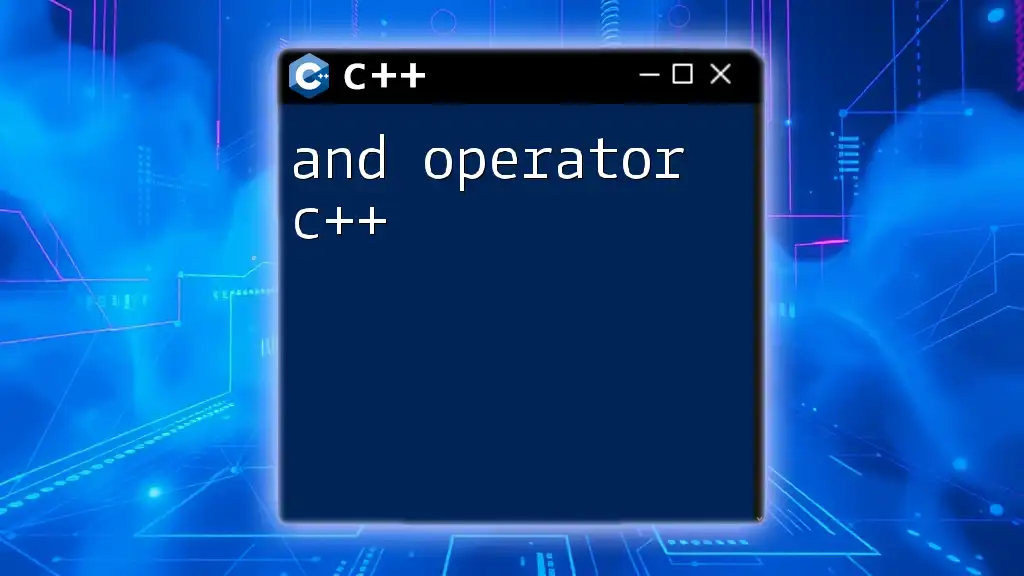
Conclusion
Recap of Key Points
In summary, understanding overflow in C++ is essential for developing reliable software. It is characterized primarily by integer and floating-point overflow, both of which can lead to undefined behavior if not adequately addressed. Developers must prioritize both static and runtime detection techniques, incorporate safe coding practices, and remain aware of the broader implications overflow can have on application security.
Final Thoughts
It is imperative for developers to commit to proactive overflow handling in all C++ applications. By prioritizing this in your coding practices, you will not only enhance the robustness of your software but also protect against the range of issues that can arise from oversights regarding overflow.
Suggested Further Reading
Explore resources such as documentation on C++ standards, books on software security, or online courses focusing on safe programming practices to deepen your understanding of overflow and its implications within C++.