`std::move` is a C++ utility that enables the transfer of ownership of resources from one object to another by converting an lvalue into an rvalue, thus allowing for efficient moves instead of expensive copies.
Here’s a simple example:
#include <iostream>
#include <utility> // for std::move
#include <vector>
class MyClass {
public:
MyClass(int size) : data(size) {
std::cout << "Constructor called\n";
}
// Move constructor
MyClass(MyClass&& other) noexcept : data(std::move(other.data)) {
std::cout << "Move constructor called\n";
}
private:
std::vector<int> data;
};
int main() {
MyClass obj1(10); // Constructor called
MyClass obj2(std::move(obj1)); // Move constructor called
return 0;
}
Understanding C++ Move Semantics
Move semantics is a powerful feature in C++ that allows for the transfer of resources from one object to another without incurring the overhead of copying. Unlike traditional copy semantics, which involves creating a duplicate of an object, move semantics enables programmers to "move" resources, such as dynamic memory, from one object and leave the original object in a valid but unspecified state. This is especially advantageous when dealing with large resources, thus ensuring better performance and efficient resource management.
Benefits of Using Move Semantics
Using move semantics enhances the performance of your programs in several ways:
- Reduced Overhead: Moving resources reduces the need for deep copying of large data structures, resulting in faster execution times.
- Better Resource Management: Move semantics allows for more effective management of dynamic memory, preventing resource leaks and fragmentation.
- Optimized Object Lifecycle: Transitioning resources using move semantics facilitates smoother object lifecycle management and reduces the complexity of constructors and destructors.
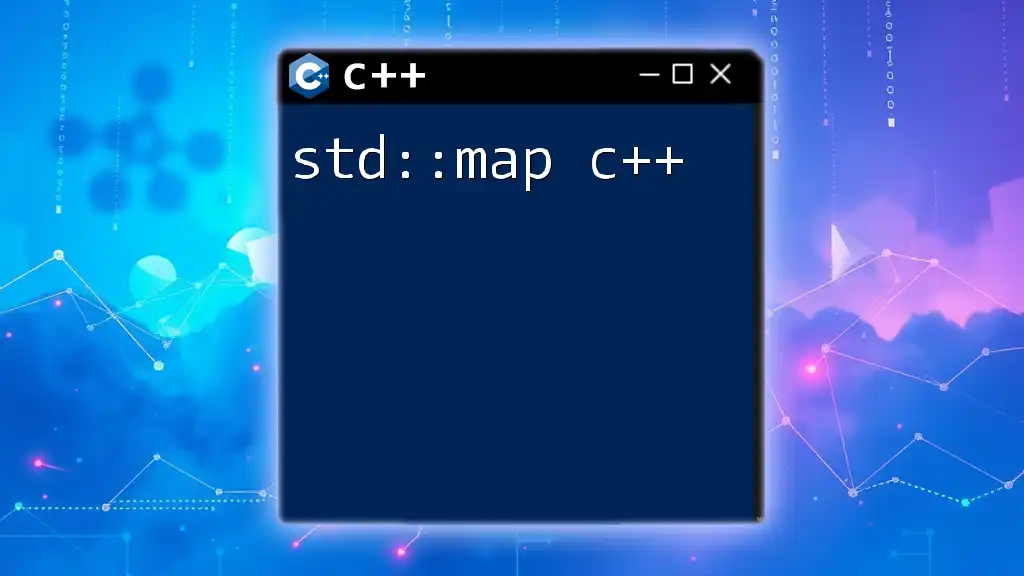
The Role of std::move
The `std::move` function is integral to implementing move semantics in C++. It casts an object to an rvalue reference, indicating that the object can be "moved" from.
Understanding `std::move`:
- Purpose: It enables the transfer of ownership of resources from one object to another.
- Function Signature: The function is defined as follows:
template <typename T>
typename std::decay<T>::type&& move(T&& t) noexcept;
- This function doesn’t actually move anything; rather, it casts the object to an rvalue, signaling that it can be moved from, thus delegates the actual move operation to the move constructor or move assignment operator of the object type.
Syntax and Usage of std::move
The syntax for using `std::move` is straightforward:
std::move(value);
When you pass an object to `std::move`, you're indicating that the object is an rvalue and that you are willing to let it be moved.
Value Categories: Lvalues vs Rvalues
To effectively utilize `std::move`, understanding value categories is crucial.
- Lvalues are objects that occupy identifiable locations in memory (e.g., variables).
- Rvalues are temporary objects that do not have a persistent state beyond the expression that uses them.
`std::move` allows you to treat lvalues as rvalues, facilitating the transfer of resources.
When to Use std::move
Using `std::move` is ideal in scenarios such as:
-
Returning Objects from Functions: Returning large objects by value can be significantly improved by moving them instead of copying.
-
Passing Objects to Functions by Value: Using `std::move` when passing arguments to functions can reduce overhead, especially in scenarios with extensive data.
Best Practices for Using std::move:
- Always ensure that the object you are moving from is either no longer needed or is in a valid, but unspecified state after the move operation.
- Avoid moving from an object that you will access afterward, as this leads to undefined behavior.
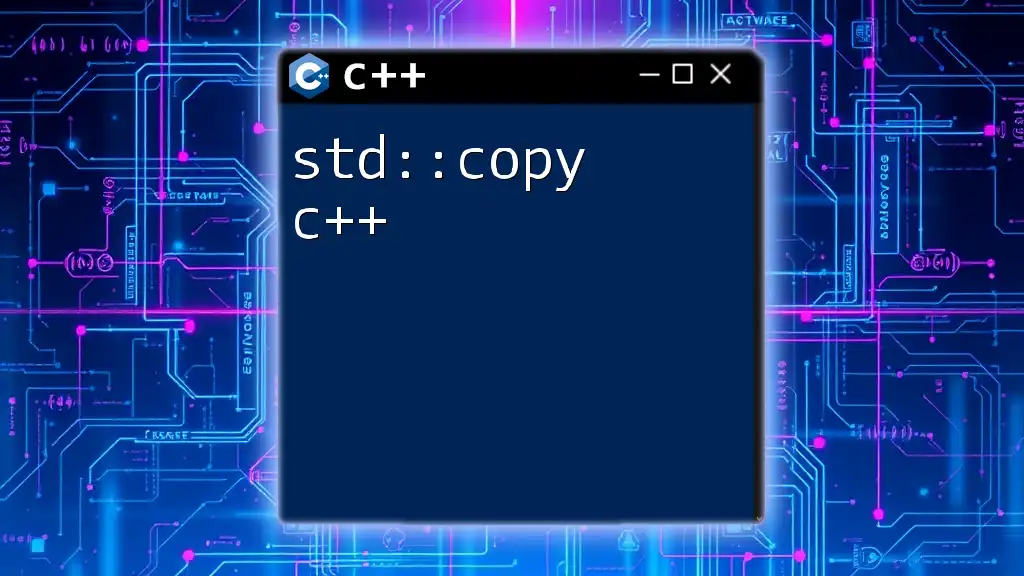
Example Implementations of std::move
Example 1: Moving Objects
Let’s consider a simple example with a class that has a move constructor:
#include <iostream>
#include <utility> // for std::move
class MyClass {
public:
MyClass(int data) : data_(data) { }
MyClass(MyClass&& other) noexcept : data_(other.data_) {
other.data_ = 0; // Leave 'other' in a valid but unspecified state
}
int data_;
};
int main() {
MyClass obj1(42);
MyClass obj2(std::move(obj1)); // Move obj1 into obj2
std::cout << "obj1 data: " << obj1.data_ << std::endl; // Outputs 0
std::cout << "obj2 data: " << obj2.data_ << std::endl; // Outputs 42
}
In this example, `obj1` is moved into `obj2`, transferring ownership of the resource. The move constructor takes care of transferring the `data_` member while leaving `obj1` in a valid but unspecified state where `data_` is set to 0.
Example 2: Move in Containers
You can also utilize `std::move` when working with containers in the Standard Template Library (STL). Here's how:
#include <iostream>
#include <vector>
#include <utility>
int main() {
std::vector<int> vec1 = {1, 2, 3};
std::vector<int> vec2 = std::move(vec1); // Move the contents of vec1 to vec2
std::cout << "vec1 size: " << vec1.size() << std::endl; // Outputs 0
std::cout << "vec2 size: " << vec2.size() << std::endl; // Outputs 3
}
Here, `vec1` is moved into `vec2`. After the move operation, `vec1` is left empty, and `vec2` holds all the elements. This operation avoids the potentially costly deep copy of the contents of the vector, demonstrating a core advantage of utilizing `std::move`.
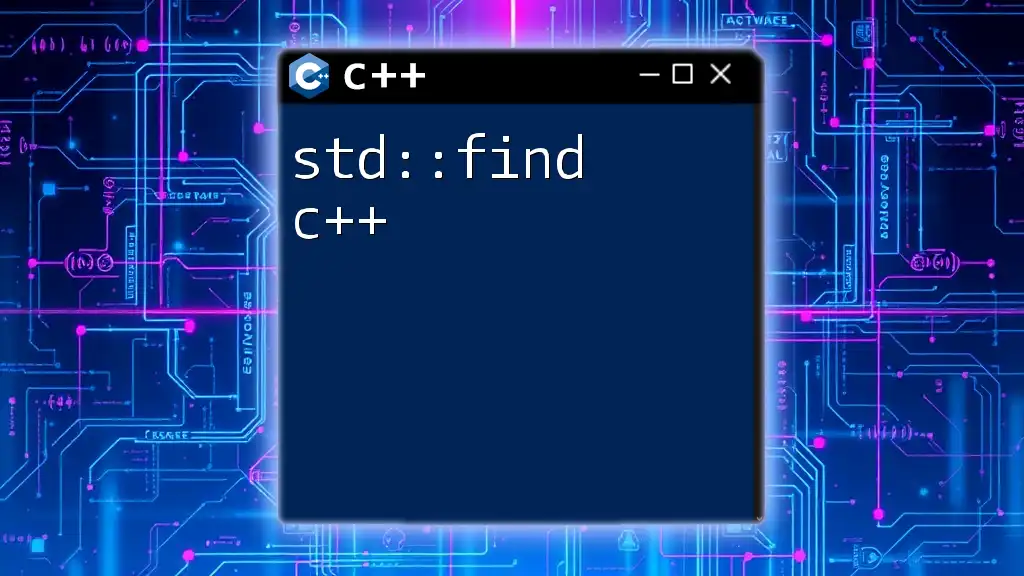
Potential Pitfalls of std::move
While `std::move` is incredibly beneficial, misuse can lead to problems:
-
Moving from a Still-in-Use Object: If you move an object that you later access, you might encounter undefined behavior.
-
Moved-From States: Objects that have been moved from should be treated carefully since they can be in a valid but unspecified state. Always avoid further access unless you're resetting the state.
-
Move-Only Types: Be cautious when designing classes that are move-only. While such types can lead to performance benefits, they can also complicate your design if not managed correctly.
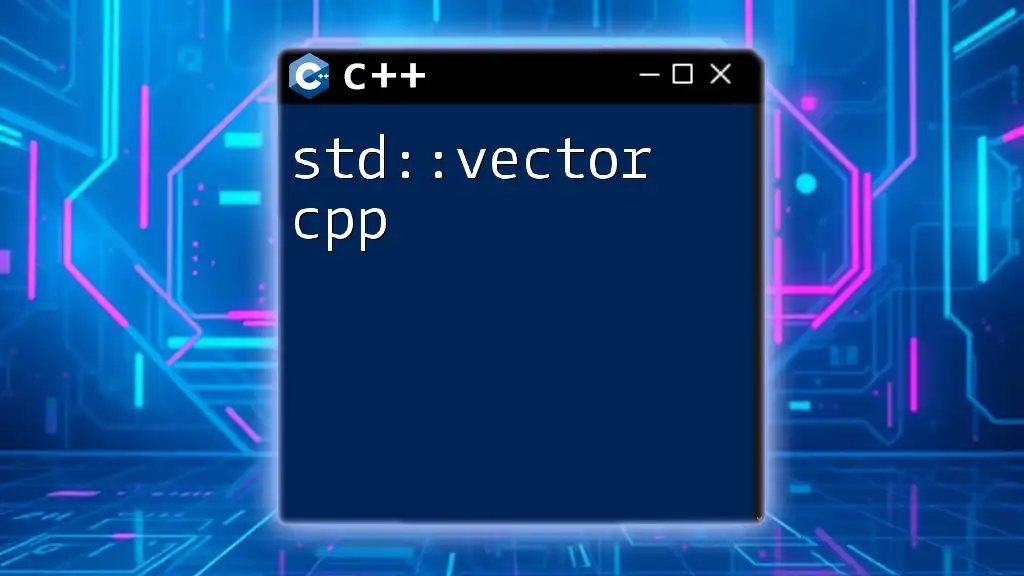
Summary and Conclusion
In conclusion, `std::move c++` is an essential tool in modern C++ for effective resource management and improved performance. By understanding and implementing move semantics alongside `std::move`, programmers can enhance their applications significantly, especially those that deal with large amounts of data or complex resources.
By adhering to best practices and understanding the significance of move semantics, you can harness the power of C++ to create efficient and optimized applications.
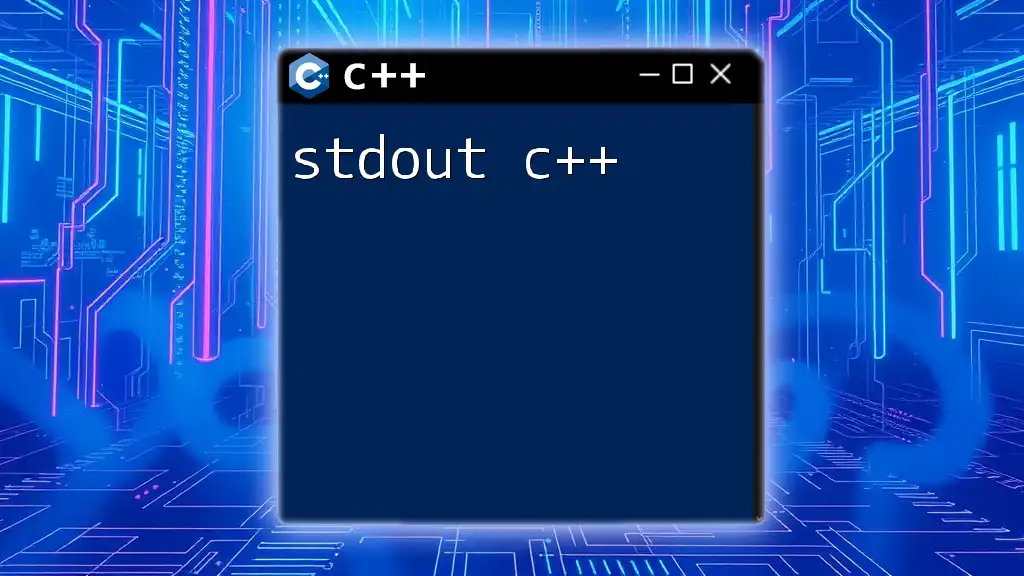
Additional Resources
To deepen your understanding of `std::move` and move semantics, consider checking the following resources:
- [cppreference - std::move](https://en.cppreference.com/w/cpp/memory/move)
- Books: "Effective Modern C++" by Scott Meyers, which contains insights on move semantics.
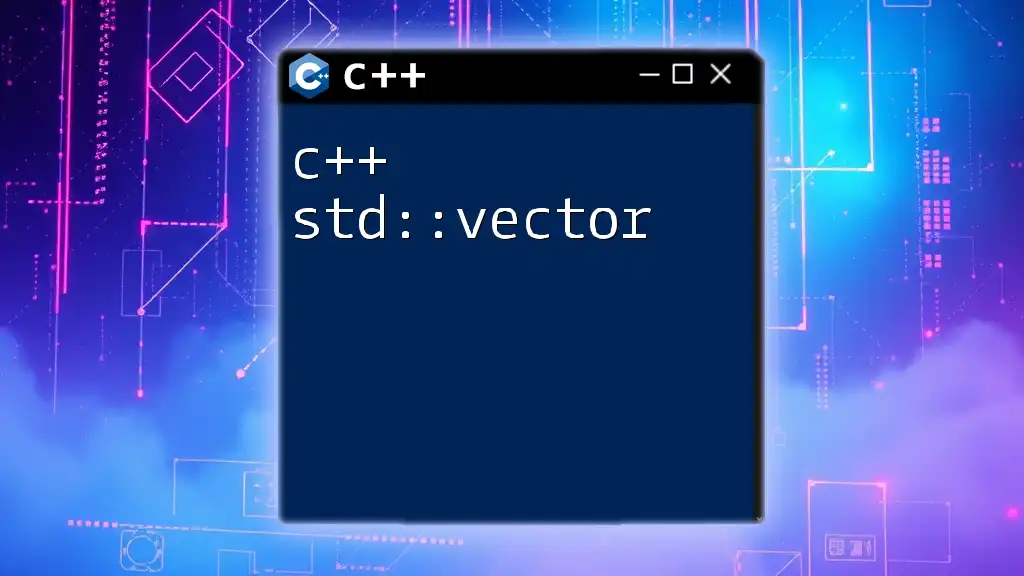
Call to Action
As you explore the intricacies of `std::move`, practice utilizing this feature in your own code. Implement the examples discussed, experiment with your classes, and engage with the community by sharing your experiences and questions!