`std::map` in C++ is a sorted associative container that stores key-value pairs, allowing for fast retrieval based on unique keys.
#include <iostream>
#include <map>
int main() {
std::map<std::string, int> ageMap;
ageMap["Alice"] = 30;
ageMap["Bob"] = 25;
for (const auto& pair : ageMap) {
std::cout << pair.first << ": " << pair.second << std::endl;
}
return 0;
}
Understanding std::map
Characteristics of std::map
Key-Value Pairs
In a `std::map`, data is stored in pairs, referred to as key-value pairs. Each key is unique, which means that if you try to insert a key that already exists in the map, it won't overwrite the existing value; instead, the insertion will fail. This uniqueness makes `std::map` ideal for situations where you need to quickly look up values based on a specific identifier.
Ordered Structure
A `std::map` maintains its elements in a sorted order based on the keys. This inherent ordering feature allows for efficient searching, insertion, and deletion, as well as the ability to easily traverse in sorted order. In contrast, a `std::set` also maintains unique elements but does not store them in key-value pairs.
Important Features
Associative Container
An associative container, like `std::map`, allows you to access elements via keys rather than indices. This feature streamlines the process of looking up values, making it efficient to find data without needing to traverse through an array or vector.
Automatic Sorting
When elements are inserted into a `std::map`, they are automatically sorted based on the comparator defined (by default, this is done using the `<` operator). This sorting ensures that you always know the order of elements, which is beneficial in numerous algorithms requiring sorted data.
Dynamic Size
The size of a `std::map` can grow and contract dynamically as elements are added or removed. This flexibility means that you don’t have to worry about the size of your data structure in advance; you can insert as many elements as needed without the overhead of manual memory management.
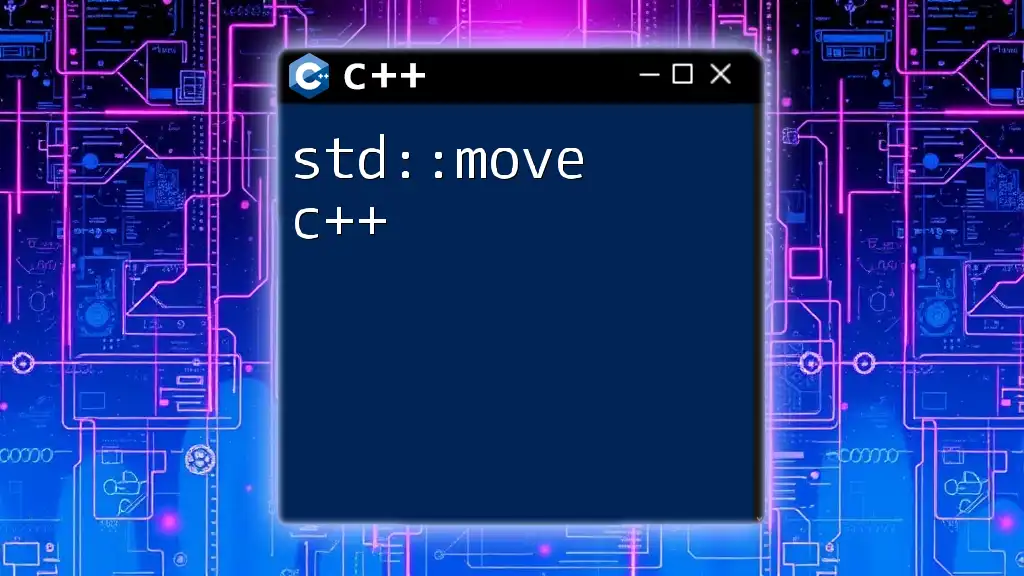
Basic Operations with std::map
Creating a std::map
To create a `std::map`, you need to specify the types for the keys and values. The basic syntax for declaring a map is:
std::map<KeyType, ValueType> mapName;
Example: Creating a simple map
std::map<int, std::string> idNameMap;
This line of code creates a map where the keys are of type `int` and the values are of type `std::string`.
Inserting Elements
To insert elements into a `std::map`, you have several methods at your disposal:
- Using `insert()`: The `insert()` function adds a key-value pair to the map. If the key already exists, the insertion will be ignored.
idNameMap.insert(std::make_pair(1, "Alice"));
- Using the subscript operator (`[]`): This method not only adds a new element if the key does not exist but also allows you to modify the value of an existing key.
idNameMap[2] = "Bob";
Accessing Elements
To retrieve values from a `std::map`, you can use:
- The subscript operator: This will either return the value associated with the key or insert a new key with a default value if the key does not exist.
std::cout << "ID 1: " << idNameMap[1] << std::endl; // Prints: Alice
- The `at()` method: This method provides access to the element at the specified key. It throws an exception if the key does not exist.
std::cout << "ID 2: " << idNameMap.at(2) << std::endl; // Prints: Bob
Erasing Elements
To remove elements from a `std::map`, you can use:
- `erase()`: This function takes a key and removes its associated key-value pair from the map. If the key does not exist, no operation is performed.
idNameMap.erase(1); // Removes the entry with key 1
- `clear()`: To remove all elements from the map, `clear()` can be used.
Iterating through std::map
Iterating over a `std::map` can be done using iterators, making it easy to access each key-value pair.
- Using Iterators: The standard iterator approach allows you to traverse through the map.
for (auto it = idNameMap.begin(); it != idNameMap.end(); ++it) {
std::cout << it->first << ": " << it->second << std::endl;
}
- Using Range-based For Loop: This method simplifies the syntax for iterating over a map.
for (const auto& pair : idNameMap) {
std::cout << "ID: " << pair.first << ", Name: " << pair.second << std::endl;
}
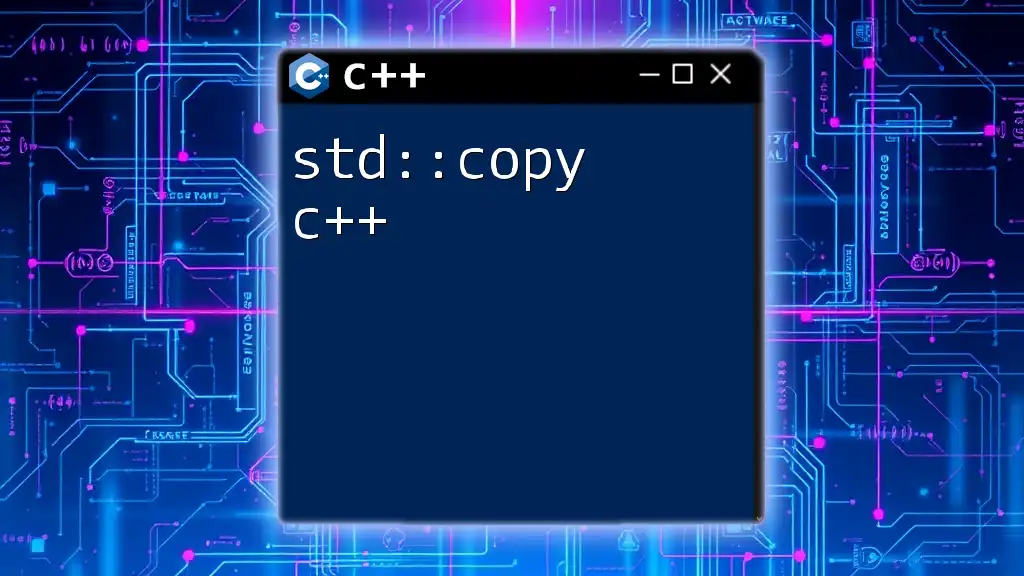
Advanced Features of std::map
Custom Sorting
While `std::map` uses a default sorting mechanism based on the keys, you can implement your own custom sorting logic by defining a comparator.
- Custom Comparator Example:
struct Compare {
bool operator()(const int& a, const int& b) const {
return a > b; // Sort in descending order
}
};
std::map<int, std::string, Compare> customMap;
In this case, the map will now sort elements in descending order based on the keys, thanks to the custom comparator.
Finding Elements
If you need to check for the presence of or retrieve an element without invoking an exception for a missing key, you can use the `find()` method.
auto it = idNameMap.find(1);
if (it != idNameMap.end()) {
std::cout << "Found: " << it->second << std::endl; // Key exists
}
If the key is found, `find()` returns an iterator pointing to that element; otherwise, it returns `mapName.end()`.
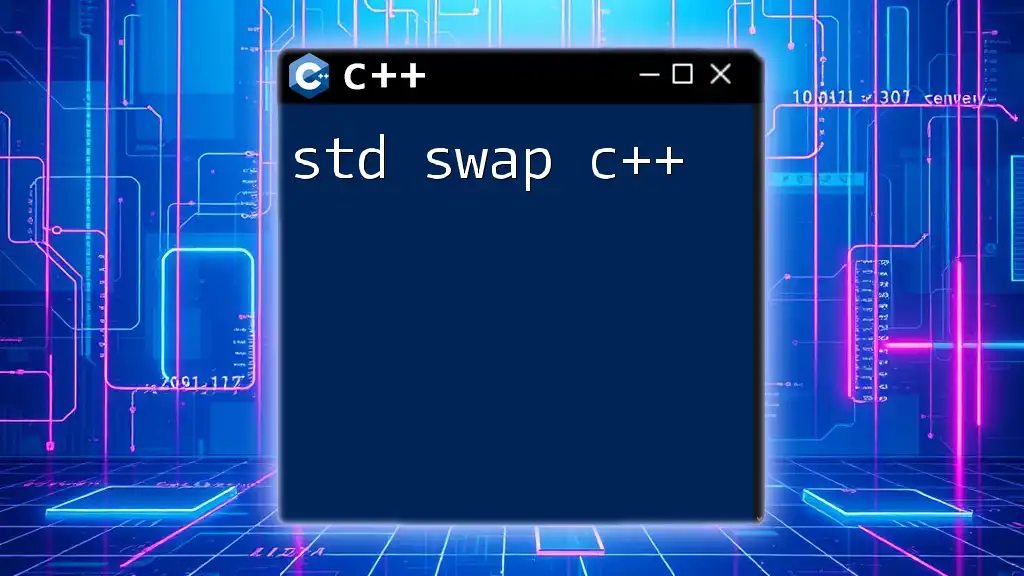
Performance Considerations
Time Complexity
Understanding the time complexities associated with operations in `std::map` is critical for efficient software design. The average time complexity for insertion, deletion, and access is O(log n) due to the underlying balanced binary tree structure of a map. The worst-case scenario remains O(log n) since it uses a self-balancing tree.
Memory Usage
`std::map` tends to consume more memory than `std::unordered_map` due to the overhead of maintaining sorted order and pointers for each element in its tree structure. Knowing when to use each is essential for optimizing both performance and memory usage.
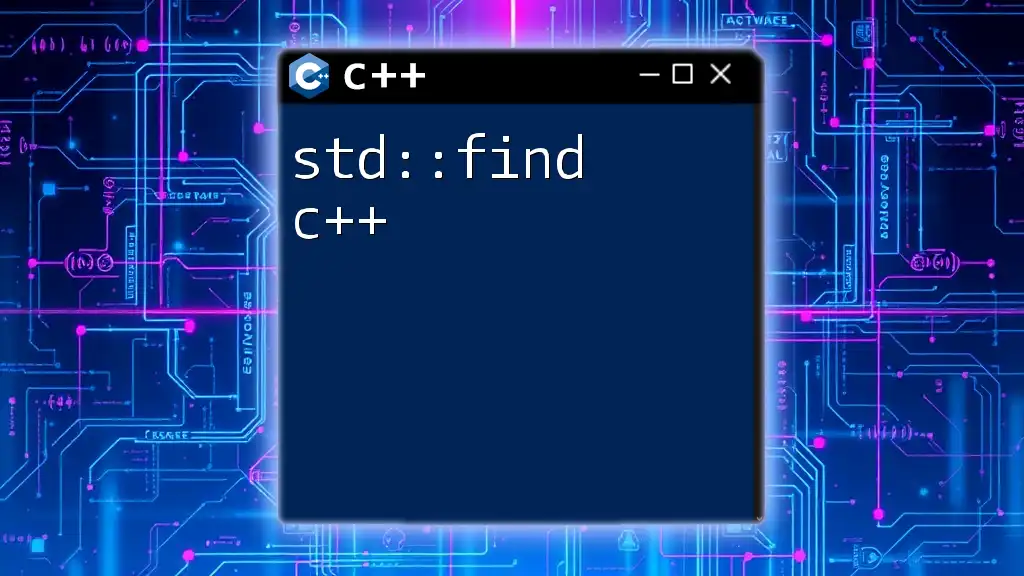
Common Use Cases
When to Use std::map
`std::map` is particularly useful in scenarios where:
- Associative Lookup is Required: When you need to access data via a key rather than an index, such as storing configuration settings or user preferences.
- Ordering is Important: If your application requires sorted output, using a `std::map` simplifies this process, ensuring you always have ordered data.
Practical Applications
A common application of `std::map` includes counting word frequencies or managing user information.
Example: Counting Frequencies
std::map<std::string, int> wordCount;
// Sample code to populate the wordCount map
std::string text = "hello world hello";
std::istringstream iss(text);
std::string word;
while (iss >> word) {
wordCount[word]++;
}
In this example, `wordCount` stores the frequency of each word found in the given string, demonstrating how `std::map` can efficiently manage associative data.
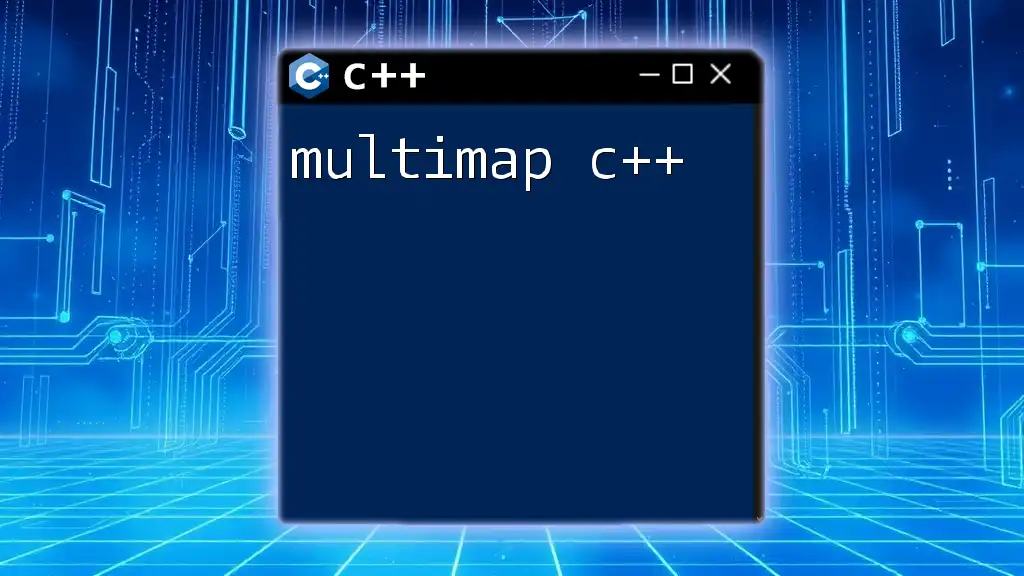
Conclusion
Understanding the power of `std::map` in C++ allows you to handle complex data manipulation with ease. From creating associative containers to advanced features like custom sorting and efficient searching, `std::map` serves as a versatile tool in a C++ programmer's toolkit. As you grow more familiar with its operations, you can leverage its capabilities in countless applications, enhancing both your coding experience and software performance.
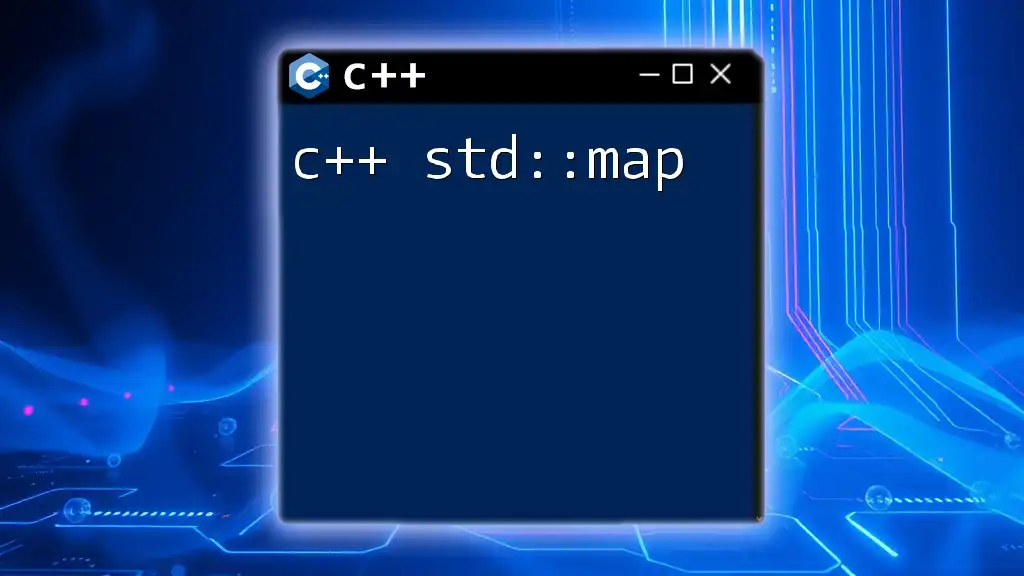
Further Resources
For a more in-depth understanding, consider exploring further tutorials and the official C++ documentation. Practice is the key to mastery, so dive into code samples and challenges to get more comfortable with `std::map` in your projects.