The `std::max` function in C++ returns the larger of two specified values, which can be used with various data types.
Here’s a simple code snippet demonstrating its usage:
#include <iostream>
#include <algorithm> // for std::max
int main() {
int a = 5, b = 10;
std::cout << "The maximum value is: " << std::max(a, b) << std::endl; // Outputs 10
return 0;
}
Understanding the Basics of std::max
`std::max` is a standard library function that helps you determine the maximum of two values. Its utility in everyday C++ programming cannot be overstated, as it offers a succinct way to perform comparisons between values without the verbosity of traditional control structures. This function is part of the C++ standard library and can be used with a wide variety of data types, making it an essential tool for developers.
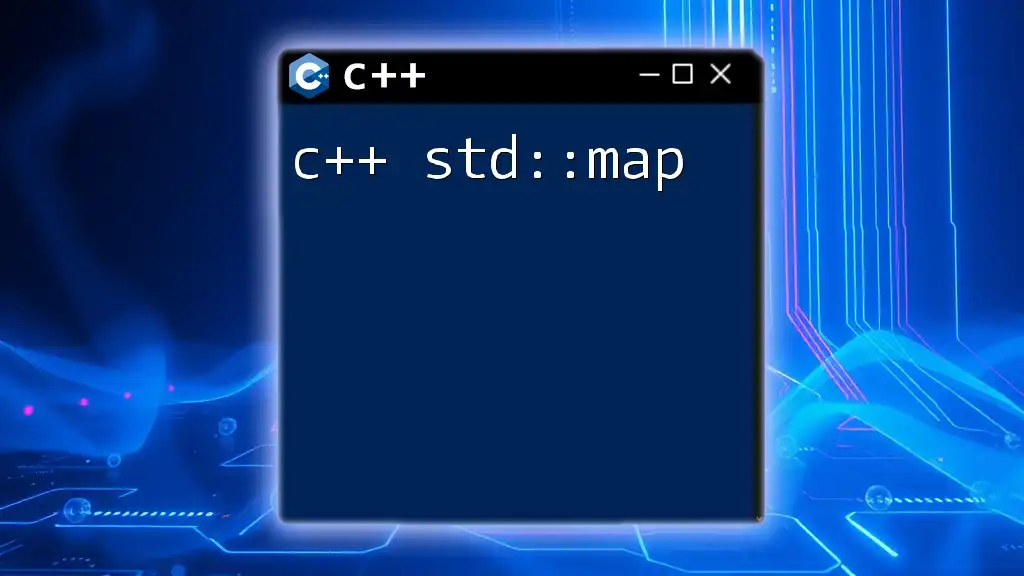
The Syntax of std::max
The basic syntax for `std::max` is as follows:
std::max<T>(a, b);
In this structure:
- `<T>` denotes the type of the arguments `a` and `b`.
- `a` and `b` are the two values you want to compare.
- The function returns the larger of the two values, with the return type being the same as the type of the arguments passed.
This clarity in syntax makes it easy to incorporate into various types of C++ projects.
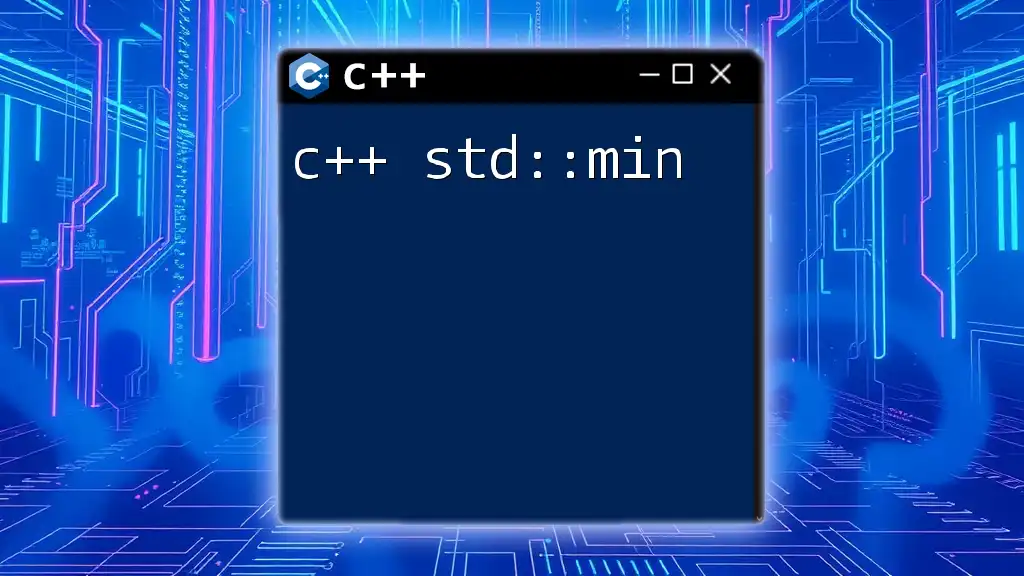
Using std::max with Different Data Types
`std::max` can be utilized with multiple data types, including integers, floating-point numbers, and even characters. Here are some examples to illustrate its versatility:
std::max with Integers
Consider the following example:
int a = 5, b = 10;
int maximum = std::max(a, b);
In this case, the `maximum` variable will hold the value `10`, being the larger of the two integers. This showcases how straightforward it is to obtain a maximum value in a single line of code.
std::max with Floating-point Numbers
Similarly, `std::max` works seamlessly with floating-point numbers:
double x = 5.5, y = 3.3;
double maxVal = std::max(x, y);
Here, `maxVal` will receive the value `5.5`. Notably, `std::max` retains the precision of floating-point calculations, making it a reliable option for numerical comparisons.
std::max with Characters
Interestingly, `std::max` can also compare characters based on their ASCII values:
char char1 = 'a', char2 = 'z';
char maxChar = std::max(char1, char2);
In this snippet, `maxChar` ends up storing the character `'z'`, which has a higher ASCII value compared to `'a'`. This demonstrates the utility of `std::max` beyond just numeric types.
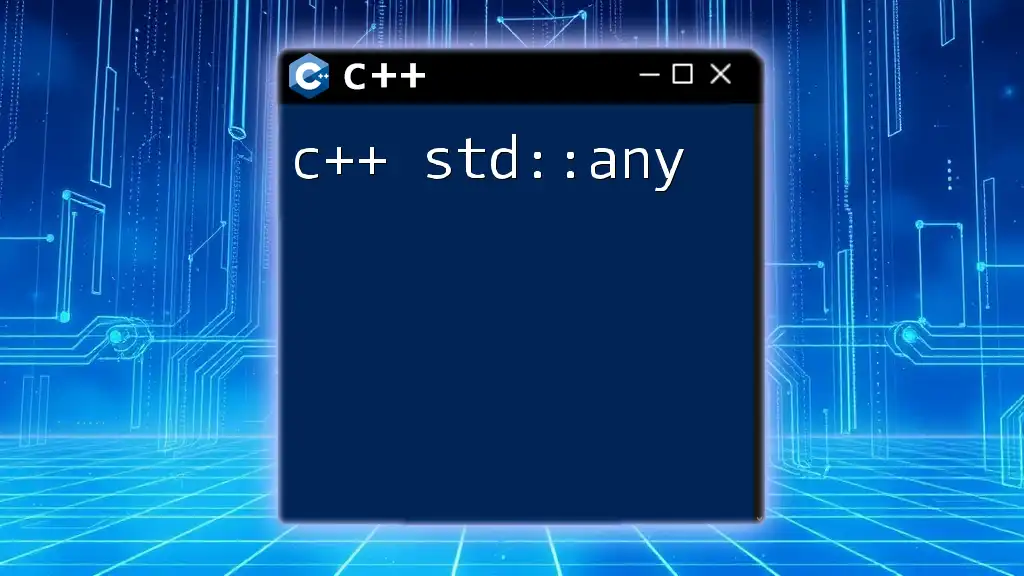
Comparing std::max with Other Approaches
When evaluating the efficacy of `std::max`, it’s helpful to compare it with traditional if-else statements:
int max;
if (a > b) {
max = a;
} else {
max = b;
}
While this method is effective, it’s significantly more verbose than using `std::max`. The simplicity and conciseness of `std::max` not only improve code readability but also make it less error-prone.
Performance Analysis of std::max vs Custom Functions
Using `std::max` often results in improved performance, as it reduces the overhead associated with writing custom maximum functions or verbose if-else constructs. Generally, `std::max` is optimized and tested for various scenarios, making it a preferable choice for developers aiming for efficiency.
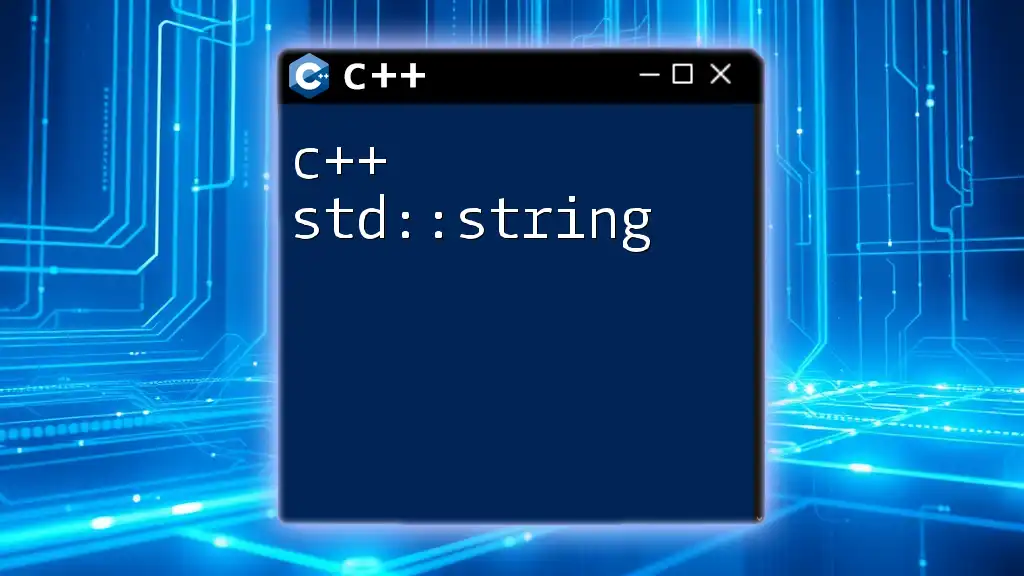
Working with std::max in Complex Scenarios
`std::max` can be employed in more complex scenarios, such as comparing elements in an array or dealing with objects that have custom comparisons.
Using std::max with Arrays
A common scenario involves obtaining the maximum element from an array. You can combine `std::max` with `std::max_element` as shown below:
#include <algorithm>
int arr[] = {1, 3, 5, 7};
int maxElement = *std::max_element(arr, arr + 4);
In this example, the function `std::max_element` traverses the array to identify the maximum element, which is then dereferenced to get the value. This method illustrates the power of the standard library in simplifying complex tasks.
Using std::max with Objects
You can even overload operators in a class to use `std::max` meaningfully with objects. Here’s a simple illustration:
class Box {
int volume;
public:
Box(int v) : volume(v) {}
bool operator<(const Box &b) const {
return volume < b.volume;
}
};
Box box1(10), box2(20);
Box maxBox = std::max(box1, box2);
In this example, we define a `Box` class with an overloaded `<` operator to compare based on volume. This allows for intuitive use of `std::max` to get the box with the greater volume.
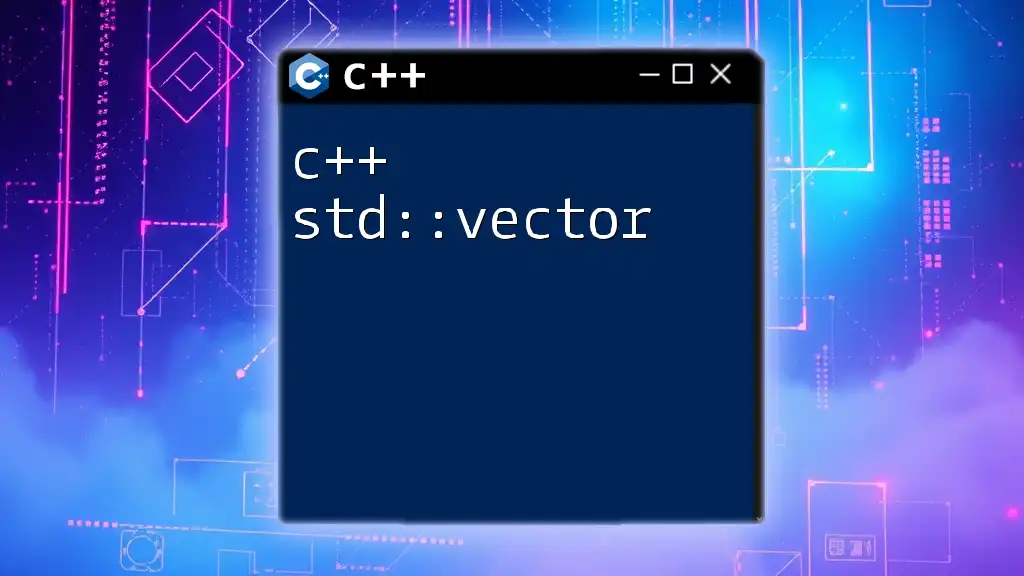
Common Pitfalls to Avoid with std::max
While using `std::max` can be very convenient, it has some pitfalls.
- Misunderstandings with Data Types: Ensure the data types of the two arguments passed are the same. If they differ, you may run into unexpected behavior or type conversions that lead to errors.
- Using Arguments of Different Types: Mixing types can result in unintended results, so always ensure consistency.
- Performance Implications of Complex Comparisons: Overly complicated comparison functions can inhibit the performance benefits that `std::max` provides. Strive for simplicity and clarity.
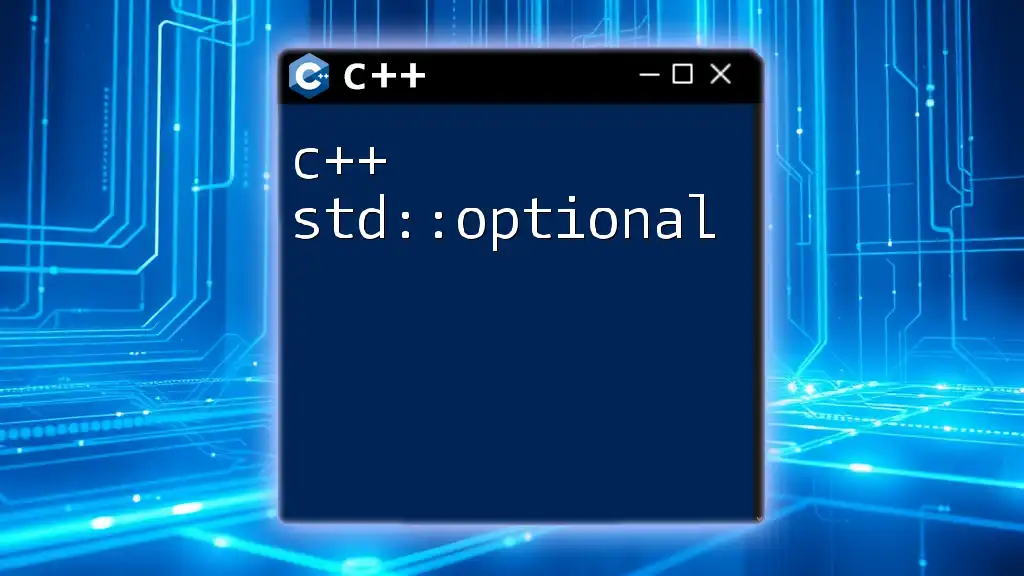
Best Practices for Using std::max
-
Utilize std::max in Templates: Implementing `std::max` in template functions can significantly enhance code reusability. By leveraging templates, your implementations can handle various data types without additional code.
-
Guidelines for Readability and Maintainability: Always prioritize readability and maintainability in your code. Using `std::max` can make your code cleaner and easier for others to read and understand.
-
Recommendations on When to Use std::max: Use `std::max` whenever you require a simple comparison between two values. It’s particularly useful in algorithms where maximum value retrieval is required.
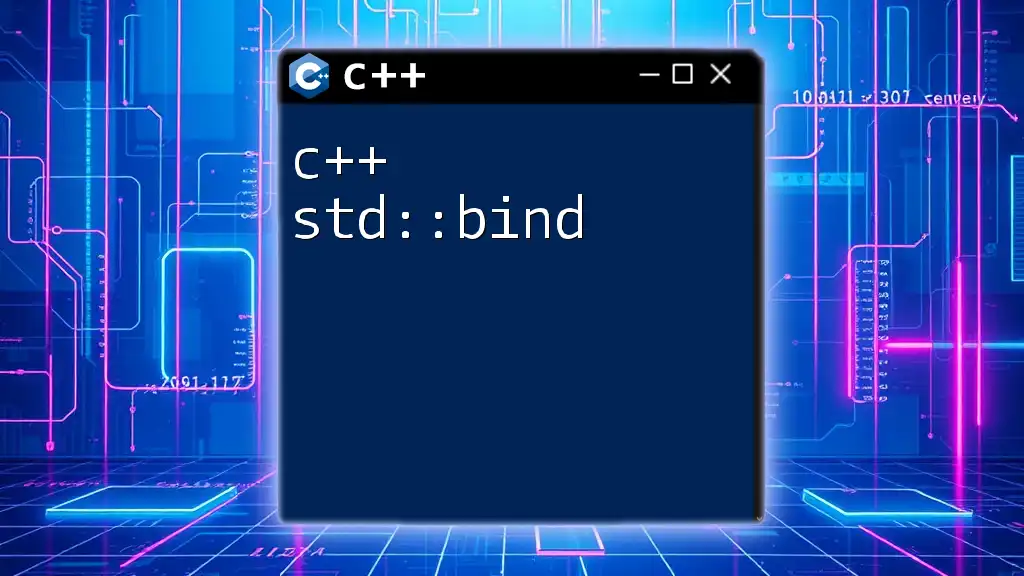
Conclusion
In summary, `c++ std::max` is not just a function but a powerful tool in your C++ programming arsenal. Its concise syntax and ability to handle various data types make it a staple in data comparison tasks. Embracing `std::max` can significantly enhance both the readability and efficiency of your code. I encourage you to practice using `std::max` in your projects and witness the ease and clarity it brings to your coding experience.