`std::span` is a lightweight, non-owning view over a contiguous sequence of elements that provides a safe way to access an array or a range of elements in C++.
#include <span>
#include <iostream>
void printArray(std::span<int> arr) {
for (int value : arr) {
std::cout << value << " ";
}
}
int main() {
int myArray[] = {1, 2, 3, 4, 5};
printArray(myArray);
return 0;
}
Understanding c++ std span
Definition of c++ std span
`std::span` is a versatile and lightweight reference wrapper designed to provide a view into a contiguous block of data such as arrays and standard containers. Unlike raw pointers, which can lead to complex memory management and potential pitfalls, `std::span` abstracts these concerns by acting as a type-safe means of accessing array elements without owning the data itself. This feature allows developers to write cleaner and more maintainable code.
Key Features of c++ std span
No ownership semantics: One of the standout features of `std::span` is its lack of ownership of the data it references. This means that you don't have to worry about the memory being freed unexpectedly or leaks occurring since `std::span` does not manage the underlying data.
Compile-time and runtime flexibility: `std::span` can be initialized with arrays of fixed size and also with dynamically-sized containers. This flexibility ensures that your code remains efficient without compromising type-safety or performance. For instance, you can easily pass a standard vector to a function as a `std::span` without copying the entire vector.
Size and bounds checking: `std::span` provides simple methods for determining the size of the underlying data and allows bounds checking, enhancing safety. You can easily obtain the size with the `size()` function and use the `empty()` method to check if the span contains any elements, preventing potential out-of-bounds access.

Creating and Using c++ std span
How to Create a c++ std span
Creating a `std::span` is straightforward. Here's how you can create a span from a C-style array:
#include <span>
void example() {
int arr[] = {1, 2, 3, 4, 5};
std::span<int> mySpan(arr); // Creating a span
}
In this example, `mySpan` can be used to access all elements in `arr` without any additional overhead.
You can also create a `std::span` from a `std::vector`:
#include <vector>
#include <span>
void example() {
std::vector<int> vec = {1, 2, 3, 4, 5};
std::span<int> mySpan(vec.data(), vec.size()); // Creating a span from vector
}
This flexibility means you can easily interact with different data structures without the need for custom wrapper functions.
Accessing Elements in c++ std span
Accessing elements in a `std::span` can be done using the indexing operator, similar to how you would access elements in an array:
int value = mySpan[2]; // Accesses the third element (value will be 3)
It is important to note that you can create a constant span if you want to prevent modification of the data:
const std::span<const int> constSpan(arr);
int constValue = constSpan[2]; // Read-only access
This ensures that your span can be safely passed to functions that should not modify the underlying data.
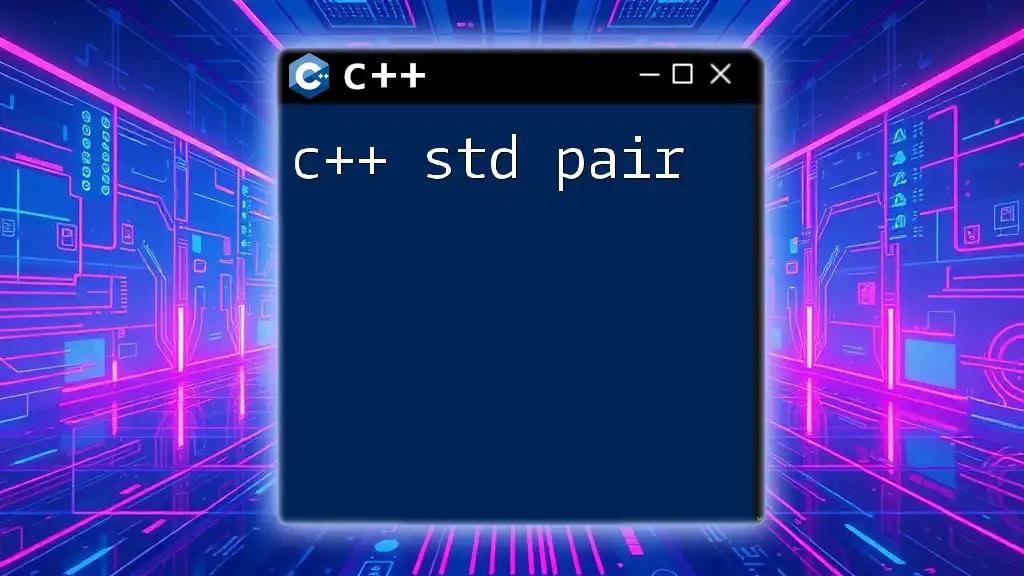
The Benefits of Using c++ std span
Memory Safety
`std::span` enhances memory safety by providing a layer of abstraction over raw pointers. This prevents common errors such as dangling pointers, as it does not manage the underlying data but rather provides a view. For example, consider the following code snippet with raw pointers:
void processArray(int* arr, size_t size) {
for (size_t i = 0; i < size; ++i) {
// Process each element
}
}
Using `std::span`, we can make this safer by eliminating potential size mismatches:
void processSpan(std::span<int> sp) {
for (auto& elem : sp) {
// Process each element
}
}
This approach inherently encapsulates size information, reducing the risk of buffer overflows.
Simplified Function Signatures
One of the significant advantages of using `std::span` is the simplified function signatures when passing arrays or other collections. Instead of passing pointers and sizes separately, you can encapsulate the data in a single, type-safe parameter. For instance:
void printSpan(std::span<int> sp) {
for (const auto& value : sp) {
std::cout << value << " ";
}
}
This clarity not only reduces cognitive load for reading and maintaining code but also makes it less error-prone.
Performance Considerations
Performance is a critical aspect of any application, and `std::span` optimizes this by avoiding unnecessary copies while still providing access to the data. When you pass a `std::span` to a function, you are simply passing a lightweight reference, ensuring that performance remains high.
For example, consider this function that operates on a span and sorts its elements:
#include <algorithm>
void sortSpan(std::span<int> sp) {
std::sort(sp.begin(), sp.end());
}
Here, the call to `std::sort` operates directly on the span without needing to copy the underlying data.

Common Use Cases for c++ std span
Passing Dynamic Arrays to Functions
`std::span` excels at allowing developers to pass dynamic arrays to functions seamlessly. This ability reduces the need for adapting function signatures every time you deal with different data structures. Instead of using separate overloads for `std::vector`, raw arrays, and other types, simply use `std::span`.
Working with Subarrays
Creating subarrays is straightforward with `std::span` thanks to the `subspan` method. This method allows you to create a new span that refers only to a portion of the original data:
std::span<int> subSpan = mySpan.subspan(1, 3); // View middle three elements
In this case, `subSpan` will reference elements {2, 3, 4} from `mySpan`, making it easy to operate on just a section of your data without copying.
Integrating with Standard Library Algorithms
`std::span` is compatible with standard library algorithms, allowing for elegant and efficient data manipulation. For example, you can leverage algorithms like `std::for_each` or `std::sort` directly on spans:
std::vector<int> vec = {5, 3, 2, 8, 1};
std::span<int> mySpan(vec.data(), vec.size());
std::sort(mySpan.begin(), mySpan.end());
This integration combines the strengths of both `std::span` and the STL, promoting a modern C++ coding style.
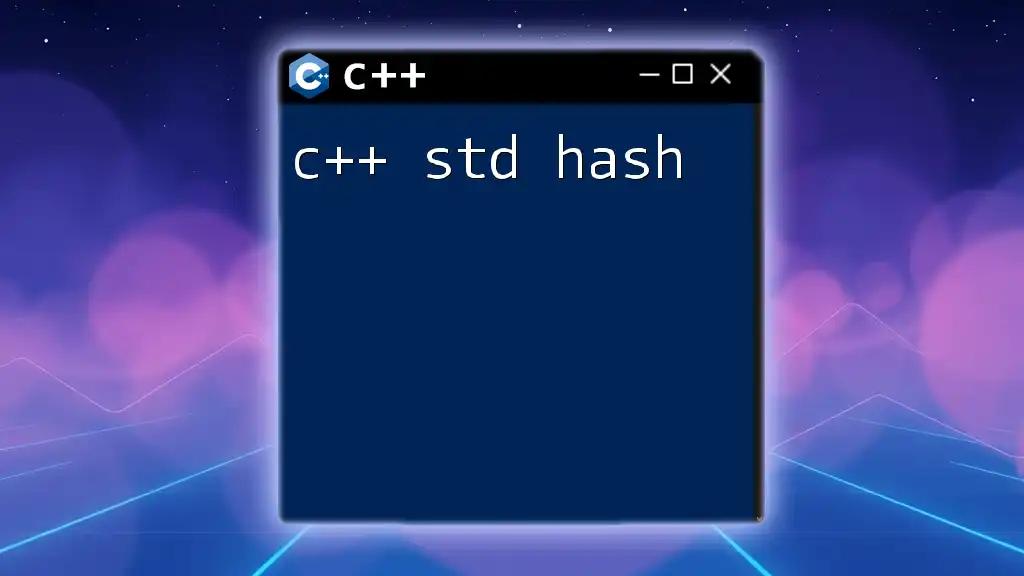
Limitations of c++ std span
No Ownership of Data
While the lack of ownership can be a significant advantage, it's important to understand its limitations. Since `std::span` does not manage the underlying data, you must be vigilant about the lifetime of the data it references. If the underlying data goes out of scope, the span becomes invalid.
Element Type Constraints
`std::span` requires a contiguous block of memory, which means it isn't suitable for all container types. You can only use it with arrays, `std::vector`, `std::array`, and similar structures. Non-contiguous data structures, such as linked lists or sets, cannot be directly represented as a `std::span`.
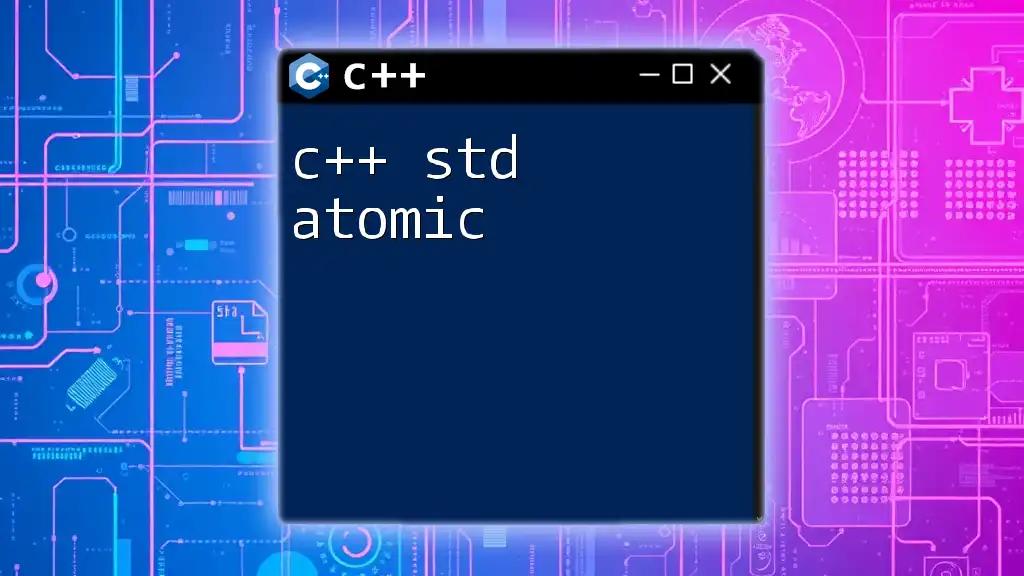
Conclusion
In summary, `c++ std span` is a powerful tool that encapsulates a view into contiguous data structures, simplifying memory management, enhancing safety, and improving performance. By incorporating `std::span` into your C++ projects, you can write cleaner, safer, and more efficient code. Embrace the benefits of `std::span` and explore its applications in your codebase, contributing to more modern and robust C++ programming practices.

Further Reading
For further exploration of `std::span` and modern C++ practices, consider visiting the official C++ standard library documentation or engaging with literature focused on C++ developments. Understanding these resources will give you a fuller grasp of how and when to effectively use `std::span` in your programming endeavors.