The C++ `std::stack` is a container adaptor that provides a LIFO (last-in, first-out) data structure, allowing you to push and pop elements efficiently.
Here’s a simple example demonstrating its use:
#include <iostream>
#include <stack>
int main() {
std::stack<int> s;
s.push(1);
s.push(2);
s.push(3);
std::cout << "Top element: " << s.top() << std::endl; // Outputs: Top element: 3
s.pop();
std::cout << "Top element after pop: " << s.top() << std::endl; // Outputs: Top element after pop: 2
return 0;
}
Understanding Stacks
A stack is a fundamental data structure that operates on the principle of Last In, First Out (LIFO). This means that the last element added to the stack will be the first one to be removed. Real-world analogies, such as a stack of plates or a pile of books, illustrate how this structure works—only the top item is accessible without disturbing the other items below it. Stacks are extensively used in programming for various purposes, including managing function calls, implementing undo functionalities in applications, and parsing expressions.
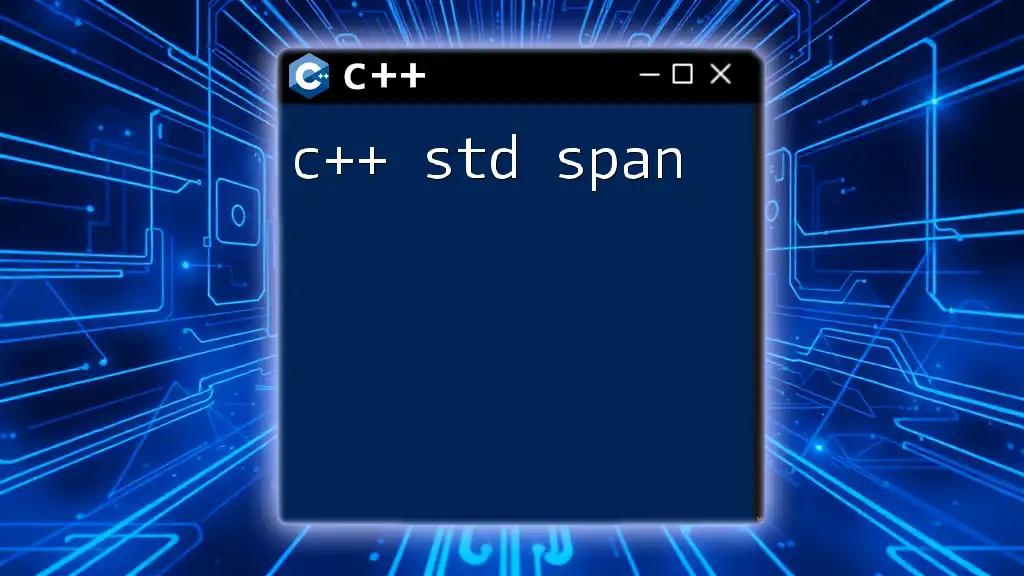
C++ std::stack Overview
The C++ Standard Library provides a convenient and efficient implementation of the stack data structure through `std::stack`. Using `std::stack` simplifies stack operations compared to creating a custom stack implementation from scratch. It encapsulates the core functions of a stack and relies on an underlying container, enabling developers to focus on their logic without managing memory and other complexities.
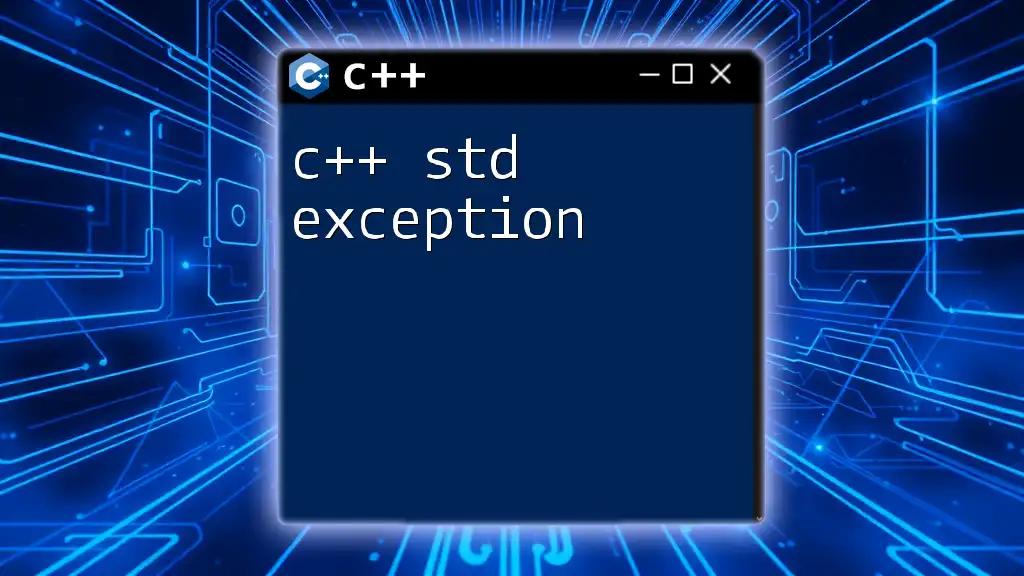
Creating and Using std::stack
Including the Required Header
To utilize `std::stack`, you need to include the appropriate header file:
#include <stack>
Declaration of std::stack
You can declare a stack by specifying the data type it will hold. For example, to create a stack of integers, use the following syntax:
std::stack<int> intStack;
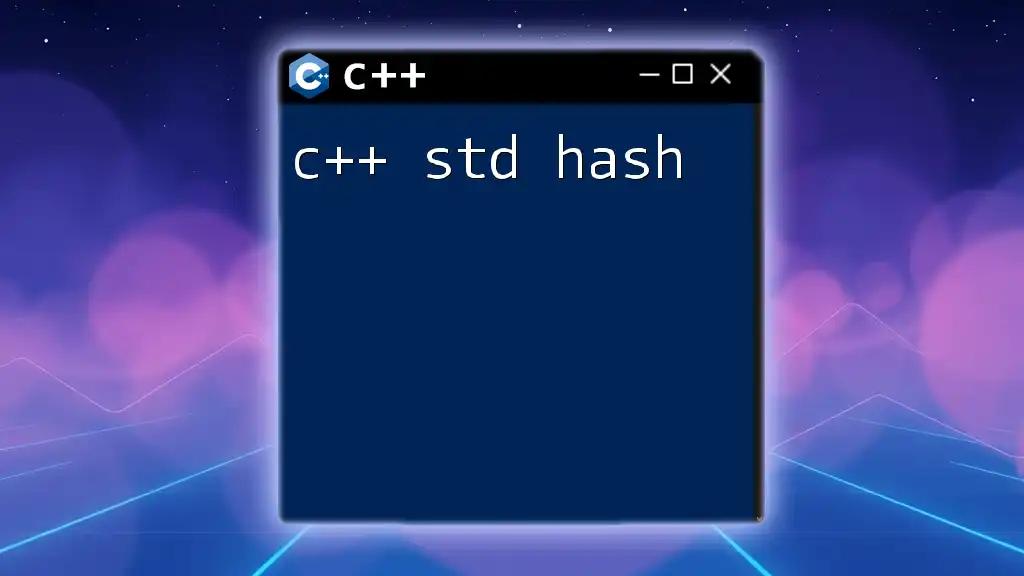
Basic Operations of std::stack
Pushing Elements onto the Stack
The `push` operation allows you to add elements to the top of the stack. This is essential when you want to build up your stack:
intStack.push(1);
intStack.push(2);
intStack.push(3);
Popping Elements from the Stack
The `pop` operation removes the top element from the stack. It’s important to understand that this operation does not return the value being removed; it simply deletes it from the stack. For instance:
intStack.pop(); // This will remove the top element (3)
Accessing the Top Element
To retrieve the top element without removing it from the stack, use the `top()` function. This operation is essential for inspecting the most recently added element:
int topElement = intStack.top(); // Retrieves the top element (2)
Checking if the Stack is Empty
Before performing operations like `pop()` or `top()`, it's crucial to check if the stack is empty using the `empty()` method. Accessing an element of an empty stack can lead to runtime errors:
if (intStack.empty()) {
std::cout << "Stack is empty";
} else {
std::cout << "Stack is not empty";
}
Size of the Stack
To find out how many elements are currently stored in the stack, you can use the `size()` method:
std::cout << "Current stack size: " << intStack.size();
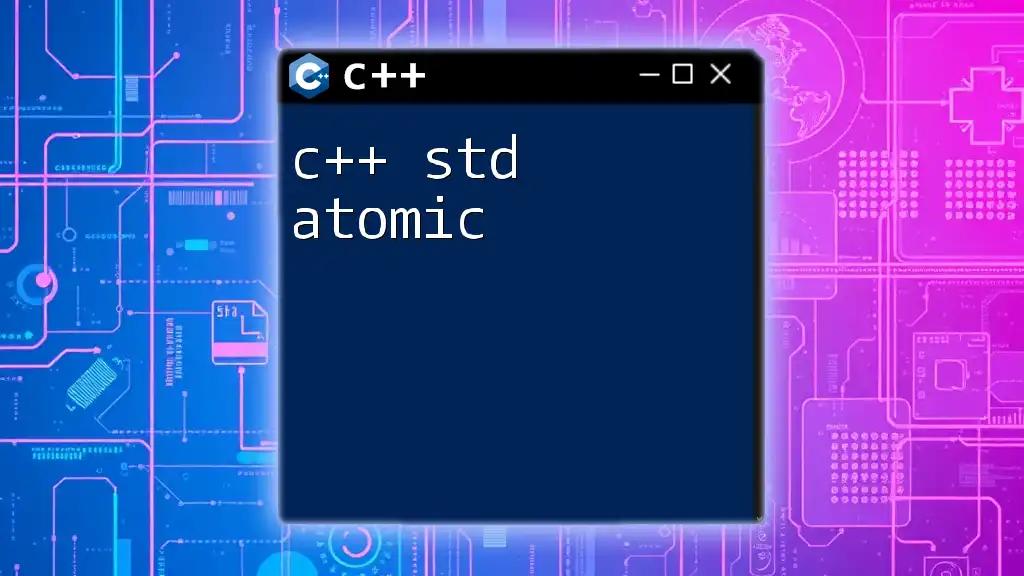
Iterating Through the Stack
One challenge with `std::stack` is that it does not support direct iteration over its elements due to its restricted access. However, you can create a temporary stack to access its elements. This method allows you to store and manage stack elements safely:
std::stack<int> tempStack;
while (!intStack.empty()) {
tempStack.push(intStack.top());
intStack.pop();
}
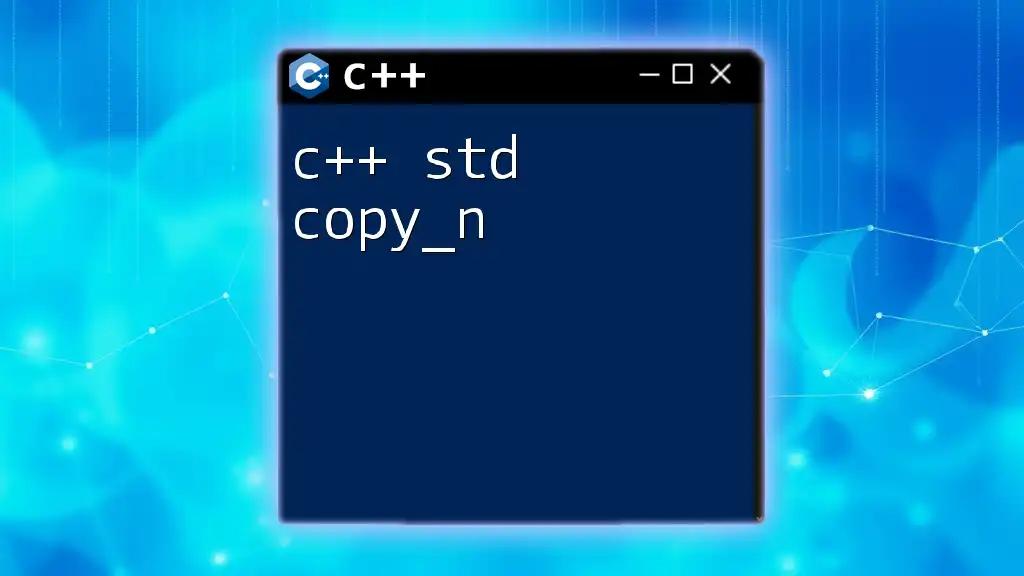
Advanced Usage of std::stack
Using std::stack with Custom Types
Besides basic data types, `std::stack` can manage user-defined types as well. For instance, let’s say you want to create a stack to hold `Person` objects:
struct Person {
std::string name;
int age;
};
std::stack<Person> personStack;
This capability allows you to utilize the stack structure for more complex data management tasks.
Stack Operations with Other STL Containers
While the default underlying container for `std::stack` is `std::deque`, you can also use other containers, like `std::vector` or `std::list`. Here’s how to declare a stack using `std::deque`:
std::stack<int, std::deque<int>> dequeStack;
This flexibility lets you select the best-performing container based on your application’s needs.
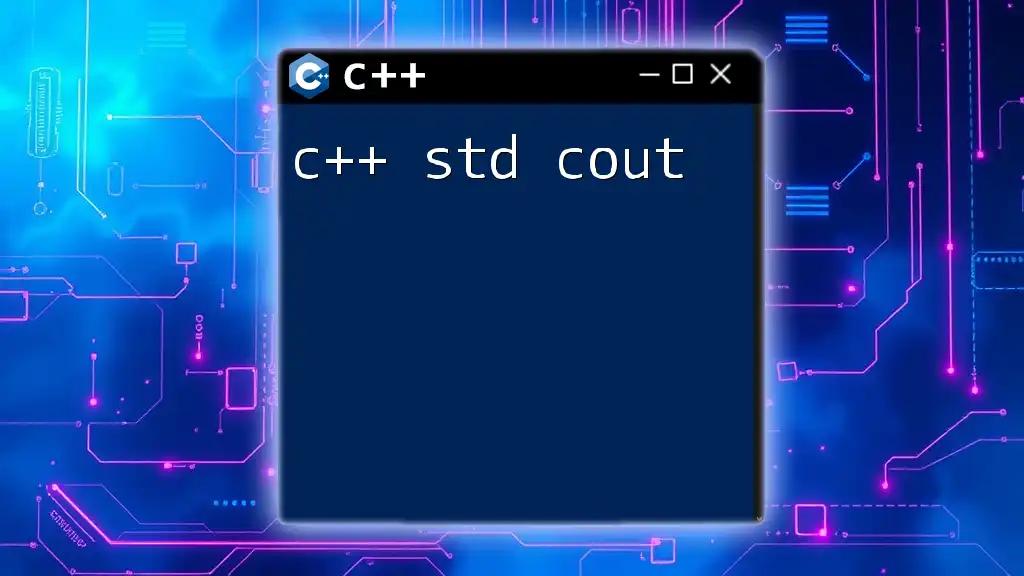
Pros and Cons of Using std::stack
Advantages
- Simplifies Stack Operations: `std::stack` provides a straightforward interface for handling stack operations without the complexity of implementing these features manually.
- Built-In Memory Management: With standard library containers, memory is handled automatically, reducing the risks of memory leaks and other allocation errors.
Disadvantages
- Limited Access to Elements: Direct access to all elements is not possible; only the top element can be accessed. This can be a limiting factor in certain scenarios.
- Performance Considerations: While generally efficient, depending on the underlying container, performance can vary. For example, `std::list` may incur overhead compared to `std::deque`.
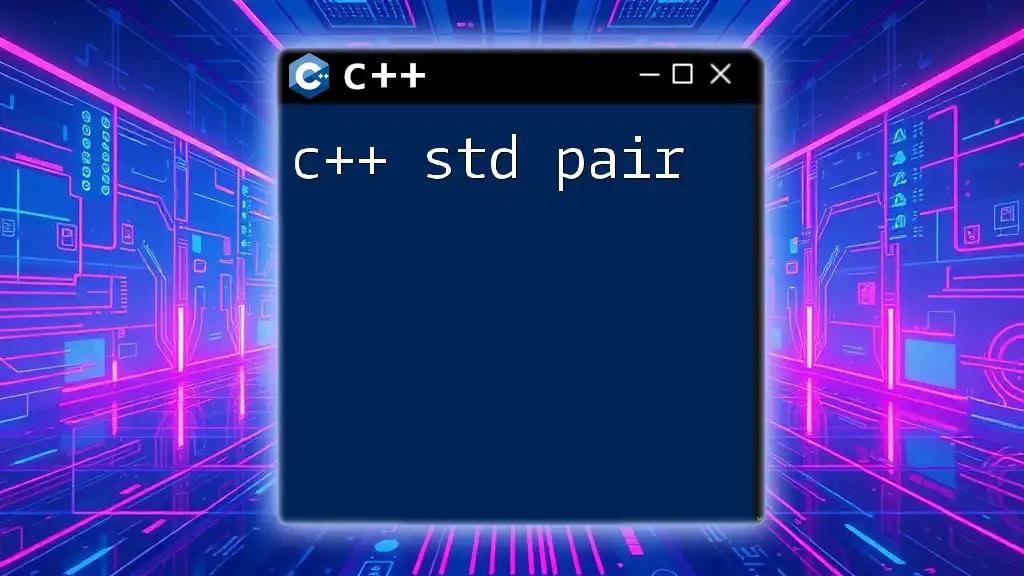
Common Mistakes to Avoid
Failing to Check for Empty Stack
One frequent error new programmers make is not checking if the stack is empty before calling `pop()` or `top()`. These operations on an empty stack lead to undefined behavior, potentially crashing the program.
Misusing push() and pop()
It’s essential to understand that every time you call `pop()`, the top element is removed permanently. Always ensure you don't accidentally remove items you might still need for further operations.
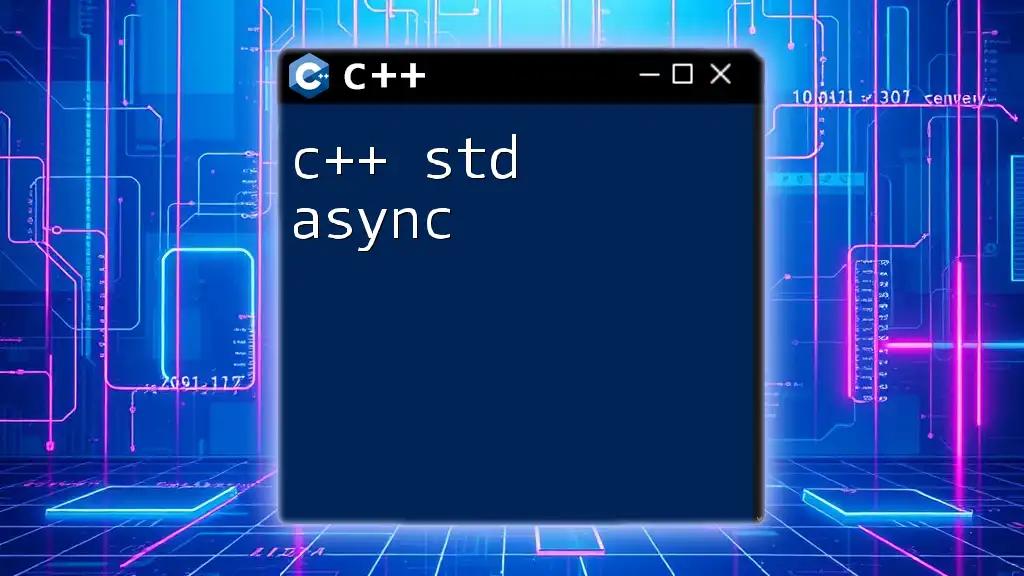
Best Practices
When to Use std::stack
Utilize `std::stack` when you need a simple LIFO structure without the requirement for random access to elements. It’s particularly useful in scenarios such as managing state in applications or parsing tasks where needing the most recent data is essential.
Debugging Tips
When working with `std::stack`, employ debugging techniques like checking stack content before critical operations, and ensure to use logging or debugging statements to trace the state of the stack during execution. This approach helps pinpoint issues related to stack operations effectively.
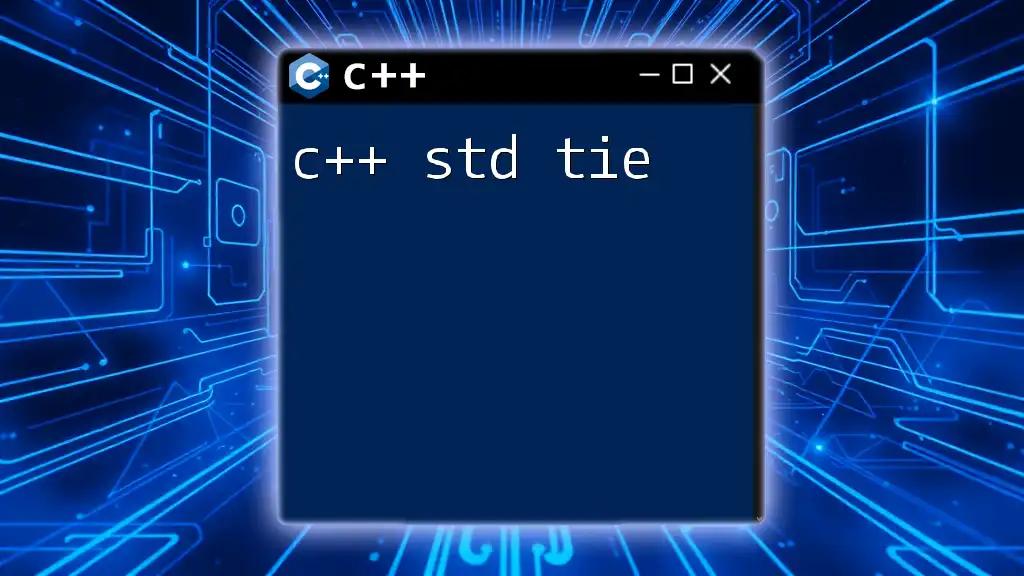
Conclusion
The `std::stack` in C++ provides a powerful yet straightforward way to manage collections of elements following the LIFO principle. Understanding its operations and best practices enhances code reliability and efficiency. As you gain experience with `std::stack`, experiment with its capabilities in various programming contexts to fully appreciate its versatility and power.
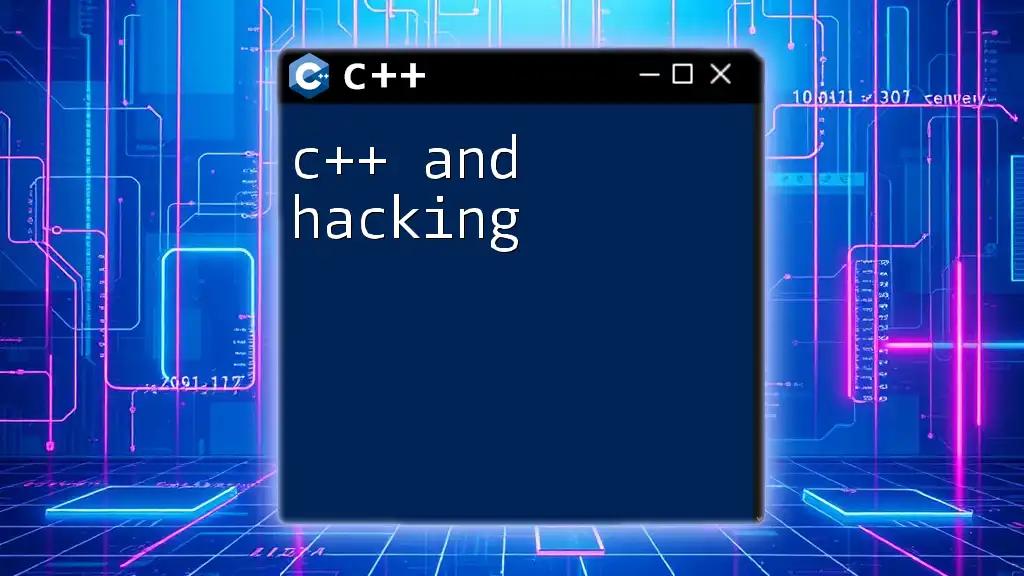
Additional Resources
For further guidance on `std::stack`, refer to the official C++ documentation and explore comprehensive resources such as programming books and online courses dedicated to C++. Engaging with these materials will strengthen your understanding and application of stacks in C++.