The `std::hash` in C++ is a template class that provides a way to generate hash values for different data types, making it useful for hash-based containers like `std::unordered_map`.
#include <iostream>
#include <string>
#include <functional>
int main() {
std::hash<std::string> hash_fn;
std::string key = "example";
size_t hash_value = hash_fn(key);
std::cout << "Hash value of \"" << key << "\" is: " << hash_value << std::endl;
return 0;
}
Understanding Hashing
Hashing is a fundamental concept in programming that involves the transformation of data into a fixed-size value, typically referred to as a hash code or hash value. This process ensures that even a small modification in the input data will produce a significantly different hash value. The significance of hash functions extends to various applications, including data retrieval, cryptography, and hashing algorithms utilized in different data structures.
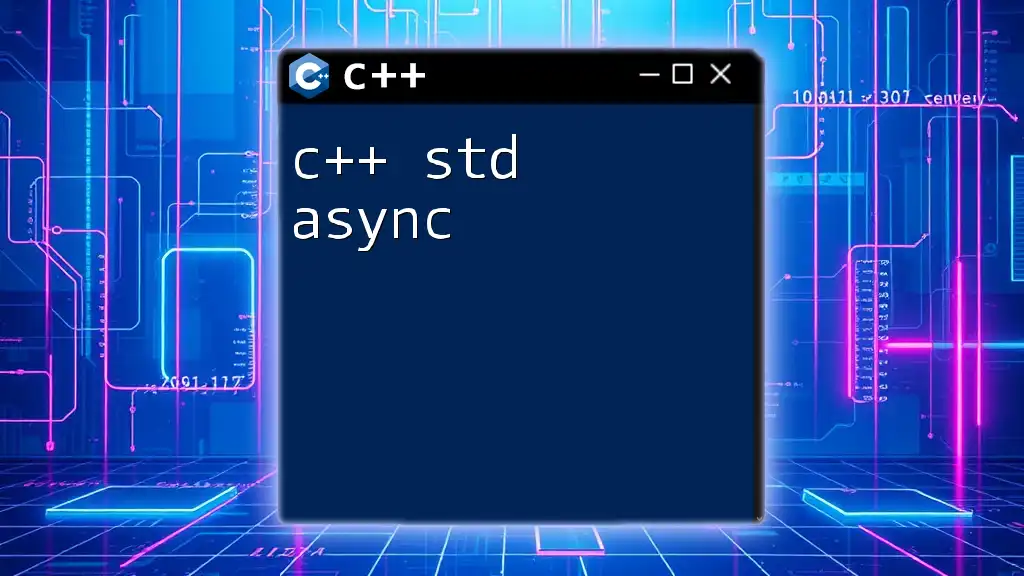
What is C++ std::hash?
The C++ std::hash is a part of the C++ Standard Library that provides a predefined mechanism to compute hash values for various data types. This functionality is crucial for creating hashed data structures like hash tables, where quick data retrieval is necessary. By leveraging std::hash, developers can efficiently utilize hash values in a variety of contexts within their applications.
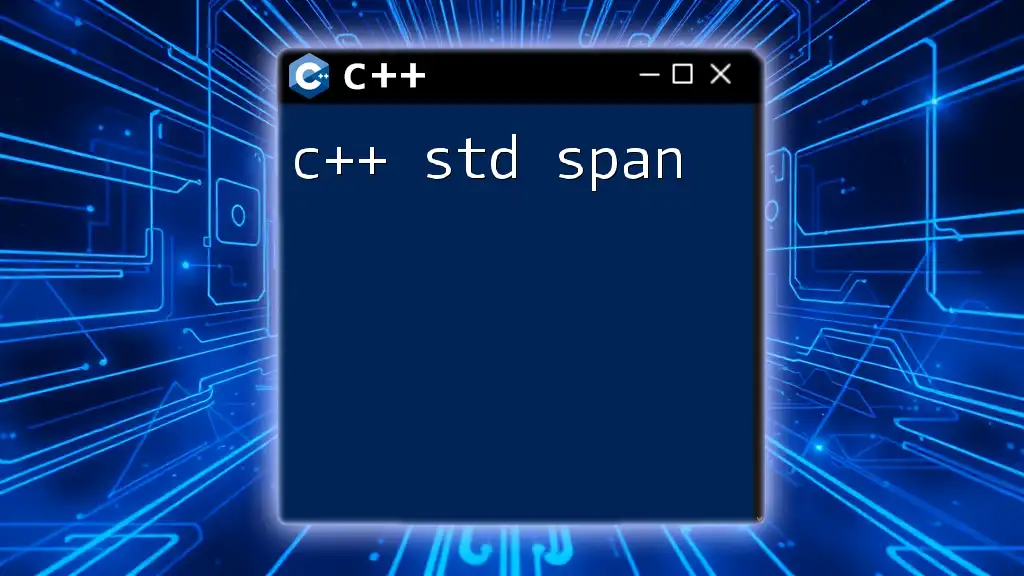
Fundamentals of C++ Hash Functions
What is a Hash Function?
A hash function is a specific type of algorithm that converts input data of arbitrary size into a fixed-size value. The characteristics of an effective hash function include:
- Determinism: The same input should consistently produce the same output.
- Uniformity: The output should be uniformly distributed to minimize collisions.
- Efficiency: It should compute the hash quickly for all input data types.
How Hash Functions Work
At its core, a hash function takes an input (sometimes referred to as a key) and maps it to a fixed-size output. This output can be an integer, a string, or another data type. However, hash functions can encounter collisions—a situation where two different inputs yield the same hash value. Handling collisions efficiently is essential for maintaining the performance of hash tables and other hash-based data structures.
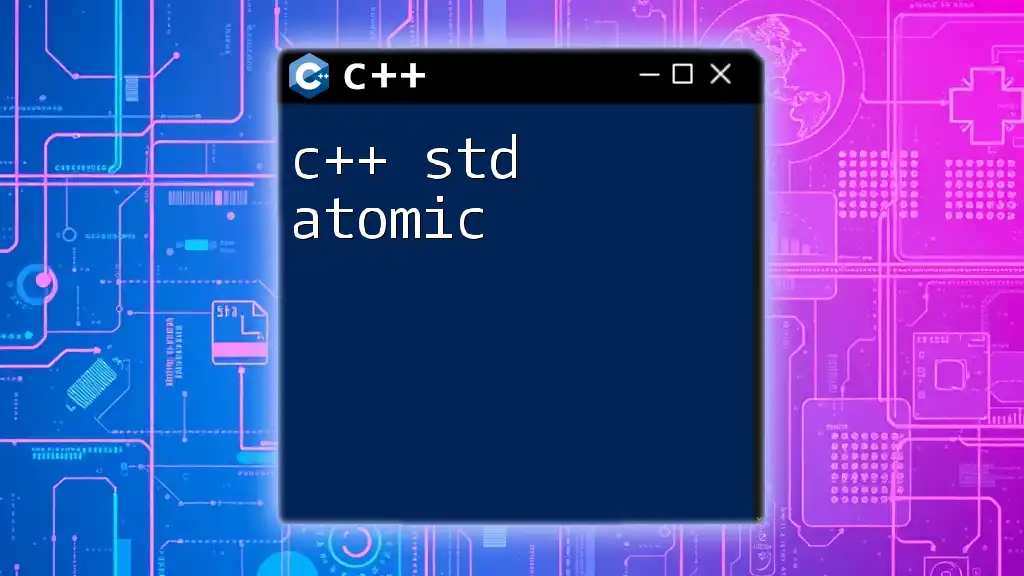
Exploring the std::hash
Overview of the std::hash Class
The std::hash class is designed to provide hash functions for built-in data types and some standard library types. By utilizing this class, programmers can avoid the complexity of implementing their own hash functions for common types. In addition, std::hash guarantees a level of performance and uniformity suitable for most applications.
Common Data Types Supported by std::hash
The std::hash class natively supports various fundamental types, including:
- Primitive types: These include integers (`int`, `long`), floating-point numbers (`float`, `double`), and boolean values (`bool`).
- Standard library types: Common data structures such as `std::string`, `std::vector`, and other containers.
Example: Using std::hash with Primitive Types
Here is a simple example demonstrating how to use std::hash with primitive types:
#include <iostream>
#include <functional>
int main() {
std::hash<int> hash_fn;
std::cout << "Hash of 42: " << hash_fn(42) << std::endl;
return 0;
}
In this example, we create a hash function for an `int` and then compute the hash value of the integer 42. The output will display the hash value generated by the std::hash function for this integer.
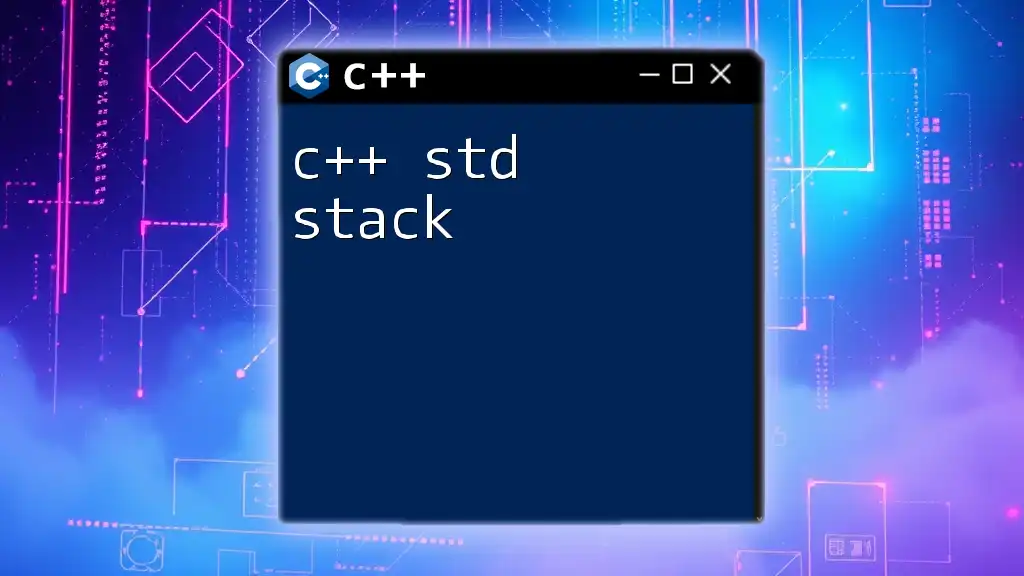
Custom Hash Functions in C++
Creating Custom Hash Functions
While std::hash provides convenient hashing for many types, there are situations where the developer might need to create custom hash functions—especially when dealing with user-defined data types like structures or classes. A custom hash function allows for specific handling of the unique attributes of these types, ensuring that the generated hash values are efficient and meaningful.
Example: Custom Hash Function for a Struct
To illustrate the creation of a custom hash function, consider a struct that represents points in a two-dimensional space:
#include <iostream>
#include <functional>
struct Point {
int x;
int y;
};
struct HashPoint {
size_t operator()(const Point& p) const {
return std::hash<int>()(p.x) ^ (std::hash<int>()(p.y) << 1);
}
};
In this example, we define a `Point` struct with `x` and `y` coordinates. The custom hash function, `HashPoint`, combines the hash values of `x` and `y` using bitwise operations to produce a unique hash for each Point instance. This ensures efficient distribution and reduces the risk of collisions.
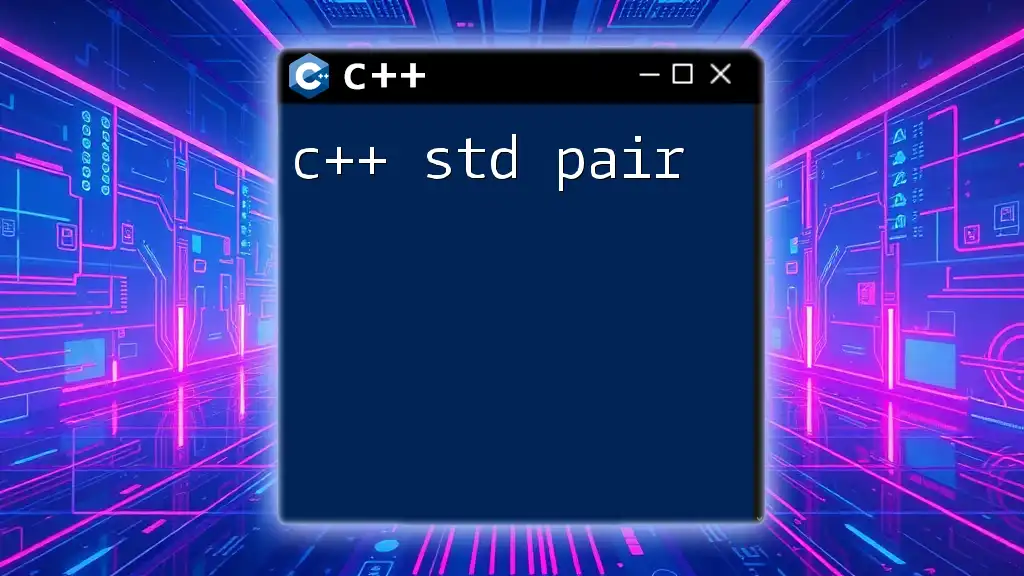
Using std::hash with Containers
Hashing in STL Containers
The integration of std::hash with STL containers, specifically unordered containers like `std::unordered_map` and `std::unordered_set`, showcases the true power of hash functions. These containers rely on hash values to achieve average-case constant time complexity for many operations, such as insertions and lookups.
Example: Using std::unordered_map with std::hash
Here’s an example of how to use std::unordered_map with std::hash:
#include <iostream>
#include <unordered_map>
int main() {
std::unordered_map<std::string, int> my_map;
my_map["apple"] = 1;
my_map["banana"] = 2;
std::cout << "Hash of 'apple': " << std::hash<std::string>()("apple") << std::endl;
return 0;
}
In this snippet, we create an unordered map and associate string keys with integer values. Additionally, we compute and print the hash of the string "apple" using std::hash. The hash value printed helps clarify how the keys are maintained within the unordered_map.
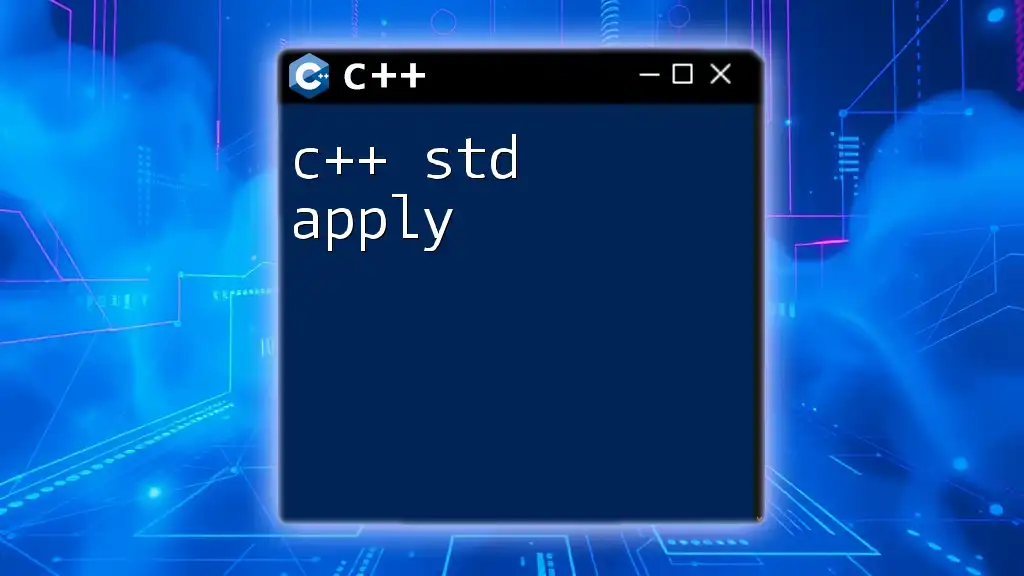
Performance and Efficiency of Hash Functions
Understanding Time Complexity
The time complexity of hash functions is generally O(1) for average cases concerning insertions, deletions, and searches in hash-based structures. However, in worst-case scenarios, where many collisions occur, it can degrade to O(n) if the same hash value is produced for multiple inputs. Developers must choose hash functions that minimize these collision occurrences.
Choosing an Appropriate Hash Function
Choosing the right hash function involves considering several factors:
- Performance: Ensure it computes efficiently for all input sizes.
- Uniformity: Aim for a minimal chance of collision for similar input data.
- Application-specific needs: Some applications may require cryptographic security, while others simply need quick access.
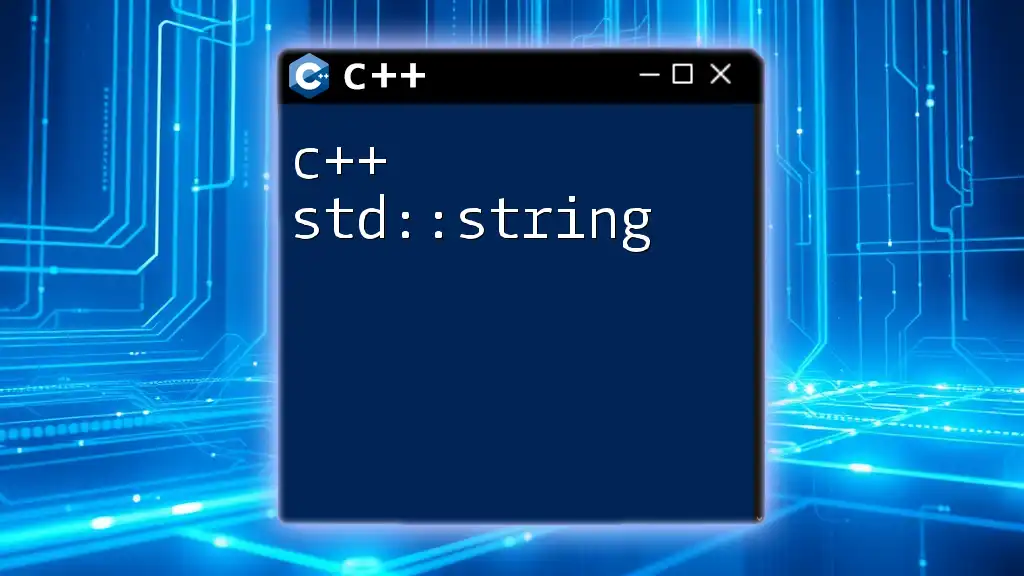
Common Mistakes and Pitfalls
Common Issues with std::hash
Developers using std::hash should be aware of certain common pitfalls. For instance, relying solely on the default behaviors for non-standard data types can result in inefficient hashing and poor performance in hash tables.
Best Practices for Hashing in C++
- Prefer std::hash for built-in types: This ensures you benefit from optimized hashing implementations.
- Design custom hash functions carefully: Pay close attention to uniformity and avoid significantly overlapping outputs for distinct inputs.
- Test hash function performance: Regularly evaluate the speed and efficiency of your hash functions within the application context to detect any performance issues early on.
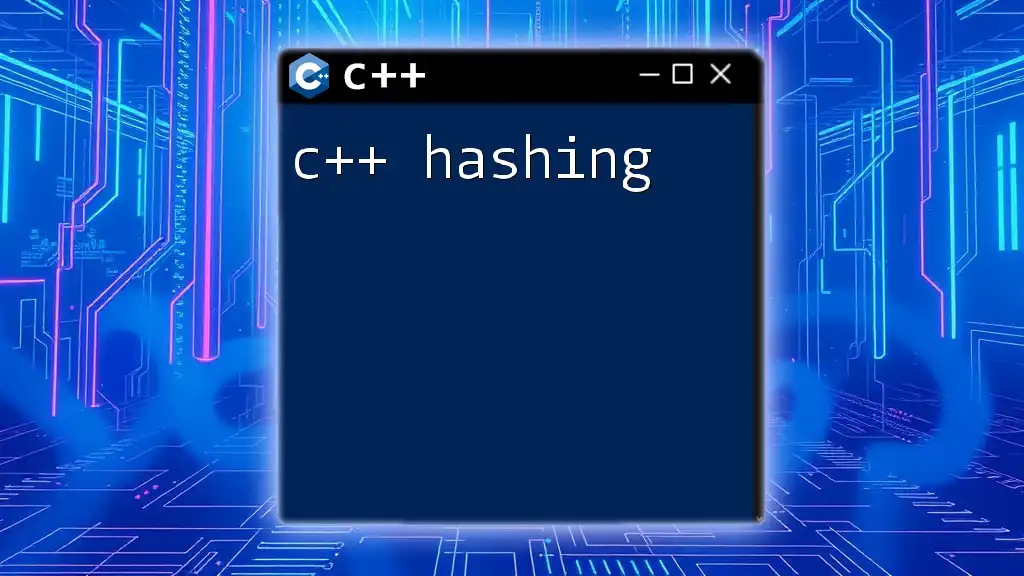
Conclusion
In summary, mastering C++ std::hash is crucial for any programmer working with modern C++. By understanding both the built-in features and the ability to create custom hash functions, developers can implement efficient algorithms and data structures that leverage the power of hashing.
Practice is essential. Implement various hash functions and explore their integration with different data structures. You’ll expand your knowledge and proficiency in building robust C++ applications.
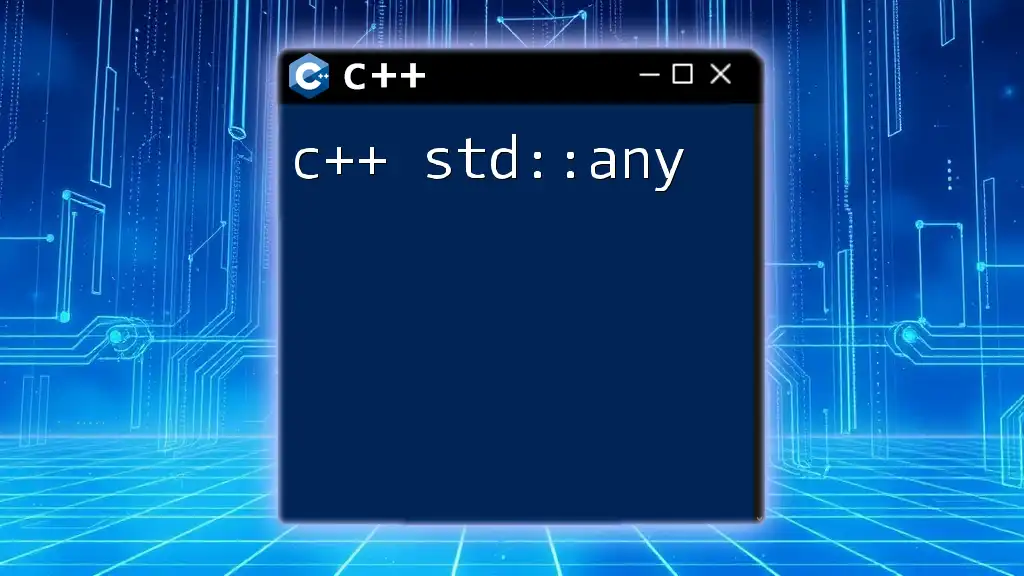
Appendix
Further Reading
For more information, consider exploring the official C++ Standard documentation or dedicated C++ programming books that discuss hashing and data structures in greater detail.
Glossary of Terms
- Hash Function: An algorithm that converts input to a fixed-size value.
- Collision: Occurs when two distinct inputs produce the same hash output.
- STL Containers: Standard Template Library containers that store collections of objects.