The `stdlib.h` header in C++ provides functions for memory allocation, process control, conversions, and others, making it essential for general-purpose programming.
#include <stdlib.h>
#include <iostream>
int main() {
int* arr = (int*)malloc(5 * sizeof(int)); // Allocate memory for 5 integers
if (arr == NULL) {
std::cerr << "Memory allocation failed\n";
return 1;
}
// Use the array...
free(arr); // Free allocated memory
return 0;
}
What is stdlib.h?
The `stdlib.h` header file is one of the essential C standard library headers that is widely utilized in C++ programming. It provides a rich collection of functions that are crucial for tasks such as memory allocation, process control, data conversion, and more. By incorporating stdlib.h into your C++ programs, you can greatly enhance your coding efficiency and manage resources effectively.
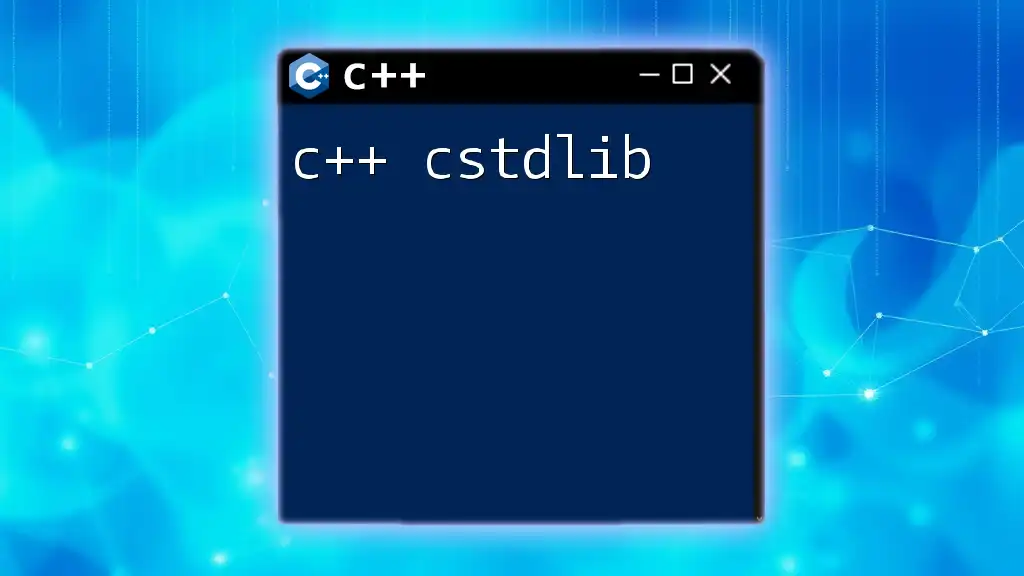
Why Use stdlib.h in C++?
Using `stdlib.h` allows developers to employ a variety of standard functions that simplify complex programming tasks. It is particularly valuable for writing code that requires dynamic memory management, decision-making, and data type conversions. The header file promotes structured programming and better resource management by providing functions that handle fundamental operations without reinventing the wheel each time.
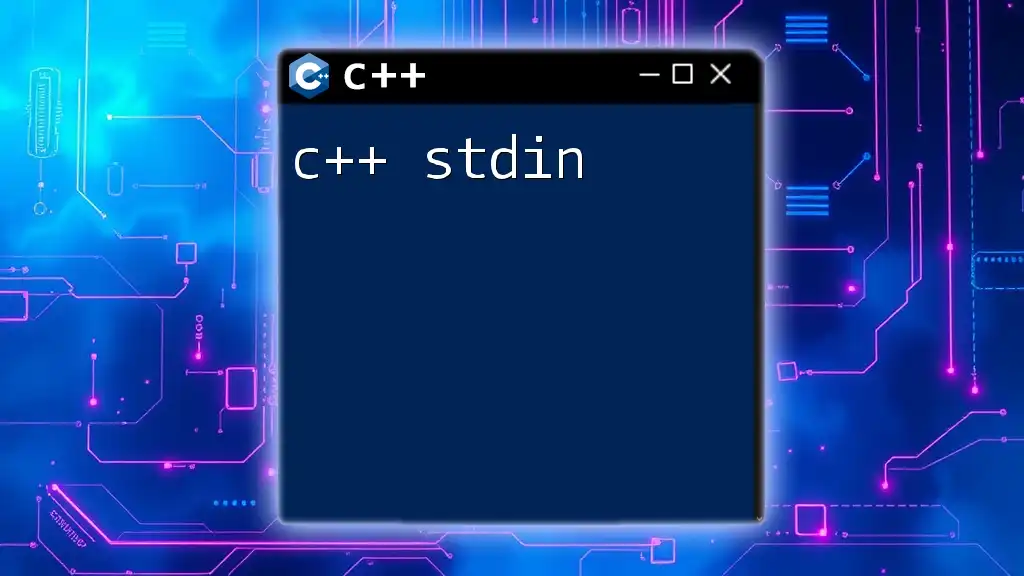
Key Functionalities of stdlib.h
Memory Management Functions
Dynamic Memory Allocation
The ability to allocate memory dynamically is one of the central functionalities provided by `stdlib.h`. It allows programmers to request memory from the heap during program execution, which is particularly useful for handling arrays and data structures whose sizes may not be known at compile time.
The key functions include:
- `malloc(size_t size)`: Allocates a block of memory of the specified size and returns a pointer to the beginning of the block.
- `calloc(size_t num, size_t size)`: Allocates a block of memory for an array, initializing all bytes to zero.
- *`realloc(void ptr, size_t newSize)`**: Resizes the memory block pointed to by `ptr` to `newSize`.
- *`free(void ptr)`**: Deallocates the memory previously allocated by `malloc`, `calloc`, or `realloc`.
Code Example:
#include <stdlib.h>
#include <iostream>
int main() {
int* arr = (int*)malloc(5 * sizeof(int)); // Allocating memory for 5 integers
if (arr == nullptr) {
std::cerr << "Memory allocation failed!";
return 1;
}
// Use the allocated memory
for (int i = 0; i < 5; ++i) {
arr[i] = i + 1; // Assigning values to allocated memory
std::cout << arr[i] << " "; // Outputting values
}
std::cout << std::endl;
free(arr); // Deallocating the memory
return 0;
}
In this example, `malloc` is used to allocate memory for an array of integers, which is essential for scenarios where the array size may vary. It’s important to check if the allocation was successful and to free the allocated memory afterward to prevent memory leaks.
Process Control Functions
Exiting a Program
`stdlib.h` also provides functions to manage program execution and process termination. The `exit()` function is paramount for gracefully terminating a program.
Code Example:
#include <stdlib.h>
#include <iostream>
int main() {
std::cout << "Program is exiting...\n";
exit(0); // Exiting the program with a success code
}
Using `exit()` allows developers to indicate success or failure by defining an exit status. Passing a value of 0 typically signifies a successful execution, while any non-zero value indicates an error.
Conversion Functions
String to Numeric Conversions
Converting strings to numeric types is a common scenario, especially when processing user input. The functions provided by `stdlib.h` facilitate these conversions effectively.
Key functions include:
- *`atoi(const char str)`**: Converts a string to an integer.
- *`atof(const char str)`**: Converts a string to a floating-point number.
- *`atol(const char str)`**: Converts a string to a long integer.
- `strtol(const char str, char* endptr, int base)`**: Converts a string to a long integer, allowing for a specified base.
Code Example:
#include <stdlib.h>
#include <iostream>
int main() {
const char* str = "123.45";
double num = atof(str); // Converts string to double
std::cout << "Converted number: " << num << std::endl;
return 0;
}
In this snippet, `atof` is used to convert a string representation of a number into its floating-point equivalent. This conversion is vital for applications that require numerical computations based on user input.
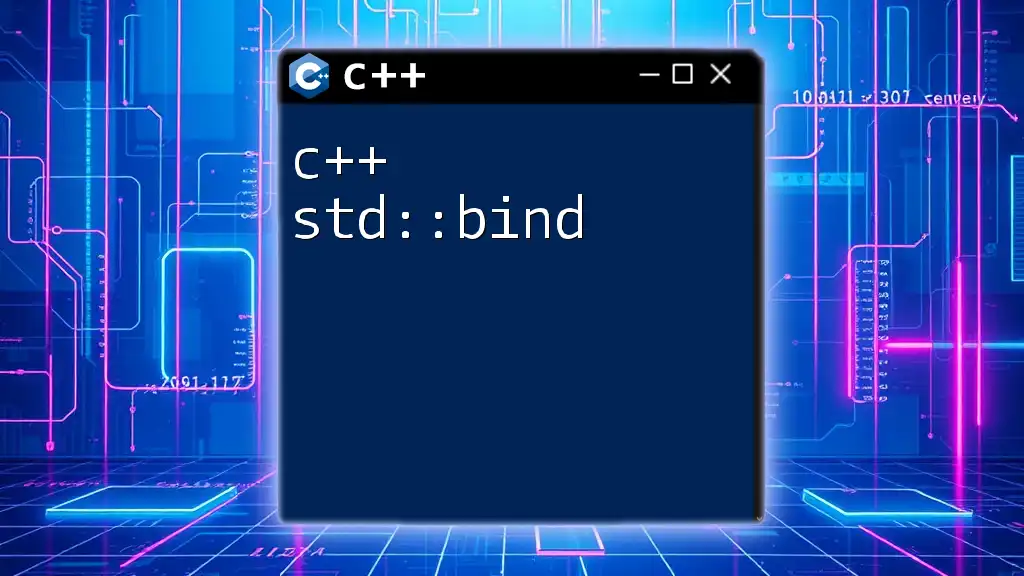
Error Handling with stdlib.h
Error Reporting with errno
Proper error handling is crucial in any program. Functions in `stdlib.h` can set the `errno` variable, allowing developers to diagnose issues effectively.
Understanding errno
The `errno` variable is set to indicate what went wrong during various library calls. After a function that sets `errno`, you can check its value to determine the type of error that occurred.
Code Example:
#include <stdlib.h>
#include <iostream>
#include <errno.h>
int main() {
int num = 5;
int result = 42 / num;
if (result == 0 && errno != 0) {
std::cerr << "Error occurred: " << strerror(errno);
}
return 0;
}
This code illustrates how `errno` is checked after a potential failure in a calculation, alongside using `strerror` to provide a clear error message. Utilize this best practice to ensure that your programs have robust error handling.
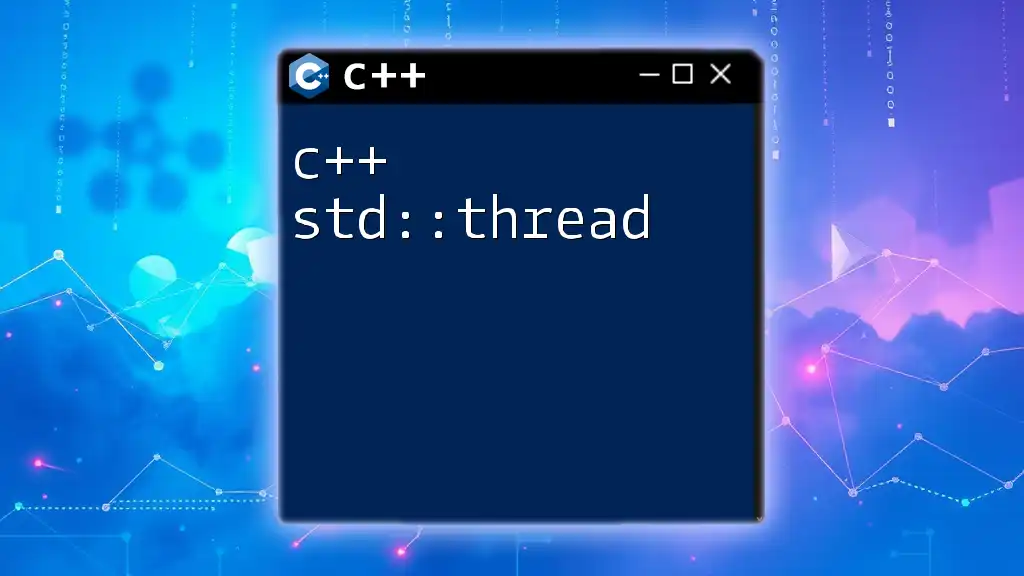
Additional Functions in stdlib.h
Environmental Functions
`stdlib.h` provides functions to interact with the environment in which a program runs. One such function is `getenv()`, which retrieves values of environment variables.
Code Example:
#include <stdlib.h>
#include <iostream>
int main() {
const char* path = getenv("PATH"); // Getting the PATH environment variable
if (path) {
std::cout << "PATH: " << path << std::endl;
} else {
std::cout << "PATH variable not found." << std::endl;
}
return 0;
}
Environment variables can be essential in configuring how programs run, making `getenv` a valuable function when you need to adapt program behavior based on system settings or user-defined configurations.
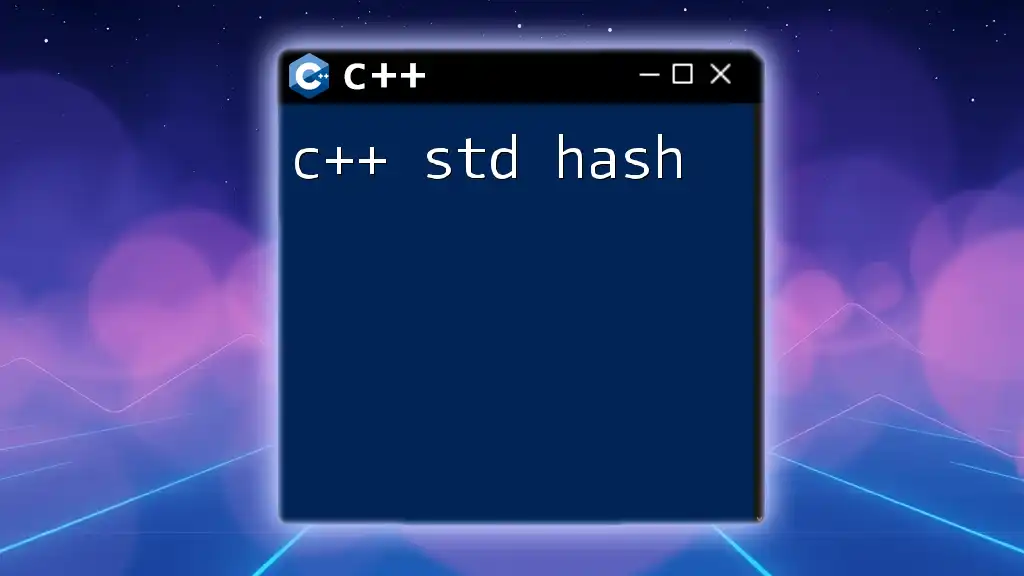
Best Practices for Using stdlib.h
Avoiding Memory Leaks
Managing memory effectively is vital to prevent leaks that can degrade system performance. Always ensure that any memory allocated with `malloc`, `calloc`, or `realloc` is properly deallocated using `free`.
Best Practice Tips:
- Use dynamic memory only when necessary.
- Always check for successful memory allocation.
- Implement RAII principles using C++ features like smart pointers to manage memory automatically wherever possible.
Ensuring Type Safety
Type safety prevents errors that can arise from improper data handling. Always ensure appropriate casting and be cautious about data converting functions. Using explicit conversions reduces the likelihood of encountering unexpected behavior in your programs.
Encourage the use of C++ best practices, such as using smart pointers, which manage memory automatically and help prevent many common memory management issues.
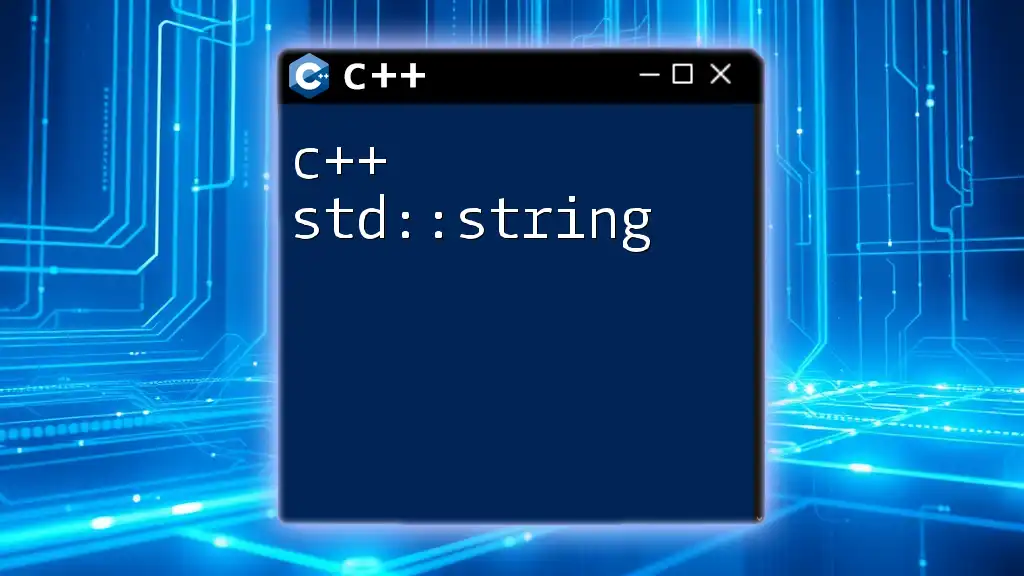
Conclusion
The `stdlib.h` header file is invaluable in C++ programming, providing essential functions that facilitate a range of tasks, from memory management to process control and data conversion. By understanding and mastering the functions within this header, developers can write more efficient, robust, and cleaner code.
I encourage you to explore the examples provided and practice implementing these concepts in your projects. If you have any experiences or questions about using `stdlib.h`, feel free to share them for further discussion.