The `std::map` in C++ is a sorted associative container that stores key-value pairs, where each key is unique, allowing for fast retrieval based on the key.
#include <iostream>
#include <map>
int main() {
std::map<std::string, int> ageMap;
ageMap["Alice"] = 30;
ageMap["Bob"] = 25;
for (const auto& pair : ageMap) {
std::cout << pair.first << " is " << pair.second << " years old.\n";
}
return 0;
}
What is `std::map`?
`std::map` is a part of the C++ Standard Library that provides an associative container to store elements in key-value pairs. It is an essential data structure for scenarios where you need to associate unique keys with specific values efficiently.
Key characteristics of `std::map`:
- Ordered nature: Unlike some other containers, `std::map` maintains its elements in a sorted order based on the keys. This allows for efficient searching and retrieval.
- Unique keys: Each key within a `std::map` must be unique; if an attempt is made to insert a duplicate key, the existing value associated with that key will be replaced.
- Key-value pairs: A `std::map` stores elements as pairs, where each pair consists of a key and its corresponding value.
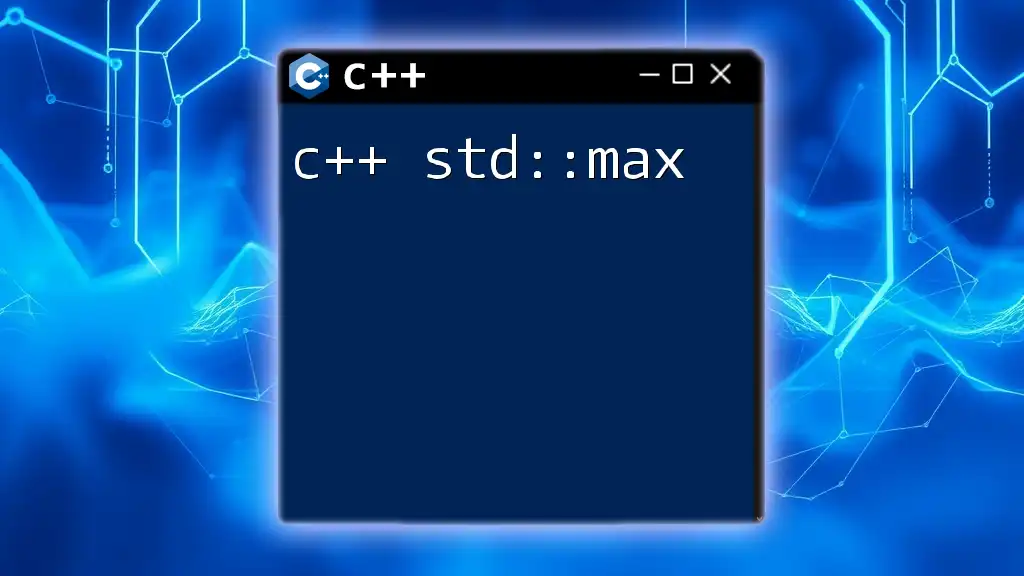
How to Include the Header
To use `std::map`, you need to include the appropriate header in your C++ program. The header file is straightforward, as shown below:
#include <map>
This inclusion allows you to leverage the functionalities provided by the `std::map` class without any additional setup.
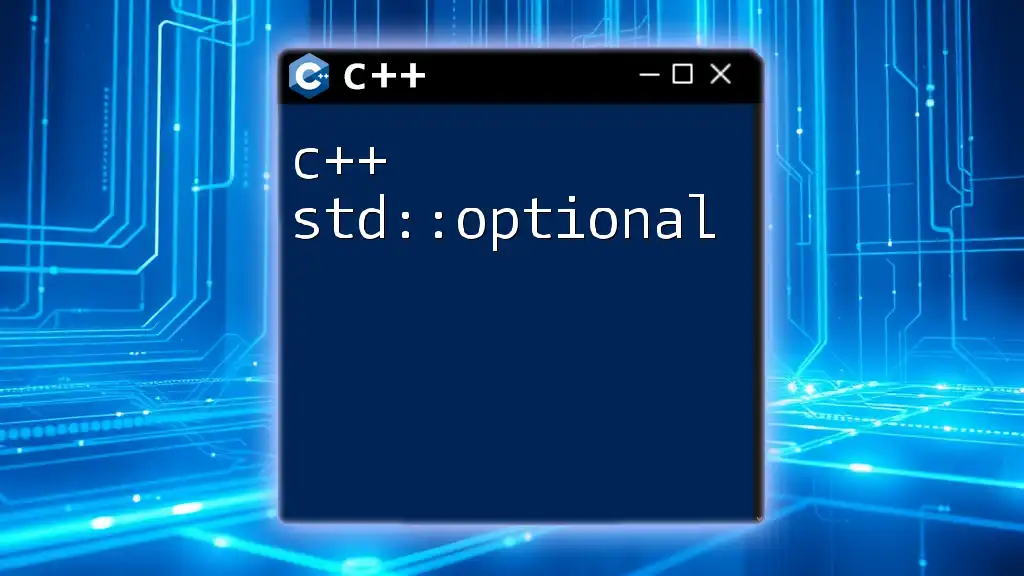
Creating a `std::map`
Creating a `std::map` is simple and follows a straightforward syntax:
std::map<int, std::string> exampleMap;
In this example, `int` is the data type of the key, and `std::string` is the type for the value. You can customize the data types according to your needs, allowing for flexible usage.
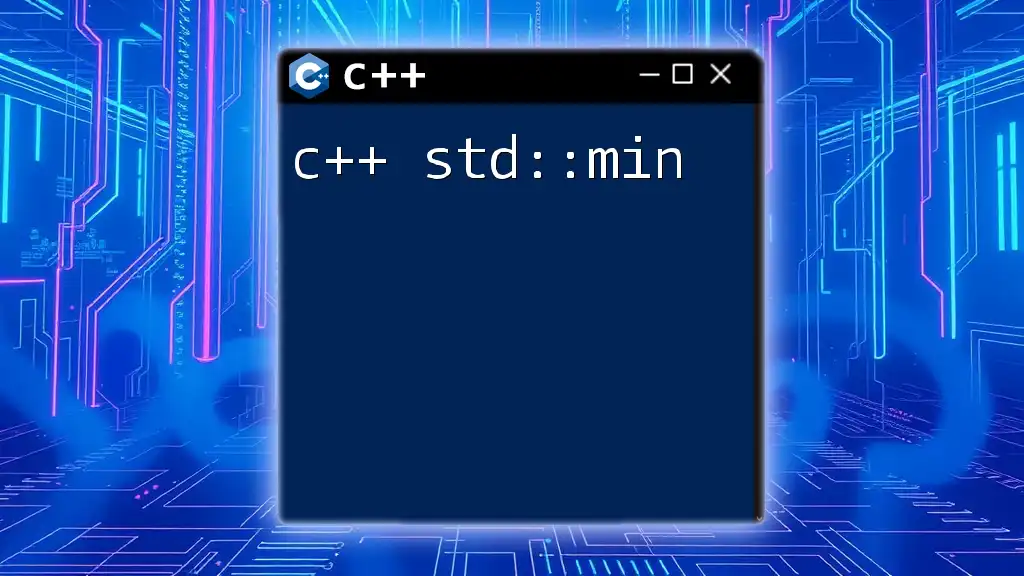
Inserting Elements into a `std::map`
Inserting elements into a `std::map` can be accomplished through several methods:
Using `insert()` Method
The `insert()` method allows you to add a key-value pair effectively. For instance:
exampleMap.insert(std::make_pair(1, "One"));
Using Subscript Operator `[]`
You can also use the subscript operator `[]` to insert a key-value pair. This operator will automatically create a new entry if the key does not exist:
exampleMap[2] = "Two";
Using `emplace()` Method
The `emplace()` method constructs a pair in place, reducing the need for a temporary object. This method is often more efficient:
exampleMap.emplace(3, "Three");
Explanation of Each Method
-
`insert()`: This method should be used when you want to add a specific pair and check for conflicts. If you attempt to insert an existing key, the operation will fail silently.
-
Subscript Operator `[]`: This operator is user-friendly, as it allows you to both retrieve and insert elements in a single line. Be cautious: using this operator on a non-existing key will add that key to the map with a default value.
-
`emplace()`: This method is particularly advantageous when you want to optimize your performance. It constructs elements directly in the map, eliminating unnecessary object copying.
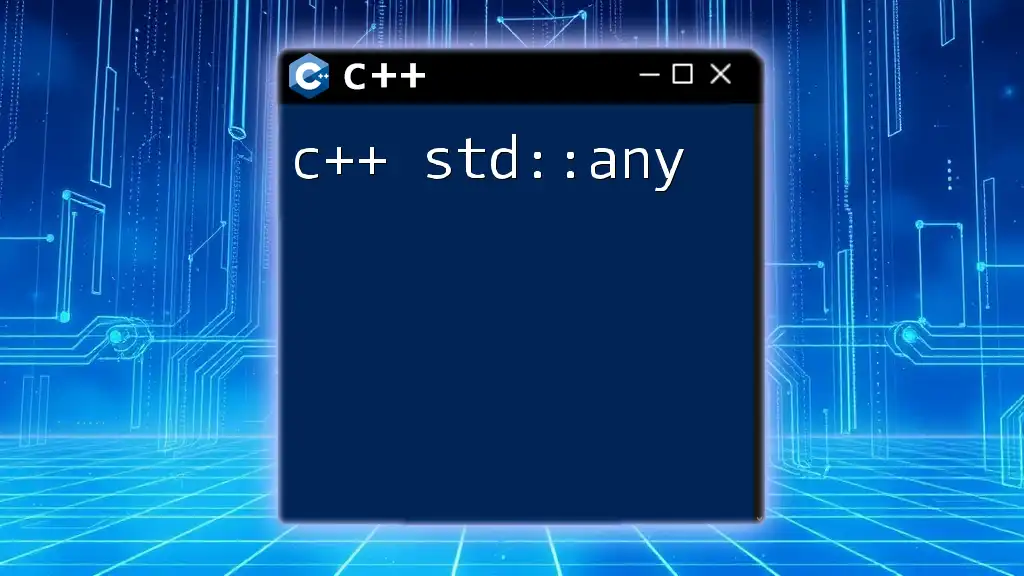
Accessing Elements in a `std::map`
There are different methods available for accessing elements stored in a `std::map`:
- Direct access using the subscript operator `[]`.
- Using the `at()` method for safe access.
- Utilizing iterators for iteration.
Example Code: Accessing Elements
std::cout << exampleMap[1]; // Outputs: One
std::cout << exampleMap.at(2); // Outputs: Two
Explanation of Access Methods
The subscript operator `[]` will automatically create a new entry with a default value if the key does not exist. In contrast, the `at()` method will throw an `std::out_of_range` exception if the requested key is not found, providing a safer alternative for access.
Iterators are essential for iterating through all elements. They offer a flexible way to access elements using the `begin()` and `end()` methods.
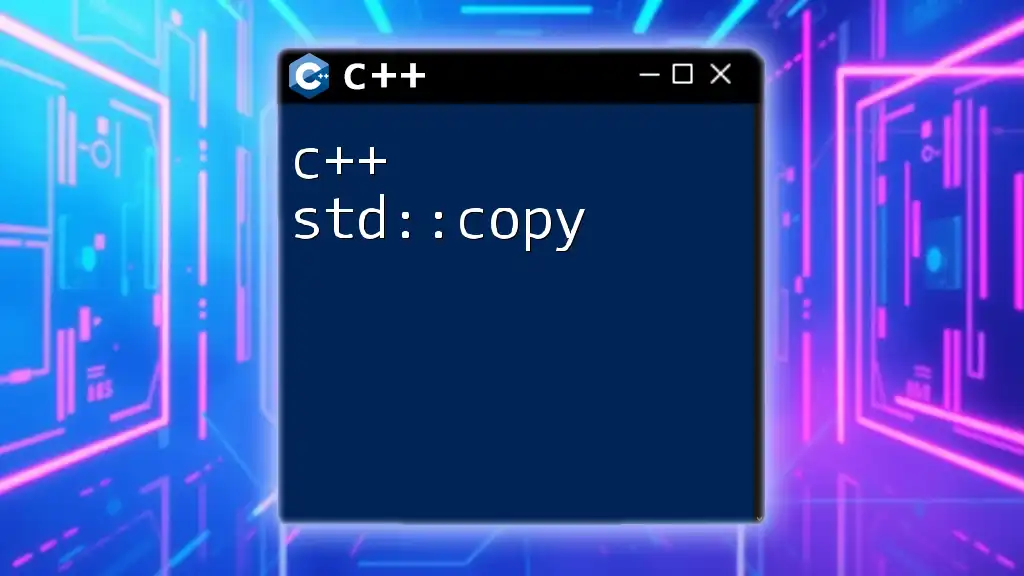
Iterating Over a `std::map`
You can iterate over a `std::map` using various techniques.
Using Range-Based For Loops
Range-based for loops provide a concise syntax for iterating over maps:
for (const auto& pair : exampleMap) {
std::cout << pair.first << ": " << pair.second << std::endl;
}
Using Iterators
Alternatively, you can use iterators if you prefer a more traditional way of looping:
for (auto it = exampleMap.begin(); it != exampleMap.end(); ++it) {
std::cout << it->first << ": " << it->second << std::endl;
}
Explanation of Iteration Techniques
Utilizing range-based for loops simplifies the syntax, making your code cleaner and more readable. On the other hand, iterators provide more control and flexibility, especially if you plan to manipulate the iterator during traversal.
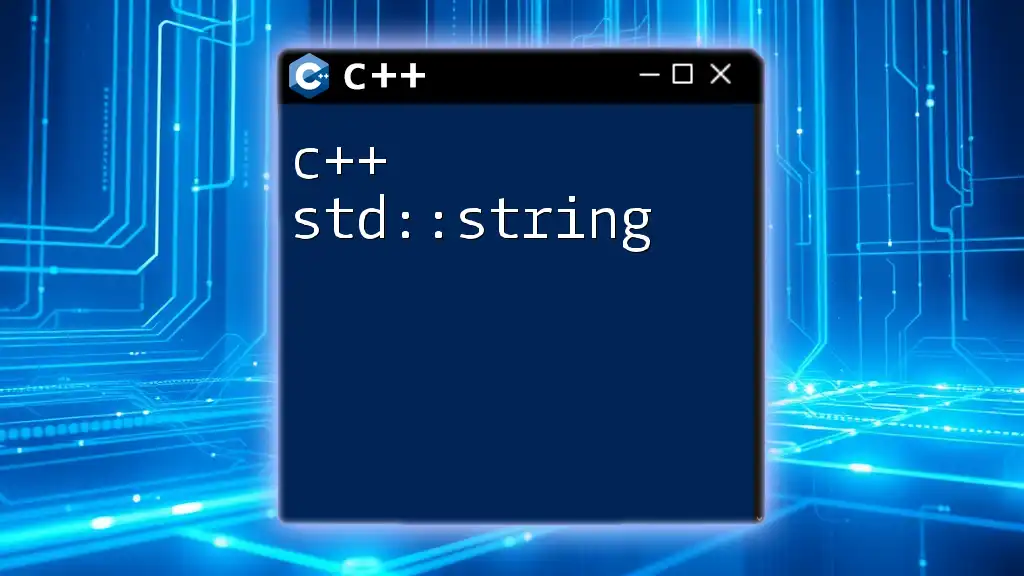
Modifying Elements in a `std::map`
Modifying an existing element is just as straightforward as inserting one. To change the value associated with a specific key, simply use the subscript operator:
exampleMap[1] = "Uno"; // Changes the value of key 1
This operation will replace the old value with the new one seamlessly.
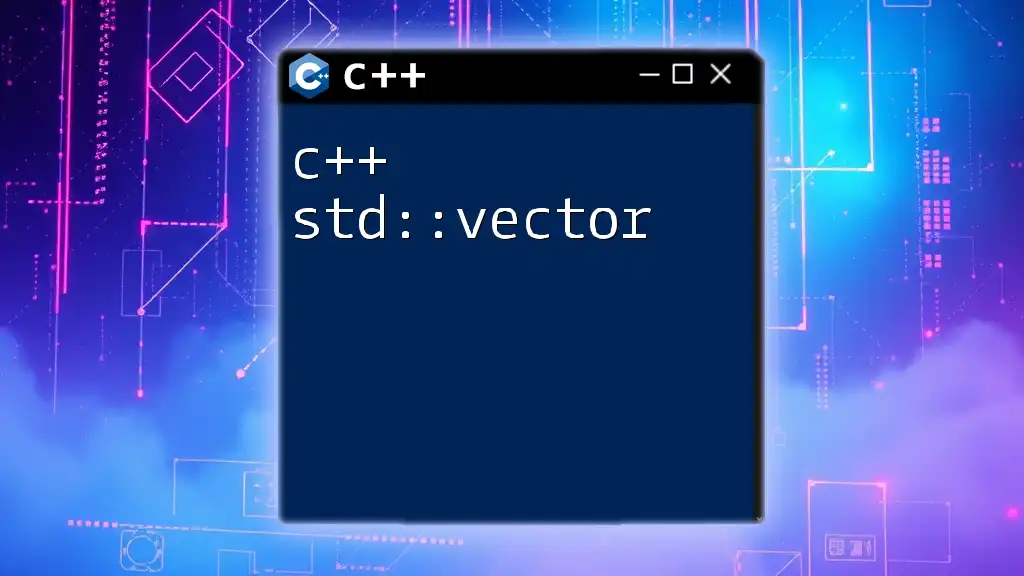
Removing Elements from a `std::map`
There are two primary methods for removing elements from `std::map`:
Using `erase()`
To remove a specific element, you can use the `erase()` method:
exampleMap.erase(2); // Removes the key-value pair with key 2
Using `clear()`
If you want to remove all elements from the map, simply call the `clear()` method:
exampleMap.clear(); // Clears the entire map
Explanation of Erasing Elements
The `erase()` method only removes the specified key and its value, while the `clear()` method empties the entire map, releasing all allocated memory. Be mindful of performance considerations when using these methods, especially in large maps.
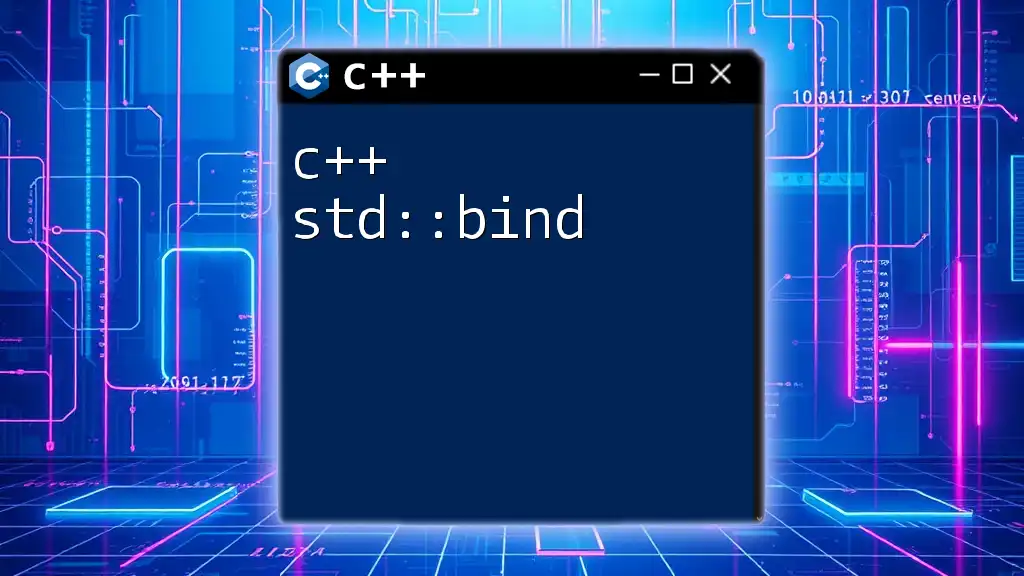
Common Use Cases for `std::map`
`std::map` shines in various scenarios:
- Counting occurrences: It can effectively keep track of how many times each element occurs in a dataset.
- Storing configuration settings: You can manage key-value pairs related to application configurations, providing easy access and modifications.
When you need ordered key-value storage where lookup, insertion, and deletion operations are efficient, `std::map` should be your go-to choice.
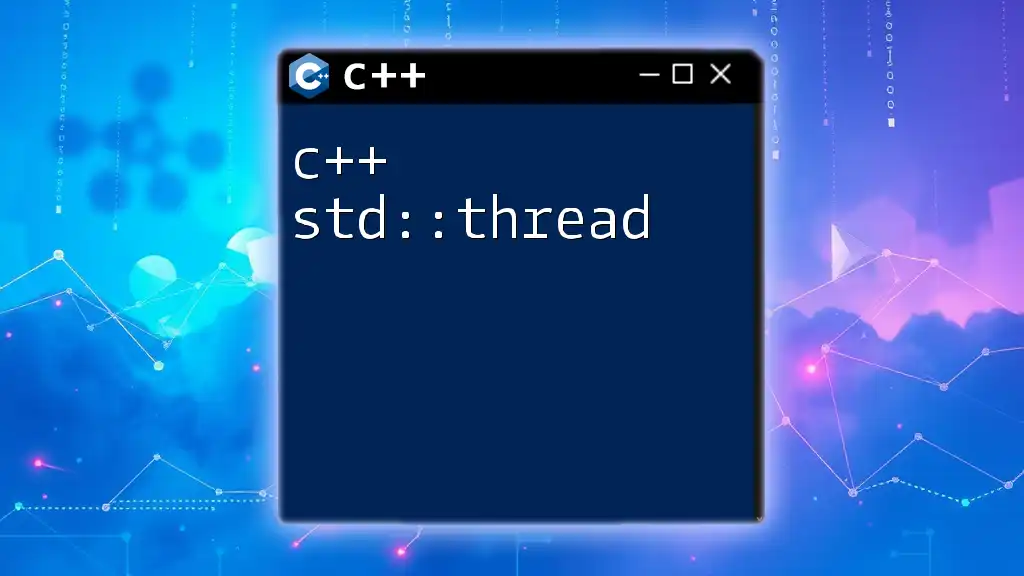
Performance Considerations
The versatility of `std::map` comes with performance characteristics worth considering. Operations such as insertion, deletion, and searching generally have a logarithmic time complexity, thanks to the underlying balanced tree structure.
However, if you don't require ordering, consider using `std::unordered_map`, which offers constant time complexity for the same operations but does not maintain element order.
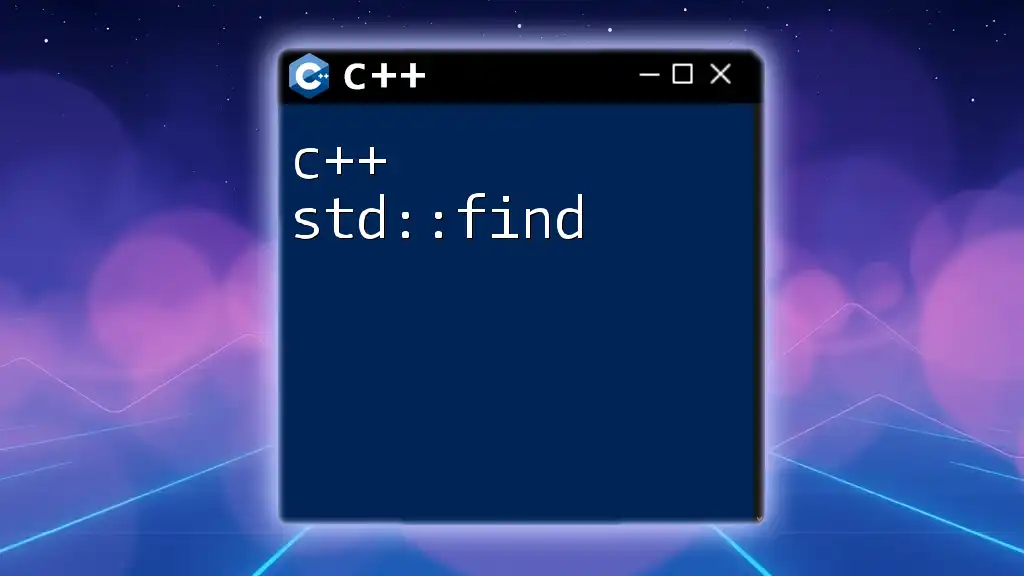
Conclusion
In summary, `std::map` is a powerful associative container in C++ that provides an efficient way to store and manage pairs of related data. Understanding its functionalities, operations, and performance considerations allows you to utilize it effectively in various programming scenarios. With this knowledge, you're well-equipped to implement `std::map` in your own C++ projects.
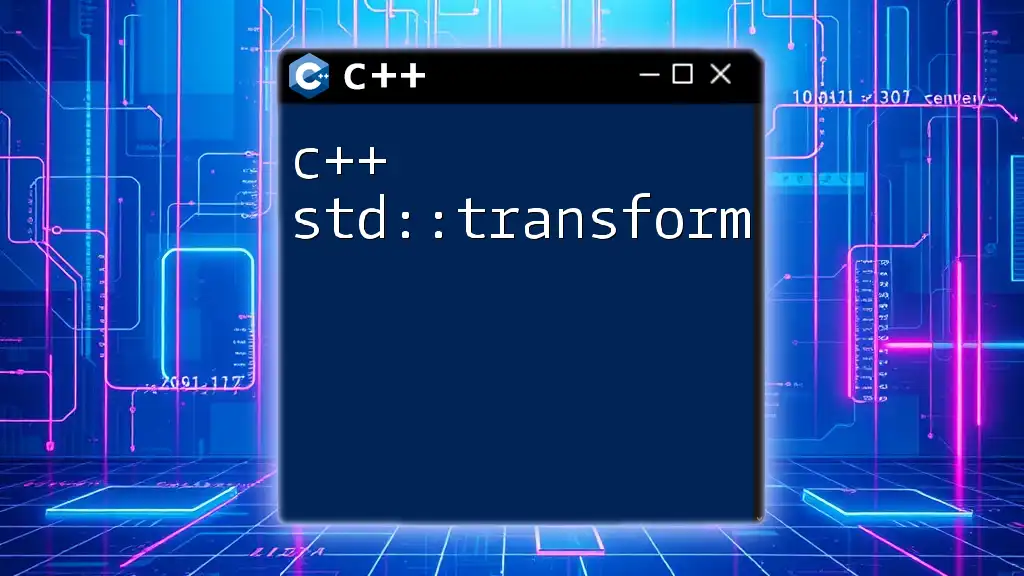
Additional Resources
To further enhance your understanding, consider referring to the official C++ documentation for `std::map`, as well as exploring recommended books and tutorials focused on mastering the C++ Standard Template Library (STL).