The `std::find` algorithm in C++ is used to search for a value within a range of elements and returns an iterator to the first occurrence of the value or the end of the range if not found.
#include <algorithm>
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
auto it = std::find(numbers.begin(), numbers.end(), 3);
if (it != numbers.end()) {
std::cout << "Found: " << *it << std::endl;
} else {
std::cout << "Not found." << std::endl;
}
return 0;
}
What is std::find?
`std::find` is a function template in C++, part of the Standard Template Library (STL), which helps locate an element in a range. It takes a range defined by two iterators (the beginning and the end) and a value to search for, returning an iterator to the found element or the end iterator if the element is not found.
Use Case
You might find `std::find` remarkably useful in various scenarios, such as when searching through a list of items to check for the existence of a specific value, making it an essential tool in your C++ toolkit.
Return Type
Understanding the return type is crucial for effectively utilizing `std::find`. The function returns an iterator pointing to the first occurrence of the specified element. If the element is not found, it returns the `last` iterator, which indicates the end of the search range.
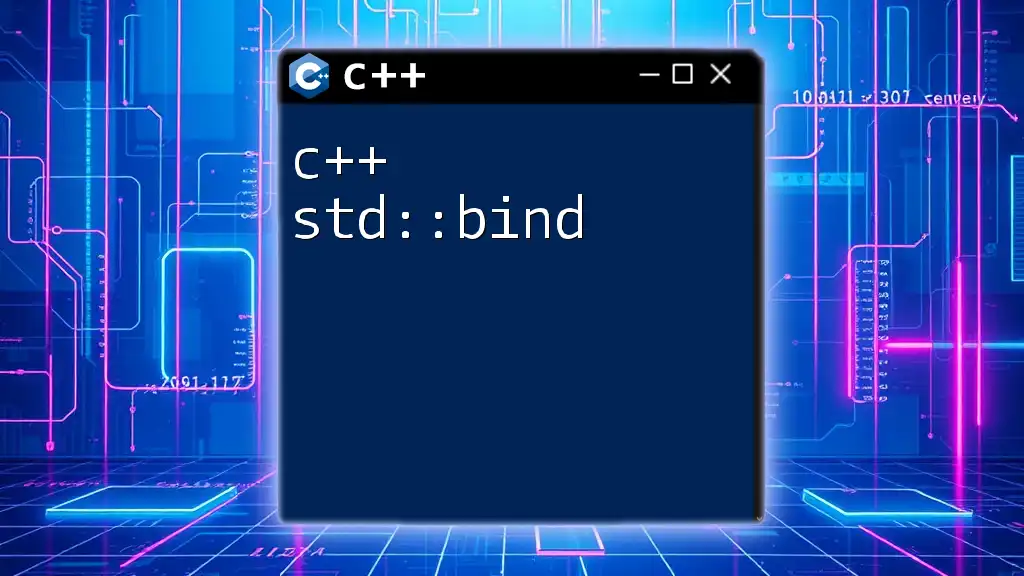
How to Include std::find
Before you start using `std::find`, you need to include the appropriate header. To utilize this function, include the following directive at the top of your C++ source file:
#include <algorithm>
This line ensures that your program can access all the functionalities of the STL algorithms, including `std::find`. Moreover, you generally need to use the `std` namespace to access `std::find`, or you can simply declare `using namespace std;` if you prefer.
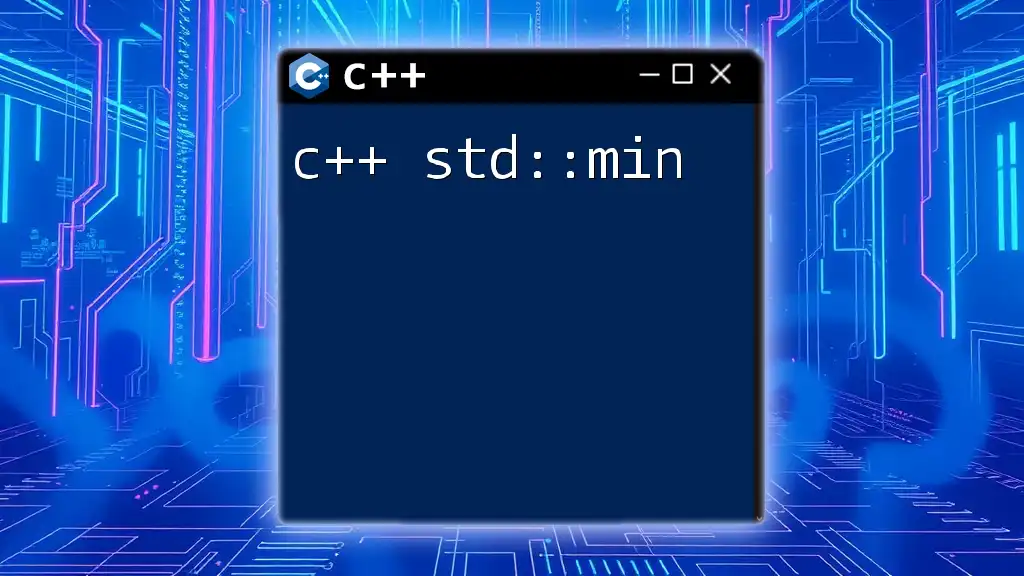
Syntax of std::find
The syntax of `std::find` is straightforward, allowing you to find elements concisely. Here's the basic syntax:
auto result = std::find(first, last, value);
Parameters Explained
- `first`: This is an iterator marking the beginning of the range you intend to search.
- `last`: This iterator marks the end of the search range (exclusive).
- `value`: The value you are searching for within the specified range.
Return Value
The return value can be interpreted as follows:
- If `std::find` finds the element, it returns an iterator pointing to the first occurrence.
- If the value is not found, it returns the same value as `last`, which helps easily check for presence.
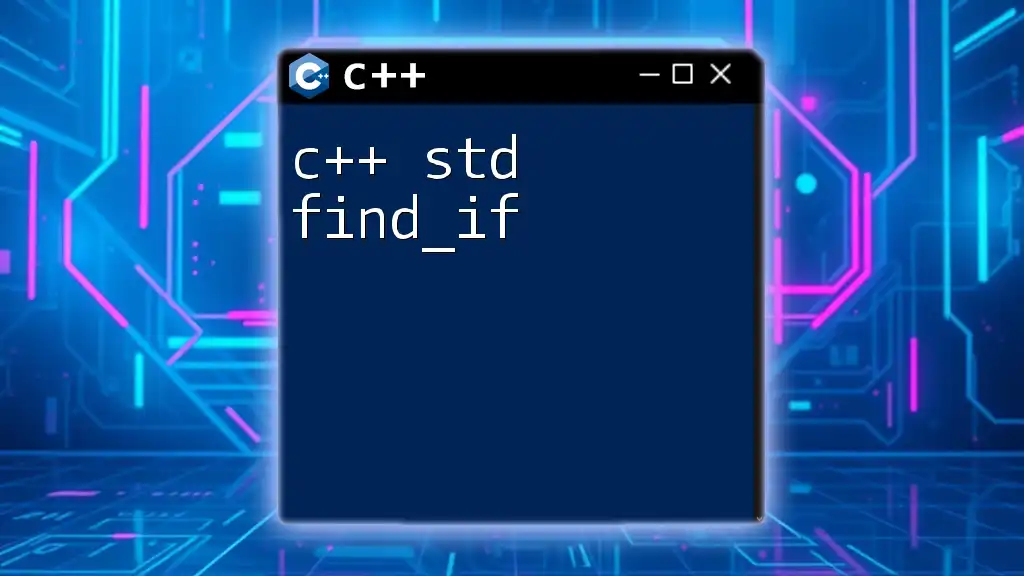
Examples of std::find
Example 1: Finding an Element in a Vector
To illustrate how `std::find` operates, let’s consider a simple example where we search for an element in a vector:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
auto it = std::find(vec.begin(), vec.end(), 3);
if (it != vec.end()) {
std::cout << "Element found: " << *it << std::endl;
} else {
std::cout << "Element not found." << std::endl;
}
return 0;
}
In this example, we define a vector containing integers from 1 to 5. We then search for the integer 3. If `3` is found, the program prints "Element found: 3"; otherwise, it prints "Element not found." Here, using `vec.end()` as the end iterator allows us to compare whether the search was successful.
Example 2: Handling Element Not Found
Continuing with our previous concept, let’s handle a case where the searched element doesn’t exist in the container:
// Continuation from previous example
auto itNotFound = std::find(vec.begin(), vec.end(), 6);
if (itNotFound == vec.end()) {
std::cout << "Element not found (6)." << std::endl;
}
When we search for 6 in the vector, since it does not exist, the output will indicate that the value is absent. This behavior highlights the versatility and effectiveness of `std::find` for both existing and non-existing elements.
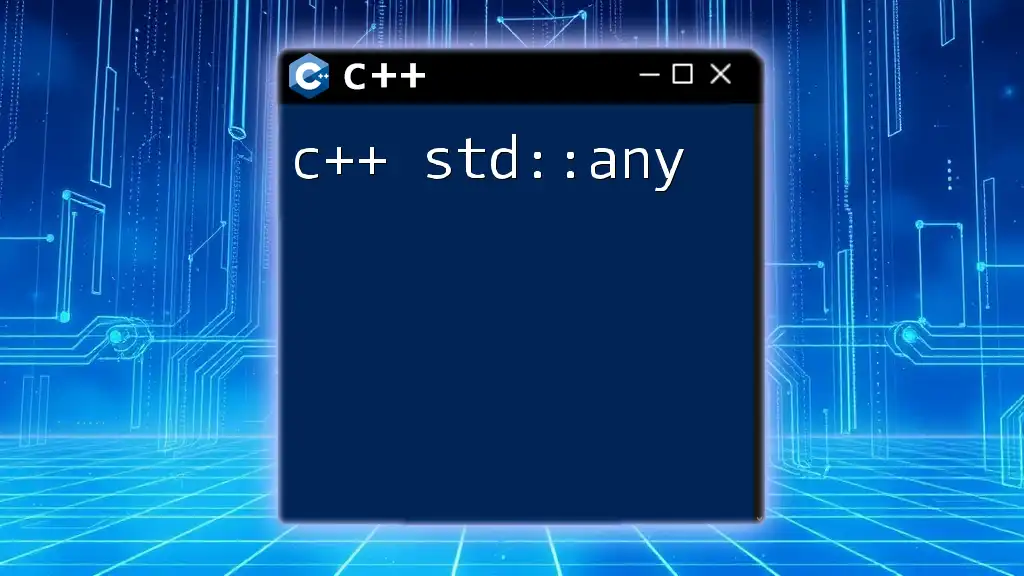
Advanced Usage of std::find
Finding Unique Elements
While `std::find` is excellent for straightforward searches, you might combine it with algorithms like `std::unique` to identify unique elements in a list. Using `std::find` in conjunction with such algorithms enhances your data handling capabilities in C++.
Custom Search with Predicate
For more complex searches, consider using `std::find_if`, which allows for a custom search based on specified conditions. This method takes a predicate (a function or lambda expression) to determine if an element meets particular criteria. For example:
auto it = std::find_if(vec.begin(), vec.end(), [](int x) { return x > 3; });
In this snippet, we search for the first element greater than 3. Utilizing `std::find_if` provides much flexibility for tailored searches, allowing for specific criteria to be met.
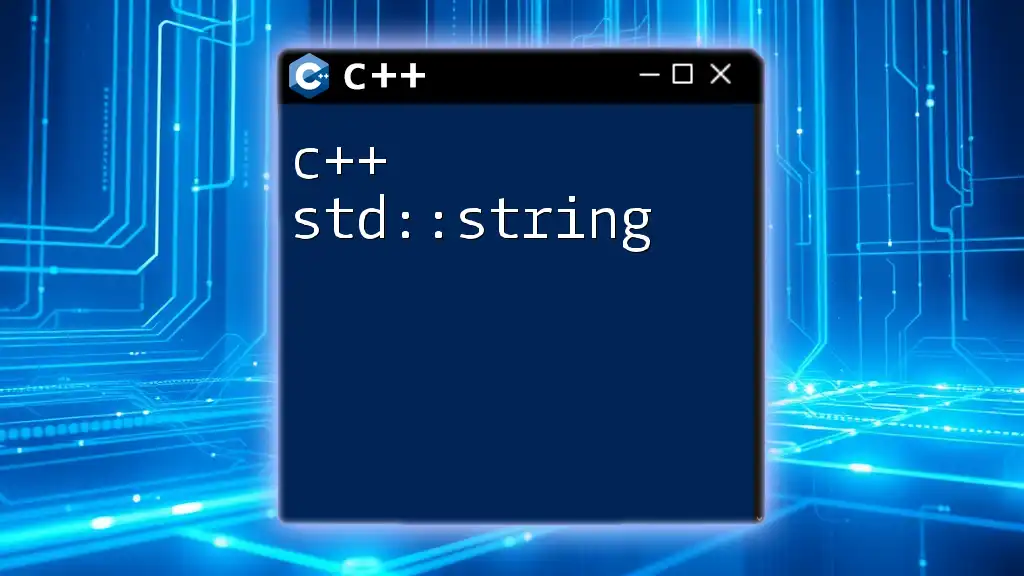
Performance Considerations
When employing `std::find`, it is essential to understand its performance implications. The complexity of `std::find` is O(n), meaning it may require traversing the entire range in the worst-case scenario. Thus, while `std::find` is simple and effective for small datasets, consider other more efficient algorithms if your dataset is larger or performance-critical.
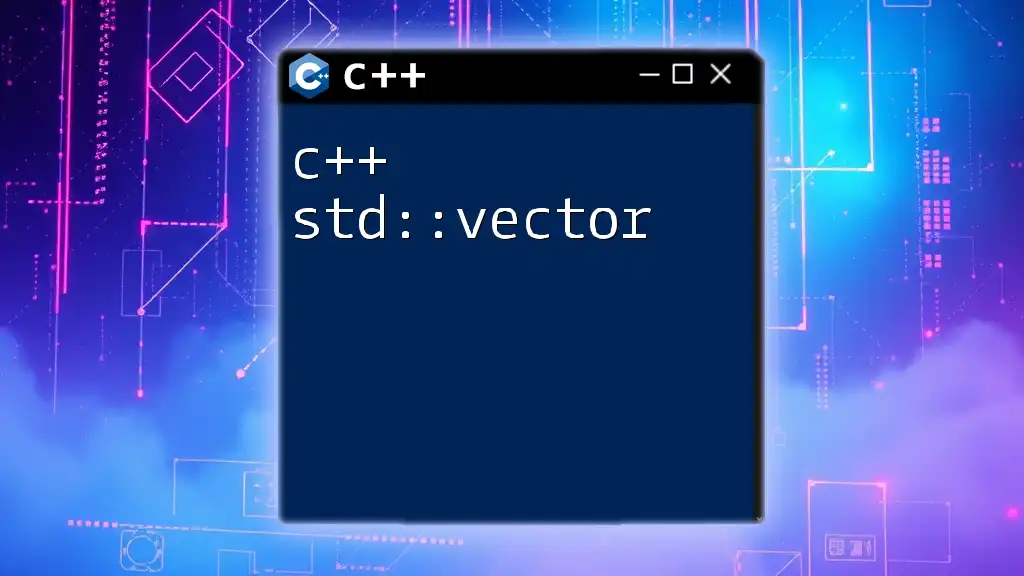
Common Mistakes
A few common pitfalls can hinder the proper use of `std::find`:
- Iterators: Always ensure that you are using valid iterators. Accessing an invalid iterator will lead to undefined behavior.
- Type Mismatches: Ensure that the type of the element being searched for matches the type contained within the collection. Mismatched types can lead to unexpected results.
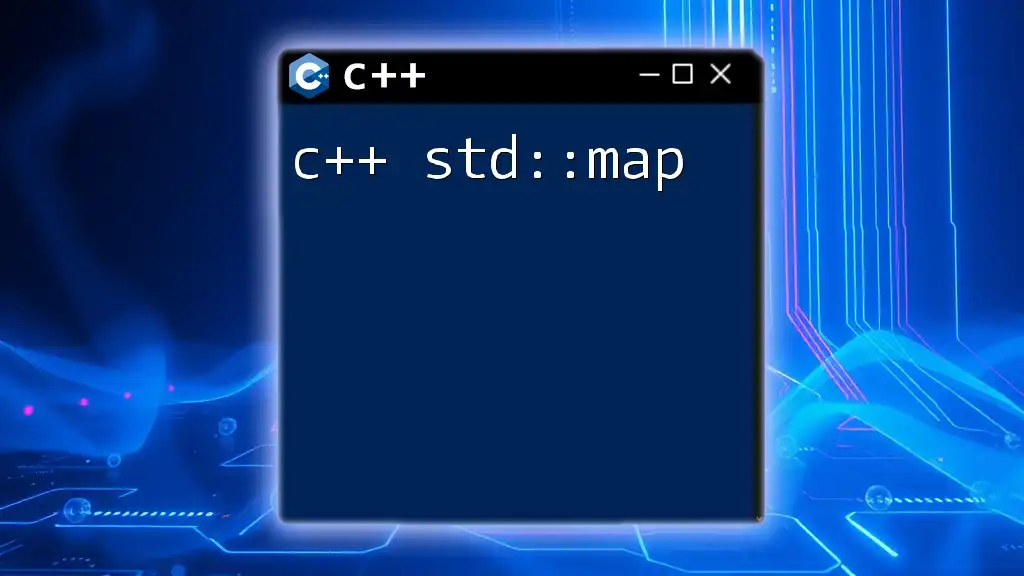
Conclusion
In summary, `c++ std::find` is a vital tool in your C++ arsenal, facilitating easy and efficient searches through collections. By understanding its workings and best practices, you can significantly enhance your programming capabilities. Embrace this functionality to streamline your C++ development process and effectively manage data.
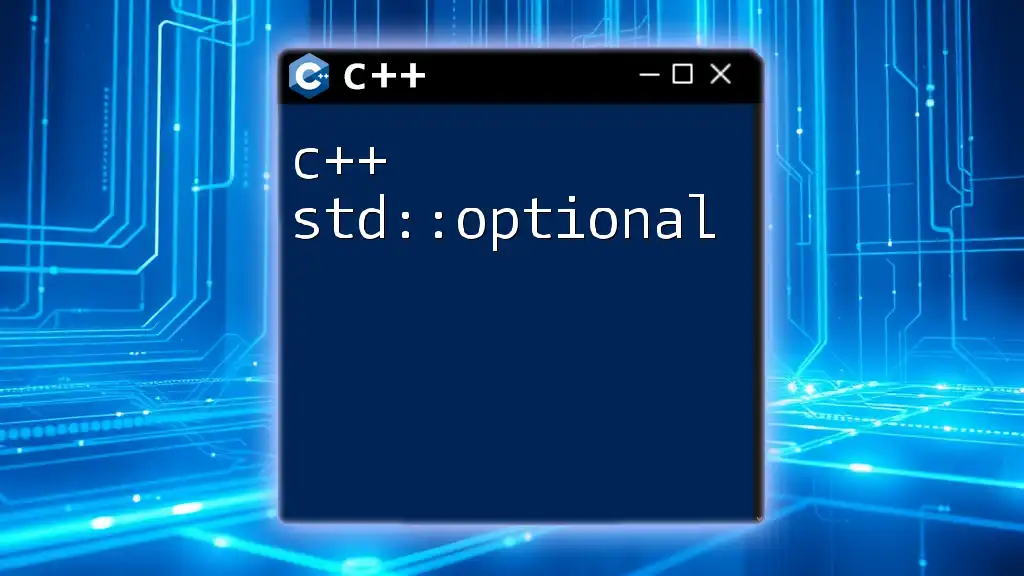
Additional Resources
For further exploration, consult the official STL documentation and consider engaging with books or tutorials dedicated to C++ algorithms to deepen your knowledge and expertise.