The `std::find_if` function in C++ is used to search for the first element in a range that satisfies a specified predicate (condition).
Here’s a code snippet demonstrating its usage:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5, 6};
auto it = std::find_if(numbers.begin(), numbers.end(), [](int n) { return n > 4; });
if (it != numbers.end()) {
std::cout << "First number greater than 4 is: " << *it << std::endl;
}
return 0;
}
Understanding `std::find_if`
Definition of `std::find_if`
`std::find_if` is a part of the C++ Standard Library and is included in the `<algorithm>` header. It is designed to search through a range of elements to find the first element that satisfies a specified condition or predicate. This makes it an invaluable tool for efficient searching within containers.
When to Use `std::find_if`
You should consider using `std::find_if` when you need to locate an element that meets specific criteria, rather than just matching an exact value. Its flexibility allows for complex conditions through user-defined predicates, making it useful in various programming scenarios.
How `std::find_if` Works
The function works by taking three primary parameters: two iterators that define the range of search, and a predicate (a condition that returns true or false). `std::find_if` then iterates over the elements within the specified range until it finds one that meets the predicate's criteria.
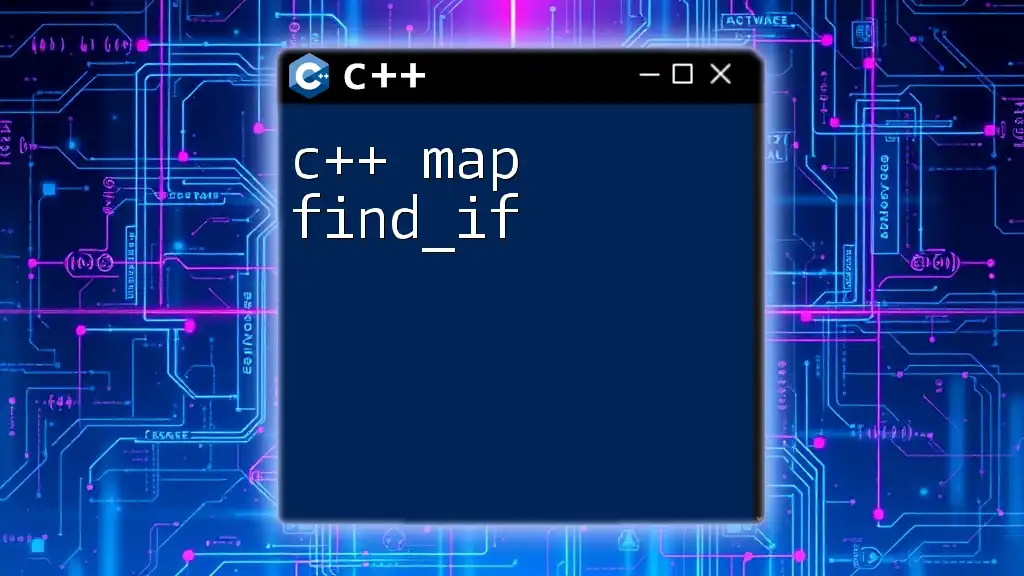
Syntax of `std::find_if`
Basic Syntax
The syntax for `std::find_if` is as follows:
auto result = std::find_if(begin, end, predicate);
- begin: An iterator pointing to the first element of the range.
- end: An iterator pointing to one past the last element of the range.
- predicate: A callable object (like a function or lambda expression) that determines the condition for element selection.
Return Value
The function returns an iterator to the first element that satisfies the predicate if found; otherwise, it returns the end iterator (indicating that no element was found).
Template Parameters
`std::find_if` is a templated function. This means it can work with any data type as long as the data type's elements can be compared with the conditions defined in the predicate.
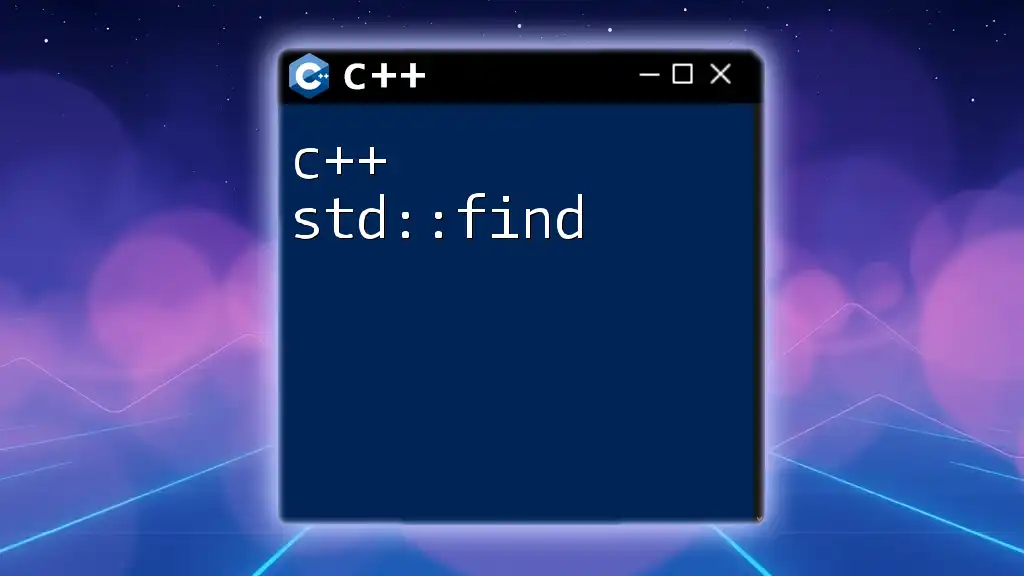
Setting Up Your Environment
Including Necessary Headers
To use `std::find_if`, make sure to include the `<algorithm>` header, as well as any container headers you plan on using. For example:
#include <algorithm>
#include <vector>
Using Namespaces
One common practice in C++ is to simplify code by applying the namespace directive:
using namespace std;
While this can make your code cleaner, it's essential to be mindful of potential naming conflicts.
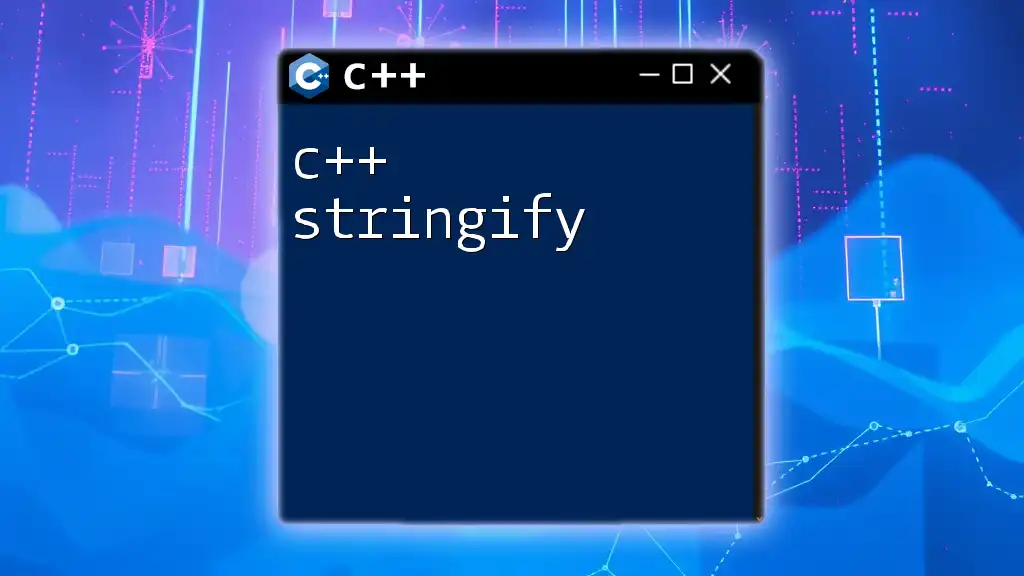
Practical Examples of Using `std::find_if`
Example: Finding Odd Numbers in a Vector
Here's how `std::find_if` can be used to find the first odd number in a vector of integers:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> numbers = {2, 4, 6, 7, 8};
auto it = std::find_if(numbers.begin(), numbers.end(), [](int n) { return n % 2 != 0; });
if (it != numbers.end()) {
std::cout << "First odd number: " << *it << std::endl;
} else {
std::cout << "No odd numbers found." << std::endl;
}
return 0;
}
Explanation of the Code
In this example, we define a vector of integers and use a lambda function as the predicate. The lambda checks if a number is odd (`n % 2 != 0`). If an odd number is found, we print it out; otherwise, we notify the user that no odd numbers were found. This showcases the versatility of predicates in `std::find_if`.
Example: Finding a String in a List
Let's extend our understanding by finding the first name longer than five characters in a list:
#include <iostream>
#include <list>
#include <algorithm>
#include <string>
int main() {
std::list<std::string> names = {"Alice", "Bob", "Charlie", "Diana"};
auto it = std::find_if(names.begin(), names.end(), [](const std::string& name) { return name.length() > 5; });
if (it != names.end()) {
std::cout << "First name longer than 5 characters: " << *it << std::endl;
} else {
std::cout << "No names longer than 5 characters found." << std::endl;
}
return 0;
}
Explanation of the Code
In this snippet, we use a list of strings. The function looks for the first name that exceeds five characters in length. By employing a lambda function as our predicate, `std::find_if` allows us to encapsulate our condition concisely. Once again, we check if the iterator `it` is valid before accessing the found name.
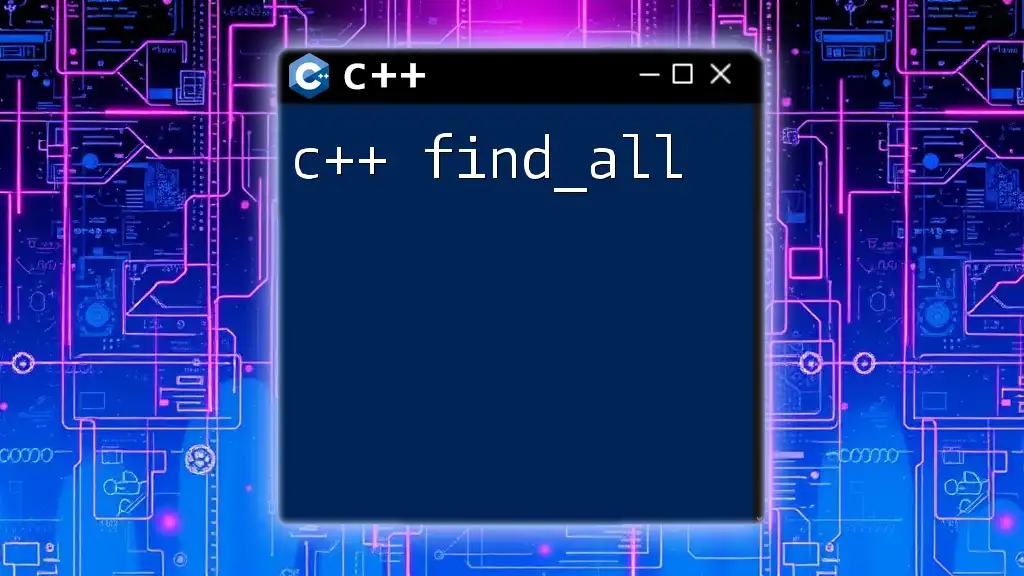
Performance Considerations
Time Complexity
The time complexity of `std::find_if` is O(n), where `n` is the number of elements in the range. This means that in the worst-case scenario, it will need to check each element until it finds a match or reaches the end.
When to Optimize
If you frequently perform searches in static collections or require significant performance for a large dataset, consider using other data structures or algorithms, such as binary search (for sorted collections) or a hash table for constant-time lookups.
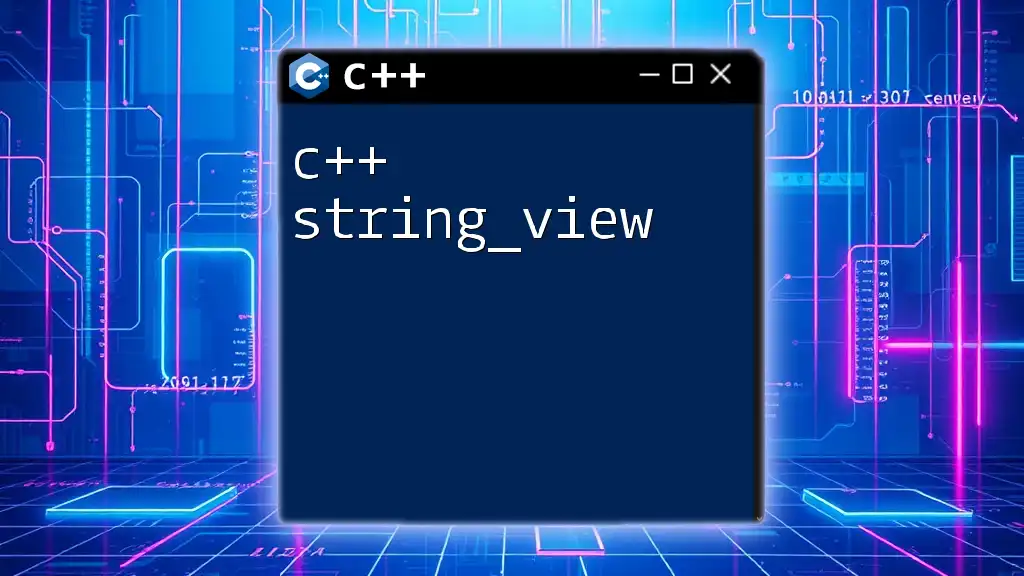
Error Handling
What Happens When the Element is Not Found
If no elements in the specified range meet the predicate's condition, `std::find_if` returns an iterator equal to `end`. It's essential to check for this condition before dereferencing the iterator to avoid accessing invalid memory.
Best Practices in Error Checking
Always validate your iterators. Use the following pattern:
if (it != container.end()) {
// Safe to dereference
} else {
// Handle the case where no match was found
}
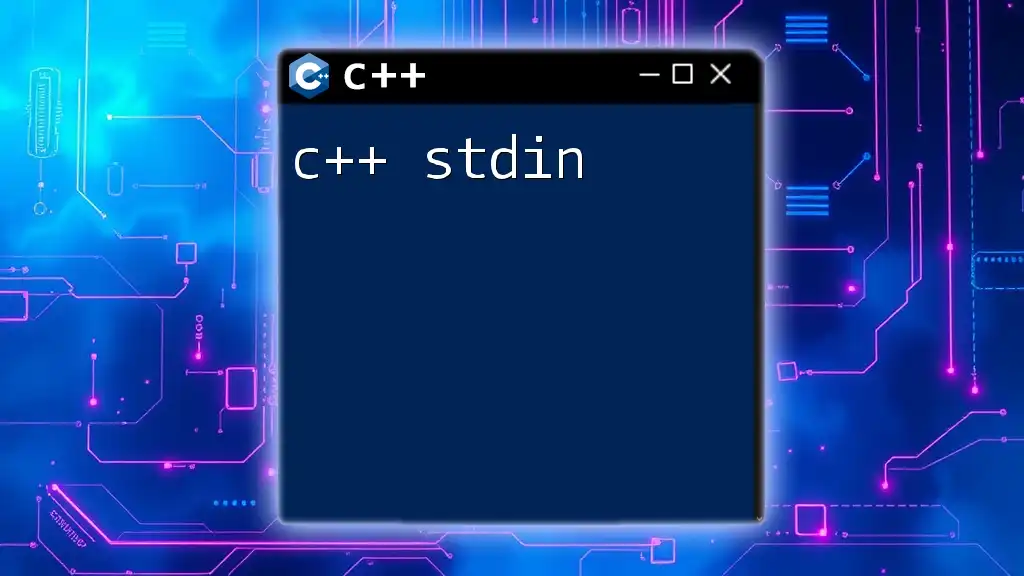
Summary
In summary, `std::find_if` is a powerful function within the C++ Standard Library that allows you to search through containers using custom predicates. Its ability to work with any data structure and flexible use of callable objects make it invaluable when searching for elements that meet specific criteria. Remember to consider performance implications in large collections, and ensure that you handle potential errors gracefully.
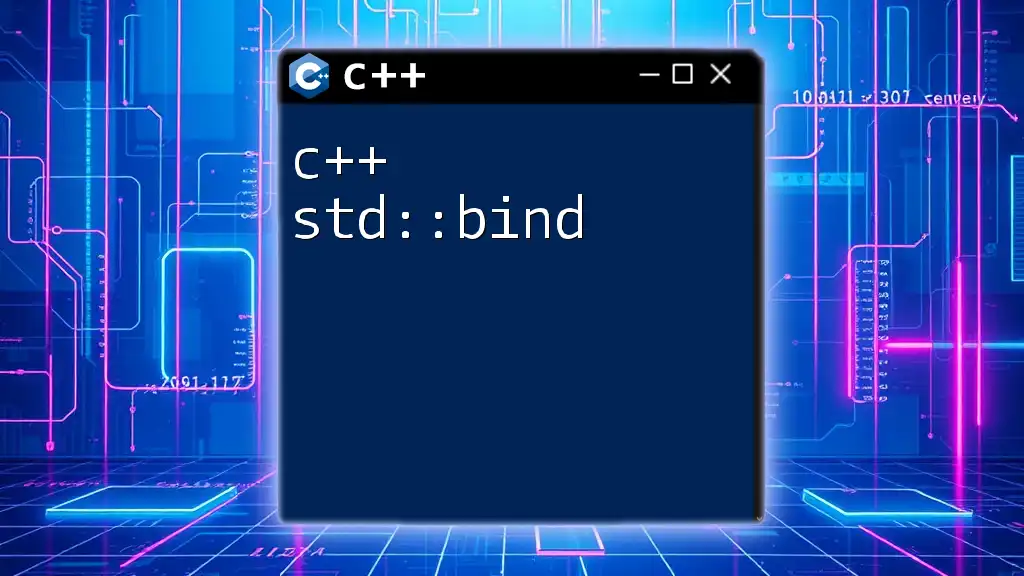
Additional Resources
For further exploration of `std::find_if` and the C++ Standard Library, consult the official C++ documentation or refer to reputable C++ programming books and online courses. Getting familiar with this function will significantly enhance your capabilities in mastering C++.
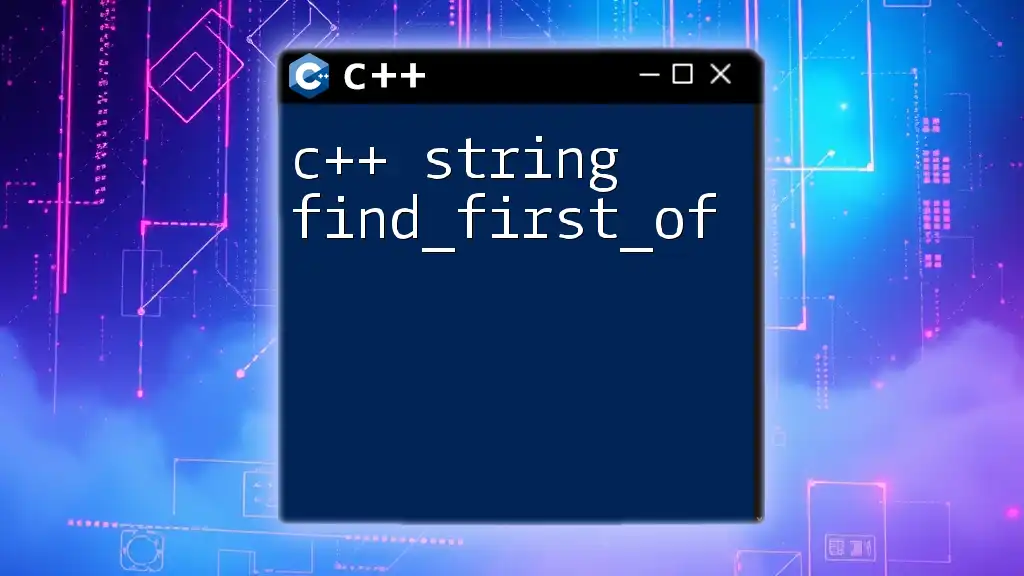
Call to Action
Experiment with the examples provided, modifying them to suit your needs and criteria. Share your experiences in the comments, and don't hesitate to reach out for more tips and tutorials on mastering C++!