In C++, the `find_if` function can be used to search for an element in a `map` that satisfies a specified predicate, returning an iterator to the first element that meets the condition, or the end iterator if no such element is found.
Here’s a code snippet demonstrating its usage:
#include <iostream>
#include <map>
#include <algorithm> // for std::find_if
int main() {
std::map<int, std::string> myMap = {{1, "one"}, {2, "two"}, {3, "three"}};
auto it = std::find_if(myMap.begin(), myMap.end(), [](const auto& pair) {
return pair.first > 1; // Predicate to find keys greater than 1
});
if (it != myMap.end()) {
std::cout << "Found: " << it->first << " -> " << it->second << std::endl;
} else {
std::cout << "No element found." << std::endl;
}
return 0;
}
Understanding `std::map`
What is `std::map`?
`std::map` is an associative container in C++ that stores elements as key-value pairs, where each key is unique and serves as an identifier for the corresponding value. This structure is particularly useful when you need to maintain a sorted collection of elements and quickly access them using keys.
The key characteristics of `std::map` include:
- Ordered: Elements in a `std::map` are stored in a specific order based on the key. The default behavior is to store the keys in ascending order.
- Unique Keys: Each key in a `std::map` must be unique. If you attempt to insert an element with a key that already exists, the new value will replace the existing one.
- Complex Keys: You can use complex data types as keys, as long as they define a strict weak ordering.
Choosing to use `std::map` can help you efficiently manage data that needs quick lookup times, such as databases or dictionaries.
Basic Operations of `std::map`
To make the most of `std::map`, it is essential to understand its basic operations:
- Insertion of Elements: You can add elements to a `std::map` using the `insert()` function or the subscript operator `[]`.
- Deletion of Elements: Elements can be removed using `erase()`, providing both key and iterator options.
- Accessing Elements by Key: You can retrieve values by their keys using the subscript operator or `at()` method.

The `find_if` Algorithm
What is `find_if`?
The `find_if` algorithm in the C++ Standard Library is a powerful tool for searching through a range of elements. It accepts a range (like iterators) and a predicate (a function or lambda that returns a boolean), and it searches for the first element that matches the condition defined by the predicate.
The significance of `find_if` lies in its flexibility, allowing developers to specify their search criteria through predicates, enabling easy customization of search operations.
How `find_if` Works
The `find_if` function employs iterators to traverse a specified range. It checks each element against the predicate and stops when it finds the first match, returning an iterator to that element.
Using `find_if` with `std::map`
Syntax and Parameters
When using `find_if` with `std::map`, the syntax generally looks like this:
std::find_if(map.begin(), map.end(), predicate);
Parameters:
- The input range is defined by the `begin()` and `end()` methods of the map, allowing you to sequentially access each element.
- The predicate is a function or lambda expression that accepts a `std::pair<const KeyType, ValueType>` and returns a boolean.
Example Usage of `find_if`
Simple Example
Here's a basic example demonstrating how to use `find_if` with a `std::map`:
#include <iostream>
#include <map>
#include <algorithm>
int main() {
std::map<int, std::string> myMap = {{1, "Apple"}, {2, "Banana"}, {3, "Cherry"}};
auto it = std::find_if(myMap.begin(), myMap.end(), [](const std::pair<int, std::string>& element) {
return element.second == "Banana";
});
if (it != myMap.end()) {
std::cout << "Found: " << it->first << " : " << it->second << '\n';
} else {
std::cout << "Not found\n";
}
return 0;
}
In this example, we have a `std::map` of integers to strings representing fruit names. The `find_if` function utilizes a lambda function as a predicate to search for the key associated with "Banana".
If found, it displays the corresponding key; if not found, it outputs "Not found". This mechanism provides a systematic way to search based on specific conditions.
Advanced Example
Let's consider a more advanced example where we create a custom predicate to search for fruits with names longer than three characters:
#include <iostream>
#include <map>
#include <algorithm>
bool isLongerThanThree(const std::pair<int, std::string>& element) {
return element.second.length() > 3;
}
int main() {
std::map<int, std::string> myMap = {{1, "Apple"}, {2, "Banana"}, {3, "Kiwi"}};
auto it = std::find_if(myMap.begin(), myMap.end(), isLongerThanThree);
if (it != myMap.end()) {
std::cout << "Found: " << it->first << " : " << it->second << '\n';
} else {
std::cout << "Not found\n";
}
return 0;
}
In this scenario, the `isLongerThanThree` function checks if the length of a fruit name exceeds three characters. When `find_if` is executed, it finds the first fruit whose name is longer than three characters and outputs its key and value.
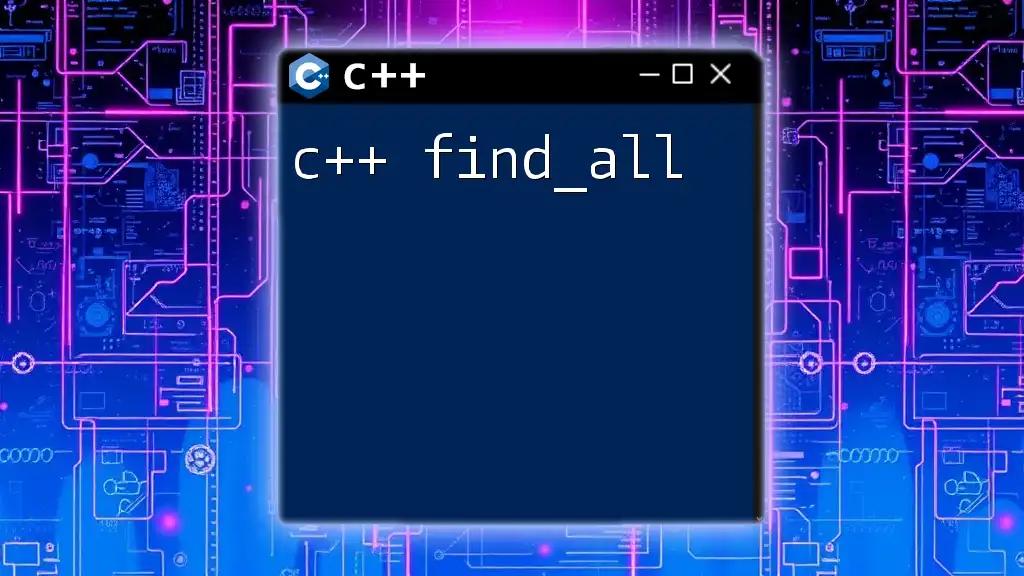
Performance Considerations
Time Complexity
The time complexity of the `find_if` algorithm when used with `std::map` is O(N) in the worst case, as it may need to examine all elements. While `std::map` provides logarithmic time complexity for standard operations like insertion and access, `find_if` iterates linearly through the elements because it doesn't take advantage of the map's ordered structure.
When to Use `find_if`
Use `find_if` when you have complex search criteria that go beyond simple key access. It's particularly useful when working with custom predicates but remember to consider the performance implications due to its linear search nature.
If you need simple key-based lookups, consider using the `find()` method or the subscript operator instead, which will yield better performance.
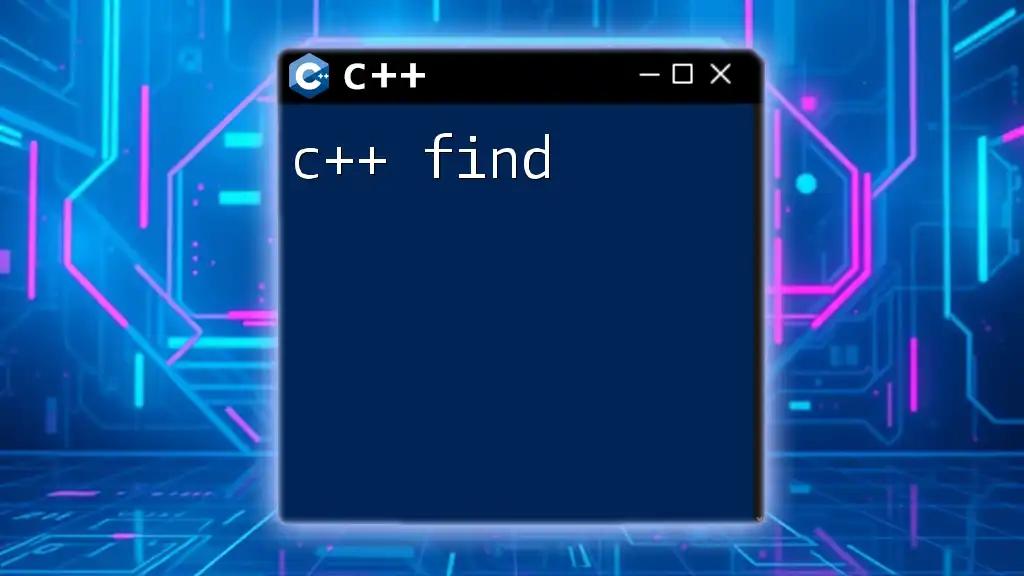
Conclusion
In summary, the `c++ map find_if` functionality is crucial for finding elements in a `std::map` based on a specified condition. Understanding how to use this algorithm effectively can greatly enhance the efficiency and readability of your code, allowing more flexible and powerful searches within your data structures. Practice with different predicates and examples to deepen your understanding of this powerful C++ feature.
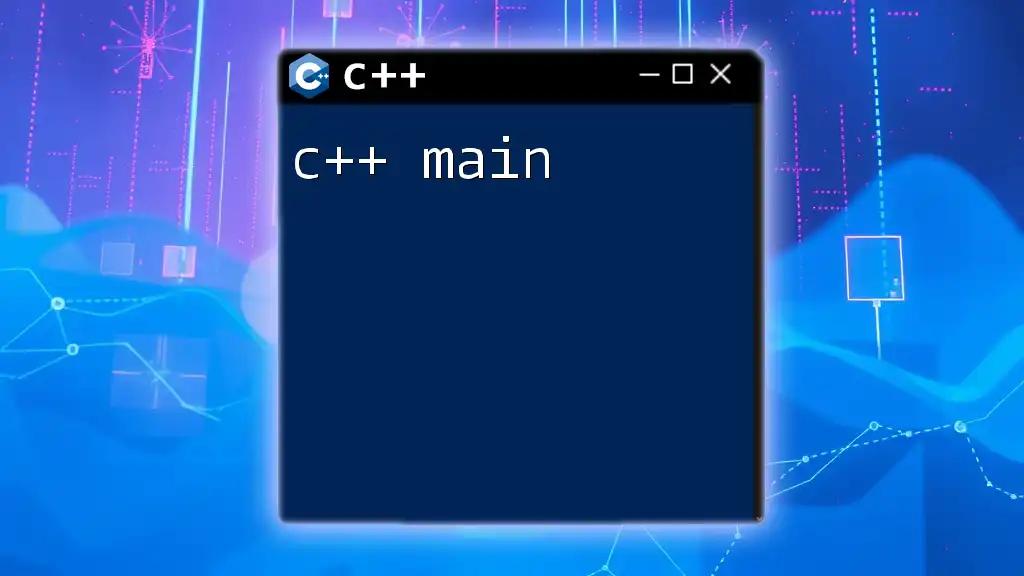
Additional Resources
For further exploration, refer to the official C++ documentation on `std::map` and `find_if`. Engaging with community resources and forums can also provide valuable insights and support as you continue to develop your C++ skills.