The `find_all` functionality in C++ is often used to retrieve all occurrences of a specific element in a container, typically achieved through the standard library's algorithms.
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 2, 5, 2};
std::vector<int>::iterator it = std::find(numbers.begin(), numbers.end(), 2);
while (it != numbers.end()) {
std::cout << "Found 2 at index: " << std::distance(numbers.begin(), it) << std::endl;
it = std::find(std::next(it), numbers.end(), 2);
}
return 0;
}
What is C++?
C++ is a powerful general-purpose programming language that offers high performance and flexibility. It is widely used in system/software development, game programming, and in applications requiring real-time processing due to its efficiency. C++ supports object-oriented programming principles, enabling better organization and modularity of code—a crucial aspect for managing large and complex applications.
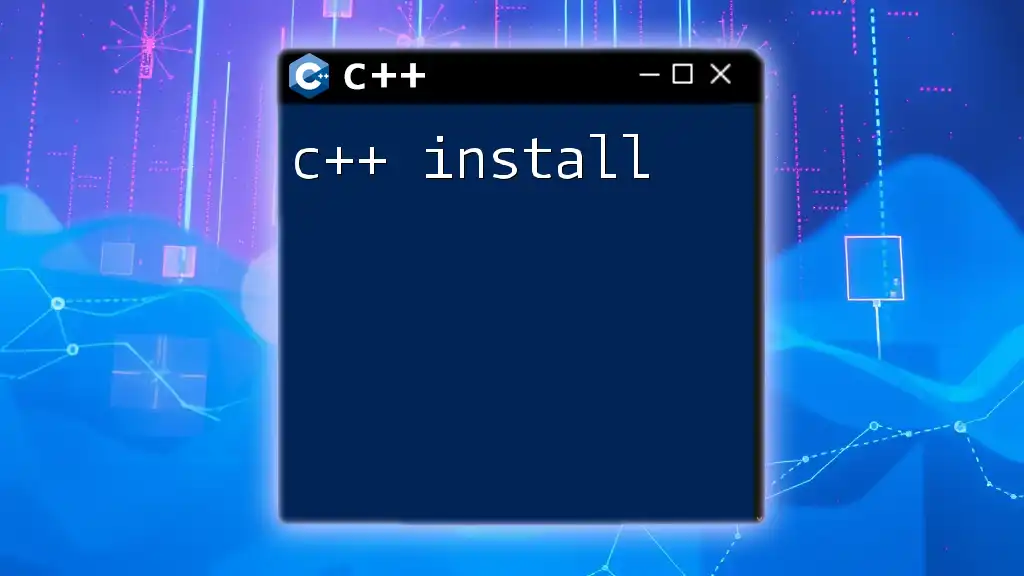
Importance of String Manipulation
String manipulation is fundamental in programming because it allows developers to handle text efficiently. Whether it’s validating user input, parsing data, or performing search operations, manipulating strings is an everyday task. Using the right tools and techniques can significantly enhance performance and ease of coding.
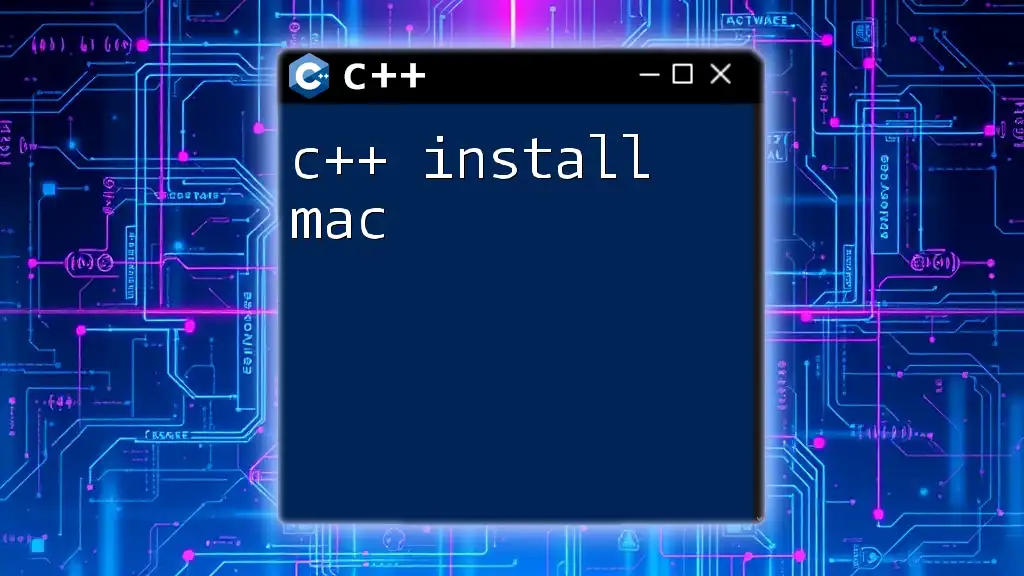
Understanding find_all in C++
What is find_all?
The term "find_all" refers to a function or utility that detects the locations of all occurrences of a specified substring within a given string. In C++, while the standard library provides functionalities to find substrings, having a custom `find_all` implementation greatly simplifies this process, especially when you need to collect all indices where a substring appears.
Basic Concepts
In C++, strings are represented by the `std::string` class, which provides a rich set of functionalities. The standard string library (`<string>`) is essential for any string manipulation tasks and includes methods like `find()`, which can locate a substring's first occurrence.
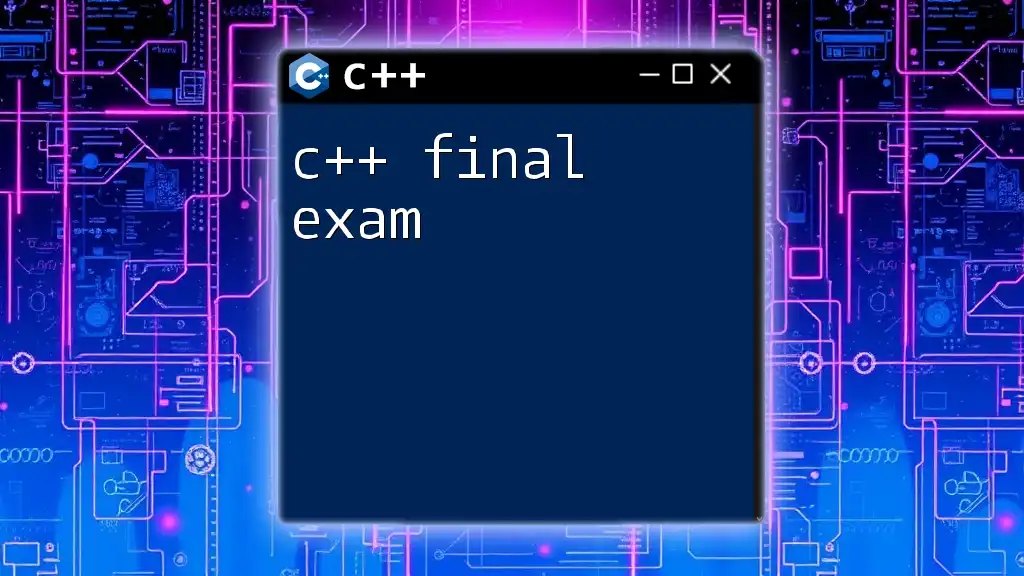
Developing Your Own find_all Function
Requirements
When creating a `find_all` function, the primary requirements include:
- An input string in which the search will take place.
- A substring (the key string) that you want to find.
Crafting the Function
Here's how to implement a custom `find_all` function in C++.
Code Snippet
#include <iostream>
#include <string>
#include <vector>
std::vector<size_t> find_all(const std::string& str, const std::string& to_find) {
std::vector<size_t> positions;
size_t pos = str.find(to_find);
while (pos != std::string::npos) {
positions.push_back(pos);
pos = str.find(to_find, pos + to_find.length());
}
return positions;
}
Explanation of the Code
- Include Necessary Headers: The code snippet starts with including the `<iostream>`, `<string>`, and `<vector>` headers which are required for input/output operations, string manipulation, and dynamic arrays (vector).
- Function Parameters: The function `find_all` takes two parameters: a constant reference to the string `str` that needs to be searched and another constant reference for the substring `to_find`. It returns a vector of size_t containing the positions of all found occurrences.
- Using std::string::find(): The `find` method is essential here as it searches for the `to_find` substring in the given string `str`. It returns the position of the first occurrence or `std::string::npos` if the substring is not found.
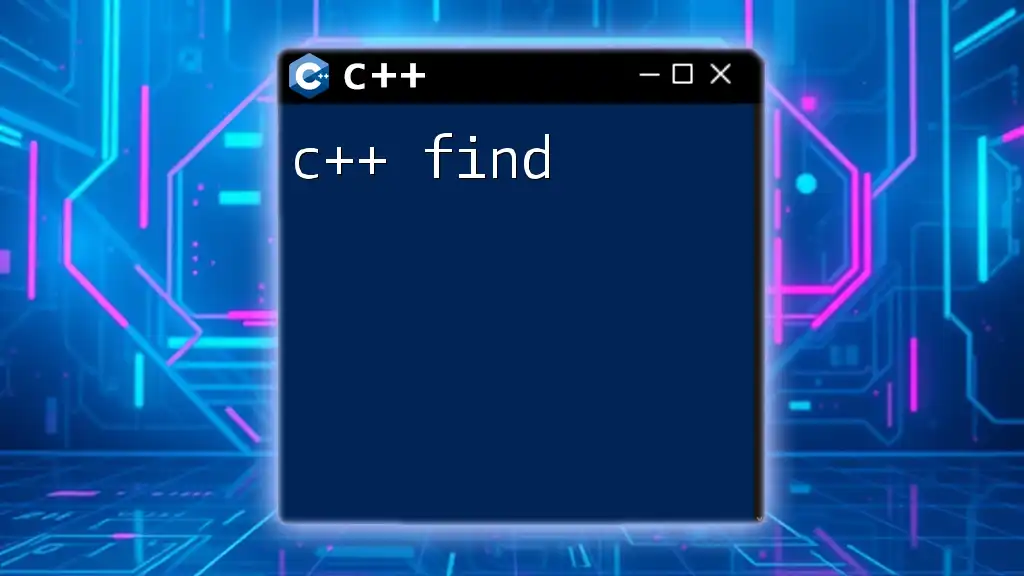
Testing the find_all Function
Example Use Case
Let's illustrate the use of our `find_all` function with a practical example where we need to find all occurrences of the word "find" in a given sentence.
Code Snippet
int main() {
std::string text = "Find the find_all occurrences of the word find.";
std::vector<size_t> positions = find_all(text, "find");
for (size_t pos : positions) {
std::cout << "Found at position: " << pos << std::endl;
}
return 0;
}
Running the Program
To compile and run this C++ program, you can use any C++ compiler. For example, with `g++`, you can run:
g++ -o find_example find_example.cpp
./find_example
The expected output of this program would be:
Found at position: 0
Found at position: 35
Found at position: 41
This output indicates the positions where the substring "find" appears within the text.
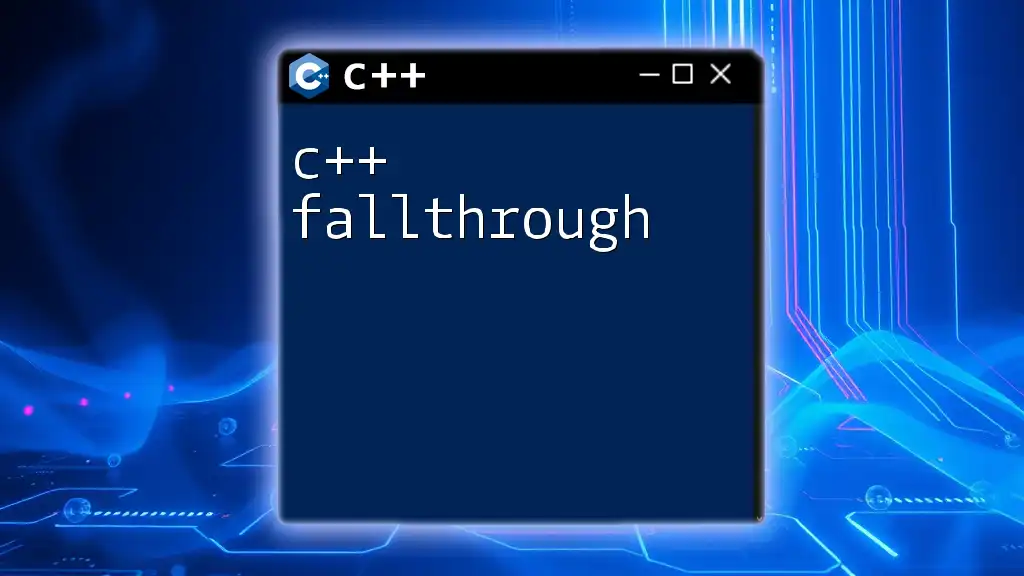
Advantages of the Custom find_all Function
Flexibility
One significant advantage of crafting your own `find_all` function is flexibility. Developers can easily adjust the function to incorporate case sensitivity or even enhance it by adding the capability to find overlapping occurrences.
Performance Considerations
Although the provided implementation is straightforward, it’s essential to keep performance in mind. Searching through large strings multiple times can be inefficient. Hence, looking into potential optimizations, such as using substring hashing or advanced algorithms like Knuth-Morris-Pratt, may be beneficial as your usage scale increases.
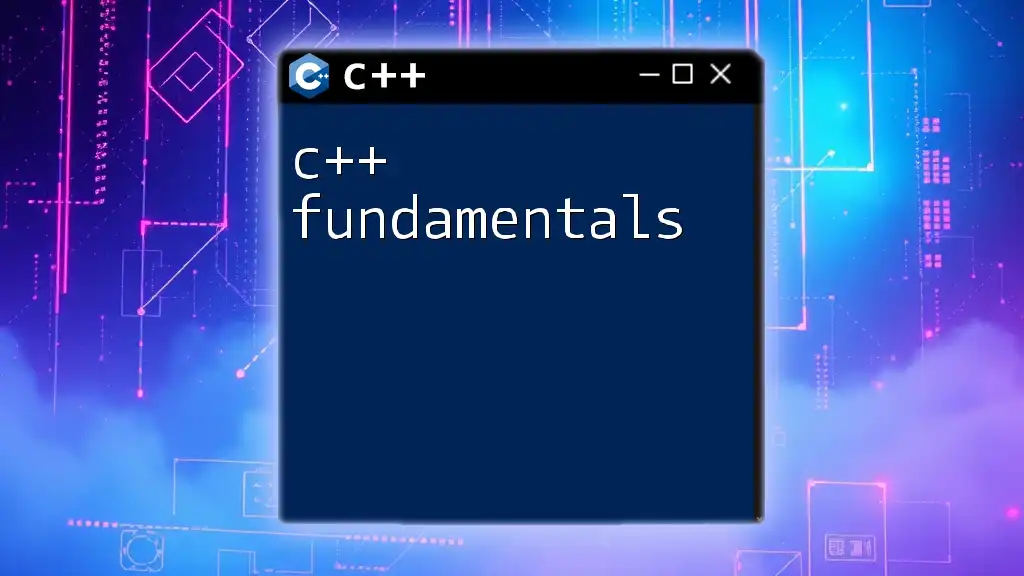
Alternatives to find_all in C++
Using Regular Expressions
For cases where patterns or more complex searches are involved, C++ offers the `<regex>` library for regular expression handling. Using regular expressions can simplify the process of finding patterns in text.
Code Snippet
#include <iostream>
#include <regex>
void find_with_regex(const std::string& text, const std::string& pattern) {
std::regex regex_pattern(pattern);
auto words_begin = std::sregex_iterator(text.begin(), text.end(), regex_pattern);
auto words_end = std::sregex_iterator();
for (std::sregex_iterator i = words_begin; i != words_end; ++i) {
std::cout << "Found: " << i->str() << std::endl;
}
}
When to Use Alternatives
Regular expressions come in handy when you need to search for patterns, such as finding words with specific prefixes or suffixes. However, for straightforward substring searches, a `find_all` implementation remains efficient and easy to understand.
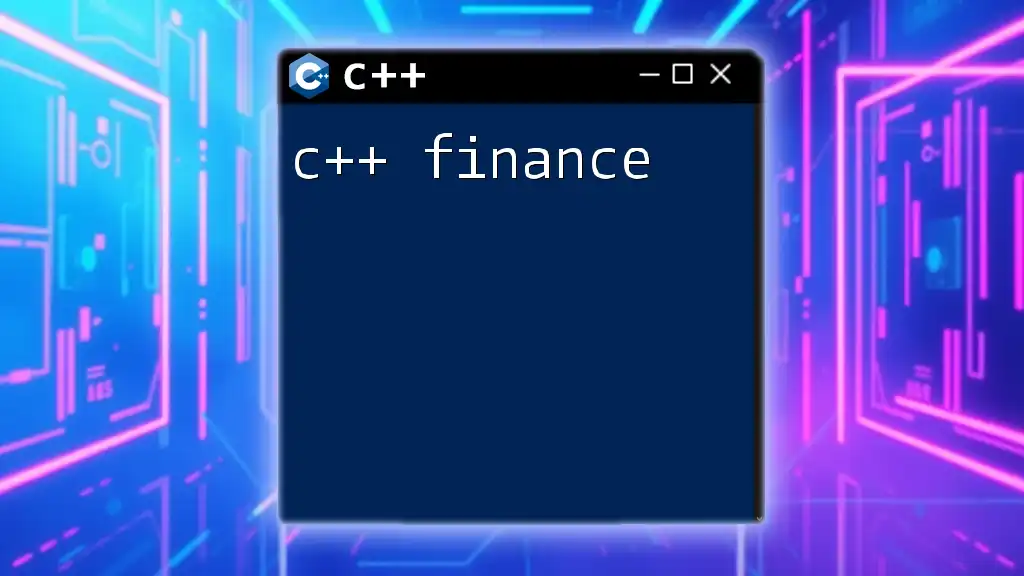
Conclusion
In conclusion, the function `c++ find_all` is a valuable tool for any developer working with strings. It allows for efficient searching and indexing of substrings, thus streamlining text processing tasks. By practicing and experimenting with this function, you enable yourself to master string manipulation—an essential skill for any programmer.
As you embark on this journey of learning C++, consider exploring more about string techniques, other search algorithms, and advanced programming concepts specific to C++. Engaging with these topics will undoubtedly enhance your skill set and prepare you for tackling complex problems in real-world applications.