C++ on Windows allows developers to create powerful applications by leveraging the Windows API for system-level programming and user interface integration.
Here's a simple example of a C++ program that creates a message box in a Windows application:
#include <windows.h>
int main() {
MessageBox(0, "Hello, Windows!", "C++ on Windows", MB_OK);
return 0;
}
Understanding Windows Programming Basics
What is Windows Programming?
Windows programming refers to the creation of software applications that run on Microsoft Windows operating systems. It encompasses a range of technologies and languages, with C++ being a prominent choice due to its powerful features and performance capabilities. Windows programming has evolved significantly since the early versions, making use of advanced graphical interfaces, networking capabilities, and improved system-level access.
Key Concepts in Windows Environment
Understanding the underlying principles of the Windows architecture is crucial for effective programming. The Windows environment operates on an architecture composed of processes, threads, and a message-driven system.
-
Processes: A process is an instance of a running application. Each process contains its own memory space, ensuring stability and security for the application.
-
Threads: Threads are the smallest unit of execution within a process. In C++ Windows programming, using multiple threads can enhance performance and responsiveness in applications, especially in tasks like file I/O or GUI operations.
-
Event-driven Programming: C++ applications in Windows are primarily event-driven. This means they wait for events (like user input or messages from the operating system) and respond accordingly. This model enhances the user experience by keeping the interfaces responsive.
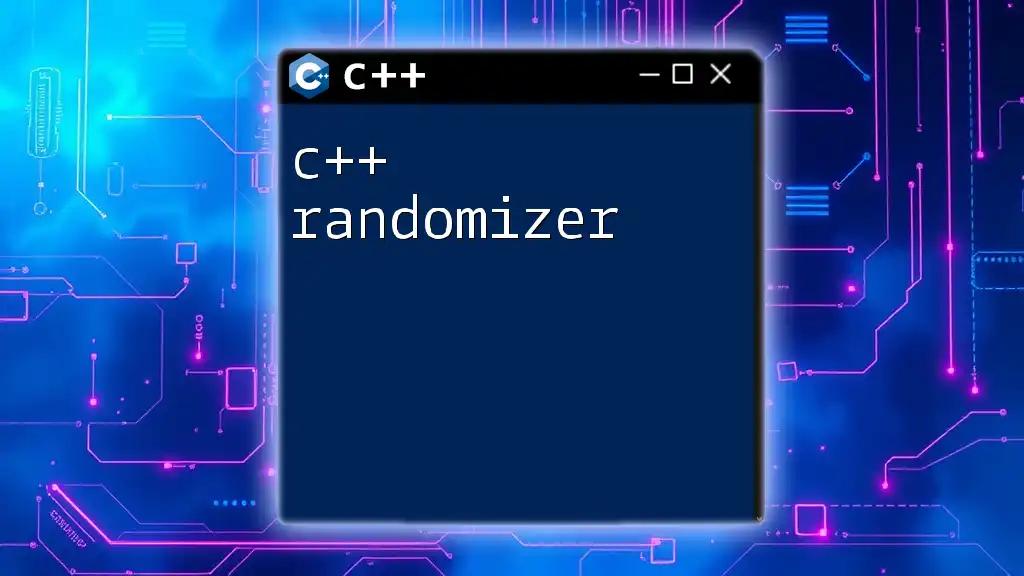
Setting Up Your Development Environment
Choosing the Right IDE
An Integrated Development Environment (IDE) can significantly ease your workflow when developing C++ applications for Windows. Popular IDEs like Visual Studio and Code::Blocks offer powerful features such as debugging tools, syntax highlighting, and GUI designers.
Visual Studio is particularly renowned for its deep integration with the Windows API and efficient debugging and profiling tools. In contrast, Code::Blocks is lightweight and customizable, making it a great choice for beginners.
Installing Necessary Tools
To kickstart C++ development on Windows, follow these steps to install Visual Studio:
-
Download Visual Studio: Visit the official Microsoft website to download the Community edition, which is free for individual developers.
-
Install the IDE: Follow the installation instructions, ensuring to select the "Desktop development with C++" workload. This installs the necessary tools and libraries for C++ Windows programming.
-
Configure Environment Variables: Ensure that your system's PATH includes the paths to build tools and binaries, allowing for seamless command-line operations.
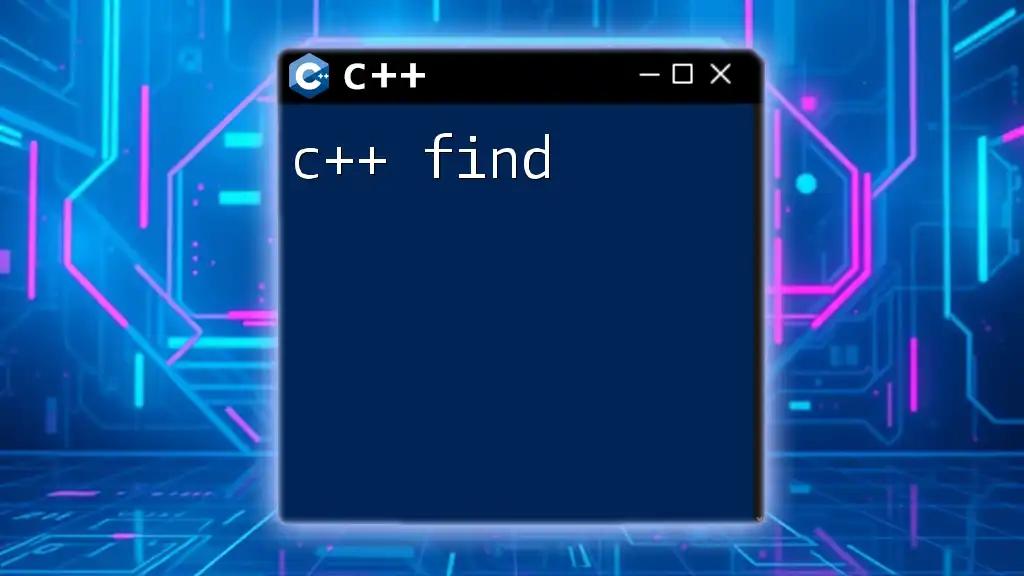
Creating Your First Windows Application
Introduction to Windows API
The Windows API (Application Programming Interface) forms the foundation for interacting with the Windows operating system. It provides a set of functions and tools to create windows, manage input/output, and handle system events. Comprehensive documentation on the Windows API is available on the Microsoft Developer Network (MSDN), which will be vital for your development process.
Writing a Simple "Hello, World!" Application
To demonstrate the basics of Windows programming, let’s create a simple "Hello, World!" application using C++ and the Windows API.
Code Snippet:
#include <windows.h>
LRESULT CALLBACK WindowProc(HWND hwnd, UINT uMsg, WPARAM wParam, LPARAM lParam) {
switch (uMsg) {
case WM_DESTROY:
PostQuitMessage(0);
return 0;
case WM_PAINT:
{
PAINTSTRUCT ps;
HDC hdc = BeginPaint(hwnd, &ps);
FillRect(hdc, &ps.rcPaint, (HBRUSH)(COLOR_WINDOW + 1));
EndPaint(hwnd, &ps);
}
return 0;
}
return DefWindowProc(hwnd, uMsg, wParam, lParam);
}
int WINAPI WinMain(HINSTANCE hInstance, HINSTANCE, LPSTR, int nShowCmd) {
const char CLASS_NAME[] = "Sample Window Class";
WNDCLASS wc = {};
wc.lpfnWndProc = WindowProc;
wc.hInstance = hInstance;
wc.lpszClassName = CLASS_NAME;
RegisterClass(&wc);
HWND hwnd = CreateWindowEx(0, CLASS_NAME, "Hello, Windows!", WS_OVERLAPPEDWINDOW,
CW_USEDEFAULT, CW_USEDEFAULT, CW_USEDEFAULT, CW_USEDEFAULT,
NULL, NULL, hInstance, NULL);
ShowWindow(hwnd, nShowCmd);
UpdateWindow(hwnd);
MSG msg = {};
while (GetMessage(&msg, NULL, 0, 0)) {
TranslateMessage(&msg);
DispatchMessage(&msg);
}
return 0;
}
Explanation: This code sample demonstrates the essential components of a Windows application. The `WinMain` function acts as the entry point, where you define and register a window class, create the window, and enter the message loop. The `WindowProc` function handles messages, including painting and window destruction.
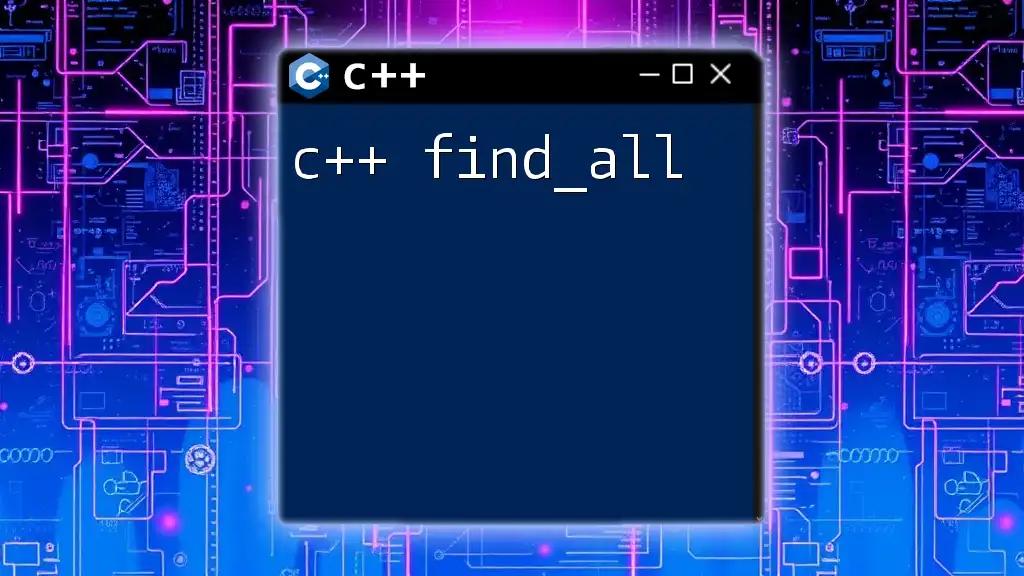
Key Components of a Windows Application
Windows Messages
In Windows programming, messages are the primary means of communication between the operating system and the application. Understanding how to handle these messages is vital for creating responsive applications. Common messages include:
- WM_PAINT: Indicates that the window needs to be redrawn.
- WM_DESTROY: Signals that the window is being closed.
By utilizing these messages effectively, you can ensure your application can respond to user actions and maintain a smooth experience.
GDI: Graphics Device Interface
The Graphics Device Interface (GDI) is crucial for rendering graphics in Windows applications. It provides functions for drawing shapes, text, and images.
As a simple example, you can use GDI functions in the `WM_PAINT` section of your `WindowProc` to draw a filled rectangle or text response to user actions.
Managing Resources
Any professional Windows application will require various resources such as icons, bitmaps, and fonts. These resources can be included in your project using resource scripts (`.rc` files). Loading and managing these resources properly ensures your application is visually appealing and functionally rich.
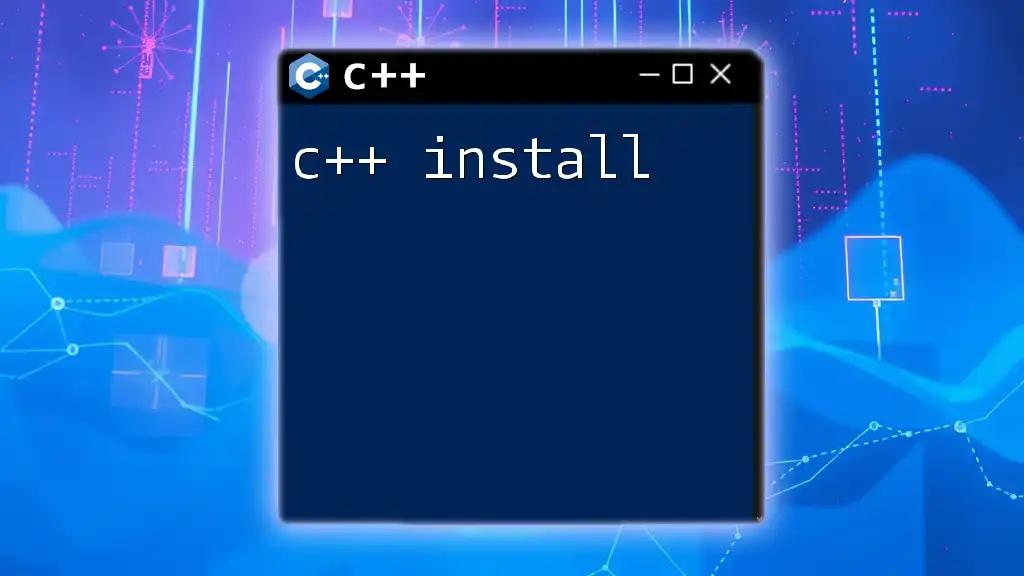
Building User Interfaces with C++
Introduction to Dialogs and Controls
Creating user-friendly interfaces is essential. Dialogs and controls (like buttons, text boxes, and list views) provide a coherent way for users to interact with your application. Windows allows for these elements to be managed through specific classes and messages, adding a layer of interactivity.
Example: Creating a Simple Dialog Box
To create a dialog box in C++, you will define a dialog resource in a `.rc` file and implement a dialog procedure that responds to messages triggered by user input.
Code Snippet:
// Example code to define and display a dialog box
Setting up a simple dialog involves registering the dialog, using the `DialogBox` function, and handling the messages received via the dialog procedure.
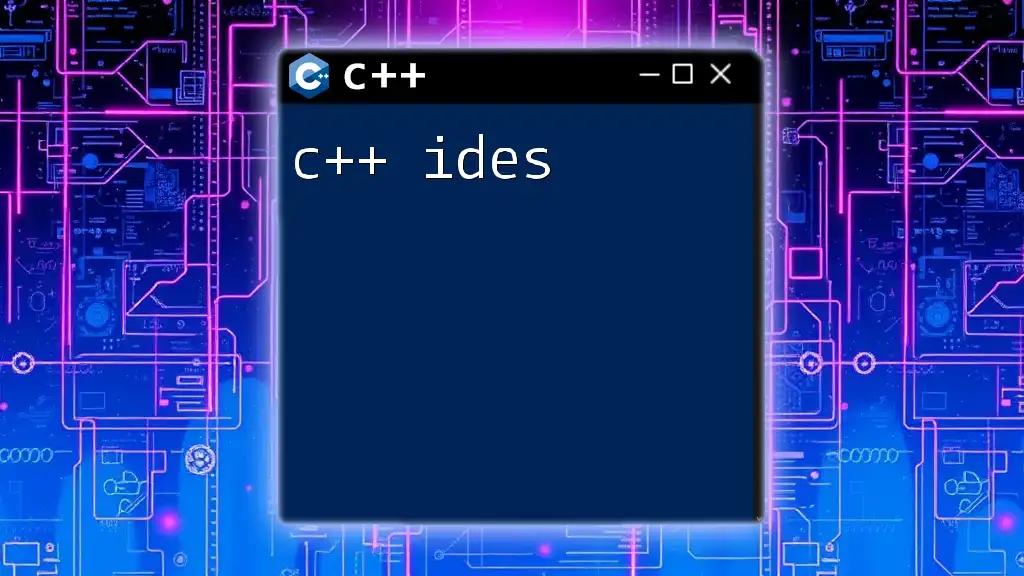
Advanced Windows Programming Concepts
Multithreading in C++
For applications requiring high performance and responsiveness, leveraging multithreading can be immensely beneficial. In C++, you can create threads using the `<thread>` header, allowing you to perform tasks such as data processing or network calls without freezing the user interface.
Using COM in C++
The Component Object Model (COM) enables the interaction of software components across various programming languages. In C++, you can utilize COM to create reusable components in a Windows environment, facilitating features like automation, user interfaces, and access to system services.
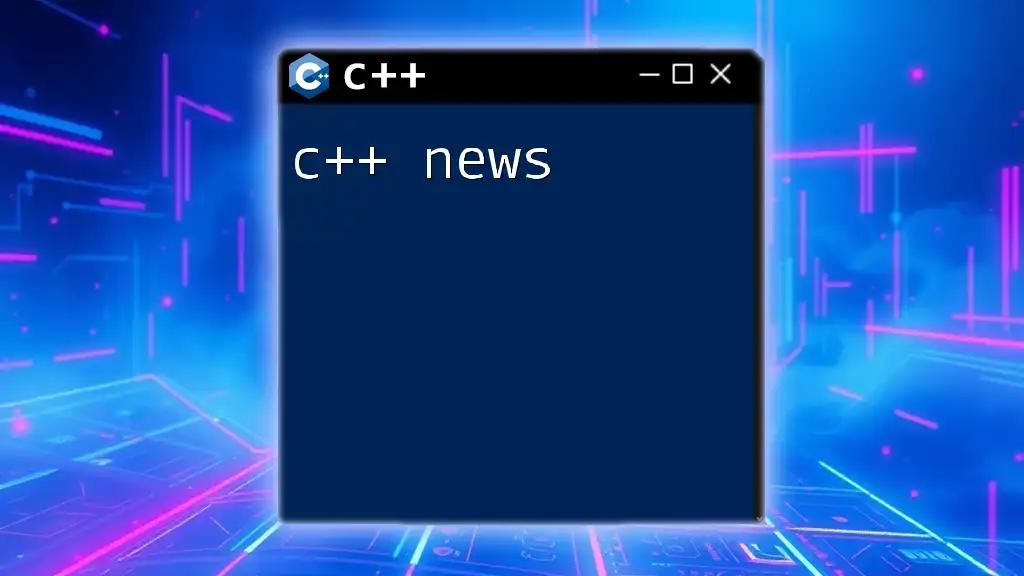
Debugging and Error Handling
Common Debugging Techniques
Debugging is a critical aspect of C++ Windows programming. Visual Studio provides robust debugging tools, allowing you to set breakpoints, inspect variables, and step through code execution. Familiarizing yourself with these tools can significantly improve your development efficiency.
Handling Exceptions in C++
Proper error handling is paramount for creating robust applications. In C++, utilize `try`, `catch`, and `throw` blocks to manage exceptions gracefully, ensuring that your application can handle errors without crashing.
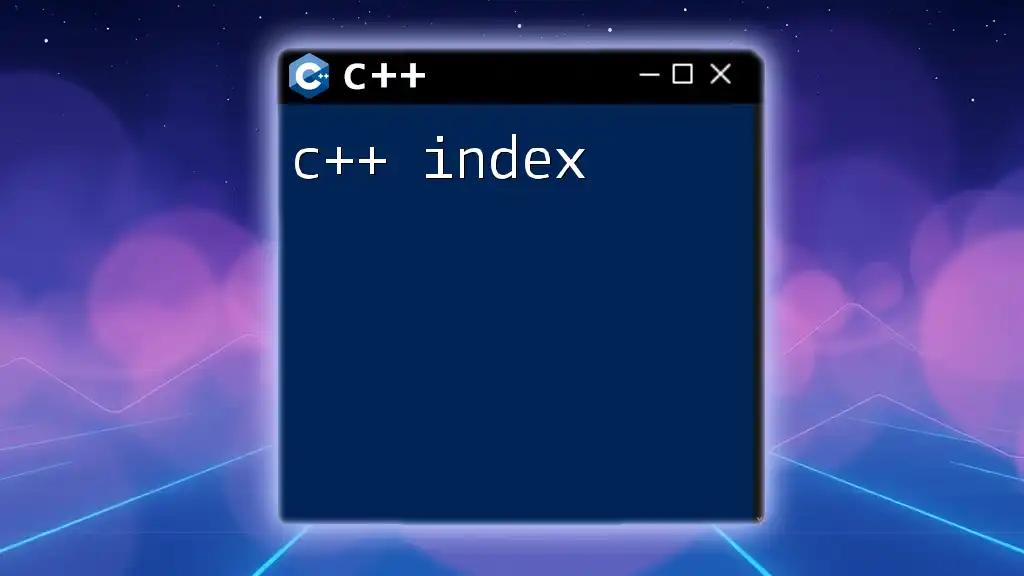
Resources for Further Learning
Recommended Books and Tutorials
To deepen your knowledge of C++ Windows programming, consider exploring these resources:
- “Programming Windows” by Charles Petzold
- Online courses from platforms such as Coursera or Udemy
- Microsoft’s official documentation on Windows API and C++
Online Communities and Support
Becoming part of developer forums like Stack Overflow, CodeProject, or the Microsoft Developer Network can provide ongoing support, guidance, and collaborative opportunities in your C++ journey.
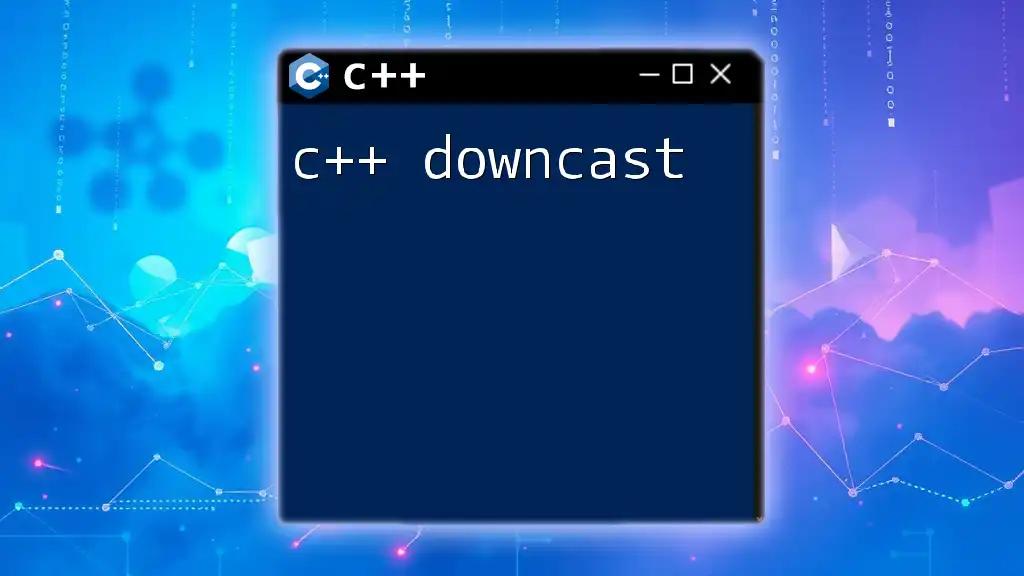
Conclusion
Throughout this guide, we've covered essential concepts of C++ Windows programming, from setting up your environment to advanced topics such as multithreading and COM. With a robust understanding of these principles, you are well-equipped to create powerful, responsive applications tailored for the Windows platform.

Call to Action
Now is the perfect time to delve deeper into C++ Windows programming. Consider exploring more advanced topics, or join hands-on workshops to refine your skills and enhance your understanding of C++ commands in the context of building Windows applications.