C++ on iOS allows developers to leverage the power and performance of C++ in creating high-performance applications for Apple's mobile platform, often using the Objective-C or Swift bridge for integration.
Here’s a simple code snippet demonstrating how to incorporate a C++ function in an iOS app:
#include <iostream>
extern "C" {
void helloWorld() {
std::cout << "Hello, iOS from C++!" << std::endl;
}
}
To call this `helloWorld` function from your Objective-C or Swift code, you would need to set up your bridging appropriately.
Understanding C++ in the Context of iOS
What is C++?
C++ is a powerful programming language that extends the capabilities of the C programming language with features such as object-oriented programming, generic programming, and powerful data abstraction. These capabilities make C++ a preferred choice for system-level programming, performance-critical applications, and game development. The language combines high-level and low-level programming capabilities, allowing developers to manage memory more efficiently and create robust applications.
The Importance of C++ for iOS
When it comes to iOS development, C++ offers distinct advantages. It provides a performance boost through faster execution times and efficient memory management. Additionally, its ability to interact seamlessly with Objective-C and Swift allows developers to leverage existing C++ codebases or libraries, enhancing functionality without rewriting code. This hybrid approach can significantly expedite development due to code reuse.
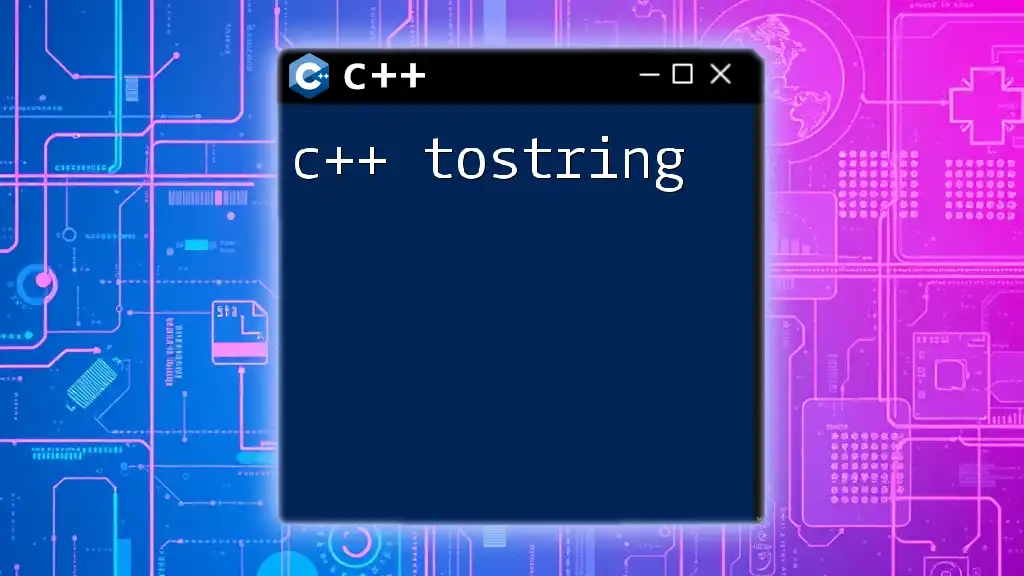
Getting Started with C++ on iOS
Setting Up Your Development Environment
To start working with C++ in iOS, download and install Xcode, Apple's integrated development environment (IDE). Once installed, set up your environment:
- Open Xcode and select "Create a new Xcode project."
- In the template dialog, choose macOS and select Command Line Tool. This allows you to work with C++ in a console application before integrating it into an iOS app.
- Set the project name and ensure the language is set to C++.
Building Your First C++ Application for iOS
Now that your environment is ready, let's create a simple "Hello World" application:
- Open your newly created project.
- Replace the contents of `main.cpp` with the following code:
#include <iostream>
int main() {
std::cout << "Hello, World from C++ on iOS!" << std::endl;
return 0;
}
- Build and run the project using Cmd + R. You should see the output in the Xcode console.
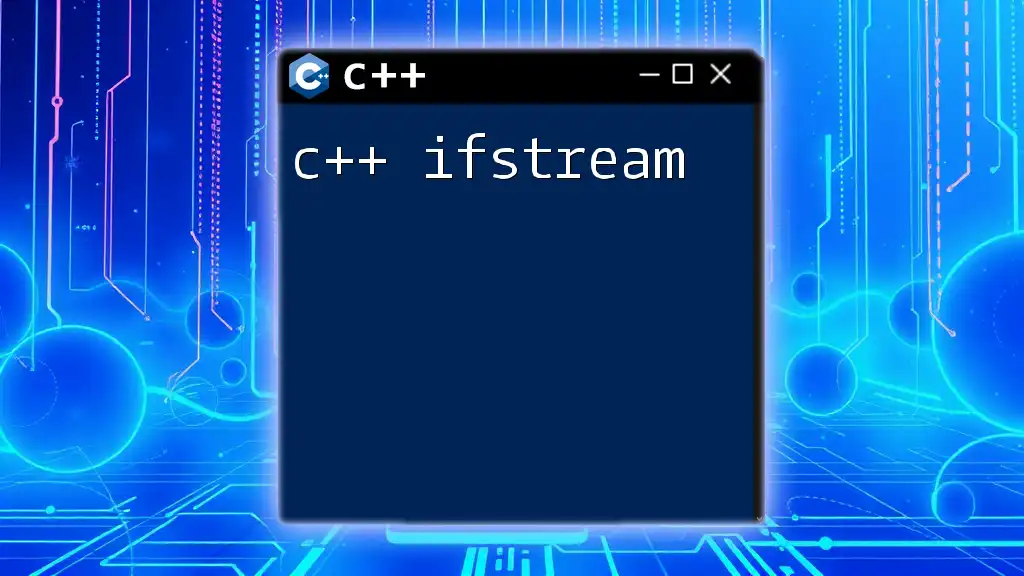
C++ Basics for iOS Development
Key Features of C++
Understanding the fundamental features of C++ is crucial for successful iOS development. Here are some core concepts:
- Data Types: C++ supports various data types such as `int`, `float`, `char`, and user-defined types like `structs` and `classes`.
int age = 30;
float salary = 50000.50;
char grade = 'A';
- Control Structures: C++ includes powerful control structures like `if`, `for`, and `while`, enabling developers to implement logic in their applications.
for(int i = 0; i < 5; i++) {
std::cout << "Iteration " << i << std::endl;
}
- Functions: Functions allow you to encapsulate code for reusability and organization.
int add(int a, int b) {
return a + b;
}
Object-Oriented Programming in C++
C++ is known for its object-oriented programming (OOP) features. Here’s how it applies to iOS:
- Classes and Objects: Define a class to create custom data types and functionality.
class Car {
public:
std::string brand;
void honk() {
std::cout << "Beep! Beep!" << std::endl;
}
};
Car myCar;
myCar.brand = "Toyota";
myCar.honk();
Using OOP enhances code organization and maintenance, making it easier to manage complex applications.
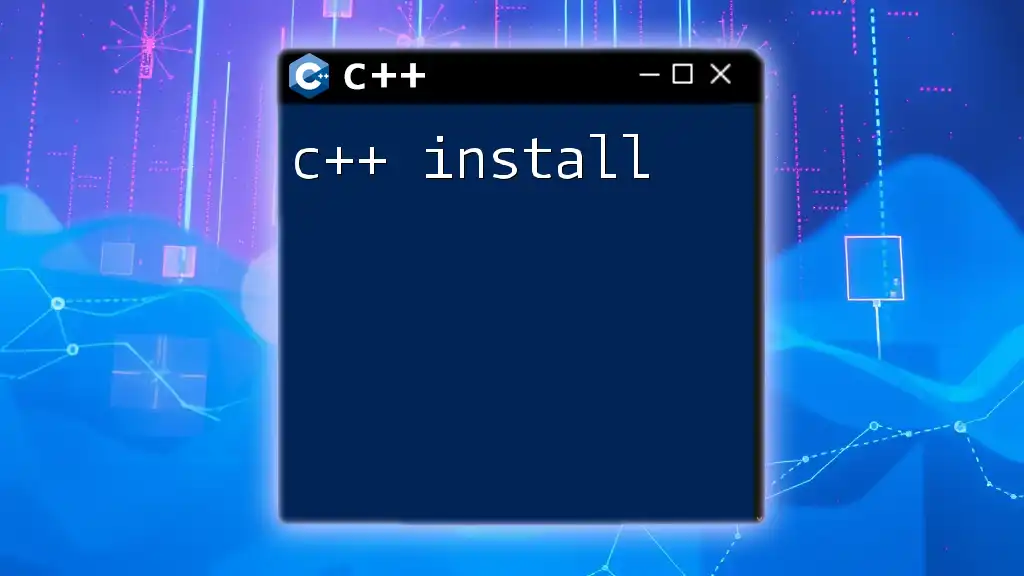
Integration of C++ with iOS
Bridging C++ and Objective-C
To utilize C++ within an iOS app, you can create Objective-C++ files (with the .mm extension). This allows you to blend C++ code with Objective-C seamlessly.
- Create a new file and choose Objective-C++. Here’s an example of how to interact between Objective-C and C++:
// MyClass.h (Objective-C)
#import <Foundation/Foundation.h>
@interface MyClass : NSObject
- (void)callCPPFunction;
@end
// MyClass.mm
#include "MyClass.h"
#include <iostream>
void cppFunction() {
std::cout << "Called from C++!" << std::endl;
}
@implementation MyClass
- (void)callCPPFunction {
cppFunction();
}
@end
This example shows how to define a method in Objective-C that calls a C++ function.
Using C++ Libraries in iOS Apps
C++ libraries can greatly expand the functionality of your iOS app. To incorporate libraries:
- Add the Library: Drag the C++ library files into your Xcode project.
- Configure Build Settings: Ensure that your Xcode project settings are configured to include C++ standard libraries.
Here's a simple example of using a third-party C++ library:
#include "SomeCPPLibrary.h"
int main() {
SomeCPPClass obj;
obj.performAction();
return 0;
}
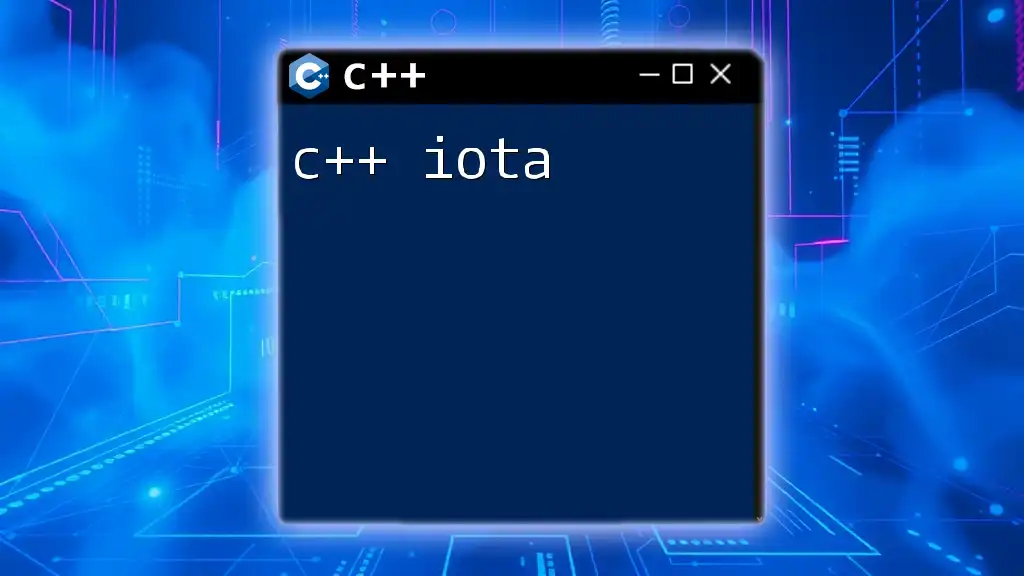
Advanced C++ Concepts Relevant to iOS
Templates in C++
Templates provide a powerful way to create generic and reusable code. This is particularly useful in applications requiring data structures such as linked lists or vectors.
template <typename T>
class Box {
private:
T value;
public:
Box(T val) : value(val) {}
T getValue() { return value; }
};
In this example, a template class `Box` can hold any data type, enhancing flexibility in your applications.
Exception Handling in C++
Effective error handling ensures a robust application. C++ supports exception handling, allowing you to catch and manage errors gracefully.
try {
throw std::runtime_error("An error occurred");
} catch (const std::runtime_error& e) {
std::cerr << "Exception: " << e.what() << std::endl;
}
Use exceptions to keep your application stable and avoid crashes.
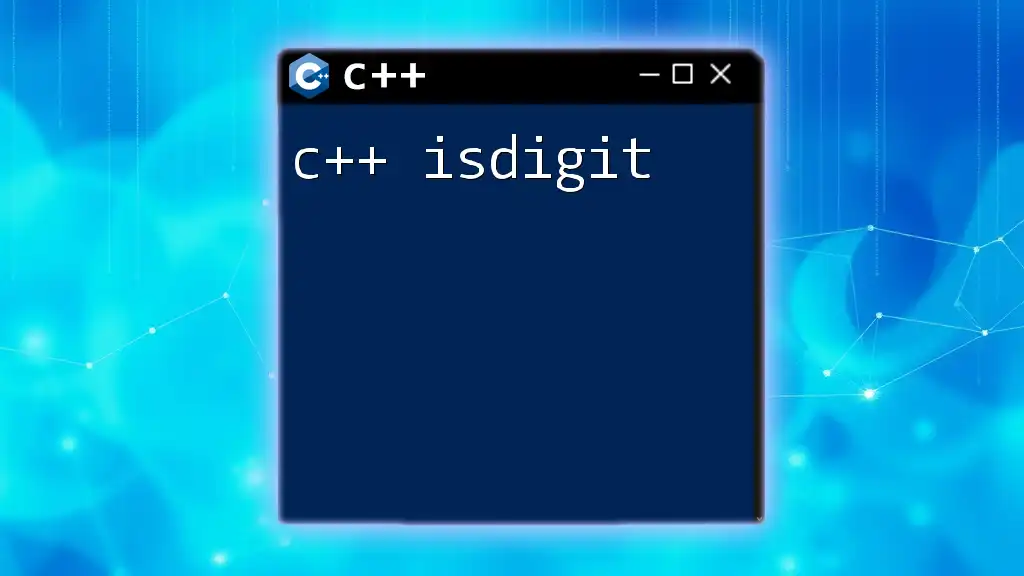
Performance Optimization with C++
Memory Management
In C++, manual memory management is a key consideration. Using smart pointers (e.g., `std::unique_ptr`) can help prevent memory leaks.
#include <memory>
void smartPointerExample() {
std::unique_ptr<int> ptr = std::make_unique<int>(10);
std::cout << *ptr << std::endl;
} // Automatically freed when out of scope
Using smart pointers also simplifies ownership semantics in your code.
Multithreading in C++
C++ offers robust support for multithreading, crucial for performance-sensitive applications. You can create and manage multiple threads for concurrent tasks.
#include <thread>
void threadFunction() {
std::cout << "Thread is running!" << std::endl;
}
int main() {
std::thread t(threadFunction);
t.join(); // Wait for the thread to finish
return 0;
}
Utilizing multithreading effectively can significantly improve application responsiveness.
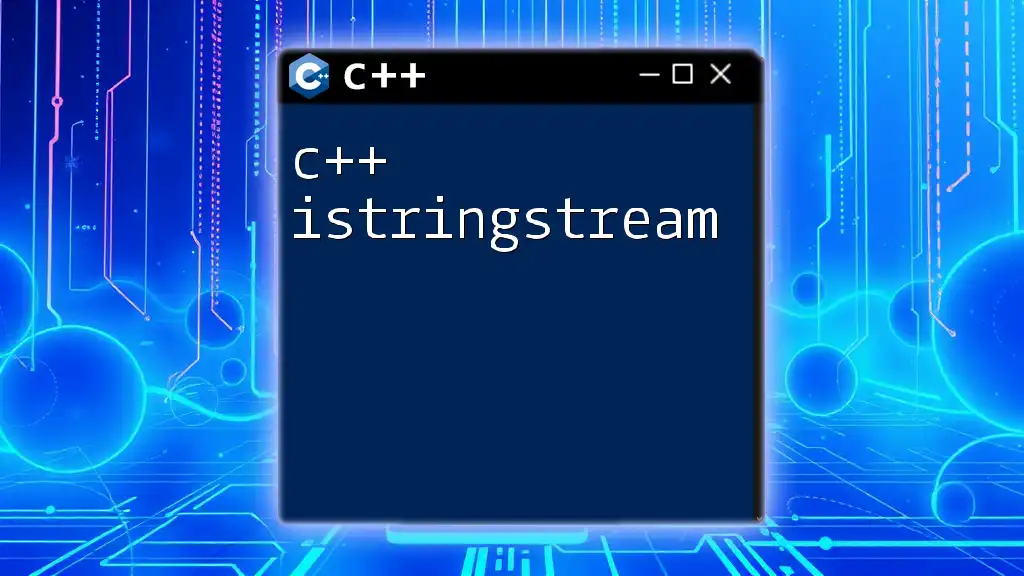
Best Practices for Using C++ in iOS Development
Code Organization
Organizing your code is essential for maintainability. Use a consistent file structure and naming conventions. Group related classes in folders and separate interface and implementation files.
Debugging C++ in Xcode
Debugging C++ in Xcode involves utilizing its built-in debugging tools:
- Set breakpoints by clicking the gutter next to the line numbers.
- Use the Debug navigator to inspect variable values.
- Step through your code with Step Over and Step Into functionalities.
These tools will help you diagnose issues quickly and effectively.
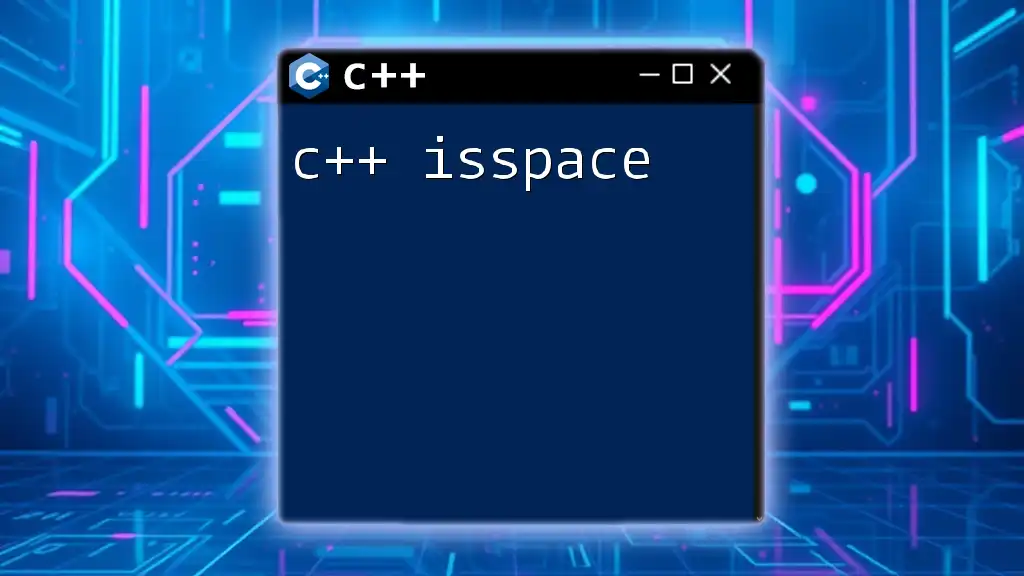
Conclusion
C++ provides a powerful toolkit for iOS development. By understanding its core concepts, integrating it with existing Objective-C and Swift code, and employing advanced techniques, you can unlock the full potential of your applications. Embrace the advantages of C++ in your iOS projects, and take your coding skills to new heights.