In C++, an instance refers to a specific object created from a class, which allows you to access the properties and methods defined within that class.
Here’s a simple code snippet illustrating how to create an instance of a class:
class Dog {
public:
void bark() {
std::cout << "Woof!" << std::endl;
}
};
int main() {
Dog myDog; // Creating an instance of Dog
myDog.bark(); // Calling a method on the instance
return 0;
}
What is an Instance in C++?
An instance is a specific realization of any object or class. It represents a concrete occurrence of a class definition, effectively treating the class as a blueprint from which objects or instances can be created. In the realm of object-oriented programming (OOP), understanding the concept of instances is crucial because it forms the backbone of how we manage and organize our code.
In a way, you may think of a class as a cookie cutter and instances as the cookies made from that cutter. Both share the same attributes and behaviors, yet each cookie is an individual entity.
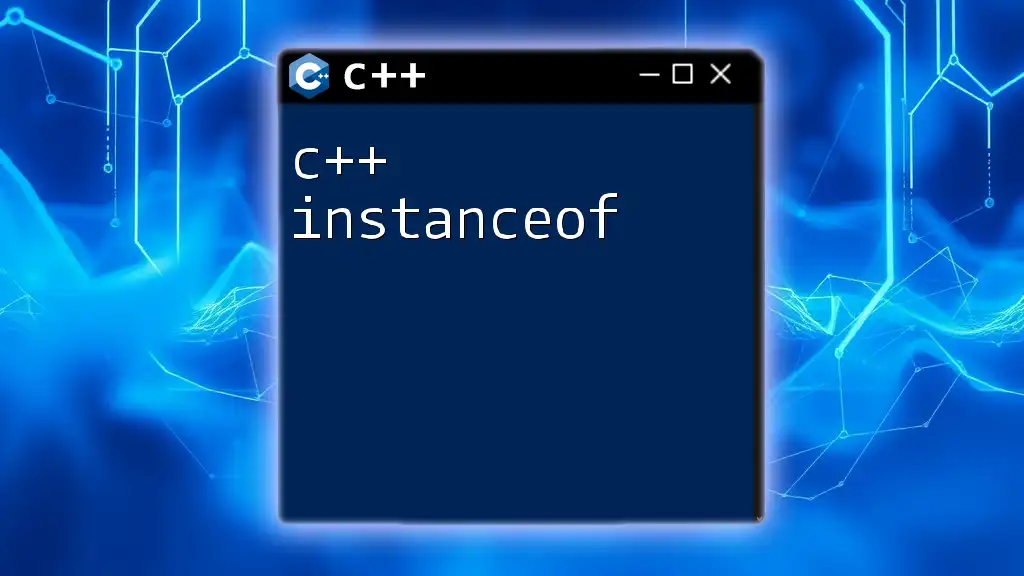
Creating Instances in C++
To create an instance in C++, you must first define a class that outlines the properties and behaviors required. Let's start with a simple class definition.
Defining Classes
A class definition is a template for what the created objects will look like. Below is an example of creating a class named Car.
class Car {
public:
std::string brand;
std::string model;
int year;
void displayInfo() {
std::cout << year << " " << brand << " " << model << std::endl;
}
};
This `Car` class has three public attributes (`brand`, `model`, and `year`) and one public method (`displayInfo`) that prints the car's details.
Instantiating an Object
Once a class is defined, you can create an instance of that class using the following syntax:
Car myCar; // Creating an instance of Car
myCar.brand = "Toyota";
myCar.model = "Corolla";
myCar.year = 2020;
myCar.displayInfo(); // Output: 2020 Toyota Corolla
In this example, `myCar` is an instance of the `Car` class. Here, we assign values to its properties and call the `displayInfo` method to see the output.
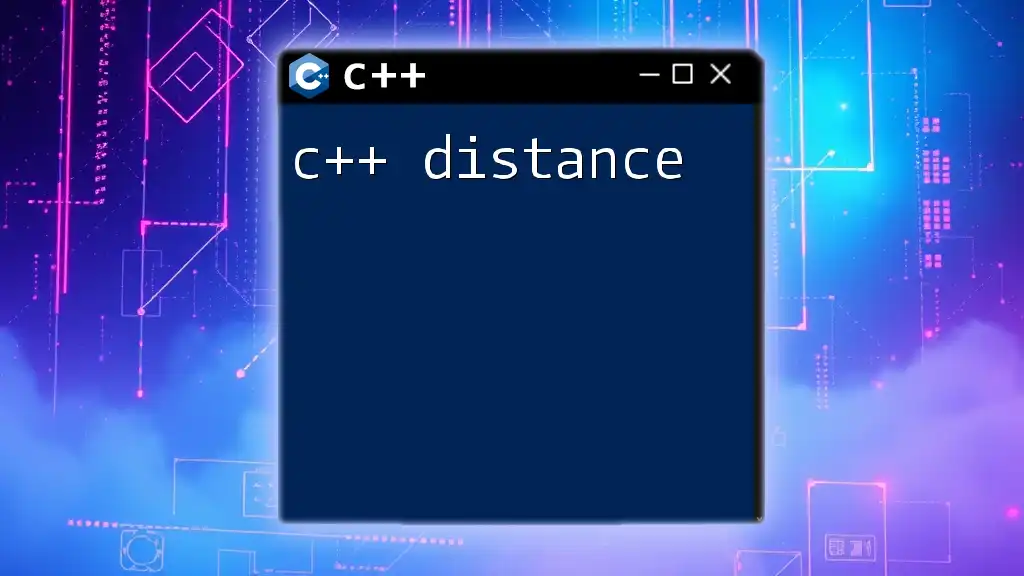
Types of Instances in C++
C++ allows for the creation of instances in different contexts: stack allocation and heap allocation. Understanding these contexts can optimize memory usage in larger programs.
Stack vs. Heap Allocation
- Stack Allocation: Instances created in the stack are automatically managed by the system. When the scope that created the instance ends, the memory is released. This allocation is generally faster due to less overhead. An example is shown below:
Car myCar; // Stack allocation
- Heap Allocation: Instances created in the heap require manual management. Use `new` to create an instance and `delete` to free the memory once it’s no longer needed. Here's how it's done:
Car* myCarHeap = new Car(); // Heap allocation
myCarHeap->brand = "Honda";
myCarHeap->model = "Civic";
myCarHeap->year = 2021;
myCarHeap->displayInfo(); // Output: 2021 Honda Civic
delete myCarHeap; // Free memory
In this example, `myCarHeap` is pointed to an instance of `Car` created on the heap. Remember to free any dynamically allocated memory to avoid memory leaks.
Static vs. Dynamic Instances
Instances can also be classified based on their traits, notably whether they are static or dynamic.
- Static Instances: These are instances whose data or methods stay associated with the class itself rather than individual instances. Static members are shared across all instances of a class. Here’s an example:
class StaticExample {
public:
static int instanceCount; // Static member
StaticExample() { instanceCount++; }
};
int StaticExample::instanceCount = 0; // Initialization
- Dynamic Instances: These are created during runtime and can change based on program conditions. They offer flexibility but require careful management.
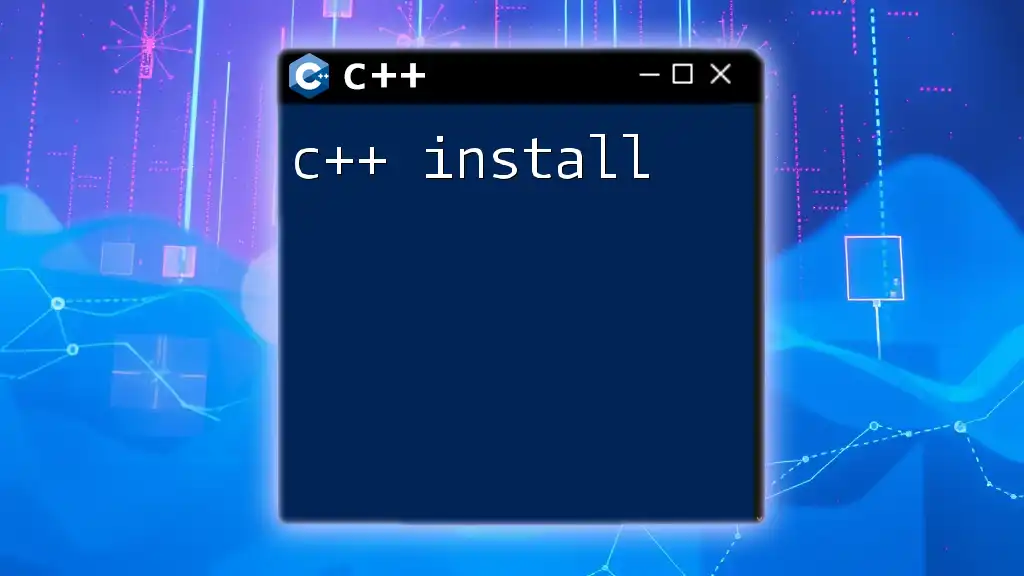
Accessing Instance Members
Instances can have various access levels dictated by access specifiers such as public, private, and protected. Understanding these will help maintain proper encapsulation.
Public, Private, and Protected Members
- Public Members: Accessible from anywhere in the program.
- Private Members: Restricted to the class itself, promoting encapsulation.
- Protected Members: Accessible by the class itself and its derived classes.
Here’s a code example using different access specifiers:
class Person {
private:
std::string ssn; // private member
public:
std::string name; // public member
void setSSN(std::string s) { ssn = s; }
std::string getSSN() { return ssn; }
};
In this `Person` class, `ssn` is a private member, while `name` is public. We use setter and getter functions to access `ssn`, ensuring encapsulation.
Using Accessors and Mutators
Accessors (getters) and mutators (setters) are essential for interacting with private members. They not only provide a way to manipulate these members but also allow for validation and other checks when setting values.
Person person;
person.name = "Alice"; // Accessing public member
person.setSSN("123-45-6789"); // Using mutator
std::cout << "Name: " << person.name << ", SSN: " << person.getSSN() << std::endl;
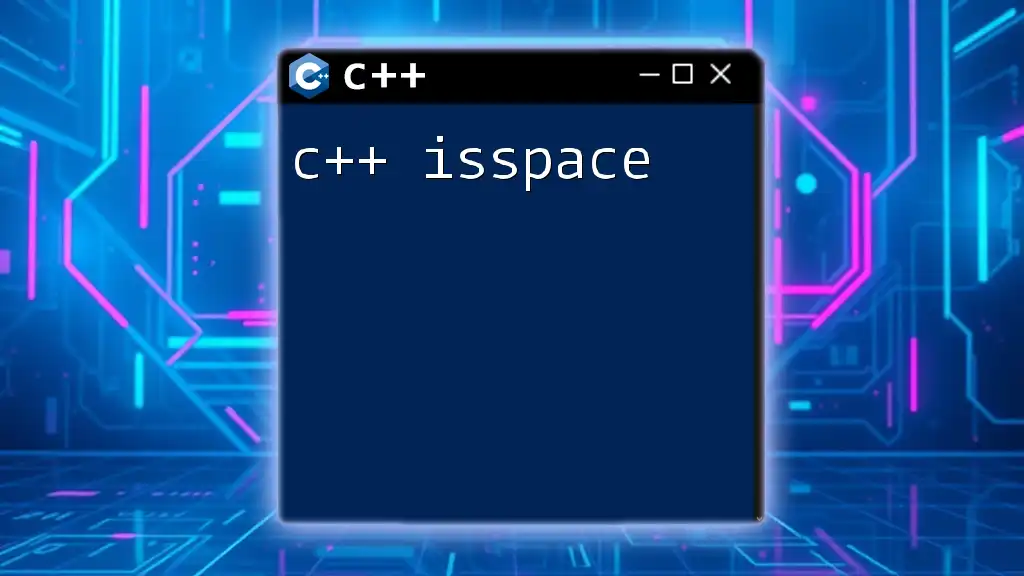
Instance Methods
Instance methods are functions defined within a class that operate on its instances. They enable instances to exhibit behavior, interacting with their attributes effectively.
Definition of Instance Methods
Instance methods have access to the instance variables and can modify their state. Here is an example of a class with instance methods:
class Rectangle {
public:
int width, height;
int area() {
return width * height;
}
};
Calling Instance Methods
Instantiating objects allows you to call its methods. You can easily calculate the area of the rectangle instance like this:
Rectangle rect;
rect.width = 5;
rect.height = 10;
std::cout << "Area: " << rect.area() << std::endl; // Output: Area: 50
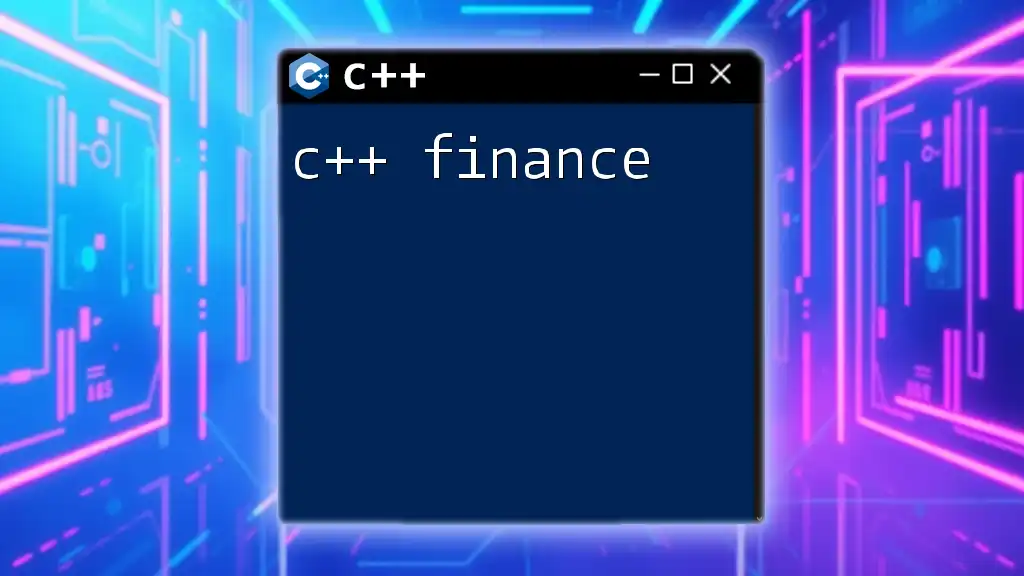
Best Practices for Using Instances
Naming Conventions
Consistency is key when naming instances. Good naming practices enhance code readability and maintainability. For example, instead of naming an instance `c`, consider naming it `myCar` for more context.
Memory Management
Careful memory management is crucial, especially for heap-allocated instances. Always remember to free up memory allocated using `new` with `delete` to avoid memory leaks:
Car* myCarHeap = new Car();
//... use myCarHeap
delete myCarHeap; // Always clean up
Encapsulation
Encapsulation is central to OOP principles. Keep members private and expose them through public methods to safeguard data integrity. Utilizing accessors and mutators as shown in the Person class is a good practice.
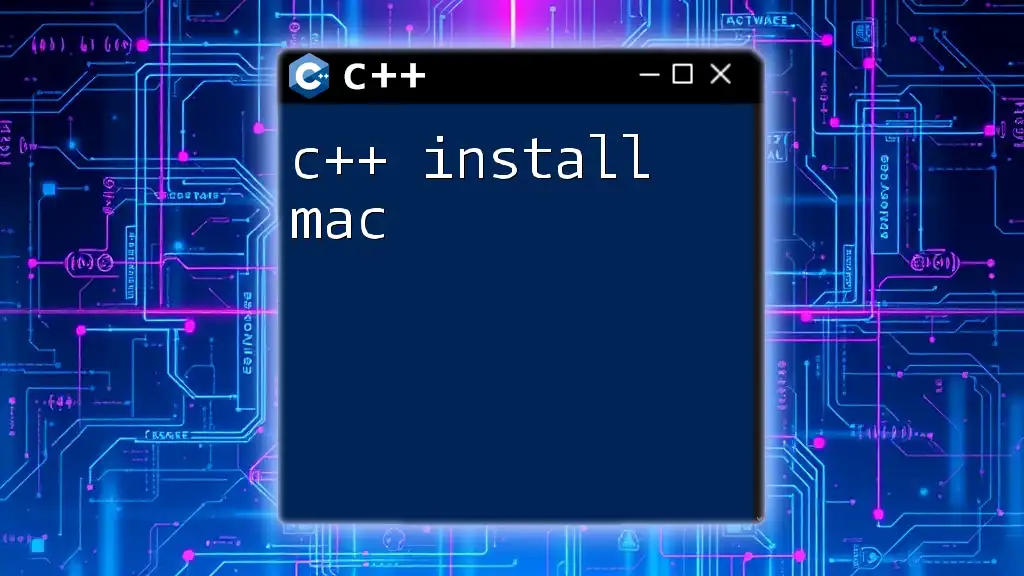
Common Pitfalls When Working with Instances
Misunderstanding Object Ownership
It's essential to grasp the ownership concepts related to instances. Mismanagement can lead to dangling pointers or unintentional memory leaks. Ensure that you know which part of your code is responsible for managing the memory of instances.
Memory Leaks
A memory leak occurs when allocated memory is not properly released. This can lead to reduced performance and crashes. Follow good practices by employing smart pointers (like `std::unique_ptr` or `std::shared_ptr`) when possible to automatically manage memory.
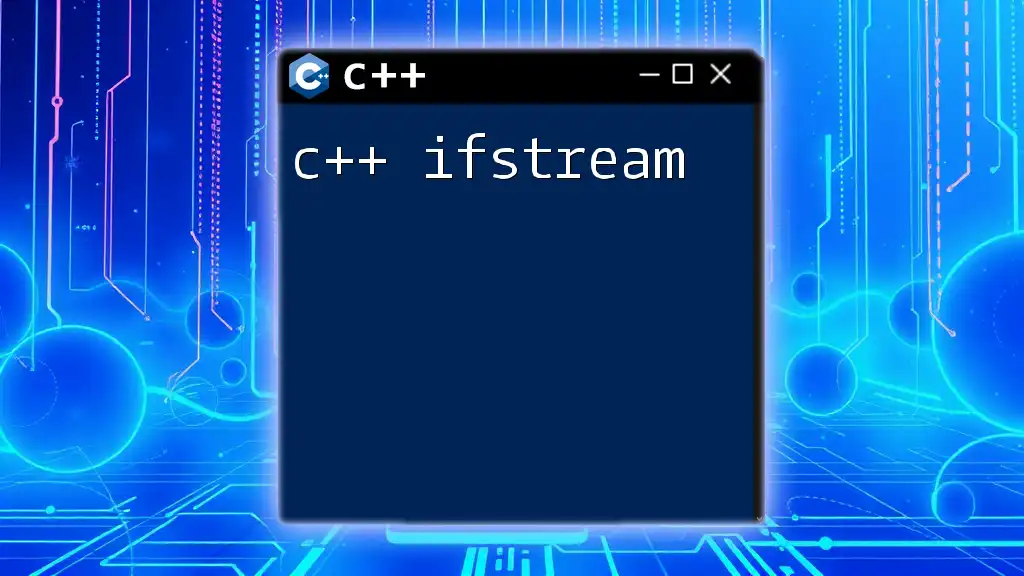
Conclusion
Understanding C++ instances is paramount in utilizing the full power of object-oriented programming. By grasping how to create, manage, and operate on instances, you create robust, maintainable, and efficient code. With diligent practice, the fundamentals of instances in C++ will become second nature, enhancing your programming effectiveness.
Utilize this knowledge to tackle real-world problems by creating well-defined classes and efficient instances that mimic complex systems elegantly. As you refine your skills, continue exploring advanced topics like polymorphism and inheritance to further enrich your coding repertoire.