The `ifstream` class in C++ is used to read data from files, allowing you to extract information easily.
Here’s a simple code snippet demonstrating its use:
#include <iostream>
#include <fstream>
#include <string>
int main() {
std::ifstream inputFile("example.txt");
std::string line;
if (inputFile.is_open()) {
while (getline(inputFile, line)) {
std::cout << line << std::endl;
}
inputFile.close();
} else {
std::cout << "Unable to open file" << std::endl;
}
return 0;
}
What is ifstream?
`ifstream` stands for "input file stream," and it is a part of the C++ standard library used for input operations from files. The primary purpose of `ifstream` is to allow programmers to read data from files, making it an essential feature for any application that requires data persistence or manipulation.
Using `ifstream` provides several benefits, such as the ability to read structured data, handle large datasets, or read data in various formats. Understanding how to use `ifstream` effectively can significantly enhance your programming capabilities in C++.
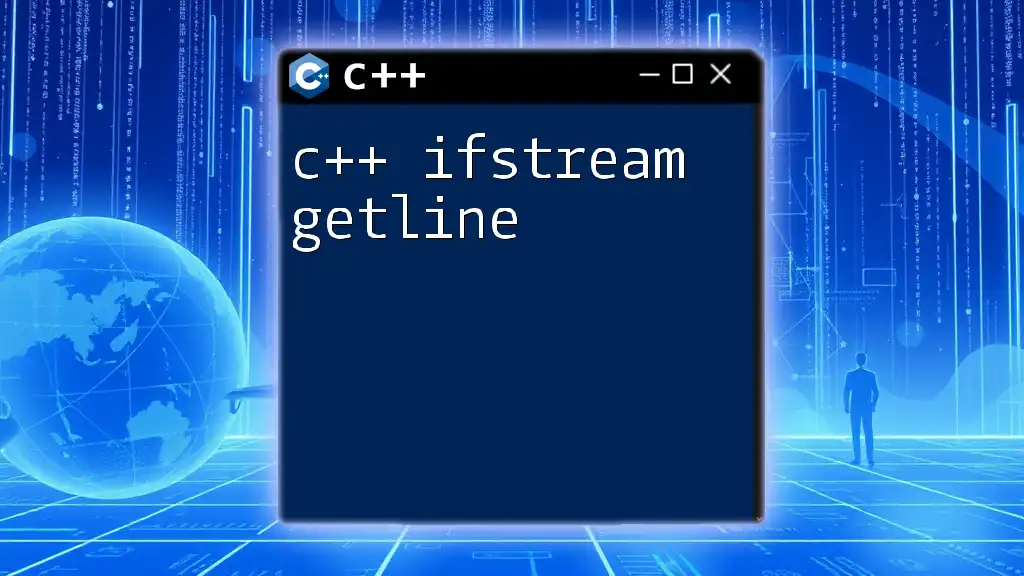
Basic Concepts of Input File Streams
In C++, a "stream" is essentially a flow of data between the program and an input/output device, like a file. Stream classes in C++ can be categorized into input streams and output streams. Specifically, `ifstream` is an input stream, which means that it is designed to read data from files or other input sources.
Difference Between Input and Output Streams
-
Input Streams: An input stream is used to fetch data into the program. `ifstream` is the specific class used for reading from files.
-
Output Streams: An output stream, such as `ofstream`, is utilized to send data out of the program, generally to a file or console.
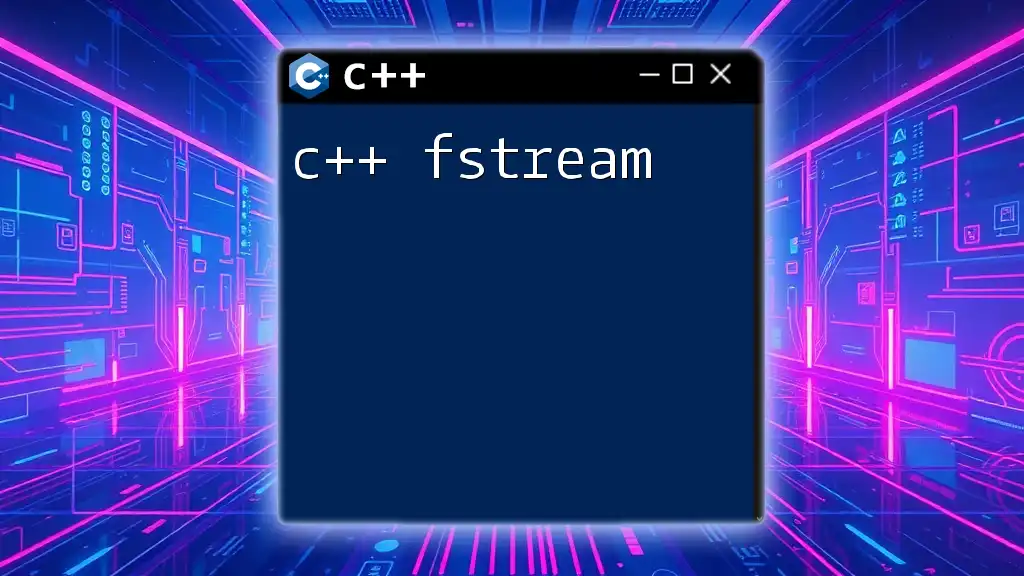
Setting Up ifstream
Including Headers
To work with `ifstream`, you must include the appropriate header files. The key header for file operations is `<fstream>`. Additionally, `<iostream>` may be included for console output, especially when you need to report errors or display output.
#include <iostream>
#include <fstream>
#include <string>
Creating an ifstream Object
Creating an `ifstream` object requires invoking its constructor, which can be done by directly passing the file name as a parameter. The syntax looks as follows:
std::ifstream inputFile("example.txt");
Opening a File
Opening Files with ifstream
After declaring the `ifstream` object, you may also want to explicitly open a file using the `open()` function. This function takes the filename as an argument, enabling you to open a file dynamically.
inputFile.open("example.txt");
Checking if the File is Open
It is crucial to check if the file opened successfully. Attempting to read from a file that is not open can lead to runtime errors. You can use the `is_open()` function for this purpose:
if (!inputFile.is_open()) {
std::cerr << "Error opening file!" << std::endl;
}
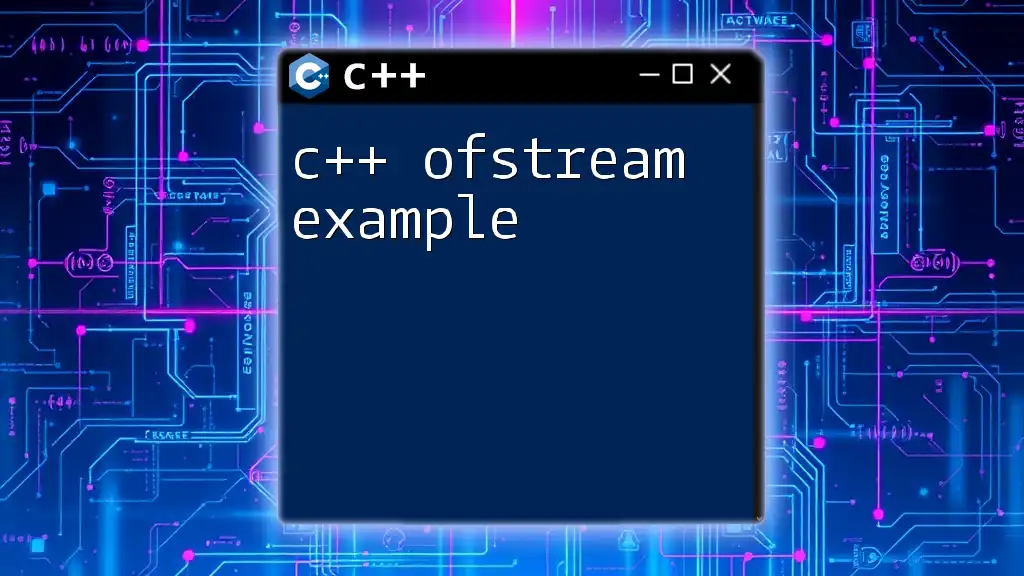
Reading Data from Files
Reading Strings
The `getline()` function is commonly used to read whole lines from a file until a newline character is encountered. This method helps capture entire data fields in a single read:
std::string line;
while (getline(inputFile, line)) {
std::cout << line << std::endl;
}
Reading Numbers
C++ provides overloaded operators that make reading common data types, such as integers and floats, straightforward. You can read numbers directly from the `ifstream` object:
int number;
inputFile >> number;
Reading Mixed Data Types
When reading data from a file that contains mixed types (e.g., a name followed by an age), you can read them sequentially using the input stream operators:
std::string name;
int age;
inputFile >> name >> age;
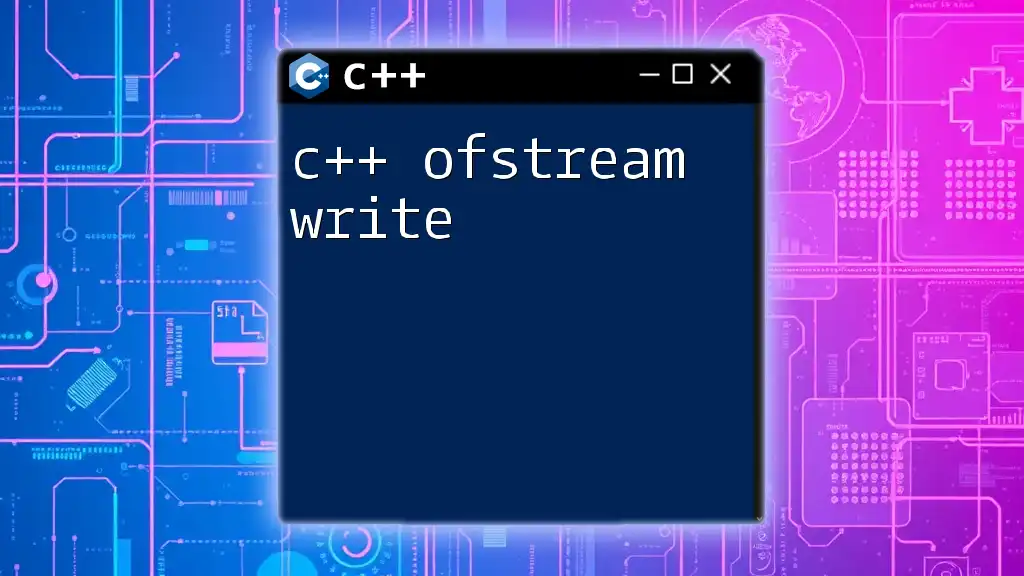
Practical Example of ifstream
Sample Code Snippet
Here’s a comprehensive example that incorporates reading from a file utilizing different data types. This code snippet demonstrates the flow of reading a file named `data.txt`.
#include <iostream>
#include <fstream>
#include <string>
int main() {
std::ifstream inputFile("data.txt");
std::string line;
// Check for file open
if (!inputFile.is_open()) {
std::cerr << "Error opening file!" << std::endl;
return 1;
}
while (getline(inputFile, line)) {
std::cout << line << std::endl;
}
inputFile.close(); // Closing the file
return 0;
}
Explanation of Sample Code
- The program begins by including the necessary headers for I/O operations.
- An `ifstream` object is created to attempt opening the file `data.txt`.
- An error check follows to confirm whether the file opened successfully. If not, an error message is printed to standard error output.
- As long as the file is open, the program uses `getline()` in a loop to read each line from the file until the end of the file is reached.
- Finally, the file is closed to free resources.
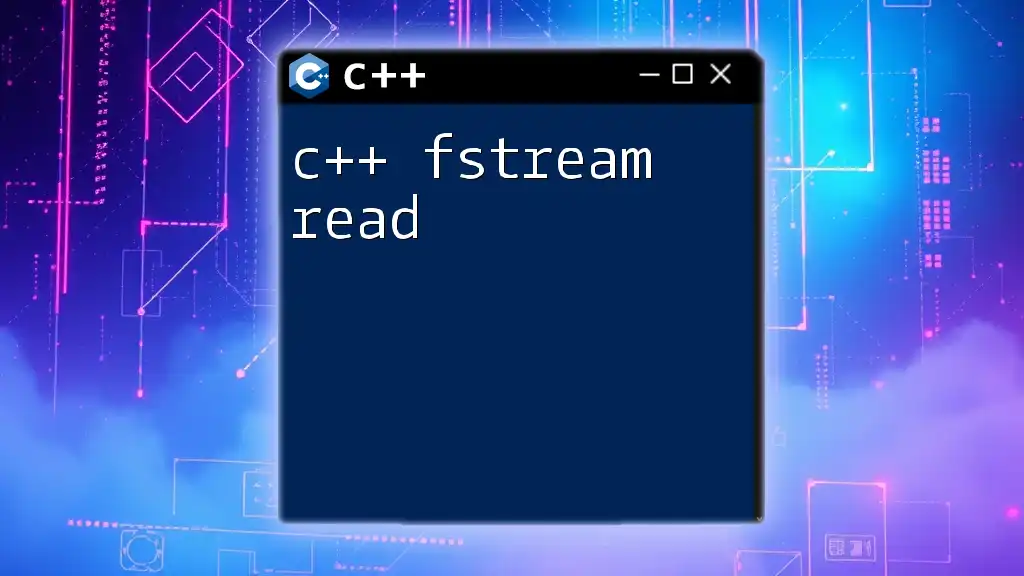
Error Handling with ifstream
Common Errors in File Handling
When working with files, encountering errors such as a missing file or insufficient permissions is common. Logging and handling these errors gracefully leads to more robust applications. Always check if the file has opened successfully before reading.
Exception Handling
Utilizing exception handling mechanisms avoids program crashes due to file-related errors. You can employ try-catch blocks to catch exceptions that might be thrown during file operations:
try {
std::ifstream inputFile("data.txt");
if (!inputFile.is_open()) {
throw std::ios_base::failure("Failed to open file");
}
// Further file reading operations
} catch (const std::ifstream::failure& e) {
std::cerr << "Exception opening file: " << e.what() << std::endl;
}
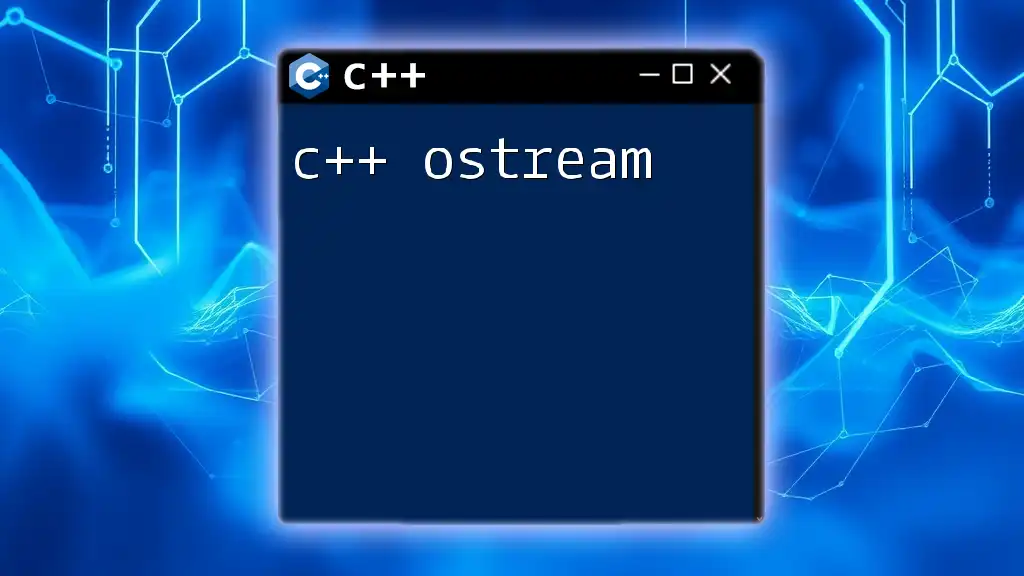
Best Practices for Using ifstream
Clean Code Recommendations
When using `ifstream`, it is essential to maintain clean and comprehensible code. This includes writing helpful comments explaining the purpose of blocks of code, adhering to naming conventions, and using whitespace appropriately for better readability.
Performance Considerations
When dealing with large files, consider optimizing your read operations. For example, reading data in chunks rather than one line at a time can minimize overhead and improve performance. Efficient use of buffers can also help when processing large datasets.
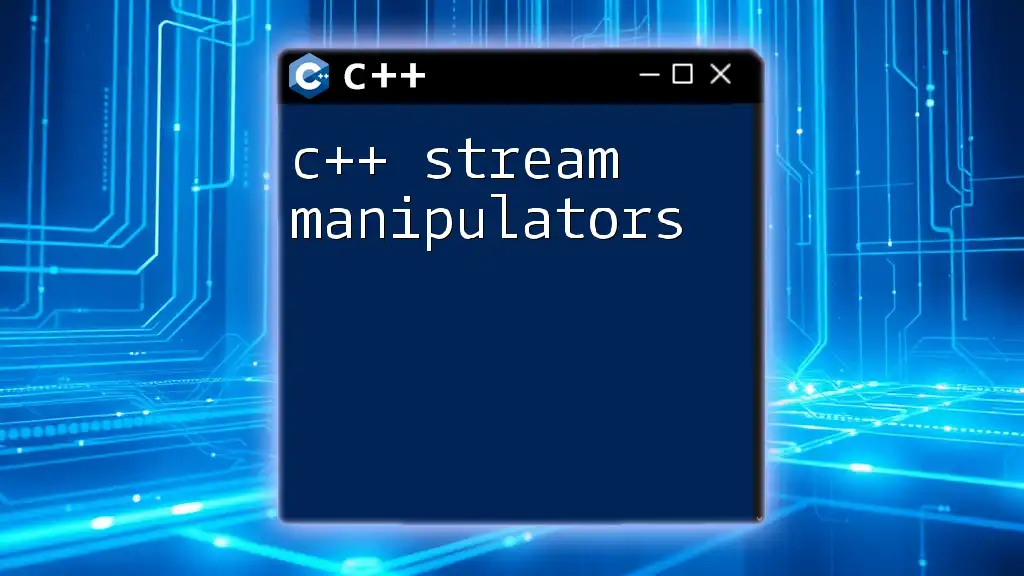
Conclusion
In summary, `ifstream` is a powerful class in C++ that enables effective file handling for reading data. Mastering its usage not only equips developers to create more functional applications but also fosters better error handling and performance optimizations.
For those looking to expand their programming skills further, exploring advanced file manipulation techniques, other file formats (e.g., binary files), or even different data processing libraries can be highly beneficial. Practicing with various data input scenarios will solidify your understanding of `ifstream` and file handling in C++.
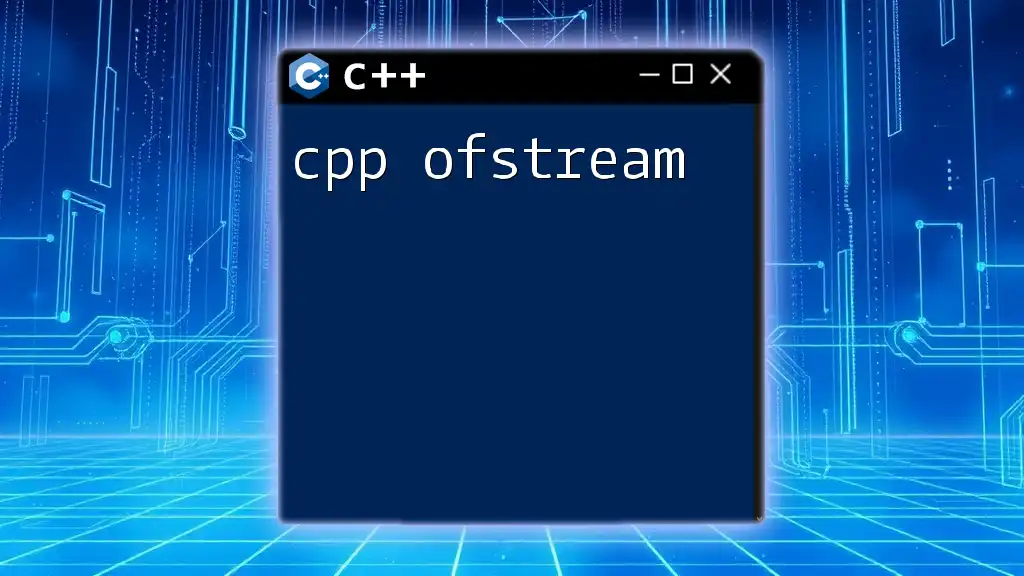
Additional Resources
Recommended Books and Online Materials
Consider exploring the following resources for further learning:
- Books on C++ programming focusing on data structures and file I/O operations.
- Online courses or tutorials specifically focusing on C++ file handling techniques.
Community Forums and Support
Engaging with online communities can provide additional support and insights. Consider joining forums or platforms like Stack Overflow or dedicated C++ communities where you can ask questions and share experiences.