The C++ `system` command allows you to execute system commands directly from your C++ program, facilitating interaction with the operating system.
Here's a simple code snippet demonstrating the usage of the `system` command:
#include <cstdlib> // Required for the system function
int main() {
system("echo Hello, World!"); // This will print "Hello, World!" in the terminal
return 0;
}
Understanding the C++ System
What is the C++ System?
The term C++ system encompasses the broader capabilities of C++ that enable developers to interact directly with system-level resources, such as the file system, system calls, and hardware. C++ is a general-purpose language known for its high performance and control over memory, making it suitable for low-level system programming. Understanding how C++ interacts with the system is crucial for tasks such as implementing drivers, building operating systems, or developing applications that require direct manipulation of hardware.
Why Use C++ for System Programming?
Using C++ for system programming provides notable advantages:
- Performance: C++ is compiled to machine code, which allows it to run with excellent performance compared to interpreted languages.
- Memory Management: With features like pointers, references, and dynamic memory allocation, C++ gives developers fine control over how memory is utilized.
- Direct Hardware Access: C++ can directly manipulate bytes and addresses, making it ideal for applications that require low-level hardware control.

Setting Up Your C++ Environment
Choosing the Right Compiler
To start developing with C++, selecting the right compiler is vital. Common compilers include:
- GCC (GNU Compiler Collection): Available on multiple platforms, it is open-source and widely used in the Linux environment.
- Clang: Another open-source compiler, known for its modular architecture and faster compilation times.
- MSVC (Microsoft Visual C++): A comprehensive IDE for Windows applications, providing extensive debugging tools.
Installing and configuring the chosen compiler depends on the platform you are using but usually involves downloading the installation package and following the setup instructions.
Setting Up an IDE
An Integrated Development Environment (IDE) simplifies the process of writing and managing C++ code. Some popular IDEs include:
- Visual Studio is highly recommended for Windows users, offering robust debugging and productivity features.
- Code::Blocks is lightweight and suitable for beginners.
- CLion is a powerful cross-platform IDE from JetBrains that supports CMake project management.
Configuration typically involves setting the path to your compiler and ensuring the IDE recognizes all associated libraries.
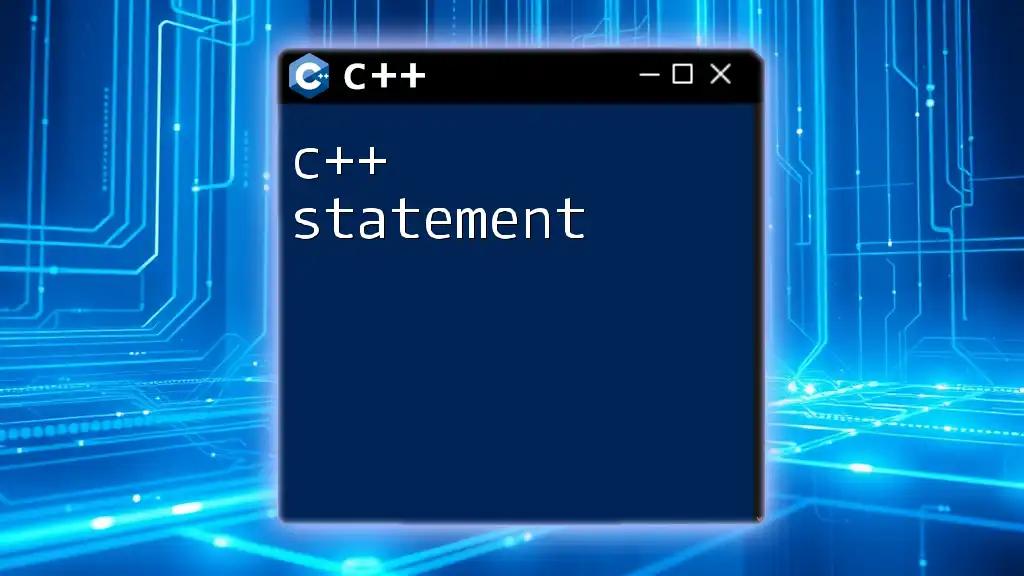
Key C++ System Commands
Opening and Closing the System Console
To interact with the command line or system console, you can use the `<cstdlib>` header and its `system()` function. This function executes a command specified in a string, allowing you to open the console in different modes.
For example, the following code opens the default command prompt:
#include <cstdlib>
int main() {
system("start"); // Opens the default command prompt
return 0;
}
File Management Commands
File handling is critical in system programming. The `<fstream>` library facilitates easy reading from and writing to files. Here’s a basic example of how to manage files:
#include <fstream>
#include <iostream>
int main() {
// Write to a file
std::ofstream outfile("example.txt");
outfile << "Hello, C++ System!";
outfile.close();
// Read from a file
std::ifstream infile("example.txt");
std::string line;
while (std::getline(infile, line)) {
std::cout << line << std::endl; // Output each line read from the file
}
infile.close();
return 0;
}
This example demonstrates the essential operations of opening, writing, and reading files, emphasizing how straightforward file management can be in C++.
Execution of System Commands
Beyond file management, C++ can also execute system commands and capture their output via the `popen()` function. This function creates a pipe to read or write to the process launched by the command.
Here’s how you can execute a shell command and retrieve the output:
#include <cstdio>
#include <iostream>
#include <array>
int main() {
std::array<char, 128> buffer;
std::string result;
// Use popen to execute a command
FILE* pipe = popen("ls", "r"); // "ls" lists files in a directory
if (!pipe) {
std::cerr << "popen() failed!" << std::endl;
return 1;
}
// Read the output of the command
while (fgets(buffer.data(), buffer.size(), pipe) != nullptr) {
result += buffer.data();
}
pclose(pipe);
std::cout << result; // Output the result of the command
return 0;
}
With this code, you can execute a system command (like listing the files in a directory) and collect its output, showcasing the versatility of C++ system commands.
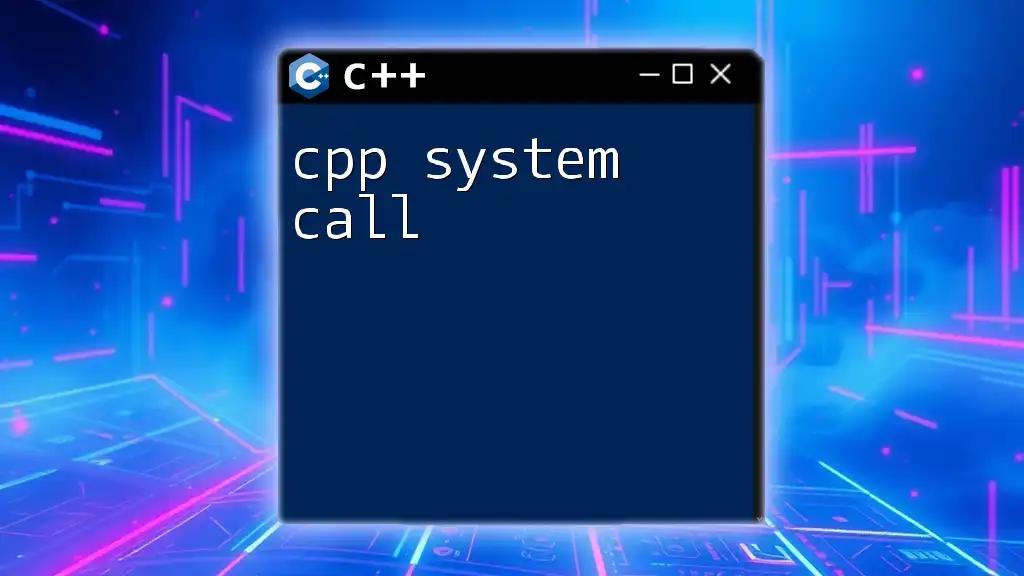
Advanced System Programming
Multi-threading in C++
Multithreaded programming enhances the performance of applications by allowing multiple threads to execute concurrently. The C++ Standard Library provides `std::thread` to facilitate thread management.
Here’s a simple example of using threads in C++:
#include <iostream>
#include <thread>
void threadFunction() {
std::cout << "Thread is executing!\n";
}
int main() {
std::thread t1(threadFunction); // Create a new thread
t1.join(); // Wait for thread to finish
return 0;
}
This code snippet demonstrates how to create and manage a thread. The `join()` method ensures the main program waits for the thread to complete its execution.
Network Programming with C++
Network programming allows for communication between processes across a network. With C++, you can implement basic socket programming to create servers and clients.
Here’s an example of a simple TCP echo server:
// A simplified echo server code
#include <iostream>
#include <cstring>
#include <sys/socket.h>
#include <netinet/in.h>
#include <unistd.h>
int main() {
int server_fd, new_socket;
struct sockaddr_in address;
int opt = 1;
int addrlen = sizeof(address);
// Create a socket
server_fd = socket(AF_INET, SOCK_STREAM, 0);
setsockopt(server_fd, SOL_SOCKET, SO_REUSEADDR, &opt, sizeof(opt));
address.sin_family = AF_INET;
address.sin_addr.s_addr = INADDR_ANY;
address.sin_port = htons(8080);
// Bind and listen
bind(server_fd, (struct sockaddr *)&address, sizeof(address));
listen(server_fd, 3);
new_socket = accept(server_fd, (struct sockaddr *)&address, (socklen_t*)&addrlen);
// Echo back the received message
char buffer[1024] = {0};
read(new_socket, buffer, 1024);
send(new_socket, buffer, strlen(buffer), 0); // Send back the same message
// Cleanup
close(new_socket);
close(server_fd);
return 0;
}
In this code, a server listens for incoming connections, reads messages from clients, and echoes them back. This demonstrates basic networking capabilities within C++.
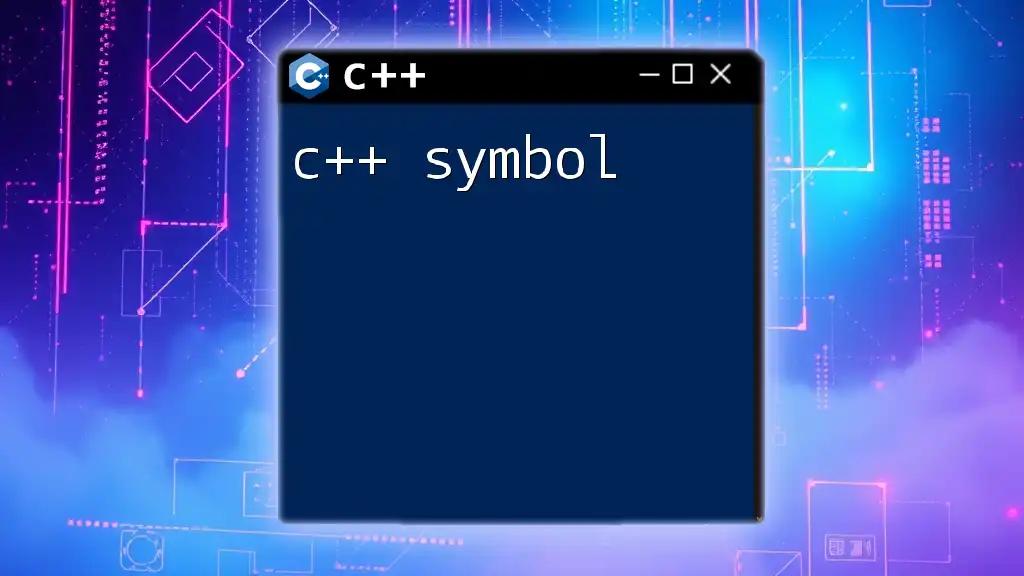
Debugging and Optimization
Common Debugging Techniques
Debugging is an essential part of software development. Leveraging debugging tools available in modern IDEs can help catch errors early. Common strategies include:
- Utilize breakpoints to pause execution and inspect variables.
- Step through code line by line to understand flow and identify bugs.
Optimizing Performance in System Code
Performance is crucial in system-level programming. Efficient memory management can drastically improve your program’s performance. Inspection and profiling tools like Valgrind and gprof can help identify memory leaks and performance bottlenecks.
To optimize your code:
- Use appropriate data structures to manage memory effectively.
- Minimize dynamic memory allocations where possible, favoring stack allocation for performance-critical sections.
In conclusion, mastering the C++ system commands and programming techniques can significantly empower your software development efforts. By combining efficient coding practices with advanced system interactions, you can build robust applications capable of leveraging the full power of the underlying hardware. Keep exploring, practicing, and learning to enhance your C++ system programming skills!