The C++ `system` command allows you to execute operating system commands directly from your C++ program, facilitating interaction with the command line.
#include <cstdlib>
int main() {
system("ls"); // For Unix/Linux; use "dir" for Windows to list directory contents
return 0;
}
Understanding the C++ System Command
What is the `system()` Function?
The `system()` function is a powerful feature in C++ that allows programmers to execute system commands directly from their C++ programs. The function is defined in the C standard library and its syntax is as follows:
int system(const char* command);
When you call `system()`, you pass a C-style string (null-terminated character array) as a command to be executed by the operating system's shell. Upon successful execution, the function returns an integer value, which is generally the return code of the command executed. A return value of zero typically indicates success, while any non-zero value signifies an error.
How the System Command Works
When you invoke the `system()` function, it interacts with the underlying operating system to create a child process, which executes the specified command. The command is passed to the system shell (like Bash in Linux or Command Prompt in Windows), making it highly versatile because it can execute any command that would normally work in those environments.
For instance, in Unix-based systems, calling `system("ls")` would generate a list of files and directories in the current working directory, similar to typing `ls` in the terminal.
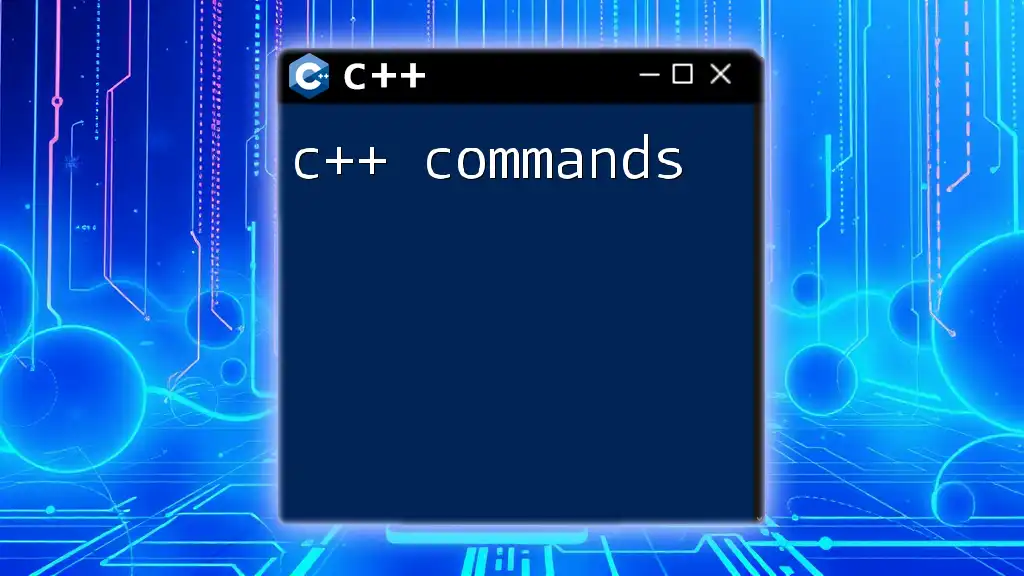
Advantages of Using System Command
Flexibility with Operating System Commands
Using the C++ system command adds significant flexibility to your programs. It enables developers to leverage existing operating system features without needing to write extensive code for tasks like file management, process handling, and more. This is particularly useful for automating repetitive tasks and enhances the efficiency of programming efforts.
Examples of System Commands
Here are a couple of simple yet effective examples demonstrating how you can utilize the system command in C++:
Example 1: Listing all files in a directory
#include <cstdlib>
int main() {
system("ls -l"); // Unix/Linux command
return 0;
}
In the example above, the `ls -l` command lists files in a detailed format. This could be beneficial for quickly checking the contents of a directory.
Example 2: Opening a text file in the default editor
#include <cstdlib>
int main() {
system("notepad.exe myfile.txt"); // Windows command
return 0;
}
In this instance, we are opening a file called `myfile.txt` in Notepad on a Windows system, providing a straightforward way to engage with files directly from a C++ program.
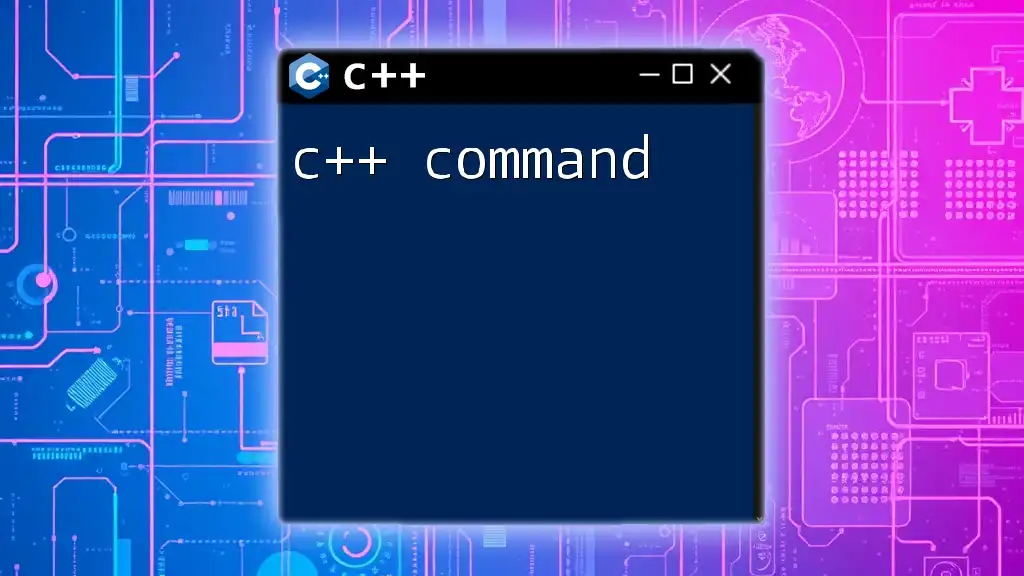
Cautions When Using System Command
Security Risks
While using `system()` can be incredibly convenient, it also introduces security vulnerabilities. One major risk is command injection, where an attacker could manipulate input and execute harmful commands on your system. It is crucial to always sanitize user inputs when dynamically constructing commands for execution. For instance, rather than directly appending user input to the command string, consider validating it or using safer alternatives.
Portability Issues
Another important concern when working with the `system()` function is portability. Commands and their syntax will vary across different operating systems. For example, a command working in a Linux environment may not function in Windows without revision. This can pose challenges when developing cross-platform applications, necessitating careful consideration during the design phase to ensure compatibility.
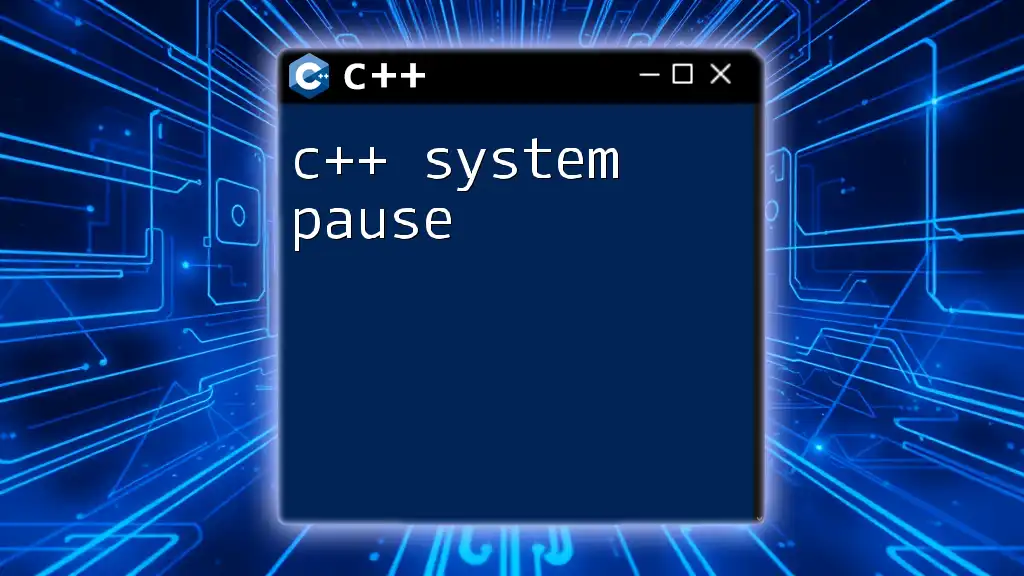
Best Practices for Using the System Command in C++
Minimizing Use of System Commands
Though `system()` is useful, over-reliance on it can lead to poorly structured code and potential safety risks. It's advisable to use system commands sparingly and only when there's no alternative. In many cases, you can achieve the desired outcomes using standard library functions or other system calls, which are typically safer and more efficient.
Error Handling and Return Values
Whenever you use the `system()` function, it's essential to handle errors appropriately. By checking the return values, you can respond to any issues that occur during command execution. Here’s how you can incorporate error handling in your code:
#include <cstdlib>
#include <iostream>
int main() {
int returnCode = system("some_command");
if (returnCode != 0) {
std::cerr << "Command failed with return code: " << returnCode << std::endl;
}
return 0;
}
In this code snippet, if the command fails (i.e., returns a non-zero value), an error message is printed to the standard error stream, helping with debugging and issue identification.
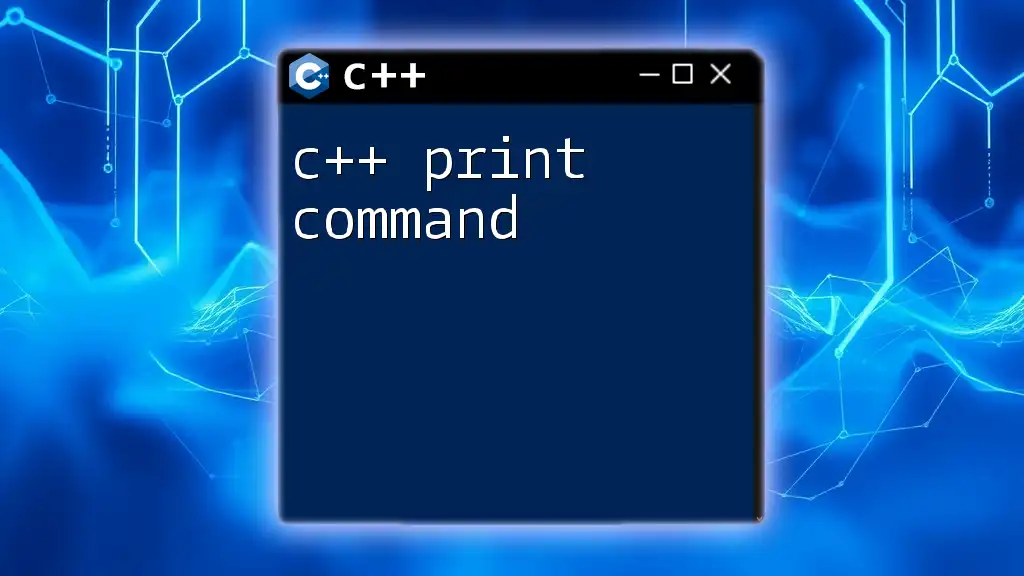
Conclusion
The `system()` command in C++ provides developers with a quick and effective tool for leveraging operating system features directly from their programs. While it's a powerful addition, it comes with responsibilities concerning security and portability. Therefore, it is critical to practice safe coding techniques and consider alternative methods when feasible.
By understanding the implications of using the C++ system command, you can enhance your programming toolkit while ensuring that your applications remain robust and secure. As you continue your exploration of system programming, consider delving into related topics such as process management and threading to deepen your expertise.