The least common multiple (LCM) of two integers in C++ can be calculated using the formula LCM(a, b) = abs(a * b) / GCD(a, b), where GCD is the greatest common divisor.
Here’s a simple code snippet to find the LCM of two numbers:
#include <iostream>
using namespace std;
// Function to calculate GCD
int gcd(int a, int b) {
return b == 0 ? a : gcd(b, a % b);
}
// Function to calculate LCM
int lcm(int a, int b) {
return abs(a * b) / gcd(a, b);
}
int main() {
int num1 = 12, num2 = 15;
cout << "LCM of " << num1 << " and " << num2 << " is " << lcm(num1, num2) << endl;
return 0;
}
Understanding Least Common Multiple
Least Common Multiple (LCM) is a fundamental concept in mathematics that finds significant application in programming, especially in C++. The LCM of two integers is defined as the smallest positive integer that is divisible by both numbers. Understanding LCM is vital for solving problems related to scheduling and synchronization in programming.
For example, consider the integers 12 and 15. The multiples of 12 are 12, 24, 36, 48, etc., while the multiples of 15 are 15, 30, 45, 60, and so on. The smallest common multiple is 60, hence LCM(12, 15) = 60.
What is LCM?
The LCM is essential when dealing with fractions, ratios, or any scenario where you need to find a common timeframe. Additionally, the Greatest Common Divisor (GCD) complements the LCM, allowing us to determine the LCM using its mathematical relationship. The formula is:
LCM(a, b) = |a * b| / GCD(a, b)
Mathematical Properties of LCM
Some essential properties of LCM include:
- LCM is commutative: LCM(a, b) = LCM(b, a)
- LCM is associative: LCM(a, LCM(b, c)) = LCM(LCM(a, b), c)
- LCM of any number with zero is zero: LCM(a, 0) = 0
Understanding these properties can simplify problem-solving and code implementation.
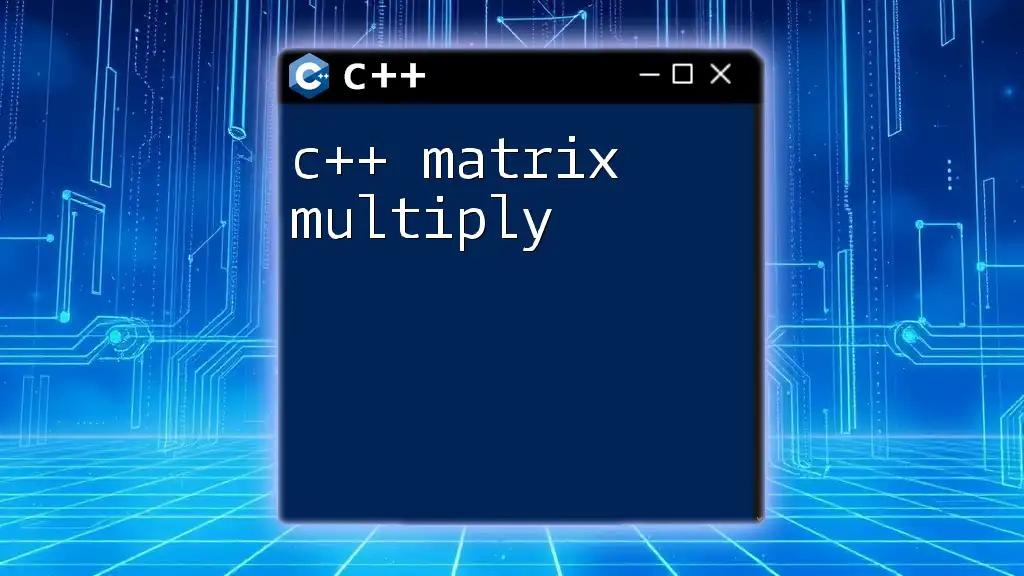
Methods to Calculate LCM in C++
There are multiple methods to calculate the C++ least common multiple. We will explore three primary approaches: using the mathematical formula, implementing an iterative method, and leveraging function templates.
Using the Mathematical Formula
The mathematical formula for calculating LCM is direct and efficient. Here’s the implementation in C++:
#include <iostream>
#include <numeric> // for std::gcd
int lcm(int a, int b) {
return std::abs(a * b) / std::gcd(a, b);
}
int main() {
int a = 12, b = 15;
std::cout << "LCM of " << a << " and " << b << " is " << lcm(a, b) << std::endl;
return 0;
}
Explanation of Code:
- The program includes the `<numeric>` header for access to the `std::gcd` function that computes the greatest common divisor.
- The lcm function calculates LCM using the relation with GCD, leveraging `std::abs()` to ensure the multiplication results in a positive number.
- In the main function, it prints the LCM of two defined integers.
Iterative Method
Another approach to compute LCM is via an iterative method, which involves incrementally checking for the least multiple. This method provides a clear understanding of how LCM is derived:
int lcm_iterative(int a, int b) {
int lcm = std::max(a, b);
while (true) {
if (lcm % a == 0 && lcm % b == 0)
return lcm;
lcm++;
}
}
Explanation of Code:
- The lcm_iterative function starts with the maximum of a or b and continually checks if the current value is divisible by both.
- The loop continues until it finds the least common multiple.
- This method, while easy to understand, may not be the most performance-efficient for larger integers.
Using a Function Template
Function templates allow for greater flexibility, especially when working with multiple data types. Here’s how you can implement LCM using a template:
template <typename T>
T lcm(T a, T b) {
return std::abs(a * b) / std::gcd(a, b);
}
Explanation of Code:
- The template keyword allows lcm to accept arguments of various types, making it versatile across different numeric types.
- By using `std::abs()` and `std::gcd`, we ensure a robust solution that avoids type conflicts.
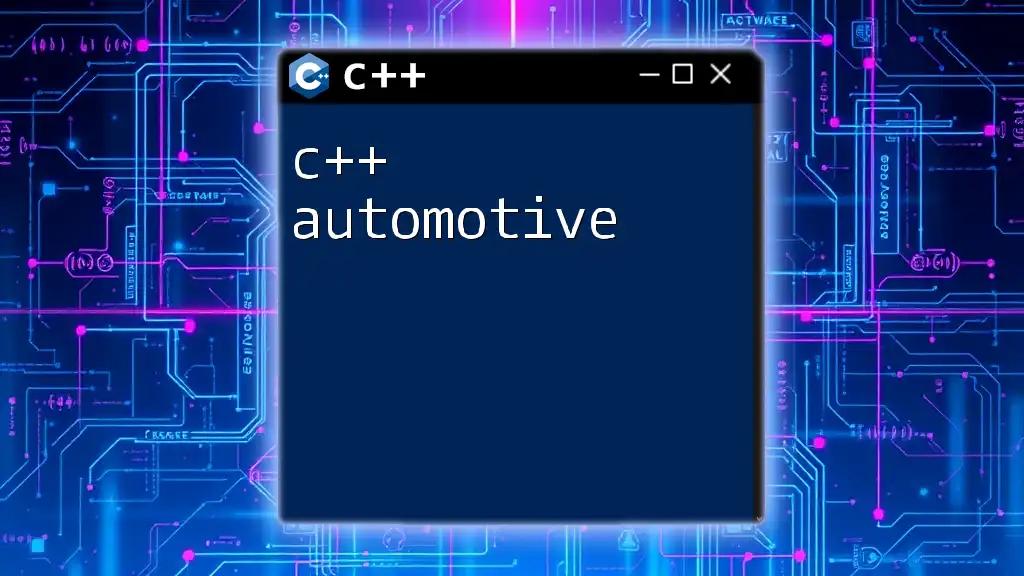
Practical Applications of LCM in C++
Real-World Examples
Understanding how to implement C++ least common multiple can help in various real-world scenarios. One significant application is in scheduling problems, where you need to determine when events will coincide.
Consider a scenario where you have two events: one occurs every 5 days and another every 7 days. To find when they coincide again, you can use the LCM:
int main() {
int interval1 = 5; // every 5 days
int interval2 = 7; // every 7 days
std::cout << "Next event will occur in " << lcm(interval1, interval2) << " days." << std::endl;
return 0;
}
Analysis of the Example:
- This short C++ program effectively illustrates how LCM is used in practical scheduling. After calculating, it informs the user when the two events will next align.
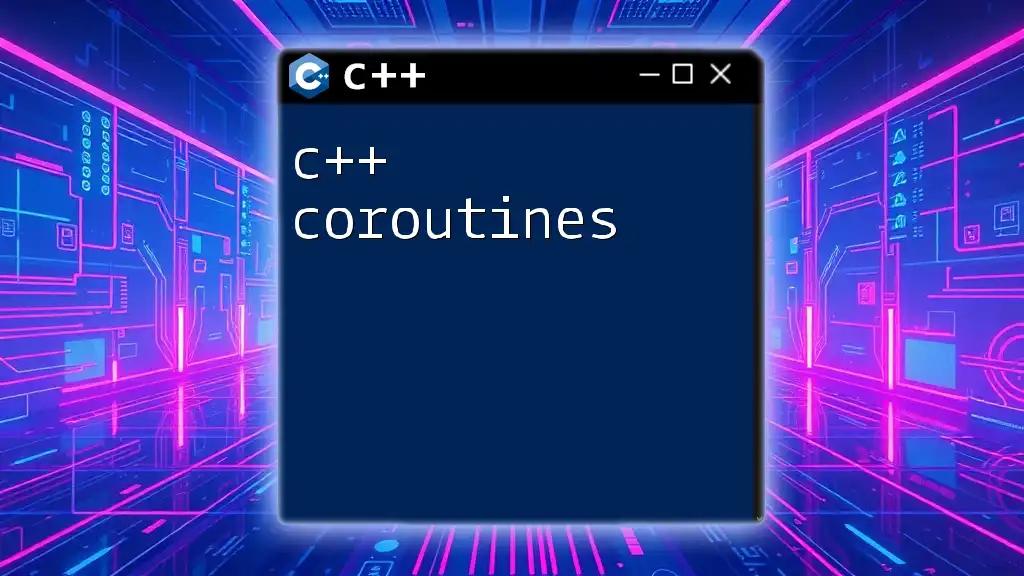
Performance Considerations
When implementing LCM in C++, understanding the complexity of different methods is crucial. The mathematical formula method is efficient, operating in logarithmic time due to the GCD calculation. Conversely, the iterative method can become inefficient for large integers, as it may require many iterations.
Best Practices
- It's essential to choose the appropriate method based on the expected input size.
- When calculating LCM for two numbers repeatedly, utilizing the mathematical formula is generally advisable for efficiency.

Conclusion
In summary, mastering the C++ least common multiple equips you with the knowledge to tackle various mathematical and scheduling problems effectively. By exploring methods using both the mathematical formula and iterative approaches, you can choose the best technique suited for your specific use case.
I encourage you to practice implementing LCM in your own projects, as this foundational concept will enhance your overall programming skills. For further learning, consider exploring more in-depth resources or online courses dedicated to C++ and mathematical algorithms.
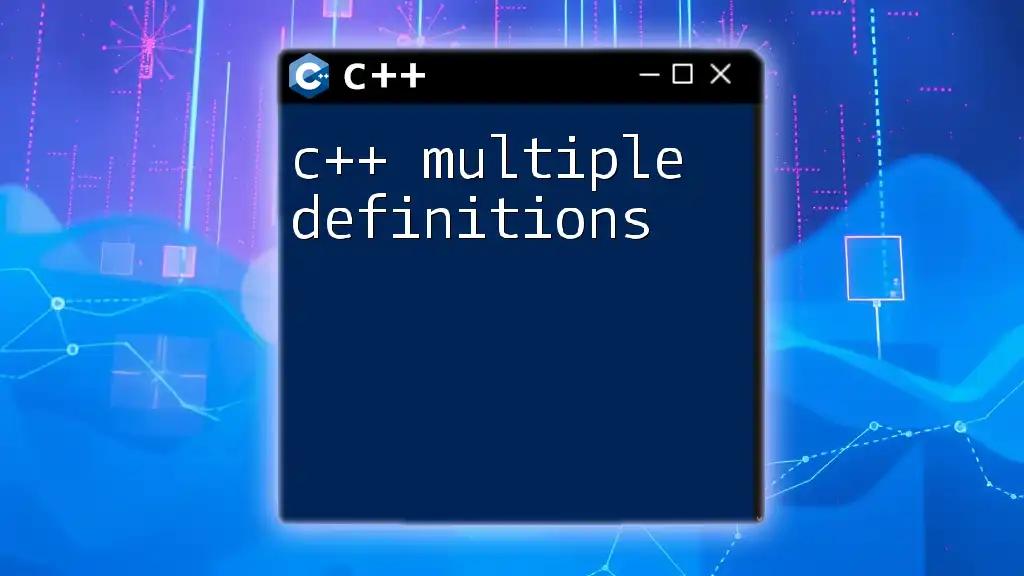
FAQs
What is the least common multiple of 0?
- The LCM of any number with zero is zero because zero is not a multiple of any number.
Can LCM be calculated for more than two numbers?
- Yes, LCM can be calculated for more than two numbers by repeatedly applying the LCM function: LCM(a, LCM(b, c)).
How does LCM relate to fractions?
- LCM is used to find a common denominator when adding or comparing fractions, ensuring each fraction can be expressed in terms of the other.