In C++, the `complex` class from the `<complex>` header is used to represent and manipulate complex numbers, allowing for operations involving both real and imaginary components.
#include <iostream>
#include <complex>
int main() {
std::complex<double> num1(3.0, 2.0); // 3 + 2i
std::complex<double> num2(1.0, 4.0); // 1 + 4i
std::complex<double> sum = num1 + num2; // Addition
std::cout << "Sum: " << sum << std::endl; // Output: (4, 6)
return 0;
}
What are Complex Numbers?
Complex numbers are defined as a number of the form a + bi, where a represents the real part, b represents the imaginary part, and i is the imaginary unit, satisfying the property \(i^2 = -1\). Complex numbers extend the idea of one-dimensional number lines into the two-dimensional complex plane, allowing for new mathematical operations and interpretations.
In various fields such as engineering, physics, and computer graphics, complex numbers are indispensable. They’re utilized in signal processing, quantum mechanics, and even in algorithms for rendering graphics. This versatility makes understanding complex numbers crucial for modern programming, especially in languages like C++.
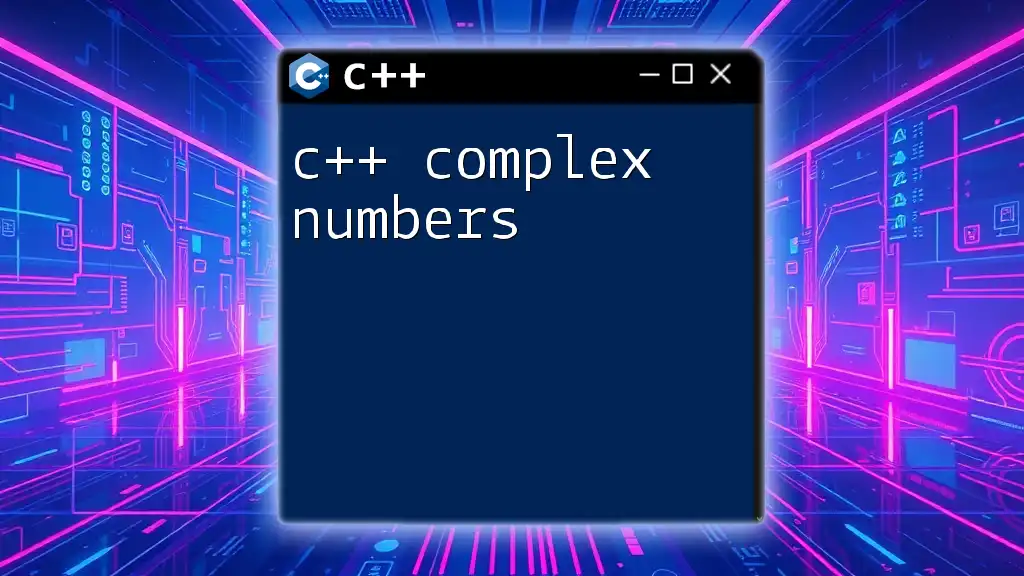
Importance of Complex Numbers in C++
Complex numbers are frequently encountered in calculations that involve oscillations, waves, and other phenomena that can be represented in two dimensions. C++ provides a built-in way to handle complex numbers through the `<complex>` library, thus making it easier for programmers to work with these mathematical constructs without getting bogged down in the nitty-gritty details of their implementations.
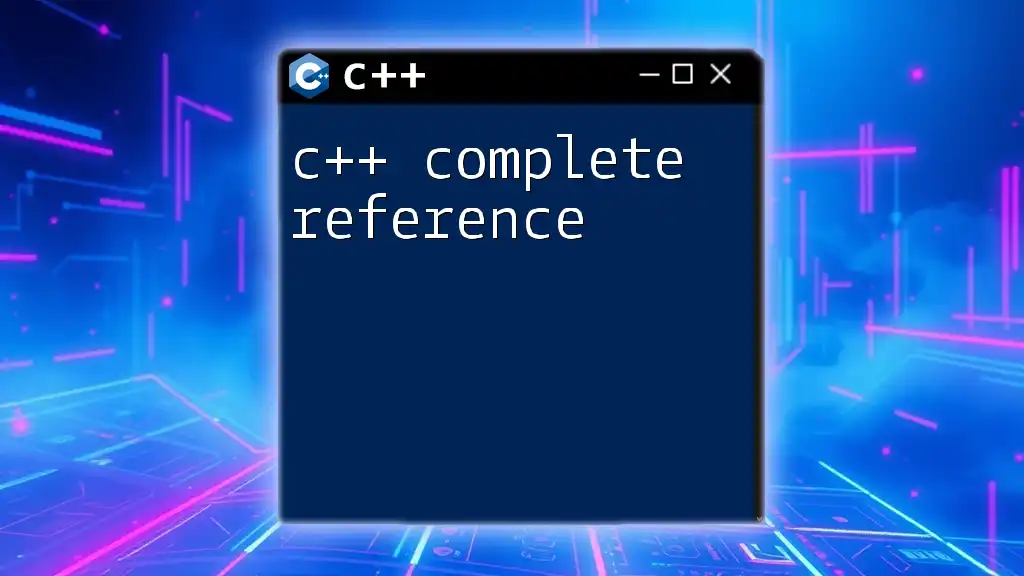
Understanding the `<complex>` Library
Overview of the `<complex>` Header
The `<complex>` library in C++ is specifically designed to handle complex number operations smoothly and effectively. To utilize the functionalities that it offers, you need to include this header in your program:
#include <complex>
This inclusion gives you access to a template class called `std::complex<T>`, where `T` could be any floating-point type like `float`, `double`, or `long double`. This flexibility allows programmers to choose the precision that best fits their needs.
Basic Types of Complex Numbers
To declare a complex number in C++, you can use the `std::complex` template. For instance, to create a complex number with real part 3 and imaginary part 4, you would write:
std::complex<double> c1(3.0, 4.0); // Creates a complex number 3 + 4i
This construction allows you to leverage all mathematical operations and functions designed for complex numbers in C++.
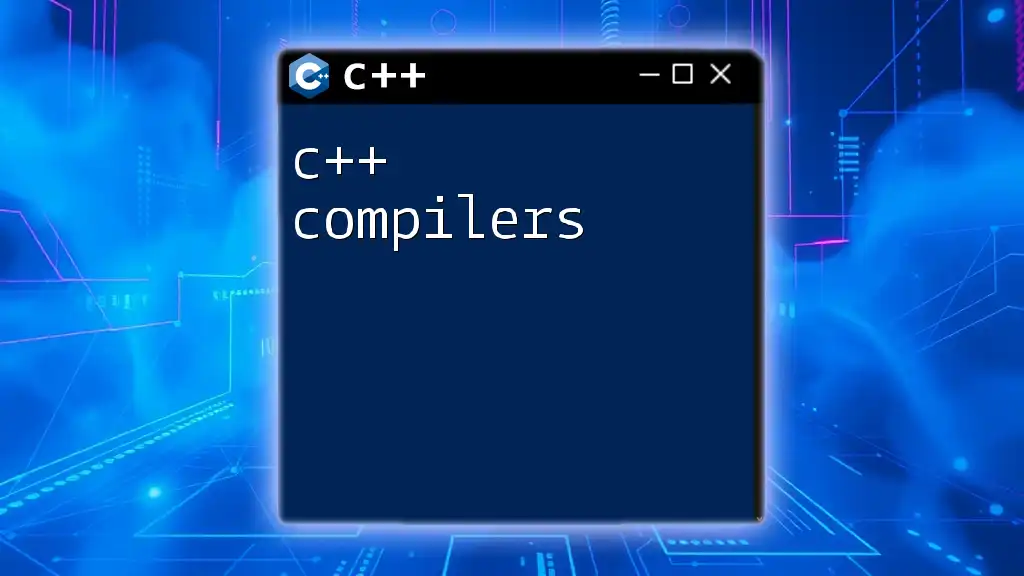
Operations with Complex Numbers
Arithmetic Operations
Addition and Subtraction
Complex numbers can be added or subtracted just like real numbers, except that the imaginary parts are also included. For instance, if you have:
std::complex<double> c2(1.0, 2.0);
auto sum = c1 + c2; // (3 + 4i) + (1 + 2i) = 4 + 6i
auto diff = c1 - c2; // (3 + 4i) - (1 + 2i) = 2 + 2i
In these examples, the real parts are added separately, and the imaginary parts are added separately.
Multiplication and Division
The multiplication and division of complex numbers are defined by the distributive property:
auto product = c1 * c2; // (3 + 4i)(1 + 2i) = -5 + 10i
auto quotient = c1 / c2; // (3 + 4i)/(1 + 2i) = 2 - 1i
This notation illustrates that you multiply and divide the real and imaginary parts according to established mathematical rules.
Advanced Mathematical Operations
Magnitude and Angle
Understanding the magnitude (or modulus) and angle (or argument) of a complex number can be crucial in fields like engineering and physics. You can compute these values using the following code:
double magnitude = std::abs(c1); // Computes |c1| = sqrt(3^2 + 4^2) = 5
double angle = std::arg(c1); // Computes the angle in radians
Conjugate of Complex Numbers
The conjugate of a complex number is obtained by changing the sign of its imaginary part. For example, the conjugate of `c1` (3 + 4i) is:
auto conjugate = std::conj(c1); // Gives (3 - 4i)
This concept is often used in complex division and various mathematical computations.
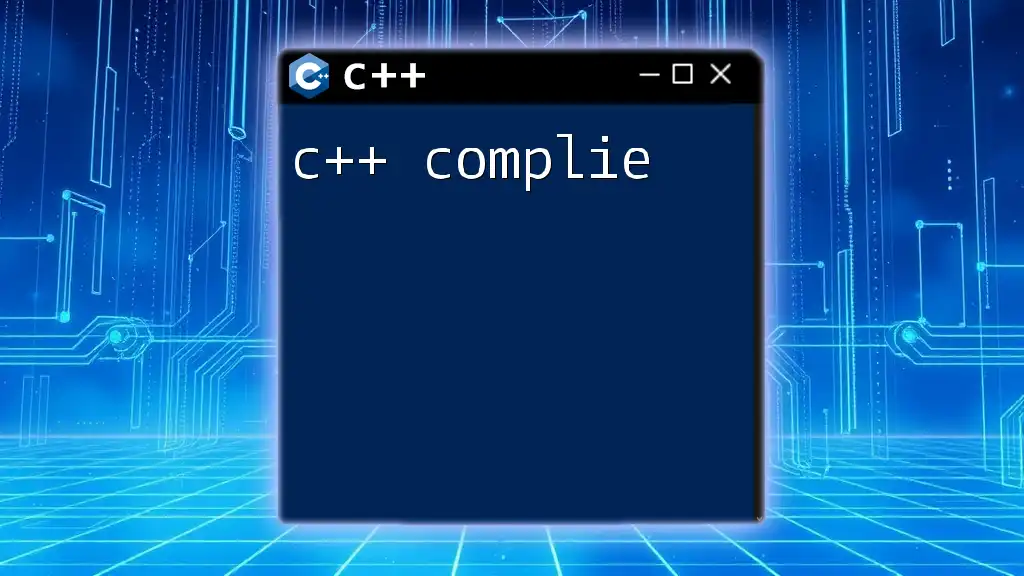
Using C++ Complex Numbers in Functions
Passing Complex Numbers as Function Parameters
Complex numbers can be efficiently passed to functions using constant references. This is beneficial for performance, as it avoids unnecessary copying:
void printComplex(const std::complex<double>& c) {
std::cout << c << std::endl; // Prints the complex number in standard format
}
Returning Complex Numbers from Functions
Functions can also return complex numbers. Here’s how you can create a function that adds two complex numbers and returns the result:
std::complex<double> addComplex(const std::complex<double>& a, const std::complex<double>& b) {
return a + b; // Returns the sum of two complex numbers
}
This approach emphasizes the functional programming paradigm, whereby complex numbers are manipulated elegantly.
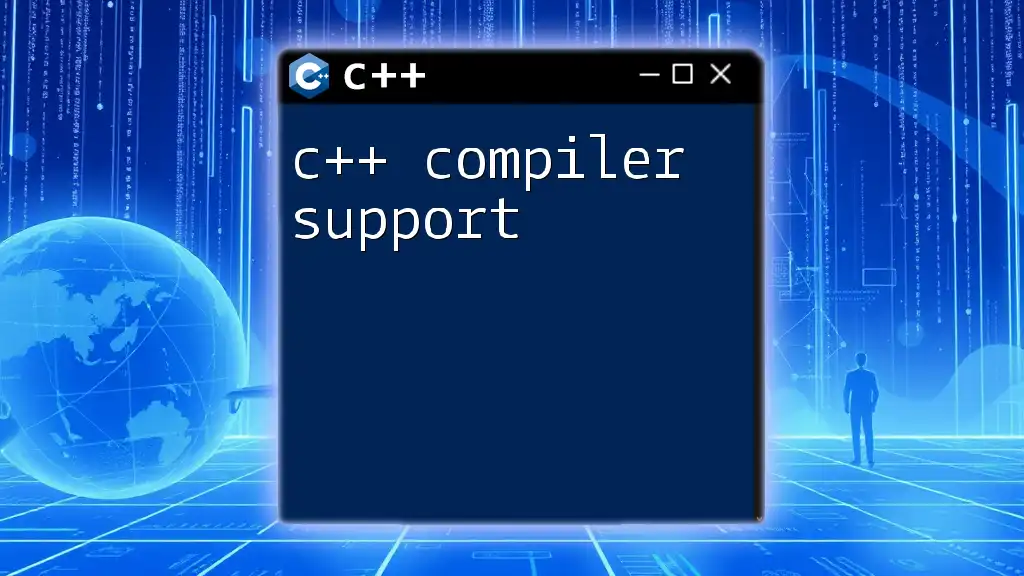
Practical Applications of C++ Complex Numbers
Image Processing and Computer Graphics
In digital image processing, complex numbers are often used to represent pixel transformations and filtering processes. The Fourier Transform, which is pivotal in frequency analysis, uses complex numbers extensively. C++ manages this analytically, making it indispensable for developers working with graphics.
Solving Differential Equations
Complex numbers also play a crucial role in scientific computing, particularly for solving differential equations. Many physical phenomena can be described effectively using complex analysis, allowing engineers and scientists to model real-world systems with precision.
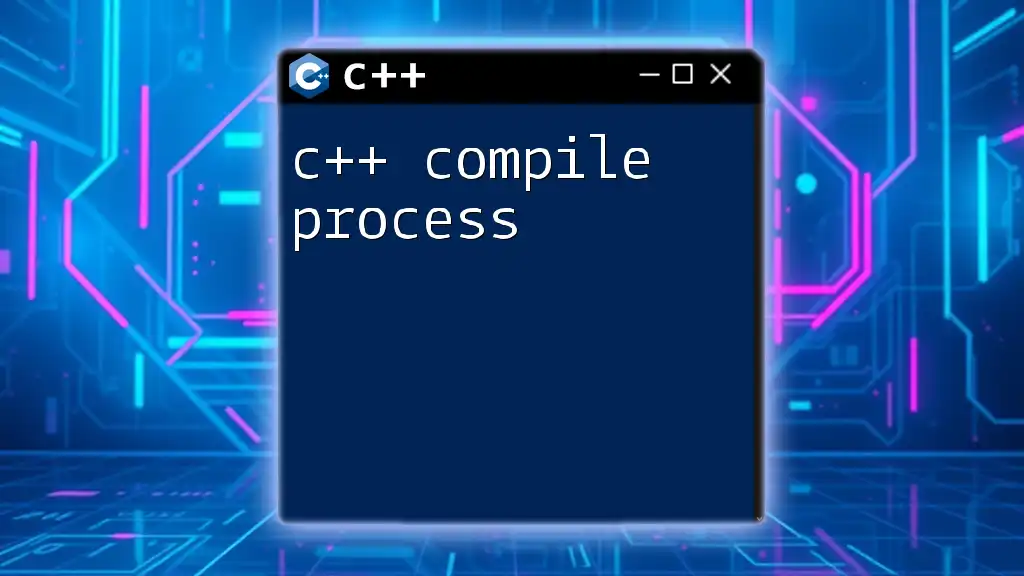
Common Pitfalls and Best Practices
Common Errors When Using Complex Numbers
One of the most frequent mistakes is confusing the real and imaginary parts when performing arithmetic operations. For instance, treating a complex number as a real one could yield unexpected results.
Best Practices
When working with complex numbers, use the functionalities provided by the `<complex>` library optimally. Always prefer passing complex numbers by const reference to improve performance. Rely on the built-in functions for mathematical operations to prevent manual errors and keep your code cleaner and more maintainable.
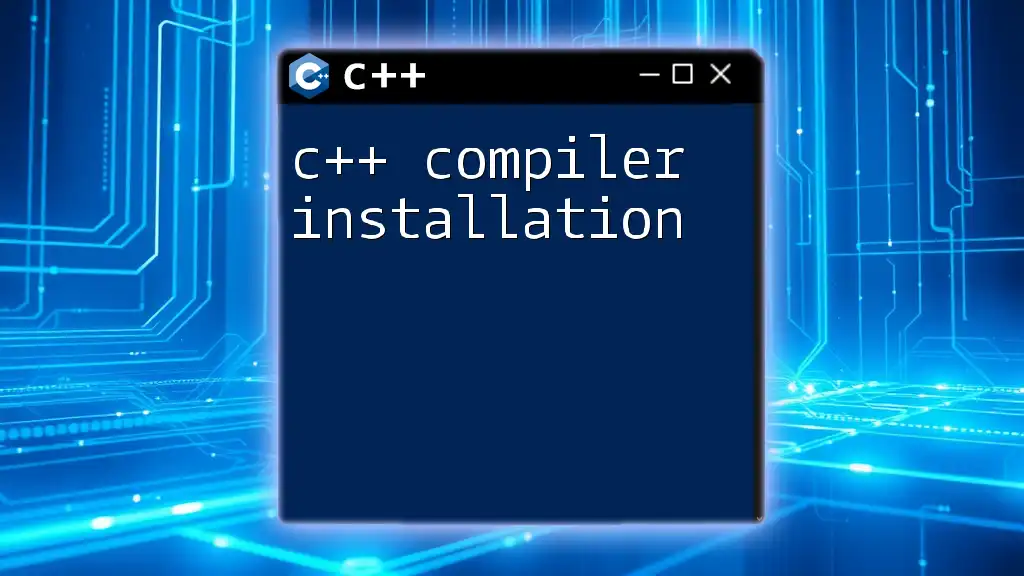
Conclusion
Understanding C++ complex numbers opens up a world of possibilities in both mathematical computation and practical applications. From advanced graphics to solving multifaceted equations, the complex number library in C++ provides a robust solution. By diving deep into this guide, you can harness the power of complex numbers to enhance your programming toolkit and build more sophisticated applications.
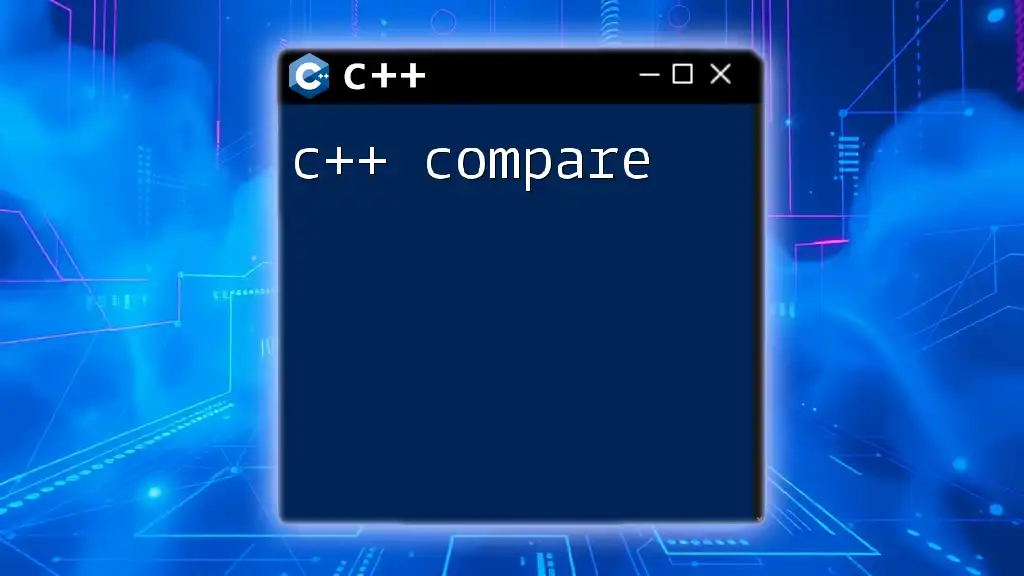
Additional Resources
For those seeking to expand their knowledge further, consider exploring resources such as community forums, online tutorials, and official C++ documentation. They provide an excellent foundation and support for continuous learning in the complexities of C++ programming.