C++ codes are a series of instructions written in the C++ programming language, enabling developers to perform a variety of tasks, such as creating variables, defining functions, and managing data.
Here's an example of a simple C++ code snippet that demonstrates how to declare a variable and print its value:
#include <iostream>
using namespace std;
int main() {
int a = 10;
cout << "The value of a is: " << a << endl;
return 0;
}
Getting Started with C++
Setting Up Your Environment
To dive into C++ codes, you first need to set up your development environment. Popular Integrated Development Environments (IDEs) such as Visual Studio, Code::Blocks, and GCC (GNU Compiler Collection) are excellent choices. Here are the steps to install and set up one of these IDEs:
- Download the desired IDE from its official website.
- Install the IDE by following the on-screen instructions.
- Configure the compiler if it doesn’t automatically setup (requires specifying the path in the IDE settings).
Basic Syntax Overview
C++ has its own syntax rules that a programmer should adhere to. Most importantly, C++ is case-sensitive, meaning that Variable and variable would be considered two different identifiers. Also, understand the significance of delimiters (like braces `{}` and semicolons `;`) which are essential for separating instructions.
There are several commonly used terms to know:
- Keywords: Reserved words in C++ that have special meaning (e.g., `int`, `return`, `if`).
- Identifiers: Names you create to represent variables, functions, etc.
By understanding these foundational elements, you're well-prepared to write simple C++ codes.
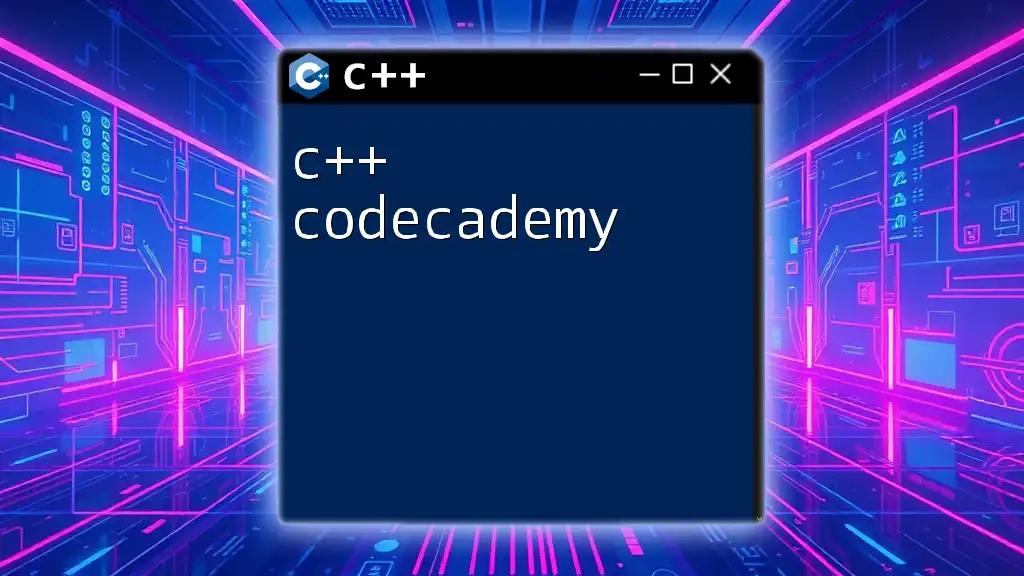
Fundamental C++ Codes
Hello World Program
A classic kickoff to learning any programming language is the proverbial "Hello, World!" program, which illustrates the most basic structure of a C++ program. Below is an example:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Breakdown of the Code:
- `#include <iostream>`: This line includes the Input/Output stream library necessary for outputting text to the console.
- `int main()`: The main function where execution begins.
- `std::cout`: Standard output stream used to display text.
- `std::endl`: Adds a new line after the output.
- `return 0;`: Terminates the program and signals successful execution.
Variables and Data Types
Variables are fundamental in storing data in a program. In C++, you must declare a variable with its respective data type before using it. Common data types include:
- int: for integers.
- float: for floating-point numbers.
- char: for single characters.
- string: for sequences of characters.
Here's an example of how to declare and initialize variables:
int age = 25;
float height = 5.9;
char initial = 'A';
In this code:
- `int age = 25;` declares an integer variable `age` and initializes it with the value 25.
- `float height = 5.9;` declares a floating-point variable `height`.
- `char initial = 'A';` declares a character variable `initial`.
Control Structures
Conditional Statements
Control structures allow you to dictate the flow of your program based on conditions. One common type is the if statement. The following code checks if a person is an adult:
if (age >= 18) {
std::cout << "Adult";
} else {
std::cout << "Minor";
}
In this example, if `age` is 18 or older, it outputs "Adult"; otherwise, it outputs "Minor".
Loops
Loops are used for repeating a block of code. C++ offers several types, including for, while, and do-while loops. Here’s an example of a simple for loop that prints numbers from 0 to 4:
for (int i = 0; i < 5; i++) {
std::cout << i << std::endl;
}
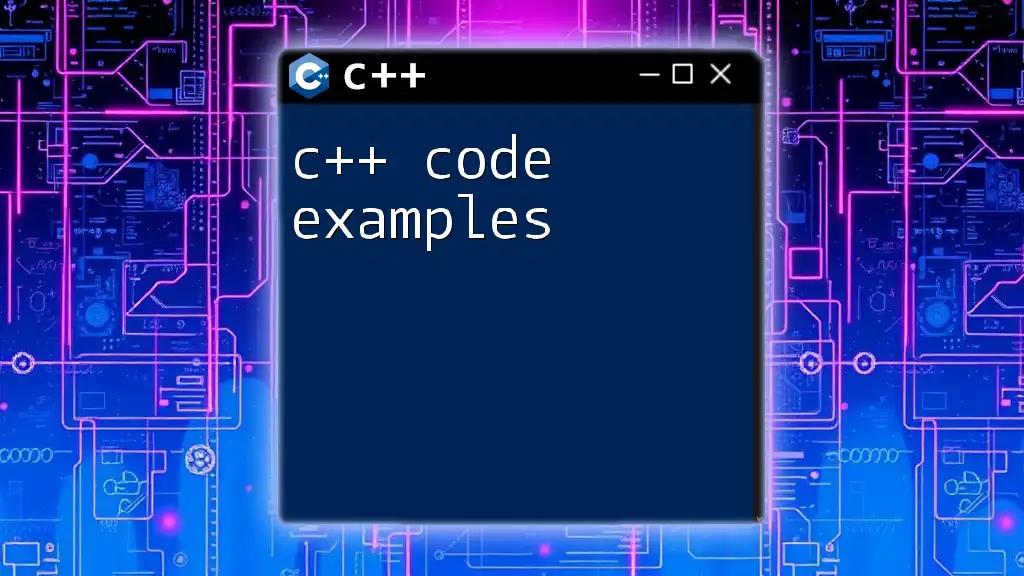
Advanced C++ Concepts
Functions
Functions break your code into reusable chunks. They improve modularity and help manage complexity. Here’s a simple function to add two integers:
int add(int a, int b) {
return a + b;
}
In this code, `add` takes two integer parameters and returns their sum.
Object-Oriented Programming (OOP) in C++
Classes and Objects
C++ is an object-oriented language, allowing you to create classes that encapsulate data and functionalities. Below is a basic example of a class:
class Dog {
public:
void bark() {
std::cout << "Woof!" << std::endl;
}
};
Usage: You can create an object of this class and call its methods:
Dog myDog;
myDog.bark(); // Outputs "Woof!"
Inheritance
Inheritance enables one class to inherit properties from another, promoting code reuse. Below is an example of a base class and a derived class:
class Animal {
public:
void sound() {
std::cout << "Some sound" << std::endl;
}
};
class Cat : public Animal {
public:
void sound() {
std::cout << "Meow" << std::endl;
}
};
Here, `Cat` inherits from `Animal`, and you can call `sound()` from both classes.
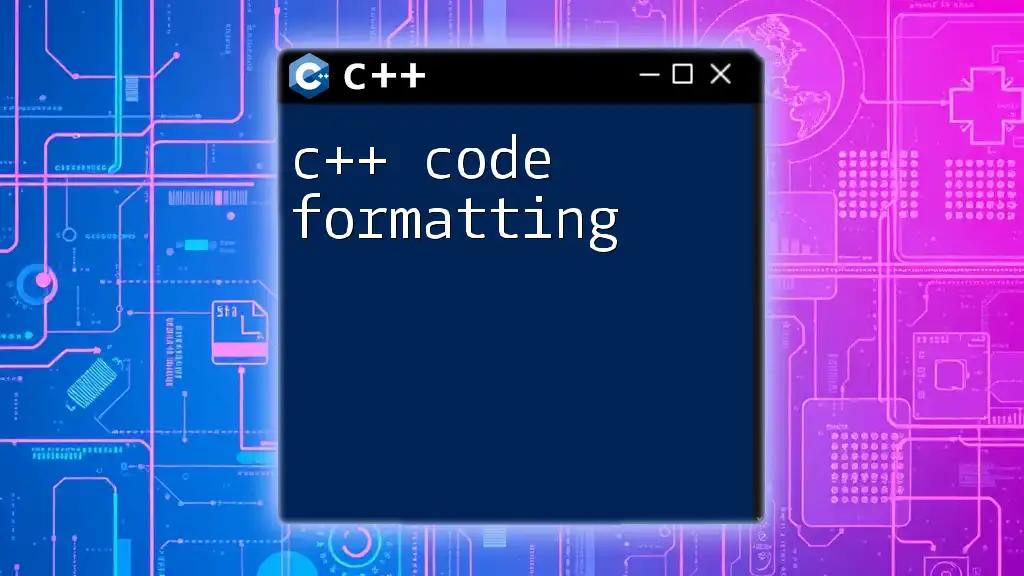
Important C++ Features
Templates
Templates enable you to write generic and reusable code. Below is a function template that adds two values of the same type:
template <typename T>
T add(T a, T b) {
return a + b;
}
Using this template, you can add integers, floats, or any type that supports the `+` operator.
Standard Template Library (STL)
The Standard Template Library provides a collection of useful classes and functions. Vectors, for example, are dynamic arrays that can grow in size as needed. Here's how to use them:
#include <vector>
std::vector<int> numbers = {1, 2, 3};
for (int num : numbers) {
std::cout << num << std::endl;
}
In this snippet, a vector of integers is printed using a range-based for loop.
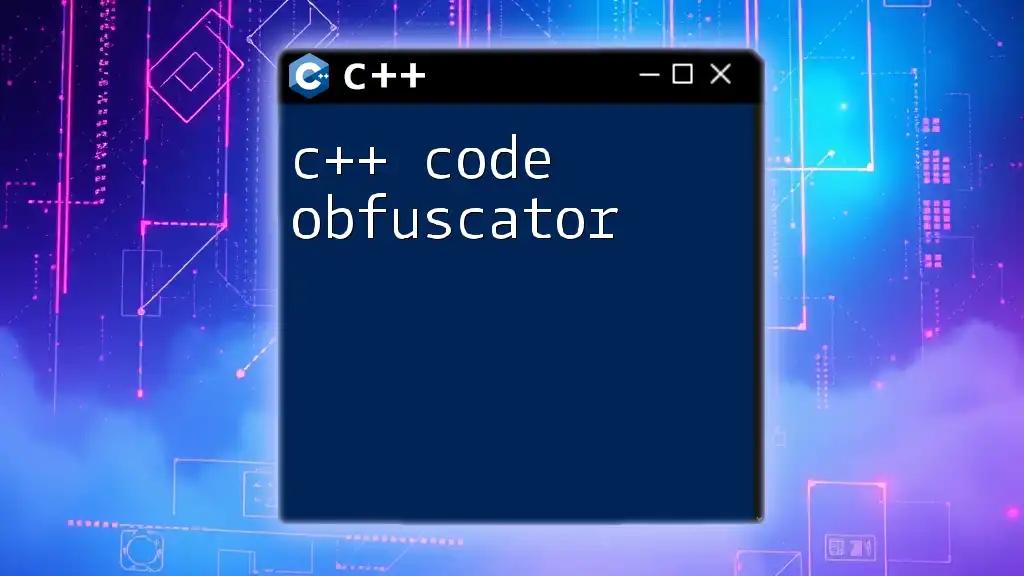
Debugging and Error Handling
Common Compilation Errors
Compilation errors can often stem from syntax issues, such as missing semicolons or unmatched braces. Understanding and resolving these errors is a critical part of programming.
Debugging Techniques
Integrated development environments come with built-in debugging tools. Utilize breakpoints, step execution, and watch variables to identify and resolve issues.
Exception Handling
C++ provides mechanisms to catch exceptions and handle errors gracefully. Below is an example using a try-catch block:
try {
throw std::runtime_error("An error occurred.");
} catch (const std::exception& e) {
std::cout << e.what() << std::endl; // Outputs the exception message.
}
In this code, if an exception is thrown, the catch block allows you to handle the error without terminating the program unexpectedly.
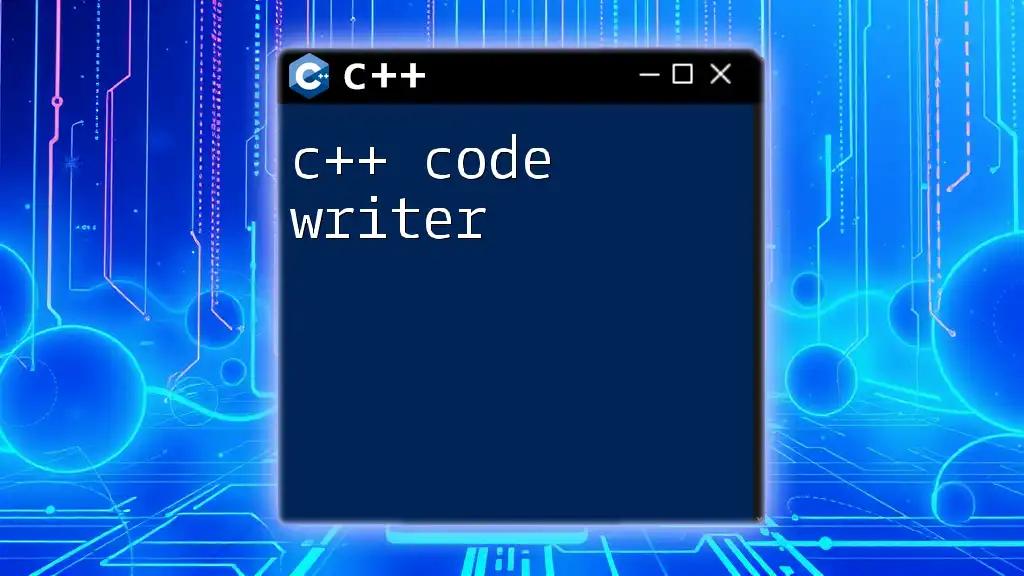
Best Practices for Writing C++ Code
Code Readability
Writing readable code is crucial for collaboration and maintenance. Use comments judiciously and choose meaningful variable names to clarify your code's intent.
Optimization Tips
Avoid unnecessary computations in loops and always prefer algorithms with lower time complexity. Profiling your code can identify hotspots that need improvement, ensuring better performance.
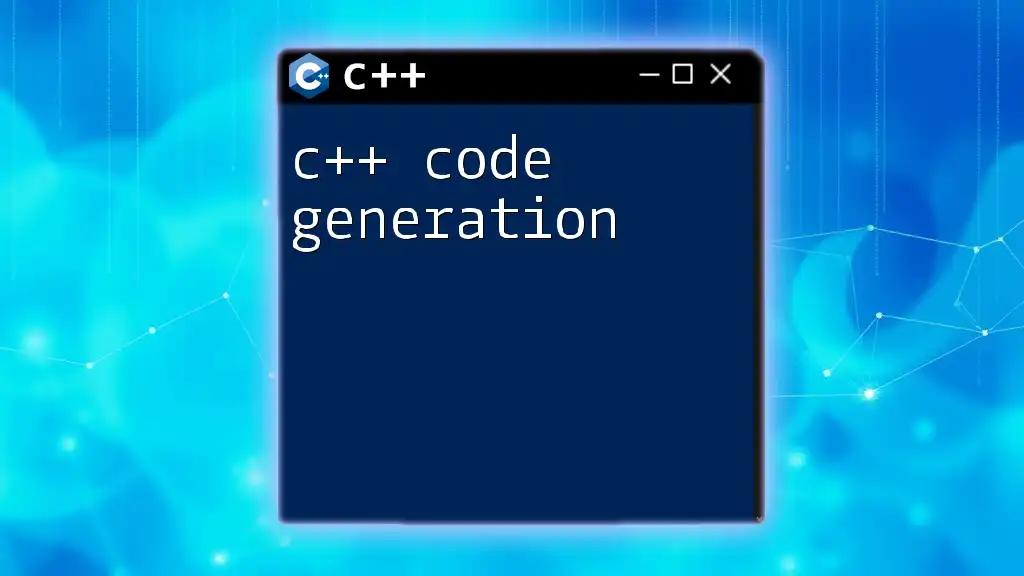
Conclusion
Mastering C++ codes allows you to unlock the immense potential of programming. As you progress, continually refine your skills and seek resources for further learning. With practice and curiosity, you will become proficient in this powerful language.
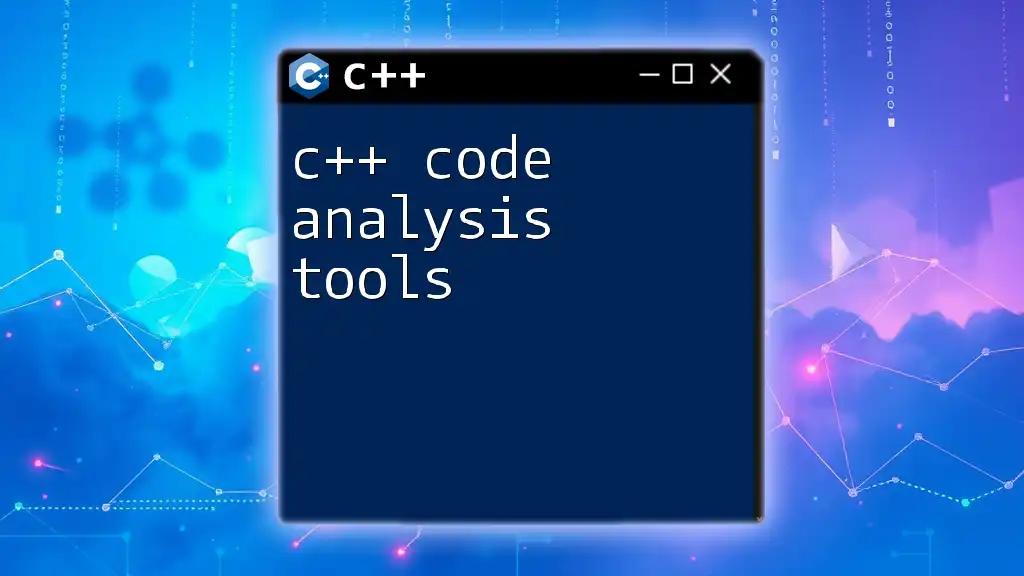
Resources
For further enhancement of your C++ knowledge, consider exploring useful online resources and books. Documentation like [cplusplus.com](http://cplusplus.com) and [cppreference.com](http://cppreference.com) can be invaluable, along with in-depth programming books that guide you through best practices and advanced concepts.