A C++ code obfuscator is a tool that transforms readable source code into a more complex and less understandable version, making it harder to reverse-engineer or understand the original logic.
Here's an example of a simple C++ code snippet before and after obfuscation:
Original Code:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
Obfuscated Code:
#include <iostream>
#define A main
#define B cout
#define C "Hello, World!"
#define D endl
int A() { B << C << D; return 0; }
What is Code Obfuscation?
Code obfuscation is a crucial practice in software development that involves deliberately making code more difficult for humans to understand while preserving its functionality. This process serves multiple purposes, particularly in protecting proprietary code and minimizing risks associated with reverse engineering.
When it comes to C++, the language's capability to interact with system-level functions makes it particularly appealing for developers seeking to safeguard their algorithms and intellectual property. In this context, a C++ code obfuscator plays an essential role by transforming readable code into a version that is much harder for others to interpret, thereby deterring potential theft or misuse.
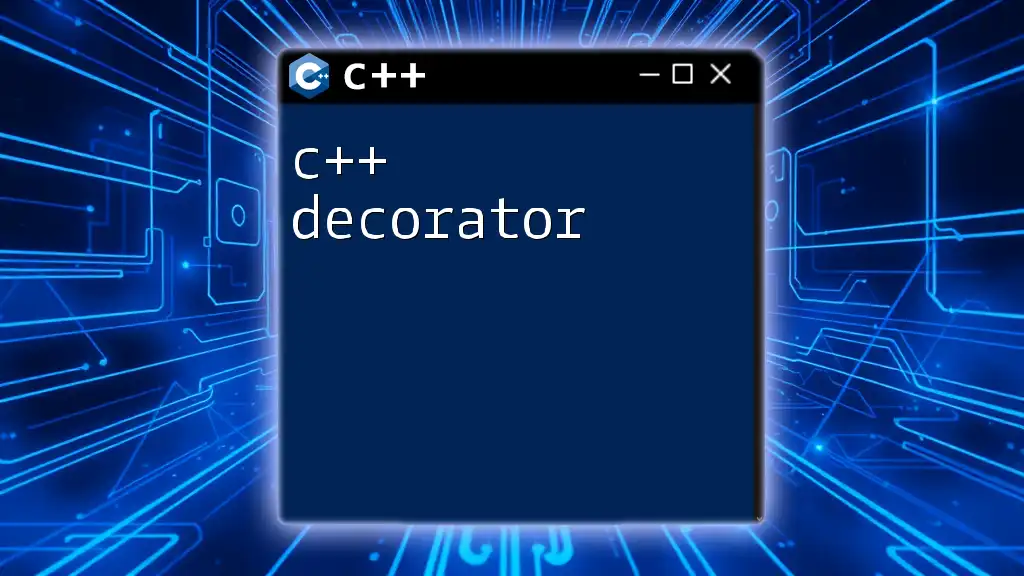
Understanding C++ Code Obfuscators
C++ code obfuscators are tools or processes that manipulate C++ source code to protect its logic and flow. These obfuscators do not merely scramble code; they fundamentally alter the structure and appearance of the code while maintaining its original execution path. It’s important to distinguish that while obfuscation modifies the readability of code, it does not provide the same level of security as encryption.
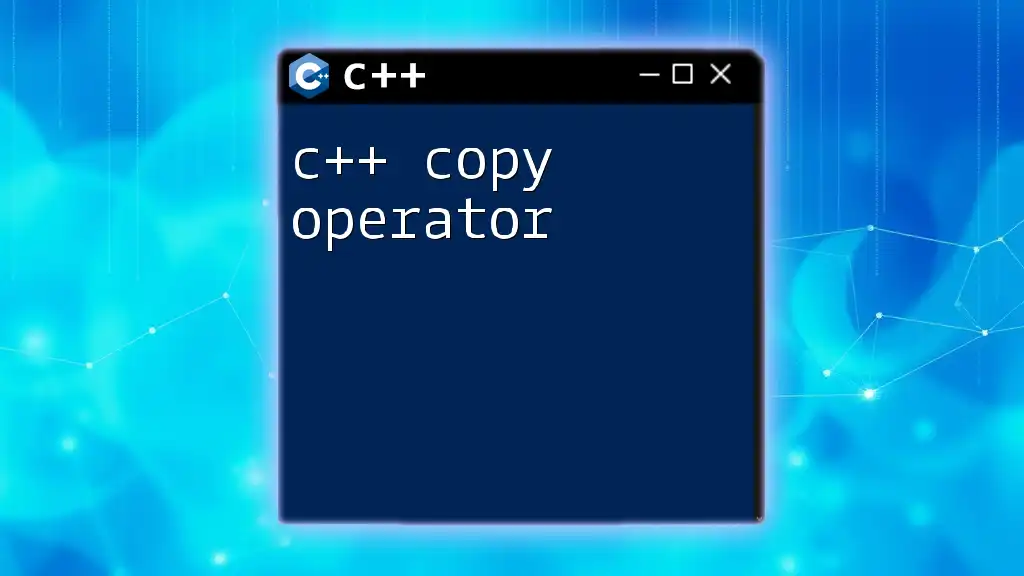
Why Use a C++ Obfuscator?
Protecting Intellectual Property
In an increasingly competitive software industry, safeguarding your code can mean the difference between success and failure. A C++ code obfuscator helps protect intellectual property by making it challenging for malicious actors to glean insights into proprietary algorithms or business logic.
Enhanced Security
Obfuscated code offers a layer of security against unauthorized access. For instance, if sensitive algorithms are widely shared, anyone can exploit them if they understand their functionality. Using a C++ obfuscator can significantly reduce the likelihood of this happening, shielding valuable assets from prying eyes.
Improving Software Reliability
C++ code obfuscation can also contribute to software reliability. By making code less understandable, it becomes harder to introduce unintentional vulnerabilities through malicious alterations. Additionally, code obfuscation can assist in software version management by covering changes thus providing a natural deterrent against casual inspection.
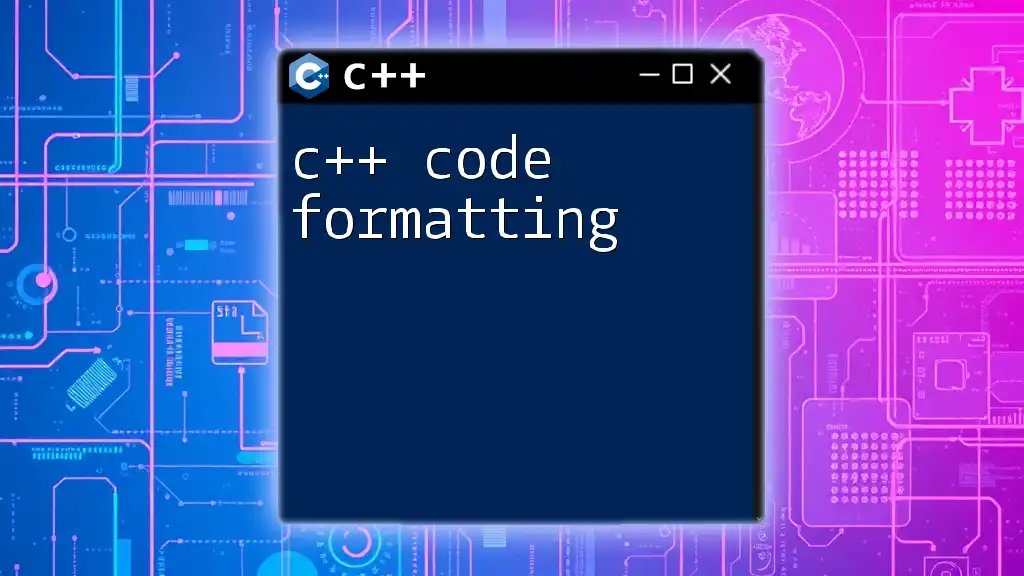
Types of C++ Obfuscation Techniques
Control Flow Obfuscation
Control flow obfuscation modifies the component structures that manage code execution paths. This technique confuses users while keeping the underlying logic intact.
Here’s an example:
// Original Code
if (x > 10) {
y = x * 2;
} else {
y = x / 2;
}
// Obfuscated Code
y = (x > 10) ? (x * 2) : (x / 2);
In this example, while the logic remains the same, the obfuscated version is less clear about the conditions leading to the final outcome.
Data Obfuscation
Data obfuscation focuses on transforming or hiding data structures and their types. This technique can involve renaming variables or substituting straightforward data with less recognizable formats.
Example:
// Original Code
int userAge = 30;
// Obfuscated Code
char obf[8] = {0x1E, 0x0A, 0x78, 0x02, 0x03, 0x06, 0x00, 0x00}; // Example of a hidden value
In this case, the original variable with a meaningful name has been replaced with byte-level representations, making it much less meaningful to a casual observer.
Variable Renaming
Changing the names of variables can further degrade code legibility. Renaming variables to cryptic identifiers makes it hard to discern their purpose or function without a deeper analysis.
A simple example:
// Original Code
int totalSum = calculateSum();
// Obfuscated Code
int a1b2c3 = calculateSum();
Here, the variable `totalSum`, which has a clear purpose, has been transformed into `a1b2c3`, obscuring its intention.
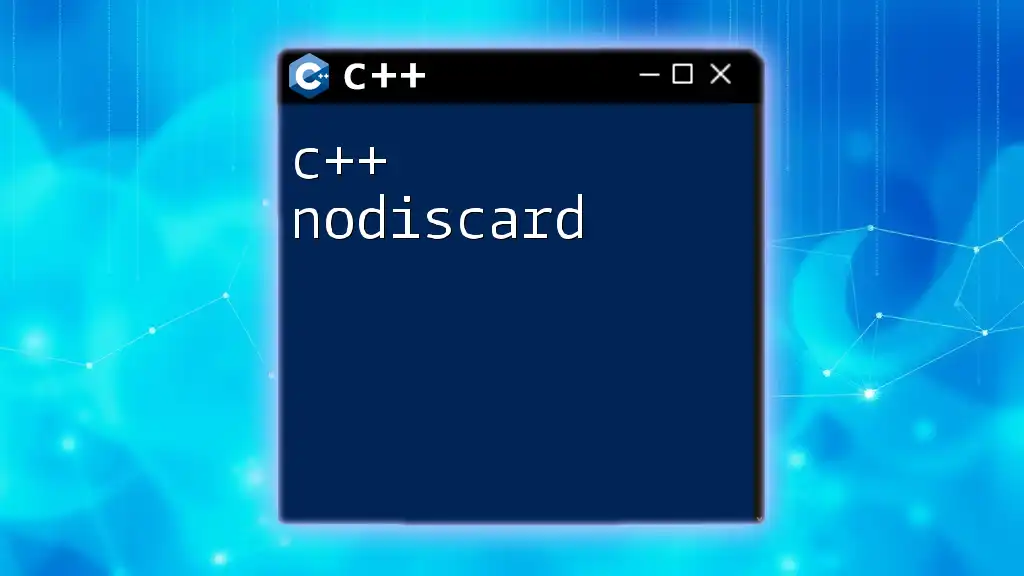
Popular C++ Obfuscators
Overview of Tools
There are numerous tools available for C++ code obfuscation, ranging from commercial products to open-source solutions. Some popular commercial tools include:
- Dotfuscator: Known for its robustness and additional features such as debugging.
- ConfuserEx: Offers various protection methods and flexibility in configuration.
On the open-source side, notable tools include:
- Obfuscator-LLVM: Integrates with the LLVM compiler framework to obfuscate C/C++ code efficiently.
- Stunnix: A versatile C/C++ obfuscator that can rearrange code structurally.
Tool Comparison and Recommendations
When choosing a C++ code obfuscator, consider the following features:
- Complexity of obfuscation techniques available
- Compatibility with various C++ standards
- Customization options for the level of obfuscation
It is advisable to read reviews and possibly experiment with a few options to find the best fit based on your specific project needs.
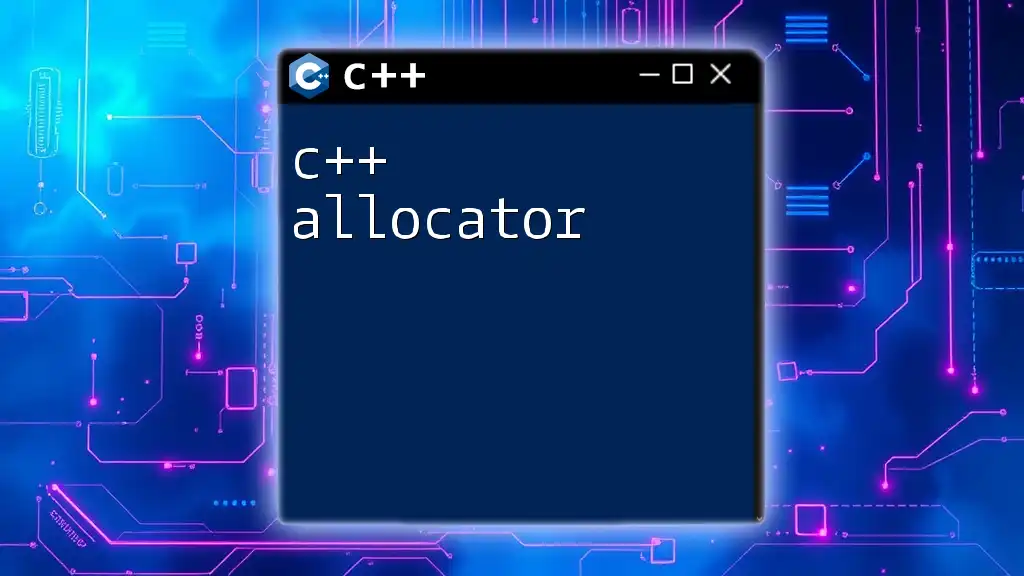
How to Use a C++ Code Obfuscator
Installation and Setup
Using a C++ code obfuscator typically begins with installation. Select a tool that aligns with your project needs and follow the guidelines provided by the tool's documentation to install it successfully.
Running the Obfuscator
Once your chosen obfuscator is set up, you can start the obfuscation process. Most tools come with command-line interfaces that allow you to execute fundamental commands. For example:
obfuscator --input file.cpp --output obfuscated_file.cpp
After running this command, review the output generated by the obfuscator to ensure that your code functions as intended while being obfuscated.
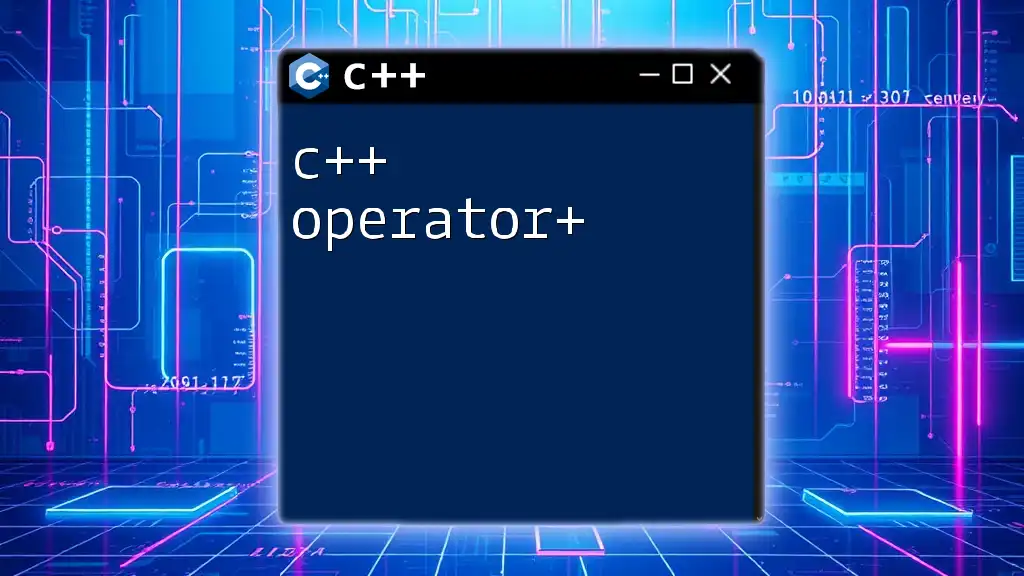
Limitations of C++ Code Obfuscation
While the benefits of a C++ code obfuscator are clear, there are limitations to consider:
Performance Concerns
Depending on the level of obfuscation, there may be performance trade-offs. More comprehensive obfuscation processes can slow down the execution speed of the software. It’s essential to strike a balance between security and performance efficiency.
Obfuscation Isn’t Foolproof
It’s critical to understand that code obfuscation does not guarantee absolute security. Determined attackers can still make their way through obfuscated code, especially with sophisticated reverse engineering techniques. Thus, treat obfuscation as one component of a broader security strategy.
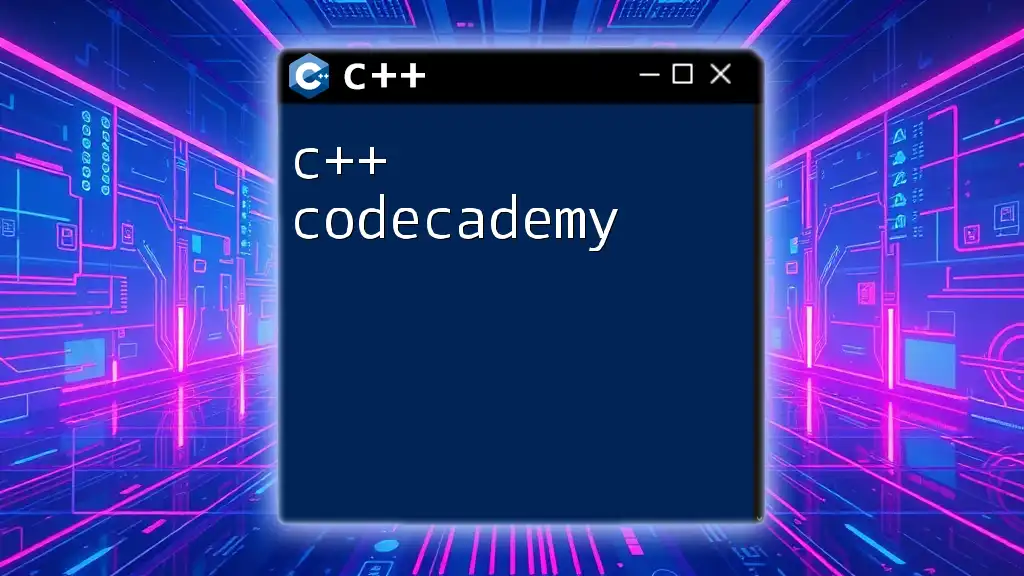
Conclusion
In summary, using a C++ code obfuscator can significantly enhance the security of software applications by protecting intellectual property, improving reliability, and making it difficult for potential adversaries to exploit sensitive algorithms. However, always remember the limitations that come with obfuscation; being aware of these can help you implement a more robust protection strategy for your software.
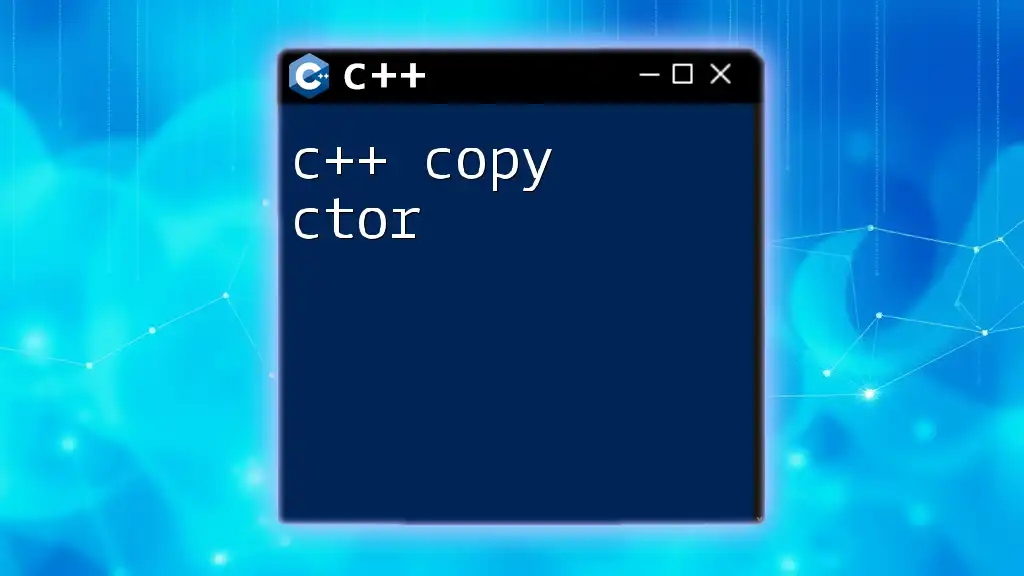
Additional Resources
For further learning about C++ code obfuscation and related best practices, consider exploring various tutorials, articles, and forums. Here, you can engage with communities and professionals who share similar interests.
Frequently Asked Questions
Addressing common questions can help clarify further intricacies regarding C++ obfuscation. Engaging with your audience through a Q&A format can foster a deeper understanding of this important topic.
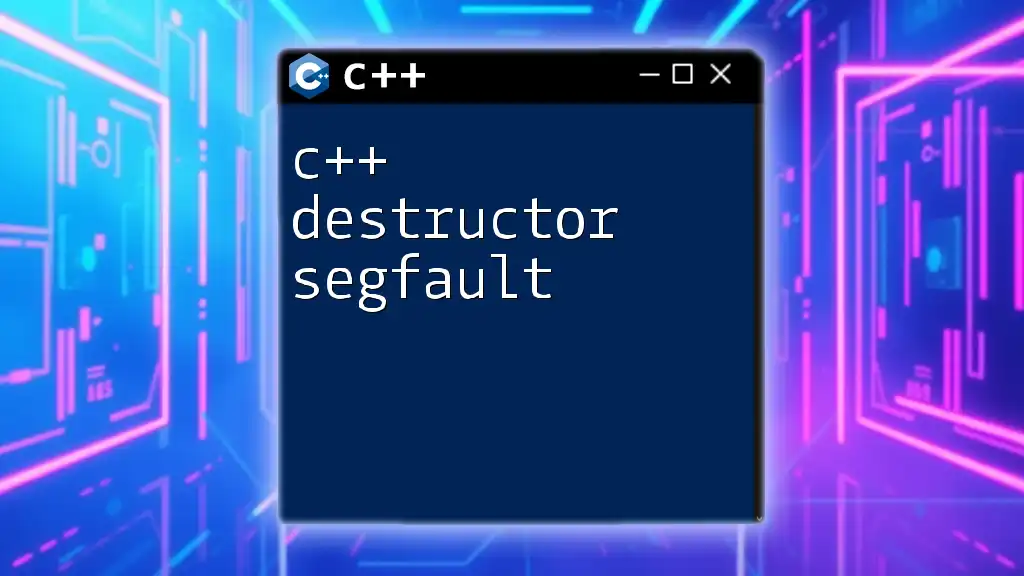
Call to Action
We invite you to share your thoughts, experiences, or any questions you have regarding C++ code obfuscation. Join our classes to learn more about C++ commands and usage, and enhance your programming skills today!